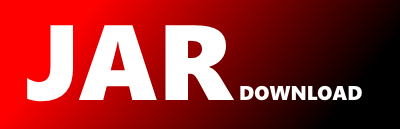
org.apache.maven.api.settings.Server Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of maven-api-settings Show documentation
Show all versions of maven-api-settings Show documentation
Maven 4 API - Immutable Settings model.
// =================== DO NOT EDIT THIS FILE ====================
// Generated by Modello Velocity from model.vm
// template, any modifications will be overwritten.
// ==============================================================
package org.apache.maven.api.settings;
import java.io.Serializable;
import java.util.Collections;
import java.util.HashMap;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.Set;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import org.apache.maven.api.annotations.Experimental;
import org.apache.maven.api.annotations.Generated;
import org.apache.maven.api.annotations.Immutable;
import org.apache.maven.api.annotations.Nonnull;
import org.apache.maven.api.annotations.NotThreadSafe;
import org.apache.maven.api.annotations.ThreadSafe;
import org.apache.maven.api.xml.XmlNode;
/**
* The {@code } element contains information required to a server settings.
*/
@Experimental
@Generated @ThreadSafe @Immutable
public class Server
extends IdentifiableBase
implements Serializable, InputLocationTracker
{
/**
* The username used to authenticate.
*/
final String username;
/**
* The password used in conjunction with the username to authenticate.
*/
final String password;
/**
* The private key location used to authenticate.
*/
final String privateKey;
/**
* The passphrase used in conjunction with the privateKey to authenticate.
*/
final String passphrase;
/**
* The permissions for files when they are created.
*/
final String filePermissions;
/**
* The permissions for directories when they are created.
*/
final String directoryPermissions;
/**
* Extra configuration for the transport layer.
*/
final XmlNode configuration;
/**
* Constructor for this class, to be called from its subclasses and {@link Builder}.
* @see Builder#build()
*/
protected Server(Builder builder) {
super(builder);
this.username = builder.username != null ? builder.username : (builder.base != null ? builder.base.username : null);
this.password = builder.password != null ? builder.password : (builder.base != null ? builder.base.password : null);
this.privateKey = builder.privateKey != null ? builder.privateKey : (builder.base != null ? builder.base.privateKey : null);
this.passphrase = builder.passphrase != null ? builder.passphrase : (builder.base != null ? builder.base.passphrase : null);
this.filePermissions = builder.filePermissions != null ? builder.filePermissions : (builder.base != null ? builder.base.filePermissions : null);
this.directoryPermissions = builder.directoryPermissions != null ? builder.directoryPermissions : (builder.base != null ? builder.base.directoryPermissions : null);
this.configuration = builder.configuration != null ? builder.configuration : (builder.base != null ? builder.base.configuration : null);
}
/**
* The username used to authenticate.
*
* @return a {@code String}
*/
public String getUsername() {
return this.username;
}
/**
* The password used in conjunction with the username to authenticate.
*
* @return a {@code String}
*/
public String getPassword() {
return this.password;
}
/**
* The private key location used to authenticate.
*
* @return a {@code String}
*/
public String getPrivateKey() {
return this.privateKey;
}
/**
* The passphrase used in conjunction with the privateKey to authenticate.
*
* @return a {@code String}
*/
public String getPassphrase() {
return this.passphrase;
}
/**
* The permissions for files when they are created.
*
* @return a {@code String}
*/
public String getFilePermissions() {
return this.filePermissions;
}
/**
* The permissions for directories when they are created.
*
* @return a {@code String}
*/
public String getDirectoryPermissions() {
return this.directoryPermissions;
}
/**
* Extra configuration for the transport layer.
*
* @return a {@code XmlNode}
*/
public XmlNode getConfiguration() {
return this.configuration;
}
/**
* Creates a new builder with this object as the basis.
*
* @return a {@code Builder}
*/
@Nonnull
public Builder with() {
return newBuilder(this);
}
/**
* Creates a new {@code Server} instance using the specified id.
*
* @param id the new {@code String} to use
* @return a {@code Server} with the specified id
*/
@Nonnull
public Server withId(String id) {
return newBuilder(this, true).id(id).build();
}
/**
* Creates a new {@code Server} instance using the specified username.
*
* @param username the new {@code String} to use
* @return a {@code Server} with the specified username
*/
@Nonnull
public Server withUsername(String username) {
return newBuilder(this, true).username(username).build();
}
/**
* Creates a new {@code Server} instance using the specified password.
*
* @param password the new {@code String} to use
* @return a {@code Server} with the specified password
*/
@Nonnull
public Server withPassword(String password) {
return newBuilder(this, true).password(password).build();
}
/**
* Creates a new {@code Server} instance using the specified privateKey.
*
* @param privateKey the new {@code String} to use
* @return a {@code Server} with the specified privateKey
*/
@Nonnull
public Server withPrivateKey(String privateKey) {
return newBuilder(this, true).privateKey(privateKey).build();
}
/**
* Creates a new {@code Server} instance using the specified passphrase.
*
* @param passphrase the new {@code String} to use
* @return a {@code Server} with the specified passphrase
*/
@Nonnull
public Server withPassphrase(String passphrase) {
return newBuilder(this, true).passphrase(passphrase).build();
}
/**
* Creates a new {@code Server} instance using the specified filePermissions.
*
* @param filePermissions the new {@code String} to use
* @return a {@code Server} with the specified filePermissions
*/
@Nonnull
public Server withFilePermissions(String filePermissions) {
return newBuilder(this, true).filePermissions(filePermissions).build();
}
/**
* Creates a new {@code Server} instance using the specified directoryPermissions.
*
* @param directoryPermissions the new {@code String} to use
* @return a {@code Server} with the specified directoryPermissions
*/
@Nonnull
public Server withDirectoryPermissions(String directoryPermissions) {
return newBuilder(this, true).directoryPermissions(directoryPermissions).build();
}
/**
* Creates a new {@code Server} instance using the specified configuration.
*
* @param configuration the new {@code XmlNode} to use
* @return a {@code Server} with the specified configuration
*/
@Nonnull
public Server withConfiguration(XmlNode configuration) {
return newBuilder(this, true).configuration(configuration).build();
}
/**
* Creates a new {@code Server} instance.
* Equivalent to {@code newInstance(true)}.
* @see #newInstance(boolean)
*
* @return a new {@code Server}
*/
@Nonnull
public static Server newInstance() {
return newInstance(true);
}
/**
* Creates a new {@code Server} instance using default values or not.
* Equivalent to {@code newBuilder(withDefaults).build()}.
*
* @param withDefaults the boolean indicating whether default values should be used
* @return a new {@code Server}
*/
@Nonnull
public static Server newInstance(boolean withDefaults) {
return newBuilder(withDefaults).build();
}
/**
* Creates a new {@code Server} builder instance.
* Equivalent to {@code newBuilder(true)}.
* @see #newBuilder(boolean)
*
* @return a new {@code Builder}
*/
@Nonnull
public static Builder newBuilder() {
return newBuilder(true);
}
/**
* Creates a new {@code Server} builder instance using default values or not.
*
* @param withDefaults the boolean indicating whether default values should be used
* @return a new {@code Builder}
*/
@Nonnull
public static Builder newBuilder(boolean withDefaults) {
return new Builder(withDefaults);
}
/**
* Creates a new {@code Server} builder instance using the specified object as a basis.
* Equivalent to {@code newBuilder(from, false)}.
*
* @param from the {@code Server} instance to use as a basis
* @return a new {@code Builder}
*/
@Nonnull
public static Builder newBuilder(Server from) {
return newBuilder(from, false);
}
/**
* Creates a new {@code Server} builder instance using the specified object as a basis.
*
* @param from the {@code Server} instance to use as a basis
* @param forceCopy the boolean indicating if a copy should be forced
* @return a new {@code Builder}
*/
@Nonnull
public static Builder newBuilder(Server from, boolean forceCopy) {
return new Builder(from, forceCopy);
}
/**
* Builder class used to create Server instances.
* @see #with()
* @see #newBuilder()
*/
@NotThreadSafe
public static class Builder
extends IdentifiableBase.Builder
{
Server base;
String username;
String password;
String privateKey;
String passphrase;
String filePermissions;
String directoryPermissions;
XmlNode configuration;
protected Builder(boolean withDefaults) {
super(withDefaults);
if (withDefaults) {
}
}
protected Builder(Server base, boolean forceCopy) {
super(base, forceCopy);
if (forceCopy) {
this.username = base.username;
this.password = base.password;
this.privateKey = base.privateKey;
this.passphrase = base.passphrase;
this.filePermissions = base.filePermissions;
this.directoryPermissions = base.directoryPermissions;
this.configuration = base.configuration;
this.locations = base.locations;
this.importedFrom = base.importedFrom;
} else {
this.base = base;
}
}
@Nonnull
public Builder id(String id) {
this.id = id;
return this;
}
@Nonnull
public Builder username(String username) {
this.username = username;
return this;
}
@Nonnull
public Builder password(String password) {
this.password = password;
return this;
}
@Nonnull
public Builder privateKey(String privateKey) {
this.privateKey = privateKey;
return this;
}
@Nonnull
public Builder passphrase(String passphrase) {
this.passphrase = passphrase;
return this;
}
@Nonnull
public Builder filePermissions(String filePermissions) {
this.filePermissions = filePermissions;
return this;
}
@Nonnull
public Builder directoryPermissions(String directoryPermissions) {
this.directoryPermissions = directoryPermissions;
return this;
}
@Nonnull
public Builder configuration(XmlNode configuration) {
this.configuration = configuration;
return this;
}
@Nonnull
public Builder location(Object key, InputLocation location) {
if (location != null) {
if (!(this.locations instanceof HashMap)) {
this.locations = this.locations != null ? new HashMap<>(this.locations) : new HashMap<>();
}
this.locations.put(key, location);
}
return this;
}
@Nonnull
public Builder importedFrom(InputLocation importedFrom) {
this.importedFrom = importedFrom;
return this;
}
@Nonnull
public Server build() {
// this method should not contain any logic other than creating (or reusing) an object in order to ease subclassing
if (base != null
&& (id == null || id == base.id)
&& (username == null || username == base.username)
&& (password == null || password == base.password)
&& (privateKey == null || privateKey == base.privateKey)
&& (passphrase == null || passphrase == base.passphrase)
&& (filePermissions == null || filePermissions == base.filePermissions)
&& (directoryPermissions == null || directoryPermissions == base.directoryPermissions)
&& (configuration == null || configuration == base.configuration)
) {
return base;
}
return new Server(this);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy