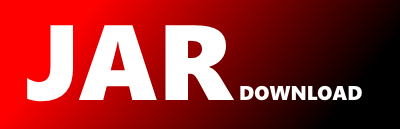
org.apache.maven.model.Dependency Maven / Gradle / Ivy
// =================== DO NOT EDIT THIS FILE ====================
// Generated by Modello Velocity from model-v3.vm
// template, any modifications will be overwritten.
// ==============================================================
package org.apache.maven.model;
import java.io.Serializable;
import java.util.AbstractList;
import java.util.ArrayList;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import org.apache.maven.api.annotations.Generated;
import org.apache.maven.api.annotations.Nonnull;
@Generated
public class Dependency
extends BaseObject
{
public Dependency() {
this(org.apache.maven.api.model.Dependency.newInstance());
}
public Dependency(org.apache.maven.api.model.Dependency delegate) {
this(delegate, null);
}
public Dependency(org.apache.maven.api.model.Dependency delegate, BaseObject parent) {
super(delegate, parent);
}
public Dependency clone(){
return new Dependency(getDelegate());
}
public org.apache.maven.api.model.Dependency getDelegate() {
return (org.apache.maven.api.model.Dependency) super.getDelegate();
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || !(o instanceof Dependency)) {
return false;
}
Dependency that = (Dependency) o;
return Objects.equals(this.delegate, that.delegate);
}
@Override
public int hashCode() {
return getDelegate().hashCode();
}
public String getGroupId() {
return getDelegate().getGroupId();
}
public void setGroupId(String groupId) {
if (!Objects.equals(groupId, getGroupId())) {
update(getDelegate().withGroupId(groupId));
}
}
public String getArtifactId() {
return getDelegate().getArtifactId();
}
public void setArtifactId(String artifactId) {
if (!Objects.equals(artifactId, getArtifactId())) {
update(getDelegate().withArtifactId(artifactId));
}
}
public String getVersion() {
return getDelegate().getVersion();
}
public void setVersion(String version) {
if (!Objects.equals(version, getVersion())) {
update(getDelegate().withVersion(version));
}
}
public String getType() {
return getDelegate().getType();
}
public void setType(String type) {
if (!Objects.equals(type, getType())) {
update(getDelegate().withType(type));
}
}
public String getClassifier() {
return getDelegate().getClassifier();
}
public void setClassifier(String classifier) {
if (!Objects.equals(classifier, getClassifier())) {
update(getDelegate().withClassifier(classifier));
}
}
public String getScope() {
return getDelegate().getScope();
}
public void setScope(String scope) {
if (!Objects.equals(scope, getScope())) {
update(getDelegate().withScope(scope));
}
}
public String getSystemPath() {
return getDelegate().getSystemPath();
}
public void setSystemPath(String systemPath) {
if (!Objects.equals(systemPath, getSystemPath())) {
update(getDelegate().withSystemPath(systemPath));
}
}
@Nonnull
public List getExclusions() {
return new WrapperList(
() -> getDelegate().getExclusions(), l -> update(getDelegate().withExclusions(l)),
d -> new Exclusion(d, this), Exclusion::getDelegate);
}
public void setExclusions(List exclusions) {
if (exclusions == null) {
exclusions = Collections.emptyList();
}
if (!Objects.equals(exclusions, getExclusions())) {
update(getDelegate().withExclusions(
exclusions.stream().map(c -> c.getDelegate()).collect(Collectors.toList())));
exclusions.forEach(e -> e.childrenTracking = this::replace);
}
}
public void addExclusion(Exclusion exclusion) {
update(getDelegate().withExclusions(
Stream.concat(getDelegate().getExclusions().stream(), Stream.of(exclusion.getDelegate()))
.collect(Collectors.toList())));
exclusion.childrenTracking = this::replace;
}
public void removeExclusion(Exclusion exclusion) {
update(getDelegate().withExclusions(
getDelegate().getExclusions().stream()
.filter(e -> !Objects.equals(e, exclusion))
.collect(Collectors.toList())));
exclusion.childrenTracking = null;
}
public String getOptional() {
return getDelegate().getOptional();
}
public void setOptional(String optional) {
if (!Objects.equals(optional, getOptional())) {
update(getDelegate().withOptional(optional));
}
}
public InputLocation getLocation(Object key) {
org.apache.maven.api.model.InputLocation loc = getDelegate().getLocation(key);
return loc != null ? new InputLocation(loc) : null;
}
public void setLocation(Object key, InputLocation location) {
update(org.apache.maven.api.model.Dependency.newBuilder(getDelegate(), true)
.location(key, location.toApiLocation()).build());
}
protected boolean replace(Object oldDelegate, Object newDelegate) {
if (super.replace(oldDelegate, newDelegate)) {
return true;
}
if (getDelegate().getExclusions().contains(oldDelegate)) {
List list = new ArrayList<>(getDelegate().getExclusions());
list.replaceAll(d -> d == oldDelegate ? (org.apache.maven.api.model.Exclusion) newDelegate : d);
update(getDelegate().withExclusions(list));
return true;
}
return false;
}
public static List dependencyToApiV4(List list) {
return list != null ? new WrapperList<>(list, Dependency::getDelegate, Dependency::new) : null;
}
public static List dependencyToApiV3(List list) {
return list != null ? new WrapperList<>(list, Dependency::new, Dependency::getDelegate) : null;
}
public boolean isOptional()
{
return ( getOptional() != null ) ? Boolean.parseBoolean( getOptional() ) : false;
}
public void setOptional( boolean optional )
{
setOptional( String.valueOf( optional ) );
}
/**
* @see java.lang.Object#toString()
*/
public String toString()
{
return "Dependency {groupId=" + getGroupId() + ", artifactId=" + getArtifactId() + ", version=" + getVersion() + ", type=" + getType() + "}";
}
private volatile String managementKey;
/**
* @return the management key as {@code groupId:artifactId:type}
*/
public String getManagementKey()
{
if ( managementKey == null )
{
managementKey = getGroupId() + ":" + getArtifactId() + ":" + getType() + ( getClassifier() != null ? ":" + getClassifier() : "" );
}
return managementKey;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy