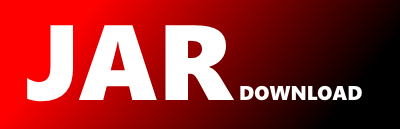
org.apache.maven.api.settings.Mirror Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of maven-api-settings Show documentation
Show all versions of maven-api-settings Show documentation
Maven 4 API - Immutable Settings model.
// =================== DO NOT EDIT THIS FILE ====================
// Generated by Modello Velocity from model.vm
// template, any modifications will be overwritten.
// ==============================================================
package org.apache.maven.api.settings;
import java.io.Serializable;
import java.util.Collections;
import java.util.HashMap;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.Set;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import org.apache.maven.api.annotations.Experimental;
import org.apache.maven.api.annotations.Generated;
import org.apache.maven.api.annotations.Immutable;
import org.apache.maven.api.annotations.Nonnull;
import org.apache.maven.api.annotations.NotThreadSafe;
import org.apache.maven.api.annotations.ThreadSafe;
/**
* A download mirror for a given repository.
*/
@Experimental
@Generated @ThreadSafe @Immutable
public class Mirror
extends IdentifiableBase
implements Serializable, InputLocationTracker
{
/**
* A repository id or (since Maven 2.0.9) an expression matching one or many repository ids to mirror, e.g.,
* central
or *,!repo1
.
* *
(since Maven 2.0.5), external:*
(since Maven 2.0.9) and external:http:*
(since Maven 3.8.0) have
* a special meaning: see Mirror Settings guide.
*/
final String mirrorOf;
/**
* The optional name that describes the mirror.
*/
final String name;
/**
* The URL of the mirror repository.
*/
final String url;
/**
* The layout of the mirror repository.
* @since Maven 3.
*/
final String layout;
/**
* The layouts of repositories being mirrored. This value can be used to restrict the usage
* of the mirror to repositories with a matching layout (apart from a matching id).
* @since Maven 3.
*/
final String mirrorOfLayouts;
/**
* Whether this mirror should be blocked from any download request but fail the download process, explaining why.
*
Default value is: false
*
Since: Maven 3.8.0
*/
final boolean blocked;
/**
* Constructor for this class, to be called from its subclasses and {@link Builder}.
* @see Builder#build()
*/
protected Mirror(Builder builder) {
super(builder);
this.mirrorOf = builder.mirrorOf != null ? builder.mirrorOf : (builder.base != null ? builder.base.mirrorOf : null);
this.name = builder.name != null ? builder.name : (builder.base != null ? builder.base.name : null);
this.url = builder.url != null ? builder.url : (builder.base != null ? builder.base.url : null);
this.layout = builder.layout != null ? builder.layout : (builder.base != null ? builder.base.layout : null);
this.mirrorOfLayouts = builder.mirrorOfLayouts != null ? builder.mirrorOfLayouts : (builder.base != null ? builder.base.mirrorOfLayouts : null);
this.blocked = builder.blocked != null ? builder.blocked : (builder.base != null ? builder.base.blocked : false);
}
/**
* A repository id or (since Maven 2.0.9) an expression matching one or many repository ids to mirror, e.g.,
* central
or *,!repo1
.
* *
(since Maven 2.0.5), external:*
(since Maven 2.0.9) and external:http:*
(since Maven 3.8.0) have
* a special meaning: see Mirror Settings guide.
*
* @return a {@code String}
*/
public String getMirrorOf() {
return this.mirrorOf;
}
/**
* The optional name that describes the mirror.
*
* @return a {@code String}
*/
public String getName() {
return this.name;
}
/**
* The URL of the mirror repository.
*
* @return a {@code String}
*/
public String getUrl() {
return this.url;
}
/**
* The layout of the mirror repository.
* @since Maven 3.
*
* @return a {@code String}
*/
public String getLayout() {
return this.layout;
}
/**
* The layouts of repositories being mirrored. This value can be used to restrict the usage
* of the mirror to repositories with a matching layout (apart from a matching id).
* @since Maven 3.
*
* @return a {@code String}
*/
public String getMirrorOfLayouts() {
return this.mirrorOfLayouts;
}
/**
* Whether this mirror should be blocked from any download request but fail the download process, explaining why.
*
Default value is: false
*
Since: Maven 3.8.0
*
* @return a {@code boolean}
*/
public boolean isBlocked() {
return this.blocked;
}
/**
* Creates a new builder with this object as the basis.
*
* @return a {@code Builder}
*/
@Nonnull
public Builder with() {
return newBuilder(this);
}
/**
* Creates a new {@code Mirror} instance using the specified id.
*
* @param id the new {@code String} to use
* @return a {@code Mirror} with the specified id
*/
@Nonnull
public Mirror withId(String id) {
return newBuilder(this, true).id(id).build();
}
/**
* Creates a new {@code Mirror} instance using the specified mirrorOf.
*
* @param mirrorOf the new {@code String} to use
* @return a {@code Mirror} with the specified mirrorOf
*/
@Nonnull
public Mirror withMirrorOf(String mirrorOf) {
return newBuilder(this, true).mirrorOf(mirrorOf).build();
}
/**
* Creates a new {@code Mirror} instance using the specified name.
*
* @param name the new {@code String} to use
* @return a {@code Mirror} with the specified name
*/
@Nonnull
public Mirror withName(String name) {
return newBuilder(this, true).name(name).build();
}
/**
* Creates a new {@code Mirror} instance using the specified url.
*
* @param url the new {@code String} to use
* @return a {@code Mirror} with the specified url
*/
@Nonnull
public Mirror withUrl(String url) {
return newBuilder(this, true).url(url).build();
}
/**
* Creates a new {@code Mirror} instance using the specified layout.
*
* @param layout the new {@code String} to use
* @return a {@code Mirror} with the specified layout
*/
@Nonnull
public Mirror withLayout(String layout) {
return newBuilder(this, true).layout(layout).build();
}
/**
* Creates a new {@code Mirror} instance using the specified mirrorOfLayouts.
*
* @param mirrorOfLayouts the new {@code String} to use
* @return a {@code Mirror} with the specified mirrorOfLayouts
*/
@Nonnull
public Mirror withMirrorOfLayouts(String mirrorOfLayouts) {
return newBuilder(this, true).mirrorOfLayouts(mirrorOfLayouts).build();
}
/**
* Creates a new {@code Mirror} instance using the specified blocked.
*
* @param blocked the new {@code boolean} to use
* @return a {@code Mirror} with the specified blocked
*/
@Nonnull
public Mirror withBlocked(boolean blocked) {
return newBuilder(this, true).blocked(blocked).build();
}
/**
* Creates a new {@code Mirror} instance.
* Equivalent to {@code newInstance(true)}.
* @see #newInstance(boolean)
*
* @return a new {@code Mirror}
*/
@Nonnull
public static Mirror newInstance() {
return newInstance(true);
}
/**
* Creates a new {@code Mirror} instance using default values or not.
* Equivalent to {@code newBuilder(withDefaults).build()}.
*
* @param withDefaults the boolean indicating whether default values should be used
* @return a new {@code Mirror}
*/
@Nonnull
public static Mirror newInstance(boolean withDefaults) {
return newBuilder(withDefaults).build();
}
/**
* Creates a new {@code Mirror} builder instance.
* Equivalent to {@code newBuilder(true)}.
* @see #newBuilder(boolean)
*
* @return a new {@code Builder}
*/
@Nonnull
public static Builder newBuilder() {
return newBuilder(true);
}
/**
* Creates a new {@code Mirror} builder instance using default values or not.
*
* @param withDefaults the boolean indicating whether default values should be used
* @return a new {@code Builder}
*/
@Nonnull
public static Builder newBuilder(boolean withDefaults) {
return new Builder(withDefaults);
}
/**
* Creates a new {@code Mirror} builder instance using the specified object as a basis.
* Equivalent to {@code newBuilder(from, false)}.
*
* @param from the {@code Mirror} instance to use as a basis
* @return a new {@code Builder}
*/
@Nonnull
public static Builder newBuilder(Mirror from) {
return newBuilder(from, false);
}
/**
* Creates a new {@code Mirror} builder instance using the specified object as a basis.
*
* @param from the {@code Mirror} instance to use as a basis
* @param forceCopy the boolean indicating if a copy should be forced
* @return a new {@code Builder}
*/
@Nonnull
public static Builder newBuilder(Mirror from, boolean forceCopy) {
return new Builder(from, forceCopy);
}
/**
* Builder class used to create Mirror instances.
* @see #with()
* @see #newBuilder()
*/
@NotThreadSafe
public static class Builder
extends IdentifiableBase.Builder
{
Mirror base;
String mirrorOf;
String name;
String url;
String layout;
String mirrorOfLayouts;
Boolean blocked;
protected Builder(boolean withDefaults) {
super(withDefaults);
if (withDefaults) {
this.layout = "default";
this.mirrorOfLayouts = "default,legacy";
this.blocked = false;
}
}
protected Builder(Mirror base, boolean forceCopy) {
super(base, forceCopy);
if (forceCopy) {
this.mirrorOf = base.mirrorOf;
this.name = base.name;
this.url = base.url;
this.layout = base.layout;
this.mirrorOfLayouts = base.mirrorOfLayouts;
this.blocked = base.blocked;
this.locations = base.locations;
this.importedFrom = base.importedFrom;
} else {
this.base = base;
}
}
@Nonnull
public Builder id(String id) {
this.id = id;
return this;
}
@Nonnull
public Builder mirrorOf(String mirrorOf) {
this.mirrorOf = mirrorOf;
return this;
}
@Nonnull
public Builder name(String name) {
this.name = name;
return this;
}
@Nonnull
public Builder url(String url) {
this.url = url;
return this;
}
@Nonnull
public Builder layout(String layout) {
this.layout = layout;
return this;
}
@Nonnull
public Builder mirrorOfLayouts(String mirrorOfLayouts) {
this.mirrorOfLayouts = mirrorOfLayouts;
return this;
}
@Nonnull
public Builder blocked(boolean blocked) {
this.blocked = blocked;
return this;
}
@Nonnull
public Builder location(Object key, InputLocation location) {
if (location != null) {
if (!(this.locations instanceof HashMap)) {
this.locations = this.locations != null ? new HashMap<>(this.locations) : new HashMap<>();
}
this.locations.put(key, location);
}
return this;
}
@Nonnull
public Builder importedFrom(InputLocation importedFrom) {
this.importedFrom = importedFrom;
return this;
}
@Nonnull
public Mirror build() {
// this method should not contain any logic other than creating (or reusing) an object in order to ease subclassing
if (base != null
&& (id == null || id == base.id)
&& (mirrorOf == null || mirrorOf == base.mirrorOf)
&& (name == null || name == base.name)
&& (url == null || url == base.url)
&& (layout == null || layout == base.layout)
&& (mirrorOfLayouts == null || mirrorOfLayouts == base.mirrorOfLayouts)
&& (blocked == null || blocked == base.blocked)
) {
return base;
}
return new Mirror(this);
}
}
public String toString() {
StringBuilder sb = new StringBuilder(128);
sb.append("Mirror[");
sb.append("id=").append(this.getId());
sb.append(",mirrorOf=").append(this.getMirrorOf());
sb.append(",url=").append(this.getUrl());
sb.append(",name=").append(this.getName());
if (isBlocked()) {
sb.append(",blocked");
}
sb.append("]");
return sb.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy