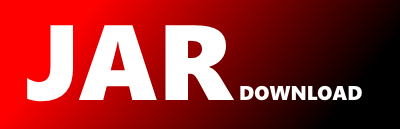
org.apache.maven.profiles.Profile Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of maven-project Show documentation
Show all versions of maven-project Show documentation
This library is used to not only read Maven project object model files, but to assemble inheritence
and to retrieve remote models as required.
The newest version!
/*
* $Id$
*/
package org.apache.maven.profiles;
//---------------------------------/
//- Imported classes and packages -/
//---------------------------------/
import java.util.Date;
/**
*
* Modifications to the build process which is keyed on
* some
* sort of environmental parameter.
*
*
* @version $Revision$ $Date$
*/
public class Profile implements java.io.Serializable {
//--------------------------/
//- Class/Member Variables -/
//--------------------------/
/**
* The ID of this build profile, for activation
* purposes.
*/
private String id = "default";
/**
* The conditional logic which will automatically
* trigger the inclusion of this profile.
*/
private Activation activation;
/**
* Field properties.
*/
private java.util.Properties properties;
/**
* Field repositories.
*/
private java.util.List repositories;
/**
* This may be removed or relocated in the near
* future. It is undecided whether plugins really
* need a remote
* repository set of their own.
*/
private java.util.List pluginRepositories;
//-----------/
//- Methods -/
//-----------/
/**
* Method addPluginRepository.
*
* @param repository
*/
public void addPluginRepository( Repository repository )
{
if ( !(repository instanceof Repository) )
{
throw new ClassCastException( "Profile.addPluginRepositories(repository) parameter must be instanceof " + Repository.class.getName() );
}
getPluginRepositories().add( repository );
} //-- void addPluginRepository( Repository )
/**
* Method addProperty.
*
* @param key
* @param value
*/
public void addProperty( String key, String value )
{
getProperties().put( key, value );
} //-- void addProperty( String, String )
/**
* Method addRepository.
*
* @param repository
*/
public void addRepository( Repository repository )
{
if ( !(repository instanceof Repository) )
{
throw new ClassCastException( "Profile.addRepositories(repository) parameter must be instanceof " + Repository.class.getName() );
}
getRepositories().add( repository );
} //-- void addRepository( Repository )
/**
* Get the conditional logic which will automatically
* trigger the inclusion of this profile.
*
* @return Activation
*/
public Activation getActivation()
{
return this.activation;
} //-- Activation getActivation()
/**
* Get the ID of this build profile, for activation
* purposes.
*
* @return String
*/
public String getId()
{
return this.id;
} //-- String getId()
/**
* Method getPluginRepositories.
*
* @return java.util.List
*/
public java.util.List getPluginRepositories()
{
if ( this.pluginRepositories == null )
{
this.pluginRepositories = new java.util.ArrayList();
}
return this.pluginRepositories;
} //-- java.util.List getPluginRepositories()
/**
* Method getProperties.
*
* @return java.util.Properties
*/
public java.util.Properties getProperties()
{
if ( this.properties == null )
{
this.properties = new java.util.Properties();
}
return this.properties;
} //-- java.util.Properties getProperties()
/**
* Method getRepositories.
*
* @return java.util.List
*/
public java.util.List getRepositories()
{
if ( this.repositories == null )
{
this.repositories = new java.util.ArrayList();
}
return this.repositories;
} //-- java.util.List getRepositories()
/**
* Method removePluginRepository.
*
* @param repository
*/
public void removePluginRepository( Repository repository )
{
if ( !(repository instanceof Repository) )
{
throw new ClassCastException( "Profile.removePluginRepositories(repository) parameter must be instanceof " + Repository.class.getName() );
}
getPluginRepositories().remove( repository );
} //-- void removePluginRepository( Repository )
/**
* Method removeRepository.
*
* @param repository
*/
public void removeRepository( Repository repository )
{
if ( !(repository instanceof Repository) )
{
throw new ClassCastException( "Profile.removeRepositories(repository) parameter must be instanceof " + Repository.class.getName() );
}
getRepositories().remove( repository );
} //-- void removeRepository( Repository )
/**
* Set the conditional logic which will automatically
* trigger the inclusion of this profile.
*
* @param activation
*/
public void setActivation( Activation activation )
{
this.activation = activation;
} //-- void setActivation( Activation )
/**
* Set the ID of this build profile, for activation
* purposes.
*
* @param id
*/
public void setId( String id )
{
this.id = id;
} //-- void setId( String )
/**
* Set
* The lists of the remote repositories for
* discovering plugins
* .
*
* @param pluginRepositories
*/
public void setPluginRepositories( java.util.List pluginRepositories )
{
this.pluginRepositories = pluginRepositories;
} //-- void setPluginRepositories( java.util.List )
/**
* Set extended configuration specific to this profile goes
* here.
*
* @param properties
*/
public void setProperties( java.util.Properties properties )
{
this.properties = properties;
} //-- void setProperties( java.util.Properties )
/**
* Set the lists of the remote repositories
* .
*
* @param repositories
*/
public void setRepositories( java.util.List repositories )
{
this.repositories = repositories;
} //-- void setRepositories( java.util.List )
private String modelEncoding = "UTF-8";
/**
* Set an encoding used for reading/writing the model.
*
* @param modelEncoding the encoding used when reading/writing the model.
*/
public void setModelEncoding( String modelEncoding )
{
this.modelEncoding = modelEncoding;
}
/**
* @return the current encoding used when reading/writing this model.
*/
public String getModelEncoding()
{
return modelEncoding;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy