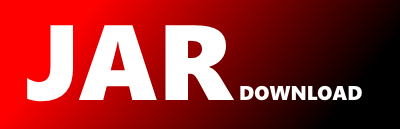
org.apache.maven.plugin.tools.model.Parameter Maven / Gradle / Ivy
/*
* $Id$
*/
package org.apache.maven.plugin.tools.model;
/**
*
* A parameter used by a mojo, and configurable from the
* command line or POM configuration sections.
*
*
* @version $Revision$ $Date$
*/
public class Parameter implements java.io.Serializable {
//--------------------------/
//- Class/Member Variables -/
//--------------------------/
/**
* Field name
*/
private String name;
/**
* Field alias
*/
private String alias;
/**
* Field property
*/
private String property;
/**
* Field required
*/
private boolean required = false;
/**
* Field readonly
*/
private boolean readonly = false;
/**
* Field expression
*/
private String expression;
/**
* Field defaultValue
*/
private String defaultValue;
/**
* Field type
*/
private String type;
/**
* Field description
*/
private String description;
/**
* Field deprecation
*/
private String deprecation;
//-----------/
//- Methods -/
//-----------/
/**
* Get An alternate name for the parameter.
*/
public String getAlias()
{
return this.alias;
} //-- String getAlias()
/**
* Get
* An expression in the form ${instance.property}
* for extracting a value for this parameter, especially from
* a runtime instance within the build system. (eg.
* ${project.build.directory} references
* project.getBuild().getDirectory())
*
*/
public String getDefaultValue()
{
return this.defaultValue;
} //-- String getDefaultValue()
/**
* Get A deprecation message for this mojo parameter.
*/
public String getDeprecation()
{
return this.deprecation;
} //-- String getDeprecation()
/**
* Get The description for this parameter.
*/
public String getDescription()
{
return this.description;
} //-- String getDescription()
/**
* Get
* The command-line reference to this parameter.
*
*/
public String getExpression()
{
return this.expression;
} //-- String getExpression()
/**
* Get The parameter name
*/
public String getName()
{
return this.name;
} //-- String getName()
/**
* Get The JavaBeans property name to use to configure the mojo
* with this parameter.
*/
public String getProperty()
{
return this.property;
} //-- String getProperty()
/**
* Get
* The java type for this parameter.
*
*/
public String getType()
{
return this.type;
} //-- String getType()
/**
* Get
* Whether this parameter can be directly edited.
* If false, this param is either derived from another POM
* element, or refers to a runtime instance of the build
* system.
*
*/
public boolean isReadonly()
{
return this.readonly;
} //-- boolean isReadonly()
/**
* Get Whether this parameter is required.
*/
public boolean isRequired()
{
return this.required;
} //-- boolean isRequired()
/**
* Set An alternate name for the parameter.
*
* @param alias
*/
public void setAlias(String alias)
{
this.alias = alias;
} //-- void setAlias(String)
/**
* Set
* An expression in the form ${instance.property}
* for extracting a value for this parameter, especially from
* a runtime instance within the build system. (eg.
* ${project.build.directory} references
* project.getBuild().getDirectory())
*
*
* @param defaultValue
*/
public void setDefaultValue(String defaultValue)
{
this.defaultValue = defaultValue;
} //-- void setDefaultValue(String)
/**
* Set A deprecation message for this mojo parameter.
*
* @param deprecation
*/
public void setDeprecation(String deprecation)
{
this.deprecation = deprecation;
} //-- void setDeprecation(String)
/**
* Set The description for this parameter.
*
* @param description
*/
public void setDescription(String description)
{
this.description = description;
} //-- void setDescription(String)
/**
* Set
* The command-line reference to this parameter.
*
*
* @param expression
*/
public void setExpression(String expression)
{
this.expression = expression;
} //-- void setExpression(String)
/**
* Set The parameter name
*
* @param name
*/
public void setName(String name)
{
this.name = name;
} //-- void setName(String)
/**
* Set The JavaBeans property name to use to configure the mojo
* with this parameter.
*
* @param property
*/
public void setProperty(String property)
{
this.property = property;
} //-- void setProperty(String)
/**
* Set
* Whether this parameter can be directly edited.
* If false, this param is either derived from another POM
* element, or refers to a runtime instance of the build
* system.
*
*
* @param readonly
*/
public void setReadonly(boolean readonly)
{
this.readonly = readonly;
} //-- void setReadonly(boolean)
/**
* Set Whether this parameter is required.
*
* @param required
*/
public void setRequired(boolean required)
{
this.required = required;
} //-- void setRequired(boolean)
/**
* Set
* The java type for this parameter.
*
*
* @param type
*/
public void setType(String type)
{
this.type = type;
} //-- void setType(String)
private String modelEncoding = "UTF-8";
public void setModelEncoding( String modelEncoding )
{
this.modelEncoding = modelEncoding;
}
public String getModelEncoding()
{
return modelEncoding;
}}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy