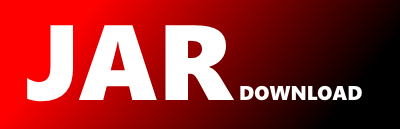
org.apache.maven.api.metadata.Snapshot Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of maven-api-metadata Show documentation
Show all versions of maven-api-metadata Show documentation
Maven 4 API - Immutable Repository Metadata model.
// =================== DO NOT EDIT THIS FILE ====================
// Generated by Modello Velocity from model.vm
// template, any modifications will be overwritten.
// ==============================================================
package org.apache.maven.api.metadata;
import java.io.Serializable;
import java.util.Collections;
import java.util.HashMap;
import java.util.Map;
import java.util.Set;
import org.apache.maven.api.annotations.Experimental;
import org.apache.maven.api.annotations.Generated;
import org.apache.maven.api.annotations.Immutable;
import org.apache.maven.api.annotations.Nonnull;
import org.apache.maven.api.annotations.NotThreadSafe;
import org.apache.maven.api.annotations.ThreadSafe;
/**
* Snapshot data for the last artifact corresponding to the SNAPSHOT base version
*/
@Experimental
@Generated @ThreadSafe @Immutable
public class Snapshot
implements Serializable
{
/**
* The timestamp when this version was deployed. The timestamp is expressed using UTC in the format yyyyMMdd.HHmmss.
*/
final String timestamp;
/**
* The incremental build number
*/
final int buildNumber;
/**
* Whether to use a local copy instead (with filename that includes the base version)
*/
final boolean localCopy;
/**
* Constructor for this class, to be called from its subclasses and {@link Builder}.
* @see Builder#build()
*/
protected Snapshot(Builder builder) {
this.timestamp = builder.timestamp != null ? builder.timestamp : (builder.base != null ? builder.base.timestamp : null);
this.buildNumber = builder.buildNumber != null ? builder.buildNumber : (builder.base != null ? builder.base.buildNumber : 0);
this.localCopy = builder.localCopy != null ? builder.localCopy : (builder.base != null ? builder.base.localCopy : false);
}
/**
* The timestamp when this version was deployed. The timestamp is expressed using UTC in the format yyyyMMdd.HHmmss.
*
* @return a {@code String}
*/
public String getTimestamp() {
return this.timestamp;
}
/**
* The incremental build number
*
* @return a {@code int}
*/
public int getBuildNumber() {
return this.buildNumber;
}
/**
* Whether to use a local copy instead (with filename that includes the base version)
*
* @return a {@code boolean}
*/
public boolean isLocalCopy() {
return this.localCopy;
}
/**
* Creates a new builder with this object as the basis.
*
* @return a {@code Builder}
*/
@Nonnull
public Builder with() {
return newBuilder(this);
}
/**
* Creates a new {@code Snapshot} instance using the specified timestamp.
*
* @param timestamp the new {@code String} to use
* @return a {@code Snapshot} with the specified timestamp
*/
@Nonnull
public Snapshot withTimestamp(String timestamp) {
return newBuilder(this, true).timestamp(timestamp).build();
}
/**
* Creates a new {@code Snapshot} instance using the specified buildNumber.
*
* @param buildNumber the new {@code int} to use
* @return a {@code Snapshot} with the specified buildNumber
*/
@Nonnull
public Snapshot withBuildNumber(int buildNumber) {
return newBuilder(this, true).buildNumber(buildNumber).build();
}
/**
* Creates a new {@code Snapshot} instance using the specified localCopy.
*
* @param localCopy the new {@code boolean} to use
* @return a {@code Snapshot} with the specified localCopy
*/
@Nonnull
public Snapshot withLocalCopy(boolean localCopy) {
return newBuilder(this, true).localCopy(localCopy).build();
}
/**
* Creates a new {@code Snapshot} instance.
* Equivalent to {@code newInstance(true)}.
* @see #newInstance(boolean)
*
* @return a new {@code Snapshot}
*/
@Nonnull
public static Snapshot newInstance() {
return newInstance(true);
}
/**
* Creates a new {@code Snapshot} instance using default values or not.
* Equivalent to {@code newBuilder(withDefaults).build()}.
*
* @param withDefaults the boolean indicating whether default values should be used
* @return a new {@code Snapshot}
*/
@Nonnull
public static Snapshot newInstance(boolean withDefaults) {
return newBuilder(withDefaults).build();
}
/**
* Creates a new {@code Snapshot} builder instance.
* Equivalent to {@code newBuilder(true)}.
* @see #newBuilder(boolean)
*
* @return a new {@code Builder}
*/
@Nonnull
public static Builder newBuilder() {
return newBuilder(true);
}
/**
* Creates a new {@code Snapshot} builder instance using default values or not.
*
* @param withDefaults the boolean indicating whether default values should be used
* @return a new {@code Builder}
*/
@Nonnull
public static Builder newBuilder(boolean withDefaults) {
return new Builder(withDefaults);
}
/**
* Creates a new {@code Snapshot} builder instance using the specified object as a basis.
* Equivalent to {@code newBuilder(from, false)}.
*
* @param from the {@code Snapshot} instance to use as a basis
* @return a new {@code Builder}
*/
@Nonnull
public static Builder newBuilder(Snapshot from) {
return newBuilder(from, false);
}
/**
* Creates a new {@code Snapshot} builder instance using the specified object as a basis.
*
* @param from the {@code Snapshot} instance to use as a basis
* @param forceCopy the boolean indicating if a copy should be forced
* @return a new {@code Builder}
*/
@Nonnull
public static Builder newBuilder(Snapshot from, boolean forceCopy) {
return new Builder(from, forceCopy);
}
/**
* Builder class used to create Snapshot instances.
* @see #with()
* @see #newBuilder()
*/
@NotThreadSafe
public static class Builder
{
Snapshot base;
String timestamp;
Integer buildNumber;
Boolean localCopy;
protected Builder(boolean withDefaults) {
if (withDefaults) {
this.buildNumber = 0;
this.localCopy = false;
}
}
protected Builder(Snapshot base, boolean forceCopy) {
if (forceCopy) {
this.timestamp = base.timestamp;
this.buildNumber = base.buildNumber;
this.localCopy = base.localCopy;
} else {
this.base = base;
}
}
@Nonnull
public Builder timestamp(String timestamp) {
this.timestamp = timestamp;
return this;
}
@Nonnull
public Builder buildNumber(int buildNumber) {
this.buildNumber = buildNumber;
return this;
}
@Nonnull
public Builder localCopy(boolean localCopy) {
this.localCopy = localCopy;
return this;
}
@Nonnull
public Snapshot build() {
// this method should not contain any logic other than creating (or reusing) an object in order to ease subclassing
if (base != null
&& (timestamp == null || timestamp == base.timestamp)
&& (buildNumber == null || buildNumber == base.buildNumber)
&& (localCopy == null || localCopy == base.localCopy)
) {
return base;
}
return new Snapshot(this);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy