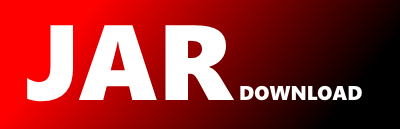
org.apache.maven.cling.invoker.LayeredOptions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of maven-cli Show documentation
Show all versions of maven-cli Show documentation
Maven CLI component, with CLI and logging support.
The newest version!
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package org.apache.maven.cling.invoker;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import java.util.function.Consumer;
import java.util.function.Function;
import org.apache.maven.api.cli.Options;
import org.apache.maven.api.cli.ParserRequest;
/**
* Options that are "layered" by precedence order.
*
* @param The type of options.
*/
public abstract class LayeredOptions implements Options {
protected final List options;
protected LayeredOptions(List options) {
this.options = new ArrayList<>(options);
}
@Override
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy