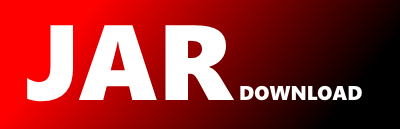
org.apache.maven.plugin.lifecycle.io.LifecycleStaxWriter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of maven-plugin-api Show documentation
Show all versions of maven-plugin-api Show documentation
The API for plugins - Mojos - development.
// =================== DO NOT EDIT THIS FILE ====================
// Generated by Modello Velocity from writer-stax.vm
// template, any modifications will be overwritten.
// ==============================================================
package org.apache.maven.plugin.lifecycle.io;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.io.Reader;
import java.io.Writer;
import java.text.DateFormat;
import java.util.ArrayList;
import java.util.Date;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Properties;
import java.util.Set;
import javax.xml.stream.XMLOutputFactory;
import javax.xml.stream.XMLStreamException;
import javax.xml.stream.XMLStreamWriter;
import org.apache.maven.api.annotations.Generated;
import org.apache.maven.api.xml.XmlNode;
import org.apache.maven.internal.xml.XmlNodeBuilder;
import org.apache.maven.api.plugin.descriptor.lifecycle.LifecycleConfiguration;
import org.apache.maven.api.plugin.descriptor.lifecycle.Lifecycle;
import org.apache.maven.api.plugin.descriptor.lifecycle.Phase;
import org.apache.maven.api.plugin.descriptor.lifecycle.Execution;
import org.codehaus.stax2.util.StreamWriterDelegate;
import static javax.xml.XMLConstants.W3C_XML_SCHEMA_INSTANCE_NS_URI;
@Generated
public class LifecycleStaxWriter {
//--------------------------/
//- Class/Member Variables -/
//--------------------------/
/**
* Default namespace.
*/
private static final String NAMESPACE = "http://maven.apache.org/LIFECYCLE/1.0.0";
/**
* Default schemaLocation.
*/
private static final String SCHEMA_LOCATION = "http://maven.apache.org/xsd/lifecycle-1.0.0.xsd";
/**
* Field namespace.
*/
private String namespace = NAMESPACE;
/**
* Field schemaLocation.
*/
private String schemaLocation = SCHEMA_LOCATION;
/**
* Field fileComment.
*/
private String fileComment = null;
//-----------/
//- Methods -/
//-----------/
/**
* Method setNamespace.
*
* @param namespace the namespace to use.
*/
public void setNamespace(String namespace) {
this.namespace = Objects.requireNonNull(namespace);
} //-- void setNamespace(String)
/**
* Method setSchemaLocation.
*
* @param schemaLocation the schema location to use.
*/
public void setSchemaLocation(String schemaLocation) {
this.schemaLocation = Objects.requireNonNull(schemaLocation);
} //-- void setSchemaLocation(String)
/**
* Method setFileComment.
*
* @param fileComment a fileComment object.
*/
public void setFileComment(String fileComment) {
this.fileComment = fileComment;
} //-- void setFileComment(String)
/**
* Method write.
*
* @param writer a writer object
* @param lifecycleConfiguration a LifecycleConfiguration object
* @throws IOException IOException if any
*/
public void write(Writer writer, LifecycleConfiguration lifecycleConfiguration) throws IOException, XMLStreamException {
XMLOutputFactory factory = new com.ctc.wstx.stax.WstxOutputFactory();
factory.setProperty(XMLOutputFactory.IS_REPAIRING_NAMESPACES, false);
factory.setProperty(com.ctc.wstx.api.WstxOutputProperties.P_USE_DOUBLE_QUOTES_IN_XML_DECL, true);
factory.setProperty(com.ctc.wstx.api.WstxOutputProperties.P_ADD_SPACE_AFTER_EMPTY_ELEM, true);
XMLStreamWriter serializer = new IndentingXMLStreamWriter(factory.createXMLStreamWriter(writer));
serializer.writeStartDocument(lifecycleConfiguration.getModelEncoding(), null);
writeLifecycleConfiguration("lifecycles", lifecycleConfiguration, serializer);
serializer.writeEndDocument();
} //-- void write(Writer, LifecycleConfiguration)
/**
* Method write.
*
* @param stream a stream object
* @param lifecycleConfiguration a LifecycleConfiguration object
* @throws IOException IOException if any
*/
public void write(OutputStream stream, LifecycleConfiguration lifecycleConfiguration) throws IOException, XMLStreamException {
XMLOutputFactory factory = new com.ctc.wstx.stax.WstxOutputFactory();
factory.setProperty(XMLOutputFactory.IS_REPAIRING_NAMESPACES, false);
factory.setProperty(com.ctc.wstx.api.WstxOutputProperties.P_USE_DOUBLE_QUOTES_IN_XML_DECL, true);
factory.setProperty(com.ctc.wstx.api.WstxOutputProperties.P_ADD_SPACE_AFTER_EMPTY_ELEM, true);
XMLStreamWriter serializer = new IndentingXMLStreamWriter(factory.createXMLStreamWriter(stream, lifecycleConfiguration.getModelEncoding()));
serializer.writeStartDocument(lifecycleConfiguration.getModelEncoding(), null);
writeLifecycleConfiguration("lifecycles", lifecycleConfiguration, serializer);
serializer.writeEndDocument();
} //-- void write(OutputStream, LifecycleConfiguration)
private void writeLifecycleConfiguration(String tagName, LifecycleConfiguration lifecycleConfiguration, XMLStreamWriter serializer)
throws IOException, XMLStreamException {
if (lifecycleConfiguration != null) {
if (this.fileComment != null) {
serializer.writeCharacters("\n");
serializer.writeComment(this.fileComment);
serializer.writeCharacters("\n");
}
serializer.writeStartElement("", tagName, namespace);
serializer.writeNamespace("", namespace);
serializer.writeNamespace("xsi", W3C_XML_SCHEMA_INSTANCE_NS_URI);
serializer.writeAttribute(W3C_XML_SCHEMA_INSTANCE_NS_URI, "schemaLocation", namespace + " " + schemaLocation);
writeList("lifecycles", true, lifecycleConfiguration.getLifecycles(), serializer,
t -> writeLifecycle("lifecycle", t, serializer));
serializer.writeEndElement();
}
}
private void writeLifecycle(String tagName, Lifecycle lifecycle, XMLStreamWriter serializer)
throws IOException, XMLStreamException {
if (lifecycle != null) {
serializer.writeStartElement(namespace, tagName);
writeTag("id", null, lifecycle.getId(), serializer);
writeList("phases", false, lifecycle.getPhases(), serializer,
t -> writePhase("phase", t, serializer));
serializer.writeEndElement();
}
}
private void writePhase(String tagName, Phase phase, XMLStreamWriter serializer)
throws IOException, XMLStreamException {
if (phase != null) {
serializer.writeStartElement(namespace, tagName);
writeTag("id", null, phase.getId(), serializer);
writeList("executions", false, phase.getExecutions(), serializer,
t -> writeExecution("execution", t, serializer));
writeDom(phase.getConfiguration(), serializer);
serializer.writeEndElement();
}
}
private void writeExecution(String tagName, Execution execution, XMLStreamWriter serializer)
throws IOException, XMLStreamException {
if (execution != null) {
serializer.writeStartElement(namespace, tagName);
writeDom(execution.getConfiguration(), serializer);
writeList("goals", execution.getGoals(), serializer,
t -> writeTag("goal", null, t, serializer));
serializer.writeEndElement();
}
}
@FunctionalInterface
private interface ElementWriter {
public void write(T t) throws IOException, XMLStreamException;
}
private void writeList(String tagName, List list, XMLStreamWriter serializer, ElementWriter writer) throws IOException, XMLStreamException {
writeList(tagName, false, list, serializer, writer);
}
private void writeList(String tagName, boolean flat, List list, XMLStreamWriter serializer, ElementWriter writer) throws IOException, XMLStreamException {
if (list != null && !list.isEmpty()) {
if (!flat) {
serializer.writeStartElement(namespace, tagName);
}
int index = 0;
for (T t : list) {
writer.write(t);
}
if (!flat) {
serializer.writeEndElement();
}
}
}
private void writeProperties(String tagName, Map props, XMLStreamWriter serializer) throws IOException, XMLStreamException {
if (props != null && !props.isEmpty()) {
serializer.writeStartElement(namespace, tagName);
for (Map.Entry entry : props.entrySet()) {
String key = entry.getKey();
writeTag(key, null, entry.getValue(), serializer);
}
serializer.writeEndElement();
}
}
private void writeDom(XmlNode dom, XMLStreamWriter serializer) throws IOException, XMLStreamException {
if (dom != null) {
serializer.writeStartElement(namespace, dom.getName());
for (Map.Entry attr : dom.getAttributes().entrySet()) {
if (attr.getKey().startsWith("xml:")) {
serializer.writeAttribute("http://www.w3.org/XML/1998/namespace",
attr.getKey().substring(4), attr.getValue());
} else {
serializer.writeAttribute(attr.getKey(), attr.getValue());
}
}
for (XmlNode child : dom.getChildren()) {
writeDom(child, serializer);
}
String value = dom.getValue();
if (value != null) {
serializer.writeCharacters(value);
}
serializer.writeEndElement();
}
}
private void writeTag(String tagName, String defaultValue, String value, XMLStreamWriter serializer) throws IOException, XMLStreamException {
if (value != null && !Objects.equals(defaultValue, value)) {
serializer.writeStartElement(namespace, tagName);
serializer.writeCharacters(value);
serializer.writeEndElement();
}
}
private void writeAttr(String attrName, String value, XMLStreamWriter serializer) throws IOException, XMLStreamException {
if (value != null) {
serializer.writeAttribute(attrName, value);
}
}
static class IndentingXMLStreamWriter extends StreamWriterDelegate {
int depth = 0;
boolean hasChildren = false;
public IndentingXMLStreamWriter(XMLStreamWriter parent) {
super(parent);
}
@Override
public void writeEmptyElement(String localName) throws XMLStreamException {
indent();
super.writeEmptyElement(localName);
hasChildren = true;
}
@Override
public void writeEmptyElement(String namespaceURI, String localName) throws XMLStreamException {
indent();
super.writeEmptyElement(namespaceURI, localName);
hasChildren = true;
}
@Override
public void writeEmptyElement(String prefix, String localName, String namespaceURI) throws XMLStreamException {
indent();
super.writeEmptyElement(prefix, localName, namespaceURI);
hasChildren = true;
}
@Override
public void writeStartElement(String localName) throws XMLStreamException {
indent();
super.writeStartElement(localName);
depth++;
hasChildren = false;
}
@Override
public void writeStartElement(String namespaceURI, String localName) throws XMLStreamException {
indent();
super.writeStartElement(namespaceURI, localName);
depth++;
hasChildren = false;
}
@Override
public void writeStartElement(String prefix, String localName, String namespaceURI) throws XMLStreamException {
indent();
super.writeStartElement(prefix, localName, namespaceURI);
depth++;
hasChildren = false;
}
@Override
public void writeEndElement() throws XMLStreamException {
depth--;
if (hasChildren) {
indent();
}
super.writeEndElement();
hasChildren = true;
}
private void indent() throws XMLStreamException {
super.writeCharacters("\n");
for (int i = 0; i < depth; i++) {
super.writeCharacters(" ");
}
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy