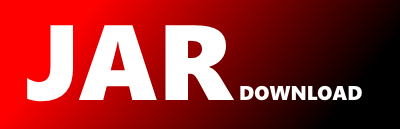
org.apache.maven.internal.impl.model.DefaultProfileActivationContext Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of maven-impl Show documentation
Show all versions of maven-impl Show documentation
Provides the implementation classes for the Maven API
The newest version!
Please wait ...
© 2015 - 2025 Weber Informatics LLC | Privacy Policy