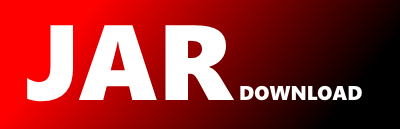
org.apache.maven.plugin.tools.model.Mojo Maven / Gradle / Ivy
/*
* $Id$
*/
package org.apache.maven.plugin.tools.model;
/**
* Mojo descriptor definition.
*
* @version $Revision$ $Date$
*/
public class Mojo implements java.io.Serializable {
//--------------------------/
//- Class/Member Variables -/
//--------------------------/
/**
* Field goal
*/
private String goal;
/**
* Field phase
*/
private String phase;
/**
* Field aggregator
*/
private boolean aggregator = false;
/**
* Field requiresDependencyResolution
*/
private String requiresDependencyResolution;
/**
* Field requiresProject
*/
private boolean requiresProject = false;
/**
* Field requiresReports
*/
private boolean requiresReports = false;
/**
* Field requiresOnline
*/
private boolean requiresOnline = false;
/**
* Field inheritByDefault
*/
private boolean inheritByDefault = false;
/**
* Field requiresDirectInvocation
*/
private boolean requiresDirectInvocation = false;
/**
* Field execution
*/
private LifecycleExecution execution;
/**
* Field components
*/
private java.util.List components;
/**
* Field parameters
*/
private java.util.List parameters;
/**
* Field description
*/
private String description;
/**
* Field deprecation
*/
private String deprecation;
/**
* Field call
*/
private String call;
//-----------/
//- Methods -/
//-----------/
/**
* Method addComponent
*
* @param component
*/
public void addComponent(Component component)
{
getComponents().add( component );
} //-- void addComponent(Component)
/**
* Method addParameter
*
* @param parameter
*/
public void addParameter(Parameter parameter)
{
getParameters().add( parameter );
} //-- void addParameter(Parameter)
/**
* Get The target/method within the script to call when this
* mojo executes.
*/
public String getCall()
{
return this.call;
} //-- String getCall()
/**
* Method getComponents
*/
public java.util.List getComponents()
{
if ( this.components == null )
{
this.components = new java.util.ArrayList();
}
return this.components;
} //-- java.util.List getComponents()
/**
* Get A deprecation message for this mojo parameter.
*/
public String getDeprecation()
{
return this.deprecation;
} //-- String getDeprecation()
/**
* Get The description for this parameter.
*/
public String getDescription()
{
return this.description;
} //-- String getDescription()
/**
* Get Information about a sub-execution of the Maven lifecycle
* which should be processed.
*/
public LifecycleExecution getExecution()
{
return this.execution;
} //-- LifecycleExecution getExecution()
/**
* Get The name of the goal used to invoke this mojo.
*/
public String getGoal()
{
return this.goal;
} //-- String getGoal()
/**
* Method getParameters
*/
public java.util.List getParameters()
{
if ( this.parameters == null )
{
this.parameters = new java.util.ArrayList();
}
return this.parameters;
} //-- java.util.List getParameters()
/**
* Get The phase to which this mojo should be bound by default.
*/
public String getPhase()
{
return this.phase;
} //-- String getPhase()
/**
* Get The scope of dependencies that this mojo requires to
* have resolved.
*/
public String getRequiresDependencyResolution()
{
return this.requiresDependencyResolution;
} //-- String getRequiresDependencyResolution()
/**
* Get Whether this mojo operates as an aggregator when the
* reactor is run. That is, only runs once.
*/
public boolean isAggregator()
{
return this.aggregator;
} //-- boolean isAggregator()
/**
* Get Whether this mojo's configuration should propagate down
* the POM inheritance chain by default.
*/
public boolean isInheritByDefault()
{
return this.inheritByDefault;
} //-- boolean isInheritByDefault()
/**
* Get If true, this mojo can only be directly invoked (eg.
* specified directly on the command line).
*/
public boolean isRequiresDirectInvocation()
{
return this.requiresDirectInvocation;
} //-- boolean isRequiresDirectInvocation()
/**
* Get Whether this mojo requires online mode to operate
* normally.
*/
public boolean isRequiresOnline()
{
return this.requiresOnline;
} //-- boolean isRequiresOnline()
/**
* Get Whether this mojo requires a project instance in order
* to execute.
*/
public boolean isRequiresProject()
{
return this.requiresProject;
} //-- boolean isRequiresProject()
/**
* Get Whether this mojo requires a reports section in the POM.
*/
public boolean isRequiresReports()
{
return this.requiresReports;
} //-- boolean isRequiresReports()
/**
* Method removeComponent
*
* @param component
*/
public void removeComponent(Component component)
{
getComponents().remove( component );
} //-- void removeComponent(Component)
/**
* Method removeParameter
*
* @param parameter
*/
public void removeParameter(Parameter parameter)
{
getParameters().remove( parameter );
} //-- void removeParameter(Parameter)
/**
* Set Whether this mojo operates as an aggregator when the
* reactor is run. That is, only runs once.
*
* @param aggregator
*/
public void setAggregator(boolean aggregator)
{
this.aggregator = aggregator;
} //-- void setAggregator(boolean)
/**
* Set The target/method within the script to call when this
* mojo executes.
*
* @param call
*/
public void setCall(String call)
{
this.call = call;
} //-- void setCall(String)
/**
* Set List of plexus components required by this mojo.
*
* @param components
*/
public void setComponents(java.util.List components)
{
this.components = components;
} //-- void setComponents(java.util.List)
/**
* Set A deprecation message for this mojo parameter.
*
* @param deprecation
*/
public void setDeprecation(String deprecation)
{
this.deprecation = deprecation;
} //-- void setDeprecation(String)
/**
* Set The description for this parameter.
*
* @param description
*/
public void setDescription(String description)
{
this.description = description;
} //-- void setDescription(String)
/**
* Set Information about a sub-execution of the Maven lifecycle
* which should be processed.
*
* @param execution
*/
public void setExecution(LifecycleExecution execution)
{
this.execution = execution;
} //-- void setExecution(LifecycleExecution)
/**
* Set The name of the goal used to invoke this mojo.
*
* @param goal
*/
public void setGoal(String goal)
{
this.goal = goal;
} //-- void setGoal(String)
/**
* Set Whether this mojo's configuration should propagate down
* the POM inheritance chain by default.
*
* @param inheritByDefault
*/
public void setInheritByDefault(boolean inheritByDefault)
{
this.inheritByDefault = inheritByDefault;
} //-- void setInheritByDefault(boolean)
/**
* Set List of parameters used by this mojo.
*
* @param parameters
*/
public void setParameters(java.util.List parameters)
{
this.parameters = parameters;
} //-- void setParameters(java.util.List)
/**
* Set The phase to which this mojo should be bound by default.
*
* @param phase
*/
public void setPhase(String phase)
{
this.phase = phase;
} //-- void setPhase(String)
/**
* Set The scope of dependencies that this mojo requires to
* have resolved.
*
* @param requiresDependencyResolution
*/
public void setRequiresDependencyResolution(String requiresDependencyResolution)
{
this.requiresDependencyResolution = requiresDependencyResolution;
} //-- void setRequiresDependencyResolution(String)
/**
* Set If true, this mojo can only be directly invoked (eg.
* specified directly on the command line).
*
* @param requiresDirectInvocation
*/
public void setRequiresDirectInvocation(boolean requiresDirectInvocation)
{
this.requiresDirectInvocation = requiresDirectInvocation;
} //-- void setRequiresDirectInvocation(boolean)
/**
* Set Whether this mojo requires online mode to operate
* normally.
*
* @param requiresOnline
*/
public void setRequiresOnline(boolean requiresOnline)
{
this.requiresOnline = requiresOnline;
} //-- void setRequiresOnline(boolean)
/**
* Set Whether this mojo requires a project instance in order
* to execute.
*
* @param requiresProject
*/
public void setRequiresProject(boolean requiresProject)
{
this.requiresProject = requiresProject;
} //-- void setRequiresProject(boolean)
/**
* Set Whether this mojo requires a reports section in the POM.
*
* @param requiresReports
*/
public void setRequiresReports(boolean requiresReports)
{
this.requiresReports = requiresReports;
} //-- void setRequiresReports(boolean)
private String modelEncoding = "UTF-8";
public void setModelEncoding( String modelEncoding )
{
this.modelEncoding = modelEncoding;
}
public String getModelEncoding()
{
return modelEncoding;
}}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy