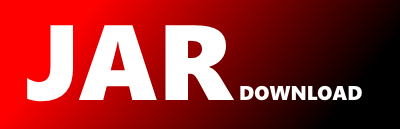
org.apache.maven.api.toolchain.ToolchainModel Maven / Gradle / Ivy
Show all versions of maven-api-toolchain Show documentation
// =================== DO NOT EDIT THIS FILE ====================
// Generated by Modello Velocity from model.vm
// template, any modifications will be overwritten.
// ==============================================================
package org.apache.maven.api.toolchain;
import java.io.Serializable;
import java.util.Collections;
import java.util.HashMap;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.Set;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import org.apache.maven.api.annotations.Experimental;
import org.apache.maven.api.annotations.Generated;
import org.apache.maven.api.annotations.Immutable;
import org.apache.maven.api.annotations.Nonnull;
import org.apache.maven.api.annotations.NotThreadSafe;
import org.apache.maven.api.annotations.ThreadSafe;
import org.apache.maven.api.xml.XmlNode;
/**
* Definition of a toolchain instance.
*/
@Experimental
@Generated @ThreadSafe @Immutable
public class ToolchainModel
extends TrackableBase
implements Serializable, InputLocationTracker
{
/**
* Type of toolchain:
* - {@code jdk} for JDK Standard Toolchain,
* - other value for Custom Toolchain
*
*/
final String type;
/**
* Toolchain identification information, which will be matched against project requirements.
* For Maven 2.0.9 to 3.2.3, the actual content structure was completely open: each toolchain type would
* define its own format and semantics. This was generally a properties format.
*
Since Maven 3.2.4, the type for this field has been changed to Properties to match the de-facto
* format.
*
Each toolchain defines its own properties names and semantics.
*/
final Map provides;
/**
* Toolchain configuration information, like location or any information that is to be retrieved.
* Actual content structure is completely open: each toolchain type will define its own format and
* semantics.
*
In general, this is a properties format: {@code value } with per-toolchain
* defined properties names.
*/
final XmlNode configuration;
/**
* Constructor for this class, to be called from its subclasses and {@link Builder}.
* @see Builder#build()
*/
protected ToolchainModel(Builder builder) {
super(builder);
this.type = builder.type != null ? builder.type : (builder.base != null ? builder.base.type : null);
this.provides = ImmutableCollections.copy(builder.provides != null ? builder.provides : (builder.base != null ? builder.base.provides : null));
this.configuration = builder.configuration != null ? builder.configuration : (builder.base != null ? builder.base.configuration : null);
}
/**
* Type of toolchain:
* - {@code jdk} for JDK Standard Toolchain,
* - other value for Custom Toolchain
*
*
* @return a {@code String}
*/
public String getType() {
return this.type;
}
/**
* Toolchain identification information, which will be matched against project requirements.
* For Maven 2.0.9 to 3.2.3, the actual content structure was completely open: each toolchain type would
* define its own format and semantics. This was generally a properties format.
*
Since Maven 3.2.4, the type for this field has been changed to Properties to match the de-facto
* format.
*
Each toolchain defines its own properties names and semantics.
*
* @return a {@code Map}
*/
@Nonnull
public Map getProvides() {
return this.provides;
}
/**
* Toolchain configuration information, like location or any information that is to be retrieved.
* Actual content structure is completely open: each toolchain type will define its own format and
* semantics.
*
In general, this is a properties format: {@code value } with per-toolchain
* defined properties names.
*
* @return a {@code XmlNode}
*/
public XmlNode getConfiguration() {
return this.configuration;
}
/**
* Creates a new builder with this object as the basis.
*
* @return a {@code Builder}
*/
@Nonnull
public Builder with() {
return newBuilder(this);
}
/**
* Creates a new {@code ToolchainModel} instance using the specified type.
*
* @param type the new {@code String} to use
* @return a {@code ToolchainModel} with the specified type
*/
@Nonnull
public ToolchainModel withType(String type) {
return newBuilder(this, true).type(type).build();
}
/**
* Creates a new {@code ToolchainModel} instance using the specified provides.
*
* @param provides the new {@code Map} to use
* @return a {@code ToolchainModel} with the specified provides
*/
@Nonnull
public ToolchainModel withProvides(Map provides) {
return newBuilder(this, true).provides(provides).build();
}
/**
* Creates a new {@code ToolchainModel} instance using the specified configuration.
*
* @param configuration the new {@code XmlNode} to use
* @return a {@code ToolchainModel} with the specified configuration
*/
@Nonnull
public ToolchainModel withConfiguration(XmlNode configuration) {
return newBuilder(this, true).configuration(configuration).build();
}
/**
* Creates a new {@code ToolchainModel} instance.
* Equivalent to {@code newInstance(true)}.
* @see #newInstance(boolean)
*
* @return a new {@code ToolchainModel}
*/
@Nonnull
public static ToolchainModel newInstance() {
return newInstance(true);
}
/**
* Creates a new {@code ToolchainModel} instance using default values or not.
* Equivalent to {@code newBuilder(withDefaults).build()}.
*
* @param withDefaults the boolean indicating whether default values should be used
* @return a new {@code ToolchainModel}
*/
@Nonnull
public static ToolchainModel newInstance(boolean withDefaults) {
return newBuilder(withDefaults).build();
}
/**
* Creates a new {@code ToolchainModel} builder instance.
* Equivalent to {@code newBuilder(true)}.
* @see #newBuilder(boolean)
*
* @return a new {@code Builder}
*/
@Nonnull
public static Builder newBuilder() {
return newBuilder(true);
}
/**
* Creates a new {@code ToolchainModel} builder instance using default values or not.
*
* @param withDefaults the boolean indicating whether default values should be used
* @return a new {@code Builder}
*/
@Nonnull
public static Builder newBuilder(boolean withDefaults) {
return new Builder(withDefaults);
}
/**
* Creates a new {@code ToolchainModel} builder instance using the specified object as a basis.
* Equivalent to {@code newBuilder(from, false)}.
*
* @param from the {@code ToolchainModel} instance to use as a basis
* @return a new {@code Builder}
*/
@Nonnull
public static Builder newBuilder(ToolchainModel from) {
return newBuilder(from, false);
}
/**
* Creates a new {@code ToolchainModel} builder instance using the specified object as a basis.
*
* @param from the {@code ToolchainModel} instance to use as a basis
* @param forceCopy the boolean indicating if a copy should be forced
* @return a new {@code Builder}
*/
@Nonnull
public static Builder newBuilder(ToolchainModel from, boolean forceCopy) {
return new Builder(from, forceCopy);
}
/**
* Builder class used to create ToolchainModel instances.
* @see #with()
* @see #newBuilder()
*/
@NotThreadSafe
public static class Builder
extends TrackableBase.Builder
{
ToolchainModel base;
String type;
Map provides;
XmlNode configuration;
protected Builder(boolean withDefaults) {
super(withDefaults);
if (withDefaults) {
}
}
protected Builder(ToolchainModel base, boolean forceCopy) {
super(base, forceCopy);
if (forceCopy) {
this.type = base.type;
this.provides = base.provides;
this.configuration = base.configuration;
this.locations = base.locations;
this.importedFrom = base.importedFrom;
} else {
this.base = base;
}
}
@Nonnull
public Builder type(String type) {
this.type = type;
return this;
}
@Nonnull
public Builder provides(Map provides) {
this.provides = provides;
return this;
}
@Nonnull
public Builder configuration(XmlNode configuration) {
this.configuration = configuration;
return this;
}
@Nonnull
public Builder location(Object key, InputLocation location) {
if (location != null) {
if (!(this.locations instanceof HashMap)) {
this.locations = this.locations != null ? new HashMap<>(this.locations) : new HashMap<>();
}
this.locations.put(key, location);
}
return this;
}
@Nonnull
public Builder importedFrom(InputLocation importedFrom) {
this.importedFrom = importedFrom;
return this;
}
@Nonnull
public ToolchainModel build() {
// this method should not contain any logic other than creating (or reusing) an object in order to ease subclassing
if (base != null
&& (type == null || type == base.type)
&& (provides == null || provides == base.provides)
&& (configuration == null || configuration == base.configuration)
) {
return base;
}
return new ToolchainModel(this);
}
}
/**
* Computes a hash value based on {@link #getType()} and {@link #getProvides()} values.
*/
public int hashCode() {
return java.util.Objects.hash(getType(), getProvides());
} //-- int hashCode()
/**
* Checks equality based on {@link #getType()} and {@link #getProvides()} values.
*/
public boolean equals(Object other) {
if (this == other) {
return true;
} else if (!(other instanceof ToolchainModel)) {
return false;
} else {
ToolchainModel that = (ToolchainModel) other;
return java.util.Objects.equals(this.getType(), that.getType())
&& java.util.Objects.equals(this.getProvides(), that.getProvides());
}
} //-- boolean equals(Object)
}