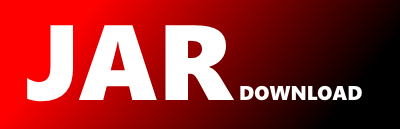
org.apache.maven.api.settings.Settings Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of maven-api-settings Show documentation
Show all versions of maven-api-settings Show documentation
Maven 4 API - Immutable Settings model.
// =================== DO NOT EDIT THIS FILE ====================
// Generated by Modello Velocity from model.vm
// template, any modifications will be overwritten.
// ==============================================================
package org.apache.maven.api.settings;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.Set;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import org.apache.maven.api.annotations.Experimental;
import org.apache.maven.api.annotations.Generated;
import org.apache.maven.api.annotations.Immutable;
import org.apache.maven.api.annotations.Nonnull;
import org.apache.maven.api.annotations.NotThreadSafe;
import org.apache.maven.api.annotations.ThreadSafe;
/**
* Root element of the user configuration file.
*/
@Experimental
@Generated @ThreadSafe @Immutable
public class Settings
extends TrackableBase
implements Serializable, InputLocationTracker
{
final String namespaceUri;
final String modelEncoding;
/**
* The local repository.
Default value is: {@code ${user.home}/.m2/repository}
*/
final String localRepository;
/**
* Whether Maven should attempt to interact with the user for input.
*/
final boolean interactiveMode;
/**
* Whether Maven should use the {@code plugin-registry.xml} file to manage plugin versions.
*/
final boolean usePluginRegistry;
/**
* Indicate whether maven should operate in offline mode full-time.
*/
final boolean offline;
/**
* Configuration for different proxy profiles. Multiple proxy profiles
* might come in handy for anyone working from a notebook or other
* mobile platform, to enable easy switching of entire proxy
* configurations by simply specifying the profile id, again either from
* the command line or from the defaults section below.
*/
final List proxies;
/**
* Configuration of server-specific settings, mainly authentication
* method. This allows configuration of authentication on a per-server
* basis.
*/
final List servers;
/**
* Configuration of download mirrors for repositories.
*/
final List mirrors;
/**
* The lists of the remote repositories.
*/
final List repositories;
/**
* The lists of the remote repositories for discovering plugins.
*/
final List pluginRepositories;
/**
* Configuration of build profiles for adjusting the build
* according to environmental parameters.
*/
final List profiles;
/**
* List of manually-activated build profiles, specified in the order in which
* they should be applied.
*/
final List activeProfiles;
/**
* List of groupIds to search for a plugin when that plugin
* groupId is not explicitly provided.
*/
final List pluginGroups;
/**
* Constructor for this class, to be called from its subclasses and {@link Builder}.
* @see Builder#build()
*/
protected Settings(Builder builder) {
super(builder);
this.namespaceUri = builder.namespaceUri != null ? builder.namespaceUri : (builder.base != null ? builder.base.namespaceUri : null);
this.modelEncoding = builder.modelEncoding != null ? builder.modelEncoding : (builder.base != null ? builder.base.modelEncoding : "UTF-8");
this.localRepository = builder.localRepository != null ? builder.localRepository : (builder.base != null ? builder.base.localRepository : null);
this.interactiveMode = builder.interactiveMode != null ? builder.interactiveMode : (builder.base != null ? builder.base.interactiveMode : true);
this.usePluginRegistry = builder.usePluginRegistry != null ? builder.usePluginRegistry : (builder.base != null ? builder.base.usePluginRegistry : false);
this.offline = builder.offline != null ? builder.offline : (builder.base != null ? builder.base.offline : false);
this.proxies = ImmutableCollections.copy(builder.proxies != null ? builder.proxies : (builder.base != null ? builder.base.proxies : null));
this.servers = ImmutableCollections.copy(builder.servers != null ? builder.servers : (builder.base != null ? builder.base.servers : null));
this.mirrors = ImmutableCollections.copy(builder.mirrors != null ? builder.mirrors : (builder.base != null ? builder.base.mirrors : null));
this.repositories = ImmutableCollections.copy(builder.repositories != null ? builder.repositories : (builder.base != null ? builder.base.repositories : null));
this.pluginRepositories = ImmutableCollections.copy(builder.pluginRepositories != null ? builder.pluginRepositories : (builder.base != null ? builder.base.pluginRepositories : null));
this.profiles = ImmutableCollections.copy(builder.profiles != null ? builder.profiles : (builder.base != null ? builder.base.profiles : null));
this.activeProfiles = ImmutableCollections.copy(builder.activeProfiles != null ? builder.activeProfiles : (builder.base != null ? builder.base.activeProfiles : null));
this.pluginGroups = ImmutableCollections.copy(builder.pluginGroups != null ? builder.pluginGroups : (builder.base != null ? builder.base.pluginGroups : null));
}
public String getNamespaceUri() {
return namespaceUri;
}
public String getModelEncoding() {
return modelEncoding;
}
/**
* The local repository.
Default value is: {@code ${user.home}/.m2/repository}
*
* @return a {@code String}
*/
public String getLocalRepository() {
return this.localRepository;
}
/**
* Whether Maven should attempt to interact with the user for input.
*
* @return a {@code boolean}
*/
public boolean isInteractiveMode() {
return this.interactiveMode;
}
/**
* Whether Maven should use the {@code plugin-registry.xml} file to manage plugin versions.
*
* @return a {@code boolean}
*/
public boolean isUsePluginRegistry() {
return this.usePluginRegistry;
}
/**
* Indicate whether maven should operate in offline mode full-time.
*
* @return a {@code boolean}
*/
public boolean isOffline() {
return this.offline;
}
/**
* Configuration for different proxy profiles. Multiple proxy profiles
* might come in handy for anyone working from a notebook or other
* mobile platform, to enable easy switching of entire proxy
* configurations by simply specifying the profile id, again either from
* the command line or from the defaults section below.
*
* @return a {@code List}
*/
@Nonnull
public List getProxies() {
return this.proxies;
}
/**
* Configuration of server-specific settings, mainly authentication
* method. This allows configuration of authentication on a per-server
* basis.
*
* @return a {@code List}
*/
@Nonnull
public List getServers() {
return this.servers;
}
/**
* Configuration of download mirrors for repositories.
*
* @return a {@code List}
*/
@Nonnull
public List getMirrors() {
return this.mirrors;
}
/**
* The lists of the remote repositories.
*
* @return a {@code List}
*/
@Nonnull
public List getRepositories() {
return this.repositories;
}
/**
* The lists of the remote repositories for discovering plugins.
*
* @return a {@code List}
*/
@Nonnull
public List getPluginRepositories() {
return this.pluginRepositories;
}
/**
* Configuration of build profiles for adjusting the build
* according to environmental parameters.
*
* @return a {@code List}
*/
@Nonnull
public List getProfiles() {
return this.profiles;
}
/**
* List of manually-activated build profiles, specified in the order in which
* they should be applied.
*
* @return a {@code List}
*/
@Nonnull
public List getActiveProfiles() {
return this.activeProfiles;
}
/**
* List of groupIds to search for a plugin when that plugin
* groupId is not explicitly provided.
*
* @return a {@code List}
*/
@Nonnull
public List getPluginGroups() {
return this.pluginGroups;
}
/**
* Creates a new builder with this object as the basis.
*
* @return a {@code Builder}
*/
@Nonnull
public Builder with() {
return newBuilder(this);
}
/**
* Creates a new {@code Settings} instance using the specified localRepository.
*
* @param localRepository the new {@code String} to use
* @return a {@code Settings} with the specified localRepository
*/
@Nonnull
public Settings withLocalRepository(String localRepository) {
return newBuilder(this, true).localRepository(localRepository).build();
}
/**
* Creates a new {@code Settings} instance using the specified interactiveMode.
*
* @param interactiveMode the new {@code boolean} to use
* @return a {@code Settings} with the specified interactiveMode
*/
@Nonnull
public Settings withInteractiveMode(boolean interactiveMode) {
return newBuilder(this, true).interactiveMode(interactiveMode).build();
}
/**
* Creates a new {@code Settings} instance using the specified usePluginRegistry.
*
* @param usePluginRegistry the new {@code boolean} to use
* @return a {@code Settings} with the specified usePluginRegistry
*/
@Nonnull
public Settings withUsePluginRegistry(boolean usePluginRegistry) {
return newBuilder(this, true).usePluginRegistry(usePluginRegistry).build();
}
/**
* Creates a new {@code Settings} instance using the specified offline.
*
* @param offline the new {@code boolean} to use
* @return a {@code Settings} with the specified offline
*/
@Nonnull
public Settings withOffline(boolean offline) {
return newBuilder(this, true).offline(offline).build();
}
/**
* Creates a new {@code Settings} instance using the specified proxies.
*
* @param proxies the new {@code Collection} to use
* @return a {@code Settings} with the specified proxies
*/
@Nonnull
public Settings withProxies(Collection proxies) {
return newBuilder(this, true).proxies(proxies).build();
}
/**
* Creates a new {@code Settings} instance using the specified servers.
*
* @param servers the new {@code Collection} to use
* @return a {@code Settings} with the specified servers
*/
@Nonnull
public Settings withServers(Collection servers) {
return newBuilder(this, true).servers(servers).build();
}
/**
* Creates a new {@code Settings} instance using the specified mirrors.
*
* @param mirrors the new {@code Collection} to use
* @return a {@code Settings} with the specified mirrors
*/
@Nonnull
public Settings withMirrors(Collection mirrors) {
return newBuilder(this, true).mirrors(mirrors).build();
}
/**
* Creates a new {@code Settings} instance using the specified repositories.
*
* @param repositories the new {@code Collection} to use
* @return a {@code Settings} with the specified repositories
*/
@Nonnull
public Settings withRepositories(Collection repositories) {
return newBuilder(this, true).repositories(repositories).build();
}
/**
* Creates a new {@code Settings} instance using the specified pluginRepositories.
*
* @param pluginRepositories the new {@code Collection} to use
* @return a {@code Settings} with the specified pluginRepositories
*/
@Nonnull
public Settings withPluginRepositories(Collection pluginRepositories) {
return newBuilder(this, true).pluginRepositories(pluginRepositories).build();
}
/**
* Creates a new {@code Settings} instance using the specified profiles.
*
* @param profiles the new {@code Collection} to use
* @return a {@code Settings} with the specified profiles
*/
@Nonnull
public Settings withProfiles(Collection profiles) {
return newBuilder(this, true).profiles(profiles).build();
}
/**
* Creates a new {@code Settings} instance using the specified activeProfiles.
*
* @param activeProfiles the new {@code Collection} to use
* @return a {@code Settings} with the specified activeProfiles
*/
@Nonnull
public Settings withActiveProfiles(Collection activeProfiles) {
return newBuilder(this, true).activeProfiles(activeProfiles).build();
}
/**
* Creates a new {@code Settings} instance using the specified pluginGroups.
*
* @param pluginGroups the new {@code Collection} to use
* @return a {@code Settings} with the specified pluginGroups
*/
@Nonnull
public Settings withPluginGroups(Collection pluginGroups) {
return newBuilder(this, true).pluginGroups(pluginGroups).build();
}
/**
* Creates a new {@code Settings} instance.
* Equivalent to {@code newInstance(true)}.
* @see #newInstance(boolean)
*
* @return a new {@code Settings}
*/
@Nonnull
public static Settings newInstance() {
return newInstance(true);
}
/**
* Creates a new {@code Settings} instance using default values or not.
* Equivalent to {@code newBuilder(withDefaults).build()}.
*
* @param withDefaults the boolean indicating whether default values should be used
* @return a new {@code Settings}
*/
@Nonnull
public static Settings newInstance(boolean withDefaults) {
return newBuilder(withDefaults).build();
}
/**
* Creates a new {@code Settings} builder instance.
* Equivalent to {@code newBuilder(true)}.
* @see #newBuilder(boolean)
*
* @return a new {@code Builder}
*/
@Nonnull
public static Builder newBuilder() {
return newBuilder(true);
}
/**
* Creates a new {@code Settings} builder instance using default values or not.
*
* @param withDefaults the boolean indicating whether default values should be used
* @return a new {@code Builder}
*/
@Nonnull
public static Builder newBuilder(boolean withDefaults) {
return new Builder(withDefaults);
}
/**
* Creates a new {@code Settings} builder instance using the specified object as a basis.
* Equivalent to {@code newBuilder(from, false)}.
*
* @param from the {@code Settings} instance to use as a basis
* @return a new {@code Builder}
*/
@Nonnull
public static Builder newBuilder(Settings from) {
return newBuilder(from, false);
}
/**
* Creates a new {@code Settings} builder instance using the specified object as a basis.
*
* @param from the {@code Settings} instance to use as a basis
* @param forceCopy the boolean indicating if a copy should be forced
* @return a new {@code Builder}
*/
@Nonnull
public static Builder newBuilder(Settings from, boolean forceCopy) {
return new Builder(from, forceCopy);
}
/**
* Builder class used to create Settings instances.
* @see #with()
* @see #newBuilder()
*/
@NotThreadSafe
public static class Builder
extends TrackableBase.Builder
{
Settings base;
String namespaceUri;
String modelEncoding;
String localRepository;
Boolean interactiveMode;
Boolean usePluginRegistry;
Boolean offline;
Collection proxies;
Collection servers;
Collection mirrors;
Collection repositories;
Collection pluginRepositories;
Collection profiles;
Collection activeProfiles;
Collection pluginGroups;
protected Builder(boolean withDefaults) {
super(withDefaults);
if (withDefaults) {
this.interactiveMode = true;
this.usePluginRegistry = false;
this.offline = false;
}
}
protected Builder(Settings base, boolean forceCopy) {
super(base, forceCopy);
this.namespaceUri = base.namespaceUri;
this.modelEncoding = base.modelEncoding;
if (forceCopy) {
this.localRepository = base.localRepository;
this.interactiveMode = base.interactiveMode;
this.usePluginRegistry = base.usePluginRegistry;
this.offline = base.offline;
this.proxies = base.proxies;
this.servers = base.servers;
this.mirrors = base.mirrors;
this.repositories = base.repositories;
this.pluginRepositories = base.pluginRepositories;
this.profiles = base.profiles;
this.activeProfiles = base.activeProfiles;
this.pluginGroups = base.pluginGroups;
this.locations = base.locations;
this.importedFrom = base.importedFrom;
} else {
this.base = base;
}
}
@Nonnull
public Builder namespaceUri(String namespaceUri) {
this.namespaceUri = namespaceUri;
return this;
}
@Nonnull
public Builder modelEncoding(String modelEncoding) {
this.modelEncoding = modelEncoding;
return this;
}
@Nonnull
public Builder localRepository(String localRepository) {
this.localRepository = localRepository;
return this;
}
@Nonnull
public Builder interactiveMode(boolean interactiveMode) {
this.interactiveMode = interactiveMode;
return this;
}
@Nonnull
public Builder usePluginRegistry(boolean usePluginRegistry) {
this.usePluginRegistry = usePluginRegistry;
return this;
}
@Nonnull
public Builder offline(boolean offline) {
this.offline = offline;
return this;
}
@Nonnull
public Builder proxies(Collection proxies) {
this.proxies = proxies;
return this;
}
@Nonnull
public Builder servers(Collection servers) {
this.servers = servers;
return this;
}
@Nonnull
public Builder mirrors(Collection mirrors) {
this.mirrors = mirrors;
return this;
}
@Nonnull
public Builder repositories(Collection repositories) {
this.repositories = repositories;
return this;
}
@Nonnull
public Builder pluginRepositories(Collection pluginRepositories) {
this.pluginRepositories = pluginRepositories;
return this;
}
@Nonnull
public Builder profiles(Collection profiles) {
this.profiles = profiles;
return this;
}
@Nonnull
public Builder activeProfiles(Collection activeProfiles) {
this.activeProfiles = activeProfiles;
return this;
}
@Nonnull
public Builder pluginGroups(Collection pluginGroups) {
this.pluginGroups = pluginGroups;
return this;
}
@Nonnull
public Builder location(Object key, InputLocation location) {
if (location != null) {
if (!(this.locations instanceof HashMap)) {
this.locations = this.locations != null ? new HashMap<>(this.locations) : new HashMap<>();
}
this.locations.put(key, location);
}
return this;
}
@Nonnull
public Builder importedFrom(InputLocation importedFrom) {
this.importedFrom = importedFrom;
return this;
}
@Nonnull
public Settings build() {
// this method should not contain any logic other than creating (or reusing) an object in order to ease subclassing
if (base != null
&& (localRepository == null || localRepository == base.localRepository)
&& (interactiveMode == null || interactiveMode == base.interactiveMode)
&& (usePluginRegistry == null || usePluginRegistry == base.usePluginRegistry)
&& (offline == null || offline == base.offline)
&& (proxies == null || proxies == base.proxies)
&& (servers == null || servers == base.servers)
&& (mirrors == null || mirrors == base.mirrors)
&& (repositories == null || repositories == base.repositories)
&& (pluginRepositories == null || pluginRepositories == base.pluginRepositories)
&& (profiles == null || profiles == base.profiles)
&& (activeProfiles == null || activeProfiles == base.activeProfiles)
&& (pluginGroups == null || pluginGroups == base.pluginGroups)
) {
return base;
}
return new Settings(this);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy