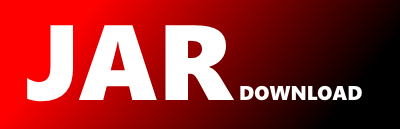
org.apache.maven.api.toolchain.PersistedToolchains Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of maven-api-toolchain Show documentation
Show all versions of maven-api-toolchain Show documentation
Maven 4 API - Immutable Toolchain model.
The newest version!
// =================== DO NOT EDIT THIS FILE ====================
// Generated by Modello Velocity from model.vm
// template, any modifications will be overwritten.
// ==============================================================
package org.apache.maven.api.toolchain;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.Set;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import org.apache.maven.api.annotations.Experimental;
import org.apache.maven.api.annotations.Generated;
import org.apache.maven.api.annotations.Immutable;
import org.apache.maven.api.annotations.Nonnull;
import org.apache.maven.api.annotations.NotThreadSafe;
import org.apache.maven.api.annotations.ThreadSafe;
/**
* The {@code } element is the root of the descriptor.
* The following table lists all the possible child elements.
*/
@Experimental
@Generated @ThreadSafe @Immutable
public class PersistedToolchains
extends TrackableBase
implements Serializable, InputLocationTracker
{
final String namespaceUri;
final String modelEncoding;
/**
* The toolchain instance definition.
*/
final List toolchains;
/**
* Constructor for this class, to be called from its subclasses and {@link Builder}.
* @see Builder#build()
*/
protected PersistedToolchains(Builder builder) {
super(builder);
this.namespaceUri = builder.namespaceUri != null ? builder.namespaceUri : (builder.base != null ? builder.base.namespaceUri : null);
this.modelEncoding = builder.modelEncoding != null ? builder.modelEncoding : (builder.base != null ? builder.base.modelEncoding : "UTF-8");
this.toolchains = ImmutableCollections.copy(builder.toolchains != null ? builder.toolchains : (builder.base != null ? builder.base.toolchains : null));
}
public String getNamespaceUri() {
return namespaceUri;
}
public String getModelEncoding() {
return modelEncoding;
}
/**
* The toolchain instance definition.
*
* @return a {@code List}
*/
@Nonnull
public List getToolchains() {
return this.toolchains;
}
/**
* Creates a new builder with this object as the basis.
*
* @return a {@code Builder}
*/
@Nonnull
public Builder with() {
return newBuilder(this);
}
/**
* Creates a new {@code PersistedToolchains} instance using the specified toolchains.
*
* @param toolchains the new {@code Collection} to use
* @return a {@code PersistedToolchains} with the specified toolchains
*/
@Nonnull
public PersistedToolchains withToolchains(Collection toolchains) {
return newBuilder(this, true).toolchains(toolchains).build();
}
/**
* Creates a new {@code PersistedToolchains} instance.
* Equivalent to {@code newInstance(true)}.
* @see #newInstance(boolean)
*
* @return a new {@code PersistedToolchains}
*/
@Nonnull
public static PersistedToolchains newInstance() {
return newInstance(true);
}
/**
* Creates a new {@code PersistedToolchains} instance using default values or not.
* Equivalent to {@code newBuilder(withDefaults).build()}.
*
* @param withDefaults the boolean indicating whether default values should be used
* @return a new {@code PersistedToolchains}
*/
@Nonnull
public static PersistedToolchains newInstance(boolean withDefaults) {
return newBuilder(withDefaults).build();
}
/**
* Creates a new {@code PersistedToolchains} builder instance.
* Equivalent to {@code newBuilder(true)}.
* @see #newBuilder(boolean)
*
* @return a new {@code Builder}
*/
@Nonnull
public static Builder newBuilder() {
return newBuilder(true);
}
/**
* Creates a new {@code PersistedToolchains} builder instance using default values or not.
*
* @param withDefaults the boolean indicating whether default values should be used
* @return a new {@code Builder}
*/
@Nonnull
public static Builder newBuilder(boolean withDefaults) {
return new Builder(withDefaults);
}
/**
* Creates a new {@code PersistedToolchains} builder instance using the specified object as a basis.
* Equivalent to {@code newBuilder(from, false)}.
*
* @param from the {@code PersistedToolchains} instance to use as a basis
* @return a new {@code Builder}
*/
@Nonnull
public static Builder newBuilder(PersistedToolchains from) {
return newBuilder(from, false);
}
/**
* Creates a new {@code PersistedToolchains} builder instance using the specified object as a basis.
*
* @param from the {@code PersistedToolchains} instance to use as a basis
* @param forceCopy the boolean indicating if a copy should be forced
* @return a new {@code Builder}
*/
@Nonnull
public static Builder newBuilder(PersistedToolchains from, boolean forceCopy) {
return new Builder(from, forceCopy);
}
/**
* Builder class used to create PersistedToolchains instances.
* @see #with()
* @see #newBuilder()
*/
@NotThreadSafe
public static class Builder
extends TrackableBase.Builder
{
PersistedToolchains base;
String namespaceUri;
String modelEncoding;
Collection toolchains;
protected Builder(boolean withDefaults) {
super(withDefaults);
if (withDefaults) {
}
}
protected Builder(PersistedToolchains base, boolean forceCopy) {
super(base, forceCopy);
this.namespaceUri = base.namespaceUri;
this.modelEncoding = base.modelEncoding;
if (forceCopy) {
this.toolchains = base.toolchains;
this.locations = base.locations;
this.importedFrom = base.importedFrom;
} else {
this.base = base;
}
}
@Nonnull
public Builder namespaceUri(String namespaceUri) {
this.namespaceUri = namespaceUri;
return this;
}
@Nonnull
public Builder modelEncoding(String modelEncoding) {
this.modelEncoding = modelEncoding;
return this;
}
@Nonnull
public Builder toolchains(Collection toolchains) {
this.toolchains = toolchains;
return this;
}
@Nonnull
public Builder location(Object key, InputLocation location) {
if (location != null) {
if (!(this.locations instanceof HashMap)) {
this.locations = this.locations != null ? new HashMap<>(this.locations) : new HashMap<>();
}
this.locations.put(key, location);
}
return this;
}
@Nonnull
public Builder importedFrom(InputLocation importedFrom) {
this.importedFrom = importedFrom;
return this;
}
@Nonnull
public PersistedToolchains build() {
// this method should not contain any logic other than creating (or reusing) an object in order to ease subclassing
if (base != null
&& (toolchains == null || toolchains == base.toolchains)
) {
return base;
}
return new PersistedToolchains(this);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy