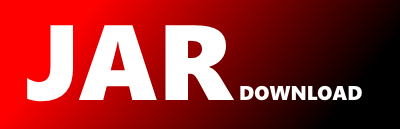
org.apache.maven.settings.Settings Maven / Gradle / Ivy
The newest version!
// =================== DO NOT EDIT THIS FILE ====================
// Generated by Modello Velocity from model-v3.vm
// template, any modifications will be overwritten.
// ==============================================================
package org.apache.maven.settings;
import java.io.Serializable;
import java.util.AbstractList;
import java.util.ArrayList;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Set;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import org.apache.maven.api.annotations.Generated;
import org.apache.maven.api.annotations.Nonnull;
@Generated
public class Settings
extends TrackableBase
implements Serializable, Cloneable
{
public Settings() {
this(org.apache.maven.api.settings.Settings.newInstance());
}
public Settings(org.apache.maven.api.settings.Settings delegate) {
this(delegate, null);
}
public Settings(org.apache.maven.api.settings.Settings delegate, BaseObject parent) {
super(delegate, parent);
}
public Settings clone(){
return new Settings(getDelegate());
}
@Override
public org.apache.maven.api.settings.Settings getDelegate() {
return (org.apache.maven.api.settings.Settings) super.getDelegate();
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || !(o instanceof Settings)) {
return false;
}
Settings that = (Settings) o;
return Objects.equals(this.delegate, that.delegate);
}
@Override
public int hashCode() {
return getDelegate().hashCode();
}
public String getModelEncoding() {
return getDelegate().getModelEncoding();
}
public String getLocalRepository() {
return getDelegate().getLocalRepository();
}
public void setLocalRepository(String localRepository) {
if (!Objects.equals(localRepository, getLocalRepository())) {
update(getDelegate().withLocalRepository(localRepository));
}
}
public boolean isInteractiveMode() {
return getDelegate().isInteractiveMode();
}
public void setInteractiveMode(boolean interactiveMode) {
if (!Objects.equals(interactiveMode, isInteractiveMode())) {
update(getDelegate().withInteractiveMode(interactiveMode));
}
}
public boolean isUsePluginRegistry() {
return getDelegate().isUsePluginRegistry();
}
public void setUsePluginRegistry(boolean usePluginRegistry) {
if (!Objects.equals(usePluginRegistry, isUsePluginRegistry())) {
update(getDelegate().withUsePluginRegistry(usePluginRegistry));
}
}
public boolean isOffline() {
return getDelegate().isOffline();
}
public void setOffline(boolean offline) {
if (!Objects.equals(offline, isOffline())) {
update(getDelegate().withOffline(offline));
}
}
@Nonnull
public List getProxies() {
return new WrapperList(
() -> getDelegate().getProxies(), l -> update(getDelegate().withProxies(l)),
d -> new Proxy(d, this), Proxy::getDelegate);
}
public void setProxies(List proxies) {
if (proxies == null) {
proxies = Collections.emptyList();
}
if (!Objects.equals(proxies, getProxies())) {
update(getDelegate().withProxies(
proxies.stream().map(c -> c.getDelegate()).collect(Collectors.toList())));
proxies.forEach(e -> e.childrenTracking = this::replace);
}
}
public void addProxy(Proxy proxy) {
update(getDelegate().withProxies(
Stream.concat(getDelegate().getProxies().stream(), Stream.of(proxy.getDelegate()))
.collect(Collectors.toList())));
proxy.childrenTracking = this::replace;
}
public void removeProxy(Proxy proxy) {
update(getDelegate().withProxies(
getDelegate().getProxies().stream()
.filter(e -> !Objects.equals(e, proxy))
.collect(Collectors.toList())));
proxy.childrenTracking = null;
}
@Nonnull
public List getServers() {
return new WrapperList(
() -> getDelegate().getServers(), l -> update(getDelegate().withServers(l)),
d -> new Server(d, this), Server::getDelegate);
}
public void setServers(List servers) {
if (servers == null) {
servers = Collections.emptyList();
}
if (!Objects.equals(servers, getServers())) {
update(getDelegate().withServers(
servers.stream().map(c -> c.getDelegate()).collect(Collectors.toList())));
servers.forEach(e -> e.childrenTracking = this::replace);
}
}
public void addServer(Server server) {
update(getDelegate().withServers(
Stream.concat(getDelegate().getServers().stream(), Stream.of(server.getDelegate()))
.collect(Collectors.toList())));
server.childrenTracking = this::replace;
}
public void removeServer(Server server) {
update(getDelegate().withServers(
getDelegate().getServers().stream()
.filter(e -> !Objects.equals(e, server))
.collect(Collectors.toList())));
server.childrenTracking = null;
}
@Nonnull
public List getMirrors() {
return new WrapperList(
() -> getDelegate().getMirrors(), l -> update(getDelegate().withMirrors(l)),
d -> new Mirror(d, this), Mirror::getDelegate);
}
public void setMirrors(List mirrors) {
if (mirrors == null) {
mirrors = Collections.emptyList();
}
if (!Objects.equals(mirrors, getMirrors())) {
update(getDelegate().withMirrors(
mirrors.stream().map(c -> c.getDelegate()).collect(Collectors.toList())));
mirrors.forEach(e -> e.childrenTracking = this::replace);
}
}
public void addMirror(Mirror mirror) {
update(getDelegate().withMirrors(
Stream.concat(getDelegate().getMirrors().stream(), Stream.of(mirror.getDelegate()))
.collect(Collectors.toList())));
mirror.childrenTracking = this::replace;
}
public void removeMirror(Mirror mirror) {
update(getDelegate().withMirrors(
getDelegate().getMirrors().stream()
.filter(e -> !Objects.equals(e, mirror))
.collect(Collectors.toList())));
mirror.childrenTracking = null;
}
@Nonnull
public List getRepositories() {
return new WrapperList(
() -> getDelegate().getRepositories(), l -> update(getDelegate().withRepositories(l)),
d -> new Repository(d, this), Repository::getDelegate);
}
public void setRepositories(List repositories) {
if (repositories == null) {
repositories = Collections.emptyList();
}
if (!Objects.equals(repositories, getRepositories())) {
update(getDelegate().withRepositories(
repositories.stream().map(c -> c.getDelegate()).collect(Collectors.toList())));
repositories.forEach(e -> e.childrenTracking = this::replace);
}
}
public void addRepository(Repository repository) {
update(getDelegate().withRepositories(
Stream.concat(getDelegate().getRepositories().stream(), Stream.of(repository.getDelegate()))
.collect(Collectors.toList())));
repository.childrenTracking = this::replace;
}
public void removeRepository(Repository repository) {
update(getDelegate().withRepositories(
getDelegate().getRepositories().stream()
.filter(e -> !Objects.equals(e, repository))
.collect(Collectors.toList())));
repository.childrenTracking = null;
}
@Nonnull
public List getPluginRepositories() {
return new WrapperList(
() -> getDelegate().getPluginRepositories(), l -> update(getDelegate().withPluginRepositories(l)),
d -> new Repository(d, this), Repository::getDelegate);
}
public void setPluginRepositories(List pluginRepositories) {
if (pluginRepositories == null) {
pluginRepositories = Collections.emptyList();
}
if (!Objects.equals(pluginRepositories, getPluginRepositories())) {
update(getDelegate().withPluginRepositories(
pluginRepositories.stream().map(c -> c.getDelegate()).collect(Collectors.toList())));
pluginRepositories.forEach(e -> e.childrenTracking = this::replace);
}
}
public void addPluginRepository(Repository pluginRepository) {
update(getDelegate().withPluginRepositories(
Stream.concat(getDelegate().getPluginRepositories().stream(), Stream.of(pluginRepository.getDelegate()))
.collect(Collectors.toList())));
pluginRepository.childrenTracking = this::replace;
}
public void removePluginRepository(Repository pluginRepository) {
update(getDelegate().withPluginRepositories(
getDelegate().getPluginRepositories().stream()
.filter(e -> !Objects.equals(e, pluginRepository))
.collect(Collectors.toList())));
pluginRepository.childrenTracking = null;
}
@Nonnull
public List getProfiles() {
return new WrapperList(
() -> getDelegate().getProfiles(), l -> update(getDelegate().withProfiles(l)),
d -> new Profile(d, this), Profile::getDelegate);
}
public void setProfiles(List profiles) {
if (profiles == null) {
profiles = Collections.emptyList();
}
if (!Objects.equals(profiles, getProfiles())) {
update(getDelegate().withProfiles(
profiles.stream().map(c -> c.getDelegate()).collect(Collectors.toList())));
profiles.forEach(e -> e.childrenTracking = this::replace);
}
}
public void addProfile(Profile profile) {
update(getDelegate().withProfiles(
Stream.concat(getDelegate().getProfiles().stream(), Stream.of(profile.getDelegate()))
.collect(Collectors.toList())));
profile.childrenTracking = this::replace;
}
public void removeProfile(Profile profile) {
update(getDelegate().withProfiles(
getDelegate().getProfiles().stream()
.filter(e -> !Objects.equals(e, profile))
.collect(Collectors.toList())));
profile.childrenTracking = null;
}
@Nonnull
public List getActiveProfiles() {
return new WrapperList(() -> getDelegate().getActiveProfiles(), this::setActiveProfiles, s -> s, s -> s);
}
public void setActiveProfiles(List activeProfiles) {
if (!Objects.equals(activeProfiles, getActiveProfiles())) {
update(getDelegate().withActiveProfiles(activeProfiles));
}
}
public void addActiveProfile(String activeProfile) {
update(getDelegate().withActiveProfiles(
Stream.concat(getDelegate().getActiveProfiles().stream(), Stream.of(activeProfile))
.collect(Collectors.toList())));
}
public void removeActiveProfile(String activeProfile) {
update(getDelegate().withActiveProfiles(
getDelegate().getActiveProfiles().stream()
.filter(e -> !Objects.equals(e, activeProfile))
.collect(Collectors.toList())));
}
@Nonnull
public List getPluginGroups() {
return new WrapperList(() -> getDelegate().getPluginGroups(), this::setPluginGroups, s -> s, s -> s);
}
public void setPluginGroups(List pluginGroups) {
if (!Objects.equals(pluginGroups, getPluginGroups())) {
update(getDelegate().withPluginGroups(pluginGroups));
}
}
public void addPluginGroup(String pluginGroup) {
update(getDelegate().withPluginGroups(
Stream.concat(getDelegate().getPluginGroups().stream(), Stream.of(pluginGroup))
.collect(Collectors.toList())));
}
public void removePluginGroup(String pluginGroup) {
update(getDelegate().withPluginGroups(
getDelegate().getPluginGroups().stream()
.filter(e -> !Objects.equals(e, pluginGroup))
.collect(Collectors.toList())));
}
protected boolean replace(Object oldDelegate, Object newDelegate) {
if (super.replace(oldDelegate, newDelegate)) {
return true;
}
if (getDelegate().getProxies().contains(oldDelegate)) {
List list = new ArrayList<>(getDelegate().getProxies());
list.replaceAll(d -> d == oldDelegate ? (org.apache.maven.api.settings.Proxy) newDelegate : d);
update(getDelegate().withProxies(list));
return true;
}
if (getDelegate().getServers().contains(oldDelegate)) {
List list = new ArrayList<>(getDelegate().getServers());
list.replaceAll(d -> d == oldDelegate ? (org.apache.maven.api.settings.Server) newDelegate : d);
update(getDelegate().withServers(list));
return true;
}
if (getDelegate().getMirrors().contains(oldDelegate)) {
List list = new ArrayList<>(getDelegate().getMirrors());
list.replaceAll(d -> d == oldDelegate ? (org.apache.maven.api.settings.Mirror) newDelegate : d);
update(getDelegate().withMirrors(list));
return true;
}
if (getDelegate().getRepositories().contains(oldDelegate)) {
List list = new ArrayList<>(getDelegate().getRepositories());
list.replaceAll(d -> d == oldDelegate ? (org.apache.maven.api.settings.Repository) newDelegate : d);
update(getDelegate().withRepositories(list));
return true;
}
if (getDelegate().getPluginRepositories().contains(oldDelegate)) {
List list = new ArrayList<>(getDelegate().getPluginRepositories());
list.replaceAll(d -> d == oldDelegate ? (org.apache.maven.api.settings.Repository) newDelegate : d);
update(getDelegate().withPluginRepositories(list));
return true;
}
if (getDelegate().getProfiles().contains(oldDelegate)) {
List list = new ArrayList<>(getDelegate().getProfiles());
list.replaceAll(d -> d == oldDelegate ? (org.apache.maven.api.settings.Profile) newDelegate : d);
update(getDelegate().withProfiles(list));
return true;
}
return false;
}
public static List settingsToApiV4(List list) {
return list != null ? new WrapperList<>(list, Settings::getDelegate, Settings::new) : null;
}
public static List settingsToApiV3(List list) {
return list != null ? new WrapperList<>(list, Settings::new, Settings::getDelegate) : null;
}
public Boolean getInteractiveMode() {
return Boolean.valueOf(isInteractiveMode());
}
private Proxy activeProxy;
/**
* Reset the {@code activeProxy} field to {@code null}.
*/
public void flushActiveProxy() {
this.activeProxy = null;
}
/**
* @return the first active proxy
*/
public synchronized Proxy getActiveProxy() {
if (activeProxy == null) {
java.util.List proxies = getProxies();
if (proxies != null && !proxies.isEmpty()) {
for (Proxy proxy : proxies) {
if (proxy.isActive()) {
activeProxy = proxy;
break;
}
}
}
}
return activeProxy;
}
public Server getServer(String serverId) {
Server match = null;
java.util.List servers = getServers();
if (servers != null && serverId != null) {
for (Server server : servers) {
if (serverId.equals(server.getId())) {
match = server;
break;
}
}
}
return match;
}
@Deprecated
public Mirror getMirrorOf(String repositoryId) {
Mirror match = null;
java.util.List mirrors = getMirrors();
if (mirrors != null && repositoryId != null) {
for (Mirror mirror : mirrors) {
if (repositoryId.equals(mirror.getMirrorOf())) {
match = mirror;
break;
}
}
}
return match;
}
private java.util.Map profileMap;
/**
* Reset the {@code profileMap} field to {@code null}
*/
public void flushProfileMap() {
this.profileMap = null;
}
/**
* @return a Map of profiles field keyed by {@link Profile#getId()}
*/
public java.util.Map getProfilesAsMap() {
if (profileMap == null) {
profileMap = new java.util.LinkedHashMap();
if (getProfiles() != null) {
for (Profile profile : getProfiles()) {
profileMap.put(profile.getId(), profile);
}
}
}
return profileMap;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy