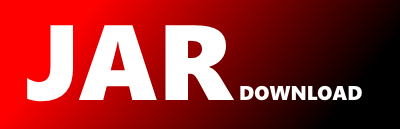
org.apache.maven.api.metadata.Metadata Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of maven-api-metadata Show documentation
Show all versions of maven-api-metadata Show documentation
Maven 4 API - Immutable Repository Metadata model.
// =================== DO NOT EDIT THIS FILE ====================
// Generated by Modello Velocity from model.vm
// template, any modifications will be overwritten.
// ==============================================================
package org.apache.maven.api.metadata;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Set;
import org.apache.maven.api.annotations.Experimental;
import org.apache.maven.api.annotations.Generated;
import org.apache.maven.api.annotations.Immutable;
import org.apache.maven.api.annotations.Nonnull;
import org.apache.maven.api.annotations.NotThreadSafe;
import org.apache.maven.api.annotations.ThreadSafe;
/**
*/
@Experimental
@Generated @ThreadSafe @Immutable
public class Metadata
implements Serializable
{
final String namespaceUri;
final String modelEncoding;
/**
* The version of the underlying metadata model.
*/
final String modelVersion;
/**
* The groupId when this directory represents "groupId/artifactId" or "groupId/artifactId/version".
*/
final String groupId;
/**
* The artifactId when this directory represents "groupId/artifactId" or "groupId/artifactId/version".
*/
final String artifactId;
/**
* Versioning information when this directory represents "groupId/artifactId" or "groupId/artifactId/version".
*/
final Versioning versioning;
/**
* The base version (i.e. ending in {@code -SNAPSHOT}) when this directory represents a "groupId/artifactId/version" for a SNAPSHOT.
*/
final String version;
/**
* The set of plugins when this directory represents a "groupId".
*/
final List plugins;
/**
* Constructor for this class, to be called from its subclasses and {@link Builder}.
* @see Builder#build()
*/
protected Metadata(Builder builder) {
this.namespaceUri = builder.namespaceUri != null ? builder.namespaceUri : (builder.base != null ? builder.base.namespaceUri : null);
this.modelEncoding = builder.modelEncoding != null ? builder.modelEncoding : (builder.base != null ? builder.base.modelEncoding : "UTF-8");
this.modelVersion = builder.modelVersion != null ? builder.modelVersion : (builder.base != null ? builder.base.modelVersion : null);
this.groupId = builder.groupId != null ? builder.groupId : (builder.base != null ? builder.base.groupId : null);
this.artifactId = builder.artifactId != null ? builder.artifactId : (builder.base != null ? builder.base.artifactId : null);
this.versioning = builder.versioning != null ? builder.versioning : (builder.base != null ? builder.base.versioning : null);
this.version = builder.version != null ? builder.version : (builder.base != null ? builder.base.version : null);
this.plugins = ImmutableCollections.copy(builder.plugins != null ? builder.plugins : (builder.base != null ? builder.base.plugins : null));
}
public String getNamespaceUri() {
return namespaceUri;
}
public String getModelEncoding() {
return modelEncoding;
}
/**
* The version of the underlying metadata model.
*
* @return a {@code String}
*/
public String getModelVersion() {
return this.modelVersion;
}
/**
* The groupId when this directory represents "groupId/artifactId" or "groupId/artifactId/version".
*
* @return a {@code String}
*/
public String getGroupId() {
return this.groupId;
}
/**
* The artifactId when this directory represents "groupId/artifactId" or "groupId/artifactId/version".
*
* @return a {@code String}
*/
public String getArtifactId() {
return this.artifactId;
}
/**
* Versioning information when this directory represents "groupId/artifactId" or "groupId/artifactId/version".
*
* @return a {@code Versioning}
*/
public Versioning getVersioning() {
return this.versioning;
}
/**
* The base version (i.e. ending in {@code -SNAPSHOT}) when this directory represents a "groupId/artifactId/version" for a SNAPSHOT.
*
* @return a {@code String}
*/
public String getVersion() {
return this.version;
}
/**
* The set of plugins when this directory represents a "groupId".
*
* @return a {@code List}
*/
@Nonnull
public List getPlugins() {
return this.plugins;
}
/**
* Creates a new builder with this object as the basis.
*
* @return a {@code Builder}
*/
@Nonnull
public Builder with() {
return newBuilder(this);
}
/**
* Creates a new {@code Metadata} instance using the specified modelVersion.
*
* @param modelVersion the new {@code String} to use
* @return a {@code Metadata} with the specified modelVersion
*/
@Nonnull
public Metadata withModelVersion(String modelVersion) {
return newBuilder(this, true).modelVersion(modelVersion).build();
}
/**
* Creates a new {@code Metadata} instance using the specified groupId.
*
* @param groupId the new {@code String} to use
* @return a {@code Metadata} with the specified groupId
*/
@Nonnull
public Metadata withGroupId(String groupId) {
return newBuilder(this, true).groupId(groupId).build();
}
/**
* Creates a new {@code Metadata} instance using the specified artifactId.
*
* @param artifactId the new {@code String} to use
* @return a {@code Metadata} with the specified artifactId
*/
@Nonnull
public Metadata withArtifactId(String artifactId) {
return newBuilder(this, true).artifactId(artifactId).build();
}
/**
* Creates a new {@code Metadata} instance using the specified versioning.
*
* @param versioning the new {@code Versioning} to use
* @return a {@code Metadata} with the specified versioning
*/
@Nonnull
public Metadata withVersioning(Versioning versioning) {
return newBuilder(this, true).versioning(versioning).build();
}
/**
* Creates a new {@code Metadata} instance using the specified version.
*
* @param version the new {@code String} to use
* @return a {@code Metadata} with the specified version
*/
@Nonnull
public Metadata withVersion(String version) {
return newBuilder(this, true).version(version).build();
}
/**
* Creates a new {@code Metadata} instance using the specified plugins.
*
* @param plugins the new {@code Collection} to use
* @return a {@code Metadata} with the specified plugins
*/
@Nonnull
public Metadata withPlugins(Collection plugins) {
return newBuilder(this, true).plugins(plugins).build();
}
/**
* Creates a new {@code Metadata} instance.
* Equivalent to {@code newInstance(true)}.
* @see #newInstance(boolean)
*
* @return a new {@code Metadata}
*/
@Nonnull
public static Metadata newInstance() {
return newInstance(true);
}
/**
* Creates a new {@code Metadata} instance using default values or not.
* Equivalent to {@code newBuilder(withDefaults).build()}.
*
* @param withDefaults the boolean indicating whether default values should be used
* @return a new {@code Metadata}
*/
@Nonnull
public static Metadata newInstance(boolean withDefaults) {
return newBuilder(withDefaults).build();
}
/**
* Creates a new {@code Metadata} builder instance.
* Equivalent to {@code newBuilder(true)}.
* @see #newBuilder(boolean)
*
* @return a new {@code Builder}
*/
@Nonnull
public static Builder newBuilder() {
return newBuilder(true);
}
/**
* Creates a new {@code Metadata} builder instance using default values or not.
*
* @param withDefaults the boolean indicating whether default values should be used
* @return a new {@code Builder}
*/
@Nonnull
public static Builder newBuilder(boolean withDefaults) {
return new Builder(withDefaults);
}
/**
* Creates a new {@code Metadata} builder instance using the specified object as a basis.
* Equivalent to {@code newBuilder(from, false)}.
*
* @param from the {@code Metadata} instance to use as a basis
* @return a new {@code Builder}
*/
@Nonnull
public static Builder newBuilder(Metadata from) {
return newBuilder(from, false);
}
/**
* Creates a new {@code Metadata} builder instance using the specified object as a basis.
*
* @param from the {@code Metadata} instance to use as a basis
* @param forceCopy the boolean indicating if a copy should be forced
* @return a new {@code Builder}
*/
@Nonnull
public static Builder newBuilder(Metadata from, boolean forceCopy) {
return new Builder(from, forceCopy);
}
/**
* Builder class used to create Metadata instances.
* @see #with()
* @see #newBuilder()
*/
@NotThreadSafe
public static class Builder
{
Metadata base;
String namespaceUri;
String modelEncoding;
String modelVersion;
String groupId;
String artifactId;
Versioning versioning;
String version;
Collection plugins;
protected Builder(boolean withDefaults) {
if (withDefaults) {
}
}
protected Builder(Metadata base, boolean forceCopy) {
this.namespaceUri = base.namespaceUri;
this.modelEncoding = base.modelEncoding;
if (forceCopy) {
this.modelVersion = base.modelVersion;
this.groupId = base.groupId;
this.artifactId = base.artifactId;
this.versioning = base.versioning;
this.version = base.version;
this.plugins = base.plugins;
} else {
this.base = base;
}
}
@Nonnull
public Builder namespaceUri(String namespaceUri) {
this.namespaceUri = namespaceUri;
return this;
}
@Nonnull
public Builder modelEncoding(String modelEncoding) {
this.modelEncoding = modelEncoding;
return this;
}
@Nonnull
public Builder modelVersion(String modelVersion) {
this.modelVersion = modelVersion;
return this;
}
@Nonnull
public Builder groupId(String groupId) {
this.groupId = groupId;
return this;
}
@Nonnull
public Builder artifactId(String artifactId) {
this.artifactId = artifactId;
return this;
}
@Nonnull
public Builder versioning(Versioning versioning) {
this.versioning = versioning;
return this;
}
@Nonnull
public Builder version(String version) {
this.version = version;
return this;
}
@Nonnull
public Builder plugins(Collection plugins) {
this.plugins = plugins;
return this;
}
@Nonnull
public Metadata build() {
// this method should not contain any logic other than creating (or reusing) an object in order to ease subclassing
if (base != null
&& (modelVersion == null || modelVersion == base.modelVersion)
&& (groupId == null || groupId == base.groupId)
&& (artifactId == null || artifactId == base.artifactId)
&& (versioning == null || versioning == base.versioning)
&& (version == null || version == base.version)
&& (plugins == null || plugins == base.plugins)
) {
return base;
}
return new Metadata(this);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy