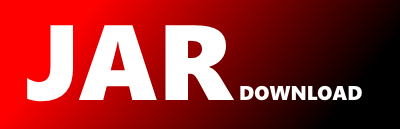
org.apache.maven.api.cli.Options Maven / Gradle / Ivy
The newest version!
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package org.apache.maven.api.cli;
import java.util.Collection;
import java.util.Map;
import java.util.Optional;
import java.util.function.Consumer;
import org.apache.maven.api.annotations.Experimental;
import org.apache.maven.api.annotations.Nonnull;
/**
* Represents the base options supported by Maven tools.
* This interface defines methods to access various configuration options
* that can be set through command-line arguments or configuration files.
*
* @since 4.0.0
*/
@Experimental
public interface Options {
/** Constant indicating that the options source is the command-line interface. */
String SOURCE_CLI = "CLI";
/**
* Returns a simple designator of the options source, such as "cli", "maven.conf", etc.
*
* @return a string representing the source of the options
*/
@Nonnull
String source();
/**
* Returns the user-defined properties for the Maven execution.
*
* @return an {@link Optional} containing the map of user properties, or empty if not set
*/
@Nonnull
Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy