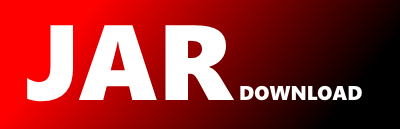
org.apache.maven.lifecycle.model.SiteBinding Maven / Gradle / Ivy
/*
* $Id$
*/
package org.apache.maven.lifecycle.model;
//---------------------------------/
//- Imported classes and packages -/
//---------------------------------/
import java.util.Date;
/**
* Class SiteBinding.
*
* @version $Revision$ $Date$
*/
public class SiteBinding extends LifecycleBinding
implements java.io.Serializable
{
//--------------------------/
//- Class/Member Variables -/
//--------------------------/
/**
* Field preSite.
*/
private Phase preSite = new Phase();
/**
* Field site.
*/
private Phase site = new Phase();
/**
* Field postSite.
*/
private Phase postSite = new Phase();
/**
* Field siteDeploy.
*/
private Phase siteDeploy = new Phase();
//-----------/
//- Methods -/
//-----------/
/**
* Get the postSite field.
*
* @return Phase
*/
public Phase getPostSite()
{
return this.postSite;
} //-- Phase getPostSite()
/**
* Get the preSite field.
*
* @return Phase
*/
public Phase getPreSite()
{
return this.preSite;
} //-- Phase getPreSite()
/**
* Get the site field.
*
* @return Phase
*/
public Phase getSite()
{
return this.site;
} //-- Phase getSite()
/**
* Get the siteDeploy field.
*
* @return Phase
*/
public Phase getSiteDeploy()
{
return this.siteDeploy;
} //-- Phase getSiteDeploy()
/**
* Set the postSite field.
*
* @param postSite
*/
public void setPostSite( Phase postSite )
{
this.postSite = postSite;
} //-- void setPostSite( Phase )
/**
* Set the preSite field.
*
* @param preSite
*/
public void setPreSite( Phase preSite )
{
this.preSite = preSite;
} //-- void setPreSite( Phase )
/**
* Set the site field.
*
* @param site
*/
public void setSite( Phase site )
{
this.site = site;
} //-- void setSite( Phase )
/**
* Set the siteDeploy field.
*
* @param siteDeploy
*/
public void setSiteDeploy( Phase siteDeploy )
{
this.siteDeploy = siteDeploy;
} //-- void setSiteDeploy( Phase )
public String getId()
{
return "site";
}
public java.util.LinkedHashMap getOrderedPhaseMapping()
{
java.util.LinkedHashMap map = new java.util.LinkedHashMap();
map.put( "pre-site", getPreSite() );
map.put( "site", getSite() );
map.put( "post-site", getPostSite() );
map.put( "site-deploy", getSiteDeploy() );
return map;
}
public java.util.List getPhasesInOrder()
{
return new java.util.ArrayList( getOrderedPhaseMapping().values() );
}
public java.util.List getPhaseNamesInOrder()
{
return new java.util.ArrayList( getOrderedPhaseMapping().keySet() );
}
private String modelEncoding = "UTF-8";
/**
* Set an encoding used for reading/writing the model.
*
* @param modelEncoding the encoding used when reading/writing the model.
*/
public void setModelEncoding( String modelEncoding )
{
this.modelEncoding = modelEncoding;
}
/**
* @return the current encoding used when reading/writing this model.
*/
public String getModelEncoding()
{
return modelEncoding;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy