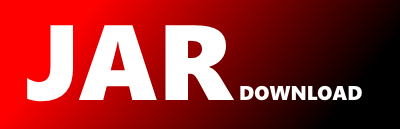
org.apache.maven.model.Model Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of maven-model Show documentation
Show all versions of maven-model Show documentation
Model for Maven POM (Project Object Model)
The newest version!
Please wait ...
© 2015 - 2025 Weber Informatics LLC | Privacy Policy