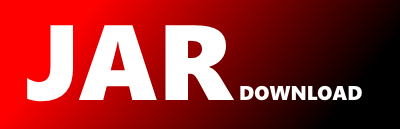
org.apache.maven.lifecycle.model.Phase Maven / Gradle / Ivy
/*
* $Id$
*/
package org.apache.maven.lifecycle.model;
//---------------------------------/
//- Imported classes and packages -/
//---------------------------------/
import java.util.Date;
/**
* Contains a series of mojo bindings for a given phase of a
* lifecycle.
*
* @version $Revision$ $Date$
*/
public class Phase implements java.io.Serializable {
//--------------------------/
//- Class/Member Variables -/
//--------------------------/
/**
* Field bindings.
*/
private java.util.List bindings;
//-----------/
//- Methods -/
//-----------/
/**
* Method addBinding.
*
* @param mojoBinding
*/
public void addBinding( MojoBinding mojoBinding )
{
if ( !(mojoBinding instanceof MojoBinding) )
{
throw new ClassCastException( "Phase.addBindings(mojoBinding) parameter must be instanceof " + MojoBinding.class.getName() );
}
getBindings().add( mojoBinding );
} //-- void addBinding( MojoBinding )
/**
* Method getBindings.
*
* @return java.util.List
*/
public java.util.List getBindings()
{
if ( this.bindings == null )
{
this.bindings = new java.util.ArrayList();
}
return this.bindings;
} //-- java.util.List getBindings()
/**
* Method removeBinding.
*
* @param mojoBinding
*/
public void removeBinding( MojoBinding mojoBinding )
{
if ( !(mojoBinding instanceof MojoBinding) )
{
throw new ClassCastException( "Phase.removeBindings(mojoBinding) parameter must be instanceof " + MojoBinding.class.getName() );
}
getBindings().remove( mojoBinding );
} //-- void removeBinding( MojoBinding )
/**
* Set collection of mojo bindings for a phase.
*
* @param bindings
*/
public void setBindings( java.util.List bindings )
{
this.bindings = bindings;
} //-- void setBindings( java.util.List )
private String name;
private LifecycleBinding lifecycleBinding;
/**
* Get the name of this phase.
*/
public String getName()
{
return name;
}
/**
* Get the LifecycleBinding instance to which this Phase belongs.
*/
public LifecycleBinding getLifecycleBinding()
{
return lifecycleBinding;
}
/**
* Set the name of this phase, and the Lifecycle instance to which is belongs.
*/
public void setLifecycleInfo( String phaseName, LifecycleBinding lifecycleBinding )
{
this.name = phaseName;
this.lifecycleBinding = lifecycleBinding;
java.util.List bindings = getBindings();
if ( bindings != null )
{
for( java.util.Iterator it = bindings.iterator(); it.hasNext(); )
{
MojoBinding binding = (MojoBinding) it.next();
binding.setLifecycleInfo( this );
}
}
}
private String modelEncoding = "UTF-8";
/**
* Set an encoding used for reading/writing the model.
*
* @param modelEncoding the encoding used when reading/writing the model.
*/
public void setModelEncoding( String modelEncoding )
{
this.modelEncoding = modelEncoding;
}
/**
* @return the current encoding used when reading/writing this model.
*/
public String getModelEncoding()
{
return modelEncoding;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy