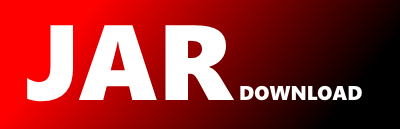
org.apache.maven.model.v4.MavenStaxWriter Maven / Gradle / Ivy
// =================== DO NOT EDIT THIS FILE ====================
// Generated by Modello Velocity from writer-stax.vm
// template, any modifications will be overwritten.
// ==============================================================
package org.apache.maven.model.v4;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.io.Reader;
import java.io.Writer;
import java.text.DateFormat;
import java.util.ArrayList;
import java.util.Date;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Properties;
import java.util.Set;
import java.util.function.Function;
import javax.xml.stream.XMLOutputFactory;
import javax.xml.stream.XMLStreamException;
import javax.xml.stream.XMLStreamWriter;
import org.apache.maven.api.annotations.Generated;
import org.apache.maven.api.model.InputLocation;
import org.apache.maven.api.model.InputLocationTracker;
import org.apache.maven.api.xml.XmlNode;
import org.apache.maven.internal.xml.XmlNodeBuilder;
import org.apache.maven.api.model.Model;
import org.apache.maven.api.model.ModelBase;
import org.apache.maven.api.model.PluginContainer;
import org.apache.maven.api.model.PluginConfiguration;
import org.apache.maven.api.model.BuildBase;
import org.apache.maven.api.model.Build;
import org.apache.maven.api.model.CiManagement;
import org.apache.maven.api.model.Notifier;
import org.apache.maven.api.model.Contributor;
import org.apache.maven.api.model.Dependency;
import org.apache.maven.api.model.Developer;
import org.apache.maven.api.model.Exclusion;
import org.apache.maven.api.model.IssueManagement;
import org.apache.maven.api.model.DistributionManagement;
import org.apache.maven.api.model.License;
import org.apache.maven.api.model.MailingList;
import org.apache.maven.api.model.Organization;
import org.apache.maven.api.model.PatternSet;
import org.apache.maven.api.model.Parent;
import org.apache.maven.api.model.Scm;
import org.apache.maven.api.model.FileSet;
import org.apache.maven.api.model.Resource;
import org.apache.maven.api.model.RepositoryBase;
import org.apache.maven.api.model.Repository;
import org.apache.maven.api.model.DeploymentRepository;
import org.apache.maven.api.model.RepositoryPolicy;
import org.apache.maven.api.model.Site;
import org.apache.maven.api.model.ConfigurationContainer;
import org.apache.maven.api.model.Plugin;
import org.apache.maven.api.model.PluginExecution;
import org.apache.maven.api.model.DependencyManagement;
import org.apache.maven.api.model.PluginManagement;
import org.apache.maven.api.model.Reporting;
import org.apache.maven.api.model.Profile;
import org.apache.maven.api.model.Activation;
import org.apache.maven.api.model.ActivationProperty;
import org.apache.maven.api.model.ActivationOS;
import org.apache.maven.api.model.ActivationFile;
import org.apache.maven.api.model.ReportPlugin;
import org.apache.maven.api.model.ReportSet;
import org.apache.maven.api.model.Prerequisites;
import org.apache.maven.api.model.Relocation;
import org.apache.maven.api.model.Extension;
import org.apache.maven.api.model.InputSource;
import org.codehaus.stax2.util.StreamWriterDelegate;
import static javax.xml.XMLConstants.W3C_XML_SCHEMA_INSTANCE_NS_URI;
@Generated
public class MavenStaxWriter {
//--------------------------/
//- Class/Member Variables -/
//--------------------------/
/**
* Default namespace.
*/
private static final String NAMESPACE = "http://maven.apache.org/POM/4.0.0";
/**
* Default schemaLocation.
*/
private static final String SCHEMA_LOCATION = "https://maven.apache.org/xsd/maven-4.0.0.xsd";
/**
* Field namespace.
*/
private String namespace = NAMESPACE;
/**
* Field schemaLocation.
*/
private String schemaLocation = SCHEMA_LOCATION;
/**
* Field fileComment.
*/
private String fileComment = null;
private boolean addLocationInformation = true;
/**
* Field stringFormatter.
*/
protected Function stringFormatter;
//-----------/
//- Methods -/
//-----------/
/**
* Method setNamespace.
*
* @param namespace the namespace to use.
*/
public void setNamespace(String namespace) {
this.namespace = Objects.requireNonNull(namespace);
} //-- void setNamespace(String)
/**
* Method setSchemaLocation.
*
* @param schemaLocation the schema location to use.
*/
public void setSchemaLocation(String schemaLocation) {
this.schemaLocation = Objects.requireNonNull(schemaLocation);
} //-- void setSchemaLocation(String)
/**
* Method setFileComment.
*
* @param fileComment a fileComment object.
*/
public void setFileComment(String fileComment) {
this.fileComment = fileComment;
} //-- void setFileComment(String)
/**
* Method setAddLocationInformation.
*/
public void setAddLocationInformation(boolean addLocationInformation) {
this.addLocationInformation = addLocationInformation;
} //-- void setAddLocationInformation(String)
/**
* Method setStringFormatter.
*
* @param stringFormatter
*/
public void setStringFormatter(Function stringFormatter) {
this.stringFormatter = stringFormatter;
} //-- void setStringFormatter(Function)
/**
* Method write.
*
* @param writer a writer object
* @param model a Model object
* @throws IOException IOException if any
*/
public void write(Writer writer, Model model) throws IOException, XMLStreamException {
XMLOutputFactory factory = new com.ctc.wstx.stax.WstxOutputFactory();
factory.setProperty(XMLOutputFactory.IS_REPAIRING_NAMESPACES, false);
factory.setProperty(com.ctc.wstx.api.WstxOutputProperties.P_USE_DOUBLE_QUOTES_IN_XML_DECL, true);
factory.setProperty(com.ctc.wstx.api.WstxOutputProperties.P_ADD_SPACE_AFTER_EMPTY_ELEM, true);
XMLStreamWriter serializer = new IndentingXMLStreamWriter(factory.createXMLStreamWriter(writer));
serializer.writeStartDocument(model.getModelEncoding(), null);
writeModel("project", model, serializer);
serializer.writeEndDocument();
} //-- void write(Writer, Model)
/**
* Method write.
*
* @param stream a stream object
* @param model a Model object
* @throws IOException IOException if any
*/
public void write(OutputStream stream, Model model) throws IOException, XMLStreamException {
XMLOutputFactory factory = new com.ctc.wstx.stax.WstxOutputFactory();
factory.setProperty(XMLOutputFactory.IS_REPAIRING_NAMESPACES, false);
factory.setProperty(com.ctc.wstx.api.WstxOutputProperties.P_USE_DOUBLE_QUOTES_IN_XML_DECL, true);
factory.setProperty(com.ctc.wstx.api.WstxOutputProperties.P_ADD_SPACE_AFTER_EMPTY_ELEM, true);
XMLStreamWriter serializer = new IndentingXMLStreamWriter(factory.createXMLStreamWriter(stream, model.getModelEncoding()));
serializer.writeStartDocument(model.getModelEncoding(), null);
writeModel("project", model, serializer);
serializer.writeEndDocument();
} //-- void write(OutputStream, Model)
private void writeModel(String tagName, Model model, XMLStreamWriter serializer)
throws IOException, XMLStreamException {
if (model != null) {
if (this.fileComment != null) {
serializer.writeCharacters("\n");
serializer.writeComment(this.fileComment);
serializer.writeCharacters("\n");
}
serializer.writeStartElement("", tagName, namespace);
serializer.writeNamespace("", namespace);
serializer.writeNamespace("xsi", W3C_XML_SCHEMA_INSTANCE_NS_URI);
serializer.writeAttribute(W3C_XML_SCHEMA_INSTANCE_NS_URI, "schemaLocation", namespace + " " + schemaLocation);
writeAttr("child.project.url.inherit.append.path", model.getChildProjectUrlInheritAppendPath(), serializer);
writeAttr("root", model.isRoot() ? "true" : null, serializer);
writeAttr("preserve.model.version", model.isPreserveModelVersion() ? "true" : null, serializer);
writeTag("modelVersion", null, model.getModelVersion(), serializer, model);
writeParent("parent", model.getParent(), serializer);
writeTag("groupId", null, model.getGroupId(), serializer, model);
writeTag("artifactId", null, model.getArtifactId(), serializer, model);
writeTag("version", null, model.getVersion(), serializer, model);
writeTag("packaging", "jar", model.getPackaging(), serializer, model);
writeTag("name", null, model.getName(), serializer, model);
writeTag("description", null, model.getDescription(), serializer, model);
writeTag("url", null, model.getUrl(), serializer, model);
writeTag("inceptionYear", null, model.getInceptionYear(), serializer, model);
writeOrganization("organization", model.getOrganization(), serializer);
writeList("licenses", false, model.getLicenses(), serializer, model,
t -> writeLicense("license", t, serializer));
writeList("developers", false, model.getDevelopers(), serializer, model,
t -> writeDeveloper("developer", t, serializer));
writeList("contributors", false, model.getContributors(), serializer, model,
t -> writeContributor("contributor", t, serializer));
writeList("mailingLists", false, model.getMailingLists(), serializer, model,
t -> writeMailingList("mailingList", t, serializer));
writePrerequisites("prerequisites", model.getPrerequisites(), serializer);
writeList("modules", model.getModules(), serializer, model,
t -> writeTag("module", null, t, serializer, null));
writeList("subprojects", model.getSubprojects(), serializer, model,
t -> writeTag("subproject", null, t, serializer, null));
writeScm("scm", model.getScm(), serializer);
writeIssueManagement("issueManagement", model.getIssueManagement(), serializer);
writeCiManagement("ciManagement", model.getCiManagement(), serializer);
writeDistributionManagement("distributionManagement", model.getDistributionManagement(), serializer);
writeProperties("properties", model.getProperties(), serializer, model);
writeDependencyManagement("dependencyManagement", model.getDependencyManagement(), serializer);
writeList("dependencies", false, model.getDependencies(), serializer, model,
t -> writeDependency("dependency", t, serializer));
writeList("repositories", false, model.getRepositories(), serializer, model,
t -> writeRepository("repository", t, serializer));
writeList("pluginRepositories", false, model.getPluginRepositories(), serializer, model,
t -> writeRepository("pluginRepository", t, serializer));
writeBuild("build", model.getBuild(), serializer);
writeReporting("reporting", model.getReporting(), serializer);
writeList("profiles", false, model.getProfiles(), serializer, model,
t -> writeProfile("profile", t, serializer));
serializer.writeEndElement();
}
}
private void writeModelBase(String tagName, ModelBase modelBase, XMLStreamWriter serializer)
throws IOException, XMLStreamException {
if (modelBase != null) {
serializer.writeStartElement(namespace, tagName);
writeList("modules", modelBase.getModules(), serializer, modelBase,
t -> writeTag("module", null, t, serializer, null));
writeList("subprojects", modelBase.getSubprojects(), serializer, modelBase,
t -> writeTag("subproject", null, t, serializer, null));
writeDistributionManagement("distributionManagement", modelBase.getDistributionManagement(), serializer);
writeProperties("properties", modelBase.getProperties(), serializer, modelBase);
writeDependencyManagement("dependencyManagement", modelBase.getDependencyManagement(), serializer);
writeList("dependencies", false, modelBase.getDependencies(), serializer, modelBase,
t -> writeDependency("dependency", t, serializer));
writeList("repositories", false, modelBase.getRepositories(), serializer, modelBase,
t -> writeRepository("repository", t, serializer));
writeList("pluginRepositories", false, modelBase.getPluginRepositories(), serializer, modelBase,
t -> writeRepository("pluginRepository", t, serializer));
writeReporting("reporting", modelBase.getReporting(), serializer);
serializer.writeEndElement();
}
}
private void writePluginContainer(String tagName, PluginContainer pluginContainer, XMLStreamWriter serializer)
throws IOException, XMLStreamException {
if (pluginContainer != null) {
serializer.writeStartElement(namespace, tagName);
writeList("plugins", false, pluginContainer.getPlugins(), serializer, pluginContainer,
t -> writePlugin("plugin", t, serializer));
serializer.writeEndElement();
}
}
private void writePluginConfiguration(String tagName, PluginConfiguration pluginConfiguration, XMLStreamWriter serializer)
throws IOException, XMLStreamException {
if (pluginConfiguration != null) {
serializer.writeStartElement(namespace, tagName);
writePluginManagement("pluginManagement", pluginConfiguration.getPluginManagement(), serializer);
writeList("plugins", false, pluginConfiguration.getPlugins(), serializer, pluginConfiguration,
t -> writePlugin("plugin", t, serializer));
serializer.writeEndElement();
}
}
private void writeBuildBase(String tagName, BuildBase buildBase, XMLStreamWriter serializer)
throws IOException, XMLStreamException {
if (buildBase != null) {
serializer.writeStartElement(namespace, tagName);
writeTag("defaultGoal", null, buildBase.getDefaultGoal(), serializer, buildBase);
writeList("resources", false, buildBase.getResources(), serializer, buildBase,
t -> writeResource("resource", t, serializer));
writeList("testResources", false, buildBase.getTestResources(), serializer, buildBase,
t -> writeResource("testResource", t, serializer));
writeTag("directory", null, buildBase.getDirectory(), serializer, buildBase);
writeTag("finalName", null, buildBase.getFinalName(), serializer, buildBase);
writeList("filters", buildBase.getFilters(), serializer, buildBase,
t -> writeTag("filter", null, t, serializer, null));
writePluginManagement("pluginManagement", buildBase.getPluginManagement(), serializer);
writeList("plugins", false, buildBase.getPlugins(), serializer, buildBase,
t -> writePlugin("plugin", t, serializer));
serializer.writeEndElement();
}
}
private void writeBuild(String tagName, Build build, XMLStreamWriter serializer)
throws IOException, XMLStreamException {
if (build != null) {
serializer.writeStartElement(namespace, tagName);
writeTag("sourceDirectory", null, build.getSourceDirectory(), serializer, build);
writeTag("scriptSourceDirectory", null, build.getScriptSourceDirectory(), serializer, build);
writeTag("testSourceDirectory", null, build.getTestSourceDirectory(), serializer, build);
writeTag("outputDirectory", null, build.getOutputDirectory(), serializer, build);
writeTag("testOutputDirectory", null, build.getTestOutputDirectory(), serializer, build);
writeList("extensions", false, build.getExtensions(), serializer, build,
t -> writeExtension("extension", t, serializer));
writeTag("defaultGoal", null, build.getDefaultGoal(), serializer, build);
writeList("resources", false, build.getResources(), serializer, build,
t -> writeResource("resource", t, serializer));
writeList("testResources", false, build.getTestResources(), serializer, build,
t -> writeResource("testResource", t, serializer));
writeTag("directory", null, build.getDirectory(), serializer, build);
writeTag("finalName", null, build.getFinalName(), serializer, build);
writeList("filters", build.getFilters(), serializer, build,
t -> writeTag("filter", null, t, serializer, null));
writePluginManagement("pluginManagement", build.getPluginManagement(), serializer);
writeList("plugins", false, build.getPlugins(), serializer, build,
t -> writePlugin("plugin", t, serializer));
serializer.writeEndElement();
}
}
private void writeCiManagement(String tagName, CiManagement ciManagement, XMLStreamWriter serializer)
throws IOException, XMLStreamException {
if (ciManagement != null) {
serializer.writeStartElement(namespace, tagName);
writeTag("system", null, ciManagement.getSystem(), serializer, ciManagement);
writeTag("url", null, ciManagement.getUrl(), serializer, ciManagement);
writeList("notifiers", false, ciManagement.getNotifiers(), serializer, ciManagement,
t -> writeNotifier("notifier", t, serializer));
serializer.writeEndElement();
}
}
private void writeNotifier(String tagName, Notifier notifier, XMLStreamWriter serializer)
throws IOException, XMLStreamException {
if (notifier != null) {
serializer.writeStartElement(namespace, tagName);
writeTag("type", "mail", notifier.getType(), serializer, notifier);
writeTag("sendOnError", "true", notifier.isSendOnError() ? null : "false", serializer, notifier);
writeTag("sendOnFailure", "true", notifier.isSendOnFailure() ? null : "false", serializer, notifier);
writeTag("sendOnSuccess", "true", notifier.isSendOnSuccess() ? null : "false", serializer, notifier);
writeTag("sendOnWarning", "true", notifier.isSendOnWarning() ? null : "false", serializer, notifier);
writeTag("address", null, notifier.getAddress(), serializer, notifier);
writeProperties("configuration", notifier.getConfiguration(), serializer, notifier);
serializer.writeEndElement();
}
}
private void writeContributor(String tagName, Contributor contributor, XMLStreamWriter serializer)
throws IOException, XMLStreamException {
if (contributor != null) {
serializer.writeStartElement(namespace, tagName);
writeTag("name", null, contributor.getName(), serializer, contributor);
writeTag("email", null, contributor.getEmail(), serializer, contributor);
writeTag("url", null, contributor.getUrl(), serializer, contributor);
writeTag("organization", null, contributor.getOrganization(), serializer, contributor);
writeTag("organizationUrl", null, contributor.getOrganizationUrl(), serializer, contributor);
writeList("roles", contributor.getRoles(), serializer, contributor,
t -> writeTag("role", null, t, serializer, null));
writeTag("timezone", null, contributor.getTimezone(), serializer, contributor);
writeProperties("properties", contributor.getProperties(), serializer, contributor);
serializer.writeEndElement();
}
}
private void writeDependency(String tagName, Dependency dependency, XMLStreamWriter serializer)
throws IOException, XMLStreamException {
if (dependency != null) {
serializer.writeStartElement(namespace, tagName);
writeTag("groupId", null, dependency.getGroupId(), serializer, dependency);
writeTag("artifactId", null, dependency.getArtifactId(), serializer, dependency);
writeTag("version", null, dependency.getVersion(), serializer, dependency);
writeTag("type", "jar", dependency.getType(), serializer, dependency);
writeTag("classifier", null, dependency.getClassifier(), serializer, dependency);
writeTag("scope", null, dependency.getScope(), serializer, dependency);
writeTag("systemPath", null, dependency.getSystemPath(), serializer, dependency);
writeList("exclusions", false, dependency.getExclusions(), serializer, dependency,
t -> writeExclusion("exclusion", t, serializer));
writeTag("optional", null, dependency.getOptional(), serializer, dependency);
serializer.writeEndElement();
}
}
private void writeDeveloper(String tagName, Developer developer, XMLStreamWriter serializer)
throws IOException, XMLStreamException {
if (developer != null) {
serializer.writeStartElement(namespace, tagName);
writeTag("id", null, developer.getId(), serializer, developer);
writeTag("name", null, developer.getName(), serializer, developer);
writeTag("email", null, developer.getEmail(), serializer, developer);
writeTag("url", null, developer.getUrl(), serializer, developer);
writeTag("organization", null, developer.getOrganization(), serializer, developer);
writeTag("organizationUrl", null, developer.getOrganizationUrl(), serializer, developer);
writeList("roles", developer.getRoles(), serializer, developer,
t -> writeTag("role", null, t, serializer, null));
writeTag("timezone", null, developer.getTimezone(), serializer, developer);
writeProperties("properties", developer.getProperties(), serializer, developer);
serializer.writeEndElement();
}
}
private void writeExclusion(String tagName, Exclusion exclusion, XMLStreamWriter serializer)
throws IOException, XMLStreamException {
if (exclusion != null) {
serializer.writeStartElement(namespace, tagName);
writeTag("groupId", null, exclusion.getGroupId(), serializer, exclusion);
writeTag("artifactId", null, exclusion.getArtifactId(), serializer, exclusion);
serializer.writeEndElement();
}
}
private void writeIssueManagement(String tagName, IssueManagement issueManagement, XMLStreamWriter serializer)
throws IOException, XMLStreamException {
if (issueManagement != null) {
serializer.writeStartElement(namespace, tagName);
writeTag("system", null, issueManagement.getSystem(), serializer, issueManagement);
writeTag("url", null, issueManagement.getUrl(), serializer, issueManagement);
serializer.writeEndElement();
}
}
private void writeDistributionManagement(String tagName, DistributionManagement distributionManagement, XMLStreamWriter serializer)
throws IOException, XMLStreamException {
if (distributionManagement != null) {
serializer.writeStartElement(namespace, tagName);
writeDeploymentRepository("repository", distributionManagement.getRepository(), serializer);
writeDeploymentRepository("snapshotRepository", distributionManagement.getSnapshotRepository(), serializer);
writeSite("site", distributionManagement.getSite(), serializer);
writeTag("downloadUrl", null, distributionManagement.getDownloadUrl(), serializer, distributionManagement);
writeRelocation("relocation", distributionManagement.getRelocation(), serializer);
writeTag("status", null, distributionManagement.getStatus(), serializer, distributionManagement);
serializer.writeEndElement();
}
}
private void writeLicense(String tagName, License license, XMLStreamWriter serializer)
throws IOException, XMLStreamException {
if (license != null) {
serializer.writeStartElement(namespace, tagName);
writeTag("name", null, license.getName(), serializer, license);
writeTag("url", null, license.getUrl(), serializer, license);
writeTag("distribution", null, license.getDistribution(), serializer, license);
writeTag("comments", null, license.getComments(), serializer, license);
serializer.writeEndElement();
}
}
private void writeMailingList(String tagName, MailingList mailingList, XMLStreamWriter serializer)
throws IOException, XMLStreamException {
if (mailingList != null) {
serializer.writeStartElement(namespace, tagName);
writeTag("name", null, mailingList.getName(), serializer, mailingList);
writeTag("subscribe", null, mailingList.getSubscribe(), serializer, mailingList);
writeTag("unsubscribe", null, mailingList.getUnsubscribe(), serializer, mailingList);
writeTag("post", null, mailingList.getPost(), serializer, mailingList);
writeTag("archive", null, mailingList.getArchive(), serializer, mailingList);
writeList("otherArchives", mailingList.getOtherArchives(), serializer, mailingList,
t -> writeTag("otherArchive", null, t, serializer, null));
serializer.writeEndElement();
}
}
private void writeOrganization(String tagName, Organization organization, XMLStreamWriter serializer)
throws IOException, XMLStreamException {
if (organization != null) {
serializer.writeStartElement(namespace, tagName);
writeTag("name", null, organization.getName(), serializer, organization);
writeTag("url", null, organization.getUrl(), serializer, organization);
serializer.writeEndElement();
}
}
private void writePatternSet(String tagName, PatternSet patternSet, XMLStreamWriter serializer)
throws IOException, XMLStreamException {
if (patternSet != null) {
serializer.writeStartElement(namespace, tagName);
writeList("includes", patternSet.getIncludes(), serializer, patternSet,
t -> writeTag("include", null, t, serializer, null));
writeList("excludes", patternSet.getExcludes(), serializer, patternSet,
t -> writeTag("exclude", null, t, serializer, null));
serializer.writeEndElement();
}
}
private void writeParent(String tagName, Parent parent, XMLStreamWriter serializer)
throws IOException, XMLStreamException {
if (parent != null) {
serializer.writeStartElement(namespace, tagName);
writeTag("groupId", null, parent.getGroupId(), serializer, parent);
writeTag("artifactId", null, parent.getArtifactId(), serializer, parent);
writeTag("version", null, parent.getVersion(), serializer, parent);
writeTag("relativePath", null, parent.getRelativePath(), serializer, parent);
serializer.writeEndElement();
}
}
private void writeScm(String tagName, Scm scm, XMLStreamWriter serializer)
throws IOException, XMLStreamException {
if (scm != null) {
serializer.writeStartElement(namespace, tagName);
writeAttr("child.scm.connection.inherit.append.path", scm.getChildScmConnectionInheritAppendPath(), serializer);
writeAttr("child.scm.developerConnection.inherit.append.path", scm.getChildScmDeveloperConnectionInheritAppendPath(), serializer);
writeAttr("child.scm.url.inherit.append.path", scm.getChildScmUrlInheritAppendPath(), serializer);
writeTag("connection", null, scm.getConnection(), serializer, scm);
writeTag("developerConnection", null, scm.getDeveloperConnection(), serializer, scm);
writeTag("tag", "HEAD", scm.getTag(), serializer, scm);
writeTag("url", null, scm.getUrl(), serializer, scm);
serializer.writeEndElement();
}
}
private void writeFileSet(String tagName, FileSet fileSet, XMLStreamWriter serializer)
throws IOException, XMLStreamException {
if (fileSet != null) {
serializer.writeStartElement(namespace, tagName);
writeTag("directory", null, fileSet.getDirectory(), serializer, fileSet);
writeList("includes", fileSet.getIncludes(), serializer, fileSet,
t -> writeTag("include", null, t, serializer, null));
writeList("excludes", fileSet.getExcludes(), serializer, fileSet,
t -> writeTag("exclude", null, t, serializer, null));
serializer.writeEndElement();
}
}
private void writeResource(String tagName, Resource resource, XMLStreamWriter serializer)
throws IOException, XMLStreamException {
if (resource != null) {
serializer.writeStartElement(namespace, tagName);
writeTag("targetPath", null, resource.getTargetPath(), serializer, resource);
writeTag("filtering", null, resource.getFiltering(), serializer, resource);
writeTag("directory", null, resource.getDirectory(), serializer, resource);
writeList("includes", resource.getIncludes(), serializer, resource,
t -> writeTag("include", null, t, serializer, null));
writeList("excludes", resource.getExcludes(), serializer, resource,
t -> writeTag("exclude", null, t, serializer, null));
serializer.writeEndElement();
}
}
private void writeRepositoryBase(String tagName, RepositoryBase repositoryBase, XMLStreamWriter serializer)
throws IOException, XMLStreamException {
if (repositoryBase != null) {
serializer.writeStartElement(namespace, tagName);
writeTag("id", null, repositoryBase.getId(), serializer, repositoryBase);
writeTag("name", null, repositoryBase.getName(), serializer, repositoryBase);
writeTag("url", null, repositoryBase.getUrl(), serializer, repositoryBase);
writeTag("layout", "default", repositoryBase.getLayout(), serializer, repositoryBase);
serializer.writeEndElement();
}
}
private void writeRepository(String tagName, Repository repository, XMLStreamWriter serializer)
throws IOException, XMLStreamException {
if (repository != null) {
serializer.writeStartElement(namespace, tagName);
writeRepositoryPolicy("releases", repository.getReleases(), serializer);
writeRepositoryPolicy("snapshots", repository.getSnapshots(), serializer);
writeTag("id", null, repository.getId(), serializer, repository);
writeTag("name", null, repository.getName(), serializer, repository);
writeTag("url", null, repository.getUrl(), serializer, repository);
writeTag("layout", "default", repository.getLayout(), serializer, repository);
serializer.writeEndElement();
}
}
private void writeDeploymentRepository(String tagName, DeploymentRepository deploymentRepository, XMLStreamWriter serializer)
throws IOException, XMLStreamException {
if (deploymentRepository != null) {
serializer.writeStartElement(namespace, tagName);
writeTag("uniqueVersion", "true", deploymentRepository.isUniqueVersion() ? null : "false", serializer, deploymentRepository);
writeRepositoryPolicy("releases", deploymentRepository.getReleases(), serializer);
writeRepositoryPolicy("snapshots", deploymentRepository.getSnapshots(), serializer);
writeTag("id", null, deploymentRepository.getId(), serializer, deploymentRepository);
writeTag("name", null, deploymentRepository.getName(), serializer, deploymentRepository);
writeTag("url", null, deploymentRepository.getUrl(), serializer, deploymentRepository);
writeTag("layout", "default", deploymentRepository.getLayout(), serializer, deploymentRepository);
serializer.writeEndElement();
}
}
private void writeRepositoryPolicy(String tagName, RepositoryPolicy repositoryPolicy, XMLStreamWriter serializer)
throws IOException, XMLStreamException {
if (repositoryPolicy != null) {
serializer.writeStartElement(namespace, tagName);
writeTag("enabled", null, repositoryPolicy.getEnabled(), serializer, repositoryPolicy);
writeTag("updatePolicy", null, repositoryPolicy.getUpdatePolicy(), serializer, repositoryPolicy);
writeTag("checksumPolicy", null, repositoryPolicy.getChecksumPolicy(), serializer, repositoryPolicy);
serializer.writeEndElement();
}
}
private void writeSite(String tagName, Site site, XMLStreamWriter serializer)
throws IOException, XMLStreamException {
if (site != null) {
serializer.writeStartElement(namespace, tagName);
writeAttr("child.site.url.inherit.append.path", site.getChildSiteUrlInheritAppendPath(), serializer);
writeTag("id", null, site.getId(), serializer, site);
writeTag("name", null, site.getName(), serializer, site);
writeTag("url", null, site.getUrl(), serializer, site);
serializer.writeEndElement();
}
}
private void writeConfigurationContainer(String tagName, ConfigurationContainer configurationContainer, XMLStreamWriter serializer)
throws IOException, XMLStreamException {
if (configurationContainer != null) {
serializer.writeStartElement(namespace, tagName);
writeTag("inherited", null, configurationContainer.getInherited(), serializer, configurationContainer);
writeDom(configurationContainer.getConfiguration(), serializer);
serializer.writeEndElement();
}
}
private void writePlugin(String tagName, Plugin plugin, XMLStreamWriter serializer)
throws IOException, XMLStreamException {
if (plugin != null) {
serializer.writeStartElement(namespace, tagName);
writeTag("groupId", "org.apache.maven.plugins", plugin.getGroupId(), serializer, plugin);
writeTag("artifactId", null, plugin.getArtifactId(), serializer, plugin);
writeTag("version", null, plugin.getVersion(), serializer, plugin);
writeTag("extensions", null, plugin.getExtensions(), serializer, plugin);
writeList("executions", false, plugin.getExecutions(), serializer, plugin,
t -> writePluginExecution("execution", t, serializer));
writeList("dependencies", false, plugin.getDependencies(), serializer, plugin,
t -> writeDependency("dependency", t, serializer));
writeTag("inherited", null, plugin.getInherited(), serializer, plugin);
writeDom(plugin.getConfiguration(), serializer);
serializer.writeEndElement();
}
}
private void writePluginExecution(String tagName, PluginExecution pluginExecution, XMLStreamWriter serializer)
throws IOException, XMLStreamException {
if (pluginExecution != null) {
serializer.writeStartElement(namespace, tagName);
writeTag("id", "default", pluginExecution.getId(), serializer, pluginExecution);
writeTag("phase", null, pluginExecution.getPhase(), serializer, pluginExecution);
writeList("goals", pluginExecution.getGoals(), serializer, pluginExecution,
t -> writeTag("goal", null, t, serializer, null));
writeTag("inherited", null, pluginExecution.getInherited(), serializer, pluginExecution);
writeDom(pluginExecution.getConfiguration(), serializer);
serializer.writeEndElement();
}
}
private void writeDependencyManagement(String tagName, DependencyManagement dependencyManagement, XMLStreamWriter serializer)
throws IOException, XMLStreamException {
if (dependencyManagement != null) {
serializer.writeStartElement(namespace, tagName);
writeList("dependencies", false, dependencyManagement.getDependencies(), serializer, dependencyManagement,
t -> writeDependency("dependency", t, serializer));
serializer.writeEndElement();
}
}
private void writePluginManagement(String tagName, PluginManagement pluginManagement, XMLStreamWriter serializer)
throws IOException, XMLStreamException {
if (pluginManagement != null) {
serializer.writeStartElement(namespace, tagName);
writeList("plugins", false, pluginManagement.getPlugins(), serializer, pluginManagement,
t -> writePlugin("plugin", t, serializer));
serializer.writeEndElement();
}
}
private void writeReporting(String tagName, Reporting reporting, XMLStreamWriter serializer)
throws IOException, XMLStreamException {
if (reporting != null) {
serializer.writeStartElement(namespace, tagName);
writeTag("excludeDefaults", null, reporting.getExcludeDefaults(), serializer, reporting);
writeTag("outputDirectory", null, reporting.getOutputDirectory(), serializer, reporting);
writeList("plugins", false, reporting.getPlugins(), serializer, reporting,
t -> writeReportPlugin("plugin", t, serializer));
serializer.writeEndElement();
}
}
private void writeProfile(String tagName, Profile profile, XMLStreamWriter serializer)
throws IOException, XMLStreamException {
if (profile != null) {
serializer.writeStartElement(namespace, tagName);
writeTag("id", "default", profile.getId(), serializer, profile);
writeActivation("activation", profile.getActivation(), serializer);
writeBuildBase("build", profile.getBuild(), serializer);
writeList("modules", profile.getModules(), serializer, profile,
t -> writeTag("module", null, t, serializer, null));
writeList("subprojects", profile.getSubprojects(), serializer, profile,
t -> writeTag("subproject", null, t, serializer, null));
writeDistributionManagement("distributionManagement", profile.getDistributionManagement(), serializer);
writeProperties("properties", profile.getProperties(), serializer, profile);
writeDependencyManagement("dependencyManagement", profile.getDependencyManagement(), serializer);
writeList("dependencies", false, profile.getDependencies(), serializer, profile,
t -> writeDependency("dependency", t, serializer));
writeList("repositories", false, profile.getRepositories(), serializer, profile,
t -> writeRepository("repository", t, serializer));
writeList("pluginRepositories", false, profile.getPluginRepositories(), serializer, profile,
t -> writeRepository("pluginRepository", t, serializer));
writeReporting("reporting", profile.getReporting(), serializer);
serializer.writeEndElement();
}
}
private void writeActivation(String tagName, Activation activation, XMLStreamWriter serializer)
throws IOException, XMLStreamException {
if (activation != null) {
serializer.writeStartElement(namespace, tagName);
writeTag("activeByDefault", "false", activation.isActiveByDefault() ? "true" : null, serializer, activation);
writeTag("jdk", null, activation.getJdk(), serializer, activation);
writeActivationOS("os", activation.getOs(), serializer);
writeActivationProperty("property", activation.getProperty(), serializer);
writeActivationFile("file", activation.getFile(), serializer);
writeTag("packaging", null, activation.getPackaging(), serializer, activation);
writeTag("condition", null, activation.getCondition(), serializer, activation);
serializer.writeEndElement();
}
}
private void writeActivationProperty(String tagName, ActivationProperty activationProperty, XMLStreamWriter serializer)
throws IOException, XMLStreamException {
if (activationProperty != null) {
serializer.writeStartElement(namespace, tagName);
writeTag("name", null, activationProperty.getName(), serializer, activationProperty);
writeTag("value", null, activationProperty.getValue(), serializer, activationProperty);
serializer.writeEndElement();
}
}
private void writeActivationOS(String tagName, ActivationOS activationOS, XMLStreamWriter serializer)
throws IOException, XMLStreamException {
if (activationOS != null) {
serializer.writeStartElement(namespace, tagName);
writeTag("name", null, activationOS.getName(), serializer, activationOS);
writeTag("family", null, activationOS.getFamily(), serializer, activationOS);
writeTag("arch", null, activationOS.getArch(), serializer, activationOS);
writeTag("version", null, activationOS.getVersion(), serializer, activationOS);
serializer.writeEndElement();
}
}
private void writeActivationFile(String tagName, ActivationFile activationFile, XMLStreamWriter serializer)
throws IOException, XMLStreamException {
if (activationFile != null) {
serializer.writeStartElement(namespace, tagName);
writeTag("missing", null, activationFile.getMissing(), serializer, activationFile);
writeTag("exists", null, activationFile.getExists(), serializer, activationFile);
serializer.writeEndElement();
}
}
private void writeReportPlugin(String tagName, ReportPlugin reportPlugin, XMLStreamWriter serializer)
throws IOException, XMLStreamException {
if (reportPlugin != null) {
serializer.writeStartElement(namespace, tagName);
writeTag("groupId", "org.apache.maven.plugins", reportPlugin.getGroupId(), serializer, reportPlugin);
writeTag("artifactId", null, reportPlugin.getArtifactId(), serializer, reportPlugin);
writeTag("version", null, reportPlugin.getVersion(), serializer, reportPlugin);
writeList("reportSets", false, reportPlugin.getReportSets(), serializer, reportPlugin,
t -> writeReportSet("reportSet", t, serializer));
writeTag("inherited", null, reportPlugin.getInherited(), serializer, reportPlugin);
writeDom(reportPlugin.getConfiguration(), serializer);
serializer.writeEndElement();
}
}
private void writeReportSet(String tagName, ReportSet reportSet, XMLStreamWriter serializer)
throws IOException, XMLStreamException {
if (reportSet != null) {
serializer.writeStartElement(namespace, tagName);
writeTag("id", "default", reportSet.getId(), serializer, reportSet);
writeList("reports", reportSet.getReports(), serializer, reportSet,
t -> writeTag("report", null, t, serializer, null));
writeTag("inherited", null, reportSet.getInherited(), serializer, reportSet);
writeDom(reportSet.getConfiguration(), serializer);
serializer.writeEndElement();
}
}
private void writePrerequisites(String tagName, Prerequisites prerequisites, XMLStreamWriter serializer)
throws IOException, XMLStreamException {
if (prerequisites != null) {
serializer.writeStartElement(namespace, tagName);
writeTag("maven", "2.0", prerequisites.getMaven(), serializer, prerequisites);
serializer.writeEndElement();
}
}
private void writeRelocation(String tagName, Relocation relocation, XMLStreamWriter serializer)
throws IOException, XMLStreamException {
if (relocation != null) {
serializer.writeStartElement(namespace, tagName);
writeTag("groupId", null, relocation.getGroupId(), serializer, relocation);
writeTag("artifactId", null, relocation.getArtifactId(), serializer, relocation);
writeTag("version", null, relocation.getVersion(), serializer, relocation);
writeTag("message", null, relocation.getMessage(), serializer, relocation);
serializer.writeEndElement();
}
}
private void writeExtension(String tagName, Extension extension, XMLStreamWriter serializer)
throws IOException, XMLStreamException {
if (extension != null) {
serializer.writeStartElement(namespace, tagName);
writeTag("groupId", null, extension.getGroupId(), serializer, extension);
writeTag("artifactId", null, extension.getArtifactId(), serializer, extension);
writeTag("version", null, extension.getVersion(), serializer, extension);
writeDom(extension.getConfiguration(), serializer);
serializer.writeEndElement();
}
}
@FunctionalInterface
private interface ElementWriter {
public void write(T t) throws IOException, XMLStreamException;
}
private void writeList(String tagName, List list, XMLStreamWriter serializer, InputLocationTracker locationTracker, ElementWriter writer) throws IOException, XMLStreamException {
writeList(tagName, false, list, serializer, locationTracker, writer);
}
private void writeList(String tagName, boolean flat, List list, XMLStreamWriter serializer, InputLocationTracker locationTracker, ElementWriter writer) throws IOException, XMLStreamException {
if (list != null && !list.isEmpty()) {
if (!flat) {
serializer.writeStartElement(namespace, tagName);
}
int index = 0;
InputLocation location = locationTracker != null ? locationTracker.getLocation(tagName) : null;
for (T t : list) {
writer.write(t);
writeLocationTracking(location, Integer.valueOf(index++), serializer);
}
if (!flat) {
serializer.writeEndElement();
}
}
}
private void writeProperties(String tagName, Map props, XMLStreamWriter serializer, InputLocationTracker locationTracker) throws IOException, XMLStreamException {
if (props != null && !props.isEmpty()) {
serializer.writeStartElement(namespace, tagName);
InputLocation location = locationTracker != null ? locationTracker.getLocation(tagName) : null;
for (Map.Entry entry : props.entrySet()) {
String key = entry.getKey();
writeTag(key, null, entry.getValue(), serializer, null);
writeLocationTracking(location, key, serializer);
}
serializer.writeEndElement();
}
}
private void writeDom(XmlNode dom, XMLStreamWriter serializer) throws IOException, XMLStreamException {
if (dom != null) {
serializer.writeStartElement(namespace, dom.getName());
for (Map.Entry attr : dom.getAttributes().entrySet()) {
if (attr.getKey().startsWith("xml:")) {
serializer.writeAttribute("http://www.w3.org/XML/1998/namespace",
attr.getKey().substring(4), attr.getValue());
} else {
serializer.writeAttribute(attr.getKey(), attr.getValue());
}
}
for (XmlNode child : dom.getChildren()) {
writeDom(child, serializer);
}
String value = dom.getValue();
if (value != null) {
serializer.writeCharacters(value);
}
serializer.writeEndElement();
if (addLocationInformation && dom.getInputLocation() instanceof InputLocation && dom.getChildren().isEmpty()) {
serializer.writeComment(toString((InputLocation) dom.getInputLocation()));
}
}
}
private void writeTag(String tagName, String defaultValue, String value, XMLStreamWriter serializer, InputLocationTracker locationTracker) throws IOException, XMLStreamException {
if (value != null && !Objects.equals(defaultValue, value)) {
serializer.writeStartElement(namespace, tagName);
serializer.writeCharacters(value);
serializer.writeEndElement();
writeLocationTracking(locationTracker, tagName, serializer);
}
}
private void writeAttr(String attrName, String value, XMLStreamWriter serializer) throws IOException, XMLStreamException {
if (value != null) {
serializer.writeAttribute(attrName, value);
}
}
/**
* Method writeLocationTracking.
*
* @param locationTracker
* @param serializer
* @param key
* @throws IOException
*/
protected void writeLocationTracking(InputLocationTracker locationTracker, Object key, XMLStreamWriter serializer) throws IOException, XMLStreamException {
if (addLocationInformation) {
InputLocation location = (locationTracker == null) ? null : locationTracker.getLocation(key);
if (location != null) {
serializer.writeComment(toString(location));
}
}
} //-- void writeLocationTracking(InputLocationTracker, Object, XMLStreamWriter)
/**
* Method toString.
*
* @param location
* @return String
*/
protected String toString(InputLocation location) {
if (stringFormatter != null) {
return stringFormatter.apply(location);
}
if (location.getSource() != null) {
return ' ' + location.getSource().toString() + ':' + location.getLineNumber() + ' ';
} else {
return " " + location.getLineNumber() + " ";
}
} //-- String toString(InputLocation)
static class IndentingXMLStreamWriter extends StreamWriterDelegate {
int depth = 0;
boolean hasChildren = false;
public IndentingXMLStreamWriter(XMLStreamWriter parent) {
super(parent);
}
@Override
public void writeEmptyElement(String localName) throws XMLStreamException {
indent();
super.writeEmptyElement(localName);
hasChildren = true;
}
@Override
public void writeEmptyElement(String namespaceURI, String localName) throws XMLStreamException {
indent();
super.writeEmptyElement(namespaceURI, localName);
hasChildren = true;
}
@Override
public void writeEmptyElement(String prefix, String localName, String namespaceURI) throws XMLStreamException {
indent();
super.writeEmptyElement(prefix, localName, namespaceURI);
hasChildren = true;
}
@Override
public void writeStartElement(String localName) throws XMLStreamException {
indent();
super.writeStartElement(localName);
depth++;
hasChildren = false;
}
@Override
public void writeStartElement(String namespaceURI, String localName) throws XMLStreamException {
indent();
super.writeStartElement(namespaceURI, localName);
depth++;
hasChildren = false;
}
@Override
public void writeStartElement(String prefix, String localName, String namespaceURI) throws XMLStreamException {
indent();
super.writeStartElement(prefix, localName, namespaceURI);
depth++;
hasChildren = false;
}
@Override
public void writeEndElement() throws XMLStreamException {
depth--;
if (hasChildren) {
indent();
}
super.writeEndElement();
hasChildren = true;
}
private void indent() throws XMLStreamException {
super.writeCharacters("\n");
for (int i = 0; i < depth; i++) {
super.writeCharacters(" ");
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy