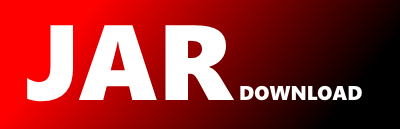
org.apache.maven.api.metadata.Plugin Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of maven-api-metadata Show documentation
Show all versions of maven-api-metadata Show documentation
Maven 4 API - Immutable Repository Metadata model.
// =================== DO NOT EDIT THIS FILE ====================
// Generated by Modello Velocity from model.vm
// template, any modifications will be overwritten.
// ==============================================================
package org.apache.maven.api.metadata;
import java.io.Serializable;
import java.util.Collections;
import java.util.HashMap;
import java.util.Map;
import java.util.Set;
import org.apache.maven.api.annotations.Experimental;
import org.apache.maven.api.annotations.Generated;
import org.apache.maven.api.annotations.Immutable;
import org.apache.maven.api.annotations.Nonnull;
import org.apache.maven.api.annotations.NotThreadSafe;
import org.apache.maven.api.annotations.ThreadSafe;
/**
* Mapping information for a single plugin within this group.
*/
@Experimental
@Generated @ThreadSafe @Immutable
public class Plugin
implements Serializable
{
/**
* Display name for the plugin.
*/
final String name;
/**
* The plugin invocation prefix (i.e. eclipse for eclipse:eclipse)
*/
final String prefix;
/**
* The plugin artifactId
*/
final String artifactId;
/**
* Constructor for this class, to be called from its subclasses and {@link Builder}.
* @see Builder#build()
*/
protected Plugin(Builder builder) {
this.name = builder.name != null ? builder.name : (builder.base != null ? builder.base.name : null);
this.prefix = builder.prefix != null ? builder.prefix : (builder.base != null ? builder.base.prefix : null);
this.artifactId = builder.artifactId != null ? builder.artifactId : (builder.base != null ? builder.base.artifactId : null);
}
/**
* Display name for the plugin.
*
* @return a {@code String}
*/
public String getName() {
return this.name;
}
/**
* The plugin invocation prefix (i.e. eclipse for eclipse:eclipse)
*
* @return a {@code String}
*/
public String getPrefix() {
return this.prefix;
}
/**
* The plugin artifactId
*
* @return a {@code String}
*/
public String getArtifactId() {
return this.artifactId;
}
/**
* Creates a new builder with this object as the basis.
*
* @return a {@code Builder}
*/
@Nonnull
public Builder with() {
return newBuilder(this);
}
/**
* Creates a new {@code Plugin} instance using the specified name.
*
* @param name the new {@code String} to use
* @return a {@code Plugin} with the specified name
*/
@Nonnull
public Plugin withName(String name) {
return newBuilder(this, true).name(name).build();
}
/**
* Creates a new {@code Plugin} instance using the specified prefix.
*
* @param prefix the new {@code String} to use
* @return a {@code Plugin} with the specified prefix
*/
@Nonnull
public Plugin withPrefix(String prefix) {
return newBuilder(this, true).prefix(prefix).build();
}
/**
* Creates a new {@code Plugin} instance using the specified artifactId.
*
* @param artifactId the new {@code String} to use
* @return a {@code Plugin} with the specified artifactId
*/
@Nonnull
public Plugin withArtifactId(String artifactId) {
return newBuilder(this, true).artifactId(artifactId).build();
}
/**
* Creates a new {@code Plugin} instance.
* Equivalent to {@code newInstance(true)}.
* @see #newInstance(boolean)
*
* @return a new {@code Plugin}
*/
@Nonnull
public static Plugin newInstance() {
return newInstance(true);
}
/**
* Creates a new {@code Plugin} instance using default values or not.
* Equivalent to {@code newBuilder(withDefaults).build()}.
*
* @param withDefaults the boolean indicating whether default values should be used
* @return a new {@code Plugin}
*/
@Nonnull
public static Plugin newInstance(boolean withDefaults) {
return newBuilder(withDefaults).build();
}
/**
* Creates a new {@code Plugin} builder instance.
* Equivalent to {@code newBuilder(true)}.
* @see #newBuilder(boolean)
*
* @return a new {@code Builder}
*/
@Nonnull
public static Builder newBuilder() {
return newBuilder(true);
}
/**
* Creates a new {@code Plugin} builder instance using default values or not.
*
* @param withDefaults the boolean indicating whether default values should be used
* @return a new {@code Builder}
*/
@Nonnull
public static Builder newBuilder(boolean withDefaults) {
return new Builder(withDefaults);
}
/**
* Creates a new {@code Plugin} builder instance using the specified object as a basis.
* Equivalent to {@code newBuilder(from, false)}.
*
* @param from the {@code Plugin} instance to use as a basis
* @return a new {@code Builder}
*/
@Nonnull
public static Builder newBuilder(Plugin from) {
return newBuilder(from, false);
}
/**
* Creates a new {@code Plugin} builder instance using the specified object as a basis.
*
* @param from the {@code Plugin} instance to use as a basis
* @param forceCopy the boolean indicating if a copy should be forced
* @return a new {@code Builder}
*/
@Nonnull
public static Builder newBuilder(Plugin from, boolean forceCopy) {
return new Builder(from, forceCopy);
}
/**
* Builder class used to create Plugin instances.
* @see #with()
* @see #newBuilder()
*/
@NotThreadSafe
public static class Builder
{
Plugin base;
String name;
String prefix;
String artifactId;
protected Builder(boolean withDefaults) {
if (withDefaults) {
}
}
protected Builder(Plugin base, boolean forceCopy) {
if (forceCopy) {
this.name = base.name;
this.prefix = base.prefix;
this.artifactId = base.artifactId;
} else {
this.base = base;
}
}
@Nonnull
public Builder name(String name) {
this.name = name;
return this;
}
@Nonnull
public Builder prefix(String prefix) {
this.prefix = prefix;
return this;
}
@Nonnull
public Builder artifactId(String artifactId) {
this.artifactId = artifactId;
return this;
}
@Nonnull
public Plugin build() {
// this method should not contain any logic other than creating (or reusing) an object in order to ease subclassing
if (base != null
&& (name == null || name == base.name)
&& (prefix == null || prefix == base.prefix)
&& (artifactId == null || artifactId == base.artifactId)
) {
return base;
}
return new Plugin(this);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy