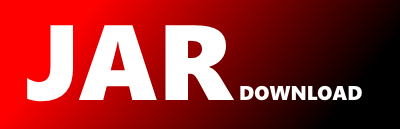
org.apache.mesos.v1.Protos Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mesos Show documentation
Show all versions of mesos Show documentation
The Apache Mesos Java API jar.
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: mesos/v1/mesos.proto
package org.apache.mesos.v1;
public final class Protos {
private Protos() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistryLite registry) {
}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
registerAllExtensions(
(com.google.protobuf.ExtensionRegistryLite) registry);
}
/**
*
**
* Status is used to indicate the state of the scheduler and executor
* driver after function calls.
*
*
* Protobuf enum {@code mesos.v1.Status}
*/
public enum Status
implements com.google.protobuf.ProtocolMessageEnum {
/**
* DRIVER_NOT_STARTED = 1;
*/
DRIVER_NOT_STARTED(1),
/**
* DRIVER_RUNNING = 2;
*/
DRIVER_RUNNING(2),
/**
* DRIVER_ABORTED = 3;
*/
DRIVER_ABORTED(3),
/**
* DRIVER_STOPPED = 4;
*/
DRIVER_STOPPED(4),
;
/**
* DRIVER_NOT_STARTED = 1;
*/
public static final int DRIVER_NOT_STARTED_VALUE = 1;
/**
* DRIVER_RUNNING = 2;
*/
public static final int DRIVER_RUNNING_VALUE = 2;
/**
* DRIVER_ABORTED = 3;
*/
public static final int DRIVER_ABORTED_VALUE = 3;
/**
* DRIVER_STOPPED = 4;
*/
public static final int DRIVER_STOPPED_VALUE = 4;
public final int getNumber() {
return value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static Status valueOf(int value) {
return forNumber(value);
}
public static Status forNumber(int value) {
switch (value) {
case 1: return DRIVER_NOT_STARTED;
case 2: return DRIVER_RUNNING;
case 3: return DRIVER_ABORTED;
case 4: return DRIVER_STOPPED;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
Status> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public Status findValueByNumber(int number) {
return Status.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return org.apache.mesos.v1.Protos.getDescriptor().getEnumTypes().get(0);
}
private static final Status[] VALUES = values();
public static Status valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int value;
private Status(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:mesos.v1.Status)
}
/**
*
**
* Describes possible task states. IMPORTANT: Mesos assumes tasks that
* enter terminal states (see below) imply the task is no longer
* running and thus clean up any thing associated with the task
* (ultimately offering any resources being consumed by that task to
* another task).
*
*
* Protobuf enum {@code mesos.v1.TaskState}
*/
public enum TaskState
implements com.google.protobuf.ProtocolMessageEnum {
/**
*
* Initial state. Framework status updates should not use.
*
*
* TASK_STAGING = 6;
*/
TASK_STAGING(6),
/**
*
* The task is being launched by the executor.
*
*
* TASK_STARTING = 0;
*/
TASK_STARTING(0),
/**
* TASK_RUNNING = 1;
*/
TASK_RUNNING(1),
/**
*
* NOTE: This should only be sent when the framework has
* the TASK_KILLING_STATE capability.
*
*
* TASK_KILLING = 8;
*/
TASK_KILLING(8),
/**
*
* The task finished successfully on its own without external interference.
*
*
* TASK_FINISHED = 2;
*/
TASK_FINISHED(2),
/**
*
* TERMINAL: The task failed to finish successfully.
*
*
* TASK_FAILED = 3;
*/
TASK_FAILED(3),
/**
*
* TERMINAL: The task was killed by the executor.
*
*
* TASK_KILLED = 4;
*/
TASK_KILLED(4),
/**
*
* TERMINAL: The task description contains an error.
*
*
* TASK_ERROR = 7;
*/
TASK_ERROR(7),
/**
*
* In Mesos 1.3, this will only be sent when the framework does NOT
* opt-in to the PARTITION_AWARE capability.
* NOTE: This state is not always terminal. For example, tasks might
* transition from TASK_LOST to TASK_RUNNING or other states when a
* partitioned agent reregisters.
*
*
* TASK_LOST = 5;
*/
TASK_LOST(5),
/**
*
* The task failed to launch because of a transient error. The
* task's executor never started running. Unlike TASK_ERROR, the
* task description is valid -- attempting to launch the task again
* may be successful.
*
*
* TASK_DROPPED = 9;
*/
TASK_DROPPED(9),
/**
*
* The task was running on an agent that has lost contact with the
* master, typically due to a network failure or partition. The task
* may or may not still be running.
*
*
* TASK_UNREACHABLE = 10;
*/
TASK_UNREACHABLE(10),
/**
*
* The task is no longer running. This can occur if the agent has
* been terminated along with all of its tasks (e.g., the host that
* was running the agent was rebooted). It might also occur if the
* task was terminated due to an agent or containerizer error, or if
* the task was preempted by the QoS controller in an
* oversubscription scenario.
*
*
* TASK_GONE = 11;
*/
TASK_GONE(11),
/**
*
* The task was running on an agent that the master cannot contact;
* the operator has asserted that the agent has been shutdown, but
* this has not been directly confirmed by the master. If the
* operator is correct, the task is not running and this is a
* terminal state; if the operator is mistaken, the task may still
* be running and might return to RUNNING in the future.
*
*
* TASK_GONE_BY_OPERATOR = 12;
*/
TASK_GONE_BY_OPERATOR(12),
/**
*
* The master has no knowledge of the task. This is typically
* because either (a) the master never had knowledge of the task, or
* (b) the master forgot about the task because it garbage collected
* its metadata about the task. The task may or may not still be
* running.
*
*
* TASK_UNKNOWN = 13;
*/
TASK_UNKNOWN(13),
;
/**
*
* Initial state. Framework status updates should not use.
*
*
* TASK_STAGING = 6;
*/
public static final int TASK_STAGING_VALUE = 6;
/**
*
* The task is being launched by the executor.
*
*
* TASK_STARTING = 0;
*/
public static final int TASK_STARTING_VALUE = 0;
/**
* TASK_RUNNING = 1;
*/
public static final int TASK_RUNNING_VALUE = 1;
/**
*
* NOTE: This should only be sent when the framework has
* the TASK_KILLING_STATE capability.
*
*
* TASK_KILLING = 8;
*/
public static final int TASK_KILLING_VALUE = 8;
/**
*
* The task finished successfully on its own without external interference.
*
*
* TASK_FINISHED = 2;
*/
public static final int TASK_FINISHED_VALUE = 2;
/**
*
* TERMINAL: The task failed to finish successfully.
*
*
* TASK_FAILED = 3;
*/
public static final int TASK_FAILED_VALUE = 3;
/**
*
* TERMINAL: The task was killed by the executor.
*
*
* TASK_KILLED = 4;
*/
public static final int TASK_KILLED_VALUE = 4;
/**
*
* TERMINAL: The task description contains an error.
*
*
* TASK_ERROR = 7;
*/
public static final int TASK_ERROR_VALUE = 7;
/**
*
* In Mesos 1.3, this will only be sent when the framework does NOT
* opt-in to the PARTITION_AWARE capability.
* NOTE: This state is not always terminal. For example, tasks might
* transition from TASK_LOST to TASK_RUNNING or other states when a
* partitioned agent reregisters.
*
*
* TASK_LOST = 5;
*/
public static final int TASK_LOST_VALUE = 5;
/**
*
* The task failed to launch because of a transient error. The
* task's executor never started running. Unlike TASK_ERROR, the
* task description is valid -- attempting to launch the task again
* may be successful.
*
*
* TASK_DROPPED = 9;
*/
public static final int TASK_DROPPED_VALUE = 9;
/**
*
* The task was running on an agent that has lost contact with the
* master, typically due to a network failure or partition. The task
* may or may not still be running.
*
*
* TASK_UNREACHABLE = 10;
*/
public static final int TASK_UNREACHABLE_VALUE = 10;
/**
*
* The task is no longer running. This can occur if the agent has
* been terminated along with all of its tasks (e.g., the host that
* was running the agent was rebooted). It might also occur if the
* task was terminated due to an agent or containerizer error, or if
* the task was preempted by the QoS controller in an
* oversubscription scenario.
*
*
* TASK_GONE = 11;
*/
public static final int TASK_GONE_VALUE = 11;
/**
*
* The task was running on an agent that the master cannot contact;
* the operator has asserted that the agent has been shutdown, but
* this has not been directly confirmed by the master. If the
* operator is correct, the task is not running and this is a
* terminal state; if the operator is mistaken, the task may still
* be running and might return to RUNNING in the future.
*
*
* TASK_GONE_BY_OPERATOR = 12;
*/
public static final int TASK_GONE_BY_OPERATOR_VALUE = 12;
/**
*
* The master has no knowledge of the task. This is typically
* because either (a) the master never had knowledge of the task, or
* (b) the master forgot about the task because it garbage collected
* its metadata about the task. The task may or may not still be
* running.
*
*
* TASK_UNKNOWN = 13;
*/
public static final int TASK_UNKNOWN_VALUE = 13;
public final int getNumber() {
return value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static TaskState valueOf(int value) {
return forNumber(value);
}
public static TaskState forNumber(int value) {
switch (value) {
case 6: return TASK_STAGING;
case 0: return TASK_STARTING;
case 1: return TASK_RUNNING;
case 8: return TASK_KILLING;
case 2: return TASK_FINISHED;
case 3: return TASK_FAILED;
case 4: return TASK_KILLED;
case 7: return TASK_ERROR;
case 5: return TASK_LOST;
case 9: return TASK_DROPPED;
case 10: return TASK_UNREACHABLE;
case 11: return TASK_GONE;
case 12: return TASK_GONE_BY_OPERATOR;
case 13: return TASK_UNKNOWN;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
TaskState> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public TaskState findValueByNumber(int number) {
return TaskState.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return org.apache.mesos.v1.Protos.getDescriptor().getEnumTypes().get(1);
}
private static final TaskState[] VALUES = values();
public static TaskState valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int value;
private TaskState(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:mesos.v1.TaskState)
}
/**
*
**
* Describes possible operation states.
*
*
* Protobuf enum {@code mesos.v1.OperationState}
*/
public enum OperationState
implements com.google.protobuf.ProtocolMessageEnum {
/**
*
* Default value if the enum is not set. See MESOS-4997.
*
*
* OPERATION_UNSUPPORTED = 0;
*/
OPERATION_UNSUPPORTED(0),
/**
*
* Initial state.
*
*
* OPERATION_PENDING = 1;
*/
OPERATION_PENDING(1),
/**
*
* TERMINAL: The operation was successfully applied.
*
*
* OPERATION_FINISHED = 2;
*/
OPERATION_FINISHED(2),
/**
*
* TERMINAL: The operation failed to apply.
*
*
* OPERATION_FAILED = 3;
*/
OPERATION_FAILED(3),
/**
*
* TERMINAL: The operation description contains an error.
*
*
* OPERATION_ERROR = 4;
*/
OPERATION_ERROR(4),
/**
*
* TERMINAL: The operation was dropped due to a transient error.
*
*
* OPERATION_DROPPED = 5;
*/
OPERATION_DROPPED(5),
/**
*
* The operation affects an agent that has lost contact with the master,
* typically due to a network failure or partition. The operation may or may
* not still be pending.
*
*
* OPERATION_UNREACHABLE = 6;
*/
OPERATION_UNREACHABLE(6),
/**
*
* The operation affected an agent that the master cannot contact;
* the operator has asserted that the agent has been shutdown, but this has
* not been directly confirmed by the master.
* If the operator is correct, the operation is not pending and this is a
* terminal state; if the operator is mistaken, the operation may still be
* pending and might return to a different state in the future.
*
*
* OPERATION_GONE_BY_OPERATOR = 7;
*/
OPERATION_GONE_BY_OPERATOR(7),
/**
*
* The operation affects an agent that the master recovered from its
* state, but that agent has not yet re-registered.
* The operation can transition to `OPERATION_UNREACHABLE` if the
* corresponding agent is marked as unreachable, and will transition to
* another status if the agent re-registers.
*
*
* OPERATION_RECOVERING = 8;
*/
OPERATION_RECOVERING(8),
/**
*
* The master has no knowledge of the operation. This is typically
* because either (a) the master never had knowledge of the operation, or
* (b) the master forgot about the operation because it garbage collected
* its metadata about the operation. The operation may or may not still be
* pending.
*
*
* OPERATION_UNKNOWN = 9;
*/
OPERATION_UNKNOWN(9),
;
/**
*
* Default value if the enum is not set. See MESOS-4997.
*
*
* OPERATION_UNSUPPORTED = 0;
*/
public static final int OPERATION_UNSUPPORTED_VALUE = 0;
/**
*
* Initial state.
*
*
* OPERATION_PENDING = 1;
*/
public static final int OPERATION_PENDING_VALUE = 1;
/**
*
* TERMINAL: The operation was successfully applied.
*
*
* OPERATION_FINISHED = 2;
*/
public static final int OPERATION_FINISHED_VALUE = 2;
/**
*
* TERMINAL: The operation failed to apply.
*
*
* OPERATION_FAILED = 3;
*/
public static final int OPERATION_FAILED_VALUE = 3;
/**
*
* TERMINAL: The operation description contains an error.
*
*
* OPERATION_ERROR = 4;
*/
public static final int OPERATION_ERROR_VALUE = 4;
/**
*
* TERMINAL: The operation was dropped due to a transient error.
*
*
* OPERATION_DROPPED = 5;
*/
public static final int OPERATION_DROPPED_VALUE = 5;
/**
*
* The operation affects an agent that has lost contact with the master,
* typically due to a network failure or partition. The operation may or may
* not still be pending.
*
*
* OPERATION_UNREACHABLE = 6;
*/
public static final int OPERATION_UNREACHABLE_VALUE = 6;
/**
*
* The operation affected an agent that the master cannot contact;
* the operator has asserted that the agent has been shutdown, but this has
* not been directly confirmed by the master.
* If the operator is correct, the operation is not pending and this is a
* terminal state; if the operator is mistaken, the operation may still be
* pending and might return to a different state in the future.
*
*
* OPERATION_GONE_BY_OPERATOR = 7;
*/
public static final int OPERATION_GONE_BY_OPERATOR_VALUE = 7;
/**
*
* The operation affects an agent that the master recovered from its
* state, but that agent has not yet re-registered.
* The operation can transition to `OPERATION_UNREACHABLE` if the
* corresponding agent is marked as unreachable, and will transition to
* another status if the agent re-registers.
*
*
* OPERATION_RECOVERING = 8;
*/
public static final int OPERATION_RECOVERING_VALUE = 8;
/**
*
* The master has no knowledge of the operation. This is typically
* because either (a) the master never had knowledge of the operation, or
* (b) the master forgot about the operation because it garbage collected
* its metadata about the operation. The operation may or may not still be
* pending.
*
*
* OPERATION_UNKNOWN = 9;
*/
public static final int OPERATION_UNKNOWN_VALUE = 9;
public final int getNumber() {
return value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static OperationState valueOf(int value) {
return forNumber(value);
}
public static OperationState forNumber(int value) {
switch (value) {
case 0: return OPERATION_UNSUPPORTED;
case 1: return OPERATION_PENDING;
case 2: return OPERATION_FINISHED;
case 3: return OPERATION_FAILED;
case 4: return OPERATION_ERROR;
case 5: return OPERATION_DROPPED;
case 6: return OPERATION_UNREACHABLE;
case 7: return OPERATION_GONE_BY_OPERATOR;
case 8: return OPERATION_RECOVERING;
case 9: return OPERATION_UNKNOWN;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
OperationState> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public OperationState findValueByNumber(int number) {
return OperationState.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return org.apache.mesos.v1.Protos.getDescriptor().getEnumTypes().get(2);
}
private static final OperationState[] VALUES = values();
public static OperationState valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int value;
private OperationState(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:mesos.v1.OperationState)
}
/**
* Protobuf enum {@code mesos.v1.DrainState}
*/
public enum DrainState
implements com.google.protobuf.ProtocolMessageEnum {
/**
* UNKNOWN = 0;
*/
UNKNOWN(0),
/**
*
* The agent is currently draining.
*
*
* DRAINING = 1;
*/
DRAINING(1),
/**
*
* The agent has been drained: all tasks have terminated, all terminal
* task status updates have been acknowledged by the frameworks, and all
* operations have finished and had their terminal updates acknowledged.
*
*
* DRAINED = 2;
*/
DRAINED(2),
;
/**
* UNKNOWN = 0;
*/
public static final int UNKNOWN_VALUE = 0;
/**
*
* The agent is currently draining.
*
*
* DRAINING = 1;
*/
public static final int DRAINING_VALUE = 1;
/**
*
* The agent has been drained: all tasks have terminated, all terminal
* task status updates have been acknowledged by the frameworks, and all
* operations have finished and had their terminal updates acknowledged.
*
*
* DRAINED = 2;
*/
public static final int DRAINED_VALUE = 2;
public final int getNumber() {
return value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static DrainState valueOf(int value) {
return forNumber(value);
}
public static DrainState forNumber(int value) {
switch (value) {
case 0: return UNKNOWN;
case 1: return DRAINING;
case 2: return DRAINED;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
DrainState> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public DrainState findValueByNumber(int number) {
return DrainState.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return org.apache.mesos.v1.Protos.getDescriptor().getEnumTypes().get(3);
}
private static final DrainState[] VALUES = values();
public static DrainState valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int value;
private DrainState(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:mesos.v1.DrainState)
}
public interface FrameworkIDOrBuilder extends
// @@protoc_insertion_point(interface_extends:mesos.v1.FrameworkID)
com.google.protobuf.MessageOrBuilder {
/**
* required string value = 1;
*/
boolean hasValue();
/**
* required string value = 1;
*/
java.lang.String getValue();
/**
* required string value = 1;
*/
com.google.protobuf.ByteString
getValueBytes();
}
/**
*
**
* A unique ID assigned to a framework. A framework can reuse this ID
* in order to do failover (see MesosSchedulerDriver).
*
*
* Protobuf type {@code mesos.v1.FrameworkID}
*/
public static final class FrameworkID extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:mesos.v1.FrameworkID)
FrameworkIDOrBuilder {
private static final long serialVersionUID = 0L;
// Use FrameworkID.newBuilder() to construct.
private FrameworkID(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private FrameworkID() {
value_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private FrameworkID(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000001;
value_ = bs;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_FrameworkID_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_FrameworkID_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.mesos.v1.Protos.FrameworkID.class, org.apache.mesos.v1.Protos.FrameworkID.Builder.class);
}
private int bitField0_;
public static final int VALUE_FIELD_NUMBER = 1;
private volatile java.lang.Object value_;
/**
* required string value = 1;
*/
public boolean hasValue() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required string value = 1;
*/
public java.lang.String getValue() {
java.lang.Object ref = value_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
value_ = s;
}
return s;
}
}
/**
* required string value = 1;
*/
public com.google.protobuf.ByteString
getValueBytes() {
java.lang.Object ref = value_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
value_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasValue()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, value_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, value_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.mesos.v1.Protos.FrameworkID)) {
return super.equals(obj);
}
org.apache.mesos.v1.Protos.FrameworkID other = (org.apache.mesos.v1.Protos.FrameworkID) obj;
boolean result = true;
result = result && (hasValue() == other.hasValue());
if (hasValue()) {
result = result && getValue()
.equals(other.getValue());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasValue()) {
hash = (37 * hash) + VALUE_FIELD_NUMBER;
hash = (53 * hash) + getValue().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.mesos.v1.Protos.FrameworkID parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.FrameworkID parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.FrameworkID parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.FrameworkID parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.FrameworkID parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.FrameworkID parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.FrameworkID parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.FrameworkID parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.FrameworkID parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.FrameworkID parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.FrameworkID parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.FrameworkID parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.apache.mesos.v1.Protos.FrameworkID prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
**
* A unique ID assigned to a framework. A framework can reuse this ID
* in order to do failover (see MesosSchedulerDriver).
*
*
* Protobuf type {@code mesos.v1.FrameworkID}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:mesos.v1.FrameworkID)
org.apache.mesos.v1.Protos.FrameworkIDOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_FrameworkID_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_FrameworkID_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.mesos.v1.Protos.FrameworkID.class, org.apache.mesos.v1.Protos.FrameworkID.Builder.class);
}
// Construct using org.apache.mesos.v1.Protos.FrameworkID.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
value_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_FrameworkID_descriptor;
}
public org.apache.mesos.v1.Protos.FrameworkID getDefaultInstanceForType() {
return org.apache.mesos.v1.Protos.FrameworkID.getDefaultInstance();
}
public org.apache.mesos.v1.Protos.FrameworkID build() {
org.apache.mesos.v1.Protos.FrameworkID result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.apache.mesos.v1.Protos.FrameworkID buildPartial() {
org.apache.mesos.v1.Protos.FrameworkID result = new org.apache.mesos.v1.Protos.FrameworkID(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.value_ = value_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.apache.mesos.v1.Protos.FrameworkID) {
return mergeFrom((org.apache.mesos.v1.Protos.FrameworkID)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.mesos.v1.Protos.FrameworkID other) {
if (other == org.apache.mesos.v1.Protos.FrameworkID.getDefaultInstance()) return this;
if (other.hasValue()) {
bitField0_ |= 0x00000001;
value_ = other.value_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
if (!hasValue()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.mesos.v1.Protos.FrameworkID parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.mesos.v1.Protos.FrameworkID) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object value_ = "";
/**
* required string value = 1;
*/
public boolean hasValue() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required string value = 1;
*/
public java.lang.String getValue() {
java.lang.Object ref = value_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
value_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* required string value = 1;
*/
public com.google.protobuf.ByteString
getValueBytes() {
java.lang.Object ref = value_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
value_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* required string value = 1;
*/
public Builder setValue(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
value_ = value;
onChanged();
return this;
}
/**
* required string value = 1;
*/
public Builder clearValue() {
bitField0_ = (bitField0_ & ~0x00000001);
value_ = getDefaultInstance().getValue();
onChanged();
return this;
}
/**
* required string value = 1;
*/
public Builder setValueBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
value_ = value;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:mesos.v1.FrameworkID)
}
// @@protoc_insertion_point(class_scope:mesos.v1.FrameworkID)
private static final org.apache.mesos.v1.Protos.FrameworkID DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.apache.mesos.v1.Protos.FrameworkID();
}
public static org.apache.mesos.v1.Protos.FrameworkID getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public FrameworkID parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new FrameworkID(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.apache.mesos.v1.Protos.FrameworkID getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface OfferIDOrBuilder extends
// @@protoc_insertion_point(interface_extends:mesos.v1.OfferID)
com.google.protobuf.MessageOrBuilder {
/**
* required string value = 1;
*/
boolean hasValue();
/**
* required string value = 1;
*/
java.lang.String getValue();
/**
* required string value = 1;
*/
com.google.protobuf.ByteString
getValueBytes();
}
/**
*
**
* A unique ID assigned to an offer.
*
*
* Protobuf type {@code mesos.v1.OfferID}
*/
public static final class OfferID extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:mesos.v1.OfferID)
OfferIDOrBuilder {
private static final long serialVersionUID = 0L;
// Use OfferID.newBuilder() to construct.
private OfferID(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private OfferID() {
value_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private OfferID(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000001;
value_ = bs;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_OfferID_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_OfferID_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.mesos.v1.Protos.OfferID.class, org.apache.mesos.v1.Protos.OfferID.Builder.class);
}
private int bitField0_;
public static final int VALUE_FIELD_NUMBER = 1;
private volatile java.lang.Object value_;
/**
* required string value = 1;
*/
public boolean hasValue() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required string value = 1;
*/
public java.lang.String getValue() {
java.lang.Object ref = value_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
value_ = s;
}
return s;
}
}
/**
* required string value = 1;
*/
public com.google.protobuf.ByteString
getValueBytes() {
java.lang.Object ref = value_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
value_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasValue()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, value_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, value_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.mesos.v1.Protos.OfferID)) {
return super.equals(obj);
}
org.apache.mesos.v1.Protos.OfferID other = (org.apache.mesos.v1.Protos.OfferID) obj;
boolean result = true;
result = result && (hasValue() == other.hasValue());
if (hasValue()) {
result = result && getValue()
.equals(other.getValue());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasValue()) {
hash = (37 * hash) + VALUE_FIELD_NUMBER;
hash = (53 * hash) + getValue().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.mesos.v1.Protos.OfferID parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.OfferID parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.OfferID parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.OfferID parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.OfferID parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.OfferID parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.OfferID parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.OfferID parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.OfferID parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.OfferID parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.OfferID parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.OfferID parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.apache.mesos.v1.Protos.OfferID prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
**
* A unique ID assigned to an offer.
*
*
* Protobuf type {@code mesos.v1.OfferID}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:mesos.v1.OfferID)
org.apache.mesos.v1.Protos.OfferIDOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_OfferID_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_OfferID_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.mesos.v1.Protos.OfferID.class, org.apache.mesos.v1.Protos.OfferID.Builder.class);
}
// Construct using org.apache.mesos.v1.Protos.OfferID.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
value_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_OfferID_descriptor;
}
public org.apache.mesos.v1.Protos.OfferID getDefaultInstanceForType() {
return org.apache.mesos.v1.Protos.OfferID.getDefaultInstance();
}
public org.apache.mesos.v1.Protos.OfferID build() {
org.apache.mesos.v1.Protos.OfferID result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.apache.mesos.v1.Protos.OfferID buildPartial() {
org.apache.mesos.v1.Protos.OfferID result = new org.apache.mesos.v1.Protos.OfferID(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.value_ = value_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.apache.mesos.v1.Protos.OfferID) {
return mergeFrom((org.apache.mesos.v1.Protos.OfferID)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.mesos.v1.Protos.OfferID other) {
if (other == org.apache.mesos.v1.Protos.OfferID.getDefaultInstance()) return this;
if (other.hasValue()) {
bitField0_ |= 0x00000001;
value_ = other.value_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
if (!hasValue()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.mesos.v1.Protos.OfferID parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.mesos.v1.Protos.OfferID) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object value_ = "";
/**
* required string value = 1;
*/
public boolean hasValue() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required string value = 1;
*/
public java.lang.String getValue() {
java.lang.Object ref = value_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
value_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* required string value = 1;
*/
public com.google.protobuf.ByteString
getValueBytes() {
java.lang.Object ref = value_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
value_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* required string value = 1;
*/
public Builder setValue(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
value_ = value;
onChanged();
return this;
}
/**
* required string value = 1;
*/
public Builder clearValue() {
bitField0_ = (bitField0_ & ~0x00000001);
value_ = getDefaultInstance().getValue();
onChanged();
return this;
}
/**
* required string value = 1;
*/
public Builder setValueBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
value_ = value;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:mesos.v1.OfferID)
}
// @@protoc_insertion_point(class_scope:mesos.v1.OfferID)
private static final org.apache.mesos.v1.Protos.OfferID DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.apache.mesos.v1.Protos.OfferID();
}
public static org.apache.mesos.v1.Protos.OfferID getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public OfferID parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new OfferID(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.apache.mesos.v1.Protos.OfferID getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface AgentIDOrBuilder extends
// @@protoc_insertion_point(interface_extends:mesos.v1.AgentID)
com.google.protobuf.MessageOrBuilder {
/**
* required string value = 1;
*/
boolean hasValue();
/**
* required string value = 1;
*/
java.lang.String getValue();
/**
* required string value = 1;
*/
com.google.protobuf.ByteString
getValueBytes();
}
/**
*
**
* A unique ID assigned to an agent. Currently, an agent gets a new ID
* whenever it (re)registers with Mesos. Framework writers shouldn't
* assume any binding between an agent ID and and a hostname.
*
*
* Protobuf type {@code mesos.v1.AgentID}
*/
public static final class AgentID extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:mesos.v1.AgentID)
AgentIDOrBuilder {
private static final long serialVersionUID = 0L;
// Use AgentID.newBuilder() to construct.
private AgentID(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private AgentID() {
value_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private AgentID(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000001;
value_ = bs;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_AgentID_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_AgentID_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.mesos.v1.Protos.AgentID.class, org.apache.mesos.v1.Protos.AgentID.Builder.class);
}
private int bitField0_;
public static final int VALUE_FIELD_NUMBER = 1;
private volatile java.lang.Object value_;
/**
* required string value = 1;
*/
public boolean hasValue() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required string value = 1;
*/
public java.lang.String getValue() {
java.lang.Object ref = value_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
value_ = s;
}
return s;
}
}
/**
* required string value = 1;
*/
public com.google.protobuf.ByteString
getValueBytes() {
java.lang.Object ref = value_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
value_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasValue()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, value_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, value_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.mesos.v1.Protos.AgentID)) {
return super.equals(obj);
}
org.apache.mesos.v1.Protos.AgentID other = (org.apache.mesos.v1.Protos.AgentID) obj;
boolean result = true;
result = result && (hasValue() == other.hasValue());
if (hasValue()) {
result = result && getValue()
.equals(other.getValue());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasValue()) {
hash = (37 * hash) + VALUE_FIELD_NUMBER;
hash = (53 * hash) + getValue().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.mesos.v1.Protos.AgentID parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.AgentID parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.AgentID parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.AgentID parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.AgentID parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.AgentID parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.AgentID parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.AgentID parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.AgentID parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.AgentID parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.AgentID parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.AgentID parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.apache.mesos.v1.Protos.AgentID prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
**
* A unique ID assigned to an agent. Currently, an agent gets a new ID
* whenever it (re)registers with Mesos. Framework writers shouldn't
* assume any binding between an agent ID and and a hostname.
*
*
* Protobuf type {@code mesos.v1.AgentID}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:mesos.v1.AgentID)
org.apache.mesos.v1.Protos.AgentIDOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_AgentID_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_AgentID_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.mesos.v1.Protos.AgentID.class, org.apache.mesos.v1.Protos.AgentID.Builder.class);
}
// Construct using org.apache.mesos.v1.Protos.AgentID.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
value_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_AgentID_descriptor;
}
public org.apache.mesos.v1.Protos.AgentID getDefaultInstanceForType() {
return org.apache.mesos.v1.Protos.AgentID.getDefaultInstance();
}
public org.apache.mesos.v1.Protos.AgentID build() {
org.apache.mesos.v1.Protos.AgentID result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.apache.mesos.v1.Protos.AgentID buildPartial() {
org.apache.mesos.v1.Protos.AgentID result = new org.apache.mesos.v1.Protos.AgentID(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.value_ = value_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.apache.mesos.v1.Protos.AgentID) {
return mergeFrom((org.apache.mesos.v1.Protos.AgentID)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.mesos.v1.Protos.AgentID other) {
if (other == org.apache.mesos.v1.Protos.AgentID.getDefaultInstance()) return this;
if (other.hasValue()) {
bitField0_ |= 0x00000001;
value_ = other.value_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
if (!hasValue()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.mesos.v1.Protos.AgentID parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.mesos.v1.Protos.AgentID) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object value_ = "";
/**
* required string value = 1;
*/
public boolean hasValue() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required string value = 1;
*/
public java.lang.String getValue() {
java.lang.Object ref = value_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
value_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* required string value = 1;
*/
public com.google.protobuf.ByteString
getValueBytes() {
java.lang.Object ref = value_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
value_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* required string value = 1;
*/
public Builder setValue(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
value_ = value;
onChanged();
return this;
}
/**
* required string value = 1;
*/
public Builder clearValue() {
bitField0_ = (bitField0_ & ~0x00000001);
value_ = getDefaultInstance().getValue();
onChanged();
return this;
}
/**
* required string value = 1;
*/
public Builder setValueBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
value_ = value;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:mesos.v1.AgentID)
}
// @@protoc_insertion_point(class_scope:mesos.v1.AgentID)
private static final org.apache.mesos.v1.Protos.AgentID DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.apache.mesos.v1.Protos.AgentID();
}
public static org.apache.mesos.v1.Protos.AgentID getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public AgentID parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new AgentID(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.apache.mesos.v1.Protos.AgentID getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface TaskIDOrBuilder extends
// @@protoc_insertion_point(interface_extends:mesos.v1.TaskID)
com.google.protobuf.MessageOrBuilder {
/**
* required string value = 1;
*/
boolean hasValue();
/**
* required string value = 1;
*/
java.lang.String getValue();
/**
* required string value = 1;
*/
com.google.protobuf.ByteString
getValueBytes();
}
/**
*
**
* A framework-generated ID to distinguish a task. The ID must remain
* unique while the task is active. A framework can reuse an ID _only_
* if the previous task with the same ID has reached a terminal state
* (e.g., TASK_FINISHED, TASK_KILLED, etc.). However, reusing task IDs
* is strongly discouraged (MESOS-2198).
*
*
* Protobuf type {@code mesos.v1.TaskID}
*/
public static final class TaskID extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:mesos.v1.TaskID)
TaskIDOrBuilder {
private static final long serialVersionUID = 0L;
// Use TaskID.newBuilder() to construct.
private TaskID(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private TaskID() {
value_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private TaskID(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000001;
value_ = bs;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_TaskID_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_TaskID_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.mesos.v1.Protos.TaskID.class, org.apache.mesos.v1.Protos.TaskID.Builder.class);
}
private int bitField0_;
public static final int VALUE_FIELD_NUMBER = 1;
private volatile java.lang.Object value_;
/**
* required string value = 1;
*/
public boolean hasValue() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required string value = 1;
*/
public java.lang.String getValue() {
java.lang.Object ref = value_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
value_ = s;
}
return s;
}
}
/**
* required string value = 1;
*/
public com.google.protobuf.ByteString
getValueBytes() {
java.lang.Object ref = value_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
value_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasValue()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, value_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, value_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.mesos.v1.Protos.TaskID)) {
return super.equals(obj);
}
org.apache.mesos.v1.Protos.TaskID other = (org.apache.mesos.v1.Protos.TaskID) obj;
boolean result = true;
result = result && (hasValue() == other.hasValue());
if (hasValue()) {
result = result && getValue()
.equals(other.getValue());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasValue()) {
hash = (37 * hash) + VALUE_FIELD_NUMBER;
hash = (53 * hash) + getValue().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.mesos.v1.Protos.TaskID parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.TaskID parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.TaskID parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.TaskID parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.TaskID parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.TaskID parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.TaskID parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.TaskID parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.TaskID parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.TaskID parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.TaskID parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.TaskID parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.apache.mesos.v1.Protos.TaskID prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
**
* A framework-generated ID to distinguish a task. The ID must remain
* unique while the task is active. A framework can reuse an ID _only_
* if the previous task with the same ID has reached a terminal state
* (e.g., TASK_FINISHED, TASK_KILLED, etc.). However, reusing task IDs
* is strongly discouraged (MESOS-2198).
*
*
* Protobuf type {@code mesos.v1.TaskID}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:mesos.v1.TaskID)
org.apache.mesos.v1.Protos.TaskIDOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_TaskID_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_TaskID_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.mesos.v1.Protos.TaskID.class, org.apache.mesos.v1.Protos.TaskID.Builder.class);
}
// Construct using org.apache.mesos.v1.Protos.TaskID.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
value_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_TaskID_descriptor;
}
public org.apache.mesos.v1.Protos.TaskID getDefaultInstanceForType() {
return org.apache.mesos.v1.Protos.TaskID.getDefaultInstance();
}
public org.apache.mesos.v1.Protos.TaskID build() {
org.apache.mesos.v1.Protos.TaskID result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.apache.mesos.v1.Protos.TaskID buildPartial() {
org.apache.mesos.v1.Protos.TaskID result = new org.apache.mesos.v1.Protos.TaskID(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.value_ = value_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.apache.mesos.v1.Protos.TaskID) {
return mergeFrom((org.apache.mesos.v1.Protos.TaskID)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.mesos.v1.Protos.TaskID other) {
if (other == org.apache.mesos.v1.Protos.TaskID.getDefaultInstance()) return this;
if (other.hasValue()) {
bitField0_ |= 0x00000001;
value_ = other.value_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
if (!hasValue()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.mesos.v1.Protos.TaskID parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.mesos.v1.Protos.TaskID) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object value_ = "";
/**
* required string value = 1;
*/
public boolean hasValue() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required string value = 1;
*/
public java.lang.String getValue() {
java.lang.Object ref = value_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
value_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* required string value = 1;
*/
public com.google.protobuf.ByteString
getValueBytes() {
java.lang.Object ref = value_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
value_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* required string value = 1;
*/
public Builder setValue(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
value_ = value;
onChanged();
return this;
}
/**
* required string value = 1;
*/
public Builder clearValue() {
bitField0_ = (bitField0_ & ~0x00000001);
value_ = getDefaultInstance().getValue();
onChanged();
return this;
}
/**
* required string value = 1;
*/
public Builder setValueBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
value_ = value;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:mesos.v1.TaskID)
}
// @@protoc_insertion_point(class_scope:mesos.v1.TaskID)
private static final org.apache.mesos.v1.Protos.TaskID DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.apache.mesos.v1.Protos.TaskID();
}
public static org.apache.mesos.v1.Protos.TaskID getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public TaskID parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new TaskID(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.apache.mesos.v1.Protos.TaskID getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ExecutorIDOrBuilder extends
// @@protoc_insertion_point(interface_extends:mesos.v1.ExecutorID)
com.google.protobuf.MessageOrBuilder {
/**
* required string value = 1;
*/
boolean hasValue();
/**
* required string value = 1;
*/
java.lang.String getValue();
/**
* required string value = 1;
*/
com.google.protobuf.ByteString
getValueBytes();
}
/**
*
**
* A framework-generated ID to distinguish an executor. Only one
* executor with the same ID can be active on the same agent at a
* time. However, reusing executor IDs is discouraged.
*
*
* Protobuf type {@code mesos.v1.ExecutorID}
*/
public static final class ExecutorID extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:mesos.v1.ExecutorID)
ExecutorIDOrBuilder {
private static final long serialVersionUID = 0L;
// Use ExecutorID.newBuilder() to construct.
private ExecutorID(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ExecutorID() {
value_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ExecutorID(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000001;
value_ = bs;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_ExecutorID_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_ExecutorID_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.mesos.v1.Protos.ExecutorID.class, org.apache.mesos.v1.Protos.ExecutorID.Builder.class);
}
private int bitField0_;
public static final int VALUE_FIELD_NUMBER = 1;
private volatile java.lang.Object value_;
/**
* required string value = 1;
*/
public boolean hasValue() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required string value = 1;
*/
public java.lang.String getValue() {
java.lang.Object ref = value_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
value_ = s;
}
return s;
}
}
/**
* required string value = 1;
*/
public com.google.protobuf.ByteString
getValueBytes() {
java.lang.Object ref = value_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
value_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasValue()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, value_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, value_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.mesos.v1.Protos.ExecutorID)) {
return super.equals(obj);
}
org.apache.mesos.v1.Protos.ExecutorID other = (org.apache.mesos.v1.Protos.ExecutorID) obj;
boolean result = true;
result = result && (hasValue() == other.hasValue());
if (hasValue()) {
result = result && getValue()
.equals(other.getValue());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasValue()) {
hash = (37 * hash) + VALUE_FIELD_NUMBER;
hash = (53 * hash) + getValue().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.mesos.v1.Protos.ExecutorID parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.ExecutorID parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.ExecutorID parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.ExecutorID parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.ExecutorID parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.ExecutorID parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.ExecutorID parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.ExecutorID parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.ExecutorID parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.ExecutorID parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.ExecutorID parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.ExecutorID parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.apache.mesos.v1.Protos.ExecutorID prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
**
* A framework-generated ID to distinguish an executor. Only one
* executor with the same ID can be active on the same agent at a
* time. However, reusing executor IDs is discouraged.
*
*
* Protobuf type {@code mesos.v1.ExecutorID}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:mesos.v1.ExecutorID)
org.apache.mesos.v1.Protos.ExecutorIDOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_ExecutorID_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_ExecutorID_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.mesos.v1.Protos.ExecutorID.class, org.apache.mesos.v1.Protos.ExecutorID.Builder.class);
}
// Construct using org.apache.mesos.v1.Protos.ExecutorID.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
value_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_ExecutorID_descriptor;
}
public org.apache.mesos.v1.Protos.ExecutorID getDefaultInstanceForType() {
return org.apache.mesos.v1.Protos.ExecutorID.getDefaultInstance();
}
public org.apache.mesos.v1.Protos.ExecutorID build() {
org.apache.mesos.v1.Protos.ExecutorID result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.apache.mesos.v1.Protos.ExecutorID buildPartial() {
org.apache.mesos.v1.Protos.ExecutorID result = new org.apache.mesos.v1.Protos.ExecutorID(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.value_ = value_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.apache.mesos.v1.Protos.ExecutorID) {
return mergeFrom((org.apache.mesos.v1.Protos.ExecutorID)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.mesos.v1.Protos.ExecutorID other) {
if (other == org.apache.mesos.v1.Protos.ExecutorID.getDefaultInstance()) return this;
if (other.hasValue()) {
bitField0_ |= 0x00000001;
value_ = other.value_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
if (!hasValue()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.mesos.v1.Protos.ExecutorID parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.mesos.v1.Protos.ExecutorID) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object value_ = "";
/**
* required string value = 1;
*/
public boolean hasValue() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required string value = 1;
*/
public java.lang.String getValue() {
java.lang.Object ref = value_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
value_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* required string value = 1;
*/
public com.google.protobuf.ByteString
getValueBytes() {
java.lang.Object ref = value_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
value_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* required string value = 1;
*/
public Builder setValue(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
value_ = value;
onChanged();
return this;
}
/**
* required string value = 1;
*/
public Builder clearValue() {
bitField0_ = (bitField0_ & ~0x00000001);
value_ = getDefaultInstance().getValue();
onChanged();
return this;
}
/**
* required string value = 1;
*/
public Builder setValueBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
value_ = value;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:mesos.v1.ExecutorID)
}
// @@protoc_insertion_point(class_scope:mesos.v1.ExecutorID)
private static final org.apache.mesos.v1.Protos.ExecutorID DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.apache.mesos.v1.Protos.ExecutorID();
}
public static org.apache.mesos.v1.Protos.ExecutorID getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public ExecutorID parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ExecutorID(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.apache.mesos.v1.Protos.ExecutorID getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ContainerIDOrBuilder extends
// @@protoc_insertion_point(interface_extends:mesos.v1.ContainerID)
com.google.protobuf.MessageOrBuilder {
/**
* required string value = 1;
*/
boolean hasValue();
/**
* required string value = 1;
*/
java.lang.String getValue();
/**
* required string value = 1;
*/
com.google.protobuf.ByteString
getValueBytes();
/**
* optional .mesos.v1.ContainerID parent = 2;
*/
boolean hasParent();
/**
* optional .mesos.v1.ContainerID parent = 2;
*/
org.apache.mesos.v1.Protos.ContainerID getParent();
/**
* optional .mesos.v1.ContainerID parent = 2;
*/
org.apache.mesos.v1.Protos.ContainerIDOrBuilder getParentOrBuilder();
}
/**
*
**
* ID used to uniquely identify a container. If the `parent` is not
* specified, the ID is a UUID generated by the agent to uniquely
* identify the container of an executor run. If the `parent` field is
* specified, it represents a nested container.
*
*
* Protobuf type {@code mesos.v1.ContainerID}
*/
public static final class ContainerID extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:mesos.v1.ContainerID)
ContainerIDOrBuilder {
private static final long serialVersionUID = 0L;
// Use ContainerID.newBuilder() to construct.
private ContainerID(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ContainerID() {
value_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ContainerID(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000001;
value_ = bs;
break;
}
case 18: {
org.apache.mesos.v1.Protos.ContainerID.Builder subBuilder = null;
if (((bitField0_ & 0x00000002) == 0x00000002)) {
subBuilder = parent_.toBuilder();
}
parent_ = input.readMessage(org.apache.mesos.v1.Protos.ContainerID.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(parent_);
parent_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000002;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_ContainerID_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_ContainerID_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.mesos.v1.Protos.ContainerID.class, org.apache.mesos.v1.Protos.ContainerID.Builder.class);
}
private int bitField0_;
public static final int VALUE_FIELD_NUMBER = 1;
private volatile java.lang.Object value_;
/**
* required string value = 1;
*/
public boolean hasValue() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required string value = 1;
*/
public java.lang.String getValue() {
java.lang.Object ref = value_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
value_ = s;
}
return s;
}
}
/**
* required string value = 1;
*/
public com.google.protobuf.ByteString
getValueBytes() {
java.lang.Object ref = value_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
value_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int PARENT_FIELD_NUMBER = 2;
private org.apache.mesos.v1.Protos.ContainerID parent_;
/**
* optional .mesos.v1.ContainerID parent = 2;
*/
public boolean hasParent() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional .mesos.v1.ContainerID parent = 2;
*/
public org.apache.mesos.v1.Protos.ContainerID getParent() {
return parent_ == null ? org.apache.mesos.v1.Protos.ContainerID.getDefaultInstance() : parent_;
}
/**
* optional .mesos.v1.ContainerID parent = 2;
*/
public org.apache.mesos.v1.Protos.ContainerIDOrBuilder getParentOrBuilder() {
return parent_ == null ? org.apache.mesos.v1.Protos.ContainerID.getDefaultInstance() : parent_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasValue()) {
memoizedIsInitialized = 0;
return false;
}
if (hasParent()) {
if (!getParent().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, value_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeMessage(2, getParent());
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, value_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getParent());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.mesos.v1.Protos.ContainerID)) {
return super.equals(obj);
}
org.apache.mesos.v1.Protos.ContainerID other = (org.apache.mesos.v1.Protos.ContainerID) obj;
boolean result = true;
result = result && (hasValue() == other.hasValue());
if (hasValue()) {
result = result && getValue()
.equals(other.getValue());
}
result = result && (hasParent() == other.hasParent());
if (hasParent()) {
result = result && getParent()
.equals(other.getParent());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasValue()) {
hash = (37 * hash) + VALUE_FIELD_NUMBER;
hash = (53 * hash) + getValue().hashCode();
}
if (hasParent()) {
hash = (37 * hash) + PARENT_FIELD_NUMBER;
hash = (53 * hash) + getParent().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.mesos.v1.Protos.ContainerID parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.ContainerID parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.ContainerID parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.ContainerID parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.ContainerID parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.ContainerID parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.ContainerID parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.ContainerID parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.ContainerID parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.ContainerID parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.ContainerID parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.ContainerID parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.apache.mesos.v1.Protos.ContainerID prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
**
* ID used to uniquely identify a container. If the `parent` is not
* specified, the ID is a UUID generated by the agent to uniquely
* identify the container of an executor run. If the `parent` field is
* specified, it represents a nested container.
*
*
* Protobuf type {@code mesos.v1.ContainerID}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:mesos.v1.ContainerID)
org.apache.mesos.v1.Protos.ContainerIDOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_ContainerID_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_ContainerID_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.mesos.v1.Protos.ContainerID.class, org.apache.mesos.v1.Protos.ContainerID.Builder.class);
}
// Construct using org.apache.mesos.v1.Protos.ContainerID.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getParentFieldBuilder();
}
}
public Builder clear() {
super.clear();
value_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
if (parentBuilder_ == null) {
parent_ = null;
} else {
parentBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_ContainerID_descriptor;
}
public org.apache.mesos.v1.Protos.ContainerID getDefaultInstanceForType() {
return org.apache.mesos.v1.Protos.ContainerID.getDefaultInstance();
}
public org.apache.mesos.v1.Protos.ContainerID build() {
org.apache.mesos.v1.Protos.ContainerID result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.apache.mesos.v1.Protos.ContainerID buildPartial() {
org.apache.mesos.v1.Protos.ContainerID result = new org.apache.mesos.v1.Protos.ContainerID(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.value_ = value_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
if (parentBuilder_ == null) {
result.parent_ = parent_;
} else {
result.parent_ = parentBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.apache.mesos.v1.Protos.ContainerID) {
return mergeFrom((org.apache.mesos.v1.Protos.ContainerID)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.mesos.v1.Protos.ContainerID other) {
if (other == org.apache.mesos.v1.Protos.ContainerID.getDefaultInstance()) return this;
if (other.hasValue()) {
bitField0_ |= 0x00000001;
value_ = other.value_;
onChanged();
}
if (other.hasParent()) {
mergeParent(other.getParent());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
if (!hasValue()) {
return false;
}
if (hasParent()) {
if (!getParent().isInitialized()) {
return false;
}
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.mesos.v1.Protos.ContainerID parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.mesos.v1.Protos.ContainerID) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object value_ = "";
/**
* required string value = 1;
*/
public boolean hasValue() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required string value = 1;
*/
public java.lang.String getValue() {
java.lang.Object ref = value_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
value_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* required string value = 1;
*/
public com.google.protobuf.ByteString
getValueBytes() {
java.lang.Object ref = value_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
value_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* required string value = 1;
*/
public Builder setValue(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
value_ = value;
onChanged();
return this;
}
/**
* required string value = 1;
*/
public Builder clearValue() {
bitField0_ = (bitField0_ & ~0x00000001);
value_ = getDefaultInstance().getValue();
onChanged();
return this;
}
/**
* required string value = 1;
*/
public Builder setValueBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
value_ = value;
onChanged();
return this;
}
private org.apache.mesos.v1.Protos.ContainerID parent_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
org.apache.mesos.v1.Protos.ContainerID, org.apache.mesos.v1.Protos.ContainerID.Builder, org.apache.mesos.v1.Protos.ContainerIDOrBuilder> parentBuilder_;
/**
* optional .mesos.v1.ContainerID parent = 2;
*/
public boolean hasParent() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional .mesos.v1.ContainerID parent = 2;
*/
public org.apache.mesos.v1.Protos.ContainerID getParent() {
if (parentBuilder_ == null) {
return parent_ == null ? org.apache.mesos.v1.Protos.ContainerID.getDefaultInstance() : parent_;
} else {
return parentBuilder_.getMessage();
}
}
/**
* optional .mesos.v1.ContainerID parent = 2;
*/
public Builder setParent(org.apache.mesos.v1.Protos.ContainerID value) {
if (parentBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
parent_ = value;
onChanged();
} else {
parentBuilder_.setMessage(value);
}
bitField0_ |= 0x00000002;
return this;
}
/**
* optional .mesos.v1.ContainerID parent = 2;
*/
public Builder setParent(
org.apache.mesos.v1.Protos.ContainerID.Builder builderForValue) {
if (parentBuilder_ == null) {
parent_ = builderForValue.build();
onChanged();
} else {
parentBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000002;
return this;
}
/**
* optional .mesos.v1.ContainerID parent = 2;
*/
public Builder mergeParent(org.apache.mesos.v1.Protos.ContainerID value) {
if (parentBuilder_ == null) {
if (((bitField0_ & 0x00000002) == 0x00000002) &&
parent_ != null &&
parent_ != org.apache.mesos.v1.Protos.ContainerID.getDefaultInstance()) {
parent_ =
org.apache.mesos.v1.Protos.ContainerID.newBuilder(parent_).mergeFrom(value).buildPartial();
} else {
parent_ = value;
}
onChanged();
} else {
parentBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000002;
return this;
}
/**
* optional .mesos.v1.ContainerID parent = 2;
*/
public Builder clearParent() {
if (parentBuilder_ == null) {
parent_ = null;
onChanged();
} else {
parentBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
/**
* optional .mesos.v1.ContainerID parent = 2;
*/
public org.apache.mesos.v1.Protos.ContainerID.Builder getParentBuilder() {
bitField0_ |= 0x00000002;
onChanged();
return getParentFieldBuilder().getBuilder();
}
/**
* optional .mesos.v1.ContainerID parent = 2;
*/
public org.apache.mesos.v1.Protos.ContainerIDOrBuilder getParentOrBuilder() {
if (parentBuilder_ != null) {
return parentBuilder_.getMessageOrBuilder();
} else {
return parent_ == null ?
org.apache.mesos.v1.Protos.ContainerID.getDefaultInstance() : parent_;
}
}
/**
* optional .mesos.v1.ContainerID parent = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.apache.mesos.v1.Protos.ContainerID, org.apache.mesos.v1.Protos.ContainerID.Builder, org.apache.mesos.v1.Protos.ContainerIDOrBuilder>
getParentFieldBuilder() {
if (parentBuilder_ == null) {
parentBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.apache.mesos.v1.Protos.ContainerID, org.apache.mesos.v1.Protos.ContainerID.Builder, org.apache.mesos.v1.Protos.ContainerIDOrBuilder>(
getParent(),
getParentForChildren(),
isClean());
parent_ = null;
}
return parentBuilder_;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:mesos.v1.ContainerID)
}
// @@protoc_insertion_point(class_scope:mesos.v1.ContainerID)
private static final org.apache.mesos.v1.Protos.ContainerID DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.apache.mesos.v1.Protos.ContainerID();
}
public static org.apache.mesos.v1.Protos.ContainerID getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public ContainerID parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ContainerID(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.apache.mesos.v1.Protos.ContainerID getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ResourceProviderIDOrBuilder extends
// @@protoc_insertion_point(interface_extends:mesos.v1.ResourceProviderID)
com.google.protobuf.MessageOrBuilder {
/**
* required string value = 1;
*/
boolean hasValue();
/**
* required string value = 1;
*/
java.lang.String getValue();
/**
* required string value = 1;
*/
com.google.protobuf.ByteString
getValueBytes();
}
/**
*
**
* A unique ID assigned to a resource provider. Currently, a resource
* provider gets a new ID whenever it (re)registers with Mesos.
*
*
* Protobuf type {@code mesos.v1.ResourceProviderID}
*/
public static final class ResourceProviderID extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:mesos.v1.ResourceProviderID)
ResourceProviderIDOrBuilder {
private static final long serialVersionUID = 0L;
// Use ResourceProviderID.newBuilder() to construct.
private ResourceProviderID(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ResourceProviderID() {
value_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ResourceProviderID(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000001;
value_ = bs;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_ResourceProviderID_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_ResourceProviderID_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.mesos.v1.Protos.ResourceProviderID.class, org.apache.mesos.v1.Protos.ResourceProviderID.Builder.class);
}
private int bitField0_;
public static final int VALUE_FIELD_NUMBER = 1;
private volatile java.lang.Object value_;
/**
* required string value = 1;
*/
public boolean hasValue() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required string value = 1;
*/
public java.lang.String getValue() {
java.lang.Object ref = value_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
value_ = s;
}
return s;
}
}
/**
* required string value = 1;
*/
public com.google.protobuf.ByteString
getValueBytes() {
java.lang.Object ref = value_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
value_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasValue()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, value_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, value_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.mesos.v1.Protos.ResourceProviderID)) {
return super.equals(obj);
}
org.apache.mesos.v1.Protos.ResourceProviderID other = (org.apache.mesos.v1.Protos.ResourceProviderID) obj;
boolean result = true;
result = result && (hasValue() == other.hasValue());
if (hasValue()) {
result = result && getValue()
.equals(other.getValue());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasValue()) {
hash = (37 * hash) + VALUE_FIELD_NUMBER;
hash = (53 * hash) + getValue().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.mesos.v1.Protos.ResourceProviderID parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.ResourceProviderID parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.ResourceProviderID parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.ResourceProviderID parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.ResourceProviderID parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.ResourceProviderID parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.ResourceProviderID parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.ResourceProviderID parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.ResourceProviderID parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.ResourceProviderID parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.ResourceProviderID parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.ResourceProviderID parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.apache.mesos.v1.Protos.ResourceProviderID prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
**
* A unique ID assigned to a resource provider. Currently, a resource
* provider gets a new ID whenever it (re)registers with Mesos.
*
*
* Protobuf type {@code mesos.v1.ResourceProviderID}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:mesos.v1.ResourceProviderID)
org.apache.mesos.v1.Protos.ResourceProviderIDOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_ResourceProviderID_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_ResourceProviderID_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.mesos.v1.Protos.ResourceProviderID.class, org.apache.mesos.v1.Protos.ResourceProviderID.Builder.class);
}
// Construct using org.apache.mesos.v1.Protos.ResourceProviderID.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
value_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_ResourceProviderID_descriptor;
}
public org.apache.mesos.v1.Protos.ResourceProviderID getDefaultInstanceForType() {
return org.apache.mesos.v1.Protos.ResourceProviderID.getDefaultInstance();
}
public org.apache.mesos.v1.Protos.ResourceProviderID build() {
org.apache.mesos.v1.Protos.ResourceProviderID result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.apache.mesos.v1.Protos.ResourceProviderID buildPartial() {
org.apache.mesos.v1.Protos.ResourceProviderID result = new org.apache.mesos.v1.Protos.ResourceProviderID(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.value_ = value_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.apache.mesos.v1.Protos.ResourceProviderID) {
return mergeFrom((org.apache.mesos.v1.Protos.ResourceProviderID)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.mesos.v1.Protos.ResourceProviderID other) {
if (other == org.apache.mesos.v1.Protos.ResourceProviderID.getDefaultInstance()) return this;
if (other.hasValue()) {
bitField0_ |= 0x00000001;
value_ = other.value_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
if (!hasValue()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.mesos.v1.Protos.ResourceProviderID parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.mesos.v1.Protos.ResourceProviderID) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object value_ = "";
/**
* required string value = 1;
*/
public boolean hasValue() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required string value = 1;
*/
public java.lang.String getValue() {
java.lang.Object ref = value_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
value_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* required string value = 1;
*/
public com.google.protobuf.ByteString
getValueBytes() {
java.lang.Object ref = value_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
value_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* required string value = 1;
*/
public Builder setValue(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
value_ = value;
onChanged();
return this;
}
/**
* required string value = 1;
*/
public Builder clearValue() {
bitField0_ = (bitField0_ & ~0x00000001);
value_ = getDefaultInstance().getValue();
onChanged();
return this;
}
/**
* required string value = 1;
*/
public Builder setValueBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
value_ = value;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:mesos.v1.ResourceProviderID)
}
// @@protoc_insertion_point(class_scope:mesos.v1.ResourceProviderID)
private static final org.apache.mesos.v1.Protos.ResourceProviderID DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.apache.mesos.v1.Protos.ResourceProviderID();
}
public static org.apache.mesos.v1.Protos.ResourceProviderID getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public ResourceProviderID parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ResourceProviderID(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.apache.mesos.v1.Protos.ResourceProviderID getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface OperationIDOrBuilder extends
// @@protoc_insertion_point(interface_extends:mesos.v1.OperationID)
com.google.protobuf.MessageOrBuilder {
/**
* required string value = 1;
*/
boolean hasValue();
/**
* required string value = 1;
*/
java.lang.String getValue();
/**
* required string value = 1;
*/
com.google.protobuf.ByteString
getValueBytes();
}
/**
*
**
* A framework-generated ID to distinguish an operation. The ID
* must be unique within the framework.
*
*
* Protobuf type {@code mesos.v1.OperationID}
*/
public static final class OperationID extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:mesos.v1.OperationID)
OperationIDOrBuilder {
private static final long serialVersionUID = 0L;
// Use OperationID.newBuilder() to construct.
private OperationID(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private OperationID() {
value_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private OperationID(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000001;
value_ = bs;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_OperationID_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_OperationID_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.mesos.v1.Protos.OperationID.class, org.apache.mesos.v1.Protos.OperationID.Builder.class);
}
private int bitField0_;
public static final int VALUE_FIELD_NUMBER = 1;
private volatile java.lang.Object value_;
/**
* required string value = 1;
*/
public boolean hasValue() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required string value = 1;
*/
public java.lang.String getValue() {
java.lang.Object ref = value_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
value_ = s;
}
return s;
}
}
/**
* required string value = 1;
*/
public com.google.protobuf.ByteString
getValueBytes() {
java.lang.Object ref = value_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
value_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasValue()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, value_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, value_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.mesos.v1.Protos.OperationID)) {
return super.equals(obj);
}
org.apache.mesos.v1.Protos.OperationID other = (org.apache.mesos.v1.Protos.OperationID) obj;
boolean result = true;
result = result && (hasValue() == other.hasValue());
if (hasValue()) {
result = result && getValue()
.equals(other.getValue());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasValue()) {
hash = (37 * hash) + VALUE_FIELD_NUMBER;
hash = (53 * hash) + getValue().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.mesos.v1.Protos.OperationID parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.OperationID parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.OperationID parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.OperationID parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.OperationID parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.OperationID parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.OperationID parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.OperationID parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.OperationID parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.OperationID parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.OperationID parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.OperationID parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.apache.mesos.v1.Protos.OperationID prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
**
* A framework-generated ID to distinguish an operation. The ID
* must be unique within the framework.
*
*
* Protobuf type {@code mesos.v1.OperationID}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:mesos.v1.OperationID)
org.apache.mesos.v1.Protos.OperationIDOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_OperationID_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_OperationID_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.mesos.v1.Protos.OperationID.class, org.apache.mesos.v1.Protos.OperationID.Builder.class);
}
// Construct using org.apache.mesos.v1.Protos.OperationID.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
value_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_OperationID_descriptor;
}
public org.apache.mesos.v1.Protos.OperationID getDefaultInstanceForType() {
return org.apache.mesos.v1.Protos.OperationID.getDefaultInstance();
}
public org.apache.mesos.v1.Protos.OperationID build() {
org.apache.mesos.v1.Protos.OperationID result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.apache.mesos.v1.Protos.OperationID buildPartial() {
org.apache.mesos.v1.Protos.OperationID result = new org.apache.mesos.v1.Protos.OperationID(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.value_ = value_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.apache.mesos.v1.Protos.OperationID) {
return mergeFrom((org.apache.mesos.v1.Protos.OperationID)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.mesos.v1.Protos.OperationID other) {
if (other == org.apache.mesos.v1.Protos.OperationID.getDefaultInstance()) return this;
if (other.hasValue()) {
bitField0_ |= 0x00000001;
value_ = other.value_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
if (!hasValue()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.mesos.v1.Protos.OperationID parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.mesos.v1.Protos.OperationID) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object value_ = "";
/**
* required string value = 1;
*/
public boolean hasValue() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required string value = 1;
*/
public java.lang.String getValue() {
java.lang.Object ref = value_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
value_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* required string value = 1;
*/
public com.google.protobuf.ByteString
getValueBytes() {
java.lang.Object ref = value_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
value_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* required string value = 1;
*/
public Builder setValue(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
value_ = value;
onChanged();
return this;
}
/**
* required string value = 1;
*/
public Builder clearValue() {
bitField0_ = (bitField0_ & ~0x00000001);
value_ = getDefaultInstance().getValue();
onChanged();
return this;
}
/**
* required string value = 1;
*/
public Builder setValueBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
value_ = value;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:mesos.v1.OperationID)
}
// @@protoc_insertion_point(class_scope:mesos.v1.OperationID)
private static final org.apache.mesos.v1.Protos.OperationID DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.apache.mesos.v1.Protos.OperationID();
}
public static org.apache.mesos.v1.Protos.OperationID getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public OperationID parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new OperationID(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.apache.mesos.v1.Protos.OperationID getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface TimeInfoOrBuilder extends
// @@protoc_insertion_point(interface_extends:mesos.v1.TimeInfo)
com.google.protobuf.MessageOrBuilder {
/**
* required int64 nanoseconds = 1;
*/
boolean hasNanoseconds();
/**
* required int64 nanoseconds = 1;
*/
long getNanoseconds();
}
/**
*
**
* Represents time since the epoch, in nanoseconds.
*
*
* Protobuf type {@code mesos.v1.TimeInfo}
*/
public static final class TimeInfo extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:mesos.v1.TimeInfo)
TimeInfoOrBuilder {
private static final long serialVersionUID = 0L;
// Use TimeInfo.newBuilder() to construct.
private TimeInfo(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private TimeInfo() {
nanoseconds_ = 0L;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private TimeInfo(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
bitField0_ |= 0x00000001;
nanoseconds_ = input.readInt64();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_TimeInfo_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_TimeInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.mesos.v1.Protos.TimeInfo.class, org.apache.mesos.v1.Protos.TimeInfo.Builder.class);
}
private int bitField0_;
public static final int NANOSECONDS_FIELD_NUMBER = 1;
private long nanoseconds_;
/**
* required int64 nanoseconds = 1;
*/
public boolean hasNanoseconds() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required int64 nanoseconds = 1;
*/
public long getNanoseconds() {
return nanoseconds_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasNanoseconds()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeInt64(1, nanoseconds_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(1, nanoseconds_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.mesos.v1.Protos.TimeInfo)) {
return super.equals(obj);
}
org.apache.mesos.v1.Protos.TimeInfo other = (org.apache.mesos.v1.Protos.TimeInfo) obj;
boolean result = true;
result = result && (hasNanoseconds() == other.hasNanoseconds());
if (hasNanoseconds()) {
result = result && (getNanoseconds()
== other.getNanoseconds());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasNanoseconds()) {
hash = (37 * hash) + NANOSECONDS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getNanoseconds());
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.mesos.v1.Protos.TimeInfo parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.TimeInfo parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.TimeInfo parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.TimeInfo parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.TimeInfo parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.TimeInfo parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.TimeInfo parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.TimeInfo parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.TimeInfo parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.TimeInfo parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.TimeInfo parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.TimeInfo parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.apache.mesos.v1.Protos.TimeInfo prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
**
* Represents time since the epoch, in nanoseconds.
*
*
* Protobuf type {@code mesos.v1.TimeInfo}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:mesos.v1.TimeInfo)
org.apache.mesos.v1.Protos.TimeInfoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_TimeInfo_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_TimeInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.mesos.v1.Protos.TimeInfo.class, org.apache.mesos.v1.Protos.TimeInfo.Builder.class);
}
// Construct using org.apache.mesos.v1.Protos.TimeInfo.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
nanoseconds_ = 0L;
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_TimeInfo_descriptor;
}
public org.apache.mesos.v1.Protos.TimeInfo getDefaultInstanceForType() {
return org.apache.mesos.v1.Protos.TimeInfo.getDefaultInstance();
}
public org.apache.mesos.v1.Protos.TimeInfo build() {
org.apache.mesos.v1.Protos.TimeInfo result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.apache.mesos.v1.Protos.TimeInfo buildPartial() {
org.apache.mesos.v1.Protos.TimeInfo result = new org.apache.mesos.v1.Protos.TimeInfo(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.nanoseconds_ = nanoseconds_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.apache.mesos.v1.Protos.TimeInfo) {
return mergeFrom((org.apache.mesos.v1.Protos.TimeInfo)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.mesos.v1.Protos.TimeInfo other) {
if (other == org.apache.mesos.v1.Protos.TimeInfo.getDefaultInstance()) return this;
if (other.hasNanoseconds()) {
setNanoseconds(other.getNanoseconds());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
if (!hasNanoseconds()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.mesos.v1.Protos.TimeInfo parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.mesos.v1.Protos.TimeInfo) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private long nanoseconds_ ;
/**
* required int64 nanoseconds = 1;
*/
public boolean hasNanoseconds() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required int64 nanoseconds = 1;
*/
public long getNanoseconds() {
return nanoseconds_;
}
/**
* required int64 nanoseconds = 1;
*/
public Builder setNanoseconds(long value) {
bitField0_ |= 0x00000001;
nanoseconds_ = value;
onChanged();
return this;
}
/**
* required int64 nanoseconds = 1;
*/
public Builder clearNanoseconds() {
bitField0_ = (bitField0_ & ~0x00000001);
nanoseconds_ = 0L;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:mesos.v1.TimeInfo)
}
// @@protoc_insertion_point(class_scope:mesos.v1.TimeInfo)
private static final org.apache.mesos.v1.Protos.TimeInfo DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.apache.mesos.v1.Protos.TimeInfo();
}
public static org.apache.mesos.v1.Protos.TimeInfo getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public TimeInfo parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new TimeInfo(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.apache.mesos.v1.Protos.TimeInfo getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface DurationInfoOrBuilder extends
// @@protoc_insertion_point(interface_extends:mesos.v1.DurationInfo)
com.google.protobuf.MessageOrBuilder {
/**
* required int64 nanoseconds = 1;
*/
boolean hasNanoseconds();
/**
* required int64 nanoseconds = 1;
*/
long getNanoseconds();
}
/**
*
**
* Represents duration in nanoseconds.
*
*
* Protobuf type {@code mesos.v1.DurationInfo}
*/
public static final class DurationInfo extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:mesos.v1.DurationInfo)
DurationInfoOrBuilder {
private static final long serialVersionUID = 0L;
// Use DurationInfo.newBuilder() to construct.
private DurationInfo(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private DurationInfo() {
nanoseconds_ = 0L;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private DurationInfo(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
bitField0_ |= 0x00000001;
nanoseconds_ = input.readInt64();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_DurationInfo_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_DurationInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.mesos.v1.Protos.DurationInfo.class, org.apache.mesos.v1.Protos.DurationInfo.Builder.class);
}
private int bitField0_;
public static final int NANOSECONDS_FIELD_NUMBER = 1;
private long nanoseconds_;
/**
* required int64 nanoseconds = 1;
*/
public boolean hasNanoseconds() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required int64 nanoseconds = 1;
*/
public long getNanoseconds() {
return nanoseconds_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasNanoseconds()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeInt64(1, nanoseconds_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(1, nanoseconds_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.mesos.v1.Protos.DurationInfo)) {
return super.equals(obj);
}
org.apache.mesos.v1.Protos.DurationInfo other = (org.apache.mesos.v1.Protos.DurationInfo) obj;
boolean result = true;
result = result && (hasNanoseconds() == other.hasNanoseconds());
if (hasNanoseconds()) {
result = result && (getNanoseconds()
== other.getNanoseconds());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasNanoseconds()) {
hash = (37 * hash) + NANOSECONDS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getNanoseconds());
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.mesos.v1.Protos.DurationInfo parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.DurationInfo parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.DurationInfo parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.DurationInfo parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.DurationInfo parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.DurationInfo parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.DurationInfo parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.DurationInfo parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.DurationInfo parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.DurationInfo parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.DurationInfo parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.DurationInfo parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.apache.mesos.v1.Protos.DurationInfo prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
**
* Represents duration in nanoseconds.
*
*
* Protobuf type {@code mesos.v1.DurationInfo}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:mesos.v1.DurationInfo)
org.apache.mesos.v1.Protos.DurationInfoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_DurationInfo_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_DurationInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.mesos.v1.Protos.DurationInfo.class, org.apache.mesos.v1.Protos.DurationInfo.Builder.class);
}
// Construct using org.apache.mesos.v1.Protos.DurationInfo.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
nanoseconds_ = 0L;
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_DurationInfo_descriptor;
}
public org.apache.mesos.v1.Protos.DurationInfo getDefaultInstanceForType() {
return org.apache.mesos.v1.Protos.DurationInfo.getDefaultInstance();
}
public org.apache.mesos.v1.Protos.DurationInfo build() {
org.apache.mesos.v1.Protos.DurationInfo result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.apache.mesos.v1.Protos.DurationInfo buildPartial() {
org.apache.mesos.v1.Protos.DurationInfo result = new org.apache.mesos.v1.Protos.DurationInfo(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.nanoseconds_ = nanoseconds_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.apache.mesos.v1.Protos.DurationInfo) {
return mergeFrom((org.apache.mesos.v1.Protos.DurationInfo)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.mesos.v1.Protos.DurationInfo other) {
if (other == org.apache.mesos.v1.Protos.DurationInfo.getDefaultInstance()) return this;
if (other.hasNanoseconds()) {
setNanoseconds(other.getNanoseconds());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
if (!hasNanoseconds()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.mesos.v1.Protos.DurationInfo parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.mesos.v1.Protos.DurationInfo) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private long nanoseconds_ ;
/**
* required int64 nanoseconds = 1;
*/
public boolean hasNanoseconds() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required int64 nanoseconds = 1;
*/
public long getNanoseconds() {
return nanoseconds_;
}
/**
* required int64 nanoseconds = 1;
*/
public Builder setNanoseconds(long value) {
bitField0_ |= 0x00000001;
nanoseconds_ = value;
onChanged();
return this;
}
/**
* required int64 nanoseconds = 1;
*/
public Builder clearNanoseconds() {
bitField0_ = (bitField0_ & ~0x00000001);
nanoseconds_ = 0L;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:mesos.v1.DurationInfo)
}
// @@protoc_insertion_point(class_scope:mesos.v1.DurationInfo)
private static final org.apache.mesos.v1.Protos.DurationInfo DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.apache.mesos.v1.Protos.DurationInfo();
}
public static org.apache.mesos.v1.Protos.DurationInfo getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public DurationInfo parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new DurationInfo(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.apache.mesos.v1.Protos.DurationInfo getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface AddressOrBuilder extends
// @@protoc_insertion_point(interface_extends:mesos.v1.Address)
com.google.protobuf.MessageOrBuilder {
/**
*
* May contain a hostname, IP address, or both.
*
*
* optional string hostname = 1;
*/
boolean hasHostname();
/**
*
* May contain a hostname, IP address, or both.
*
*
* optional string hostname = 1;
*/
java.lang.String getHostname();
/**
*
* May contain a hostname, IP address, or both.
*
*
* optional string hostname = 1;
*/
com.google.protobuf.ByteString
getHostnameBytes();
/**
* optional string ip = 2;
*/
boolean hasIp();
/**
* optional string ip = 2;
*/
java.lang.String getIp();
/**
* optional string ip = 2;
*/
com.google.protobuf.ByteString
getIpBytes();
/**
* required int32 port = 3;
*/
boolean hasPort();
/**
* required int32 port = 3;
*/
int getPort();
}
/**
*
**
* A network address.
* TODO(bmahler): Use this more widely.
*
*
* Protobuf type {@code mesos.v1.Address}
*/
public static final class Address extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:mesos.v1.Address)
AddressOrBuilder {
private static final long serialVersionUID = 0L;
// Use Address.newBuilder() to construct.
private Address(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Address() {
hostname_ = "";
ip_ = "";
port_ = 0;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Address(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000001;
hostname_ = bs;
break;
}
case 18: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000002;
ip_ = bs;
break;
}
case 24: {
bitField0_ |= 0x00000004;
port_ = input.readInt32();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_Address_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_Address_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.mesos.v1.Protos.Address.class, org.apache.mesos.v1.Protos.Address.Builder.class);
}
private int bitField0_;
public static final int HOSTNAME_FIELD_NUMBER = 1;
private volatile java.lang.Object hostname_;
/**
*
* May contain a hostname, IP address, or both.
*
*
* optional string hostname = 1;
*/
public boolean hasHostname() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
*
* May contain a hostname, IP address, or both.
*
*
* optional string hostname = 1;
*/
public java.lang.String getHostname() {
java.lang.Object ref = hostname_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
hostname_ = s;
}
return s;
}
}
/**
*
* May contain a hostname, IP address, or both.
*
*
* optional string hostname = 1;
*/
public com.google.protobuf.ByteString
getHostnameBytes() {
java.lang.Object ref = hostname_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
hostname_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int IP_FIELD_NUMBER = 2;
private volatile java.lang.Object ip_;
/**
* optional string ip = 2;
*/
public boolean hasIp() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional string ip = 2;
*/
public java.lang.String getIp() {
java.lang.Object ref = ip_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
ip_ = s;
}
return s;
}
}
/**
* optional string ip = 2;
*/
public com.google.protobuf.ByteString
getIpBytes() {
java.lang.Object ref = ip_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
ip_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int PORT_FIELD_NUMBER = 3;
private int port_;
/**
* required int32 port = 3;
*/
public boolean hasPort() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* required int32 port = 3;
*/
public int getPort() {
return port_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasPort()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, hostname_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, ip_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeInt32(3, port_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, hostname_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, ip_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(3, port_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.mesos.v1.Protos.Address)) {
return super.equals(obj);
}
org.apache.mesos.v1.Protos.Address other = (org.apache.mesos.v1.Protos.Address) obj;
boolean result = true;
result = result && (hasHostname() == other.hasHostname());
if (hasHostname()) {
result = result && getHostname()
.equals(other.getHostname());
}
result = result && (hasIp() == other.hasIp());
if (hasIp()) {
result = result && getIp()
.equals(other.getIp());
}
result = result && (hasPort() == other.hasPort());
if (hasPort()) {
result = result && (getPort()
== other.getPort());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasHostname()) {
hash = (37 * hash) + HOSTNAME_FIELD_NUMBER;
hash = (53 * hash) + getHostname().hashCode();
}
if (hasIp()) {
hash = (37 * hash) + IP_FIELD_NUMBER;
hash = (53 * hash) + getIp().hashCode();
}
if (hasPort()) {
hash = (37 * hash) + PORT_FIELD_NUMBER;
hash = (53 * hash) + getPort();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.mesos.v1.Protos.Address parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.Address parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.Address parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.Address parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.Address parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.Address parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.Address parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.Address parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.Address parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.Address parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.Address parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.Address parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.apache.mesos.v1.Protos.Address prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
**
* A network address.
* TODO(bmahler): Use this more widely.
*
*
* Protobuf type {@code mesos.v1.Address}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:mesos.v1.Address)
org.apache.mesos.v1.Protos.AddressOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_Address_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_Address_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.mesos.v1.Protos.Address.class, org.apache.mesos.v1.Protos.Address.Builder.class);
}
// Construct using org.apache.mesos.v1.Protos.Address.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
hostname_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
ip_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
port_ = 0;
bitField0_ = (bitField0_ & ~0x00000004);
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_Address_descriptor;
}
public org.apache.mesos.v1.Protos.Address getDefaultInstanceForType() {
return org.apache.mesos.v1.Protos.Address.getDefaultInstance();
}
public org.apache.mesos.v1.Protos.Address build() {
org.apache.mesos.v1.Protos.Address result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.apache.mesos.v1.Protos.Address buildPartial() {
org.apache.mesos.v1.Protos.Address result = new org.apache.mesos.v1.Protos.Address(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.hostname_ = hostname_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.ip_ = ip_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.port_ = port_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.apache.mesos.v1.Protos.Address) {
return mergeFrom((org.apache.mesos.v1.Protos.Address)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.mesos.v1.Protos.Address other) {
if (other == org.apache.mesos.v1.Protos.Address.getDefaultInstance()) return this;
if (other.hasHostname()) {
bitField0_ |= 0x00000001;
hostname_ = other.hostname_;
onChanged();
}
if (other.hasIp()) {
bitField0_ |= 0x00000002;
ip_ = other.ip_;
onChanged();
}
if (other.hasPort()) {
setPort(other.getPort());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
if (!hasPort()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.mesos.v1.Protos.Address parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.mesos.v1.Protos.Address) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object hostname_ = "";
/**
*
* May contain a hostname, IP address, or both.
*
*
* optional string hostname = 1;
*/
public boolean hasHostname() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
*
* May contain a hostname, IP address, or both.
*
*
* optional string hostname = 1;
*/
public java.lang.String getHostname() {
java.lang.Object ref = hostname_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
hostname_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* May contain a hostname, IP address, or both.
*
*
* optional string hostname = 1;
*/
public com.google.protobuf.ByteString
getHostnameBytes() {
java.lang.Object ref = hostname_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
hostname_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* May contain a hostname, IP address, or both.
*
*
* optional string hostname = 1;
*/
public Builder setHostname(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
hostname_ = value;
onChanged();
return this;
}
/**
*
* May contain a hostname, IP address, or both.
*
*
* optional string hostname = 1;
*/
public Builder clearHostname() {
bitField0_ = (bitField0_ & ~0x00000001);
hostname_ = getDefaultInstance().getHostname();
onChanged();
return this;
}
/**
*
* May contain a hostname, IP address, or both.
*
*
* optional string hostname = 1;
*/
public Builder setHostnameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
hostname_ = value;
onChanged();
return this;
}
private java.lang.Object ip_ = "";
/**
* optional string ip = 2;
*/
public boolean hasIp() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional string ip = 2;
*/
public java.lang.String getIp() {
java.lang.Object ref = ip_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
ip_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string ip = 2;
*/
public com.google.protobuf.ByteString
getIpBytes() {
java.lang.Object ref = ip_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
ip_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string ip = 2;
*/
public Builder setIp(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
ip_ = value;
onChanged();
return this;
}
/**
* optional string ip = 2;
*/
public Builder clearIp() {
bitField0_ = (bitField0_ & ~0x00000002);
ip_ = getDefaultInstance().getIp();
onChanged();
return this;
}
/**
* optional string ip = 2;
*/
public Builder setIpBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
ip_ = value;
onChanged();
return this;
}
private int port_ ;
/**
* required int32 port = 3;
*/
public boolean hasPort() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* required int32 port = 3;
*/
public int getPort() {
return port_;
}
/**
* required int32 port = 3;
*/
public Builder setPort(int value) {
bitField0_ |= 0x00000004;
port_ = value;
onChanged();
return this;
}
/**
* required int32 port = 3;
*/
public Builder clearPort() {
bitField0_ = (bitField0_ & ~0x00000004);
port_ = 0;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:mesos.v1.Address)
}
// @@protoc_insertion_point(class_scope:mesos.v1.Address)
private static final org.apache.mesos.v1.Protos.Address DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.apache.mesos.v1.Protos.Address();
}
public static org.apache.mesos.v1.Protos.Address getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public Address parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Address(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.apache.mesos.v1.Protos.Address getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface URLOrBuilder extends
// @@protoc_insertion_point(interface_extends:mesos.v1.URL)
com.google.protobuf.MessageOrBuilder {
/**
* required string scheme = 1;
*/
boolean hasScheme();
/**
* required string scheme = 1;
*/
java.lang.String getScheme();
/**
* required string scheme = 1;
*/
com.google.protobuf.ByteString
getSchemeBytes();
/**
* required .mesos.v1.Address address = 2;
*/
boolean hasAddress();
/**
* required .mesos.v1.Address address = 2;
*/
org.apache.mesos.v1.Protos.Address getAddress();
/**
* required .mesos.v1.Address address = 2;
*/
org.apache.mesos.v1.Protos.AddressOrBuilder getAddressOrBuilder();
/**
* optional string path = 3;
*/
boolean hasPath();
/**
* optional string path = 3;
*/
java.lang.String getPath();
/**
* optional string path = 3;
*/
com.google.protobuf.ByteString
getPathBytes();
/**
* repeated .mesos.v1.Parameter query = 4;
*/
java.util.List
getQueryList();
/**
* repeated .mesos.v1.Parameter query = 4;
*/
org.apache.mesos.v1.Protos.Parameter getQuery(int index);
/**
* repeated .mesos.v1.Parameter query = 4;
*/
int getQueryCount();
/**
* repeated .mesos.v1.Parameter query = 4;
*/
java.util.List extends org.apache.mesos.v1.Protos.ParameterOrBuilder>
getQueryOrBuilderList();
/**
* repeated .mesos.v1.Parameter query = 4;
*/
org.apache.mesos.v1.Protos.ParameterOrBuilder getQueryOrBuilder(
int index);
/**
* optional string fragment = 5;
*/
boolean hasFragment();
/**
* optional string fragment = 5;
*/
java.lang.String getFragment();
/**
* optional string fragment = 5;
*/
com.google.protobuf.ByteString
getFragmentBytes();
}
/**
*
**
* Represents a URL.
*
*
* Protobuf type {@code mesos.v1.URL}
*/
public static final class URL extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:mesos.v1.URL)
URLOrBuilder {
private static final long serialVersionUID = 0L;
// Use URL.newBuilder() to construct.
private URL(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private URL() {
scheme_ = "";
path_ = "";
query_ = java.util.Collections.emptyList();
fragment_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private URL(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000001;
scheme_ = bs;
break;
}
case 18: {
org.apache.mesos.v1.Protos.Address.Builder subBuilder = null;
if (((bitField0_ & 0x00000002) == 0x00000002)) {
subBuilder = address_.toBuilder();
}
address_ = input.readMessage(org.apache.mesos.v1.Protos.Address.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(address_);
address_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000002;
break;
}
case 26: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000004;
path_ = bs;
break;
}
case 34: {
if (!((mutable_bitField0_ & 0x00000008) == 0x00000008)) {
query_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000008;
}
query_.add(
input.readMessage(org.apache.mesos.v1.Protos.Parameter.PARSER, extensionRegistry));
break;
}
case 42: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000008;
fragment_ = bs;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000008) == 0x00000008)) {
query_ = java.util.Collections.unmodifiableList(query_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_URL_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_URL_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.mesos.v1.Protos.URL.class, org.apache.mesos.v1.Protos.URL.Builder.class);
}
private int bitField0_;
public static final int SCHEME_FIELD_NUMBER = 1;
private volatile java.lang.Object scheme_;
/**
* required string scheme = 1;
*/
public boolean hasScheme() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required string scheme = 1;
*/
public java.lang.String getScheme() {
java.lang.Object ref = scheme_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
scheme_ = s;
}
return s;
}
}
/**
* required string scheme = 1;
*/
public com.google.protobuf.ByteString
getSchemeBytes() {
java.lang.Object ref = scheme_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
scheme_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ADDRESS_FIELD_NUMBER = 2;
private org.apache.mesos.v1.Protos.Address address_;
/**
* required .mesos.v1.Address address = 2;
*/
public boolean hasAddress() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required .mesos.v1.Address address = 2;
*/
public org.apache.mesos.v1.Protos.Address getAddress() {
return address_ == null ? org.apache.mesos.v1.Protos.Address.getDefaultInstance() : address_;
}
/**
* required .mesos.v1.Address address = 2;
*/
public org.apache.mesos.v1.Protos.AddressOrBuilder getAddressOrBuilder() {
return address_ == null ? org.apache.mesos.v1.Protos.Address.getDefaultInstance() : address_;
}
public static final int PATH_FIELD_NUMBER = 3;
private volatile java.lang.Object path_;
/**
* optional string path = 3;
*/
public boolean hasPath() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional string path = 3;
*/
public java.lang.String getPath() {
java.lang.Object ref = path_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
path_ = s;
}
return s;
}
}
/**
* optional string path = 3;
*/
public com.google.protobuf.ByteString
getPathBytes() {
java.lang.Object ref = path_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
path_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int QUERY_FIELD_NUMBER = 4;
private java.util.List query_;
/**
* repeated .mesos.v1.Parameter query = 4;
*/
public java.util.List getQueryList() {
return query_;
}
/**
* repeated .mesos.v1.Parameter query = 4;
*/
public java.util.List extends org.apache.mesos.v1.Protos.ParameterOrBuilder>
getQueryOrBuilderList() {
return query_;
}
/**
* repeated .mesos.v1.Parameter query = 4;
*/
public int getQueryCount() {
return query_.size();
}
/**
* repeated .mesos.v1.Parameter query = 4;
*/
public org.apache.mesos.v1.Protos.Parameter getQuery(int index) {
return query_.get(index);
}
/**
* repeated .mesos.v1.Parameter query = 4;
*/
public org.apache.mesos.v1.Protos.ParameterOrBuilder getQueryOrBuilder(
int index) {
return query_.get(index);
}
public static final int FRAGMENT_FIELD_NUMBER = 5;
private volatile java.lang.Object fragment_;
/**
* optional string fragment = 5;
*/
public boolean hasFragment() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional string fragment = 5;
*/
public java.lang.String getFragment() {
java.lang.Object ref = fragment_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
fragment_ = s;
}
return s;
}
}
/**
* optional string fragment = 5;
*/
public com.google.protobuf.ByteString
getFragmentBytes() {
java.lang.Object ref = fragment_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
fragment_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasScheme()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasAddress()) {
memoizedIsInitialized = 0;
return false;
}
if (!getAddress().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
for (int i = 0; i < getQueryCount(); i++) {
if (!getQuery(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, scheme_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeMessage(2, getAddress());
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, path_);
}
for (int i = 0; i < query_.size(); i++) {
output.writeMessage(4, query_.get(i));
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 5, fragment_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, scheme_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getAddress());
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, path_);
}
for (int i = 0; i < query_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, query_.get(i));
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(5, fragment_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.mesos.v1.Protos.URL)) {
return super.equals(obj);
}
org.apache.mesos.v1.Protos.URL other = (org.apache.mesos.v1.Protos.URL) obj;
boolean result = true;
result = result && (hasScheme() == other.hasScheme());
if (hasScheme()) {
result = result && getScheme()
.equals(other.getScheme());
}
result = result && (hasAddress() == other.hasAddress());
if (hasAddress()) {
result = result && getAddress()
.equals(other.getAddress());
}
result = result && (hasPath() == other.hasPath());
if (hasPath()) {
result = result && getPath()
.equals(other.getPath());
}
result = result && getQueryList()
.equals(other.getQueryList());
result = result && (hasFragment() == other.hasFragment());
if (hasFragment()) {
result = result && getFragment()
.equals(other.getFragment());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasScheme()) {
hash = (37 * hash) + SCHEME_FIELD_NUMBER;
hash = (53 * hash) + getScheme().hashCode();
}
if (hasAddress()) {
hash = (37 * hash) + ADDRESS_FIELD_NUMBER;
hash = (53 * hash) + getAddress().hashCode();
}
if (hasPath()) {
hash = (37 * hash) + PATH_FIELD_NUMBER;
hash = (53 * hash) + getPath().hashCode();
}
if (getQueryCount() > 0) {
hash = (37 * hash) + QUERY_FIELD_NUMBER;
hash = (53 * hash) + getQueryList().hashCode();
}
if (hasFragment()) {
hash = (37 * hash) + FRAGMENT_FIELD_NUMBER;
hash = (53 * hash) + getFragment().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.mesos.v1.Protos.URL parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.URL parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.URL parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.URL parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.URL parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.URL parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.URL parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.URL parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.URL parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.URL parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.URL parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.URL parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.apache.mesos.v1.Protos.URL prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
**
* Represents a URL.
*
*
* Protobuf type {@code mesos.v1.URL}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:mesos.v1.URL)
org.apache.mesos.v1.Protos.URLOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_URL_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_URL_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.mesos.v1.Protos.URL.class, org.apache.mesos.v1.Protos.URL.Builder.class);
}
// Construct using org.apache.mesos.v1.Protos.URL.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getAddressFieldBuilder();
getQueryFieldBuilder();
}
}
public Builder clear() {
super.clear();
scheme_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
if (addressBuilder_ == null) {
address_ = null;
} else {
addressBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
path_ = "";
bitField0_ = (bitField0_ & ~0x00000004);
if (queryBuilder_ == null) {
query_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000008);
} else {
queryBuilder_.clear();
}
fragment_ = "";
bitField0_ = (bitField0_ & ~0x00000010);
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_URL_descriptor;
}
public org.apache.mesos.v1.Protos.URL getDefaultInstanceForType() {
return org.apache.mesos.v1.Protos.URL.getDefaultInstance();
}
public org.apache.mesos.v1.Protos.URL build() {
org.apache.mesos.v1.Protos.URL result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.apache.mesos.v1.Protos.URL buildPartial() {
org.apache.mesos.v1.Protos.URL result = new org.apache.mesos.v1.Protos.URL(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.scheme_ = scheme_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
if (addressBuilder_ == null) {
result.address_ = address_;
} else {
result.address_ = addressBuilder_.build();
}
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.path_ = path_;
if (queryBuilder_ == null) {
if (((bitField0_ & 0x00000008) == 0x00000008)) {
query_ = java.util.Collections.unmodifiableList(query_);
bitField0_ = (bitField0_ & ~0x00000008);
}
result.query_ = query_;
} else {
result.query_ = queryBuilder_.build();
}
if (((from_bitField0_ & 0x00000010) == 0x00000010)) {
to_bitField0_ |= 0x00000008;
}
result.fragment_ = fragment_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.apache.mesos.v1.Protos.URL) {
return mergeFrom((org.apache.mesos.v1.Protos.URL)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.mesos.v1.Protos.URL other) {
if (other == org.apache.mesos.v1.Protos.URL.getDefaultInstance()) return this;
if (other.hasScheme()) {
bitField0_ |= 0x00000001;
scheme_ = other.scheme_;
onChanged();
}
if (other.hasAddress()) {
mergeAddress(other.getAddress());
}
if (other.hasPath()) {
bitField0_ |= 0x00000004;
path_ = other.path_;
onChanged();
}
if (queryBuilder_ == null) {
if (!other.query_.isEmpty()) {
if (query_.isEmpty()) {
query_ = other.query_;
bitField0_ = (bitField0_ & ~0x00000008);
} else {
ensureQueryIsMutable();
query_.addAll(other.query_);
}
onChanged();
}
} else {
if (!other.query_.isEmpty()) {
if (queryBuilder_.isEmpty()) {
queryBuilder_.dispose();
queryBuilder_ = null;
query_ = other.query_;
bitField0_ = (bitField0_ & ~0x00000008);
queryBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getQueryFieldBuilder() : null;
} else {
queryBuilder_.addAllMessages(other.query_);
}
}
}
if (other.hasFragment()) {
bitField0_ |= 0x00000010;
fragment_ = other.fragment_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
if (!hasScheme()) {
return false;
}
if (!hasAddress()) {
return false;
}
if (!getAddress().isInitialized()) {
return false;
}
for (int i = 0; i < getQueryCount(); i++) {
if (!getQuery(i).isInitialized()) {
return false;
}
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.mesos.v1.Protos.URL parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.mesos.v1.Protos.URL) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object scheme_ = "";
/**
* required string scheme = 1;
*/
public boolean hasScheme() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required string scheme = 1;
*/
public java.lang.String getScheme() {
java.lang.Object ref = scheme_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
scheme_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* required string scheme = 1;
*/
public com.google.protobuf.ByteString
getSchemeBytes() {
java.lang.Object ref = scheme_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
scheme_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* required string scheme = 1;
*/
public Builder setScheme(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
scheme_ = value;
onChanged();
return this;
}
/**
* required string scheme = 1;
*/
public Builder clearScheme() {
bitField0_ = (bitField0_ & ~0x00000001);
scheme_ = getDefaultInstance().getScheme();
onChanged();
return this;
}
/**
* required string scheme = 1;
*/
public Builder setSchemeBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
scheme_ = value;
onChanged();
return this;
}
private org.apache.mesos.v1.Protos.Address address_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
org.apache.mesos.v1.Protos.Address, org.apache.mesos.v1.Protos.Address.Builder, org.apache.mesos.v1.Protos.AddressOrBuilder> addressBuilder_;
/**
* required .mesos.v1.Address address = 2;
*/
public boolean hasAddress() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required .mesos.v1.Address address = 2;
*/
public org.apache.mesos.v1.Protos.Address getAddress() {
if (addressBuilder_ == null) {
return address_ == null ? org.apache.mesos.v1.Protos.Address.getDefaultInstance() : address_;
} else {
return addressBuilder_.getMessage();
}
}
/**
* required .mesos.v1.Address address = 2;
*/
public Builder setAddress(org.apache.mesos.v1.Protos.Address value) {
if (addressBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
address_ = value;
onChanged();
} else {
addressBuilder_.setMessage(value);
}
bitField0_ |= 0x00000002;
return this;
}
/**
* required .mesos.v1.Address address = 2;
*/
public Builder setAddress(
org.apache.mesos.v1.Protos.Address.Builder builderForValue) {
if (addressBuilder_ == null) {
address_ = builderForValue.build();
onChanged();
} else {
addressBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000002;
return this;
}
/**
* required .mesos.v1.Address address = 2;
*/
public Builder mergeAddress(org.apache.mesos.v1.Protos.Address value) {
if (addressBuilder_ == null) {
if (((bitField0_ & 0x00000002) == 0x00000002) &&
address_ != null &&
address_ != org.apache.mesos.v1.Protos.Address.getDefaultInstance()) {
address_ =
org.apache.mesos.v1.Protos.Address.newBuilder(address_).mergeFrom(value).buildPartial();
} else {
address_ = value;
}
onChanged();
} else {
addressBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000002;
return this;
}
/**
* required .mesos.v1.Address address = 2;
*/
public Builder clearAddress() {
if (addressBuilder_ == null) {
address_ = null;
onChanged();
} else {
addressBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
/**
* required .mesos.v1.Address address = 2;
*/
public org.apache.mesos.v1.Protos.Address.Builder getAddressBuilder() {
bitField0_ |= 0x00000002;
onChanged();
return getAddressFieldBuilder().getBuilder();
}
/**
* required .mesos.v1.Address address = 2;
*/
public org.apache.mesos.v1.Protos.AddressOrBuilder getAddressOrBuilder() {
if (addressBuilder_ != null) {
return addressBuilder_.getMessageOrBuilder();
} else {
return address_ == null ?
org.apache.mesos.v1.Protos.Address.getDefaultInstance() : address_;
}
}
/**
* required .mesos.v1.Address address = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.apache.mesos.v1.Protos.Address, org.apache.mesos.v1.Protos.Address.Builder, org.apache.mesos.v1.Protos.AddressOrBuilder>
getAddressFieldBuilder() {
if (addressBuilder_ == null) {
addressBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.apache.mesos.v1.Protos.Address, org.apache.mesos.v1.Protos.Address.Builder, org.apache.mesos.v1.Protos.AddressOrBuilder>(
getAddress(),
getParentForChildren(),
isClean());
address_ = null;
}
return addressBuilder_;
}
private java.lang.Object path_ = "";
/**
* optional string path = 3;
*/
public boolean hasPath() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional string path = 3;
*/
public java.lang.String getPath() {
java.lang.Object ref = path_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
path_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string path = 3;
*/
public com.google.protobuf.ByteString
getPathBytes() {
java.lang.Object ref = path_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
path_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string path = 3;
*/
public Builder setPath(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
path_ = value;
onChanged();
return this;
}
/**
* optional string path = 3;
*/
public Builder clearPath() {
bitField0_ = (bitField0_ & ~0x00000004);
path_ = getDefaultInstance().getPath();
onChanged();
return this;
}
/**
* optional string path = 3;
*/
public Builder setPathBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
path_ = value;
onChanged();
return this;
}
private java.util.List query_ =
java.util.Collections.emptyList();
private void ensureQueryIsMutable() {
if (!((bitField0_ & 0x00000008) == 0x00000008)) {
query_ = new java.util.ArrayList(query_);
bitField0_ |= 0x00000008;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.apache.mesos.v1.Protos.Parameter, org.apache.mesos.v1.Protos.Parameter.Builder, org.apache.mesos.v1.Protos.ParameterOrBuilder> queryBuilder_;
/**
* repeated .mesos.v1.Parameter query = 4;
*/
public java.util.List getQueryList() {
if (queryBuilder_ == null) {
return java.util.Collections.unmodifiableList(query_);
} else {
return queryBuilder_.getMessageList();
}
}
/**
* repeated .mesos.v1.Parameter query = 4;
*/
public int getQueryCount() {
if (queryBuilder_ == null) {
return query_.size();
} else {
return queryBuilder_.getCount();
}
}
/**
* repeated .mesos.v1.Parameter query = 4;
*/
public org.apache.mesos.v1.Protos.Parameter getQuery(int index) {
if (queryBuilder_ == null) {
return query_.get(index);
} else {
return queryBuilder_.getMessage(index);
}
}
/**
* repeated .mesos.v1.Parameter query = 4;
*/
public Builder setQuery(
int index, org.apache.mesos.v1.Protos.Parameter value) {
if (queryBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureQueryIsMutable();
query_.set(index, value);
onChanged();
} else {
queryBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .mesos.v1.Parameter query = 4;
*/
public Builder setQuery(
int index, org.apache.mesos.v1.Protos.Parameter.Builder builderForValue) {
if (queryBuilder_ == null) {
ensureQueryIsMutable();
query_.set(index, builderForValue.build());
onChanged();
} else {
queryBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .mesos.v1.Parameter query = 4;
*/
public Builder addQuery(org.apache.mesos.v1.Protos.Parameter value) {
if (queryBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureQueryIsMutable();
query_.add(value);
onChanged();
} else {
queryBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .mesos.v1.Parameter query = 4;
*/
public Builder addQuery(
int index, org.apache.mesos.v1.Protos.Parameter value) {
if (queryBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureQueryIsMutable();
query_.add(index, value);
onChanged();
} else {
queryBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .mesos.v1.Parameter query = 4;
*/
public Builder addQuery(
org.apache.mesos.v1.Protos.Parameter.Builder builderForValue) {
if (queryBuilder_ == null) {
ensureQueryIsMutable();
query_.add(builderForValue.build());
onChanged();
} else {
queryBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .mesos.v1.Parameter query = 4;
*/
public Builder addQuery(
int index, org.apache.mesos.v1.Protos.Parameter.Builder builderForValue) {
if (queryBuilder_ == null) {
ensureQueryIsMutable();
query_.add(index, builderForValue.build());
onChanged();
} else {
queryBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .mesos.v1.Parameter query = 4;
*/
public Builder addAllQuery(
java.lang.Iterable extends org.apache.mesos.v1.Protos.Parameter> values) {
if (queryBuilder_ == null) {
ensureQueryIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, query_);
onChanged();
} else {
queryBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .mesos.v1.Parameter query = 4;
*/
public Builder clearQuery() {
if (queryBuilder_ == null) {
query_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000008);
onChanged();
} else {
queryBuilder_.clear();
}
return this;
}
/**
* repeated .mesos.v1.Parameter query = 4;
*/
public Builder removeQuery(int index) {
if (queryBuilder_ == null) {
ensureQueryIsMutable();
query_.remove(index);
onChanged();
} else {
queryBuilder_.remove(index);
}
return this;
}
/**
* repeated .mesos.v1.Parameter query = 4;
*/
public org.apache.mesos.v1.Protos.Parameter.Builder getQueryBuilder(
int index) {
return getQueryFieldBuilder().getBuilder(index);
}
/**
* repeated .mesos.v1.Parameter query = 4;
*/
public org.apache.mesos.v1.Protos.ParameterOrBuilder getQueryOrBuilder(
int index) {
if (queryBuilder_ == null) {
return query_.get(index); } else {
return queryBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .mesos.v1.Parameter query = 4;
*/
public java.util.List extends org.apache.mesos.v1.Protos.ParameterOrBuilder>
getQueryOrBuilderList() {
if (queryBuilder_ != null) {
return queryBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(query_);
}
}
/**
* repeated .mesos.v1.Parameter query = 4;
*/
public org.apache.mesos.v1.Protos.Parameter.Builder addQueryBuilder() {
return getQueryFieldBuilder().addBuilder(
org.apache.mesos.v1.Protos.Parameter.getDefaultInstance());
}
/**
* repeated .mesos.v1.Parameter query = 4;
*/
public org.apache.mesos.v1.Protos.Parameter.Builder addQueryBuilder(
int index) {
return getQueryFieldBuilder().addBuilder(
index, org.apache.mesos.v1.Protos.Parameter.getDefaultInstance());
}
/**
* repeated .mesos.v1.Parameter query = 4;
*/
public java.util.List
getQueryBuilderList() {
return getQueryFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.apache.mesos.v1.Protos.Parameter, org.apache.mesos.v1.Protos.Parameter.Builder, org.apache.mesos.v1.Protos.ParameterOrBuilder>
getQueryFieldBuilder() {
if (queryBuilder_ == null) {
queryBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
org.apache.mesos.v1.Protos.Parameter, org.apache.mesos.v1.Protos.Parameter.Builder, org.apache.mesos.v1.Protos.ParameterOrBuilder>(
query_,
((bitField0_ & 0x00000008) == 0x00000008),
getParentForChildren(),
isClean());
query_ = null;
}
return queryBuilder_;
}
private java.lang.Object fragment_ = "";
/**
* optional string fragment = 5;
*/
public boolean hasFragment() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional string fragment = 5;
*/
public java.lang.String getFragment() {
java.lang.Object ref = fragment_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
fragment_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string fragment = 5;
*/
public com.google.protobuf.ByteString
getFragmentBytes() {
java.lang.Object ref = fragment_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
fragment_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string fragment = 5;
*/
public Builder setFragment(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000010;
fragment_ = value;
onChanged();
return this;
}
/**
* optional string fragment = 5;
*/
public Builder clearFragment() {
bitField0_ = (bitField0_ & ~0x00000010);
fragment_ = getDefaultInstance().getFragment();
onChanged();
return this;
}
/**
* optional string fragment = 5;
*/
public Builder setFragmentBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000010;
fragment_ = value;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:mesos.v1.URL)
}
// @@protoc_insertion_point(class_scope:mesos.v1.URL)
private static final org.apache.mesos.v1.Protos.URL DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.apache.mesos.v1.Protos.URL();
}
public static org.apache.mesos.v1.Protos.URL getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public URL parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new URL(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.apache.mesos.v1.Protos.URL getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface UnavailabilityOrBuilder extends
// @@protoc_insertion_point(interface_extends:mesos.v1.Unavailability)
com.google.protobuf.MessageOrBuilder {
/**
* required .mesos.v1.TimeInfo start = 1;
*/
boolean hasStart();
/**
* required .mesos.v1.TimeInfo start = 1;
*/
org.apache.mesos.v1.Protos.TimeInfo getStart();
/**
* required .mesos.v1.TimeInfo start = 1;
*/
org.apache.mesos.v1.Protos.TimeInfoOrBuilder getStartOrBuilder();
/**
*
* When added to `start`, this represents the end of the interval.
* If unspecified, the duration is assumed to be infinite.
*
*
* optional .mesos.v1.DurationInfo duration = 2;
*/
boolean hasDuration();
/**
*
* When added to `start`, this represents the end of the interval.
* If unspecified, the duration is assumed to be infinite.
*
*
* optional .mesos.v1.DurationInfo duration = 2;
*/
org.apache.mesos.v1.Protos.DurationInfo getDuration();
/**
*
* When added to `start`, this represents the end of the interval.
* If unspecified, the duration is assumed to be infinite.
*
*
* optional .mesos.v1.DurationInfo duration = 2;
*/
org.apache.mesos.v1.Protos.DurationInfoOrBuilder getDurationOrBuilder();
}
/**
*
**
* Represents an interval, from a given start time over a given duration.
* This interval pertains to an unavailability event, such as maintenance,
* and is not a generic interval.
*
*
* Protobuf type {@code mesos.v1.Unavailability}
*/
public static final class Unavailability extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:mesos.v1.Unavailability)
UnavailabilityOrBuilder {
private static final long serialVersionUID = 0L;
// Use Unavailability.newBuilder() to construct.
private Unavailability(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Unavailability() {
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Unavailability(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
org.apache.mesos.v1.Protos.TimeInfo.Builder subBuilder = null;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
subBuilder = start_.toBuilder();
}
start_ = input.readMessage(org.apache.mesos.v1.Protos.TimeInfo.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(start_);
start_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000001;
break;
}
case 18: {
org.apache.mesos.v1.Protos.DurationInfo.Builder subBuilder = null;
if (((bitField0_ & 0x00000002) == 0x00000002)) {
subBuilder = duration_.toBuilder();
}
duration_ = input.readMessage(org.apache.mesos.v1.Protos.DurationInfo.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(duration_);
duration_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000002;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_Unavailability_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_Unavailability_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.mesos.v1.Protos.Unavailability.class, org.apache.mesos.v1.Protos.Unavailability.Builder.class);
}
private int bitField0_;
public static final int START_FIELD_NUMBER = 1;
private org.apache.mesos.v1.Protos.TimeInfo start_;
/**
* required .mesos.v1.TimeInfo start = 1;
*/
public boolean hasStart() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required .mesos.v1.TimeInfo start = 1;
*/
public org.apache.mesos.v1.Protos.TimeInfo getStart() {
return start_ == null ? org.apache.mesos.v1.Protos.TimeInfo.getDefaultInstance() : start_;
}
/**
* required .mesos.v1.TimeInfo start = 1;
*/
public org.apache.mesos.v1.Protos.TimeInfoOrBuilder getStartOrBuilder() {
return start_ == null ? org.apache.mesos.v1.Protos.TimeInfo.getDefaultInstance() : start_;
}
public static final int DURATION_FIELD_NUMBER = 2;
private org.apache.mesos.v1.Protos.DurationInfo duration_;
/**
*
* When added to `start`, this represents the end of the interval.
* If unspecified, the duration is assumed to be infinite.
*
*
* optional .mesos.v1.DurationInfo duration = 2;
*/
public boolean hasDuration() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
*
* When added to `start`, this represents the end of the interval.
* If unspecified, the duration is assumed to be infinite.
*
*
* optional .mesos.v1.DurationInfo duration = 2;
*/
public org.apache.mesos.v1.Protos.DurationInfo getDuration() {
return duration_ == null ? org.apache.mesos.v1.Protos.DurationInfo.getDefaultInstance() : duration_;
}
/**
*
* When added to `start`, this represents the end of the interval.
* If unspecified, the duration is assumed to be infinite.
*
*
* optional .mesos.v1.DurationInfo duration = 2;
*/
public org.apache.mesos.v1.Protos.DurationInfoOrBuilder getDurationOrBuilder() {
return duration_ == null ? org.apache.mesos.v1.Protos.DurationInfo.getDefaultInstance() : duration_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasStart()) {
memoizedIsInitialized = 0;
return false;
}
if (!getStart().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
if (hasDuration()) {
if (!getDuration().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeMessage(1, getStart());
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeMessage(2, getDuration());
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getStart());
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getDuration());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.mesos.v1.Protos.Unavailability)) {
return super.equals(obj);
}
org.apache.mesos.v1.Protos.Unavailability other = (org.apache.mesos.v1.Protos.Unavailability) obj;
boolean result = true;
result = result && (hasStart() == other.hasStart());
if (hasStart()) {
result = result && getStart()
.equals(other.getStart());
}
result = result && (hasDuration() == other.hasDuration());
if (hasDuration()) {
result = result && getDuration()
.equals(other.getDuration());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasStart()) {
hash = (37 * hash) + START_FIELD_NUMBER;
hash = (53 * hash) + getStart().hashCode();
}
if (hasDuration()) {
hash = (37 * hash) + DURATION_FIELD_NUMBER;
hash = (53 * hash) + getDuration().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.mesos.v1.Protos.Unavailability parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.Unavailability parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.Unavailability parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.Unavailability parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.Unavailability parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.Unavailability parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.Unavailability parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.Unavailability parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.Unavailability parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.Unavailability parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.Unavailability parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.Unavailability parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.apache.mesos.v1.Protos.Unavailability prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
**
* Represents an interval, from a given start time over a given duration.
* This interval pertains to an unavailability event, such as maintenance,
* and is not a generic interval.
*
*
* Protobuf type {@code mesos.v1.Unavailability}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:mesos.v1.Unavailability)
org.apache.mesos.v1.Protos.UnavailabilityOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_Unavailability_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_Unavailability_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.mesos.v1.Protos.Unavailability.class, org.apache.mesos.v1.Protos.Unavailability.Builder.class);
}
// Construct using org.apache.mesos.v1.Protos.Unavailability.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getStartFieldBuilder();
getDurationFieldBuilder();
}
}
public Builder clear() {
super.clear();
if (startBuilder_ == null) {
start_ = null;
} else {
startBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
if (durationBuilder_ == null) {
duration_ = null;
} else {
durationBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_Unavailability_descriptor;
}
public org.apache.mesos.v1.Protos.Unavailability getDefaultInstanceForType() {
return org.apache.mesos.v1.Protos.Unavailability.getDefaultInstance();
}
public org.apache.mesos.v1.Protos.Unavailability build() {
org.apache.mesos.v1.Protos.Unavailability result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.apache.mesos.v1.Protos.Unavailability buildPartial() {
org.apache.mesos.v1.Protos.Unavailability result = new org.apache.mesos.v1.Protos.Unavailability(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
if (startBuilder_ == null) {
result.start_ = start_;
} else {
result.start_ = startBuilder_.build();
}
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
if (durationBuilder_ == null) {
result.duration_ = duration_;
} else {
result.duration_ = durationBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.apache.mesos.v1.Protos.Unavailability) {
return mergeFrom((org.apache.mesos.v1.Protos.Unavailability)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.mesos.v1.Protos.Unavailability other) {
if (other == org.apache.mesos.v1.Protos.Unavailability.getDefaultInstance()) return this;
if (other.hasStart()) {
mergeStart(other.getStart());
}
if (other.hasDuration()) {
mergeDuration(other.getDuration());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
if (!hasStart()) {
return false;
}
if (!getStart().isInitialized()) {
return false;
}
if (hasDuration()) {
if (!getDuration().isInitialized()) {
return false;
}
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.mesos.v1.Protos.Unavailability parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.mesos.v1.Protos.Unavailability) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private org.apache.mesos.v1.Protos.TimeInfo start_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
org.apache.mesos.v1.Protos.TimeInfo, org.apache.mesos.v1.Protos.TimeInfo.Builder, org.apache.mesos.v1.Protos.TimeInfoOrBuilder> startBuilder_;
/**
* required .mesos.v1.TimeInfo start = 1;
*/
public boolean hasStart() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required .mesos.v1.TimeInfo start = 1;
*/
public org.apache.mesos.v1.Protos.TimeInfo getStart() {
if (startBuilder_ == null) {
return start_ == null ? org.apache.mesos.v1.Protos.TimeInfo.getDefaultInstance() : start_;
} else {
return startBuilder_.getMessage();
}
}
/**
* required .mesos.v1.TimeInfo start = 1;
*/
public Builder setStart(org.apache.mesos.v1.Protos.TimeInfo value) {
if (startBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
start_ = value;
onChanged();
} else {
startBuilder_.setMessage(value);
}
bitField0_ |= 0x00000001;
return this;
}
/**
* required .mesos.v1.TimeInfo start = 1;
*/
public Builder setStart(
org.apache.mesos.v1.Protos.TimeInfo.Builder builderForValue) {
if (startBuilder_ == null) {
start_ = builderForValue.build();
onChanged();
} else {
startBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000001;
return this;
}
/**
* required .mesos.v1.TimeInfo start = 1;
*/
public Builder mergeStart(org.apache.mesos.v1.Protos.TimeInfo value) {
if (startBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001) &&
start_ != null &&
start_ != org.apache.mesos.v1.Protos.TimeInfo.getDefaultInstance()) {
start_ =
org.apache.mesos.v1.Protos.TimeInfo.newBuilder(start_).mergeFrom(value).buildPartial();
} else {
start_ = value;
}
onChanged();
} else {
startBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000001;
return this;
}
/**
* required .mesos.v1.TimeInfo start = 1;
*/
public Builder clearStart() {
if (startBuilder_ == null) {
start_ = null;
onChanged();
} else {
startBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
/**
* required .mesos.v1.TimeInfo start = 1;
*/
public org.apache.mesos.v1.Protos.TimeInfo.Builder getStartBuilder() {
bitField0_ |= 0x00000001;
onChanged();
return getStartFieldBuilder().getBuilder();
}
/**
* required .mesos.v1.TimeInfo start = 1;
*/
public org.apache.mesos.v1.Protos.TimeInfoOrBuilder getStartOrBuilder() {
if (startBuilder_ != null) {
return startBuilder_.getMessageOrBuilder();
} else {
return start_ == null ?
org.apache.mesos.v1.Protos.TimeInfo.getDefaultInstance() : start_;
}
}
/**
* required .mesos.v1.TimeInfo start = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.apache.mesos.v1.Protos.TimeInfo, org.apache.mesos.v1.Protos.TimeInfo.Builder, org.apache.mesos.v1.Protos.TimeInfoOrBuilder>
getStartFieldBuilder() {
if (startBuilder_ == null) {
startBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.apache.mesos.v1.Protos.TimeInfo, org.apache.mesos.v1.Protos.TimeInfo.Builder, org.apache.mesos.v1.Protos.TimeInfoOrBuilder>(
getStart(),
getParentForChildren(),
isClean());
start_ = null;
}
return startBuilder_;
}
private org.apache.mesos.v1.Protos.DurationInfo duration_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
org.apache.mesos.v1.Protos.DurationInfo, org.apache.mesos.v1.Protos.DurationInfo.Builder, org.apache.mesos.v1.Protos.DurationInfoOrBuilder> durationBuilder_;
/**
*
* When added to `start`, this represents the end of the interval.
* If unspecified, the duration is assumed to be infinite.
*
*
* optional .mesos.v1.DurationInfo duration = 2;
*/
public boolean hasDuration() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
*
* When added to `start`, this represents the end of the interval.
* If unspecified, the duration is assumed to be infinite.
*
*
* optional .mesos.v1.DurationInfo duration = 2;
*/
public org.apache.mesos.v1.Protos.DurationInfo getDuration() {
if (durationBuilder_ == null) {
return duration_ == null ? org.apache.mesos.v1.Protos.DurationInfo.getDefaultInstance() : duration_;
} else {
return durationBuilder_.getMessage();
}
}
/**
*
* When added to `start`, this represents the end of the interval.
* If unspecified, the duration is assumed to be infinite.
*
*
* optional .mesos.v1.DurationInfo duration = 2;
*/
public Builder setDuration(org.apache.mesos.v1.Protos.DurationInfo value) {
if (durationBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
duration_ = value;
onChanged();
} else {
durationBuilder_.setMessage(value);
}
bitField0_ |= 0x00000002;
return this;
}
/**
*
* When added to `start`, this represents the end of the interval.
* If unspecified, the duration is assumed to be infinite.
*
*
* optional .mesos.v1.DurationInfo duration = 2;
*/
public Builder setDuration(
org.apache.mesos.v1.Protos.DurationInfo.Builder builderForValue) {
if (durationBuilder_ == null) {
duration_ = builderForValue.build();
onChanged();
} else {
durationBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000002;
return this;
}
/**
*
* When added to `start`, this represents the end of the interval.
* If unspecified, the duration is assumed to be infinite.
*
*
* optional .mesos.v1.DurationInfo duration = 2;
*/
public Builder mergeDuration(org.apache.mesos.v1.Protos.DurationInfo value) {
if (durationBuilder_ == null) {
if (((bitField0_ & 0x00000002) == 0x00000002) &&
duration_ != null &&
duration_ != org.apache.mesos.v1.Protos.DurationInfo.getDefaultInstance()) {
duration_ =
org.apache.mesos.v1.Protos.DurationInfo.newBuilder(duration_).mergeFrom(value).buildPartial();
} else {
duration_ = value;
}
onChanged();
} else {
durationBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000002;
return this;
}
/**
*
* When added to `start`, this represents the end of the interval.
* If unspecified, the duration is assumed to be infinite.
*
*
* optional .mesos.v1.DurationInfo duration = 2;
*/
public Builder clearDuration() {
if (durationBuilder_ == null) {
duration_ = null;
onChanged();
} else {
durationBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
/**
*
* When added to `start`, this represents the end of the interval.
* If unspecified, the duration is assumed to be infinite.
*
*
* optional .mesos.v1.DurationInfo duration = 2;
*/
public org.apache.mesos.v1.Protos.DurationInfo.Builder getDurationBuilder() {
bitField0_ |= 0x00000002;
onChanged();
return getDurationFieldBuilder().getBuilder();
}
/**
*
* When added to `start`, this represents the end of the interval.
* If unspecified, the duration is assumed to be infinite.
*
*
* optional .mesos.v1.DurationInfo duration = 2;
*/
public org.apache.mesos.v1.Protos.DurationInfoOrBuilder getDurationOrBuilder() {
if (durationBuilder_ != null) {
return durationBuilder_.getMessageOrBuilder();
} else {
return duration_ == null ?
org.apache.mesos.v1.Protos.DurationInfo.getDefaultInstance() : duration_;
}
}
/**
*
* When added to `start`, this represents the end of the interval.
* If unspecified, the duration is assumed to be infinite.
*
*
* optional .mesos.v1.DurationInfo duration = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.apache.mesos.v1.Protos.DurationInfo, org.apache.mesos.v1.Protos.DurationInfo.Builder, org.apache.mesos.v1.Protos.DurationInfoOrBuilder>
getDurationFieldBuilder() {
if (durationBuilder_ == null) {
durationBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.apache.mesos.v1.Protos.DurationInfo, org.apache.mesos.v1.Protos.DurationInfo.Builder, org.apache.mesos.v1.Protos.DurationInfoOrBuilder>(
getDuration(),
getParentForChildren(),
isClean());
duration_ = null;
}
return durationBuilder_;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:mesos.v1.Unavailability)
}
// @@protoc_insertion_point(class_scope:mesos.v1.Unavailability)
private static final org.apache.mesos.v1.Protos.Unavailability DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.apache.mesos.v1.Protos.Unavailability();
}
public static org.apache.mesos.v1.Protos.Unavailability getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public Unavailability parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Unavailability(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.apache.mesos.v1.Protos.Unavailability getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface MachineIDOrBuilder extends
// @@protoc_insertion_point(interface_extends:mesos.v1.MachineID)
com.google.protobuf.MessageOrBuilder {
/**
* optional string hostname = 1;
*/
boolean hasHostname();
/**
* optional string hostname = 1;
*/
java.lang.String getHostname();
/**
* optional string hostname = 1;
*/
com.google.protobuf.ByteString
getHostnameBytes();
/**
* optional string ip = 2;
*/
boolean hasIp();
/**
* optional string ip = 2;
*/
java.lang.String getIp();
/**
* optional string ip = 2;
*/
com.google.protobuf.ByteString
getIpBytes();
}
/**
*
**
* Represents a single machine, which may hold one or more agents.
* NOTE: In order to match an agent to a machine, both the `hostname` and
* `ip` must match the values advertised by the agent to the master.
* Hostname is not case-sensitive.
*
*
* Protobuf type {@code mesos.v1.MachineID}
*/
public static final class MachineID extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:mesos.v1.MachineID)
MachineIDOrBuilder {
private static final long serialVersionUID = 0L;
// Use MachineID.newBuilder() to construct.
private MachineID(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private MachineID() {
hostname_ = "";
ip_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private MachineID(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000001;
hostname_ = bs;
break;
}
case 18: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000002;
ip_ = bs;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_MachineID_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_MachineID_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.mesos.v1.Protos.MachineID.class, org.apache.mesos.v1.Protos.MachineID.Builder.class);
}
private int bitField0_;
public static final int HOSTNAME_FIELD_NUMBER = 1;
private volatile java.lang.Object hostname_;
/**
* optional string hostname = 1;
*/
public boolean hasHostname() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional string hostname = 1;
*/
public java.lang.String getHostname() {
java.lang.Object ref = hostname_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
hostname_ = s;
}
return s;
}
}
/**
* optional string hostname = 1;
*/
public com.google.protobuf.ByteString
getHostnameBytes() {
java.lang.Object ref = hostname_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
hostname_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int IP_FIELD_NUMBER = 2;
private volatile java.lang.Object ip_;
/**
* optional string ip = 2;
*/
public boolean hasIp() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional string ip = 2;
*/
public java.lang.String getIp() {
java.lang.Object ref = ip_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
ip_ = s;
}
return s;
}
}
/**
* optional string ip = 2;
*/
public com.google.protobuf.ByteString
getIpBytes() {
java.lang.Object ref = ip_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
ip_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, hostname_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, ip_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, hostname_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, ip_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.mesos.v1.Protos.MachineID)) {
return super.equals(obj);
}
org.apache.mesos.v1.Protos.MachineID other = (org.apache.mesos.v1.Protos.MachineID) obj;
boolean result = true;
result = result && (hasHostname() == other.hasHostname());
if (hasHostname()) {
result = result && getHostname()
.equals(other.getHostname());
}
result = result && (hasIp() == other.hasIp());
if (hasIp()) {
result = result && getIp()
.equals(other.getIp());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasHostname()) {
hash = (37 * hash) + HOSTNAME_FIELD_NUMBER;
hash = (53 * hash) + getHostname().hashCode();
}
if (hasIp()) {
hash = (37 * hash) + IP_FIELD_NUMBER;
hash = (53 * hash) + getIp().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.mesos.v1.Protos.MachineID parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.MachineID parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.MachineID parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.MachineID parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.MachineID parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.MachineID parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.MachineID parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.MachineID parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.MachineID parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.MachineID parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.MachineID parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.MachineID parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.apache.mesos.v1.Protos.MachineID prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
**
* Represents a single machine, which may hold one or more agents.
* NOTE: In order to match an agent to a machine, both the `hostname` and
* `ip` must match the values advertised by the agent to the master.
* Hostname is not case-sensitive.
*
*
* Protobuf type {@code mesos.v1.MachineID}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:mesos.v1.MachineID)
org.apache.mesos.v1.Protos.MachineIDOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_MachineID_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_MachineID_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.mesos.v1.Protos.MachineID.class, org.apache.mesos.v1.Protos.MachineID.Builder.class);
}
// Construct using org.apache.mesos.v1.Protos.MachineID.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
hostname_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
ip_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_MachineID_descriptor;
}
public org.apache.mesos.v1.Protos.MachineID getDefaultInstanceForType() {
return org.apache.mesos.v1.Protos.MachineID.getDefaultInstance();
}
public org.apache.mesos.v1.Protos.MachineID build() {
org.apache.mesos.v1.Protos.MachineID result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.apache.mesos.v1.Protos.MachineID buildPartial() {
org.apache.mesos.v1.Protos.MachineID result = new org.apache.mesos.v1.Protos.MachineID(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.hostname_ = hostname_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.ip_ = ip_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.apache.mesos.v1.Protos.MachineID) {
return mergeFrom((org.apache.mesos.v1.Protos.MachineID)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.mesos.v1.Protos.MachineID other) {
if (other == org.apache.mesos.v1.Protos.MachineID.getDefaultInstance()) return this;
if (other.hasHostname()) {
bitField0_ |= 0x00000001;
hostname_ = other.hostname_;
onChanged();
}
if (other.hasIp()) {
bitField0_ |= 0x00000002;
ip_ = other.ip_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.mesos.v1.Protos.MachineID parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.mesos.v1.Protos.MachineID) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object hostname_ = "";
/**
* optional string hostname = 1;
*/
public boolean hasHostname() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional string hostname = 1;
*/
public java.lang.String getHostname() {
java.lang.Object ref = hostname_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
hostname_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string hostname = 1;
*/
public com.google.protobuf.ByteString
getHostnameBytes() {
java.lang.Object ref = hostname_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
hostname_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string hostname = 1;
*/
public Builder setHostname(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
hostname_ = value;
onChanged();
return this;
}
/**
* optional string hostname = 1;
*/
public Builder clearHostname() {
bitField0_ = (bitField0_ & ~0x00000001);
hostname_ = getDefaultInstance().getHostname();
onChanged();
return this;
}
/**
* optional string hostname = 1;
*/
public Builder setHostnameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
hostname_ = value;
onChanged();
return this;
}
private java.lang.Object ip_ = "";
/**
* optional string ip = 2;
*/
public boolean hasIp() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional string ip = 2;
*/
public java.lang.String getIp() {
java.lang.Object ref = ip_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
ip_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string ip = 2;
*/
public com.google.protobuf.ByteString
getIpBytes() {
java.lang.Object ref = ip_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
ip_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string ip = 2;
*/
public Builder setIp(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
ip_ = value;
onChanged();
return this;
}
/**
* optional string ip = 2;
*/
public Builder clearIp() {
bitField0_ = (bitField0_ & ~0x00000002);
ip_ = getDefaultInstance().getIp();
onChanged();
return this;
}
/**
* optional string ip = 2;
*/
public Builder setIpBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
ip_ = value;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:mesos.v1.MachineID)
}
// @@protoc_insertion_point(class_scope:mesos.v1.MachineID)
private static final org.apache.mesos.v1.Protos.MachineID DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.apache.mesos.v1.Protos.MachineID();
}
public static org.apache.mesos.v1.Protos.MachineID getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public MachineID parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new MachineID(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.apache.mesos.v1.Protos.MachineID getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface MachineInfoOrBuilder extends
// @@protoc_insertion_point(interface_extends:mesos.v1.MachineInfo)
com.google.protobuf.MessageOrBuilder {
/**
* required .mesos.v1.MachineID id = 1;
*/
boolean hasId();
/**
* required .mesos.v1.MachineID id = 1;
*/
org.apache.mesos.v1.Protos.MachineID getId();
/**
* required .mesos.v1.MachineID id = 1;
*/
org.apache.mesos.v1.Protos.MachineIDOrBuilder getIdOrBuilder();
/**
* optional .mesos.v1.MachineInfo.Mode mode = 2;
*/
boolean hasMode();
/**
* optional .mesos.v1.MachineInfo.Mode mode = 2;
*/
org.apache.mesos.v1.Protos.MachineInfo.Mode getMode();
/**
*
* Signifies that the machine may be unavailable during the given interval.
* See comments in `Unavailability` and for the `unavailability` fields
* in `Offer` and `InverseOffer` for more information.
*
*
* optional .mesos.v1.Unavailability unavailability = 3;
*/
boolean hasUnavailability();
/**
*
* Signifies that the machine may be unavailable during the given interval.
* See comments in `Unavailability` and for the `unavailability` fields
* in `Offer` and `InverseOffer` for more information.
*
*
* optional .mesos.v1.Unavailability unavailability = 3;
*/
org.apache.mesos.v1.Protos.Unavailability getUnavailability();
/**
*
* Signifies that the machine may be unavailable during the given interval.
* See comments in `Unavailability` and for the `unavailability` fields
* in `Offer` and `InverseOffer` for more information.
*
*
* optional .mesos.v1.Unavailability unavailability = 3;
*/
org.apache.mesos.v1.Protos.UnavailabilityOrBuilder getUnavailabilityOrBuilder();
}
/**
*
**
* Holds information about a single machine, its `mode`, and any other
* relevant information which may affect the behavior of the machine.
*
*
* Protobuf type {@code mesos.v1.MachineInfo}
*/
public static final class MachineInfo extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:mesos.v1.MachineInfo)
MachineInfoOrBuilder {
private static final long serialVersionUID = 0L;
// Use MachineInfo.newBuilder() to construct.
private MachineInfo(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private MachineInfo() {
mode_ = 1;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private MachineInfo(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
org.apache.mesos.v1.Protos.MachineID.Builder subBuilder = null;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
subBuilder = id_.toBuilder();
}
id_ = input.readMessage(org.apache.mesos.v1.Protos.MachineID.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(id_);
id_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000001;
break;
}
case 16: {
int rawValue = input.readEnum();
org.apache.mesos.v1.Protos.MachineInfo.Mode value = org.apache.mesos.v1.Protos.MachineInfo.Mode.valueOf(rawValue);
if (value == null) {
unknownFields.mergeVarintField(2, rawValue);
} else {
bitField0_ |= 0x00000002;
mode_ = rawValue;
}
break;
}
case 26: {
org.apache.mesos.v1.Protos.Unavailability.Builder subBuilder = null;
if (((bitField0_ & 0x00000004) == 0x00000004)) {
subBuilder = unavailability_.toBuilder();
}
unavailability_ = input.readMessage(org.apache.mesos.v1.Protos.Unavailability.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(unavailability_);
unavailability_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000004;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_MachineInfo_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_MachineInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.mesos.v1.Protos.MachineInfo.class, org.apache.mesos.v1.Protos.MachineInfo.Builder.class);
}
/**
*
* Describes the several states that a machine can be in. A `Mode`
* applies to a machine and to all associated agents on the machine.
*
*
* Protobuf enum {@code mesos.v1.MachineInfo.Mode}
*/
public enum Mode
implements com.google.protobuf.ProtocolMessageEnum {
/**
*
* In this mode, a machine is behaving normally;
* offering resources, executing tasks, etc.
*
*
* UP = 1;
*/
UP(1),
/**
*
* In this mode, all agents on the machine are expected to cooperate with
* frameworks to drain resources. In general, draining is done ahead of
* a pending `unavailability`. The resources should be drained so as to
* maximize utilization prior to the maintenance but without knowingly
* violating the frameworks' requirements.
*
*
* DRAINING = 2;
*/
DRAINING(2),
/**
*
* In this mode, a machine is not running any tasks and will not offer
* any of its resources. Agents on the machine will not be allowed to
* register with the master.
*
*
* DOWN = 3;
*/
DOWN(3),
;
/**
*
* In this mode, a machine is behaving normally;
* offering resources, executing tasks, etc.
*
*
* UP = 1;
*/
public static final int UP_VALUE = 1;
/**
*
* In this mode, all agents on the machine are expected to cooperate with
* frameworks to drain resources. In general, draining is done ahead of
* a pending `unavailability`. The resources should be drained so as to
* maximize utilization prior to the maintenance but without knowingly
* violating the frameworks' requirements.
*
*
* DRAINING = 2;
*/
public static final int DRAINING_VALUE = 2;
/**
*
* In this mode, a machine is not running any tasks and will not offer
* any of its resources. Agents on the machine will not be allowed to
* register with the master.
*
*
* DOWN = 3;
*/
public static final int DOWN_VALUE = 3;
public final int getNumber() {
return value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static Mode valueOf(int value) {
return forNumber(value);
}
public static Mode forNumber(int value) {
switch (value) {
case 1: return UP;
case 2: return DRAINING;
case 3: return DOWN;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
Mode> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public Mode findValueByNumber(int number) {
return Mode.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return org.apache.mesos.v1.Protos.MachineInfo.getDescriptor().getEnumTypes().get(0);
}
private static final Mode[] VALUES = values();
public static Mode valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int value;
private Mode(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:mesos.v1.MachineInfo.Mode)
}
private int bitField0_;
public static final int ID_FIELD_NUMBER = 1;
private org.apache.mesos.v1.Protos.MachineID id_;
/**
* required .mesos.v1.MachineID id = 1;
*/
public boolean hasId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required .mesos.v1.MachineID id = 1;
*/
public org.apache.mesos.v1.Protos.MachineID getId() {
return id_ == null ? org.apache.mesos.v1.Protos.MachineID.getDefaultInstance() : id_;
}
/**
* required .mesos.v1.MachineID id = 1;
*/
public org.apache.mesos.v1.Protos.MachineIDOrBuilder getIdOrBuilder() {
return id_ == null ? org.apache.mesos.v1.Protos.MachineID.getDefaultInstance() : id_;
}
public static final int MODE_FIELD_NUMBER = 2;
private int mode_;
/**
* optional .mesos.v1.MachineInfo.Mode mode = 2;
*/
public boolean hasMode() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional .mesos.v1.MachineInfo.Mode mode = 2;
*/
public org.apache.mesos.v1.Protos.MachineInfo.Mode getMode() {
org.apache.mesos.v1.Protos.MachineInfo.Mode result = org.apache.mesos.v1.Protos.MachineInfo.Mode.valueOf(mode_);
return result == null ? org.apache.mesos.v1.Protos.MachineInfo.Mode.UP : result;
}
public static final int UNAVAILABILITY_FIELD_NUMBER = 3;
private org.apache.mesos.v1.Protos.Unavailability unavailability_;
/**
*
* Signifies that the machine may be unavailable during the given interval.
* See comments in `Unavailability` and for the `unavailability` fields
* in `Offer` and `InverseOffer` for more information.
*
*
* optional .mesos.v1.Unavailability unavailability = 3;
*/
public boolean hasUnavailability() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
*
* Signifies that the machine may be unavailable during the given interval.
* See comments in `Unavailability` and for the `unavailability` fields
* in `Offer` and `InverseOffer` for more information.
*
*
* optional .mesos.v1.Unavailability unavailability = 3;
*/
public org.apache.mesos.v1.Protos.Unavailability getUnavailability() {
return unavailability_ == null ? org.apache.mesos.v1.Protos.Unavailability.getDefaultInstance() : unavailability_;
}
/**
*
* Signifies that the machine may be unavailable during the given interval.
* See comments in `Unavailability` and for the `unavailability` fields
* in `Offer` and `InverseOffer` for more information.
*
*
* optional .mesos.v1.Unavailability unavailability = 3;
*/
public org.apache.mesos.v1.Protos.UnavailabilityOrBuilder getUnavailabilityOrBuilder() {
return unavailability_ == null ? org.apache.mesos.v1.Protos.Unavailability.getDefaultInstance() : unavailability_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasId()) {
memoizedIsInitialized = 0;
return false;
}
if (hasUnavailability()) {
if (!getUnavailability().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeMessage(1, getId());
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeEnum(2, mode_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeMessage(3, getUnavailability());
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getId());
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(2, mode_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getUnavailability());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.mesos.v1.Protos.MachineInfo)) {
return super.equals(obj);
}
org.apache.mesos.v1.Protos.MachineInfo other = (org.apache.mesos.v1.Protos.MachineInfo) obj;
boolean result = true;
result = result && (hasId() == other.hasId());
if (hasId()) {
result = result && getId()
.equals(other.getId());
}
result = result && (hasMode() == other.hasMode());
if (hasMode()) {
result = result && mode_ == other.mode_;
}
result = result && (hasUnavailability() == other.hasUnavailability());
if (hasUnavailability()) {
result = result && getUnavailability()
.equals(other.getUnavailability());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasId()) {
hash = (37 * hash) + ID_FIELD_NUMBER;
hash = (53 * hash) + getId().hashCode();
}
if (hasMode()) {
hash = (37 * hash) + MODE_FIELD_NUMBER;
hash = (53 * hash) + mode_;
}
if (hasUnavailability()) {
hash = (37 * hash) + UNAVAILABILITY_FIELD_NUMBER;
hash = (53 * hash) + getUnavailability().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.mesos.v1.Protos.MachineInfo parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.MachineInfo parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.MachineInfo parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.MachineInfo parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.MachineInfo parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.MachineInfo parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.MachineInfo parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.MachineInfo parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.MachineInfo parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.MachineInfo parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.MachineInfo parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.MachineInfo parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.apache.mesos.v1.Protos.MachineInfo prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
**
* Holds information about a single machine, its `mode`, and any other
* relevant information which may affect the behavior of the machine.
*
*
* Protobuf type {@code mesos.v1.MachineInfo}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:mesos.v1.MachineInfo)
org.apache.mesos.v1.Protos.MachineInfoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_MachineInfo_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_MachineInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.mesos.v1.Protos.MachineInfo.class, org.apache.mesos.v1.Protos.MachineInfo.Builder.class);
}
// Construct using org.apache.mesos.v1.Protos.MachineInfo.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getIdFieldBuilder();
getUnavailabilityFieldBuilder();
}
}
public Builder clear() {
super.clear();
if (idBuilder_ == null) {
id_ = null;
} else {
idBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
mode_ = 1;
bitField0_ = (bitField0_ & ~0x00000002);
if (unavailabilityBuilder_ == null) {
unavailability_ = null;
} else {
unavailabilityBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000004);
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_MachineInfo_descriptor;
}
public org.apache.mesos.v1.Protos.MachineInfo getDefaultInstanceForType() {
return org.apache.mesos.v1.Protos.MachineInfo.getDefaultInstance();
}
public org.apache.mesos.v1.Protos.MachineInfo build() {
org.apache.mesos.v1.Protos.MachineInfo result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.apache.mesos.v1.Protos.MachineInfo buildPartial() {
org.apache.mesos.v1.Protos.MachineInfo result = new org.apache.mesos.v1.Protos.MachineInfo(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
if (idBuilder_ == null) {
result.id_ = id_;
} else {
result.id_ = idBuilder_.build();
}
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.mode_ = mode_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
if (unavailabilityBuilder_ == null) {
result.unavailability_ = unavailability_;
} else {
result.unavailability_ = unavailabilityBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.apache.mesos.v1.Protos.MachineInfo) {
return mergeFrom((org.apache.mesos.v1.Protos.MachineInfo)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.mesos.v1.Protos.MachineInfo other) {
if (other == org.apache.mesos.v1.Protos.MachineInfo.getDefaultInstance()) return this;
if (other.hasId()) {
mergeId(other.getId());
}
if (other.hasMode()) {
setMode(other.getMode());
}
if (other.hasUnavailability()) {
mergeUnavailability(other.getUnavailability());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
if (!hasId()) {
return false;
}
if (hasUnavailability()) {
if (!getUnavailability().isInitialized()) {
return false;
}
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.mesos.v1.Protos.MachineInfo parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.mesos.v1.Protos.MachineInfo) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private org.apache.mesos.v1.Protos.MachineID id_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
org.apache.mesos.v1.Protos.MachineID, org.apache.mesos.v1.Protos.MachineID.Builder, org.apache.mesos.v1.Protos.MachineIDOrBuilder> idBuilder_;
/**
* required .mesos.v1.MachineID id = 1;
*/
public boolean hasId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required .mesos.v1.MachineID id = 1;
*/
public org.apache.mesos.v1.Protos.MachineID getId() {
if (idBuilder_ == null) {
return id_ == null ? org.apache.mesos.v1.Protos.MachineID.getDefaultInstance() : id_;
} else {
return idBuilder_.getMessage();
}
}
/**
* required .mesos.v1.MachineID id = 1;
*/
public Builder setId(org.apache.mesos.v1.Protos.MachineID value) {
if (idBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
id_ = value;
onChanged();
} else {
idBuilder_.setMessage(value);
}
bitField0_ |= 0x00000001;
return this;
}
/**
* required .mesos.v1.MachineID id = 1;
*/
public Builder setId(
org.apache.mesos.v1.Protos.MachineID.Builder builderForValue) {
if (idBuilder_ == null) {
id_ = builderForValue.build();
onChanged();
} else {
idBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000001;
return this;
}
/**
* required .mesos.v1.MachineID id = 1;
*/
public Builder mergeId(org.apache.mesos.v1.Protos.MachineID value) {
if (idBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001) &&
id_ != null &&
id_ != org.apache.mesos.v1.Protos.MachineID.getDefaultInstance()) {
id_ =
org.apache.mesos.v1.Protos.MachineID.newBuilder(id_).mergeFrom(value).buildPartial();
} else {
id_ = value;
}
onChanged();
} else {
idBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000001;
return this;
}
/**
* required .mesos.v1.MachineID id = 1;
*/
public Builder clearId() {
if (idBuilder_ == null) {
id_ = null;
onChanged();
} else {
idBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
/**
* required .mesos.v1.MachineID id = 1;
*/
public org.apache.mesos.v1.Protos.MachineID.Builder getIdBuilder() {
bitField0_ |= 0x00000001;
onChanged();
return getIdFieldBuilder().getBuilder();
}
/**
* required .mesos.v1.MachineID id = 1;
*/
public org.apache.mesos.v1.Protos.MachineIDOrBuilder getIdOrBuilder() {
if (idBuilder_ != null) {
return idBuilder_.getMessageOrBuilder();
} else {
return id_ == null ?
org.apache.mesos.v1.Protos.MachineID.getDefaultInstance() : id_;
}
}
/**
* required .mesos.v1.MachineID id = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.apache.mesos.v1.Protos.MachineID, org.apache.mesos.v1.Protos.MachineID.Builder, org.apache.mesos.v1.Protos.MachineIDOrBuilder>
getIdFieldBuilder() {
if (idBuilder_ == null) {
idBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.apache.mesos.v1.Protos.MachineID, org.apache.mesos.v1.Protos.MachineID.Builder, org.apache.mesos.v1.Protos.MachineIDOrBuilder>(
getId(),
getParentForChildren(),
isClean());
id_ = null;
}
return idBuilder_;
}
private int mode_ = 1;
/**
* optional .mesos.v1.MachineInfo.Mode mode = 2;
*/
public boolean hasMode() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional .mesos.v1.MachineInfo.Mode mode = 2;
*/
public org.apache.mesos.v1.Protos.MachineInfo.Mode getMode() {
org.apache.mesos.v1.Protos.MachineInfo.Mode result = org.apache.mesos.v1.Protos.MachineInfo.Mode.valueOf(mode_);
return result == null ? org.apache.mesos.v1.Protos.MachineInfo.Mode.UP : result;
}
/**
* optional .mesos.v1.MachineInfo.Mode mode = 2;
*/
public Builder setMode(org.apache.mesos.v1.Protos.MachineInfo.Mode value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
mode_ = value.getNumber();
onChanged();
return this;
}
/**
* optional .mesos.v1.MachineInfo.Mode mode = 2;
*/
public Builder clearMode() {
bitField0_ = (bitField0_ & ~0x00000002);
mode_ = 1;
onChanged();
return this;
}
private org.apache.mesos.v1.Protos.Unavailability unavailability_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
org.apache.mesos.v1.Protos.Unavailability, org.apache.mesos.v1.Protos.Unavailability.Builder, org.apache.mesos.v1.Protos.UnavailabilityOrBuilder> unavailabilityBuilder_;
/**
*
* Signifies that the machine may be unavailable during the given interval.
* See comments in `Unavailability` and for the `unavailability` fields
* in `Offer` and `InverseOffer` for more information.
*
*
* optional .mesos.v1.Unavailability unavailability = 3;
*/
public boolean hasUnavailability() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
*
* Signifies that the machine may be unavailable during the given interval.
* See comments in `Unavailability` and for the `unavailability` fields
* in `Offer` and `InverseOffer` for more information.
*
*
* optional .mesos.v1.Unavailability unavailability = 3;
*/
public org.apache.mesos.v1.Protos.Unavailability getUnavailability() {
if (unavailabilityBuilder_ == null) {
return unavailability_ == null ? org.apache.mesos.v1.Protos.Unavailability.getDefaultInstance() : unavailability_;
} else {
return unavailabilityBuilder_.getMessage();
}
}
/**
*
* Signifies that the machine may be unavailable during the given interval.
* See comments in `Unavailability` and for the `unavailability` fields
* in `Offer` and `InverseOffer` for more information.
*
*
* optional .mesos.v1.Unavailability unavailability = 3;
*/
public Builder setUnavailability(org.apache.mesos.v1.Protos.Unavailability value) {
if (unavailabilityBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
unavailability_ = value;
onChanged();
} else {
unavailabilityBuilder_.setMessage(value);
}
bitField0_ |= 0x00000004;
return this;
}
/**
*
* Signifies that the machine may be unavailable during the given interval.
* See comments in `Unavailability` and for the `unavailability` fields
* in `Offer` and `InverseOffer` for more information.
*
*
* optional .mesos.v1.Unavailability unavailability = 3;
*/
public Builder setUnavailability(
org.apache.mesos.v1.Protos.Unavailability.Builder builderForValue) {
if (unavailabilityBuilder_ == null) {
unavailability_ = builderForValue.build();
onChanged();
} else {
unavailabilityBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000004;
return this;
}
/**
*
* Signifies that the machine may be unavailable during the given interval.
* See comments in `Unavailability` and for the `unavailability` fields
* in `Offer` and `InverseOffer` for more information.
*
*
* optional .mesos.v1.Unavailability unavailability = 3;
*/
public Builder mergeUnavailability(org.apache.mesos.v1.Protos.Unavailability value) {
if (unavailabilityBuilder_ == null) {
if (((bitField0_ & 0x00000004) == 0x00000004) &&
unavailability_ != null &&
unavailability_ != org.apache.mesos.v1.Protos.Unavailability.getDefaultInstance()) {
unavailability_ =
org.apache.mesos.v1.Protos.Unavailability.newBuilder(unavailability_).mergeFrom(value).buildPartial();
} else {
unavailability_ = value;
}
onChanged();
} else {
unavailabilityBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000004;
return this;
}
/**
*
* Signifies that the machine may be unavailable during the given interval.
* See comments in `Unavailability` and for the `unavailability` fields
* in `Offer` and `InverseOffer` for more information.
*
*
* optional .mesos.v1.Unavailability unavailability = 3;
*/
public Builder clearUnavailability() {
if (unavailabilityBuilder_ == null) {
unavailability_ = null;
onChanged();
} else {
unavailabilityBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000004);
return this;
}
/**
*
* Signifies that the machine may be unavailable during the given interval.
* See comments in `Unavailability` and for the `unavailability` fields
* in `Offer` and `InverseOffer` for more information.
*
*
* optional .mesos.v1.Unavailability unavailability = 3;
*/
public org.apache.mesos.v1.Protos.Unavailability.Builder getUnavailabilityBuilder() {
bitField0_ |= 0x00000004;
onChanged();
return getUnavailabilityFieldBuilder().getBuilder();
}
/**
*
* Signifies that the machine may be unavailable during the given interval.
* See comments in `Unavailability` and for the `unavailability` fields
* in `Offer` and `InverseOffer` for more information.
*
*
* optional .mesos.v1.Unavailability unavailability = 3;
*/
public org.apache.mesos.v1.Protos.UnavailabilityOrBuilder getUnavailabilityOrBuilder() {
if (unavailabilityBuilder_ != null) {
return unavailabilityBuilder_.getMessageOrBuilder();
} else {
return unavailability_ == null ?
org.apache.mesos.v1.Protos.Unavailability.getDefaultInstance() : unavailability_;
}
}
/**
*
* Signifies that the machine may be unavailable during the given interval.
* See comments in `Unavailability` and for the `unavailability` fields
* in `Offer` and `InverseOffer` for more information.
*
*
* optional .mesos.v1.Unavailability unavailability = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.apache.mesos.v1.Protos.Unavailability, org.apache.mesos.v1.Protos.Unavailability.Builder, org.apache.mesos.v1.Protos.UnavailabilityOrBuilder>
getUnavailabilityFieldBuilder() {
if (unavailabilityBuilder_ == null) {
unavailabilityBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.apache.mesos.v1.Protos.Unavailability, org.apache.mesos.v1.Protos.Unavailability.Builder, org.apache.mesos.v1.Protos.UnavailabilityOrBuilder>(
getUnavailability(),
getParentForChildren(),
isClean());
unavailability_ = null;
}
return unavailabilityBuilder_;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:mesos.v1.MachineInfo)
}
// @@protoc_insertion_point(class_scope:mesos.v1.MachineInfo)
private static final org.apache.mesos.v1.Protos.MachineInfo DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.apache.mesos.v1.Protos.MachineInfo();
}
public static org.apache.mesos.v1.Protos.MachineInfo getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public MachineInfo parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new MachineInfo(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.apache.mesos.v1.Protos.MachineInfo getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface FrameworkInfoOrBuilder extends
// @@protoc_insertion_point(interface_extends:mesos.v1.FrameworkInfo)
com.google.protobuf.MessageOrBuilder {
/**
*
* Used to determine the Unix user that an executor or task should be
* launched as.
* When using the MesosSchedulerDriver, if the field is set to an
* empty string, it will automagically set it to the current user.
* When using the HTTP Scheduler API, the user has to be set
* explicitly.
*
*
* required string user = 1;
*/
boolean hasUser();
/**
*
* Used to determine the Unix user that an executor or task should be
* launched as.
* When using the MesosSchedulerDriver, if the field is set to an
* empty string, it will automagically set it to the current user.
* When using the HTTP Scheduler API, the user has to be set
* explicitly.
*
*
* required string user = 1;
*/
java.lang.String getUser();
/**
*
* Used to determine the Unix user that an executor or task should be
* launched as.
* When using the MesosSchedulerDriver, if the field is set to an
* empty string, it will automagically set it to the current user.
* When using the HTTP Scheduler API, the user has to be set
* explicitly.
*
*
* required string user = 1;
*/
com.google.protobuf.ByteString
getUserBytes();
/**
*
* Name of the framework that shows up in the Mesos Web UI.
*
*
* required string name = 2;
*/
boolean hasName();
/**
*
* Name of the framework that shows up in the Mesos Web UI.
*
*
* required string name = 2;
*/
java.lang.String getName();
/**
*
* Name of the framework that shows up in the Mesos Web UI.
*
*
* required string name = 2;
*/
com.google.protobuf.ByteString
getNameBytes();
/**
*
* Used to uniquely identify the framework.
* This field must be unset when the framework subscribes for the
* first time upon which the master will assign a new ID. To
* resubscribe after scheduler failover the framework should set
* 'id' to the ID assigned by the master. Setting 'id' to values
* not assigned by Mesos masters is unsupported.
*
*
* optional .mesos.v1.FrameworkID id = 3;
*/
boolean hasId();
/**
*
* Used to uniquely identify the framework.
* This field must be unset when the framework subscribes for the
* first time upon which the master will assign a new ID. To
* resubscribe after scheduler failover the framework should set
* 'id' to the ID assigned by the master. Setting 'id' to values
* not assigned by Mesos masters is unsupported.
*
*
* optional .mesos.v1.FrameworkID id = 3;
*/
org.apache.mesos.v1.Protos.FrameworkID getId();
/**
*
* Used to uniquely identify the framework.
* This field must be unset when the framework subscribes for the
* first time upon which the master will assign a new ID. To
* resubscribe after scheduler failover the framework should set
* 'id' to the ID assigned by the master. Setting 'id' to values
* not assigned by Mesos masters is unsupported.
*
*
* optional .mesos.v1.FrameworkID id = 3;
*/
org.apache.mesos.v1.Protos.FrameworkIDOrBuilder getIdOrBuilder();
/**
*
* The amount of time (in seconds) that the master will wait for the
* scheduler to failover before it tears down the framework by
* killing all its tasks/executors. This should be non-zero if a
* framework expects to reconnect after a failure and not lose its
* tasks/executors.
* NOTE: To avoid accidental destruction of tasks, production
* frameworks typically set this to a large value (e.g., 1 week).
*
*
* optional double failover_timeout = 4 [default = 0];
*/
boolean hasFailoverTimeout();
/**
*
* The amount of time (in seconds) that the master will wait for the
* scheduler to failover before it tears down the framework by
* killing all its tasks/executors. This should be non-zero if a
* framework expects to reconnect after a failure and not lose its
* tasks/executors.
* NOTE: To avoid accidental destruction of tasks, production
* frameworks typically set this to a large value (e.g., 1 week).
*
*
* optional double failover_timeout = 4 [default = 0];
*/
double getFailoverTimeout();
/**
*
* If set, agents running tasks started by this framework will write
* the framework pid, executor pids and status updates to disk. If
* the agent exits (e.g., due to a crash or as part of upgrading
* Mesos), this checkpointed data allows the restarted agent to
* reconnect to executors that were started by the old instance of
* the agent. Enabling checkpointing improves fault tolerance, at
* the cost of a (usually small) increase in disk I/O.
*
*
* optional bool checkpoint = 5 [default = false];
*/
boolean hasCheckpoint();
/**
*
* If set, agents running tasks started by this framework will write
* the framework pid, executor pids and status updates to disk. If
* the agent exits (e.g., due to a crash or as part of upgrading
* Mesos), this checkpointed data allows the restarted agent to
* reconnect to executors that were started by the old instance of
* the agent. Enabling checkpointing improves fault tolerance, at
* the cost of a (usually small) increase in disk I/O.
*
*
* optional bool checkpoint = 5 [default = false];
*/
boolean getCheckpoint();
/**
*
* Roles are the entities to which allocations are made.
* The framework must have at least one role in order to
* be offered resources. Note that `role` is deprecated
* in favor of `roles` and only one of these fields must
* be used. Since we cannot distinguish between empty
* `roles` and the default unset `role`, we require that
* frameworks set the `MULTI_ROLE` capability if
* setting the `roles` field.
*
*
* optional string role = 6 [default = "*", deprecated = true];
*/
@java.lang.Deprecated boolean hasRole();
/**
*
* Roles are the entities to which allocations are made.
* The framework must have at least one role in order to
* be offered resources. Note that `role` is deprecated
* in favor of `roles` and only one of these fields must
* be used. Since we cannot distinguish between empty
* `roles` and the default unset `role`, we require that
* frameworks set the `MULTI_ROLE` capability if
* setting the `roles` field.
*
*
* optional string role = 6 [default = "*", deprecated = true];
*/
@java.lang.Deprecated java.lang.String getRole();
/**
*
* Roles are the entities to which allocations are made.
* The framework must have at least one role in order to
* be offered resources. Note that `role` is deprecated
* in favor of `roles` and only one of these fields must
* be used. Since we cannot distinguish between empty
* `roles` and the default unset `role`, we require that
* frameworks set the `MULTI_ROLE` capability if
* setting the `roles` field.
*
*
* optional string role = 6 [default = "*", deprecated = true];
*/
@java.lang.Deprecated com.google.protobuf.ByteString
getRoleBytes();
/**
* repeated string roles = 12;
*/
java.util.List
getRolesList();
/**
* repeated string roles = 12;
*/
int getRolesCount();
/**
* repeated string roles = 12;
*/
java.lang.String getRoles(int index);
/**
* repeated string roles = 12;
*/
com.google.protobuf.ByteString
getRolesBytes(int index);
/**
*
* Used to indicate the current host from which the scheduler is
* registered in the Mesos Web UI. If set to an empty string Mesos
* will automagically set it to the current hostname if one is
* available.
*
*
* optional string hostname = 7;
*/
boolean hasHostname();
/**
*
* Used to indicate the current host from which the scheduler is
* registered in the Mesos Web UI. If set to an empty string Mesos
* will automagically set it to the current hostname if one is
* available.
*
*
* optional string hostname = 7;
*/
java.lang.String getHostname();
/**
*
* Used to indicate the current host from which the scheduler is
* registered in the Mesos Web UI. If set to an empty string Mesos
* will automagically set it to the current hostname if one is
* available.
*
*
* optional string hostname = 7;
*/
com.google.protobuf.ByteString
getHostnameBytes();
/**
*
* This field should match the credential's principal the framework
* uses for authentication. This field is used for framework API
* rate limiting and dynamic reservations. It should be set even
* if authentication is not enabled if these features are desired.
*
*
* optional string principal = 8;
*/
boolean hasPrincipal();
/**
*
* This field should match the credential's principal the framework
* uses for authentication. This field is used for framework API
* rate limiting and dynamic reservations. It should be set even
* if authentication is not enabled if these features are desired.
*
*
* optional string principal = 8;
*/
java.lang.String getPrincipal();
/**
*
* This field should match the credential's principal the framework
* uses for authentication. This field is used for framework API
* rate limiting and dynamic reservations. It should be set even
* if authentication is not enabled if these features are desired.
*
*
* optional string principal = 8;
*/
com.google.protobuf.ByteString
getPrincipalBytes();
/**
*
* This field allows a framework to advertise its web UI, so that
* the Mesos web UI can link to it. It is expected to be a full URL,
* for example http://my-scheduler.example.com:8080/.
*
*
* optional string webui_url = 9;
*/
boolean hasWebuiUrl();
/**
*
* This field allows a framework to advertise its web UI, so that
* the Mesos web UI can link to it. It is expected to be a full URL,
* for example http://my-scheduler.example.com:8080/.
*
*
* optional string webui_url = 9;
*/
java.lang.String getWebuiUrl();
/**
*
* This field allows a framework to advertise its web UI, so that
* the Mesos web UI can link to it. It is expected to be a full URL,
* for example http://my-scheduler.example.com:8080/.
*
*
* optional string webui_url = 9;
*/
com.google.protobuf.ByteString
getWebuiUrlBytes();
/**
*
* This field allows a framework to advertise its set of
* capabilities (e.g., ability to receive offers for revocable
* resources).
*
*
* repeated .mesos.v1.FrameworkInfo.Capability capabilities = 10;
*/
java.util.List
getCapabilitiesList();
/**
*
* This field allows a framework to advertise its set of
* capabilities (e.g., ability to receive offers for revocable
* resources).
*
*
* repeated .mesos.v1.FrameworkInfo.Capability capabilities = 10;
*/
org.apache.mesos.v1.Protos.FrameworkInfo.Capability getCapabilities(int index);
/**
*
* This field allows a framework to advertise its set of
* capabilities (e.g., ability to receive offers for revocable
* resources).
*
*
* repeated .mesos.v1.FrameworkInfo.Capability capabilities = 10;
*/
int getCapabilitiesCount();
/**
*
* This field allows a framework to advertise its set of
* capabilities (e.g., ability to receive offers for revocable
* resources).
*
*
* repeated .mesos.v1.FrameworkInfo.Capability capabilities = 10;
*/
java.util.List extends org.apache.mesos.v1.Protos.FrameworkInfo.CapabilityOrBuilder>
getCapabilitiesOrBuilderList();
/**
*
* This field allows a framework to advertise its set of
* capabilities (e.g., ability to receive offers for revocable
* resources).
*
*
* repeated .mesos.v1.FrameworkInfo.Capability capabilities = 10;
*/
org.apache.mesos.v1.Protos.FrameworkInfo.CapabilityOrBuilder getCapabilitiesOrBuilder(
int index);
/**
*
* Labels are free-form key value pairs supplied by the framework
* scheduler (e.g., to describe additional functionality offered by
* the framework). These labels are not interpreted by Mesos itself.
* Labels should not contain duplicate key-value pairs.
*
*
* optional .mesos.v1.Labels labels = 11;
*/
boolean hasLabels();
/**
*
* Labels are free-form key value pairs supplied by the framework
* scheduler (e.g., to describe additional functionality offered by
* the framework). These labels are not interpreted by Mesos itself.
* Labels should not contain duplicate key-value pairs.
*
*
* optional .mesos.v1.Labels labels = 11;
*/
org.apache.mesos.v1.Protos.Labels getLabels();
/**
*
* Labels are free-form key value pairs supplied by the framework
* scheduler (e.g., to describe additional functionality offered by
* the framework). These labels are not interpreted by Mesos itself.
* Labels should not contain duplicate key-value pairs.
*
*
* optional .mesos.v1.Labels labels = 11;
*/
org.apache.mesos.v1.Protos.LabelsOrBuilder getLabelsOrBuilder();
/**
*
* Specifc resource requirements for each of the framework's roles. This field
* is used by e.g., the default allocator to decide whether a framework is
* interested in seeing a resource of a certain shape.
*
*
* map<string, .mesos.v1.OfferFilters> offer_filters = 13;
*/
int getOfferFiltersCount();
/**
*
* Specifc resource requirements for each of the framework's roles. This field
* is used by e.g., the default allocator to decide whether a framework is
* interested in seeing a resource of a certain shape.
*
*
* map<string, .mesos.v1.OfferFilters> offer_filters = 13;
*/
boolean containsOfferFilters(
java.lang.String key);
/**
* Use {@link #getOfferFiltersMap()} instead.
*/
@java.lang.Deprecated
java.util.Map
getOfferFilters();
/**
*
* Specifc resource requirements for each of the framework's roles. This field
* is used by e.g., the default allocator to decide whether a framework is
* interested in seeing a resource of a certain shape.
*
*
* map<string, .mesos.v1.OfferFilters> offer_filters = 13;
*/
java.util.Map
getOfferFiltersMap();
/**
*
* Specifc resource requirements for each of the framework's roles. This field
* is used by e.g., the default allocator to decide whether a framework is
* interested in seeing a resource of a certain shape.
*
*
* map<string, .mesos.v1.OfferFilters> offer_filters = 13;
*/
org.apache.mesos.v1.Protos.OfferFilters getOfferFiltersOrDefault(
java.lang.String key,
org.apache.mesos.v1.Protos.OfferFilters defaultValue);
/**
*
* Specifc resource requirements for each of the framework's roles. This field
* is used by e.g., the default allocator to decide whether a framework is
* interested in seeing a resource of a certain shape.
*
*
* map<string, .mesos.v1.OfferFilters> offer_filters = 13;
*/
org.apache.mesos.v1.Protos.OfferFilters getOfferFiltersOrThrow(
java.lang.String key);
}
/**
*
**
* Describes a framework.
*
*
* Protobuf type {@code mesos.v1.FrameworkInfo}
*/
public static final class FrameworkInfo extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:mesos.v1.FrameworkInfo)
FrameworkInfoOrBuilder {
private static final long serialVersionUID = 0L;
// Use FrameworkInfo.newBuilder() to construct.
private FrameworkInfo(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private FrameworkInfo() {
user_ = "";
name_ = "";
failoverTimeout_ = 0D;
checkpoint_ = false;
role_ = "*";
roles_ = com.google.protobuf.LazyStringArrayList.EMPTY;
hostname_ = "";
principal_ = "";
webuiUrl_ = "";
capabilities_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private FrameworkInfo(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000001;
user_ = bs;
break;
}
case 18: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000002;
name_ = bs;
break;
}
case 26: {
org.apache.mesos.v1.Protos.FrameworkID.Builder subBuilder = null;
if (((bitField0_ & 0x00000004) == 0x00000004)) {
subBuilder = id_.toBuilder();
}
id_ = input.readMessage(org.apache.mesos.v1.Protos.FrameworkID.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(id_);
id_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000004;
break;
}
case 33: {
bitField0_ |= 0x00000008;
failoverTimeout_ = input.readDouble();
break;
}
case 40: {
bitField0_ |= 0x00000010;
checkpoint_ = input.readBool();
break;
}
case 50: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000020;
role_ = bs;
break;
}
case 58: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000040;
hostname_ = bs;
break;
}
case 66: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000080;
principal_ = bs;
break;
}
case 74: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000100;
webuiUrl_ = bs;
break;
}
case 82: {
if (!((mutable_bitField0_ & 0x00000400) == 0x00000400)) {
capabilities_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000400;
}
capabilities_.add(
input.readMessage(org.apache.mesos.v1.Protos.FrameworkInfo.Capability.PARSER, extensionRegistry));
break;
}
case 90: {
org.apache.mesos.v1.Protos.Labels.Builder subBuilder = null;
if (((bitField0_ & 0x00000200) == 0x00000200)) {
subBuilder = labels_.toBuilder();
}
labels_ = input.readMessage(org.apache.mesos.v1.Protos.Labels.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(labels_);
labels_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000200;
break;
}
case 98: {
com.google.protobuf.ByteString bs = input.readBytes();
if (!((mutable_bitField0_ & 0x00000040) == 0x00000040)) {
roles_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000040;
}
roles_.add(bs);
break;
}
case 106: {
if (!((mutable_bitField0_ & 0x00001000) == 0x00001000)) {
offerFilters_ = com.google.protobuf.MapField.newMapField(
OfferFiltersDefaultEntryHolder.defaultEntry);
mutable_bitField0_ |= 0x00001000;
}
com.google.protobuf.MapEntry
offerFilters__ = input.readMessage(
OfferFiltersDefaultEntryHolder.defaultEntry.getParserForType(), extensionRegistry);
offerFilters_.getMutableMap().put(
offerFilters__.getKey(), offerFilters__.getValue());
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000400) == 0x00000400)) {
capabilities_ = java.util.Collections.unmodifiableList(capabilities_);
}
if (((mutable_bitField0_ & 0x00000040) == 0x00000040)) {
roles_ = roles_.getUnmodifiableView();
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_FrameworkInfo_descriptor;
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapField internalGetMapField(
int number) {
switch (number) {
case 13:
return internalGetOfferFilters();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_FrameworkInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.mesos.v1.Protos.FrameworkInfo.class, org.apache.mesos.v1.Protos.FrameworkInfo.Builder.class);
}
public interface CapabilityOrBuilder extends
// @@protoc_insertion_point(interface_extends:mesos.v1.FrameworkInfo.Capability)
com.google.protobuf.MessageOrBuilder {
/**
*
* Enum fields should be optional, see: MESOS-4997.
*
*
* optional .mesos.v1.FrameworkInfo.Capability.Type type = 1;
*/
boolean hasType();
/**
*
* Enum fields should be optional, see: MESOS-4997.
*
*
* optional .mesos.v1.FrameworkInfo.Capability.Type type = 1;
*/
org.apache.mesos.v1.Protos.FrameworkInfo.Capability.Type getType();
}
/**
* Protobuf type {@code mesos.v1.FrameworkInfo.Capability}
*/
public static final class Capability extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:mesos.v1.FrameworkInfo.Capability)
CapabilityOrBuilder {
private static final long serialVersionUID = 0L;
// Use Capability.newBuilder() to construct.
private Capability(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Capability() {
type_ = 0;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Capability(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
int rawValue = input.readEnum();
org.apache.mesos.v1.Protos.FrameworkInfo.Capability.Type value = org.apache.mesos.v1.Protos.FrameworkInfo.Capability.Type.valueOf(rawValue);
if (value == null) {
unknownFields.mergeVarintField(1, rawValue);
} else {
bitField0_ |= 0x00000001;
type_ = rawValue;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_FrameworkInfo_Capability_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_FrameworkInfo_Capability_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.mesos.v1.Protos.FrameworkInfo.Capability.class, org.apache.mesos.v1.Protos.FrameworkInfo.Capability.Builder.class);
}
/**
* Protobuf enum {@code mesos.v1.FrameworkInfo.Capability.Type}
*/
public enum Type
implements com.google.protobuf.ProtocolMessageEnum {
/**
*
* This must be the first enum value in this list, to
* ensure that if 'type' is not set, the default value
* is UNKNOWN. This enables enum values to be added
* in a backwards-compatible way. See: MESOS-4997.
*
*
* UNKNOWN = 0;
*/
UNKNOWN(0),
/**
*
* Receive offers with revocable resources. See 'Resource'
* message for details.
*
*
* REVOCABLE_RESOURCES = 1;
*/
REVOCABLE_RESOURCES(1),
/**
*
* Receive the TASK_KILLING TaskState when a task is being
* killed by an executor. The executor will examine this
* capability to determine whether it can send TASK_KILLING.
*
*
* TASK_KILLING_STATE = 2;
*/
TASK_KILLING_STATE(2),
/**
*
* Indicates whether the framework is aware of GPU resources.
* Frameworks that are aware of GPU resources are expected to
* avoid placing non-GPU workloads on GPU agents, in order
* to avoid occupying a GPU agent and preventing GPU workloads
* from running! Currently, if a framework is unaware of GPU
* resources, it will not be offered *any* of the resources on
* an agent with GPUs. This restriction is in place because we
* do not have a revocation mechanism that ensures GPU workloads
* can evict GPU agent occupants if necessary.
* TODO(bmahler): As we add revocation we can relax the
* restriction here. See MESOS-5634 for more information.
*
*
* GPU_RESOURCES = 3;
*/
GPU_RESOURCES(3),
/**
*
* Receive offers with resources that are shared.
*
*
* SHARED_RESOURCES = 4;
*/
SHARED_RESOURCES(4),
/**
*
* Indicates that (1) the framework is prepared to handle the
* following TaskStates: TASK_UNREACHABLE, TASK_DROPPED,
* TASK_GONE, TASK_GONE_BY_OPERATOR, and TASK_UNKNOWN, and (2)
* the framework will assume responsibility for managing
* partitioned tasks that reregister with the master.
* Frameworks that enable this capability can define how they
* would like to handle partitioned tasks. Frameworks will
* receive TASK_UNREACHABLE for tasks on agents that are
* partitioned from the master.
* Without this capability, frameworks will receive TASK_LOST
* for tasks on partitioned agents.
* NOTE: Prior to Mesos 1.5, such tasks will be killed by Mesos
* when the agent reregisters (unless the master has failed over).
* However due to the lack of benefit in maintaining different
* behaviors depending on whether the master has failed over
* (see MESOS-7215), as of 1.5, Mesos will not kill these
* tasks in either case.
*
*
* PARTITION_AWARE = 5;
*/
PARTITION_AWARE(5),
/**
*
* This expresses the ability for the framework to be
* "multi-tenant" via using the newly introduced `roles`
* field, and examining `Offer.allocation_info` to determine
* which role the offers are being made to. We also
* expect that "single-tenant" schedulers eventually
* provide this and move away from the deprecated
* `role` field.
*
*
* MULTI_ROLE = 6;
*/
MULTI_ROLE(6),
/**
*
* This capability has two effects for a framework.
* (1) The framework is offered resources in a new format.
* The offered resources have the `Resource.reservations` field set
* rather than `Resource.role` and `Resource.reservation`. In short,
* an empty `reservations` field denotes unreserved resources, and
* each `ReservationInfo` in the `reservations` field denotes a
* reservation that refines the previous one.
* See the 'Resource Format' section for more details.
* (2) The framework can create refined reservations.
* A framework can refine an existing reservation via the
* `Resource.reservations` field. For example, a reservation for role
* `eng` can be refined to `eng/front_end`.
* See `ReservationInfo.reservations` for more details.
* NOTE: Without this capability, a framework is not offered resources
* that have refined reservations. A resource is said to have refined
* reservations if it uses the `Resource.reservations` field, and
* `Resource.reservations_size() > 1`.
*
*
* RESERVATION_REFINEMENT = 7;
*/
RESERVATION_REFINEMENT(7),
/**
*
* Indicates that the framework is prepared to receive offers
* for agents whose region is different from the master's
* region. Network links between hosts in different regions
* typically have higher latency and lower bandwidth than
* network links within a region, so frameworks should be
* careful to only place suitable workloads in remote regions.
* Frameworks that are not region-aware will never receive
* offers for remote agents; region-aware frameworks are assumed
* to implement their own logic to decide which workloads (if
* any) are suitable for placement on remote agents.
*
*
* REGION_AWARE = 8;
*/
REGION_AWARE(8),
;
/**
*
* This must be the first enum value in this list, to
* ensure that if 'type' is not set, the default value
* is UNKNOWN. This enables enum values to be added
* in a backwards-compatible way. See: MESOS-4997.
*
*
* UNKNOWN = 0;
*/
public static final int UNKNOWN_VALUE = 0;
/**
*
* Receive offers with revocable resources. See 'Resource'
* message for details.
*
*
* REVOCABLE_RESOURCES = 1;
*/
public static final int REVOCABLE_RESOURCES_VALUE = 1;
/**
*
* Receive the TASK_KILLING TaskState when a task is being
* killed by an executor. The executor will examine this
* capability to determine whether it can send TASK_KILLING.
*
*
* TASK_KILLING_STATE = 2;
*/
public static final int TASK_KILLING_STATE_VALUE = 2;
/**
*
* Indicates whether the framework is aware of GPU resources.
* Frameworks that are aware of GPU resources are expected to
* avoid placing non-GPU workloads on GPU agents, in order
* to avoid occupying a GPU agent and preventing GPU workloads
* from running! Currently, if a framework is unaware of GPU
* resources, it will not be offered *any* of the resources on
* an agent with GPUs. This restriction is in place because we
* do not have a revocation mechanism that ensures GPU workloads
* can evict GPU agent occupants if necessary.
* TODO(bmahler): As we add revocation we can relax the
* restriction here. See MESOS-5634 for more information.
*
*
* GPU_RESOURCES = 3;
*/
public static final int GPU_RESOURCES_VALUE = 3;
/**
*
* Receive offers with resources that are shared.
*
*
* SHARED_RESOURCES = 4;
*/
public static final int SHARED_RESOURCES_VALUE = 4;
/**
*
* Indicates that (1) the framework is prepared to handle the
* following TaskStates: TASK_UNREACHABLE, TASK_DROPPED,
* TASK_GONE, TASK_GONE_BY_OPERATOR, and TASK_UNKNOWN, and (2)
* the framework will assume responsibility for managing
* partitioned tasks that reregister with the master.
* Frameworks that enable this capability can define how they
* would like to handle partitioned tasks. Frameworks will
* receive TASK_UNREACHABLE for tasks on agents that are
* partitioned from the master.
* Without this capability, frameworks will receive TASK_LOST
* for tasks on partitioned agents.
* NOTE: Prior to Mesos 1.5, such tasks will be killed by Mesos
* when the agent reregisters (unless the master has failed over).
* However due to the lack of benefit in maintaining different
* behaviors depending on whether the master has failed over
* (see MESOS-7215), as of 1.5, Mesos will not kill these
* tasks in either case.
*
*
* PARTITION_AWARE = 5;
*/
public static final int PARTITION_AWARE_VALUE = 5;
/**
*
* This expresses the ability for the framework to be
* "multi-tenant" via using the newly introduced `roles`
* field, and examining `Offer.allocation_info` to determine
* which role the offers are being made to. We also
* expect that "single-tenant" schedulers eventually
* provide this and move away from the deprecated
* `role` field.
*
*
* MULTI_ROLE = 6;
*/
public static final int MULTI_ROLE_VALUE = 6;
/**
*
* This capability has two effects for a framework.
* (1) The framework is offered resources in a new format.
* The offered resources have the `Resource.reservations` field set
* rather than `Resource.role` and `Resource.reservation`. In short,
* an empty `reservations` field denotes unreserved resources, and
* each `ReservationInfo` in the `reservations` field denotes a
* reservation that refines the previous one.
* See the 'Resource Format' section for more details.
* (2) The framework can create refined reservations.
* A framework can refine an existing reservation via the
* `Resource.reservations` field. For example, a reservation for role
* `eng` can be refined to `eng/front_end`.
* See `ReservationInfo.reservations` for more details.
* NOTE: Without this capability, a framework is not offered resources
* that have refined reservations. A resource is said to have refined
* reservations if it uses the `Resource.reservations` field, and
* `Resource.reservations_size() > 1`.
*
*
* RESERVATION_REFINEMENT = 7;
*/
public static final int RESERVATION_REFINEMENT_VALUE = 7;
/**
*
* Indicates that the framework is prepared to receive offers
* for agents whose region is different from the master's
* region. Network links between hosts in different regions
* typically have higher latency and lower bandwidth than
* network links within a region, so frameworks should be
* careful to only place suitable workloads in remote regions.
* Frameworks that are not region-aware will never receive
* offers for remote agents; region-aware frameworks are assumed
* to implement their own logic to decide which workloads (if
* any) are suitable for placement on remote agents.
*
*
* REGION_AWARE = 8;
*/
public static final int REGION_AWARE_VALUE = 8;
public final int getNumber() {
return value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static Type valueOf(int value) {
return forNumber(value);
}
public static Type forNumber(int value) {
switch (value) {
case 0: return UNKNOWN;
case 1: return REVOCABLE_RESOURCES;
case 2: return TASK_KILLING_STATE;
case 3: return GPU_RESOURCES;
case 4: return SHARED_RESOURCES;
case 5: return PARTITION_AWARE;
case 6: return MULTI_ROLE;
case 7: return RESERVATION_REFINEMENT;
case 8: return REGION_AWARE;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
Type> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public Type findValueByNumber(int number) {
return Type.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return org.apache.mesos.v1.Protos.FrameworkInfo.Capability.getDescriptor().getEnumTypes().get(0);
}
private static final Type[] VALUES = values();
public static Type valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int value;
private Type(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:mesos.v1.FrameworkInfo.Capability.Type)
}
private int bitField0_;
public static final int TYPE_FIELD_NUMBER = 1;
private int type_;
/**
*
* Enum fields should be optional, see: MESOS-4997.
*
*
* optional .mesos.v1.FrameworkInfo.Capability.Type type = 1;
*/
public boolean hasType() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
*
* Enum fields should be optional, see: MESOS-4997.
*
*
* optional .mesos.v1.FrameworkInfo.Capability.Type type = 1;
*/
public org.apache.mesos.v1.Protos.FrameworkInfo.Capability.Type getType() {
org.apache.mesos.v1.Protos.FrameworkInfo.Capability.Type result = org.apache.mesos.v1.Protos.FrameworkInfo.Capability.Type.valueOf(type_);
return result == null ? org.apache.mesos.v1.Protos.FrameworkInfo.Capability.Type.UNKNOWN : result;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeEnum(1, type_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(1, type_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.mesos.v1.Protos.FrameworkInfo.Capability)) {
return super.equals(obj);
}
org.apache.mesos.v1.Protos.FrameworkInfo.Capability other = (org.apache.mesos.v1.Protos.FrameworkInfo.Capability) obj;
boolean result = true;
result = result && (hasType() == other.hasType());
if (hasType()) {
result = result && type_ == other.type_;
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasType()) {
hash = (37 * hash) + TYPE_FIELD_NUMBER;
hash = (53 * hash) + type_;
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.mesos.v1.Protos.FrameworkInfo.Capability parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.FrameworkInfo.Capability parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.FrameworkInfo.Capability parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.FrameworkInfo.Capability parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.FrameworkInfo.Capability parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.FrameworkInfo.Capability parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.FrameworkInfo.Capability parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.FrameworkInfo.Capability parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.FrameworkInfo.Capability parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.FrameworkInfo.Capability parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.FrameworkInfo.Capability parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.FrameworkInfo.Capability parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.apache.mesos.v1.Protos.FrameworkInfo.Capability prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code mesos.v1.FrameworkInfo.Capability}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:mesos.v1.FrameworkInfo.Capability)
org.apache.mesos.v1.Protos.FrameworkInfo.CapabilityOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_FrameworkInfo_Capability_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_FrameworkInfo_Capability_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.mesos.v1.Protos.FrameworkInfo.Capability.class, org.apache.mesos.v1.Protos.FrameworkInfo.Capability.Builder.class);
}
// Construct using org.apache.mesos.v1.Protos.FrameworkInfo.Capability.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
type_ = 0;
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_FrameworkInfo_Capability_descriptor;
}
public org.apache.mesos.v1.Protos.FrameworkInfo.Capability getDefaultInstanceForType() {
return org.apache.mesos.v1.Protos.FrameworkInfo.Capability.getDefaultInstance();
}
public org.apache.mesos.v1.Protos.FrameworkInfo.Capability build() {
org.apache.mesos.v1.Protos.FrameworkInfo.Capability result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.apache.mesos.v1.Protos.FrameworkInfo.Capability buildPartial() {
org.apache.mesos.v1.Protos.FrameworkInfo.Capability result = new org.apache.mesos.v1.Protos.FrameworkInfo.Capability(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.type_ = type_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.apache.mesos.v1.Protos.FrameworkInfo.Capability) {
return mergeFrom((org.apache.mesos.v1.Protos.FrameworkInfo.Capability)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.mesos.v1.Protos.FrameworkInfo.Capability other) {
if (other == org.apache.mesos.v1.Protos.FrameworkInfo.Capability.getDefaultInstance()) return this;
if (other.hasType()) {
setType(other.getType());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.mesos.v1.Protos.FrameworkInfo.Capability parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.mesos.v1.Protos.FrameworkInfo.Capability) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private int type_ = 0;
/**
*
* Enum fields should be optional, see: MESOS-4997.
*
*
* optional .mesos.v1.FrameworkInfo.Capability.Type type = 1;
*/
public boolean hasType() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
*
* Enum fields should be optional, see: MESOS-4997.
*
*
* optional .mesos.v1.FrameworkInfo.Capability.Type type = 1;
*/
public org.apache.mesos.v1.Protos.FrameworkInfo.Capability.Type getType() {
org.apache.mesos.v1.Protos.FrameworkInfo.Capability.Type result = org.apache.mesos.v1.Protos.FrameworkInfo.Capability.Type.valueOf(type_);
return result == null ? org.apache.mesos.v1.Protos.FrameworkInfo.Capability.Type.UNKNOWN : result;
}
/**
*
* Enum fields should be optional, see: MESOS-4997.
*
*
* optional .mesos.v1.FrameworkInfo.Capability.Type type = 1;
*/
public Builder setType(org.apache.mesos.v1.Protos.FrameworkInfo.Capability.Type value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
type_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Enum fields should be optional, see: MESOS-4997.
*
*
* optional .mesos.v1.FrameworkInfo.Capability.Type type = 1;
*/
public Builder clearType() {
bitField0_ = (bitField0_ & ~0x00000001);
type_ = 0;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:mesos.v1.FrameworkInfo.Capability)
}
// @@protoc_insertion_point(class_scope:mesos.v1.FrameworkInfo.Capability)
private static final org.apache.mesos.v1.Protos.FrameworkInfo.Capability DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.apache.mesos.v1.Protos.FrameworkInfo.Capability();
}
public static org.apache.mesos.v1.Protos.FrameworkInfo.Capability getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public Capability parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Capability(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.apache.mesos.v1.Protos.FrameworkInfo.Capability getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private int bitField0_;
public static final int USER_FIELD_NUMBER = 1;
private volatile java.lang.Object user_;
/**
*
* Used to determine the Unix user that an executor or task should be
* launched as.
* When using the MesosSchedulerDriver, if the field is set to an
* empty string, it will automagically set it to the current user.
* When using the HTTP Scheduler API, the user has to be set
* explicitly.
*
*
* required string user = 1;
*/
public boolean hasUser() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
*
* Used to determine the Unix user that an executor or task should be
* launched as.
* When using the MesosSchedulerDriver, if the field is set to an
* empty string, it will automagically set it to the current user.
* When using the HTTP Scheduler API, the user has to be set
* explicitly.
*
*
* required string user = 1;
*/
public java.lang.String getUser() {
java.lang.Object ref = user_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
user_ = s;
}
return s;
}
}
/**
*
* Used to determine the Unix user that an executor or task should be
* launched as.
* When using the MesosSchedulerDriver, if the field is set to an
* empty string, it will automagically set it to the current user.
* When using the HTTP Scheduler API, the user has to be set
* explicitly.
*
*
* required string user = 1;
*/
public com.google.protobuf.ByteString
getUserBytes() {
java.lang.Object ref = user_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
user_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int NAME_FIELD_NUMBER = 2;
private volatile java.lang.Object name_;
/**
*
* Name of the framework that shows up in the Mesos Web UI.
*
*
* required string name = 2;
*/
public boolean hasName() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
*
* Name of the framework that shows up in the Mesos Web UI.
*
*
* required string name = 2;
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
name_ = s;
}
return s;
}
}
/**
*
* Name of the framework that shows up in the Mesos Web UI.
*
*
* required string name = 2;
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ID_FIELD_NUMBER = 3;
private org.apache.mesos.v1.Protos.FrameworkID id_;
/**
*
* Used to uniquely identify the framework.
* This field must be unset when the framework subscribes for the
* first time upon which the master will assign a new ID. To
* resubscribe after scheduler failover the framework should set
* 'id' to the ID assigned by the master. Setting 'id' to values
* not assigned by Mesos masters is unsupported.
*
*
* optional .mesos.v1.FrameworkID id = 3;
*/
public boolean hasId() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
*
* Used to uniquely identify the framework.
* This field must be unset when the framework subscribes for the
* first time upon which the master will assign a new ID. To
* resubscribe after scheduler failover the framework should set
* 'id' to the ID assigned by the master. Setting 'id' to values
* not assigned by Mesos masters is unsupported.
*
*
* optional .mesos.v1.FrameworkID id = 3;
*/
public org.apache.mesos.v1.Protos.FrameworkID getId() {
return id_ == null ? org.apache.mesos.v1.Protos.FrameworkID.getDefaultInstance() : id_;
}
/**
*
* Used to uniquely identify the framework.
* This field must be unset when the framework subscribes for the
* first time upon which the master will assign a new ID. To
* resubscribe after scheduler failover the framework should set
* 'id' to the ID assigned by the master. Setting 'id' to values
* not assigned by Mesos masters is unsupported.
*
*
* optional .mesos.v1.FrameworkID id = 3;
*/
public org.apache.mesos.v1.Protos.FrameworkIDOrBuilder getIdOrBuilder() {
return id_ == null ? org.apache.mesos.v1.Protos.FrameworkID.getDefaultInstance() : id_;
}
public static final int FAILOVER_TIMEOUT_FIELD_NUMBER = 4;
private double failoverTimeout_;
/**
*
* The amount of time (in seconds) that the master will wait for the
* scheduler to failover before it tears down the framework by
* killing all its tasks/executors. This should be non-zero if a
* framework expects to reconnect after a failure and not lose its
* tasks/executors.
* NOTE: To avoid accidental destruction of tasks, production
* frameworks typically set this to a large value (e.g., 1 week).
*
*
* optional double failover_timeout = 4 [default = 0];
*/
public boolean hasFailoverTimeout() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
*
* The amount of time (in seconds) that the master will wait for the
* scheduler to failover before it tears down the framework by
* killing all its tasks/executors. This should be non-zero if a
* framework expects to reconnect after a failure and not lose its
* tasks/executors.
* NOTE: To avoid accidental destruction of tasks, production
* frameworks typically set this to a large value (e.g., 1 week).
*
*
* optional double failover_timeout = 4 [default = 0];
*/
public double getFailoverTimeout() {
return failoverTimeout_;
}
public static final int CHECKPOINT_FIELD_NUMBER = 5;
private boolean checkpoint_;
/**
*
* If set, agents running tasks started by this framework will write
* the framework pid, executor pids and status updates to disk. If
* the agent exits (e.g., due to a crash or as part of upgrading
* Mesos), this checkpointed data allows the restarted agent to
* reconnect to executors that were started by the old instance of
* the agent. Enabling checkpointing improves fault tolerance, at
* the cost of a (usually small) increase in disk I/O.
*
*
* optional bool checkpoint = 5 [default = false];
*/
public boolean hasCheckpoint() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
*
* If set, agents running tasks started by this framework will write
* the framework pid, executor pids and status updates to disk. If
* the agent exits (e.g., due to a crash or as part of upgrading
* Mesos), this checkpointed data allows the restarted agent to
* reconnect to executors that were started by the old instance of
* the agent. Enabling checkpointing improves fault tolerance, at
* the cost of a (usually small) increase in disk I/O.
*
*
* optional bool checkpoint = 5 [default = false];
*/
public boolean getCheckpoint() {
return checkpoint_;
}
public static final int ROLE_FIELD_NUMBER = 6;
private volatile java.lang.Object role_;
/**
*
* Roles are the entities to which allocations are made.
* The framework must have at least one role in order to
* be offered resources. Note that `role` is deprecated
* in favor of `roles` and only one of these fields must
* be used. Since we cannot distinguish between empty
* `roles` and the default unset `role`, we require that
* frameworks set the `MULTI_ROLE` capability if
* setting the `roles` field.
*
*
* optional string role = 6 [default = "*", deprecated = true];
*/
@java.lang.Deprecated public boolean hasRole() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
*
* Roles are the entities to which allocations are made.
* The framework must have at least one role in order to
* be offered resources. Note that `role` is deprecated
* in favor of `roles` and only one of these fields must
* be used. Since we cannot distinguish between empty
* `roles` and the default unset `role`, we require that
* frameworks set the `MULTI_ROLE` capability if
* setting the `roles` field.
*
*
* optional string role = 6 [default = "*", deprecated = true];
*/
@java.lang.Deprecated public java.lang.String getRole() {
java.lang.Object ref = role_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
role_ = s;
}
return s;
}
}
/**
*
* Roles are the entities to which allocations are made.
* The framework must have at least one role in order to
* be offered resources. Note that `role` is deprecated
* in favor of `roles` and only one of these fields must
* be used. Since we cannot distinguish between empty
* `roles` and the default unset `role`, we require that
* frameworks set the `MULTI_ROLE` capability if
* setting the `roles` field.
*
*
* optional string role = 6 [default = "*", deprecated = true];
*/
@java.lang.Deprecated public com.google.protobuf.ByteString
getRoleBytes() {
java.lang.Object ref = role_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
role_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ROLES_FIELD_NUMBER = 12;
private com.google.protobuf.LazyStringList roles_;
/**
* repeated string roles = 12;
*/
public com.google.protobuf.ProtocolStringList
getRolesList() {
return roles_;
}
/**
* repeated string roles = 12;
*/
public int getRolesCount() {
return roles_.size();
}
/**
* repeated string roles = 12;
*/
public java.lang.String getRoles(int index) {
return roles_.get(index);
}
/**
* repeated string roles = 12;
*/
public com.google.protobuf.ByteString
getRolesBytes(int index) {
return roles_.getByteString(index);
}
public static final int HOSTNAME_FIELD_NUMBER = 7;
private volatile java.lang.Object hostname_;
/**
*
* Used to indicate the current host from which the scheduler is
* registered in the Mesos Web UI. If set to an empty string Mesos
* will automagically set it to the current hostname if one is
* available.
*
*
* optional string hostname = 7;
*/
public boolean hasHostname() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
*
* Used to indicate the current host from which the scheduler is
* registered in the Mesos Web UI. If set to an empty string Mesos
* will automagically set it to the current hostname if one is
* available.
*
*
* optional string hostname = 7;
*/
public java.lang.String getHostname() {
java.lang.Object ref = hostname_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
hostname_ = s;
}
return s;
}
}
/**
*
* Used to indicate the current host from which the scheduler is
* registered in the Mesos Web UI. If set to an empty string Mesos
* will automagically set it to the current hostname if one is
* available.
*
*
* optional string hostname = 7;
*/
public com.google.protobuf.ByteString
getHostnameBytes() {
java.lang.Object ref = hostname_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
hostname_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int PRINCIPAL_FIELD_NUMBER = 8;
private volatile java.lang.Object principal_;
/**
*
* This field should match the credential's principal the framework
* uses for authentication. This field is used for framework API
* rate limiting and dynamic reservations. It should be set even
* if authentication is not enabled if these features are desired.
*
*
* optional string principal = 8;
*/
public boolean hasPrincipal() {
return ((bitField0_ & 0x00000080) == 0x00000080);
}
/**
*
* This field should match the credential's principal the framework
* uses for authentication. This field is used for framework API
* rate limiting and dynamic reservations. It should be set even
* if authentication is not enabled if these features are desired.
*
*
* optional string principal = 8;
*/
public java.lang.String getPrincipal() {
java.lang.Object ref = principal_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
principal_ = s;
}
return s;
}
}
/**
*
* This field should match the credential's principal the framework
* uses for authentication. This field is used for framework API
* rate limiting and dynamic reservations. It should be set even
* if authentication is not enabled if these features are desired.
*
*
* optional string principal = 8;
*/
public com.google.protobuf.ByteString
getPrincipalBytes() {
java.lang.Object ref = principal_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
principal_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int WEBUI_URL_FIELD_NUMBER = 9;
private volatile java.lang.Object webuiUrl_;
/**
*
* This field allows a framework to advertise its web UI, so that
* the Mesos web UI can link to it. It is expected to be a full URL,
* for example http://my-scheduler.example.com:8080/.
*
*
* optional string webui_url = 9;
*/
public boolean hasWebuiUrl() {
return ((bitField0_ & 0x00000100) == 0x00000100);
}
/**
*
* This field allows a framework to advertise its web UI, so that
* the Mesos web UI can link to it. It is expected to be a full URL,
* for example http://my-scheduler.example.com:8080/.
*
*
* optional string webui_url = 9;
*/
public java.lang.String getWebuiUrl() {
java.lang.Object ref = webuiUrl_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
webuiUrl_ = s;
}
return s;
}
}
/**
*
* This field allows a framework to advertise its web UI, so that
* the Mesos web UI can link to it. It is expected to be a full URL,
* for example http://my-scheduler.example.com:8080/.
*
*
* optional string webui_url = 9;
*/
public com.google.protobuf.ByteString
getWebuiUrlBytes() {
java.lang.Object ref = webuiUrl_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
webuiUrl_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CAPABILITIES_FIELD_NUMBER = 10;
private java.util.List capabilities_;
/**
*
* This field allows a framework to advertise its set of
* capabilities (e.g., ability to receive offers for revocable
* resources).
*
*
* repeated .mesos.v1.FrameworkInfo.Capability capabilities = 10;
*/
public java.util.List getCapabilitiesList() {
return capabilities_;
}
/**
*
* This field allows a framework to advertise its set of
* capabilities (e.g., ability to receive offers for revocable
* resources).
*
*
* repeated .mesos.v1.FrameworkInfo.Capability capabilities = 10;
*/
public java.util.List extends org.apache.mesos.v1.Protos.FrameworkInfo.CapabilityOrBuilder>
getCapabilitiesOrBuilderList() {
return capabilities_;
}
/**
*
* This field allows a framework to advertise its set of
* capabilities (e.g., ability to receive offers for revocable
* resources).
*
*
* repeated .mesos.v1.FrameworkInfo.Capability capabilities = 10;
*/
public int getCapabilitiesCount() {
return capabilities_.size();
}
/**
*
* This field allows a framework to advertise its set of
* capabilities (e.g., ability to receive offers for revocable
* resources).
*
*
* repeated .mesos.v1.FrameworkInfo.Capability capabilities = 10;
*/
public org.apache.mesos.v1.Protos.FrameworkInfo.Capability getCapabilities(int index) {
return capabilities_.get(index);
}
/**
*
* This field allows a framework to advertise its set of
* capabilities (e.g., ability to receive offers for revocable
* resources).
*
*
* repeated .mesos.v1.FrameworkInfo.Capability capabilities = 10;
*/
public org.apache.mesos.v1.Protos.FrameworkInfo.CapabilityOrBuilder getCapabilitiesOrBuilder(
int index) {
return capabilities_.get(index);
}
public static final int LABELS_FIELD_NUMBER = 11;
private org.apache.mesos.v1.Protos.Labels labels_;
/**
*
* Labels are free-form key value pairs supplied by the framework
* scheduler (e.g., to describe additional functionality offered by
* the framework). These labels are not interpreted by Mesos itself.
* Labels should not contain duplicate key-value pairs.
*
*
* optional .mesos.v1.Labels labels = 11;
*/
public boolean hasLabels() {
return ((bitField0_ & 0x00000200) == 0x00000200);
}
/**
*
* Labels are free-form key value pairs supplied by the framework
* scheduler (e.g., to describe additional functionality offered by
* the framework). These labels are not interpreted by Mesos itself.
* Labels should not contain duplicate key-value pairs.
*
*
* optional .mesos.v1.Labels labels = 11;
*/
public org.apache.mesos.v1.Protos.Labels getLabels() {
return labels_ == null ? org.apache.mesos.v1.Protos.Labels.getDefaultInstance() : labels_;
}
/**
*
* Labels are free-form key value pairs supplied by the framework
* scheduler (e.g., to describe additional functionality offered by
* the framework). These labels are not interpreted by Mesos itself.
* Labels should not contain duplicate key-value pairs.
*
*
* optional .mesos.v1.Labels labels = 11;
*/
public org.apache.mesos.v1.Protos.LabelsOrBuilder getLabelsOrBuilder() {
return labels_ == null ? org.apache.mesos.v1.Protos.Labels.getDefaultInstance() : labels_;
}
public static final int OFFER_FILTERS_FIELD_NUMBER = 13;
private static final class OfferFiltersDefaultEntryHolder {
static final com.google.protobuf.MapEntry<
java.lang.String, org.apache.mesos.v1.Protos.OfferFilters> defaultEntry =
com.google.protobuf.MapEntry
.newDefaultInstance(
org.apache.mesos.v1.Protos.internal_static_mesos_v1_FrameworkInfo_OfferFiltersEntry_descriptor,
com.google.protobuf.WireFormat.FieldType.STRING,
"",
com.google.protobuf.WireFormat.FieldType.MESSAGE,
org.apache.mesos.v1.Protos.OfferFilters.getDefaultInstance());
}
private com.google.protobuf.MapField<
java.lang.String, org.apache.mesos.v1.Protos.OfferFilters> offerFilters_;
private com.google.protobuf.MapField
internalGetOfferFilters() {
if (offerFilters_ == null) {
return com.google.protobuf.MapField.emptyMapField(
OfferFiltersDefaultEntryHolder.defaultEntry);
}
return offerFilters_;
}
public int getOfferFiltersCount() {
return internalGetOfferFilters().getMap().size();
}
/**
*
* Specifc resource requirements for each of the framework's roles. This field
* is used by e.g., the default allocator to decide whether a framework is
* interested in seeing a resource of a certain shape.
*
*
* map<string, .mesos.v1.OfferFilters> offer_filters = 13;
*/
public boolean containsOfferFilters(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
return internalGetOfferFilters().getMap().containsKey(key);
}
/**
* Use {@link #getOfferFiltersMap()} instead.
*/
@java.lang.Deprecated
public java.util.Map getOfferFilters() {
return getOfferFiltersMap();
}
/**
*
* Specifc resource requirements for each of the framework's roles. This field
* is used by e.g., the default allocator to decide whether a framework is
* interested in seeing a resource of a certain shape.
*
*
* map<string, .mesos.v1.OfferFilters> offer_filters = 13;
*/
public java.util.Map getOfferFiltersMap() {
return internalGetOfferFilters().getMap();
}
/**
*
* Specifc resource requirements for each of the framework's roles. This field
* is used by e.g., the default allocator to decide whether a framework is
* interested in seeing a resource of a certain shape.
*
*
* map<string, .mesos.v1.OfferFilters> offer_filters = 13;
*/
public org.apache.mesos.v1.Protos.OfferFilters getOfferFiltersOrDefault(
java.lang.String key,
org.apache.mesos.v1.Protos.OfferFilters defaultValue) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetOfferFilters().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
*
* Specifc resource requirements for each of the framework's roles. This field
* is used by e.g., the default allocator to decide whether a framework is
* interested in seeing a resource of a certain shape.
*
*
* map<string, .mesos.v1.OfferFilters> offer_filters = 13;
*/
public org.apache.mesos.v1.Protos.OfferFilters getOfferFiltersOrThrow(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetOfferFilters().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasUser()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasName()) {
memoizedIsInitialized = 0;
return false;
}
if (hasId()) {
if (!getId().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
if (hasLabels()) {
if (!getLabels().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
for (org.apache.mesos.v1.Protos.OfferFilters item : getOfferFiltersMap().values()) {
if (!item.isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, user_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, name_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeMessage(3, getId());
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeDouble(4, failoverTimeout_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
output.writeBool(5, checkpoint_);
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 6, role_);
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 7, hostname_);
}
if (((bitField0_ & 0x00000080) == 0x00000080)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 8, principal_);
}
if (((bitField0_ & 0x00000100) == 0x00000100)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 9, webuiUrl_);
}
for (int i = 0; i < capabilities_.size(); i++) {
output.writeMessage(10, capabilities_.get(i));
}
if (((bitField0_ & 0x00000200) == 0x00000200)) {
output.writeMessage(11, getLabels());
}
for (int i = 0; i < roles_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 12, roles_.getRaw(i));
}
com.google.protobuf.GeneratedMessageV3
.serializeStringMapTo(
output,
internalGetOfferFilters(),
OfferFiltersDefaultEntryHolder.defaultEntry,
13);
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, user_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, name_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getId());
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeDoubleSize(4, failoverTimeout_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(5, checkpoint_);
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(6, role_);
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(7, hostname_);
}
if (((bitField0_ & 0x00000080) == 0x00000080)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(8, principal_);
}
if (((bitField0_ & 0x00000100) == 0x00000100)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(9, webuiUrl_);
}
for (int i = 0; i < capabilities_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(10, capabilities_.get(i));
}
if (((bitField0_ & 0x00000200) == 0x00000200)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(11, getLabels());
}
{
int dataSize = 0;
for (int i = 0; i < roles_.size(); i++) {
dataSize += computeStringSizeNoTag(roles_.getRaw(i));
}
size += dataSize;
size += 1 * getRolesList().size();
}
for (java.util.Map.Entry entry
: internalGetOfferFilters().getMap().entrySet()) {
com.google.protobuf.MapEntry
offerFilters__ = OfferFiltersDefaultEntryHolder.defaultEntry.newBuilderForType()
.setKey(entry.getKey())
.setValue(entry.getValue())
.build();
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(13, offerFilters__);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.mesos.v1.Protos.FrameworkInfo)) {
return super.equals(obj);
}
org.apache.mesos.v1.Protos.FrameworkInfo other = (org.apache.mesos.v1.Protos.FrameworkInfo) obj;
boolean result = true;
result = result && (hasUser() == other.hasUser());
if (hasUser()) {
result = result && getUser()
.equals(other.getUser());
}
result = result && (hasName() == other.hasName());
if (hasName()) {
result = result && getName()
.equals(other.getName());
}
result = result && (hasId() == other.hasId());
if (hasId()) {
result = result && getId()
.equals(other.getId());
}
result = result && (hasFailoverTimeout() == other.hasFailoverTimeout());
if (hasFailoverTimeout()) {
result = result && (
java.lang.Double.doubleToLongBits(getFailoverTimeout())
== java.lang.Double.doubleToLongBits(
other.getFailoverTimeout()));
}
result = result && (hasCheckpoint() == other.hasCheckpoint());
if (hasCheckpoint()) {
result = result && (getCheckpoint()
== other.getCheckpoint());
}
result = result && (hasRole() == other.hasRole());
if (hasRole()) {
result = result && getRole()
.equals(other.getRole());
}
result = result && getRolesList()
.equals(other.getRolesList());
result = result && (hasHostname() == other.hasHostname());
if (hasHostname()) {
result = result && getHostname()
.equals(other.getHostname());
}
result = result && (hasPrincipal() == other.hasPrincipal());
if (hasPrincipal()) {
result = result && getPrincipal()
.equals(other.getPrincipal());
}
result = result && (hasWebuiUrl() == other.hasWebuiUrl());
if (hasWebuiUrl()) {
result = result && getWebuiUrl()
.equals(other.getWebuiUrl());
}
result = result && getCapabilitiesList()
.equals(other.getCapabilitiesList());
result = result && (hasLabels() == other.hasLabels());
if (hasLabels()) {
result = result && getLabels()
.equals(other.getLabels());
}
result = result && internalGetOfferFilters().equals(
other.internalGetOfferFilters());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasUser()) {
hash = (37 * hash) + USER_FIELD_NUMBER;
hash = (53 * hash) + getUser().hashCode();
}
if (hasName()) {
hash = (37 * hash) + NAME_FIELD_NUMBER;
hash = (53 * hash) + getName().hashCode();
}
if (hasId()) {
hash = (37 * hash) + ID_FIELD_NUMBER;
hash = (53 * hash) + getId().hashCode();
}
if (hasFailoverTimeout()) {
hash = (37 * hash) + FAILOVER_TIMEOUT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
java.lang.Double.doubleToLongBits(getFailoverTimeout()));
}
if (hasCheckpoint()) {
hash = (37 * hash) + CHECKPOINT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getCheckpoint());
}
if (hasRole()) {
hash = (37 * hash) + ROLE_FIELD_NUMBER;
hash = (53 * hash) + getRole().hashCode();
}
if (getRolesCount() > 0) {
hash = (37 * hash) + ROLES_FIELD_NUMBER;
hash = (53 * hash) + getRolesList().hashCode();
}
if (hasHostname()) {
hash = (37 * hash) + HOSTNAME_FIELD_NUMBER;
hash = (53 * hash) + getHostname().hashCode();
}
if (hasPrincipal()) {
hash = (37 * hash) + PRINCIPAL_FIELD_NUMBER;
hash = (53 * hash) + getPrincipal().hashCode();
}
if (hasWebuiUrl()) {
hash = (37 * hash) + WEBUI_URL_FIELD_NUMBER;
hash = (53 * hash) + getWebuiUrl().hashCode();
}
if (getCapabilitiesCount() > 0) {
hash = (37 * hash) + CAPABILITIES_FIELD_NUMBER;
hash = (53 * hash) + getCapabilitiesList().hashCode();
}
if (hasLabels()) {
hash = (37 * hash) + LABELS_FIELD_NUMBER;
hash = (53 * hash) + getLabels().hashCode();
}
if (!internalGetOfferFilters().getMap().isEmpty()) {
hash = (37 * hash) + OFFER_FILTERS_FIELD_NUMBER;
hash = (53 * hash) + internalGetOfferFilters().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.mesos.v1.Protos.FrameworkInfo parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.FrameworkInfo parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.FrameworkInfo parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.FrameworkInfo parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.FrameworkInfo parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.FrameworkInfo parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.FrameworkInfo parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.FrameworkInfo parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.FrameworkInfo parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.FrameworkInfo parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.FrameworkInfo parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.FrameworkInfo parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.apache.mesos.v1.Protos.FrameworkInfo prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
**
* Describes a framework.
*
*
* Protobuf type {@code mesos.v1.FrameworkInfo}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:mesos.v1.FrameworkInfo)
org.apache.mesos.v1.Protos.FrameworkInfoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_FrameworkInfo_descriptor;
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapField internalGetMapField(
int number) {
switch (number) {
case 13:
return internalGetOfferFilters();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapField internalGetMutableMapField(
int number) {
switch (number) {
case 13:
return internalGetMutableOfferFilters();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_FrameworkInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.mesos.v1.Protos.FrameworkInfo.class, org.apache.mesos.v1.Protos.FrameworkInfo.Builder.class);
}
// Construct using org.apache.mesos.v1.Protos.FrameworkInfo.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getIdFieldBuilder();
getCapabilitiesFieldBuilder();
getLabelsFieldBuilder();
}
}
public Builder clear() {
super.clear();
user_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
name_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
if (idBuilder_ == null) {
id_ = null;
} else {
idBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000004);
failoverTimeout_ = 0D;
bitField0_ = (bitField0_ & ~0x00000008);
checkpoint_ = false;
bitField0_ = (bitField0_ & ~0x00000010);
role_ = "*";
bitField0_ = (bitField0_ & ~0x00000020);
roles_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000040);
hostname_ = "";
bitField0_ = (bitField0_ & ~0x00000080);
principal_ = "";
bitField0_ = (bitField0_ & ~0x00000100);
webuiUrl_ = "";
bitField0_ = (bitField0_ & ~0x00000200);
if (capabilitiesBuilder_ == null) {
capabilities_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000400);
} else {
capabilitiesBuilder_.clear();
}
if (labelsBuilder_ == null) {
labels_ = null;
} else {
labelsBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000800);
internalGetMutableOfferFilters().clear();
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_FrameworkInfo_descriptor;
}
public org.apache.mesos.v1.Protos.FrameworkInfo getDefaultInstanceForType() {
return org.apache.mesos.v1.Protos.FrameworkInfo.getDefaultInstance();
}
public org.apache.mesos.v1.Protos.FrameworkInfo build() {
org.apache.mesos.v1.Protos.FrameworkInfo result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.apache.mesos.v1.Protos.FrameworkInfo buildPartial() {
org.apache.mesos.v1.Protos.FrameworkInfo result = new org.apache.mesos.v1.Protos.FrameworkInfo(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.user_ = user_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.name_ = name_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
if (idBuilder_ == null) {
result.id_ = id_;
} else {
result.id_ = idBuilder_.build();
}
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000008;
}
result.failoverTimeout_ = failoverTimeout_;
if (((from_bitField0_ & 0x00000010) == 0x00000010)) {
to_bitField0_ |= 0x00000010;
}
result.checkpoint_ = checkpoint_;
if (((from_bitField0_ & 0x00000020) == 0x00000020)) {
to_bitField0_ |= 0x00000020;
}
result.role_ = role_;
if (((bitField0_ & 0x00000040) == 0x00000040)) {
roles_ = roles_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x00000040);
}
result.roles_ = roles_;
if (((from_bitField0_ & 0x00000080) == 0x00000080)) {
to_bitField0_ |= 0x00000040;
}
result.hostname_ = hostname_;
if (((from_bitField0_ & 0x00000100) == 0x00000100)) {
to_bitField0_ |= 0x00000080;
}
result.principal_ = principal_;
if (((from_bitField0_ & 0x00000200) == 0x00000200)) {
to_bitField0_ |= 0x00000100;
}
result.webuiUrl_ = webuiUrl_;
if (capabilitiesBuilder_ == null) {
if (((bitField0_ & 0x00000400) == 0x00000400)) {
capabilities_ = java.util.Collections.unmodifiableList(capabilities_);
bitField0_ = (bitField0_ & ~0x00000400);
}
result.capabilities_ = capabilities_;
} else {
result.capabilities_ = capabilitiesBuilder_.build();
}
if (((from_bitField0_ & 0x00000800) == 0x00000800)) {
to_bitField0_ |= 0x00000200;
}
if (labelsBuilder_ == null) {
result.labels_ = labels_;
} else {
result.labels_ = labelsBuilder_.build();
}
result.offerFilters_ = internalGetOfferFilters();
result.offerFilters_.makeImmutable();
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.apache.mesos.v1.Protos.FrameworkInfo) {
return mergeFrom((org.apache.mesos.v1.Protos.FrameworkInfo)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.mesos.v1.Protos.FrameworkInfo other) {
if (other == org.apache.mesos.v1.Protos.FrameworkInfo.getDefaultInstance()) return this;
if (other.hasUser()) {
bitField0_ |= 0x00000001;
user_ = other.user_;
onChanged();
}
if (other.hasName()) {
bitField0_ |= 0x00000002;
name_ = other.name_;
onChanged();
}
if (other.hasId()) {
mergeId(other.getId());
}
if (other.hasFailoverTimeout()) {
setFailoverTimeout(other.getFailoverTimeout());
}
if (other.hasCheckpoint()) {
setCheckpoint(other.getCheckpoint());
}
if (other.hasRole()) {
bitField0_ |= 0x00000020;
role_ = other.role_;
onChanged();
}
if (!other.roles_.isEmpty()) {
if (roles_.isEmpty()) {
roles_ = other.roles_;
bitField0_ = (bitField0_ & ~0x00000040);
} else {
ensureRolesIsMutable();
roles_.addAll(other.roles_);
}
onChanged();
}
if (other.hasHostname()) {
bitField0_ |= 0x00000080;
hostname_ = other.hostname_;
onChanged();
}
if (other.hasPrincipal()) {
bitField0_ |= 0x00000100;
principal_ = other.principal_;
onChanged();
}
if (other.hasWebuiUrl()) {
bitField0_ |= 0x00000200;
webuiUrl_ = other.webuiUrl_;
onChanged();
}
if (capabilitiesBuilder_ == null) {
if (!other.capabilities_.isEmpty()) {
if (capabilities_.isEmpty()) {
capabilities_ = other.capabilities_;
bitField0_ = (bitField0_ & ~0x00000400);
} else {
ensureCapabilitiesIsMutable();
capabilities_.addAll(other.capabilities_);
}
onChanged();
}
} else {
if (!other.capabilities_.isEmpty()) {
if (capabilitiesBuilder_.isEmpty()) {
capabilitiesBuilder_.dispose();
capabilitiesBuilder_ = null;
capabilities_ = other.capabilities_;
bitField0_ = (bitField0_ & ~0x00000400);
capabilitiesBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getCapabilitiesFieldBuilder() : null;
} else {
capabilitiesBuilder_.addAllMessages(other.capabilities_);
}
}
}
if (other.hasLabels()) {
mergeLabels(other.getLabels());
}
internalGetMutableOfferFilters().mergeFrom(
other.internalGetOfferFilters());
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
if (!hasUser()) {
return false;
}
if (!hasName()) {
return false;
}
if (hasId()) {
if (!getId().isInitialized()) {
return false;
}
}
if (hasLabels()) {
if (!getLabels().isInitialized()) {
return false;
}
}
for (org.apache.mesos.v1.Protos.OfferFilters item : getOfferFiltersMap().values()) {
if (!item.isInitialized()) {
return false;
}
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.mesos.v1.Protos.FrameworkInfo parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.mesos.v1.Protos.FrameworkInfo) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object user_ = "";
/**
*
* Used to determine the Unix user that an executor or task should be
* launched as.
* When using the MesosSchedulerDriver, if the field is set to an
* empty string, it will automagically set it to the current user.
* When using the HTTP Scheduler API, the user has to be set
* explicitly.
*
*
* required string user = 1;
*/
public boolean hasUser() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
*
* Used to determine the Unix user that an executor or task should be
* launched as.
* When using the MesosSchedulerDriver, if the field is set to an
* empty string, it will automagically set it to the current user.
* When using the HTTP Scheduler API, the user has to be set
* explicitly.
*
*
* required string user = 1;
*/
public java.lang.String getUser() {
java.lang.Object ref = user_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
user_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Used to determine the Unix user that an executor or task should be
* launched as.
* When using the MesosSchedulerDriver, if the field is set to an
* empty string, it will automagically set it to the current user.
* When using the HTTP Scheduler API, the user has to be set
* explicitly.
*
*
* required string user = 1;
*/
public com.google.protobuf.ByteString
getUserBytes() {
java.lang.Object ref = user_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
user_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Used to determine the Unix user that an executor or task should be
* launched as.
* When using the MesosSchedulerDriver, if the field is set to an
* empty string, it will automagically set it to the current user.
* When using the HTTP Scheduler API, the user has to be set
* explicitly.
*
*
* required string user = 1;
*/
public Builder setUser(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
user_ = value;
onChanged();
return this;
}
/**
*
* Used to determine the Unix user that an executor or task should be
* launched as.
* When using the MesosSchedulerDriver, if the field is set to an
* empty string, it will automagically set it to the current user.
* When using the HTTP Scheduler API, the user has to be set
* explicitly.
*
*
* required string user = 1;
*/
public Builder clearUser() {
bitField0_ = (bitField0_ & ~0x00000001);
user_ = getDefaultInstance().getUser();
onChanged();
return this;
}
/**
*
* Used to determine the Unix user that an executor or task should be
* launched as.
* When using the MesosSchedulerDriver, if the field is set to an
* empty string, it will automagically set it to the current user.
* When using the HTTP Scheduler API, the user has to be set
* explicitly.
*
*
* required string user = 1;
*/
public Builder setUserBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
user_ = value;
onChanged();
return this;
}
private java.lang.Object name_ = "";
/**
*
* Name of the framework that shows up in the Mesos Web UI.
*
*
* required string name = 2;
*/
public boolean hasName() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
*
* Name of the framework that shows up in the Mesos Web UI.
*
*
* required string name = 2;
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
name_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Name of the framework that shows up in the Mesos Web UI.
*
*
* required string name = 2;
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Name of the framework that shows up in the Mesos Web UI.
*
*
* required string name = 2;
*/
public Builder setName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
name_ = value;
onChanged();
return this;
}
/**
*
* Name of the framework that shows up in the Mesos Web UI.
*
*
* required string name = 2;
*/
public Builder clearName() {
bitField0_ = (bitField0_ & ~0x00000002);
name_ = getDefaultInstance().getName();
onChanged();
return this;
}
/**
*
* Name of the framework that shows up in the Mesos Web UI.
*
*
* required string name = 2;
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
name_ = value;
onChanged();
return this;
}
private org.apache.mesos.v1.Protos.FrameworkID id_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
org.apache.mesos.v1.Protos.FrameworkID, org.apache.mesos.v1.Protos.FrameworkID.Builder, org.apache.mesos.v1.Protos.FrameworkIDOrBuilder> idBuilder_;
/**
*
* Used to uniquely identify the framework.
* This field must be unset when the framework subscribes for the
* first time upon which the master will assign a new ID. To
* resubscribe after scheduler failover the framework should set
* 'id' to the ID assigned by the master. Setting 'id' to values
* not assigned by Mesos masters is unsupported.
*
*
* optional .mesos.v1.FrameworkID id = 3;
*/
public boolean hasId() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
*
* Used to uniquely identify the framework.
* This field must be unset when the framework subscribes for the
* first time upon which the master will assign a new ID. To
* resubscribe after scheduler failover the framework should set
* 'id' to the ID assigned by the master. Setting 'id' to values
* not assigned by Mesos masters is unsupported.
*
*
* optional .mesos.v1.FrameworkID id = 3;
*/
public org.apache.mesos.v1.Protos.FrameworkID getId() {
if (idBuilder_ == null) {
return id_ == null ? org.apache.mesos.v1.Protos.FrameworkID.getDefaultInstance() : id_;
} else {
return idBuilder_.getMessage();
}
}
/**
*
* Used to uniquely identify the framework.
* This field must be unset when the framework subscribes for the
* first time upon which the master will assign a new ID. To
* resubscribe after scheduler failover the framework should set
* 'id' to the ID assigned by the master. Setting 'id' to values
* not assigned by Mesos masters is unsupported.
*
*
* optional .mesos.v1.FrameworkID id = 3;
*/
public Builder setId(org.apache.mesos.v1.Protos.FrameworkID value) {
if (idBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
id_ = value;
onChanged();
} else {
idBuilder_.setMessage(value);
}
bitField0_ |= 0x00000004;
return this;
}
/**
*
* Used to uniquely identify the framework.
* This field must be unset when the framework subscribes for the
* first time upon which the master will assign a new ID. To
* resubscribe after scheduler failover the framework should set
* 'id' to the ID assigned by the master. Setting 'id' to values
* not assigned by Mesos masters is unsupported.
*
*
* optional .mesos.v1.FrameworkID id = 3;
*/
public Builder setId(
org.apache.mesos.v1.Protos.FrameworkID.Builder builderForValue) {
if (idBuilder_ == null) {
id_ = builderForValue.build();
onChanged();
} else {
idBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000004;
return this;
}
/**
*
* Used to uniquely identify the framework.
* This field must be unset when the framework subscribes for the
* first time upon which the master will assign a new ID. To
* resubscribe after scheduler failover the framework should set
* 'id' to the ID assigned by the master. Setting 'id' to values
* not assigned by Mesos masters is unsupported.
*
*
* optional .mesos.v1.FrameworkID id = 3;
*/
public Builder mergeId(org.apache.mesos.v1.Protos.FrameworkID value) {
if (idBuilder_ == null) {
if (((bitField0_ & 0x00000004) == 0x00000004) &&
id_ != null &&
id_ != org.apache.mesos.v1.Protos.FrameworkID.getDefaultInstance()) {
id_ =
org.apache.mesos.v1.Protos.FrameworkID.newBuilder(id_).mergeFrom(value).buildPartial();
} else {
id_ = value;
}
onChanged();
} else {
idBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000004;
return this;
}
/**
*
* Used to uniquely identify the framework.
* This field must be unset when the framework subscribes for the
* first time upon which the master will assign a new ID. To
* resubscribe after scheduler failover the framework should set
* 'id' to the ID assigned by the master. Setting 'id' to values
* not assigned by Mesos masters is unsupported.
*
*
* optional .mesos.v1.FrameworkID id = 3;
*/
public Builder clearId() {
if (idBuilder_ == null) {
id_ = null;
onChanged();
} else {
idBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000004);
return this;
}
/**
*
* Used to uniquely identify the framework.
* This field must be unset when the framework subscribes for the
* first time upon which the master will assign a new ID. To
* resubscribe after scheduler failover the framework should set
* 'id' to the ID assigned by the master. Setting 'id' to values
* not assigned by Mesos masters is unsupported.
*
*
* optional .mesos.v1.FrameworkID id = 3;
*/
public org.apache.mesos.v1.Protos.FrameworkID.Builder getIdBuilder() {
bitField0_ |= 0x00000004;
onChanged();
return getIdFieldBuilder().getBuilder();
}
/**
*
* Used to uniquely identify the framework.
* This field must be unset when the framework subscribes for the
* first time upon which the master will assign a new ID. To
* resubscribe after scheduler failover the framework should set
* 'id' to the ID assigned by the master. Setting 'id' to values
* not assigned by Mesos masters is unsupported.
*
*
* optional .mesos.v1.FrameworkID id = 3;
*/
public org.apache.mesos.v1.Protos.FrameworkIDOrBuilder getIdOrBuilder() {
if (idBuilder_ != null) {
return idBuilder_.getMessageOrBuilder();
} else {
return id_ == null ?
org.apache.mesos.v1.Protos.FrameworkID.getDefaultInstance() : id_;
}
}
/**
*
* Used to uniquely identify the framework.
* This field must be unset when the framework subscribes for the
* first time upon which the master will assign a new ID. To
* resubscribe after scheduler failover the framework should set
* 'id' to the ID assigned by the master. Setting 'id' to values
* not assigned by Mesos masters is unsupported.
*
*
* optional .mesos.v1.FrameworkID id = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.apache.mesos.v1.Protos.FrameworkID, org.apache.mesos.v1.Protos.FrameworkID.Builder, org.apache.mesos.v1.Protos.FrameworkIDOrBuilder>
getIdFieldBuilder() {
if (idBuilder_ == null) {
idBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.apache.mesos.v1.Protos.FrameworkID, org.apache.mesos.v1.Protos.FrameworkID.Builder, org.apache.mesos.v1.Protos.FrameworkIDOrBuilder>(
getId(),
getParentForChildren(),
isClean());
id_ = null;
}
return idBuilder_;
}
private double failoverTimeout_ ;
/**
*
* The amount of time (in seconds) that the master will wait for the
* scheduler to failover before it tears down the framework by
* killing all its tasks/executors. This should be non-zero if a
* framework expects to reconnect after a failure and not lose its
* tasks/executors.
* NOTE: To avoid accidental destruction of tasks, production
* frameworks typically set this to a large value (e.g., 1 week).
*
*
* optional double failover_timeout = 4 [default = 0];
*/
public boolean hasFailoverTimeout() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
*
* The amount of time (in seconds) that the master will wait for the
* scheduler to failover before it tears down the framework by
* killing all its tasks/executors. This should be non-zero if a
* framework expects to reconnect after a failure and not lose its
* tasks/executors.
* NOTE: To avoid accidental destruction of tasks, production
* frameworks typically set this to a large value (e.g., 1 week).
*
*
* optional double failover_timeout = 4 [default = 0];
*/
public double getFailoverTimeout() {
return failoverTimeout_;
}
/**
*
* The amount of time (in seconds) that the master will wait for the
* scheduler to failover before it tears down the framework by
* killing all its tasks/executors. This should be non-zero if a
* framework expects to reconnect after a failure and not lose its
* tasks/executors.
* NOTE: To avoid accidental destruction of tasks, production
* frameworks typically set this to a large value (e.g., 1 week).
*
*
* optional double failover_timeout = 4 [default = 0];
*/
public Builder setFailoverTimeout(double value) {
bitField0_ |= 0x00000008;
failoverTimeout_ = value;
onChanged();
return this;
}
/**
*
* The amount of time (in seconds) that the master will wait for the
* scheduler to failover before it tears down the framework by
* killing all its tasks/executors. This should be non-zero if a
* framework expects to reconnect after a failure and not lose its
* tasks/executors.
* NOTE: To avoid accidental destruction of tasks, production
* frameworks typically set this to a large value (e.g., 1 week).
*
*
* optional double failover_timeout = 4 [default = 0];
*/
public Builder clearFailoverTimeout() {
bitField0_ = (bitField0_ & ~0x00000008);
failoverTimeout_ = 0D;
onChanged();
return this;
}
private boolean checkpoint_ ;
/**
*
* If set, agents running tasks started by this framework will write
* the framework pid, executor pids and status updates to disk. If
* the agent exits (e.g., due to a crash or as part of upgrading
* Mesos), this checkpointed data allows the restarted agent to
* reconnect to executors that were started by the old instance of
* the agent. Enabling checkpointing improves fault tolerance, at
* the cost of a (usually small) increase in disk I/O.
*
*
* optional bool checkpoint = 5 [default = false];
*/
public boolean hasCheckpoint() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
*
* If set, agents running tasks started by this framework will write
* the framework pid, executor pids and status updates to disk. If
* the agent exits (e.g., due to a crash or as part of upgrading
* Mesos), this checkpointed data allows the restarted agent to
* reconnect to executors that were started by the old instance of
* the agent. Enabling checkpointing improves fault tolerance, at
* the cost of a (usually small) increase in disk I/O.
*
*
* optional bool checkpoint = 5 [default = false];
*/
public boolean getCheckpoint() {
return checkpoint_;
}
/**
*
* If set, agents running tasks started by this framework will write
* the framework pid, executor pids and status updates to disk. If
* the agent exits (e.g., due to a crash or as part of upgrading
* Mesos), this checkpointed data allows the restarted agent to
* reconnect to executors that were started by the old instance of
* the agent. Enabling checkpointing improves fault tolerance, at
* the cost of a (usually small) increase in disk I/O.
*
*
* optional bool checkpoint = 5 [default = false];
*/
public Builder setCheckpoint(boolean value) {
bitField0_ |= 0x00000010;
checkpoint_ = value;
onChanged();
return this;
}
/**
*
* If set, agents running tasks started by this framework will write
* the framework pid, executor pids and status updates to disk. If
* the agent exits (e.g., due to a crash or as part of upgrading
* Mesos), this checkpointed data allows the restarted agent to
* reconnect to executors that were started by the old instance of
* the agent. Enabling checkpointing improves fault tolerance, at
* the cost of a (usually small) increase in disk I/O.
*
*
* optional bool checkpoint = 5 [default = false];
*/
public Builder clearCheckpoint() {
bitField0_ = (bitField0_ & ~0x00000010);
checkpoint_ = false;
onChanged();
return this;
}
private java.lang.Object role_ = "*";
/**
*
* Roles are the entities to which allocations are made.
* The framework must have at least one role in order to
* be offered resources. Note that `role` is deprecated
* in favor of `roles` and only one of these fields must
* be used. Since we cannot distinguish between empty
* `roles` and the default unset `role`, we require that
* frameworks set the `MULTI_ROLE` capability if
* setting the `roles` field.
*
*
* optional string role = 6 [default = "*", deprecated = true];
*/
@java.lang.Deprecated public boolean hasRole() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
*
* Roles are the entities to which allocations are made.
* The framework must have at least one role in order to
* be offered resources. Note that `role` is deprecated
* in favor of `roles` and only one of these fields must
* be used. Since we cannot distinguish between empty
* `roles` and the default unset `role`, we require that
* frameworks set the `MULTI_ROLE` capability if
* setting the `roles` field.
*
*
* optional string role = 6 [default = "*", deprecated = true];
*/
@java.lang.Deprecated public java.lang.String getRole() {
java.lang.Object ref = role_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
role_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Roles are the entities to which allocations are made.
* The framework must have at least one role in order to
* be offered resources. Note that `role` is deprecated
* in favor of `roles` and only one of these fields must
* be used. Since we cannot distinguish between empty
* `roles` and the default unset `role`, we require that
* frameworks set the `MULTI_ROLE` capability if
* setting the `roles` field.
*
*
* optional string role = 6 [default = "*", deprecated = true];
*/
@java.lang.Deprecated public com.google.protobuf.ByteString
getRoleBytes() {
java.lang.Object ref = role_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
role_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Roles are the entities to which allocations are made.
* The framework must have at least one role in order to
* be offered resources. Note that `role` is deprecated
* in favor of `roles` and only one of these fields must
* be used. Since we cannot distinguish between empty
* `roles` and the default unset `role`, we require that
* frameworks set the `MULTI_ROLE` capability if
* setting the `roles` field.
*
*
* optional string role = 6 [default = "*", deprecated = true];
*/
@java.lang.Deprecated public Builder setRole(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000020;
role_ = value;
onChanged();
return this;
}
/**
*
* Roles are the entities to which allocations are made.
* The framework must have at least one role in order to
* be offered resources. Note that `role` is deprecated
* in favor of `roles` and only one of these fields must
* be used. Since we cannot distinguish between empty
* `roles` and the default unset `role`, we require that
* frameworks set the `MULTI_ROLE` capability if
* setting the `roles` field.
*
*
* optional string role = 6 [default = "*", deprecated = true];
*/
@java.lang.Deprecated public Builder clearRole() {
bitField0_ = (bitField0_ & ~0x00000020);
role_ = getDefaultInstance().getRole();
onChanged();
return this;
}
/**
*
* Roles are the entities to which allocations are made.
* The framework must have at least one role in order to
* be offered resources. Note that `role` is deprecated
* in favor of `roles` and only one of these fields must
* be used. Since we cannot distinguish between empty
* `roles` and the default unset `role`, we require that
* frameworks set the `MULTI_ROLE` capability if
* setting the `roles` field.
*
*
* optional string role = 6 [default = "*", deprecated = true];
*/
@java.lang.Deprecated public Builder setRoleBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000020;
role_ = value;
onChanged();
return this;
}
private com.google.protobuf.LazyStringList roles_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureRolesIsMutable() {
if (!((bitField0_ & 0x00000040) == 0x00000040)) {
roles_ = new com.google.protobuf.LazyStringArrayList(roles_);
bitField0_ |= 0x00000040;
}
}
/**
* repeated string roles = 12;
*/
public com.google.protobuf.ProtocolStringList
getRolesList() {
return roles_.getUnmodifiableView();
}
/**
* repeated string roles = 12;
*/
public int getRolesCount() {
return roles_.size();
}
/**
* repeated string roles = 12;
*/
public java.lang.String getRoles(int index) {
return roles_.get(index);
}
/**
* repeated string roles = 12;
*/
public com.google.protobuf.ByteString
getRolesBytes(int index) {
return roles_.getByteString(index);
}
/**
* repeated string roles = 12;
*/
public Builder setRoles(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureRolesIsMutable();
roles_.set(index, value);
onChanged();
return this;
}
/**
* repeated string roles = 12;
*/
public Builder addRoles(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureRolesIsMutable();
roles_.add(value);
onChanged();
return this;
}
/**
* repeated string roles = 12;
*/
public Builder addAllRoles(
java.lang.Iterable values) {
ensureRolesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, roles_);
onChanged();
return this;
}
/**
* repeated string roles = 12;
*/
public Builder clearRoles() {
roles_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000040);
onChanged();
return this;
}
/**
* repeated string roles = 12;
*/
public Builder addRolesBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureRolesIsMutable();
roles_.add(value);
onChanged();
return this;
}
private java.lang.Object hostname_ = "";
/**
*
* Used to indicate the current host from which the scheduler is
* registered in the Mesos Web UI. If set to an empty string Mesos
* will automagically set it to the current hostname if one is
* available.
*
*
* optional string hostname = 7;
*/
public boolean hasHostname() {
return ((bitField0_ & 0x00000080) == 0x00000080);
}
/**
*
* Used to indicate the current host from which the scheduler is
* registered in the Mesos Web UI. If set to an empty string Mesos
* will automagically set it to the current hostname if one is
* available.
*
*
* optional string hostname = 7;
*/
public java.lang.String getHostname() {
java.lang.Object ref = hostname_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
hostname_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Used to indicate the current host from which the scheduler is
* registered in the Mesos Web UI. If set to an empty string Mesos
* will automagically set it to the current hostname if one is
* available.
*
*
* optional string hostname = 7;
*/
public com.google.protobuf.ByteString
getHostnameBytes() {
java.lang.Object ref = hostname_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
hostname_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Used to indicate the current host from which the scheduler is
* registered in the Mesos Web UI. If set to an empty string Mesos
* will automagically set it to the current hostname if one is
* available.
*
*
* optional string hostname = 7;
*/
public Builder setHostname(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000080;
hostname_ = value;
onChanged();
return this;
}
/**
*
* Used to indicate the current host from which the scheduler is
* registered in the Mesos Web UI. If set to an empty string Mesos
* will automagically set it to the current hostname if one is
* available.
*
*
* optional string hostname = 7;
*/
public Builder clearHostname() {
bitField0_ = (bitField0_ & ~0x00000080);
hostname_ = getDefaultInstance().getHostname();
onChanged();
return this;
}
/**
*
* Used to indicate the current host from which the scheduler is
* registered in the Mesos Web UI. If set to an empty string Mesos
* will automagically set it to the current hostname if one is
* available.
*
*
* optional string hostname = 7;
*/
public Builder setHostnameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000080;
hostname_ = value;
onChanged();
return this;
}
private java.lang.Object principal_ = "";
/**
*
* This field should match the credential's principal the framework
* uses for authentication. This field is used for framework API
* rate limiting and dynamic reservations. It should be set even
* if authentication is not enabled if these features are desired.
*
*
* optional string principal = 8;
*/
public boolean hasPrincipal() {
return ((bitField0_ & 0x00000100) == 0x00000100);
}
/**
*
* This field should match the credential's principal the framework
* uses for authentication. This field is used for framework API
* rate limiting and dynamic reservations. It should be set even
* if authentication is not enabled if these features are desired.
*
*
* optional string principal = 8;
*/
public java.lang.String getPrincipal() {
java.lang.Object ref = principal_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
principal_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* This field should match the credential's principal the framework
* uses for authentication. This field is used for framework API
* rate limiting and dynamic reservations. It should be set even
* if authentication is not enabled if these features are desired.
*
*
* optional string principal = 8;
*/
public com.google.protobuf.ByteString
getPrincipalBytes() {
java.lang.Object ref = principal_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
principal_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* This field should match the credential's principal the framework
* uses for authentication. This field is used for framework API
* rate limiting and dynamic reservations. It should be set even
* if authentication is not enabled if these features are desired.
*
*
* optional string principal = 8;
*/
public Builder setPrincipal(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000100;
principal_ = value;
onChanged();
return this;
}
/**
*
* This field should match the credential's principal the framework
* uses for authentication. This field is used for framework API
* rate limiting and dynamic reservations. It should be set even
* if authentication is not enabled if these features are desired.
*
*
* optional string principal = 8;
*/
public Builder clearPrincipal() {
bitField0_ = (bitField0_ & ~0x00000100);
principal_ = getDefaultInstance().getPrincipal();
onChanged();
return this;
}
/**
*
* This field should match the credential's principal the framework
* uses for authentication. This field is used for framework API
* rate limiting and dynamic reservations. It should be set even
* if authentication is not enabled if these features are desired.
*
*
* optional string principal = 8;
*/
public Builder setPrincipalBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000100;
principal_ = value;
onChanged();
return this;
}
private java.lang.Object webuiUrl_ = "";
/**
*
* This field allows a framework to advertise its web UI, so that
* the Mesos web UI can link to it. It is expected to be a full URL,
* for example http://my-scheduler.example.com:8080/.
*
*
* optional string webui_url = 9;
*/
public boolean hasWebuiUrl() {
return ((bitField0_ & 0x00000200) == 0x00000200);
}
/**
*
* This field allows a framework to advertise its web UI, so that
* the Mesos web UI can link to it. It is expected to be a full URL,
* for example http://my-scheduler.example.com:8080/.
*
*
* optional string webui_url = 9;
*/
public java.lang.String getWebuiUrl() {
java.lang.Object ref = webuiUrl_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
webuiUrl_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* This field allows a framework to advertise its web UI, so that
* the Mesos web UI can link to it. It is expected to be a full URL,
* for example http://my-scheduler.example.com:8080/.
*
*
* optional string webui_url = 9;
*/
public com.google.protobuf.ByteString
getWebuiUrlBytes() {
java.lang.Object ref = webuiUrl_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
webuiUrl_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* This field allows a framework to advertise its web UI, so that
* the Mesos web UI can link to it. It is expected to be a full URL,
* for example http://my-scheduler.example.com:8080/.
*
*
* optional string webui_url = 9;
*/
public Builder setWebuiUrl(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000200;
webuiUrl_ = value;
onChanged();
return this;
}
/**
*
* This field allows a framework to advertise its web UI, so that
* the Mesos web UI can link to it. It is expected to be a full URL,
* for example http://my-scheduler.example.com:8080/.
*
*
* optional string webui_url = 9;
*/
public Builder clearWebuiUrl() {
bitField0_ = (bitField0_ & ~0x00000200);
webuiUrl_ = getDefaultInstance().getWebuiUrl();
onChanged();
return this;
}
/**
*
* This field allows a framework to advertise its web UI, so that
* the Mesos web UI can link to it. It is expected to be a full URL,
* for example http://my-scheduler.example.com:8080/.
*
*
* optional string webui_url = 9;
*/
public Builder setWebuiUrlBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000200;
webuiUrl_ = value;
onChanged();
return this;
}
private java.util.List capabilities_ =
java.util.Collections.emptyList();
private void ensureCapabilitiesIsMutable() {
if (!((bitField0_ & 0x00000400) == 0x00000400)) {
capabilities_ = new java.util.ArrayList(capabilities_);
bitField0_ |= 0x00000400;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.apache.mesos.v1.Protos.FrameworkInfo.Capability, org.apache.mesos.v1.Protos.FrameworkInfo.Capability.Builder, org.apache.mesos.v1.Protos.FrameworkInfo.CapabilityOrBuilder> capabilitiesBuilder_;
/**
*
* This field allows a framework to advertise its set of
* capabilities (e.g., ability to receive offers for revocable
* resources).
*
*
* repeated .mesos.v1.FrameworkInfo.Capability capabilities = 10;
*/
public java.util.List getCapabilitiesList() {
if (capabilitiesBuilder_ == null) {
return java.util.Collections.unmodifiableList(capabilities_);
} else {
return capabilitiesBuilder_.getMessageList();
}
}
/**
*
* This field allows a framework to advertise its set of
* capabilities (e.g., ability to receive offers for revocable
* resources).
*
*
* repeated .mesos.v1.FrameworkInfo.Capability capabilities = 10;
*/
public int getCapabilitiesCount() {
if (capabilitiesBuilder_ == null) {
return capabilities_.size();
} else {
return capabilitiesBuilder_.getCount();
}
}
/**
*
* This field allows a framework to advertise its set of
* capabilities (e.g., ability to receive offers for revocable
* resources).
*
*
* repeated .mesos.v1.FrameworkInfo.Capability capabilities = 10;
*/
public org.apache.mesos.v1.Protos.FrameworkInfo.Capability getCapabilities(int index) {
if (capabilitiesBuilder_ == null) {
return capabilities_.get(index);
} else {
return capabilitiesBuilder_.getMessage(index);
}
}
/**
*
* This field allows a framework to advertise its set of
* capabilities (e.g., ability to receive offers for revocable
* resources).
*
*
* repeated .mesos.v1.FrameworkInfo.Capability capabilities = 10;
*/
public Builder setCapabilities(
int index, org.apache.mesos.v1.Protos.FrameworkInfo.Capability value) {
if (capabilitiesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureCapabilitiesIsMutable();
capabilities_.set(index, value);
onChanged();
} else {
capabilitiesBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* This field allows a framework to advertise its set of
* capabilities (e.g., ability to receive offers for revocable
* resources).
*
*
* repeated .mesos.v1.FrameworkInfo.Capability capabilities = 10;
*/
public Builder setCapabilities(
int index, org.apache.mesos.v1.Protos.FrameworkInfo.Capability.Builder builderForValue) {
if (capabilitiesBuilder_ == null) {
ensureCapabilitiesIsMutable();
capabilities_.set(index, builderForValue.build());
onChanged();
} else {
capabilitiesBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* This field allows a framework to advertise its set of
* capabilities (e.g., ability to receive offers for revocable
* resources).
*
*
* repeated .mesos.v1.FrameworkInfo.Capability capabilities = 10;
*/
public Builder addCapabilities(org.apache.mesos.v1.Protos.FrameworkInfo.Capability value) {
if (capabilitiesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureCapabilitiesIsMutable();
capabilities_.add(value);
onChanged();
} else {
capabilitiesBuilder_.addMessage(value);
}
return this;
}
/**
*
* This field allows a framework to advertise its set of
* capabilities (e.g., ability to receive offers for revocable
* resources).
*
*
* repeated .mesos.v1.FrameworkInfo.Capability capabilities = 10;
*/
public Builder addCapabilities(
int index, org.apache.mesos.v1.Protos.FrameworkInfo.Capability value) {
if (capabilitiesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureCapabilitiesIsMutable();
capabilities_.add(index, value);
onChanged();
} else {
capabilitiesBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* This field allows a framework to advertise its set of
* capabilities (e.g., ability to receive offers for revocable
* resources).
*
*
* repeated .mesos.v1.FrameworkInfo.Capability capabilities = 10;
*/
public Builder addCapabilities(
org.apache.mesos.v1.Protos.FrameworkInfo.Capability.Builder builderForValue) {
if (capabilitiesBuilder_ == null) {
ensureCapabilitiesIsMutable();
capabilities_.add(builderForValue.build());
onChanged();
} else {
capabilitiesBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* This field allows a framework to advertise its set of
* capabilities (e.g., ability to receive offers for revocable
* resources).
*
*
* repeated .mesos.v1.FrameworkInfo.Capability capabilities = 10;
*/
public Builder addCapabilities(
int index, org.apache.mesos.v1.Protos.FrameworkInfo.Capability.Builder builderForValue) {
if (capabilitiesBuilder_ == null) {
ensureCapabilitiesIsMutable();
capabilities_.add(index, builderForValue.build());
onChanged();
} else {
capabilitiesBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* This field allows a framework to advertise its set of
* capabilities (e.g., ability to receive offers for revocable
* resources).
*
*
* repeated .mesos.v1.FrameworkInfo.Capability capabilities = 10;
*/
public Builder addAllCapabilities(
java.lang.Iterable extends org.apache.mesos.v1.Protos.FrameworkInfo.Capability> values) {
if (capabilitiesBuilder_ == null) {
ensureCapabilitiesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, capabilities_);
onChanged();
} else {
capabilitiesBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* This field allows a framework to advertise its set of
* capabilities (e.g., ability to receive offers for revocable
* resources).
*
*
* repeated .mesos.v1.FrameworkInfo.Capability capabilities = 10;
*/
public Builder clearCapabilities() {
if (capabilitiesBuilder_ == null) {
capabilities_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000400);
onChanged();
} else {
capabilitiesBuilder_.clear();
}
return this;
}
/**
*
* This field allows a framework to advertise its set of
* capabilities (e.g., ability to receive offers for revocable
* resources).
*
*
* repeated .mesos.v1.FrameworkInfo.Capability capabilities = 10;
*/
public Builder removeCapabilities(int index) {
if (capabilitiesBuilder_ == null) {
ensureCapabilitiesIsMutable();
capabilities_.remove(index);
onChanged();
} else {
capabilitiesBuilder_.remove(index);
}
return this;
}
/**
*
* This field allows a framework to advertise its set of
* capabilities (e.g., ability to receive offers for revocable
* resources).
*
*
* repeated .mesos.v1.FrameworkInfo.Capability capabilities = 10;
*/
public org.apache.mesos.v1.Protos.FrameworkInfo.Capability.Builder getCapabilitiesBuilder(
int index) {
return getCapabilitiesFieldBuilder().getBuilder(index);
}
/**
*
* This field allows a framework to advertise its set of
* capabilities (e.g., ability to receive offers for revocable
* resources).
*
*
* repeated .mesos.v1.FrameworkInfo.Capability capabilities = 10;
*/
public org.apache.mesos.v1.Protos.FrameworkInfo.CapabilityOrBuilder getCapabilitiesOrBuilder(
int index) {
if (capabilitiesBuilder_ == null) {
return capabilities_.get(index); } else {
return capabilitiesBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* This field allows a framework to advertise its set of
* capabilities (e.g., ability to receive offers for revocable
* resources).
*
*
* repeated .mesos.v1.FrameworkInfo.Capability capabilities = 10;
*/
public java.util.List extends org.apache.mesos.v1.Protos.FrameworkInfo.CapabilityOrBuilder>
getCapabilitiesOrBuilderList() {
if (capabilitiesBuilder_ != null) {
return capabilitiesBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(capabilities_);
}
}
/**
*
* This field allows a framework to advertise its set of
* capabilities (e.g., ability to receive offers for revocable
* resources).
*
*
* repeated .mesos.v1.FrameworkInfo.Capability capabilities = 10;
*/
public org.apache.mesos.v1.Protos.FrameworkInfo.Capability.Builder addCapabilitiesBuilder() {
return getCapabilitiesFieldBuilder().addBuilder(
org.apache.mesos.v1.Protos.FrameworkInfo.Capability.getDefaultInstance());
}
/**
*
* This field allows a framework to advertise its set of
* capabilities (e.g., ability to receive offers for revocable
* resources).
*
*
* repeated .mesos.v1.FrameworkInfo.Capability capabilities = 10;
*/
public org.apache.mesos.v1.Protos.FrameworkInfo.Capability.Builder addCapabilitiesBuilder(
int index) {
return getCapabilitiesFieldBuilder().addBuilder(
index, org.apache.mesos.v1.Protos.FrameworkInfo.Capability.getDefaultInstance());
}
/**
*
* This field allows a framework to advertise its set of
* capabilities (e.g., ability to receive offers for revocable
* resources).
*
*
* repeated .mesos.v1.FrameworkInfo.Capability capabilities = 10;
*/
public java.util.List
getCapabilitiesBuilderList() {
return getCapabilitiesFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.apache.mesos.v1.Protos.FrameworkInfo.Capability, org.apache.mesos.v1.Protos.FrameworkInfo.Capability.Builder, org.apache.mesos.v1.Protos.FrameworkInfo.CapabilityOrBuilder>
getCapabilitiesFieldBuilder() {
if (capabilitiesBuilder_ == null) {
capabilitiesBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
org.apache.mesos.v1.Protos.FrameworkInfo.Capability, org.apache.mesos.v1.Protos.FrameworkInfo.Capability.Builder, org.apache.mesos.v1.Protos.FrameworkInfo.CapabilityOrBuilder>(
capabilities_,
((bitField0_ & 0x00000400) == 0x00000400),
getParentForChildren(),
isClean());
capabilities_ = null;
}
return capabilitiesBuilder_;
}
private org.apache.mesos.v1.Protos.Labels labels_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
org.apache.mesos.v1.Protos.Labels, org.apache.mesos.v1.Protos.Labels.Builder, org.apache.mesos.v1.Protos.LabelsOrBuilder> labelsBuilder_;
/**
*
* Labels are free-form key value pairs supplied by the framework
* scheduler (e.g., to describe additional functionality offered by
* the framework). These labels are not interpreted by Mesos itself.
* Labels should not contain duplicate key-value pairs.
*
*
* optional .mesos.v1.Labels labels = 11;
*/
public boolean hasLabels() {
return ((bitField0_ & 0x00000800) == 0x00000800);
}
/**
*
* Labels are free-form key value pairs supplied by the framework
* scheduler (e.g., to describe additional functionality offered by
* the framework). These labels are not interpreted by Mesos itself.
* Labels should not contain duplicate key-value pairs.
*
*
* optional .mesos.v1.Labels labels = 11;
*/
public org.apache.mesos.v1.Protos.Labels getLabels() {
if (labelsBuilder_ == null) {
return labels_ == null ? org.apache.mesos.v1.Protos.Labels.getDefaultInstance() : labels_;
} else {
return labelsBuilder_.getMessage();
}
}
/**
*
* Labels are free-form key value pairs supplied by the framework
* scheduler (e.g., to describe additional functionality offered by
* the framework). These labels are not interpreted by Mesos itself.
* Labels should not contain duplicate key-value pairs.
*
*
* optional .mesos.v1.Labels labels = 11;
*/
public Builder setLabels(org.apache.mesos.v1.Protos.Labels value) {
if (labelsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
labels_ = value;
onChanged();
} else {
labelsBuilder_.setMessage(value);
}
bitField0_ |= 0x00000800;
return this;
}
/**
*
* Labels are free-form key value pairs supplied by the framework
* scheduler (e.g., to describe additional functionality offered by
* the framework). These labels are not interpreted by Mesos itself.
* Labels should not contain duplicate key-value pairs.
*
*
* optional .mesos.v1.Labels labels = 11;
*/
public Builder setLabels(
org.apache.mesos.v1.Protos.Labels.Builder builderForValue) {
if (labelsBuilder_ == null) {
labels_ = builderForValue.build();
onChanged();
} else {
labelsBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000800;
return this;
}
/**
*
* Labels are free-form key value pairs supplied by the framework
* scheduler (e.g., to describe additional functionality offered by
* the framework). These labels are not interpreted by Mesos itself.
* Labels should not contain duplicate key-value pairs.
*
*
* optional .mesos.v1.Labels labels = 11;
*/
public Builder mergeLabels(org.apache.mesos.v1.Protos.Labels value) {
if (labelsBuilder_ == null) {
if (((bitField0_ & 0x00000800) == 0x00000800) &&
labels_ != null &&
labels_ != org.apache.mesos.v1.Protos.Labels.getDefaultInstance()) {
labels_ =
org.apache.mesos.v1.Protos.Labels.newBuilder(labels_).mergeFrom(value).buildPartial();
} else {
labels_ = value;
}
onChanged();
} else {
labelsBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000800;
return this;
}
/**
*
* Labels are free-form key value pairs supplied by the framework
* scheduler (e.g., to describe additional functionality offered by
* the framework). These labels are not interpreted by Mesos itself.
* Labels should not contain duplicate key-value pairs.
*
*
* optional .mesos.v1.Labels labels = 11;
*/
public Builder clearLabels() {
if (labelsBuilder_ == null) {
labels_ = null;
onChanged();
} else {
labelsBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000800);
return this;
}
/**
*
* Labels are free-form key value pairs supplied by the framework
* scheduler (e.g., to describe additional functionality offered by
* the framework). These labels are not interpreted by Mesos itself.
* Labels should not contain duplicate key-value pairs.
*
*
* optional .mesos.v1.Labels labels = 11;
*/
public org.apache.mesos.v1.Protos.Labels.Builder getLabelsBuilder() {
bitField0_ |= 0x00000800;
onChanged();
return getLabelsFieldBuilder().getBuilder();
}
/**
*
* Labels are free-form key value pairs supplied by the framework
* scheduler (e.g., to describe additional functionality offered by
* the framework). These labels are not interpreted by Mesos itself.
* Labels should not contain duplicate key-value pairs.
*
*
* optional .mesos.v1.Labels labels = 11;
*/
public org.apache.mesos.v1.Protos.LabelsOrBuilder getLabelsOrBuilder() {
if (labelsBuilder_ != null) {
return labelsBuilder_.getMessageOrBuilder();
} else {
return labels_ == null ?
org.apache.mesos.v1.Protos.Labels.getDefaultInstance() : labels_;
}
}
/**
*
* Labels are free-form key value pairs supplied by the framework
* scheduler (e.g., to describe additional functionality offered by
* the framework). These labels are not interpreted by Mesos itself.
* Labels should not contain duplicate key-value pairs.
*
*
* optional .mesos.v1.Labels labels = 11;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.apache.mesos.v1.Protos.Labels, org.apache.mesos.v1.Protos.Labels.Builder, org.apache.mesos.v1.Protos.LabelsOrBuilder>
getLabelsFieldBuilder() {
if (labelsBuilder_ == null) {
labelsBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.apache.mesos.v1.Protos.Labels, org.apache.mesos.v1.Protos.Labels.Builder, org.apache.mesos.v1.Protos.LabelsOrBuilder>(
getLabels(),
getParentForChildren(),
isClean());
labels_ = null;
}
return labelsBuilder_;
}
private com.google.protobuf.MapField<
java.lang.String, org.apache.mesos.v1.Protos.OfferFilters> offerFilters_;
private com.google.protobuf.MapField
internalGetOfferFilters() {
if (offerFilters_ == null) {
return com.google.protobuf.MapField.emptyMapField(
OfferFiltersDefaultEntryHolder.defaultEntry);
}
return offerFilters_;
}
private com.google.protobuf.MapField
internalGetMutableOfferFilters() {
onChanged();;
if (offerFilters_ == null) {
offerFilters_ = com.google.protobuf.MapField.newMapField(
OfferFiltersDefaultEntryHolder.defaultEntry);
}
if (!offerFilters_.isMutable()) {
offerFilters_ = offerFilters_.copy();
}
return offerFilters_;
}
public int getOfferFiltersCount() {
return internalGetOfferFilters().getMap().size();
}
/**
*
* Specifc resource requirements for each of the framework's roles. This field
* is used by e.g., the default allocator to decide whether a framework is
* interested in seeing a resource of a certain shape.
*
*
* map<string, .mesos.v1.OfferFilters> offer_filters = 13;
*/
public boolean containsOfferFilters(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
return internalGetOfferFilters().getMap().containsKey(key);
}
/**
* Use {@link #getOfferFiltersMap()} instead.
*/
@java.lang.Deprecated
public java.util.Map getOfferFilters() {
return getOfferFiltersMap();
}
/**
*
* Specifc resource requirements for each of the framework's roles. This field
* is used by e.g., the default allocator to decide whether a framework is
* interested in seeing a resource of a certain shape.
*
*
* map<string, .mesos.v1.OfferFilters> offer_filters = 13;
*/
public java.util.Map getOfferFiltersMap() {
return internalGetOfferFilters().getMap();
}
/**
*
* Specifc resource requirements for each of the framework's roles. This field
* is used by e.g., the default allocator to decide whether a framework is
* interested in seeing a resource of a certain shape.
*
*
* map<string, .mesos.v1.OfferFilters> offer_filters = 13;
*/
public org.apache.mesos.v1.Protos.OfferFilters getOfferFiltersOrDefault(
java.lang.String key,
org.apache.mesos.v1.Protos.OfferFilters defaultValue) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetOfferFilters().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
*
* Specifc resource requirements for each of the framework's roles. This field
* is used by e.g., the default allocator to decide whether a framework is
* interested in seeing a resource of a certain shape.
*
*
* map<string, .mesos.v1.OfferFilters> offer_filters = 13;
*/
public org.apache.mesos.v1.Protos.OfferFilters getOfferFiltersOrThrow(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetOfferFilters().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public Builder clearOfferFilters() {
internalGetMutableOfferFilters().getMutableMap()
.clear();
return this;
}
/**
*
* Specifc resource requirements for each of the framework's roles. This field
* is used by e.g., the default allocator to decide whether a framework is
* interested in seeing a resource of a certain shape.
*
*
* map<string, .mesos.v1.OfferFilters> offer_filters = 13;
*/
public Builder removeOfferFilters(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
internalGetMutableOfferFilters().getMutableMap()
.remove(key);
return this;
}
/**
* Use alternate mutation accessors instead.
*/
@java.lang.Deprecated
public java.util.Map
getMutableOfferFilters() {
return internalGetMutableOfferFilters().getMutableMap();
}
/**
*
* Specifc resource requirements for each of the framework's roles. This field
* is used by e.g., the default allocator to decide whether a framework is
* interested in seeing a resource of a certain shape.
*
*
* map<string, .mesos.v1.OfferFilters> offer_filters = 13;
*/
public Builder putOfferFilters(
java.lang.String key,
org.apache.mesos.v1.Protos.OfferFilters value) {
if (key == null) { throw new java.lang.NullPointerException(); }
if (value == null) { throw new java.lang.NullPointerException(); }
internalGetMutableOfferFilters().getMutableMap()
.put(key, value);
return this;
}
/**
*
* Specifc resource requirements for each of the framework's roles. This field
* is used by e.g., the default allocator to decide whether a framework is
* interested in seeing a resource of a certain shape.
*
*
* map<string, .mesos.v1.OfferFilters> offer_filters = 13;
*/
public Builder putAllOfferFilters(
java.util.Map values) {
internalGetMutableOfferFilters().getMutableMap()
.putAll(values);
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:mesos.v1.FrameworkInfo)
}
// @@protoc_insertion_point(class_scope:mesos.v1.FrameworkInfo)
private static final org.apache.mesos.v1.Protos.FrameworkInfo DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.apache.mesos.v1.Protos.FrameworkInfo();
}
public static org.apache.mesos.v1.Protos.FrameworkInfo getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public FrameworkInfo parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new FrameworkInfo(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.apache.mesos.v1.Protos.FrameworkInfo getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface CheckInfoOrBuilder extends
// @@protoc_insertion_point(interface_extends:mesos.v1.CheckInfo)
com.google.protobuf.MessageOrBuilder {
/**
*
* The type of the check.
*
*
* optional .mesos.v1.CheckInfo.Type type = 1;
*/
boolean hasType();
/**
*
* The type of the check.
*
*
* optional .mesos.v1.CheckInfo.Type type = 1;
*/
org.apache.mesos.v1.Protos.CheckInfo.Type getType();
/**
*
* Command check.
*
*
* optional .mesos.v1.CheckInfo.Command command = 2;
*/
boolean hasCommand();
/**
*
* Command check.
*
*
* optional .mesos.v1.CheckInfo.Command command = 2;
*/
org.apache.mesos.v1.Protos.CheckInfo.Command getCommand();
/**
*
* Command check.
*
*
* optional .mesos.v1.CheckInfo.Command command = 2;
*/
org.apache.mesos.v1.Protos.CheckInfo.CommandOrBuilder getCommandOrBuilder();
/**
*
* HTTP check.
*
*
* optional .mesos.v1.CheckInfo.Http http = 3;
*/
boolean hasHttp();
/**
*
* HTTP check.
*
*
* optional .mesos.v1.CheckInfo.Http http = 3;
*/
org.apache.mesos.v1.Protos.CheckInfo.Http getHttp();
/**
*
* HTTP check.
*
*
* optional .mesos.v1.CheckInfo.Http http = 3;
*/
org.apache.mesos.v1.Protos.CheckInfo.HttpOrBuilder getHttpOrBuilder();
/**
*
* TCP check.
*
*
* optional .mesos.v1.CheckInfo.Tcp tcp = 7;
*/
boolean hasTcp();
/**
*
* TCP check.
*
*
* optional .mesos.v1.CheckInfo.Tcp tcp = 7;
*/
org.apache.mesos.v1.Protos.CheckInfo.Tcp getTcp();
/**
*
* TCP check.
*
*
* optional .mesos.v1.CheckInfo.Tcp tcp = 7;
*/
org.apache.mesos.v1.Protos.CheckInfo.TcpOrBuilder getTcpOrBuilder();
/**
*
* Amount of time to wait to start checking the task after it
* transitions to `TASK_RUNNING` or `TASK_STARTING` if the latter
* is used by the executor.
*
*
* optional double delay_seconds = 4 [default = 15];
*/
boolean hasDelaySeconds();
/**
*
* Amount of time to wait to start checking the task after it
* transitions to `TASK_RUNNING` or `TASK_STARTING` if the latter
* is used by the executor.
*
*
* optional double delay_seconds = 4 [default = 15];
*/
double getDelaySeconds();
/**
*
* Interval between check attempts, i.e., amount of time to wait after
* the previous check finished or timed out to start the next check.
*
*
* optional double interval_seconds = 5 [default = 10];
*/
boolean hasIntervalSeconds();
/**
*
* Interval between check attempts, i.e., amount of time to wait after
* the previous check finished or timed out to start the next check.
*
*
* optional double interval_seconds = 5 [default = 10];
*/
double getIntervalSeconds();
/**
*
* Amount of time to wait for the check to complete. Zero means infinite
* timeout.
* After this timeout, the check attempt is aborted and no result is
* reported. Note that this may be considered a state change and hence
* may trigger a check status change delivery to the corresponding
* scheduler. See `CheckStatusInfo` for more details.
*
*
* optional double timeout_seconds = 6 [default = 20];
*/
boolean hasTimeoutSeconds();
/**
*
* Amount of time to wait for the check to complete. Zero means infinite
* timeout.
* After this timeout, the check attempt is aborted and no result is
* reported. Note that this may be considered a state change and hence
* may trigger a check status change delivery to the corresponding
* scheduler. See `CheckStatusInfo` for more details.
*
*
* optional double timeout_seconds = 6 [default = 20];
*/
double getTimeoutSeconds();
}
/**
*
**
* Describes a general non-interpreting non-killing check for a task or
* executor (or any arbitrary process/command). A type is picked by
* specifying one of the optional fields. Specifying more than one type
* is an error.
* NOTE: This API is subject to change and the related feature is experimental.
*
*
* Protobuf type {@code mesos.v1.CheckInfo}
*/
public static final class CheckInfo extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:mesos.v1.CheckInfo)
CheckInfoOrBuilder {
private static final long serialVersionUID = 0L;
// Use CheckInfo.newBuilder() to construct.
private CheckInfo(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private CheckInfo() {
type_ = 0;
delaySeconds_ = 15D;
intervalSeconds_ = 10D;
timeoutSeconds_ = 20D;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private CheckInfo(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
int rawValue = input.readEnum();
org.apache.mesos.v1.Protos.CheckInfo.Type value = org.apache.mesos.v1.Protos.CheckInfo.Type.valueOf(rawValue);
if (value == null) {
unknownFields.mergeVarintField(1, rawValue);
} else {
bitField0_ |= 0x00000001;
type_ = rawValue;
}
break;
}
case 18: {
org.apache.mesos.v1.Protos.CheckInfo.Command.Builder subBuilder = null;
if (((bitField0_ & 0x00000002) == 0x00000002)) {
subBuilder = command_.toBuilder();
}
command_ = input.readMessage(org.apache.mesos.v1.Protos.CheckInfo.Command.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(command_);
command_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000002;
break;
}
case 26: {
org.apache.mesos.v1.Protos.CheckInfo.Http.Builder subBuilder = null;
if (((bitField0_ & 0x00000004) == 0x00000004)) {
subBuilder = http_.toBuilder();
}
http_ = input.readMessage(org.apache.mesos.v1.Protos.CheckInfo.Http.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(http_);
http_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000004;
break;
}
case 33: {
bitField0_ |= 0x00000010;
delaySeconds_ = input.readDouble();
break;
}
case 41: {
bitField0_ |= 0x00000020;
intervalSeconds_ = input.readDouble();
break;
}
case 49: {
bitField0_ |= 0x00000040;
timeoutSeconds_ = input.readDouble();
break;
}
case 58: {
org.apache.mesos.v1.Protos.CheckInfo.Tcp.Builder subBuilder = null;
if (((bitField0_ & 0x00000008) == 0x00000008)) {
subBuilder = tcp_.toBuilder();
}
tcp_ = input.readMessage(org.apache.mesos.v1.Protos.CheckInfo.Tcp.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(tcp_);
tcp_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000008;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_CheckInfo_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_CheckInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.mesos.v1.Protos.CheckInfo.class, org.apache.mesos.v1.Protos.CheckInfo.Builder.class);
}
/**
* Protobuf enum {@code mesos.v1.CheckInfo.Type}
*/
public enum Type
implements com.google.protobuf.ProtocolMessageEnum {
/**
* UNKNOWN = 0;
*/
UNKNOWN(0),
/**
* COMMAND = 1;
*/
COMMAND(1),
/**
* HTTP = 2;
*/
HTTP(2),
/**
* TCP = 3;
*/
TCP(3),
;
/**
* UNKNOWN = 0;
*/
public static final int UNKNOWN_VALUE = 0;
/**
* COMMAND = 1;
*/
public static final int COMMAND_VALUE = 1;
/**
* HTTP = 2;
*/
public static final int HTTP_VALUE = 2;
/**
* TCP = 3;
*/
public static final int TCP_VALUE = 3;
public final int getNumber() {
return value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static Type valueOf(int value) {
return forNumber(value);
}
public static Type forNumber(int value) {
switch (value) {
case 0: return UNKNOWN;
case 1: return COMMAND;
case 2: return HTTP;
case 3: return TCP;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
Type> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public Type findValueByNumber(int number) {
return Type.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return org.apache.mesos.v1.Protos.CheckInfo.getDescriptor().getEnumTypes().get(0);
}
private static final Type[] VALUES = values();
public static Type valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int value;
private Type(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:mesos.v1.CheckInfo.Type)
}
public interface CommandOrBuilder extends
// @@protoc_insertion_point(interface_extends:mesos.v1.CheckInfo.Command)
com.google.protobuf.MessageOrBuilder {
/**
* required .mesos.v1.CommandInfo command = 1;
*/
boolean hasCommand();
/**
* required .mesos.v1.CommandInfo command = 1;
*/
org.apache.mesos.v1.Protos.CommandInfo getCommand();
/**
* required .mesos.v1.CommandInfo command = 1;
*/
org.apache.mesos.v1.Protos.CommandInfoOrBuilder getCommandOrBuilder();
}
/**
*
* Describes a command check. If applicable, enters mount and/or network
* namespaces of the task.
*
*
* Protobuf type {@code mesos.v1.CheckInfo.Command}
*/
public static final class Command extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:mesos.v1.CheckInfo.Command)
CommandOrBuilder {
private static final long serialVersionUID = 0L;
// Use Command.newBuilder() to construct.
private Command(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Command() {
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Command(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
org.apache.mesos.v1.Protos.CommandInfo.Builder subBuilder = null;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
subBuilder = command_.toBuilder();
}
command_ = input.readMessage(org.apache.mesos.v1.Protos.CommandInfo.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(command_);
command_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000001;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_CheckInfo_Command_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_CheckInfo_Command_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.mesos.v1.Protos.CheckInfo.Command.class, org.apache.mesos.v1.Protos.CheckInfo.Command.Builder.class);
}
private int bitField0_;
public static final int COMMAND_FIELD_NUMBER = 1;
private org.apache.mesos.v1.Protos.CommandInfo command_;
/**
* required .mesos.v1.CommandInfo command = 1;
*/
public boolean hasCommand() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required .mesos.v1.CommandInfo command = 1;
*/
public org.apache.mesos.v1.Protos.CommandInfo getCommand() {
return command_ == null ? org.apache.mesos.v1.Protos.CommandInfo.getDefaultInstance() : command_;
}
/**
* required .mesos.v1.CommandInfo command = 1;
*/
public org.apache.mesos.v1.Protos.CommandInfoOrBuilder getCommandOrBuilder() {
return command_ == null ? org.apache.mesos.v1.Protos.CommandInfo.getDefaultInstance() : command_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasCommand()) {
memoizedIsInitialized = 0;
return false;
}
if (!getCommand().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeMessage(1, getCommand());
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getCommand());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.mesos.v1.Protos.CheckInfo.Command)) {
return super.equals(obj);
}
org.apache.mesos.v1.Protos.CheckInfo.Command other = (org.apache.mesos.v1.Protos.CheckInfo.Command) obj;
boolean result = true;
result = result && (hasCommand() == other.hasCommand());
if (hasCommand()) {
result = result && getCommand()
.equals(other.getCommand());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasCommand()) {
hash = (37 * hash) + COMMAND_FIELD_NUMBER;
hash = (53 * hash) + getCommand().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.mesos.v1.Protos.CheckInfo.Command parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.CheckInfo.Command parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.CheckInfo.Command parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.CheckInfo.Command parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.CheckInfo.Command parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.CheckInfo.Command parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.CheckInfo.Command parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.CheckInfo.Command parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.CheckInfo.Command parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.CheckInfo.Command parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.CheckInfo.Command parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.CheckInfo.Command parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.apache.mesos.v1.Protos.CheckInfo.Command prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Describes a command check. If applicable, enters mount and/or network
* namespaces of the task.
*
*
* Protobuf type {@code mesos.v1.CheckInfo.Command}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:mesos.v1.CheckInfo.Command)
org.apache.mesos.v1.Protos.CheckInfo.CommandOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_CheckInfo_Command_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_CheckInfo_Command_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.mesos.v1.Protos.CheckInfo.Command.class, org.apache.mesos.v1.Protos.CheckInfo.Command.Builder.class);
}
// Construct using org.apache.mesos.v1.Protos.CheckInfo.Command.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getCommandFieldBuilder();
}
}
public Builder clear() {
super.clear();
if (commandBuilder_ == null) {
command_ = null;
} else {
commandBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_CheckInfo_Command_descriptor;
}
public org.apache.mesos.v1.Protos.CheckInfo.Command getDefaultInstanceForType() {
return org.apache.mesos.v1.Protos.CheckInfo.Command.getDefaultInstance();
}
public org.apache.mesos.v1.Protos.CheckInfo.Command build() {
org.apache.mesos.v1.Protos.CheckInfo.Command result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.apache.mesos.v1.Protos.CheckInfo.Command buildPartial() {
org.apache.mesos.v1.Protos.CheckInfo.Command result = new org.apache.mesos.v1.Protos.CheckInfo.Command(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
if (commandBuilder_ == null) {
result.command_ = command_;
} else {
result.command_ = commandBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.apache.mesos.v1.Protos.CheckInfo.Command) {
return mergeFrom((org.apache.mesos.v1.Protos.CheckInfo.Command)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.mesos.v1.Protos.CheckInfo.Command other) {
if (other == org.apache.mesos.v1.Protos.CheckInfo.Command.getDefaultInstance()) return this;
if (other.hasCommand()) {
mergeCommand(other.getCommand());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
if (!hasCommand()) {
return false;
}
if (!getCommand().isInitialized()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.mesos.v1.Protos.CheckInfo.Command parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.mesos.v1.Protos.CheckInfo.Command) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private org.apache.mesos.v1.Protos.CommandInfo command_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
org.apache.mesos.v1.Protos.CommandInfo, org.apache.mesos.v1.Protos.CommandInfo.Builder, org.apache.mesos.v1.Protos.CommandInfoOrBuilder> commandBuilder_;
/**
* required .mesos.v1.CommandInfo command = 1;
*/
public boolean hasCommand() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required .mesos.v1.CommandInfo command = 1;
*/
public org.apache.mesos.v1.Protos.CommandInfo getCommand() {
if (commandBuilder_ == null) {
return command_ == null ? org.apache.mesos.v1.Protos.CommandInfo.getDefaultInstance() : command_;
} else {
return commandBuilder_.getMessage();
}
}
/**
* required .mesos.v1.CommandInfo command = 1;
*/
public Builder setCommand(org.apache.mesos.v1.Protos.CommandInfo value) {
if (commandBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
command_ = value;
onChanged();
} else {
commandBuilder_.setMessage(value);
}
bitField0_ |= 0x00000001;
return this;
}
/**
* required .mesos.v1.CommandInfo command = 1;
*/
public Builder setCommand(
org.apache.mesos.v1.Protos.CommandInfo.Builder builderForValue) {
if (commandBuilder_ == null) {
command_ = builderForValue.build();
onChanged();
} else {
commandBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000001;
return this;
}
/**
* required .mesos.v1.CommandInfo command = 1;
*/
public Builder mergeCommand(org.apache.mesos.v1.Protos.CommandInfo value) {
if (commandBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001) &&
command_ != null &&
command_ != org.apache.mesos.v1.Protos.CommandInfo.getDefaultInstance()) {
command_ =
org.apache.mesos.v1.Protos.CommandInfo.newBuilder(command_).mergeFrom(value).buildPartial();
} else {
command_ = value;
}
onChanged();
} else {
commandBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000001;
return this;
}
/**
* required .mesos.v1.CommandInfo command = 1;
*/
public Builder clearCommand() {
if (commandBuilder_ == null) {
command_ = null;
onChanged();
} else {
commandBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
/**
* required .mesos.v1.CommandInfo command = 1;
*/
public org.apache.mesos.v1.Protos.CommandInfo.Builder getCommandBuilder() {
bitField0_ |= 0x00000001;
onChanged();
return getCommandFieldBuilder().getBuilder();
}
/**
* required .mesos.v1.CommandInfo command = 1;
*/
public org.apache.mesos.v1.Protos.CommandInfoOrBuilder getCommandOrBuilder() {
if (commandBuilder_ != null) {
return commandBuilder_.getMessageOrBuilder();
} else {
return command_ == null ?
org.apache.mesos.v1.Protos.CommandInfo.getDefaultInstance() : command_;
}
}
/**
* required .mesos.v1.CommandInfo command = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.apache.mesos.v1.Protos.CommandInfo, org.apache.mesos.v1.Protos.CommandInfo.Builder, org.apache.mesos.v1.Protos.CommandInfoOrBuilder>
getCommandFieldBuilder() {
if (commandBuilder_ == null) {
commandBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.apache.mesos.v1.Protos.CommandInfo, org.apache.mesos.v1.Protos.CommandInfo.Builder, org.apache.mesos.v1.Protos.CommandInfoOrBuilder>(
getCommand(),
getParentForChildren(),
isClean());
command_ = null;
}
return commandBuilder_;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:mesos.v1.CheckInfo.Command)
}
// @@protoc_insertion_point(class_scope:mesos.v1.CheckInfo.Command)
private static final org.apache.mesos.v1.Protos.CheckInfo.Command DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.apache.mesos.v1.Protos.CheckInfo.Command();
}
public static org.apache.mesos.v1.Protos.CheckInfo.Command getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public Command parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Command(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.apache.mesos.v1.Protos.CheckInfo.Command getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface HttpOrBuilder extends
// @@protoc_insertion_point(interface_extends:mesos.v1.CheckInfo.Http)
com.google.protobuf.MessageOrBuilder {
/**
*
* Port to send the HTTP request.
*
*
* required uint32 port = 1;
*/
boolean hasPort();
/**
*
* Port to send the HTTP request.
*
*
* required uint32 port = 1;
*/
int getPort();
/**
*
* HTTP request path.
*
*
* optional string path = 2;
*/
boolean hasPath();
/**
*
* HTTP request path.
*
*
* optional string path = 2;
*/
java.lang.String getPath();
/**
*
* HTTP request path.
*
*
* optional string path = 2;
*/
com.google.protobuf.ByteString
getPathBytes();
}
/**
*
* Describes an HTTP check. Sends a GET request to
* http://<host>:port/path. Note that <host> is not configurable and is
* resolved automatically to 127.0.0.1.
*
*
* Protobuf type {@code mesos.v1.CheckInfo.Http}
*/
public static final class Http extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:mesos.v1.CheckInfo.Http)
HttpOrBuilder {
private static final long serialVersionUID = 0L;
// Use Http.newBuilder() to construct.
private Http(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Http() {
port_ = 0;
path_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Http(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
bitField0_ |= 0x00000001;
port_ = input.readUInt32();
break;
}
case 18: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000002;
path_ = bs;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_CheckInfo_Http_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_CheckInfo_Http_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.mesos.v1.Protos.CheckInfo.Http.class, org.apache.mesos.v1.Protos.CheckInfo.Http.Builder.class);
}
private int bitField0_;
public static final int PORT_FIELD_NUMBER = 1;
private int port_;
/**
*
* Port to send the HTTP request.
*
*
* required uint32 port = 1;
*/
public boolean hasPort() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
*
* Port to send the HTTP request.
*
*
* required uint32 port = 1;
*/
public int getPort() {
return port_;
}
public static final int PATH_FIELD_NUMBER = 2;
private volatile java.lang.Object path_;
/**
*
* HTTP request path.
*
*
* optional string path = 2;
*/
public boolean hasPath() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
*
* HTTP request path.
*
*
* optional string path = 2;
*/
public java.lang.String getPath() {
java.lang.Object ref = path_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
path_ = s;
}
return s;
}
}
/**
*
* HTTP request path.
*
*
* optional string path = 2;
*/
public com.google.protobuf.ByteString
getPathBytes() {
java.lang.Object ref = path_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
path_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasPort()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeUInt32(1, port_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, path_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(1, port_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, path_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.mesos.v1.Protos.CheckInfo.Http)) {
return super.equals(obj);
}
org.apache.mesos.v1.Protos.CheckInfo.Http other = (org.apache.mesos.v1.Protos.CheckInfo.Http) obj;
boolean result = true;
result = result && (hasPort() == other.hasPort());
if (hasPort()) {
result = result && (getPort()
== other.getPort());
}
result = result && (hasPath() == other.hasPath());
if (hasPath()) {
result = result && getPath()
.equals(other.getPath());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasPort()) {
hash = (37 * hash) + PORT_FIELD_NUMBER;
hash = (53 * hash) + getPort();
}
if (hasPath()) {
hash = (37 * hash) + PATH_FIELD_NUMBER;
hash = (53 * hash) + getPath().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.mesos.v1.Protos.CheckInfo.Http parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.CheckInfo.Http parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.CheckInfo.Http parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.CheckInfo.Http parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.CheckInfo.Http parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.CheckInfo.Http parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.CheckInfo.Http parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.CheckInfo.Http parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.CheckInfo.Http parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.CheckInfo.Http parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.CheckInfo.Http parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.CheckInfo.Http parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.apache.mesos.v1.Protos.CheckInfo.Http prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Describes an HTTP check. Sends a GET request to
* http://<host>:port/path. Note that <host> is not configurable and is
* resolved automatically to 127.0.0.1.
*
*
* Protobuf type {@code mesos.v1.CheckInfo.Http}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:mesos.v1.CheckInfo.Http)
org.apache.mesos.v1.Protos.CheckInfo.HttpOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_CheckInfo_Http_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_CheckInfo_Http_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.mesos.v1.Protos.CheckInfo.Http.class, org.apache.mesos.v1.Protos.CheckInfo.Http.Builder.class);
}
// Construct using org.apache.mesos.v1.Protos.CheckInfo.Http.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
port_ = 0;
bitField0_ = (bitField0_ & ~0x00000001);
path_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_CheckInfo_Http_descriptor;
}
public org.apache.mesos.v1.Protos.CheckInfo.Http getDefaultInstanceForType() {
return org.apache.mesos.v1.Protos.CheckInfo.Http.getDefaultInstance();
}
public org.apache.mesos.v1.Protos.CheckInfo.Http build() {
org.apache.mesos.v1.Protos.CheckInfo.Http result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.apache.mesos.v1.Protos.CheckInfo.Http buildPartial() {
org.apache.mesos.v1.Protos.CheckInfo.Http result = new org.apache.mesos.v1.Protos.CheckInfo.Http(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.port_ = port_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.path_ = path_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.apache.mesos.v1.Protos.CheckInfo.Http) {
return mergeFrom((org.apache.mesos.v1.Protos.CheckInfo.Http)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.mesos.v1.Protos.CheckInfo.Http other) {
if (other == org.apache.mesos.v1.Protos.CheckInfo.Http.getDefaultInstance()) return this;
if (other.hasPort()) {
setPort(other.getPort());
}
if (other.hasPath()) {
bitField0_ |= 0x00000002;
path_ = other.path_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
if (!hasPort()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.mesos.v1.Protos.CheckInfo.Http parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.mesos.v1.Protos.CheckInfo.Http) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private int port_ ;
/**
*
* Port to send the HTTP request.
*
*
* required uint32 port = 1;
*/
public boolean hasPort() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
*
* Port to send the HTTP request.
*
*
* required uint32 port = 1;
*/
public int getPort() {
return port_;
}
/**
*
* Port to send the HTTP request.
*
*
* required uint32 port = 1;
*/
public Builder setPort(int value) {
bitField0_ |= 0x00000001;
port_ = value;
onChanged();
return this;
}
/**
*
* Port to send the HTTP request.
*
*
* required uint32 port = 1;
*/
public Builder clearPort() {
bitField0_ = (bitField0_ & ~0x00000001);
port_ = 0;
onChanged();
return this;
}
private java.lang.Object path_ = "";
/**
*
* HTTP request path.
*
*
* optional string path = 2;
*/
public boolean hasPath() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
*
* HTTP request path.
*
*
* optional string path = 2;
*/
public java.lang.String getPath() {
java.lang.Object ref = path_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
path_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* HTTP request path.
*
*
* optional string path = 2;
*/
public com.google.protobuf.ByteString
getPathBytes() {
java.lang.Object ref = path_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
path_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* HTTP request path.
*
*
* optional string path = 2;
*/
public Builder setPath(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
path_ = value;
onChanged();
return this;
}
/**
*
* HTTP request path.
*
*
* optional string path = 2;
*/
public Builder clearPath() {
bitField0_ = (bitField0_ & ~0x00000002);
path_ = getDefaultInstance().getPath();
onChanged();
return this;
}
/**
*
* HTTP request path.
*
*
* optional string path = 2;
*/
public Builder setPathBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
path_ = value;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:mesos.v1.CheckInfo.Http)
}
// @@protoc_insertion_point(class_scope:mesos.v1.CheckInfo.Http)
private static final org.apache.mesos.v1.Protos.CheckInfo.Http DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.apache.mesos.v1.Protos.CheckInfo.Http();
}
public static org.apache.mesos.v1.Protos.CheckInfo.Http getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public Http parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Http(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.apache.mesos.v1.Protos.CheckInfo.Http getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface TcpOrBuilder extends
// @@protoc_insertion_point(interface_extends:mesos.v1.CheckInfo.Tcp)
com.google.protobuf.MessageOrBuilder {
/**
* required uint32 port = 1;
*/
boolean hasPort();
/**
* required uint32 port = 1;
*/
int getPort();
}
/**
*
* Describes a TCP check, i.e. based on establishing a TCP connection to
* the specified port. Note that <host> is not configurable and is resolved
* automatically to 127.0.0.1.
*
*
* Protobuf type {@code mesos.v1.CheckInfo.Tcp}
*/
public static final class Tcp extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:mesos.v1.CheckInfo.Tcp)
TcpOrBuilder {
private static final long serialVersionUID = 0L;
// Use Tcp.newBuilder() to construct.
private Tcp(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Tcp() {
port_ = 0;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Tcp(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
bitField0_ |= 0x00000001;
port_ = input.readUInt32();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_CheckInfo_Tcp_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_CheckInfo_Tcp_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.mesos.v1.Protos.CheckInfo.Tcp.class, org.apache.mesos.v1.Protos.CheckInfo.Tcp.Builder.class);
}
private int bitField0_;
public static final int PORT_FIELD_NUMBER = 1;
private int port_;
/**
* required uint32 port = 1;
*/
public boolean hasPort() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required uint32 port = 1;
*/
public int getPort() {
return port_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasPort()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeUInt32(1, port_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(1, port_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.mesos.v1.Protos.CheckInfo.Tcp)) {
return super.equals(obj);
}
org.apache.mesos.v1.Protos.CheckInfo.Tcp other = (org.apache.mesos.v1.Protos.CheckInfo.Tcp) obj;
boolean result = true;
result = result && (hasPort() == other.hasPort());
if (hasPort()) {
result = result && (getPort()
== other.getPort());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasPort()) {
hash = (37 * hash) + PORT_FIELD_NUMBER;
hash = (53 * hash) + getPort();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.mesos.v1.Protos.CheckInfo.Tcp parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.CheckInfo.Tcp parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.CheckInfo.Tcp parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.CheckInfo.Tcp parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.CheckInfo.Tcp parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.CheckInfo.Tcp parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.CheckInfo.Tcp parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.CheckInfo.Tcp parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.CheckInfo.Tcp parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.CheckInfo.Tcp parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.CheckInfo.Tcp parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.CheckInfo.Tcp parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.apache.mesos.v1.Protos.CheckInfo.Tcp prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Describes a TCP check, i.e. based on establishing a TCP connection to
* the specified port. Note that <host> is not configurable and is resolved
* automatically to 127.0.0.1.
*
*
* Protobuf type {@code mesos.v1.CheckInfo.Tcp}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:mesos.v1.CheckInfo.Tcp)
org.apache.mesos.v1.Protos.CheckInfo.TcpOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_CheckInfo_Tcp_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_CheckInfo_Tcp_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.mesos.v1.Protos.CheckInfo.Tcp.class, org.apache.mesos.v1.Protos.CheckInfo.Tcp.Builder.class);
}
// Construct using org.apache.mesos.v1.Protos.CheckInfo.Tcp.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
port_ = 0;
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_CheckInfo_Tcp_descriptor;
}
public org.apache.mesos.v1.Protos.CheckInfo.Tcp getDefaultInstanceForType() {
return org.apache.mesos.v1.Protos.CheckInfo.Tcp.getDefaultInstance();
}
public org.apache.mesos.v1.Protos.CheckInfo.Tcp build() {
org.apache.mesos.v1.Protos.CheckInfo.Tcp result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.apache.mesos.v1.Protos.CheckInfo.Tcp buildPartial() {
org.apache.mesos.v1.Protos.CheckInfo.Tcp result = new org.apache.mesos.v1.Protos.CheckInfo.Tcp(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.port_ = port_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.apache.mesos.v1.Protos.CheckInfo.Tcp) {
return mergeFrom((org.apache.mesos.v1.Protos.CheckInfo.Tcp)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.mesos.v1.Protos.CheckInfo.Tcp other) {
if (other == org.apache.mesos.v1.Protos.CheckInfo.Tcp.getDefaultInstance()) return this;
if (other.hasPort()) {
setPort(other.getPort());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
if (!hasPort()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.mesos.v1.Protos.CheckInfo.Tcp parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.mesos.v1.Protos.CheckInfo.Tcp) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private int port_ ;
/**
* required uint32 port = 1;
*/
public boolean hasPort() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required uint32 port = 1;
*/
public int getPort() {
return port_;
}
/**
* required uint32 port = 1;
*/
public Builder setPort(int value) {
bitField0_ |= 0x00000001;
port_ = value;
onChanged();
return this;
}
/**
* required uint32 port = 1;
*/
public Builder clearPort() {
bitField0_ = (bitField0_ & ~0x00000001);
port_ = 0;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:mesos.v1.CheckInfo.Tcp)
}
// @@protoc_insertion_point(class_scope:mesos.v1.CheckInfo.Tcp)
private static final org.apache.mesos.v1.Protos.CheckInfo.Tcp DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.apache.mesos.v1.Protos.CheckInfo.Tcp();
}
public static org.apache.mesos.v1.Protos.CheckInfo.Tcp getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public Tcp parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Tcp(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.apache.mesos.v1.Protos.CheckInfo.Tcp getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private int bitField0_;
public static final int TYPE_FIELD_NUMBER = 1;
private int type_;
/**
*
* The type of the check.
*
*
* optional .mesos.v1.CheckInfo.Type type = 1;
*/
public boolean hasType() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
*
* The type of the check.
*
*
* optional .mesos.v1.CheckInfo.Type type = 1;
*/
public org.apache.mesos.v1.Protos.CheckInfo.Type getType() {
org.apache.mesos.v1.Protos.CheckInfo.Type result = org.apache.mesos.v1.Protos.CheckInfo.Type.valueOf(type_);
return result == null ? org.apache.mesos.v1.Protos.CheckInfo.Type.UNKNOWN : result;
}
public static final int COMMAND_FIELD_NUMBER = 2;
private org.apache.mesos.v1.Protos.CheckInfo.Command command_;
/**
*
* Command check.
*
*
* optional .mesos.v1.CheckInfo.Command command = 2;
*/
public boolean hasCommand() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
*
* Command check.
*
*
* optional .mesos.v1.CheckInfo.Command command = 2;
*/
public org.apache.mesos.v1.Protos.CheckInfo.Command getCommand() {
return command_ == null ? org.apache.mesos.v1.Protos.CheckInfo.Command.getDefaultInstance() : command_;
}
/**
*
* Command check.
*
*
* optional .mesos.v1.CheckInfo.Command command = 2;
*/
public org.apache.mesos.v1.Protos.CheckInfo.CommandOrBuilder getCommandOrBuilder() {
return command_ == null ? org.apache.mesos.v1.Protos.CheckInfo.Command.getDefaultInstance() : command_;
}
public static final int HTTP_FIELD_NUMBER = 3;
private org.apache.mesos.v1.Protos.CheckInfo.Http http_;
/**
*
* HTTP check.
*
*
* optional .mesos.v1.CheckInfo.Http http = 3;
*/
public boolean hasHttp() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
*
* HTTP check.
*
*
* optional .mesos.v1.CheckInfo.Http http = 3;
*/
public org.apache.mesos.v1.Protos.CheckInfo.Http getHttp() {
return http_ == null ? org.apache.mesos.v1.Protos.CheckInfo.Http.getDefaultInstance() : http_;
}
/**
*
* HTTP check.
*
*
* optional .mesos.v1.CheckInfo.Http http = 3;
*/
public org.apache.mesos.v1.Protos.CheckInfo.HttpOrBuilder getHttpOrBuilder() {
return http_ == null ? org.apache.mesos.v1.Protos.CheckInfo.Http.getDefaultInstance() : http_;
}
public static final int TCP_FIELD_NUMBER = 7;
private org.apache.mesos.v1.Protos.CheckInfo.Tcp tcp_;
/**
*
* TCP check.
*
*
* optional .mesos.v1.CheckInfo.Tcp tcp = 7;
*/
public boolean hasTcp() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
*
* TCP check.
*
*
* optional .mesos.v1.CheckInfo.Tcp tcp = 7;
*/
public org.apache.mesos.v1.Protos.CheckInfo.Tcp getTcp() {
return tcp_ == null ? org.apache.mesos.v1.Protos.CheckInfo.Tcp.getDefaultInstance() : tcp_;
}
/**
*
* TCP check.
*
*
* optional .mesos.v1.CheckInfo.Tcp tcp = 7;
*/
public org.apache.mesos.v1.Protos.CheckInfo.TcpOrBuilder getTcpOrBuilder() {
return tcp_ == null ? org.apache.mesos.v1.Protos.CheckInfo.Tcp.getDefaultInstance() : tcp_;
}
public static final int DELAY_SECONDS_FIELD_NUMBER = 4;
private double delaySeconds_;
/**
*
* Amount of time to wait to start checking the task after it
* transitions to `TASK_RUNNING` or `TASK_STARTING` if the latter
* is used by the executor.
*
*
* optional double delay_seconds = 4 [default = 15];
*/
public boolean hasDelaySeconds() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
*
* Amount of time to wait to start checking the task after it
* transitions to `TASK_RUNNING` or `TASK_STARTING` if the latter
* is used by the executor.
*
*
* optional double delay_seconds = 4 [default = 15];
*/
public double getDelaySeconds() {
return delaySeconds_;
}
public static final int INTERVAL_SECONDS_FIELD_NUMBER = 5;
private double intervalSeconds_;
/**
*
* Interval between check attempts, i.e., amount of time to wait after
* the previous check finished or timed out to start the next check.
*
*
* optional double interval_seconds = 5 [default = 10];
*/
public boolean hasIntervalSeconds() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
*
* Interval between check attempts, i.e., amount of time to wait after
* the previous check finished or timed out to start the next check.
*
*
* optional double interval_seconds = 5 [default = 10];
*/
public double getIntervalSeconds() {
return intervalSeconds_;
}
public static final int TIMEOUT_SECONDS_FIELD_NUMBER = 6;
private double timeoutSeconds_;
/**
*
* Amount of time to wait for the check to complete. Zero means infinite
* timeout.
* After this timeout, the check attempt is aborted and no result is
* reported. Note that this may be considered a state change and hence
* may trigger a check status change delivery to the corresponding
* scheduler. See `CheckStatusInfo` for more details.
*
*
* optional double timeout_seconds = 6 [default = 20];
*/
public boolean hasTimeoutSeconds() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
*
* Amount of time to wait for the check to complete. Zero means infinite
* timeout.
* After this timeout, the check attempt is aborted and no result is
* reported. Note that this may be considered a state change and hence
* may trigger a check status change delivery to the corresponding
* scheduler. See `CheckStatusInfo` for more details.
*
*
* optional double timeout_seconds = 6 [default = 20];
*/
public double getTimeoutSeconds() {
return timeoutSeconds_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (hasCommand()) {
if (!getCommand().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
if (hasHttp()) {
if (!getHttp().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
if (hasTcp()) {
if (!getTcp().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeEnum(1, type_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeMessage(2, getCommand());
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeMessage(3, getHttp());
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
output.writeDouble(4, delaySeconds_);
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
output.writeDouble(5, intervalSeconds_);
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
output.writeDouble(6, timeoutSeconds_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeMessage(7, getTcp());
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(1, type_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getCommand());
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getHttp());
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
size += com.google.protobuf.CodedOutputStream
.computeDoubleSize(4, delaySeconds_);
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
size += com.google.protobuf.CodedOutputStream
.computeDoubleSize(5, intervalSeconds_);
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
size += com.google.protobuf.CodedOutputStream
.computeDoubleSize(6, timeoutSeconds_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(7, getTcp());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.mesos.v1.Protos.CheckInfo)) {
return super.equals(obj);
}
org.apache.mesos.v1.Protos.CheckInfo other = (org.apache.mesos.v1.Protos.CheckInfo) obj;
boolean result = true;
result = result && (hasType() == other.hasType());
if (hasType()) {
result = result && type_ == other.type_;
}
result = result && (hasCommand() == other.hasCommand());
if (hasCommand()) {
result = result && getCommand()
.equals(other.getCommand());
}
result = result && (hasHttp() == other.hasHttp());
if (hasHttp()) {
result = result && getHttp()
.equals(other.getHttp());
}
result = result && (hasTcp() == other.hasTcp());
if (hasTcp()) {
result = result && getTcp()
.equals(other.getTcp());
}
result = result && (hasDelaySeconds() == other.hasDelaySeconds());
if (hasDelaySeconds()) {
result = result && (
java.lang.Double.doubleToLongBits(getDelaySeconds())
== java.lang.Double.doubleToLongBits(
other.getDelaySeconds()));
}
result = result && (hasIntervalSeconds() == other.hasIntervalSeconds());
if (hasIntervalSeconds()) {
result = result && (
java.lang.Double.doubleToLongBits(getIntervalSeconds())
== java.lang.Double.doubleToLongBits(
other.getIntervalSeconds()));
}
result = result && (hasTimeoutSeconds() == other.hasTimeoutSeconds());
if (hasTimeoutSeconds()) {
result = result && (
java.lang.Double.doubleToLongBits(getTimeoutSeconds())
== java.lang.Double.doubleToLongBits(
other.getTimeoutSeconds()));
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasType()) {
hash = (37 * hash) + TYPE_FIELD_NUMBER;
hash = (53 * hash) + type_;
}
if (hasCommand()) {
hash = (37 * hash) + COMMAND_FIELD_NUMBER;
hash = (53 * hash) + getCommand().hashCode();
}
if (hasHttp()) {
hash = (37 * hash) + HTTP_FIELD_NUMBER;
hash = (53 * hash) + getHttp().hashCode();
}
if (hasTcp()) {
hash = (37 * hash) + TCP_FIELD_NUMBER;
hash = (53 * hash) + getTcp().hashCode();
}
if (hasDelaySeconds()) {
hash = (37 * hash) + DELAY_SECONDS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
java.lang.Double.doubleToLongBits(getDelaySeconds()));
}
if (hasIntervalSeconds()) {
hash = (37 * hash) + INTERVAL_SECONDS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
java.lang.Double.doubleToLongBits(getIntervalSeconds()));
}
if (hasTimeoutSeconds()) {
hash = (37 * hash) + TIMEOUT_SECONDS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
java.lang.Double.doubleToLongBits(getTimeoutSeconds()));
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.mesos.v1.Protos.CheckInfo parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.CheckInfo parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.CheckInfo parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.CheckInfo parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.CheckInfo parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.CheckInfo parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.CheckInfo parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.CheckInfo parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.CheckInfo parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.CheckInfo parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.CheckInfo parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.CheckInfo parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.apache.mesos.v1.Protos.CheckInfo prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
**
* Describes a general non-interpreting non-killing check for a task or
* executor (or any arbitrary process/command). A type is picked by
* specifying one of the optional fields. Specifying more than one type
* is an error.
* NOTE: This API is subject to change and the related feature is experimental.
*
*
* Protobuf type {@code mesos.v1.CheckInfo}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:mesos.v1.CheckInfo)
org.apache.mesos.v1.Protos.CheckInfoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_CheckInfo_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_CheckInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.mesos.v1.Protos.CheckInfo.class, org.apache.mesos.v1.Protos.CheckInfo.Builder.class);
}
// Construct using org.apache.mesos.v1.Protos.CheckInfo.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getCommandFieldBuilder();
getHttpFieldBuilder();
getTcpFieldBuilder();
}
}
public Builder clear() {
super.clear();
type_ = 0;
bitField0_ = (bitField0_ & ~0x00000001);
if (commandBuilder_ == null) {
command_ = null;
} else {
commandBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
if (httpBuilder_ == null) {
http_ = null;
} else {
httpBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000004);
if (tcpBuilder_ == null) {
tcp_ = null;
} else {
tcpBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000008);
delaySeconds_ = 15D;
bitField0_ = (bitField0_ & ~0x00000010);
intervalSeconds_ = 10D;
bitField0_ = (bitField0_ & ~0x00000020);
timeoutSeconds_ = 20D;
bitField0_ = (bitField0_ & ~0x00000040);
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_CheckInfo_descriptor;
}
public org.apache.mesos.v1.Protos.CheckInfo getDefaultInstanceForType() {
return org.apache.mesos.v1.Protos.CheckInfo.getDefaultInstance();
}
public org.apache.mesos.v1.Protos.CheckInfo build() {
org.apache.mesos.v1.Protos.CheckInfo result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.apache.mesos.v1.Protos.CheckInfo buildPartial() {
org.apache.mesos.v1.Protos.CheckInfo result = new org.apache.mesos.v1.Protos.CheckInfo(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.type_ = type_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
if (commandBuilder_ == null) {
result.command_ = command_;
} else {
result.command_ = commandBuilder_.build();
}
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
if (httpBuilder_ == null) {
result.http_ = http_;
} else {
result.http_ = httpBuilder_.build();
}
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000008;
}
if (tcpBuilder_ == null) {
result.tcp_ = tcp_;
} else {
result.tcp_ = tcpBuilder_.build();
}
if (((from_bitField0_ & 0x00000010) == 0x00000010)) {
to_bitField0_ |= 0x00000010;
}
result.delaySeconds_ = delaySeconds_;
if (((from_bitField0_ & 0x00000020) == 0x00000020)) {
to_bitField0_ |= 0x00000020;
}
result.intervalSeconds_ = intervalSeconds_;
if (((from_bitField0_ & 0x00000040) == 0x00000040)) {
to_bitField0_ |= 0x00000040;
}
result.timeoutSeconds_ = timeoutSeconds_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.apache.mesos.v1.Protos.CheckInfo) {
return mergeFrom((org.apache.mesos.v1.Protos.CheckInfo)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.mesos.v1.Protos.CheckInfo other) {
if (other == org.apache.mesos.v1.Protos.CheckInfo.getDefaultInstance()) return this;
if (other.hasType()) {
setType(other.getType());
}
if (other.hasCommand()) {
mergeCommand(other.getCommand());
}
if (other.hasHttp()) {
mergeHttp(other.getHttp());
}
if (other.hasTcp()) {
mergeTcp(other.getTcp());
}
if (other.hasDelaySeconds()) {
setDelaySeconds(other.getDelaySeconds());
}
if (other.hasIntervalSeconds()) {
setIntervalSeconds(other.getIntervalSeconds());
}
if (other.hasTimeoutSeconds()) {
setTimeoutSeconds(other.getTimeoutSeconds());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
if (hasCommand()) {
if (!getCommand().isInitialized()) {
return false;
}
}
if (hasHttp()) {
if (!getHttp().isInitialized()) {
return false;
}
}
if (hasTcp()) {
if (!getTcp().isInitialized()) {
return false;
}
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.mesos.v1.Protos.CheckInfo parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.mesos.v1.Protos.CheckInfo) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private int type_ = 0;
/**
*
* The type of the check.
*
*
* optional .mesos.v1.CheckInfo.Type type = 1;
*/
public boolean hasType() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
*
* The type of the check.
*
*
* optional .mesos.v1.CheckInfo.Type type = 1;
*/
public org.apache.mesos.v1.Protos.CheckInfo.Type getType() {
org.apache.mesos.v1.Protos.CheckInfo.Type result = org.apache.mesos.v1.Protos.CheckInfo.Type.valueOf(type_);
return result == null ? org.apache.mesos.v1.Protos.CheckInfo.Type.UNKNOWN : result;
}
/**
*
* The type of the check.
*
*
* optional .mesos.v1.CheckInfo.Type type = 1;
*/
public Builder setType(org.apache.mesos.v1.Protos.CheckInfo.Type value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
type_ = value.getNumber();
onChanged();
return this;
}
/**
*
* The type of the check.
*
*
* optional .mesos.v1.CheckInfo.Type type = 1;
*/
public Builder clearType() {
bitField0_ = (bitField0_ & ~0x00000001);
type_ = 0;
onChanged();
return this;
}
private org.apache.mesos.v1.Protos.CheckInfo.Command command_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
org.apache.mesos.v1.Protos.CheckInfo.Command, org.apache.mesos.v1.Protos.CheckInfo.Command.Builder, org.apache.mesos.v1.Protos.CheckInfo.CommandOrBuilder> commandBuilder_;
/**
*
* Command check.
*
*
* optional .mesos.v1.CheckInfo.Command command = 2;
*/
public boolean hasCommand() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
*
* Command check.
*
*
* optional .mesos.v1.CheckInfo.Command command = 2;
*/
public org.apache.mesos.v1.Protos.CheckInfo.Command getCommand() {
if (commandBuilder_ == null) {
return command_ == null ? org.apache.mesos.v1.Protos.CheckInfo.Command.getDefaultInstance() : command_;
} else {
return commandBuilder_.getMessage();
}
}
/**
*
* Command check.
*
*
* optional .mesos.v1.CheckInfo.Command command = 2;
*/
public Builder setCommand(org.apache.mesos.v1.Protos.CheckInfo.Command value) {
if (commandBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
command_ = value;
onChanged();
} else {
commandBuilder_.setMessage(value);
}
bitField0_ |= 0x00000002;
return this;
}
/**
*
* Command check.
*
*
* optional .mesos.v1.CheckInfo.Command command = 2;
*/
public Builder setCommand(
org.apache.mesos.v1.Protos.CheckInfo.Command.Builder builderForValue) {
if (commandBuilder_ == null) {
command_ = builderForValue.build();
onChanged();
} else {
commandBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000002;
return this;
}
/**
*
* Command check.
*
*
* optional .mesos.v1.CheckInfo.Command command = 2;
*/
public Builder mergeCommand(org.apache.mesos.v1.Protos.CheckInfo.Command value) {
if (commandBuilder_ == null) {
if (((bitField0_ & 0x00000002) == 0x00000002) &&
command_ != null &&
command_ != org.apache.mesos.v1.Protos.CheckInfo.Command.getDefaultInstance()) {
command_ =
org.apache.mesos.v1.Protos.CheckInfo.Command.newBuilder(command_).mergeFrom(value).buildPartial();
} else {
command_ = value;
}
onChanged();
} else {
commandBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000002;
return this;
}
/**
*
* Command check.
*
*
* optional .mesos.v1.CheckInfo.Command command = 2;
*/
public Builder clearCommand() {
if (commandBuilder_ == null) {
command_ = null;
onChanged();
} else {
commandBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
/**
*
* Command check.
*
*
* optional .mesos.v1.CheckInfo.Command command = 2;
*/
public org.apache.mesos.v1.Protos.CheckInfo.Command.Builder getCommandBuilder() {
bitField0_ |= 0x00000002;
onChanged();
return getCommandFieldBuilder().getBuilder();
}
/**
*
* Command check.
*
*
* optional .mesos.v1.CheckInfo.Command command = 2;
*/
public org.apache.mesos.v1.Protos.CheckInfo.CommandOrBuilder getCommandOrBuilder() {
if (commandBuilder_ != null) {
return commandBuilder_.getMessageOrBuilder();
} else {
return command_ == null ?
org.apache.mesos.v1.Protos.CheckInfo.Command.getDefaultInstance() : command_;
}
}
/**
*
* Command check.
*
*
* optional .mesos.v1.CheckInfo.Command command = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.apache.mesos.v1.Protos.CheckInfo.Command, org.apache.mesos.v1.Protos.CheckInfo.Command.Builder, org.apache.mesos.v1.Protos.CheckInfo.CommandOrBuilder>
getCommandFieldBuilder() {
if (commandBuilder_ == null) {
commandBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.apache.mesos.v1.Protos.CheckInfo.Command, org.apache.mesos.v1.Protos.CheckInfo.Command.Builder, org.apache.mesos.v1.Protos.CheckInfo.CommandOrBuilder>(
getCommand(),
getParentForChildren(),
isClean());
command_ = null;
}
return commandBuilder_;
}
private org.apache.mesos.v1.Protos.CheckInfo.Http http_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
org.apache.mesos.v1.Protos.CheckInfo.Http, org.apache.mesos.v1.Protos.CheckInfo.Http.Builder, org.apache.mesos.v1.Protos.CheckInfo.HttpOrBuilder> httpBuilder_;
/**
*
* HTTP check.
*
*
* optional .mesos.v1.CheckInfo.Http http = 3;
*/
public boolean hasHttp() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
*
* HTTP check.
*
*
* optional .mesos.v1.CheckInfo.Http http = 3;
*/
public org.apache.mesos.v1.Protos.CheckInfo.Http getHttp() {
if (httpBuilder_ == null) {
return http_ == null ? org.apache.mesos.v1.Protos.CheckInfo.Http.getDefaultInstance() : http_;
} else {
return httpBuilder_.getMessage();
}
}
/**
*
* HTTP check.
*
*
* optional .mesos.v1.CheckInfo.Http http = 3;
*/
public Builder setHttp(org.apache.mesos.v1.Protos.CheckInfo.Http value) {
if (httpBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
http_ = value;
onChanged();
} else {
httpBuilder_.setMessage(value);
}
bitField0_ |= 0x00000004;
return this;
}
/**
*
* HTTP check.
*
*
* optional .mesos.v1.CheckInfo.Http http = 3;
*/
public Builder setHttp(
org.apache.mesos.v1.Protos.CheckInfo.Http.Builder builderForValue) {
if (httpBuilder_ == null) {
http_ = builderForValue.build();
onChanged();
} else {
httpBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000004;
return this;
}
/**
*
* HTTP check.
*
*
* optional .mesos.v1.CheckInfo.Http http = 3;
*/
public Builder mergeHttp(org.apache.mesos.v1.Protos.CheckInfo.Http value) {
if (httpBuilder_ == null) {
if (((bitField0_ & 0x00000004) == 0x00000004) &&
http_ != null &&
http_ != org.apache.mesos.v1.Protos.CheckInfo.Http.getDefaultInstance()) {
http_ =
org.apache.mesos.v1.Protos.CheckInfo.Http.newBuilder(http_).mergeFrom(value).buildPartial();
} else {
http_ = value;
}
onChanged();
} else {
httpBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000004;
return this;
}
/**
*
* HTTP check.
*
*
* optional .mesos.v1.CheckInfo.Http http = 3;
*/
public Builder clearHttp() {
if (httpBuilder_ == null) {
http_ = null;
onChanged();
} else {
httpBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000004);
return this;
}
/**
*
* HTTP check.
*
*
* optional .mesos.v1.CheckInfo.Http http = 3;
*/
public org.apache.mesos.v1.Protos.CheckInfo.Http.Builder getHttpBuilder() {
bitField0_ |= 0x00000004;
onChanged();
return getHttpFieldBuilder().getBuilder();
}
/**
*
* HTTP check.
*
*
* optional .mesos.v1.CheckInfo.Http http = 3;
*/
public org.apache.mesos.v1.Protos.CheckInfo.HttpOrBuilder getHttpOrBuilder() {
if (httpBuilder_ != null) {
return httpBuilder_.getMessageOrBuilder();
} else {
return http_ == null ?
org.apache.mesos.v1.Protos.CheckInfo.Http.getDefaultInstance() : http_;
}
}
/**
*
* HTTP check.
*
*
* optional .mesos.v1.CheckInfo.Http http = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.apache.mesos.v1.Protos.CheckInfo.Http, org.apache.mesos.v1.Protos.CheckInfo.Http.Builder, org.apache.mesos.v1.Protos.CheckInfo.HttpOrBuilder>
getHttpFieldBuilder() {
if (httpBuilder_ == null) {
httpBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.apache.mesos.v1.Protos.CheckInfo.Http, org.apache.mesos.v1.Protos.CheckInfo.Http.Builder, org.apache.mesos.v1.Protos.CheckInfo.HttpOrBuilder>(
getHttp(),
getParentForChildren(),
isClean());
http_ = null;
}
return httpBuilder_;
}
private org.apache.mesos.v1.Protos.CheckInfo.Tcp tcp_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
org.apache.mesos.v1.Protos.CheckInfo.Tcp, org.apache.mesos.v1.Protos.CheckInfo.Tcp.Builder, org.apache.mesos.v1.Protos.CheckInfo.TcpOrBuilder> tcpBuilder_;
/**
*
* TCP check.
*
*
* optional .mesos.v1.CheckInfo.Tcp tcp = 7;
*/
public boolean hasTcp() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
*
* TCP check.
*
*
* optional .mesos.v1.CheckInfo.Tcp tcp = 7;
*/
public org.apache.mesos.v1.Protos.CheckInfo.Tcp getTcp() {
if (tcpBuilder_ == null) {
return tcp_ == null ? org.apache.mesos.v1.Protos.CheckInfo.Tcp.getDefaultInstance() : tcp_;
} else {
return tcpBuilder_.getMessage();
}
}
/**
*
* TCP check.
*
*
* optional .mesos.v1.CheckInfo.Tcp tcp = 7;
*/
public Builder setTcp(org.apache.mesos.v1.Protos.CheckInfo.Tcp value) {
if (tcpBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
tcp_ = value;
onChanged();
} else {
tcpBuilder_.setMessage(value);
}
bitField0_ |= 0x00000008;
return this;
}
/**
*
* TCP check.
*
*
* optional .mesos.v1.CheckInfo.Tcp tcp = 7;
*/
public Builder setTcp(
org.apache.mesos.v1.Protos.CheckInfo.Tcp.Builder builderForValue) {
if (tcpBuilder_ == null) {
tcp_ = builderForValue.build();
onChanged();
} else {
tcpBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000008;
return this;
}
/**
*
* TCP check.
*
*
* optional .mesos.v1.CheckInfo.Tcp tcp = 7;
*/
public Builder mergeTcp(org.apache.mesos.v1.Protos.CheckInfo.Tcp value) {
if (tcpBuilder_ == null) {
if (((bitField0_ & 0x00000008) == 0x00000008) &&
tcp_ != null &&
tcp_ != org.apache.mesos.v1.Protos.CheckInfo.Tcp.getDefaultInstance()) {
tcp_ =
org.apache.mesos.v1.Protos.CheckInfo.Tcp.newBuilder(tcp_).mergeFrom(value).buildPartial();
} else {
tcp_ = value;
}
onChanged();
} else {
tcpBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000008;
return this;
}
/**
*
* TCP check.
*
*
* optional .mesos.v1.CheckInfo.Tcp tcp = 7;
*/
public Builder clearTcp() {
if (tcpBuilder_ == null) {
tcp_ = null;
onChanged();
} else {
tcpBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000008);
return this;
}
/**
*
* TCP check.
*
*
* optional .mesos.v1.CheckInfo.Tcp tcp = 7;
*/
public org.apache.mesos.v1.Protos.CheckInfo.Tcp.Builder getTcpBuilder() {
bitField0_ |= 0x00000008;
onChanged();
return getTcpFieldBuilder().getBuilder();
}
/**
*
* TCP check.
*
*
* optional .mesos.v1.CheckInfo.Tcp tcp = 7;
*/
public org.apache.mesos.v1.Protos.CheckInfo.TcpOrBuilder getTcpOrBuilder() {
if (tcpBuilder_ != null) {
return tcpBuilder_.getMessageOrBuilder();
} else {
return tcp_ == null ?
org.apache.mesos.v1.Protos.CheckInfo.Tcp.getDefaultInstance() : tcp_;
}
}
/**
*
* TCP check.
*
*
* optional .mesos.v1.CheckInfo.Tcp tcp = 7;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.apache.mesos.v1.Protos.CheckInfo.Tcp, org.apache.mesos.v1.Protos.CheckInfo.Tcp.Builder, org.apache.mesos.v1.Protos.CheckInfo.TcpOrBuilder>
getTcpFieldBuilder() {
if (tcpBuilder_ == null) {
tcpBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.apache.mesos.v1.Protos.CheckInfo.Tcp, org.apache.mesos.v1.Protos.CheckInfo.Tcp.Builder, org.apache.mesos.v1.Protos.CheckInfo.TcpOrBuilder>(
getTcp(),
getParentForChildren(),
isClean());
tcp_ = null;
}
return tcpBuilder_;
}
private double delaySeconds_ = 15D;
/**
*
* Amount of time to wait to start checking the task after it
* transitions to `TASK_RUNNING` or `TASK_STARTING` if the latter
* is used by the executor.
*
*
* optional double delay_seconds = 4 [default = 15];
*/
public boolean hasDelaySeconds() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
*
* Amount of time to wait to start checking the task after it
* transitions to `TASK_RUNNING` or `TASK_STARTING` if the latter
* is used by the executor.
*
*
* optional double delay_seconds = 4 [default = 15];
*/
public double getDelaySeconds() {
return delaySeconds_;
}
/**
*
* Amount of time to wait to start checking the task after it
* transitions to `TASK_RUNNING` or `TASK_STARTING` if the latter
* is used by the executor.
*
*
* optional double delay_seconds = 4 [default = 15];
*/
public Builder setDelaySeconds(double value) {
bitField0_ |= 0x00000010;
delaySeconds_ = value;
onChanged();
return this;
}
/**
*
* Amount of time to wait to start checking the task after it
* transitions to `TASK_RUNNING` or `TASK_STARTING` if the latter
* is used by the executor.
*
*
* optional double delay_seconds = 4 [default = 15];
*/
public Builder clearDelaySeconds() {
bitField0_ = (bitField0_ & ~0x00000010);
delaySeconds_ = 15D;
onChanged();
return this;
}
private double intervalSeconds_ = 10D;
/**
*
* Interval between check attempts, i.e., amount of time to wait after
* the previous check finished or timed out to start the next check.
*
*
* optional double interval_seconds = 5 [default = 10];
*/
public boolean hasIntervalSeconds() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
*
* Interval between check attempts, i.e., amount of time to wait after
* the previous check finished or timed out to start the next check.
*
*
* optional double interval_seconds = 5 [default = 10];
*/
public double getIntervalSeconds() {
return intervalSeconds_;
}
/**
*
* Interval between check attempts, i.e., amount of time to wait after
* the previous check finished or timed out to start the next check.
*
*
* optional double interval_seconds = 5 [default = 10];
*/
public Builder setIntervalSeconds(double value) {
bitField0_ |= 0x00000020;
intervalSeconds_ = value;
onChanged();
return this;
}
/**
*
* Interval between check attempts, i.e., amount of time to wait after
* the previous check finished or timed out to start the next check.
*
*
* optional double interval_seconds = 5 [default = 10];
*/
public Builder clearIntervalSeconds() {
bitField0_ = (bitField0_ & ~0x00000020);
intervalSeconds_ = 10D;
onChanged();
return this;
}
private double timeoutSeconds_ = 20D;
/**
*
* Amount of time to wait for the check to complete. Zero means infinite
* timeout.
* After this timeout, the check attempt is aborted and no result is
* reported. Note that this may be considered a state change and hence
* may trigger a check status change delivery to the corresponding
* scheduler. See `CheckStatusInfo` for more details.
*
*
* optional double timeout_seconds = 6 [default = 20];
*/
public boolean hasTimeoutSeconds() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
*
* Amount of time to wait for the check to complete. Zero means infinite
* timeout.
* After this timeout, the check attempt is aborted and no result is
* reported. Note that this may be considered a state change and hence
* may trigger a check status change delivery to the corresponding
* scheduler. See `CheckStatusInfo` for more details.
*
*
* optional double timeout_seconds = 6 [default = 20];
*/
public double getTimeoutSeconds() {
return timeoutSeconds_;
}
/**
*
* Amount of time to wait for the check to complete. Zero means infinite
* timeout.
* After this timeout, the check attempt is aborted and no result is
* reported. Note that this may be considered a state change and hence
* may trigger a check status change delivery to the corresponding
* scheduler. See `CheckStatusInfo` for more details.
*
*
* optional double timeout_seconds = 6 [default = 20];
*/
public Builder setTimeoutSeconds(double value) {
bitField0_ |= 0x00000040;
timeoutSeconds_ = value;
onChanged();
return this;
}
/**
*
* Amount of time to wait for the check to complete. Zero means infinite
* timeout.
* After this timeout, the check attempt is aborted and no result is
* reported. Note that this may be considered a state change and hence
* may trigger a check status change delivery to the corresponding
* scheduler. See `CheckStatusInfo` for more details.
*
*
* optional double timeout_seconds = 6 [default = 20];
*/
public Builder clearTimeoutSeconds() {
bitField0_ = (bitField0_ & ~0x00000040);
timeoutSeconds_ = 20D;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:mesos.v1.CheckInfo)
}
// @@protoc_insertion_point(class_scope:mesos.v1.CheckInfo)
private static final org.apache.mesos.v1.Protos.CheckInfo DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.apache.mesos.v1.Protos.CheckInfo();
}
public static org.apache.mesos.v1.Protos.CheckInfo getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public CheckInfo parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new CheckInfo(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.apache.mesos.v1.Protos.CheckInfo getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface HealthCheckOrBuilder extends
// @@protoc_insertion_point(interface_extends:mesos.v1.HealthCheck)
com.google.protobuf.MessageOrBuilder {
/**
*
* Amount of time to wait to start health checking the task after it
* transitions to `TASK_RUNNING` or `TASK_STATING` if the latter is
* used by the executor.
*
*
* optional double delay_seconds = 2 [default = 15];
*/
boolean hasDelaySeconds();
/**
*
* Amount of time to wait to start health checking the task after it
* transitions to `TASK_RUNNING` or `TASK_STATING` if the latter is
* used by the executor.
*
*
* optional double delay_seconds = 2 [default = 15];
*/
double getDelaySeconds();
/**
*
* Interval between health checks, i.e., amount of time to wait after
* the previous health check finished or timed out to start the next
* health check.
*
*
* optional double interval_seconds = 3 [default = 10];
*/
boolean hasIntervalSeconds();
/**
*
* Interval between health checks, i.e., amount of time to wait after
* the previous health check finished or timed out to start the next
* health check.
*
*
* optional double interval_seconds = 3 [default = 10];
*/
double getIntervalSeconds();
/**
*
* Amount of time to wait for the health check to complete. After this
* timeout, the health check is aborted and treated as a failure. Zero
* means infinite timeout.
*
*
* optional double timeout_seconds = 4 [default = 20];
*/
boolean hasTimeoutSeconds();
/**
*
* Amount of time to wait for the health check to complete. After this
* timeout, the health check is aborted and treated as a failure. Zero
* means infinite timeout.
*
*
* optional double timeout_seconds = 4 [default = 20];
*/
double getTimeoutSeconds();
/**
*
* Number of consecutive failures until the task is killed by the executor.
*
*
* optional uint32 consecutive_failures = 5 [default = 3];
*/
boolean hasConsecutiveFailures();
/**
*
* Number of consecutive failures until the task is killed by the executor.
*
*
* optional uint32 consecutive_failures = 5 [default = 3];
*/
int getConsecutiveFailures();
/**
*
* Amount of time after the task is launched during which health check
* failures are ignored. Once a check succeeds for the first time,
* the grace period does not apply anymore. Note that it includes
* `delay_seconds`, i.e., setting `grace_period_seconds` < `delay_seconds`
* has no effect.
*
*
* optional double grace_period_seconds = 6 [default = 10];
*/
boolean hasGracePeriodSeconds();
/**
*
* Amount of time after the task is launched during which health check
* failures are ignored. Once a check succeeds for the first time,
* the grace period does not apply anymore. Note that it includes
* `delay_seconds`, i.e., setting `grace_period_seconds` < `delay_seconds`
* has no effect.
*
*
* optional double grace_period_seconds = 6 [default = 10];
*/
double getGracePeriodSeconds();
/**
*
* The type of health check.
*
*
* optional .mesos.v1.HealthCheck.Type type = 8;
*/
boolean hasType();
/**
*
* The type of health check.
*
*
* optional .mesos.v1.HealthCheck.Type type = 8;
*/
org.apache.mesos.v1.Protos.HealthCheck.Type getType();
/**
*
* Command health check.
*
*
* optional .mesos.v1.CommandInfo command = 7;
*/
boolean hasCommand();
/**
*
* Command health check.
*
*
* optional .mesos.v1.CommandInfo command = 7;
*/
org.apache.mesos.v1.Protos.CommandInfo getCommand();
/**
*
* Command health check.
*
*
* optional .mesos.v1.CommandInfo command = 7;
*/
org.apache.mesos.v1.Protos.CommandInfoOrBuilder getCommandOrBuilder();
/**
*
* HTTP health check.
*
*
* optional .mesos.v1.HealthCheck.HTTPCheckInfo http = 1;
*/
boolean hasHttp();
/**
*
* HTTP health check.
*
*
* optional .mesos.v1.HealthCheck.HTTPCheckInfo http = 1;
*/
org.apache.mesos.v1.Protos.HealthCheck.HTTPCheckInfo getHttp();
/**
*
* HTTP health check.
*
*
* optional .mesos.v1.HealthCheck.HTTPCheckInfo http = 1;
*/
org.apache.mesos.v1.Protos.HealthCheck.HTTPCheckInfoOrBuilder getHttpOrBuilder();
/**
*
* TCP health check.
*
*
* optional .mesos.v1.HealthCheck.TCPCheckInfo tcp = 9;
*/
boolean hasTcp();
/**
*
* TCP health check.
*
*
* optional .mesos.v1.HealthCheck.TCPCheckInfo tcp = 9;
*/
org.apache.mesos.v1.Protos.HealthCheck.TCPCheckInfo getTcp();
/**
*
* TCP health check.
*
*
* optional .mesos.v1.HealthCheck.TCPCheckInfo tcp = 9;
*/
org.apache.mesos.v1.Protos.HealthCheck.TCPCheckInfoOrBuilder getTcpOrBuilder();
}
/**
*
**
* Describes a health check for a task or executor (or any arbitrary
* process/command). A type is picked by specifying one of the
* optional fields. Specifying more than one type is an error.
*
*
* Protobuf type {@code mesos.v1.HealthCheck}
*/
public static final class HealthCheck extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:mesos.v1.HealthCheck)
HealthCheckOrBuilder {
private static final long serialVersionUID = 0L;
// Use HealthCheck.newBuilder() to construct.
private HealthCheck(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private HealthCheck() {
delaySeconds_ = 15D;
intervalSeconds_ = 10D;
timeoutSeconds_ = 20D;
consecutiveFailures_ = 3;
gracePeriodSeconds_ = 10D;
type_ = 0;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private HealthCheck(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
org.apache.mesos.v1.Protos.HealthCheck.HTTPCheckInfo.Builder subBuilder = null;
if (((bitField0_ & 0x00000080) == 0x00000080)) {
subBuilder = http_.toBuilder();
}
http_ = input.readMessage(org.apache.mesos.v1.Protos.HealthCheck.HTTPCheckInfo.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(http_);
http_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000080;
break;
}
case 17: {
bitField0_ |= 0x00000001;
delaySeconds_ = input.readDouble();
break;
}
case 25: {
bitField0_ |= 0x00000002;
intervalSeconds_ = input.readDouble();
break;
}
case 33: {
bitField0_ |= 0x00000004;
timeoutSeconds_ = input.readDouble();
break;
}
case 40: {
bitField0_ |= 0x00000008;
consecutiveFailures_ = input.readUInt32();
break;
}
case 49: {
bitField0_ |= 0x00000010;
gracePeriodSeconds_ = input.readDouble();
break;
}
case 58: {
org.apache.mesos.v1.Protos.CommandInfo.Builder subBuilder = null;
if (((bitField0_ & 0x00000040) == 0x00000040)) {
subBuilder = command_.toBuilder();
}
command_ = input.readMessage(org.apache.mesos.v1.Protos.CommandInfo.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(command_);
command_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000040;
break;
}
case 64: {
int rawValue = input.readEnum();
org.apache.mesos.v1.Protos.HealthCheck.Type value = org.apache.mesos.v1.Protos.HealthCheck.Type.valueOf(rawValue);
if (value == null) {
unknownFields.mergeVarintField(8, rawValue);
} else {
bitField0_ |= 0x00000020;
type_ = rawValue;
}
break;
}
case 74: {
org.apache.mesos.v1.Protos.HealthCheck.TCPCheckInfo.Builder subBuilder = null;
if (((bitField0_ & 0x00000100) == 0x00000100)) {
subBuilder = tcp_.toBuilder();
}
tcp_ = input.readMessage(org.apache.mesos.v1.Protos.HealthCheck.TCPCheckInfo.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(tcp_);
tcp_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000100;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_HealthCheck_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_HealthCheck_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.mesos.v1.Protos.HealthCheck.class, org.apache.mesos.v1.Protos.HealthCheck.Builder.class);
}
/**
* Protobuf enum {@code mesos.v1.HealthCheck.Type}
*/
public enum Type
implements com.google.protobuf.ProtocolMessageEnum {
/**
* UNKNOWN = 0;
*/
UNKNOWN(0),
/**
* COMMAND = 1;
*/
COMMAND(1),
/**
* HTTP = 2;
*/
HTTP(2),
/**
* TCP = 3;
*/
TCP(3),
;
/**
* UNKNOWN = 0;
*/
public static final int UNKNOWN_VALUE = 0;
/**
* COMMAND = 1;
*/
public static final int COMMAND_VALUE = 1;
/**
* HTTP = 2;
*/
public static final int HTTP_VALUE = 2;
/**
* TCP = 3;
*/
public static final int TCP_VALUE = 3;
public final int getNumber() {
return value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static Type valueOf(int value) {
return forNumber(value);
}
public static Type forNumber(int value) {
switch (value) {
case 0: return UNKNOWN;
case 1: return COMMAND;
case 2: return HTTP;
case 3: return TCP;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
Type> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public Type findValueByNumber(int number) {
return Type.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return org.apache.mesos.v1.Protos.HealthCheck.getDescriptor().getEnumTypes().get(0);
}
private static final Type[] VALUES = values();
public static Type valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int value;
private Type(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:mesos.v1.HealthCheck.Type)
}
public interface HTTPCheckInfoOrBuilder extends
// @@protoc_insertion_point(interface_extends:mesos.v1.HealthCheck.HTTPCheckInfo)
com.google.protobuf.MessageOrBuilder {
/**
* optional .mesos.v1.NetworkInfo.Protocol protocol = 5 [default = IPv4];
*/
boolean hasProtocol();
/**
* optional .mesos.v1.NetworkInfo.Protocol protocol = 5 [default = IPv4];
*/
org.apache.mesos.v1.Protos.NetworkInfo.Protocol getProtocol();
/**
*
* Currently "http" and "https" are supported.
*
*
* optional string scheme = 3;
*/
boolean hasScheme();
/**
*
* Currently "http" and "https" are supported.
*
*
* optional string scheme = 3;
*/
java.lang.String getScheme();
/**
*
* Currently "http" and "https" are supported.
*
*
* optional string scheme = 3;
*/
com.google.protobuf.ByteString
getSchemeBytes();
/**
*
* Port to send the HTTP request.
*
*
* required uint32 port = 1;
*/
boolean hasPort();
/**
*
* Port to send the HTTP request.
*
*
* required uint32 port = 1;
*/
int getPort();
/**
*
* HTTP request path.
*
*
* optional string path = 2;
*/
boolean hasPath();
/**
*
* HTTP request path.
*
*
* optional string path = 2;
*/
java.lang.String getPath();
/**
*
* HTTP request path.
*
*
* optional string path = 2;
*/
com.google.protobuf.ByteString
getPathBytes();
/**
*
* NOTE: It is up to the custom executor to interpret and act on this
* field. Setting this field has no effect on the default executors.
* TODO(haosdent): Deprecate this field when we add better support for
* success and possibly failure statuses, e.g. ranges of success and
* failure statuses.
*
*
* repeated uint32 statuses = 4;
*/
java.util.List getStatusesList();
/**
*
* NOTE: It is up to the custom executor to interpret and act on this
* field. Setting this field has no effect on the default executors.
* TODO(haosdent): Deprecate this field when we add better support for
* success and possibly failure statuses, e.g. ranges of success and
* failure statuses.
*
*
* repeated uint32 statuses = 4;
*/
int getStatusesCount();
/**
*
* NOTE: It is up to the custom executor to interpret and act on this
* field. Setting this field has no effect on the default executors.
* TODO(haosdent): Deprecate this field when we add better support for
* success and possibly failure statuses, e.g. ranges of success and
* failure statuses.
*
*
* repeated uint32 statuses = 4;
*/
int getStatuses(int index);
}
/**
*
* Describes an HTTP health check. Sends a GET request to
* scheme://<host>:port/path. Note that <host> is not configurable and is
* resolved automatically, in most cases to 127.0.0.1. Default executors
* treat return codes between 200 and 399 as success; custom executors
* may employ a different strategy, e.g. leveraging the `statuses` field.
*
*
* Protobuf type {@code mesos.v1.HealthCheck.HTTPCheckInfo}
*/
public static final class HTTPCheckInfo extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:mesos.v1.HealthCheck.HTTPCheckInfo)
HTTPCheckInfoOrBuilder {
private static final long serialVersionUID = 0L;
// Use HTTPCheckInfo.newBuilder() to construct.
private HTTPCheckInfo(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private HTTPCheckInfo() {
protocol_ = 1;
scheme_ = "";
port_ = 0;
path_ = "";
statuses_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private HTTPCheckInfo(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
bitField0_ |= 0x00000004;
port_ = input.readUInt32();
break;
}
case 18: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000008;
path_ = bs;
break;
}
case 26: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000002;
scheme_ = bs;
break;
}
case 32: {
if (!((mutable_bitField0_ & 0x00000010) == 0x00000010)) {
statuses_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000010;
}
statuses_.add(input.readUInt32());
break;
}
case 34: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!((mutable_bitField0_ & 0x00000010) == 0x00000010) && input.getBytesUntilLimit() > 0) {
statuses_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000010;
}
while (input.getBytesUntilLimit() > 0) {
statuses_.add(input.readUInt32());
}
input.popLimit(limit);
break;
}
case 40: {
int rawValue = input.readEnum();
org.apache.mesos.v1.Protos.NetworkInfo.Protocol value = org.apache.mesos.v1.Protos.NetworkInfo.Protocol.valueOf(rawValue);
if (value == null) {
unknownFields.mergeVarintField(5, rawValue);
} else {
bitField0_ |= 0x00000001;
protocol_ = rawValue;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000010) == 0x00000010)) {
statuses_ = java.util.Collections.unmodifiableList(statuses_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_HealthCheck_HTTPCheckInfo_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_HealthCheck_HTTPCheckInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.mesos.v1.Protos.HealthCheck.HTTPCheckInfo.class, org.apache.mesos.v1.Protos.HealthCheck.HTTPCheckInfo.Builder.class);
}
private int bitField0_;
public static final int PROTOCOL_FIELD_NUMBER = 5;
private int protocol_;
/**
* optional .mesos.v1.NetworkInfo.Protocol protocol = 5 [default = IPv4];
*/
public boolean hasProtocol() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional .mesos.v1.NetworkInfo.Protocol protocol = 5 [default = IPv4];
*/
public org.apache.mesos.v1.Protos.NetworkInfo.Protocol getProtocol() {
org.apache.mesos.v1.Protos.NetworkInfo.Protocol result = org.apache.mesos.v1.Protos.NetworkInfo.Protocol.valueOf(protocol_);
return result == null ? org.apache.mesos.v1.Protos.NetworkInfo.Protocol.IPv4 : result;
}
public static final int SCHEME_FIELD_NUMBER = 3;
private volatile java.lang.Object scheme_;
/**
*
* Currently "http" and "https" are supported.
*
*
* optional string scheme = 3;
*/
public boolean hasScheme() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
*
* Currently "http" and "https" are supported.
*
*
* optional string scheme = 3;
*/
public java.lang.String getScheme() {
java.lang.Object ref = scheme_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
scheme_ = s;
}
return s;
}
}
/**
*
* Currently "http" and "https" are supported.
*
*
* optional string scheme = 3;
*/
public com.google.protobuf.ByteString
getSchemeBytes() {
java.lang.Object ref = scheme_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
scheme_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int PORT_FIELD_NUMBER = 1;
private int port_;
/**
*
* Port to send the HTTP request.
*
*
* required uint32 port = 1;
*/
public boolean hasPort() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
*
* Port to send the HTTP request.
*
*
* required uint32 port = 1;
*/
public int getPort() {
return port_;
}
public static final int PATH_FIELD_NUMBER = 2;
private volatile java.lang.Object path_;
/**
*
* HTTP request path.
*
*
* optional string path = 2;
*/
public boolean hasPath() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
*
* HTTP request path.
*
*
* optional string path = 2;
*/
public java.lang.String getPath() {
java.lang.Object ref = path_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
path_ = s;
}
return s;
}
}
/**
*
* HTTP request path.
*
*
* optional string path = 2;
*/
public com.google.protobuf.ByteString
getPathBytes() {
java.lang.Object ref = path_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
path_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int STATUSES_FIELD_NUMBER = 4;
private java.util.List statuses_;
/**
*
* NOTE: It is up to the custom executor to interpret and act on this
* field. Setting this field has no effect on the default executors.
* TODO(haosdent): Deprecate this field when we add better support for
* success and possibly failure statuses, e.g. ranges of success and
* failure statuses.
*
*
* repeated uint32 statuses = 4;
*/
public java.util.List
getStatusesList() {
return statuses_;
}
/**
*
* NOTE: It is up to the custom executor to interpret and act on this
* field. Setting this field has no effect on the default executors.
* TODO(haosdent): Deprecate this field when we add better support for
* success and possibly failure statuses, e.g. ranges of success and
* failure statuses.
*
*
* repeated uint32 statuses = 4;
*/
public int getStatusesCount() {
return statuses_.size();
}
/**
*
* NOTE: It is up to the custom executor to interpret and act on this
* field. Setting this field has no effect on the default executors.
* TODO(haosdent): Deprecate this field when we add better support for
* success and possibly failure statuses, e.g. ranges of success and
* failure statuses.
*
*
* repeated uint32 statuses = 4;
*/
public int getStatuses(int index) {
return statuses_.get(index);
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasPort()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeUInt32(1, port_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, path_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, scheme_);
}
for (int i = 0; i < statuses_.size(); i++) {
output.writeUInt32(4, statuses_.get(i));
}
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeEnum(5, protocol_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(1, port_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, path_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, scheme_);
}
{
int dataSize = 0;
for (int i = 0; i < statuses_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeUInt32SizeNoTag(statuses_.get(i));
}
size += dataSize;
size += 1 * getStatusesList().size();
}
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(5, protocol_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.mesos.v1.Protos.HealthCheck.HTTPCheckInfo)) {
return super.equals(obj);
}
org.apache.mesos.v1.Protos.HealthCheck.HTTPCheckInfo other = (org.apache.mesos.v1.Protos.HealthCheck.HTTPCheckInfo) obj;
boolean result = true;
result = result && (hasProtocol() == other.hasProtocol());
if (hasProtocol()) {
result = result && protocol_ == other.protocol_;
}
result = result && (hasScheme() == other.hasScheme());
if (hasScheme()) {
result = result && getScheme()
.equals(other.getScheme());
}
result = result && (hasPort() == other.hasPort());
if (hasPort()) {
result = result && (getPort()
== other.getPort());
}
result = result && (hasPath() == other.hasPath());
if (hasPath()) {
result = result && getPath()
.equals(other.getPath());
}
result = result && getStatusesList()
.equals(other.getStatusesList());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasProtocol()) {
hash = (37 * hash) + PROTOCOL_FIELD_NUMBER;
hash = (53 * hash) + protocol_;
}
if (hasScheme()) {
hash = (37 * hash) + SCHEME_FIELD_NUMBER;
hash = (53 * hash) + getScheme().hashCode();
}
if (hasPort()) {
hash = (37 * hash) + PORT_FIELD_NUMBER;
hash = (53 * hash) + getPort();
}
if (hasPath()) {
hash = (37 * hash) + PATH_FIELD_NUMBER;
hash = (53 * hash) + getPath().hashCode();
}
if (getStatusesCount() > 0) {
hash = (37 * hash) + STATUSES_FIELD_NUMBER;
hash = (53 * hash) + getStatusesList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.mesos.v1.Protos.HealthCheck.HTTPCheckInfo parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.HealthCheck.HTTPCheckInfo parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.HealthCheck.HTTPCheckInfo parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.HealthCheck.HTTPCheckInfo parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.HealthCheck.HTTPCheckInfo parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.HealthCheck.HTTPCheckInfo parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.HealthCheck.HTTPCheckInfo parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.HealthCheck.HTTPCheckInfo parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.HealthCheck.HTTPCheckInfo parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.HealthCheck.HTTPCheckInfo parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.HealthCheck.HTTPCheckInfo parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.HealthCheck.HTTPCheckInfo parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.apache.mesos.v1.Protos.HealthCheck.HTTPCheckInfo prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Describes an HTTP health check. Sends a GET request to
* scheme://<host>:port/path. Note that <host> is not configurable and is
* resolved automatically, in most cases to 127.0.0.1. Default executors
* treat return codes between 200 and 399 as success; custom executors
* may employ a different strategy, e.g. leveraging the `statuses` field.
*
*
* Protobuf type {@code mesos.v1.HealthCheck.HTTPCheckInfo}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:mesos.v1.HealthCheck.HTTPCheckInfo)
org.apache.mesos.v1.Protos.HealthCheck.HTTPCheckInfoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_HealthCheck_HTTPCheckInfo_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_HealthCheck_HTTPCheckInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.mesos.v1.Protos.HealthCheck.HTTPCheckInfo.class, org.apache.mesos.v1.Protos.HealthCheck.HTTPCheckInfo.Builder.class);
}
// Construct using org.apache.mesos.v1.Protos.HealthCheck.HTTPCheckInfo.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
protocol_ = 1;
bitField0_ = (bitField0_ & ~0x00000001);
scheme_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
port_ = 0;
bitField0_ = (bitField0_ & ~0x00000004);
path_ = "";
bitField0_ = (bitField0_ & ~0x00000008);
statuses_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000010);
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_HealthCheck_HTTPCheckInfo_descriptor;
}
public org.apache.mesos.v1.Protos.HealthCheck.HTTPCheckInfo getDefaultInstanceForType() {
return org.apache.mesos.v1.Protos.HealthCheck.HTTPCheckInfo.getDefaultInstance();
}
public org.apache.mesos.v1.Protos.HealthCheck.HTTPCheckInfo build() {
org.apache.mesos.v1.Protos.HealthCheck.HTTPCheckInfo result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.apache.mesos.v1.Protos.HealthCheck.HTTPCheckInfo buildPartial() {
org.apache.mesos.v1.Protos.HealthCheck.HTTPCheckInfo result = new org.apache.mesos.v1.Protos.HealthCheck.HTTPCheckInfo(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.protocol_ = protocol_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.scheme_ = scheme_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.port_ = port_;
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000008;
}
result.path_ = path_;
if (((bitField0_ & 0x00000010) == 0x00000010)) {
statuses_ = java.util.Collections.unmodifiableList(statuses_);
bitField0_ = (bitField0_ & ~0x00000010);
}
result.statuses_ = statuses_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.apache.mesos.v1.Protos.HealthCheck.HTTPCheckInfo) {
return mergeFrom((org.apache.mesos.v1.Protos.HealthCheck.HTTPCheckInfo)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.mesos.v1.Protos.HealthCheck.HTTPCheckInfo other) {
if (other == org.apache.mesos.v1.Protos.HealthCheck.HTTPCheckInfo.getDefaultInstance()) return this;
if (other.hasProtocol()) {
setProtocol(other.getProtocol());
}
if (other.hasScheme()) {
bitField0_ |= 0x00000002;
scheme_ = other.scheme_;
onChanged();
}
if (other.hasPort()) {
setPort(other.getPort());
}
if (other.hasPath()) {
bitField0_ |= 0x00000008;
path_ = other.path_;
onChanged();
}
if (!other.statuses_.isEmpty()) {
if (statuses_.isEmpty()) {
statuses_ = other.statuses_;
bitField0_ = (bitField0_ & ~0x00000010);
} else {
ensureStatusesIsMutable();
statuses_.addAll(other.statuses_);
}
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
if (!hasPort()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.mesos.v1.Protos.HealthCheck.HTTPCheckInfo parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.mesos.v1.Protos.HealthCheck.HTTPCheckInfo) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private int protocol_ = 1;
/**
* optional .mesos.v1.NetworkInfo.Protocol protocol = 5 [default = IPv4];
*/
public boolean hasProtocol() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional .mesos.v1.NetworkInfo.Protocol protocol = 5 [default = IPv4];
*/
public org.apache.mesos.v1.Protos.NetworkInfo.Protocol getProtocol() {
org.apache.mesos.v1.Protos.NetworkInfo.Protocol result = org.apache.mesos.v1.Protos.NetworkInfo.Protocol.valueOf(protocol_);
return result == null ? org.apache.mesos.v1.Protos.NetworkInfo.Protocol.IPv4 : result;
}
/**
* optional .mesos.v1.NetworkInfo.Protocol protocol = 5 [default = IPv4];
*/
public Builder setProtocol(org.apache.mesos.v1.Protos.NetworkInfo.Protocol value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
protocol_ = value.getNumber();
onChanged();
return this;
}
/**
* optional .mesos.v1.NetworkInfo.Protocol protocol = 5 [default = IPv4];
*/
public Builder clearProtocol() {
bitField0_ = (bitField0_ & ~0x00000001);
protocol_ = 1;
onChanged();
return this;
}
private java.lang.Object scheme_ = "";
/**
*
* Currently "http" and "https" are supported.
*
*
* optional string scheme = 3;
*/
public boolean hasScheme() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
*
* Currently "http" and "https" are supported.
*
*
* optional string scheme = 3;
*/
public java.lang.String getScheme() {
java.lang.Object ref = scheme_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
scheme_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Currently "http" and "https" are supported.
*
*
* optional string scheme = 3;
*/
public com.google.protobuf.ByteString
getSchemeBytes() {
java.lang.Object ref = scheme_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
scheme_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Currently "http" and "https" are supported.
*
*
* optional string scheme = 3;
*/
public Builder setScheme(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
scheme_ = value;
onChanged();
return this;
}
/**
*
* Currently "http" and "https" are supported.
*
*
* optional string scheme = 3;
*/
public Builder clearScheme() {
bitField0_ = (bitField0_ & ~0x00000002);
scheme_ = getDefaultInstance().getScheme();
onChanged();
return this;
}
/**
*
* Currently "http" and "https" are supported.
*
*
* optional string scheme = 3;
*/
public Builder setSchemeBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
scheme_ = value;
onChanged();
return this;
}
private int port_ ;
/**
*
* Port to send the HTTP request.
*
*
* required uint32 port = 1;
*/
public boolean hasPort() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
*
* Port to send the HTTP request.
*
*
* required uint32 port = 1;
*/
public int getPort() {
return port_;
}
/**
*
* Port to send the HTTP request.
*
*
* required uint32 port = 1;
*/
public Builder setPort(int value) {
bitField0_ |= 0x00000004;
port_ = value;
onChanged();
return this;
}
/**
*
* Port to send the HTTP request.
*
*
* required uint32 port = 1;
*/
public Builder clearPort() {
bitField0_ = (bitField0_ & ~0x00000004);
port_ = 0;
onChanged();
return this;
}
private java.lang.Object path_ = "";
/**
*
* HTTP request path.
*
*
* optional string path = 2;
*/
public boolean hasPath() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
*
* HTTP request path.
*
*
* optional string path = 2;
*/
public java.lang.String getPath() {
java.lang.Object ref = path_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
path_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* HTTP request path.
*
*
* optional string path = 2;
*/
public com.google.protobuf.ByteString
getPathBytes() {
java.lang.Object ref = path_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
path_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* HTTP request path.
*
*
* optional string path = 2;
*/
public Builder setPath(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
path_ = value;
onChanged();
return this;
}
/**
*
* HTTP request path.
*
*
* optional string path = 2;
*/
public Builder clearPath() {
bitField0_ = (bitField0_ & ~0x00000008);
path_ = getDefaultInstance().getPath();
onChanged();
return this;
}
/**
*
* HTTP request path.
*
*
* optional string path = 2;
*/
public Builder setPathBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
path_ = value;
onChanged();
return this;
}
private java.util.List statuses_ = java.util.Collections.emptyList();
private void ensureStatusesIsMutable() {
if (!((bitField0_ & 0x00000010) == 0x00000010)) {
statuses_ = new java.util.ArrayList(statuses_);
bitField0_ |= 0x00000010;
}
}
/**
*
* NOTE: It is up to the custom executor to interpret and act on this
* field. Setting this field has no effect on the default executors.
* TODO(haosdent): Deprecate this field when we add better support for
* success and possibly failure statuses, e.g. ranges of success and
* failure statuses.
*
*
* repeated uint32 statuses = 4;
*/
public java.util.List
getStatusesList() {
return java.util.Collections.unmodifiableList(statuses_);
}
/**
*
* NOTE: It is up to the custom executor to interpret and act on this
* field. Setting this field has no effect on the default executors.
* TODO(haosdent): Deprecate this field when we add better support for
* success and possibly failure statuses, e.g. ranges of success and
* failure statuses.
*
*
* repeated uint32 statuses = 4;
*/
public int getStatusesCount() {
return statuses_.size();
}
/**
*
* NOTE: It is up to the custom executor to interpret and act on this
* field. Setting this field has no effect on the default executors.
* TODO(haosdent): Deprecate this field when we add better support for
* success and possibly failure statuses, e.g. ranges of success and
* failure statuses.
*
*
* repeated uint32 statuses = 4;
*/
public int getStatuses(int index) {
return statuses_.get(index);
}
/**
*
* NOTE: It is up to the custom executor to interpret and act on this
* field. Setting this field has no effect on the default executors.
* TODO(haosdent): Deprecate this field when we add better support for
* success and possibly failure statuses, e.g. ranges of success and
* failure statuses.
*
*
* repeated uint32 statuses = 4;
*/
public Builder setStatuses(
int index, int value) {
ensureStatusesIsMutable();
statuses_.set(index, value);
onChanged();
return this;
}
/**
*
* NOTE: It is up to the custom executor to interpret and act on this
* field. Setting this field has no effect on the default executors.
* TODO(haosdent): Deprecate this field when we add better support for
* success and possibly failure statuses, e.g. ranges of success and
* failure statuses.
*
*
* repeated uint32 statuses = 4;
*/
public Builder addStatuses(int value) {
ensureStatusesIsMutable();
statuses_.add(value);
onChanged();
return this;
}
/**
*
* NOTE: It is up to the custom executor to interpret and act on this
* field. Setting this field has no effect on the default executors.
* TODO(haosdent): Deprecate this field when we add better support for
* success and possibly failure statuses, e.g. ranges of success and
* failure statuses.
*
*
* repeated uint32 statuses = 4;
*/
public Builder addAllStatuses(
java.lang.Iterable extends java.lang.Integer> values) {
ensureStatusesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, statuses_);
onChanged();
return this;
}
/**
*
* NOTE: It is up to the custom executor to interpret and act on this
* field. Setting this field has no effect on the default executors.
* TODO(haosdent): Deprecate this field when we add better support for
* success and possibly failure statuses, e.g. ranges of success and
* failure statuses.
*
*
* repeated uint32 statuses = 4;
*/
public Builder clearStatuses() {
statuses_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000010);
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:mesos.v1.HealthCheck.HTTPCheckInfo)
}
// @@protoc_insertion_point(class_scope:mesos.v1.HealthCheck.HTTPCheckInfo)
private static final org.apache.mesos.v1.Protos.HealthCheck.HTTPCheckInfo DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.apache.mesos.v1.Protos.HealthCheck.HTTPCheckInfo();
}
public static org.apache.mesos.v1.Protos.HealthCheck.HTTPCheckInfo getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public HTTPCheckInfo parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new HTTPCheckInfo(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.apache.mesos.v1.Protos.HealthCheck.HTTPCheckInfo getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface TCPCheckInfoOrBuilder extends
// @@protoc_insertion_point(interface_extends:mesos.v1.HealthCheck.TCPCheckInfo)
com.google.protobuf.MessageOrBuilder {
/**
* optional .mesos.v1.NetworkInfo.Protocol protocol = 2 [default = IPv4];
*/
boolean hasProtocol();
/**
* optional .mesos.v1.NetworkInfo.Protocol protocol = 2 [default = IPv4];
*/
org.apache.mesos.v1.Protos.NetworkInfo.Protocol getProtocol();
/**
*
* Port expected to be open.
*
*
* required uint32 port = 1;
*/
boolean hasPort();
/**
*
* Port expected to be open.
*
*
* required uint32 port = 1;
*/
int getPort();
}
/**
*
* Describes a TCP health check, i.e. based on establishing
* a TCP connection to the specified port.
*
*
* Protobuf type {@code mesos.v1.HealthCheck.TCPCheckInfo}
*/
public static final class TCPCheckInfo extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:mesos.v1.HealthCheck.TCPCheckInfo)
TCPCheckInfoOrBuilder {
private static final long serialVersionUID = 0L;
// Use TCPCheckInfo.newBuilder() to construct.
private TCPCheckInfo(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private TCPCheckInfo() {
protocol_ = 1;
port_ = 0;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private TCPCheckInfo(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
bitField0_ |= 0x00000002;
port_ = input.readUInt32();
break;
}
case 16: {
int rawValue = input.readEnum();
org.apache.mesos.v1.Protos.NetworkInfo.Protocol value = org.apache.mesos.v1.Protos.NetworkInfo.Protocol.valueOf(rawValue);
if (value == null) {
unknownFields.mergeVarintField(2, rawValue);
} else {
bitField0_ |= 0x00000001;
protocol_ = rawValue;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_HealthCheck_TCPCheckInfo_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_HealthCheck_TCPCheckInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.mesos.v1.Protos.HealthCheck.TCPCheckInfo.class, org.apache.mesos.v1.Protos.HealthCheck.TCPCheckInfo.Builder.class);
}
private int bitField0_;
public static final int PROTOCOL_FIELD_NUMBER = 2;
private int protocol_;
/**
* optional .mesos.v1.NetworkInfo.Protocol protocol = 2 [default = IPv4];
*/
public boolean hasProtocol() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional .mesos.v1.NetworkInfo.Protocol protocol = 2 [default = IPv4];
*/
public org.apache.mesos.v1.Protos.NetworkInfo.Protocol getProtocol() {
org.apache.mesos.v1.Protos.NetworkInfo.Protocol result = org.apache.mesos.v1.Protos.NetworkInfo.Protocol.valueOf(protocol_);
return result == null ? org.apache.mesos.v1.Protos.NetworkInfo.Protocol.IPv4 : result;
}
public static final int PORT_FIELD_NUMBER = 1;
private int port_;
/**
*
* Port expected to be open.
*
*
* required uint32 port = 1;
*/
public boolean hasPort() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
*
* Port expected to be open.
*
*
* required uint32 port = 1;
*/
public int getPort() {
return port_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasPort()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeUInt32(1, port_);
}
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeEnum(2, protocol_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(1, port_);
}
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(2, protocol_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.mesos.v1.Protos.HealthCheck.TCPCheckInfo)) {
return super.equals(obj);
}
org.apache.mesos.v1.Protos.HealthCheck.TCPCheckInfo other = (org.apache.mesos.v1.Protos.HealthCheck.TCPCheckInfo) obj;
boolean result = true;
result = result && (hasProtocol() == other.hasProtocol());
if (hasProtocol()) {
result = result && protocol_ == other.protocol_;
}
result = result && (hasPort() == other.hasPort());
if (hasPort()) {
result = result && (getPort()
== other.getPort());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasProtocol()) {
hash = (37 * hash) + PROTOCOL_FIELD_NUMBER;
hash = (53 * hash) + protocol_;
}
if (hasPort()) {
hash = (37 * hash) + PORT_FIELD_NUMBER;
hash = (53 * hash) + getPort();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.mesos.v1.Protos.HealthCheck.TCPCheckInfo parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.HealthCheck.TCPCheckInfo parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.HealthCheck.TCPCheckInfo parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.HealthCheck.TCPCheckInfo parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.HealthCheck.TCPCheckInfo parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.HealthCheck.TCPCheckInfo parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.HealthCheck.TCPCheckInfo parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.HealthCheck.TCPCheckInfo parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.HealthCheck.TCPCheckInfo parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.HealthCheck.TCPCheckInfo parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.HealthCheck.TCPCheckInfo parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.HealthCheck.TCPCheckInfo parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.apache.mesos.v1.Protos.HealthCheck.TCPCheckInfo prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Describes a TCP health check, i.e. based on establishing
* a TCP connection to the specified port.
*
*
* Protobuf type {@code mesos.v1.HealthCheck.TCPCheckInfo}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:mesos.v1.HealthCheck.TCPCheckInfo)
org.apache.mesos.v1.Protos.HealthCheck.TCPCheckInfoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_HealthCheck_TCPCheckInfo_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_HealthCheck_TCPCheckInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.mesos.v1.Protos.HealthCheck.TCPCheckInfo.class, org.apache.mesos.v1.Protos.HealthCheck.TCPCheckInfo.Builder.class);
}
// Construct using org.apache.mesos.v1.Protos.HealthCheck.TCPCheckInfo.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
protocol_ = 1;
bitField0_ = (bitField0_ & ~0x00000001);
port_ = 0;
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_HealthCheck_TCPCheckInfo_descriptor;
}
public org.apache.mesos.v1.Protos.HealthCheck.TCPCheckInfo getDefaultInstanceForType() {
return org.apache.mesos.v1.Protos.HealthCheck.TCPCheckInfo.getDefaultInstance();
}
public org.apache.mesos.v1.Protos.HealthCheck.TCPCheckInfo build() {
org.apache.mesos.v1.Protos.HealthCheck.TCPCheckInfo result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.apache.mesos.v1.Protos.HealthCheck.TCPCheckInfo buildPartial() {
org.apache.mesos.v1.Protos.HealthCheck.TCPCheckInfo result = new org.apache.mesos.v1.Protos.HealthCheck.TCPCheckInfo(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.protocol_ = protocol_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.port_ = port_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.apache.mesos.v1.Protos.HealthCheck.TCPCheckInfo) {
return mergeFrom((org.apache.mesos.v1.Protos.HealthCheck.TCPCheckInfo)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.mesos.v1.Protos.HealthCheck.TCPCheckInfo other) {
if (other == org.apache.mesos.v1.Protos.HealthCheck.TCPCheckInfo.getDefaultInstance()) return this;
if (other.hasProtocol()) {
setProtocol(other.getProtocol());
}
if (other.hasPort()) {
setPort(other.getPort());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
if (!hasPort()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.mesos.v1.Protos.HealthCheck.TCPCheckInfo parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.mesos.v1.Protos.HealthCheck.TCPCheckInfo) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private int protocol_ = 1;
/**
* optional .mesos.v1.NetworkInfo.Protocol protocol = 2 [default = IPv4];
*/
public boolean hasProtocol() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional .mesos.v1.NetworkInfo.Protocol protocol = 2 [default = IPv4];
*/
public org.apache.mesos.v1.Protos.NetworkInfo.Protocol getProtocol() {
org.apache.mesos.v1.Protos.NetworkInfo.Protocol result = org.apache.mesos.v1.Protos.NetworkInfo.Protocol.valueOf(protocol_);
return result == null ? org.apache.mesos.v1.Protos.NetworkInfo.Protocol.IPv4 : result;
}
/**
* optional .mesos.v1.NetworkInfo.Protocol protocol = 2 [default = IPv4];
*/
public Builder setProtocol(org.apache.mesos.v1.Protos.NetworkInfo.Protocol value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
protocol_ = value.getNumber();
onChanged();
return this;
}
/**
* optional .mesos.v1.NetworkInfo.Protocol protocol = 2 [default = IPv4];
*/
public Builder clearProtocol() {
bitField0_ = (bitField0_ & ~0x00000001);
protocol_ = 1;
onChanged();
return this;
}
private int port_ ;
/**
*
* Port expected to be open.
*
*
* required uint32 port = 1;
*/
public boolean hasPort() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
*
* Port expected to be open.
*
*
* required uint32 port = 1;
*/
public int getPort() {
return port_;
}
/**
*
* Port expected to be open.
*
*
* required uint32 port = 1;
*/
public Builder setPort(int value) {
bitField0_ |= 0x00000002;
port_ = value;
onChanged();
return this;
}
/**
*
* Port expected to be open.
*
*
* required uint32 port = 1;
*/
public Builder clearPort() {
bitField0_ = (bitField0_ & ~0x00000002);
port_ = 0;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:mesos.v1.HealthCheck.TCPCheckInfo)
}
// @@protoc_insertion_point(class_scope:mesos.v1.HealthCheck.TCPCheckInfo)
private static final org.apache.mesos.v1.Protos.HealthCheck.TCPCheckInfo DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.apache.mesos.v1.Protos.HealthCheck.TCPCheckInfo();
}
public static org.apache.mesos.v1.Protos.HealthCheck.TCPCheckInfo getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public TCPCheckInfo parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new TCPCheckInfo(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.apache.mesos.v1.Protos.HealthCheck.TCPCheckInfo getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private int bitField0_;
public static final int DELAY_SECONDS_FIELD_NUMBER = 2;
private double delaySeconds_;
/**
*
* Amount of time to wait to start health checking the task after it
* transitions to `TASK_RUNNING` or `TASK_STATING` if the latter is
* used by the executor.
*
*
* optional double delay_seconds = 2 [default = 15];
*/
public boolean hasDelaySeconds() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
*
* Amount of time to wait to start health checking the task after it
* transitions to `TASK_RUNNING` or `TASK_STATING` if the latter is
* used by the executor.
*
*
* optional double delay_seconds = 2 [default = 15];
*/
public double getDelaySeconds() {
return delaySeconds_;
}
public static final int INTERVAL_SECONDS_FIELD_NUMBER = 3;
private double intervalSeconds_;
/**
*
* Interval between health checks, i.e., amount of time to wait after
* the previous health check finished or timed out to start the next
* health check.
*
*
* optional double interval_seconds = 3 [default = 10];
*/
public boolean hasIntervalSeconds() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
*
* Interval between health checks, i.e., amount of time to wait after
* the previous health check finished or timed out to start the next
* health check.
*
*
* optional double interval_seconds = 3 [default = 10];
*/
public double getIntervalSeconds() {
return intervalSeconds_;
}
public static final int TIMEOUT_SECONDS_FIELD_NUMBER = 4;
private double timeoutSeconds_;
/**
*
* Amount of time to wait for the health check to complete. After this
* timeout, the health check is aborted and treated as a failure. Zero
* means infinite timeout.
*
*
* optional double timeout_seconds = 4 [default = 20];
*/
public boolean hasTimeoutSeconds() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
*
* Amount of time to wait for the health check to complete. After this
* timeout, the health check is aborted and treated as a failure. Zero
* means infinite timeout.
*
*
* optional double timeout_seconds = 4 [default = 20];
*/
public double getTimeoutSeconds() {
return timeoutSeconds_;
}
public static final int CONSECUTIVE_FAILURES_FIELD_NUMBER = 5;
private int consecutiveFailures_;
/**
*
* Number of consecutive failures until the task is killed by the executor.
*
*
* optional uint32 consecutive_failures = 5 [default = 3];
*/
public boolean hasConsecutiveFailures() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
*
* Number of consecutive failures until the task is killed by the executor.
*
*
* optional uint32 consecutive_failures = 5 [default = 3];
*/
public int getConsecutiveFailures() {
return consecutiveFailures_;
}
public static final int GRACE_PERIOD_SECONDS_FIELD_NUMBER = 6;
private double gracePeriodSeconds_;
/**
*
* Amount of time after the task is launched during which health check
* failures are ignored. Once a check succeeds for the first time,
* the grace period does not apply anymore. Note that it includes
* `delay_seconds`, i.e., setting `grace_period_seconds` < `delay_seconds`
* has no effect.
*
*
* optional double grace_period_seconds = 6 [default = 10];
*/
public boolean hasGracePeriodSeconds() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
*
* Amount of time after the task is launched during which health check
* failures are ignored. Once a check succeeds for the first time,
* the grace period does not apply anymore. Note that it includes
* `delay_seconds`, i.e., setting `grace_period_seconds` < `delay_seconds`
* has no effect.
*
*
* optional double grace_period_seconds = 6 [default = 10];
*/
public double getGracePeriodSeconds() {
return gracePeriodSeconds_;
}
public static final int TYPE_FIELD_NUMBER = 8;
private int type_;
/**
*
* The type of health check.
*
*
* optional .mesos.v1.HealthCheck.Type type = 8;
*/
public boolean hasType() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
*
* The type of health check.
*
*
* optional .mesos.v1.HealthCheck.Type type = 8;
*/
public org.apache.mesos.v1.Protos.HealthCheck.Type getType() {
org.apache.mesos.v1.Protos.HealthCheck.Type result = org.apache.mesos.v1.Protos.HealthCheck.Type.valueOf(type_);
return result == null ? org.apache.mesos.v1.Protos.HealthCheck.Type.UNKNOWN : result;
}
public static final int COMMAND_FIELD_NUMBER = 7;
private org.apache.mesos.v1.Protos.CommandInfo command_;
/**
*
* Command health check.
*
*
* optional .mesos.v1.CommandInfo command = 7;
*/
public boolean hasCommand() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
*
* Command health check.
*
*
* optional .mesos.v1.CommandInfo command = 7;
*/
public org.apache.mesos.v1.Protos.CommandInfo getCommand() {
return command_ == null ? org.apache.mesos.v1.Protos.CommandInfo.getDefaultInstance() : command_;
}
/**
*
* Command health check.
*
*
* optional .mesos.v1.CommandInfo command = 7;
*/
public org.apache.mesos.v1.Protos.CommandInfoOrBuilder getCommandOrBuilder() {
return command_ == null ? org.apache.mesos.v1.Protos.CommandInfo.getDefaultInstance() : command_;
}
public static final int HTTP_FIELD_NUMBER = 1;
private org.apache.mesos.v1.Protos.HealthCheck.HTTPCheckInfo http_;
/**
*
* HTTP health check.
*
*
* optional .mesos.v1.HealthCheck.HTTPCheckInfo http = 1;
*/
public boolean hasHttp() {
return ((bitField0_ & 0x00000080) == 0x00000080);
}
/**
*
* HTTP health check.
*
*
* optional .mesos.v1.HealthCheck.HTTPCheckInfo http = 1;
*/
public org.apache.mesos.v1.Protos.HealthCheck.HTTPCheckInfo getHttp() {
return http_ == null ? org.apache.mesos.v1.Protos.HealthCheck.HTTPCheckInfo.getDefaultInstance() : http_;
}
/**
*
* HTTP health check.
*
*
* optional .mesos.v1.HealthCheck.HTTPCheckInfo http = 1;
*/
public org.apache.mesos.v1.Protos.HealthCheck.HTTPCheckInfoOrBuilder getHttpOrBuilder() {
return http_ == null ? org.apache.mesos.v1.Protos.HealthCheck.HTTPCheckInfo.getDefaultInstance() : http_;
}
public static final int TCP_FIELD_NUMBER = 9;
private org.apache.mesos.v1.Protos.HealthCheck.TCPCheckInfo tcp_;
/**
*
* TCP health check.
*
*
* optional .mesos.v1.HealthCheck.TCPCheckInfo tcp = 9;
*/
public boolean hasTcp() {
return ((bitField0_ & 0x00000100) == 0x00000100);
}
/**
*
* TCP health check.
*
*
* optional .mesos.v1.HealthCheck.TCPCheckInfo tcp = 9;
*/
public org.apache.mesos.v1.Protos.HealthCheck.TCPCheckInfo getTcp() {
return tcp_ == null ? org.apache.mesos.v1.Protos.HealthCheck.TCPCheckInfo.getDefaultInstance() : tcp_;
}
/**
*
* TCP health check.
*
*
* optional .mesos.v1.HealthCheck.TCPCheckInfo tcp = 9;
*/
public org.apache.mesos.v1.Protos.HealthCheck.TCPCheckInfoOrBuilder getTcpOrBuilder() {
return tcp_ == null ? org.apache.mesos.v1.Protos.HealthCheck.TCPCheckInfo.getDefaultInstance() : tcp_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (hasCommand()) {
if (!getCommand().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
if (hasHttp()) {
if (!getHttp().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
if (hasTcp()) {
if (!getTcp().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000080) == 0x00000080)) {
output.writeMessage(1, getHttp());
}
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeDouble(2, delaySeconds_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeDouble(3, intervalSeconds_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeDouble(4, timeoutSeconds_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeUInt32(5, consecutiveFailures_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
output.writeDouble(6, gracePeriodSeconds_);
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
output.writeMessage(7, getCommand());
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
output.writeEnum(8, type_);
}
if (((bitField0_ & 0x00000100) == 0x00000100)) {
output.writeMessage(9, getTcp());
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000080) == 0x00000080)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getHttp());
}
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeDoubleSize(2, delaySeconds_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeDoubleSize(3, intervalSeconds_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeDoubleSize(4, timeoutSeconds_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(5, consecutiveFailures_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
size += com.google.protobuf.CodedOutputStream
.computeDoubleSize(6, gracePeriodSeconds_);
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(7, getCommand());
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(8, type_);
}
if (((bitField0_ & 0x00000100) == 0x00000100)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(9, getTcp());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.mesos.v1.Protos.HealthCheck)) {
return super.equals(obj);
}
org.apache.mesos.v1.Protos.HealthCheck other = (org.apache.mesos.v1.Protos.HealthCheck) obj;
boolean result = true;
result = result && (hasDelaySeconds() == other.hasDelaySeconds());
if (hasDelaySeconds()) {
result = result && (
java.lang.Double.doubleToLongBits(getDelaySeconds())
== java.lang.Double.doubleToLongBits(
other.getDelaySeconds()));
}
result = result && (hasIntervalSeconds() == other.hasIntervalSeconds());
if (hasIntervalSeconds()) {
result = result && (
java.lang.Double.doubleToLongBits(getIntervalSeconds())
== java.lang.Double.doubleToLongBits(
other.getIntervalSeconds()));
}
result = result && (hasTimeoutSeconds() == other.hasTimeoutSeconds());
if (hasTimeoutSeconds()) {
result = result && (
java.lang.Double.doubleToLongBits(getTimeoutSeconds())
== java.lang.Double.doubleToLongBits(
other.getTimeoutSeconds()));
}
result = result && (hasConsecutiveFailures() == other.hasConsecutiveFailures());
if (hasConsecutiveFailures()) {
result = result && (getConsecutiveFailures()
== other.getConsecutiveFailures());
}
result = result && (hasGracePeriodSeconds() == other.hasGracePeriodSeconds());
if (hasGracePeriodSeconds()) {
result = result && (
java.lang.Double.doubleToLongBits(getGracePeriodSeconds())
== java.lang.Double.doubleToLongBits(
other.getGracePeriodSeconds()));
}
result = result && (hasType() == other.hasType());
if (hasType()) {
result = result && type_ == other.type_;
}
result = result && (hasCommand() == other.hasCommand());
if (hasCommand()) {
result = result && getCommand()
.equals(other.getCommand());
}
result = result && (hasHttp() == other.hasHttp());
if (hasHttp()) {
result = result && getHttp()
.equals(other.getHttp());
}
result = result && (hasTcp() == other.hasTcp());
if (hasTcp()) {
result = result && getTcp()
.equals(other.getTcp());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasDelaySeconds()) {
hash = (37 * hash) + DELAY_SECONDS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
java.lang.Double.doubleToLongBits(getDelaySeconds()));
}
if (hasIntervalSeconds()) {
hash = (37 * hash) + INTERVAL_SECONDS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
java.lang.Double.doubleToLongBits(getIntervalSeconds()));
}
if (hasTimeoutSeconds()) {
hash = (37 * hash) + TIMEOUT_SECONDS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
java.lang.Double.doubleToLongBits(getTimeoutSeconds()));
}
if (hasConsecutiveFailures()) {
hash = (37 * hash) + CONSECUTIVE_FAILURES_FIELD_NUMBER;
hash = (53 * hash) + getConsecutiveFailures();
}
if (hasGracePeriodSeconds()) {
hash = (37 * hash) + GRACE_PERIOD_SECONDS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
java.lang.Double.doubleToLongBits(getGracePeriodSeconds()));
}
if (hasType()) {
hash = (37 * hash) + TYPE_FIELD_NUMBER;
hash = (53 * hash) + type_;
}
if (hasCommand()) {
hash = (37 * hash) + COMMAND_FIELD_NUMBER;
hash = (53 * hash) + getCommand().hashCode();
}
if (hasHttp()) {
hash = (37 * hash) + HTTP_FIELD_NUMBER;
hash = (53 * hash) + getHttp().hashCode();
}
if (hasTcp()) {
hash = (37 * hash) + TCP_FIELD_NUMBER;
hash = (53 * hash) + getTcp().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.mesos.v1.Protos.HealthCheck parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.HealthCheck parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.HealthCheck parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.HealthCheck parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.HealthCheck parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.HealthCheck parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.HealthCheck parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.HealthCheck parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.HealthCheck parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.HealthCheck parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.HealthCheck parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.HealthCheck parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.apache.mesos.v1.Protos.HealthCheck prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
**
* Describes a health check for a task or executor (or any arbitrary
* process/command). A type is picked by specifying one of the
* optional fields. Specifying more than one type is an error.
*
*
* Protobuf type {@code mesos.v1.HealthCheck}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:mesos.v1.HealthCheck)
org.apache.mesos.v1.Protos.HealthCheckOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_HealthCheck_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_HealthCheck_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.mesos.v1.Protos.HealthCheck.class, org.apache.mesos.v1.Protos.HealthCheck.Builder.class);
}
// Construct using org.apache.mesos.v1.Protos.HealthCheck.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getCommandFieldBuilder();
getHttpFieldBuilder();
getTcpFieldBuilder();
}
}
public Builder clear() {
super.clear();
delaySeconds_ = 15D;
bitField0_ = (bitField0_ & ~0x00000001);
intervalSeconds_ = 10D;
bitField0_ = (bitField0_ & ~0x00000002);
timeoutSeconds_ = 20D;
bitField0_ = (bitField0_ & ~0x00000004);
consecutiveFailures_ = 3;
bitField0_ = (bitField0_ & ~0x00000008);
gracePeriodSeconds_ = 10D;
bitField0_ = (bitField0_ & ~0x00000010);
type_ = 0;
bitField0_ = (bitField0_ & ~0x00000020);
if (commandBuilder_ == null) {
command_ = null;
} else {
commandBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000040);
if (httpBuilder_ == null) {
http_ = null;
} else {
httpBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000080);
if (tcpBuilder_ == null) {
tcp_ = null;
} else {
tcpBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000100);
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_HealthCheck_descriptor;
}
public org.apache.mesos.v1.Protos.HealthCheck getDefaultInstanceForType() {
return org.apache.mesos.v1.Protos.HealthCheck.getDefaultInstance();
}
public org.apache.mesos.v1.Protos.HealthCheck build() {
org.apache.mesos.v1.Protos.HealthCheck result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.apache.mesos.v1.Protos.HealthCheck buildPartial() {
org.apache.mesos.v1.Protos.HealthCheck result = new org.apache.mesos.v1.Protos.HealthCheck(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.delaySeconds_ = delaySeconds_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.intervalSeconds_ = intervalSeconds_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.timeoutSeconds_ = timeoutSeconds_;
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000008;
}
result.consecutiveFailures_ = consecutiveFailures_;
if (((from_bitField0_ & 0x00000010) == 0x00000010)) {
to_bitField0_ |= 0x00000010;
}
result.gracePeriodSeconds_ = gracePeriodSeconds_;
if (((from_bitField0_ & 0x00000020) == 0x00000020)) {
to_bitField0_ |= 0x00000020;
}
result.type_ = type_;
if (((from_bitField0_ & 0x00000040) == 0x00000040)) {
to_bitField0_ |= 0x00000040;
}
if (commandBuilder_ == null) {
result.command_ = command_;
} else {
result.command_ = commandBuilder_.build();
}
if (((from_bitField0_ & 0x00000080) == 0x00000080)) {
to_bitField0_ |= 0x00000080;
}
if (httpBuilder_ == null) {
result.http_ = http_;
} else {
result.http_ = httpBuilder_.build();
}
if (((from_bitField0_ & 0x00000100) == 0x00000100)) {
to_bitField0_ |= 0x00000100;
}
if (tcpBuilder_ == null) {
result.tcp_ = tcp_;
} else {
result.tcp_ = tcpBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.apache.mesos.v1.Protos.HealthCheck) {
return mergeFrom((org.apache.mesos.v1.Protos.HealthCheck)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.mesos.v1.Protos.HealthCheck other) {
if (other == org.apache.mesos.v1.Protos.HealthCheck.getDefaultInstance()) return this;
if (other.hasDelaySeconds()) {
setDelaySeconds(other.getDelaySeconds());
}
if (other.hasIntervalSeconds()) {
setIntervalSeconds(other.getIntervalSeconds());
}
if (other.hasTimeoutSeconds()) {
setTimeoutSeconds(other.getTimeoutSeconds());
}
if (other.hasConsecutiveFailures()) {
setConsecutiveFailures(other.getConsecutiveFailures());
}
if (other.hasGracePeriodSeconds()) {
setGracePeriodSeconds(other.getGracePeriodSeconds());
}
if (other.hasType()) {
setType(other.getType());
}
if (other.hasCommand()) {
mergeCommand(other.getCommand());
}
if (other.hasHttp()) {
mergeHttp(other.getHttp());
}
if (other.hasTcp()) {
mergeTcp(other.getTcp());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
if (hasCommand()) {
if (!getCommand().isInitialized()) {
return false;
}
}
if (hasHttp()) {
if (!getHttp().isInitialized()) {
return false;
}
}
if (hasTcp()) {
if (!getTcp().isInitialized()) {
return false;
}
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.mesos.v1.Protos.HealthCheck parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.mesos.v1.Protos.HealthCheck) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private double delaySeconds_ = 15D;
/**
*
* Amount of time to wait to start health checking the task after it
* transitions to `TASK_RUNNING` or `TASK_STATING` if the latter is
* used by the executor.
*
*
* optional double delay_seconds = 2 [default = 15];
*/
public boolean hasDelaySeconds() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
*
* Amount of time to wait to start health checking the task after it
* transitions to `TASK_RUNNING` or `TASK_STATING` if the latter is
* used by the executor.
*
*
* optional double delay_seconds = 2 [default = 15];
*/
public double getDelaySeconds() {
return delaySeconds_;
}
/**
*
* Amount of time to wait to start health checking the task after it
* transitions to `TASK_RUNNING` or `TASK_STATING` if the latter is
* used by the executor.
*
*
* optional double delay_seconds = 2 [default = 15];
*/
public Builder setDelaySeconds(double value) {
bitField0_ |= 0x00000001;
delaySeconds_ = value;
onChanged();
return this;
}
/**
*
* Amount of time to wait to start health checking the task after it
* transitions to `TASK_RUNNING` or `TASK_STATING` if the latter is
* used by the executor.
*
*
* optional double delay_seconds = 2 [default = 15];
*/
public Builder clearDelaySeconds() {
bitField0_ = (bitField0_ & ~0x00000001);
delaySeconds_ = 15D;
onChanged();
return this;
}
private double intervalSeconds_ = 10D;
/**
*
* Interval between health checks, i.e., amount of time to wait after
* the previous health check finished or timed out to start the next
* health check.
*
*
* optional double interval_seconds = 3 [default = 10];
*/
public boolean hasIntervalSeconds() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
*
* Interval between health checks, i.e., amount of time to wait after
* the previous health check finished or timed out to start the next
* health check.
*
*
* optional double interval_seconds = 3 [default = 10];
*/
public double getIntervalSeconds() {
return intervalSeconds_;
}
/**
*
* Interval between health checks, i.e., amount of time to wait after
* the previous health check finished or timed out to start the next
* health check.
*
*
* optional double interval_seconds = 3 [default = 10];
*/
public Builder setIntervalSeconds(double value) {
bitField0_ |= 0x00000002;
intervalSeconds_ = value;
onChanged();
return this;
}
/**
*
* Interval between health checks, i.e., amount of time to wait after
* the previous health check finished or timed out to start the next
* health check.
*
*
* optional double interval_seconds = 3 [default = 10];
*/
public Builder clearIntervalSeconds() {
bitField0_ = (bitField0_ & ~0x00000002);
intervalSeconds_ = 10D;
onChanged();
return this;
}
private double timeoutSeconds_ = 20D;
/**
*
* Amount of time to wait for the health check to complete. After this
* timeout, the health check is aborted and treated as a failure. Zero
* means infinite timeout.
*
*
* optional double timeout_seconds = 4 [default = 20];
*/
public boolean hasTimeoutSeconds() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
*
* Amount of time to wait for the health check to complete. After this
* timeout, the health check is aborted and treated as a failure. Zero
* means infinite timeout.
*
*
* optional double timeout_seconds = 4 [default = 20];
*/
public double getTimeoutSeconds() {
return timeoutSeconds_;
}
/**
*
* Amount of time to wait for the health check to complete. After this
* timeout, the health check is aborted and treated as a failure. Zero
* means infinite timeout.
*
*
* optional double timeout_seconds = 4 [default = 20];
*/
public Builder setTimeoutSeconds(double value) {
bitField0_ |= 0x00000004;
timeoutSeconds_ = value;
onChanged();
return this;
}
/**
*
* Amount of time to wait for the health check to complete. After this
* timeout, the health check is aborted and treated as a failure. Zero
* means infinite timeout.
*
*
* optional double timeout_seconds = 4 [default = 20];
*/
public Builder clearTimeoutSeconds() {
bitField0_ = (bitField0_ & ~0x00000004);
timeoutSeconds_ = 20D;
onChanged();
return this;
}
private int consecutiveFailures_ = 3;
/**
*
* Number of consecutive failures until the task is killed by the executor.
*
*
* optional uint32 consecutive_failures = 5 [default = 3];
*/
public boolean hasConsecutiveFailures() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
*
* Number of consecutive failures until the task is killed by the executor.
*
*
* optional uint32 consecutive_failures = 5 [default = 3];
*/
public int getConsecutiveFailures() {
return consecutiveFailures_;
}
/**
*
* Number of consecutive failures until the task is killed by the executor.
*
*
* optional uint32 consecutive_failures = 5 [default = 3];
*/
public Builder setConsecutiveFailures(int value) {
bitField0_ |= 0x00000008;
consecutiveFailures_ = value;
onChanged();
return this;
}
/**
*
* Number of consecutive failures until the task is killed by the executor.
*
*
* optional uint32 consecutive_failures = 5 [default = 3];
*/
public Builder clearConsecutiveFailures() {
bitField0_ = (bitField0_ & ~0x00000008);
consecutiveFailures_ = 3;
onChanged();
return this;
}
private double gracePeriodSeconds_ = 10D;
/**
*
* Amount of time after the task is launched during which health check
* failures are ignored. Once a check succeeds for the first time,
* the grace period does not apply anymore. Note that it includes
* `delay_seconds`, i.e., setting `grace_period_seconds` < `delay_seconds`
* has no effect.
*
*
* optional double grace_period_seconds = 6 [default = 10];
*/
public boolean hasGracePeriodSeconds() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
*
* Amount of time after the task is launched during which health check
* failures are ignored. Once a check succeeds for the first time,
* the grace period does not apply anymore. Note that it includes
* `delay_seconds`, i.e., setting `grace_period_seconds` < `delay_seconds`
* has no effect.
*
*
* optional double grace_period_seconds = 6 [default = 10];
*/
public double getGracePeriodSeconds() {
return gracePeriodSeconds_;
}
/**
*
* Amount of time after the task is launched during which health check
* failures are ignored. Once a check succeeds for the first time,
* the grace period does not apply anymore. Note that it includes
* `delay_seconds`, i.e., setting `grace_period_seconds` < `delay_seconds`
* has no effect.
*
*
* optional double grace_period_seconds = 6 [default = 10];
*/
public Builder setGracePeriodSeconds(double value) {
bitField0_ |= 0x00000010;
gracePeriodSeconds_ = value;
onChanged();
return this;
}
/**
*
* Amount of time after the task is launched during which health check
* failures are ignored. Once a check succeeds for the first time,
* the grace period does not apply anymore. Note that it includes
* `delay_seconds`, i.e., setting `grace_period_seconds` < `delay_seconds`
* has no effect.
*
*
* optional double grace_period_seconds = 6 [default = 10];
*/
public Builder clearGracePeriodSeconds() {
bitField0_ = (bitField0_ & ~0x00000010);
gracePeriodSeconds_ = 10D;
onChanged();
return this;
}
private int type_ = 0;
/**
*
* The type of health check.
*
*
* optional .mesos.v1.HealthCheck.Type type = 8;
*/
public boolean hasType() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
*
* The type of health check.
*
*
* optional .mesos.v1.HealthCheck.Type type = 8;
*/
public org.apache.mesos.v1.Protos.HealthCheck.Type getType() {
org.apache.mesos.v1.Protos.HealthCheck.Type result = org.apache.mesos.v1.Protos.HealthCheck.Type.valueOf(type_);
return result == null ? org.apache.mesos.v1.Protos.HealthCheck.Type.UNKNOWN : result;
}
/**
*
* The type of health check.
*
*
* optional .mesos.v1.HealthCheck.Type type = 8;
*/
public Builder setType(org.apache.mesos.v1.Protos.HealthCheck.Type value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000020;
type_ = value.getNumber();
onChanged();
return this;
}
/**
*
* The type of health check.
*
*
* optional .mesos.v1.HealthCheck.Type type = 8;
*/
public Builder clearType() {
bitField0_ = (bitField0_ & ~0x00000020);
type_ = 0;
onChanged();
return this;
}
private org.apache.mesos.v1.Protos.CommandInfo command_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
org.apache.mesos.v1.Protos.CommandInfo, org.apache.mesos.v1.Protos.CommandInfo.Builder, org.apache.mesos.v1.Protos.CommandInfoOrBuilder> commandBuilder_;
/**
*
* Command health check.
*
*
* optional .mesos.v1.CommandInfo command = 7;
*/
public boolean hasCommand() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
*
* Command health check.
*
*
* optional .mesos.v1.CommandInfo command = 7;
*/
public org.apache.mesos.v1.Protos.CommandInfo getCommand() {
if (commandBuilder_ == null) {
return command_ == null ? org.apache.mesos.v1.Protos.CommandInfo.getDefaultInstance() : command_;
} else {
return commandBuilder_.getMessage();
}
}
/**
*
* Command health check.
*
*
* optional .mesos.v1.CommandInfo command = 7;
*/
public Builder setCommand(org.apache.mesos.v1.Protos.CommandInfo value) {
if (commandBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
command_ = value;
onChanged();
} else {
commandBuilder_.setMessage(value);
}
bitField0_ |= 0x00000040;
return this;
}
/**
*
* Command health check.
*
*
* optional .mesos.v1.CommandInfo command = 7;
*/
public Builder setCommand(
org.apache.mesos.v1.Protos.CommandInfo.Builder builderForValue) {
if (commandBuilder_ == null) {
command_ = builderForValue.build();
onChanged();
} else {
commandBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000040;
return this;
}
/**
*
* Command health check.
*
*
* optional .mesos.v1.CommandInfo command = 7;
*/
public Builder mergeCommand(org.apache.mesos.v1.Protos.CommandInfo value) {
if (commandBuilder_ == null) {
if (((bitField0_ & 0x00000040) == 0x00000040) &&
command_ != null &&
command_ != org.apache.mesos.v1.Protos.CommandInfo.getDefaultInstance()) {
command_ =
org.apache.mesos.v1.Protos.CommandInfo.newBuilder(command_).mergeFrom(value).buildPartial();
} else {
command_ = value;
}
onChanged();
} else {
commandBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000040;
return this;
}
/**
*
* Command health check.
*
*
* optional .mesos.v1.CommandInfo command = 7;
*/
public Builder clearCommand() {
if (commandBuilder_ == null) {
command_ = null;
onChanged();
} else {
commandBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000040);
return this;
}
/**
*
* Command health check.
*
*
* optional .mesos.v1.CommandInfo command = 7;
*/
public org.apache.mesos.v1.Protos.CommandInfo.Builder getCommandBuilder() {
bitField0_ |= 0x00000040;
onChanged();
return getCommandFieldBuilder().getBuilder();
}
/**
*
* Command health check.
*
*
* optional .mesos.v1.CommandInfo command = 7;
*/
public org.apache.mesos.v1.Protos.CommandInfoOrBuilder getCommandOrBuilder() {
if (commandBuilder_ != null) {
return commandBuilder_.getMessageOrBuilder();
} else {
return command_ == null ?
org.apache.mesos.v1.Protos.CommandInfo.getDefaultInstance() : command_;
}
}
/**
*
* Command health check.
*
*
* optional .mesos.v1.CommandInfo command = 7;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.apache.mesos.v1.Protos.CommandInfo, org.apache.mesos.v1.Protos.CommandInfo.Builder, org.apache.mesos.v1.Protos.CommandInfoOrBuilder>
getCommandFieldBuilder() {
if (commandBuilder_ == null) {
commandBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.apache.mesos.v1.Protos.CommandInfo, org.apache.mesos.v1.Protos.CommandInfo.Builder, org.apache.mesos.v1.Protos.CommandInfoOrBuilder>(
getCommand(),
getParentForChildren(),
isClean());
command_ = null;
}
return commandBuilder_;
}
private org.apache.mesos.v1.Protos.HealthCheck.HTTPCheckInfo http_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
org.apache.mesos.v1.Protos.HealthCheck.HTTPCheckInfo, org.apache.mesos.v1.Protos.HealthCheck.HTTPCheckInfo.Builder, org.apache.mesos.v1.Protos.HealthCheck.HTTPCheckInfoOrBuilder> httpBuilder_;
/**
*
* HTTP health check.
*
*
* optional .mesos.v1.HealthCheck.HTTPCheckInfo http = 1;
*/
public boolean hasHttp() {
return ((bitField0_ & 0x00000080) == 0x00000080);
}
/**
*
* HTTP health check.
*
*
* optional .mesos.v1.HealthCheck.HTTPCheckInfo http = 1;
*/
public org.apache.mesos.v1.Protos.HealthCheck.HTTPCheckInfo getHttp() {
if (httpBuilder_ == null) {
return http_ == null ? org.apache.mesos.v1.Protos.HealthCheck.HTTPCheckInfo.getDefaultInstance() : http_;
} else {
return httpBuilder_.getMessage();
}
}
/**
*
* HTTP health check.
*
*
* optional .mesos.v1.HealthCheck.HTTPCheckInfo http = 1;
*/
public Builder setHttp(org.apache.mesos.v1.Protos.HealthCheck.HTTPCheckInfo value) {
if (httpBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
http_ = value;
onChanged();
} else {
httpBuilder_.setMessage(value);
}
bitField0_ |= 0x00000080;
return this;
}
/**
*
* HTTP health check.
*
*
* optional .mesos.v1.HealthCheck.HTTPCheckInfo http = 1;
*/
public Builder setHttp(
org.apache.mesos.v1.Protos.HealthCheck.HTTPCheckInfo.Builder builderForValue) {
if (httpBuilder_ == null) {
http_ = builderForValue.build();
onChanged();
} else {
httpBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000080;
return this;
}
/**
*
* HTTP health check.
*
*
* optional .mesos.v1.HealthCheck.HTTPCheckInfo http = 1;
*/
public Builder mergeHttp(org.apache.mesos.v1.Protos.HealthCheck.HTTPCheckInfo value) {
if (httpBuilder_ == null) {
if (((bitField0_ & 0x00000080) == 0x00000080) &&
http_ != null &&
http_ != org.apache.mesos.v1.Protos.HealthCheck.HTTPCheckInfo.getDefaultInstance()) {
http_ =
org.apache.mesos.v1.Protos.HealthCheck.HTTPCheckInfo.newBuilder(http_).mergeFrom(value).buildPartial();
} else {
http_ = value;
}
onChanged();
} else {
httpBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000080;
return this;
}
/**
*
* HTTP health check.
*
*
* optional .mesos.v1.HealthCheck.HTTPCheckInfo http = 1;
*/
public Builder clearHttp() {
if (httpBuilder_ == null) {
http_ = null;
onChanged();
} else {
httpBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000080);
return this;
}
/**
*
* HTTP health check.
*
*
* optional .mesos.v1.HealthCheck.HTTPCheckInfo http = 1;
*/
public org.apache.mesos.v1.Protos.HealthCheck.HTTPCheckInfo.Builder getHttpBuilder() {
bitField0_ |= 0x00000080;
onChanged();
return getHttpFieldBuilder().getBuilder();
}
/**
*
* HTTP health check.
*
*
* optional .mesos.v1.HealthCheck.HTTPCheckInfo http = 1;
*/
public org.apache.mesos.v1.Protos.HealthCheck.HTTPCheckInfoOrBuilder getHttpOrBuilder() {
if (httpBuilder_ != null) {
return httpBuilder_.getMessageOrBuilder();
} else {
return http_ == null ?
org.apache.mesos.v1.Protos.HealthCheck.HTTPCheckInfo.getDefaultInstance() : http_;
}
}
/**
*
* HTTP health check.
*
*
* optional .mesos.v1.HealthCheck.HTTPCheckInfo http = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.apache.mesos.v1.Protos.HealthCheck.HTTPCheckInfo, org.apache.mesos.v1.Protos.HealthCheck.HTTPCheckInfo.Builder, org.apache.mesos.v1.Protos.HealthCheck.HTTPCheckInfoOrBuilder>
getHttpFieldBuilder() {
if (httpBuilder_ == null) {
httpBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.apache.mesos.v1.Protos.HealthCheck.HTTPCheckInfo, org.apache.mesos.v1.Protos.HealthCheck.HTTPCheckInfo.Builder, org.apache.mesos.v1.Protos.HealthCheck.HTTPCheckInfoOrBuilder>(
getHttp(),
getParentForChildren(),
isClean());
http_ = null;
}
return httpBuilder_;
}
private org.apache.mesos.v1.Protos.HealthCheck.TCPCheckInfo tcp_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
org.apache.mesos.v1.Protos.HealthCheck.TCPCheckInfo, org.apache.mesos.v1.Protos.HealthCheck.TCPCheckInfo.Builder, org.apache.mesos.v1.Protos.HealthCheck.TCPCheckInfoOrBuilder> tcpBuilder_;
/**
*
* TCP health check.
*
*
* optional .mesos.v1.HealthCheck.TCPCheckInfo tcp = 9;
*/
public boolean hasTcp() {
return ((bitField0_ & 0x00000100) == 0x00000100);
}
/**
*
* TCP health check.
*
*
* optional .mesos.v1.HealthCheck.TCPCheckInfo tcp = 9;
*/
public org.apache.mesos.v1.Protos.HealthCheck.TCPCheckInfo getTcp() {
if (tcpBuilder_ == null) {
return tcp_ == null ? org.apache.mesos.v1.Protos.HealthCheck.TCPCheckInfo.getDefaultInstance() : tcp_;
} else {
return tcpBuilder_.getMessage();
}
}
/**
*
* TCP health check.
*
*
* optional .mesos.v1.HealthCheck.TCPCheckInfo tcp = 9;
*/
public Builder setTcp(org.apache.mesos.v1.Protos.HealthCheck.TCPCheckInfo value) {
if (tcpBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
tcp_ = value;
onChanged();
} else {
tcpBuilder_.setMessage(value);
}
bitField0_ |= 0x00000100;
return this;
}
/**
*
* TCP health check.
*
*
* optional .mesos.v1.HealthCheck.TCPCheckInfo tcp = 9;
*/
public Builder setTcp(
org.apache.mesos.v1.Protos.HealthCheck.TCPCheckInfo.Builder builderForValue) {
if (tcpBuilder_ == null) {
tcp_ = builderForValue.build();
onChanged();
} else {
tcpBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000100;
return this;
}
/**
*
* TCP health check.
*
*
* optional .mesos.v1.HealthCheck.TCPCheckInfo tcp = 9;
*/
public Builder mergeTcp(org.apache.mesos.v1.Protos.HealthCheck.TCPCheckInfo value) {
if (tcpBuilder_ == null) {
if (((bitField0_ & 0x00000100) == 0x00000100) &&
tcp_ != null &&
tcp_ != org.apache.mesos.v1.Protos.HealthCheck.TCPCheckInfo.getDefaultInstance()) {
tcp_ =
org.apache.mesos.v1.Protos.HealthCheck.TCPCheckInfo.newBuilder(tcp_).mergeFrom(value).buildPartial();
} else {
tcp_ = value;
}
onChanged();
} else {
tcpBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000100;
return this;
}
/**
*
* TCP health check.
*
*
* optional .mesos.v1.HealthCheck.TCPCheckInfo tcp = 9;
*/
public Builder clearTcp() {
if (tcpBuilder_ == null) {
tcp_ = null;
onChanged();
} else {
tcpBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000100);
return this;
}
/**
*
* TCP health check.
*
*
* optional .mesos.v1.HealthCheck.TCPCheckInfo tcp = 9;
*/
public org.apache.mesos.v1.Protos.HealthCheck.TCPCheckInfo.Builder getTcpBuilder() {
bitField0_ |= 0x00000100;
onChanged();
return getTcpFieldBuilder().getBuilder();
}
/**
*
* TCP health check.
*
*
* optional .mesos.v1.HealthCheck.TCPCheckInfo tcp = 9;
*/
public org.apache.mesos.v1.Protos.HealthCheck.TCPCheckInfoOrBuilder getTcpOrBuilder() {
if (tcpBuilder_ != null) {
return tcpBuilder_.getMessageOrBuilder();
} else {
return tcp_ == null ?
org.apache.mesos.v1.Protos.HealthCheck.TCPCheckInfo.getDefaultInstance() : tcp_;
}
}
/**
*
* TCP health check.
*
*
* optional .mesos.v1.HealthCheck.TCPCheckInfo tcp = 9;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.apache.mesos.v1.Protos.HealthCheck.TCPCheckInfo, org.apache.mesos.v1.Protos.HealthCheck.TCPCheckInfo.Builder, org.apache.mesos.v1.Protos.HealthCheck.TCPCheckInfoOrBuilder>
getTcpFieldBuilder() {
if (tcpBuilder_ == null) {
tcpBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.apache.mesos.v1.Protos.HealthCheck.TCPCheckInfo, org.apache.mesos.v1.Protos.HealthCheck.TCPCheckInfo.Builder, org.apache.mesos.v1.Protos.HealthCheck.TCPCheckInfoOrBuilder>(
getTcp(),
getParentForChildren(),
isClean());
tcp_ = null;
}
return tcpBuilder_;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:mesos.v1.HealthCheck)
}
// @@protoc_insertion_point(class_scope:mesos.v1.HealthCheck)
private static final org.apache.mesos.v1.Protos.HealthCheck DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.apache.mesos.v1.Protos.HealthCheck();
}
public static org.apache.mesos.v1.Protos.HealthCheck getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public HealthCheck parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new HealthCheck(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.apache.mesos.v1.Protos.HealthCheck getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface KillPolicyOrBuilder extends
// @@protoc_insertion_point(interface_extends:mesos.v1.KillPolicy)
com.google.protobuf.MessageOrBuilder {
/**
*
* The grace period specifies how long to wait before forcibly
* killing the task. It is recommended to attempt to gracefully
* kill the task (and send TASK_KILLING) to indicate that the
* graceful kill is in progress. Once the grace period elapses,
* if the task has not terminated, a forcible kill should occur.
* The task should not assume that it will always be allotted
* the full grace period. For example, the executor may be
* shutdown more quickly by the agent, or failures / forcible
* terminations may occur.
*
*
* optional .mesos.v1.DurationInfo grace_period = 1;
*/
boolean hasGracePeriod();
/**
*
* The grace period specifies how long to wait before forcibly
* killing the task. It is recommended to attempt to gracefully
* kill the task (and send TASK_KILLING) to indicate that the
* graceful kill is in progress. Once the grace period elapses,
* if the task has not terminated, a forcible kill should occur.
* The task should not assume that it will always be allotted
* the full grace period. For example, the executor may be
* shutdown more quickly by the agent, or failures / forcible
* terminations may occur.
*
*
* optional .mesos.v1.DurationInfo grace_period = 1;
*/
org.apache.mesos.v1.Protos.DurationInfo getGracePeriod();
/**
*
* The grace period specifies how long to wait before forcibly
* killing the task. It is recommended to attempt to gracefully
* kill the task (and send TASK_KILLING) to indicate that the
* graceful kill is in progress. Once the grace period elapses,
* if the task has not terminated, a forcible kill should occur.
* The task should not assume that it will always be allotted
* the full grace period. For example, the executor may be
* shutdown more quickly by the agent, or failures / forcible
* terminations may occur.
*
*
* optional .mesos.v1.DurationInfo grace_period = 1;
*/
org.apache.mesos.v1.Protos.DurationInfoOrBuilder getGracePeriodOrBuilder();
}
/**
*
**
* Describes a kill policy for a task. Currently does not express
* different policies (e.g. hitting HTTP endpoints), only controls
* how long to wait between graceful and forcible task kill:
* graceful kill --------------> forcible kill
* grace_period
* Kill policies are best-effort, because machine failures / forcible
* terminations may occur.
* NOTE: For executor-less command-based tasks, the kill is performed
* via sending a signal to the task process: SIGTERM for the graceful
* kill and SIGKILL for the forcible kill. For the docker executor-less
* tasks the grace period is passed to 'docker stop --time'.
*
*
* Protobuf type {@code mesos.v1.KillPolicy}
*/
public static final class KillPolicy extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:mesos.v1.KillPolicy)
KillPolicyOrBuilder {
private static final long serialVersionUID = 0L;
// Use KillPolicy.newBuilder() to construct.
private KillPolicy(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private KillPolicy() {
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private KillPolicy(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
org.apache.mesos.v1.Protos.DurationInfo.Builder subBuilder = null;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
subBuilder = gracePeriod_.toBuilder();
}
gracePeriod_ = input.readMessage(org.apache.mesos.v1.Protos.DurationInfo.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(gracePeriod_);
gracePeriod_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000001;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_KillPolicy_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_KillPolicy_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.mesos.v1.Protos.KillPolicy.class, org.apache.mesos.v1.Protos.KillPolicy.Builder.class);
}
private int bitField0_;
public static final int GRACE_PERIOD_FIELD_NUMBER = 1;
private org.apache.mesos.v1.Protos.DurationInfo gracePeriod_;
/**
*
* The grace period specifies how long to wait before forcibly
* killing the task. It is recommended to attempt to gracefully
* kill the task (and send TASK_KILLING) to indicate that the
* graceful kill is in progress. Once the grace period elapses,
* if the task has not terminated, a forcible kill should occur.
* The task should not assume that it will always be allotted
* the full grace period. For example, the executor may be
* shutdown more quickly by the agent, or failures / forcible
* terminations may occur.
*
*
* optional .mesos.v1.DurationInfo grace_period = 1;
*/
public boolean hasGracePeriod() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
*
* The grace period specifies how long to wait before forcibly
* killing the task. It is recommended to attempt to gracefully
* kill the task (and send TASK_KILLING) to indicate that the
* graceful kill is in progress. Once the grace period elapses,
* if the task has not terminated, a forcible kill should occur.
* The task should not assume that it will always be allotted
* the full grace period. For example, the executor may be
* shutdown more quickly by the agent, or failures / forcible
* terminations may occur.
*
*
* optional .mesos.v1.DurationInfo grace_period = 1;
*/
public org.apache.mesos.v1.Protos.DurationInfo getGracePeriod() {
return gracePeriod_ == null ? org.apache.mesos.v1.Protos.DurationInfo.getDefaultInstance() : gracePeriod_;
}
/**
*
* The grace period specifies how long to wait before forcibly
* killing the task. It is recommended to attempt to gracefully
* kill the task (and send TASK_KILLING) to indicate that the
* graceful kill is in progress. Once the grace period elapses,
* if the task has not terminated, a forcible kill should occur.
* The task should not assume that it will always be allotted
* the full grace period. For example, the executor may be
* shutdown more quickly by the agent, or failures / forcible
* terminations may occur.
*
*
* optional .mesos.v1.DurationInfo grace_period = 1;
*/
public org.apache.mesos.v1.Protos.DurationInfoOrBuilder getGracePeriodOrBuilder() {
return gracePeriod_ == null ? org.apache.mesos.v1.Protos.DurationInfo.getDefaultInstance() : gracePeriod_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (hasGracePeriod()) {
if (!getGracePeriod().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeMessage(1, getGracePeriod());
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getGracePeriod());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.mesos.v1.Protos.KillPolicy)) {
return super.equals(obj);
}
org.apache.mesos.v1.Protos.KillPolicy other = (org.apache.mesos.v1.Protos.KillPolicy) obj;
boolean result = true;
result = result && (hasGracePeriod() == other.hasGracePeriod());
if (hasGracePeriod()) {
result = result && getGracePeriod()
.equals(other.getGracePeriod());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasGracePeriod()) {
hash = (37 * hash) + GRACE_PERIOD_FIELD_NUMBER;
hash = (53 * hash) + getGracePeriod().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.mesos.v1.Protos.KillPolicy parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.KillPolicy parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.KillPolicy parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.KillPolicy parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.KillPolicy parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.KillPolicy parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.KillPolicy parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.KillPolicy parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.KillPolicy parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.KillPolicy parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.KillPolicy parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.KillPolicy parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.apache.mesos.v1.Protos.KillPolicy prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
**
* Describes a kill policy for a task. Currently does not express
* different policies (e.g. hitting HTTP endpoints), only controls
* how long to wait between graceful and forcible task kill:
* graceful kill --------------> forcible kill
* grace_period
* Kill policies are best-effort, because machine failures / forcible
* terminations may occur.
* NOTE: For executor-less command-based tasks, the kill is performed
* via sending a signal to the task process: SIGTERM for the graceful
* kill and SIGKILL for the forcible kill. For the docker executor-less
* tasks the grace period is passed to 'docker stop --time'.
*
*
* Protobuf type {@code mesos.v1.KillPolicy}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:mesos.v1.KillPolicy)
org.apache.mesos.v1.Protos.KillPolicyOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_KillPolicy_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_KillPolicy_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.mesos.v1.Protos.KillPolicy.class, org.apache.mesos.v1.Protos.KillPolicy.Builder.class);
}
// Construct using org.apache.mesos.v1.Protos.KillPolicy.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getGracePeriodFieldBuilder();
}
}
public Builder clear() {
super.clear();
if (gracePeriodBuilder_ == null) {
gracePeriod_ = null;
} else {
gracePeriodBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_KillPolicy_descriptor;
}
public org.apache.mesos.v1.Protos.KillPolicy getDefaultInstanceForType() {
return org.apache.mesos.v1.Protos.KillPolicy.getDefaultInstance();
}
public org.apache.mesos.v1.Protos.KillPolicy build() {
org.apache.mesos.v1.Protos.KillPolicy result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.apache.mesos.v1.Protos.KillPolicy buildPartial() {
org.apache.mesos.v1.Protos.KillPolicy result = new org.apache.mesos.v1.Protos.KillPolicy(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
if (gracePeriodBuilder_ == null) {
result.gracePeriod_ = gracePeriod_;
} else {
result.gracePeriod_ = gracePeriodBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.apache.mesos.v1.Protos.KillPolicy) {
return mergeFrom((org.apache.mesos.v1.Protos.KillPolicy)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.mesos.v1.Protos.KillPolicy other) {
if (other == org.apache.mesos.v1.Protos.KillPolicy.getDefaultInstance()) return this;
if (other.hasGracePeriod()) {
mergeGracePeriod(other.getGracePeriod());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
if (hasGracePeriod()) {
if (!getGracePeriod().isInitialized()) {
return false;
}
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.mesos.v1.Protos.KillPolicy parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.mesos.v1.Protos.KillPolicy) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private org.apache.mesos.v1.Protos.DurationInfo gracePeriod_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
org.apache.mesos.v1.Protos.DurationInfo, org.apache.mesos.v1.Protos.DurationInfo.Builder, org.apache.mesos.v1.Protos.DurationInfoOrBuilder> gracePeriodBuilder_;
/**
*
* The grace period specifies how long to wait before forcibly
* killing the task. It is recommended to attempt to gracefully
* kill the task (and send TASK_KILLING) to indicate that the
* graceful kill is in progress. Once the grace period elapses,
* if the task has not terminated, a forcible kill should occur.
* The task should not assume that it will always be allotted
* the full grace period. For example, the executor may be
* shutdown more quickly by the agent, or failures / forcible
* terminations may occur.
*
*
* optional .mesos.v1.DurationInfo grace_period = 1;
*/
public boolean hasGracePeriod() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
*
* The grace period specifies how long to wait before forcibly
* killing the task. It is recommended to attempt to gracefully
* kill the task (and send TASK_KILLING) to indicate that the
* graceful kill is in progress. Once the grace period elapses,
* if the task has not terminated, a forcible kill should occur.
* The task should not assume that it will always be allotted
* the full grace period. For example, the executor may be
* shutdown more quickly by the agent, or failures / forcible
* terminations may occur.
*
*
* optional .mesos.v1.DurationInfo grace_period = 1;
*/
public org.apache.mesos.v1.Protos.DurationInfo getGracePeriod() {
if (gracePeriodBuilder_ == null) {
return gracePeriod_ == null ? org.apache.mesos.v1.Protos.DurationInfo.getDefaultInstance() : gracePeriod_;
} else {
return gracePeriodBuilder_.getMessage();
}
}
/**
*
* The grace period specifies how long to wait before forcibly
* killing the task. It is recommended to attempt to gracefully
* kill the task (and send TASK_KILLING) to indicate that the
* graceful kill is in progress. Once the grace period elapses,
* if the task has not terminated, a forcible kill should occur.
* The task should not assume that it will always be allotted
* the full grace period. For example, the executor may be
* shutdown more quickly by the agent, or failures / forcible
* terminations may occur.
*
*
* optional .mesos.v1.DurationInfo grace_period = 1;
*/
public Builder setGracePeriod(org.apache.mesos.v1.Protos.DurationInfo value) {
if (gracePeriodBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
gracePeriod_ = value;
onChanged();
} else {
gracePeriodBuilder_.setMessage(value);
}
bitField0_ |= 0x00000001;
return this;
}
/**
*
* The grace period specifies how long to wait before forcibly
* killing the task. It is recommended to attempt to gracefully
* kill the task (and send TASK_KILLING) to indicate that the
* graceful kill is in progress. Once the grace period elapses,
* if the task has not terminated, a forcible kill should occur.
* The task should not assume that it will always be allotted
* the full grace period. For example, the executor may be
* shutdown more quickly by the agent, or failures / forcible
* terminations may occur.
*
*
* optional .mesos.v1.DurationInfo grace_period = 1;
*/
public Builder setGracePeriod(
org.apache.mesos.v1.Protos.DurationInfo.Builder builderForValue) {
if (gracePeriodBuilder_ == null) {
gracePeriod_ = builderForValue.build();
onChanged();
} else {
gracePeriodBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000001;
return this;
}
/**
*
* The grace period specifies how long to wait before forcibly
* killing the task. It is recommended to attempt to gracefully
* kill the task (and send TASK_KILLING) to indicate that the
* graceful kill is in progress. Once the grace period elapses,
* if the task has not terminated, a forcible kill should occur.
* The task should not assume that it will always be allotted
* the full grace period. For example, the executor may be
* shutdown more quickly by the agent, or failures / forcible
* terminations may occur.
*
*
* optional .mesos.v1.DurationInfo grace_period = 1;
*/
public Builder mergeGracePeriod(org.apache.mesos.v1.Protos.DurationInfo value) {
if (gracePeriodBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001) &&
gracePeriod_ != null &&
gracePeriod_ != org.apache.mesos.v1.Protos.DurationInfo.getDefaultInstance()) {
gracePeriod_ =
org.apache.mesos.v1.Protos.DurationInfo.newBuilder(gracePeriod_).mergeFrom(value).buildPartial();
} else {
gracePeriod_ = value;
}
onChanged();
} else {
gracePeriodBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000001;
return this;
}
/**
*
* The grace period specifies how long to wait before forcibly
* killing the task. It is recommended to attempt to gracefully
* kill the task (and send TASK_KILLING) to indicate that the
* graceful kill is in progress. Once the grace period elapses,
* if the task has not terminated, a forcible kill should occur.
* The task should not assume that it will always be allotted
* the full grace period. For example, the executor may be
* shutdown more quickly by the agent, or failures / forcible
* terminations may occur.
*
*
* optional .mesos.v1.DurationInfo grace_period = 1;
*/
public Builder clearGracePeriod() {
if (gracePeriodBuilder_ == null) {
gracePeriod_ = null;
onChanged();
} else {
gracePeriodBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
/**
*
* The grace period specifies how long to wait before forcibly
* killing the task. It is recommended to attempt to gracefully
* kill the task (and send TASK_KILLING) to indicate that the
* graceful kill is in progress. Once the grace period elapses,
* if the task has not terminated, a forcible kill should occur.
* The task should not assume that it will always be allotted
* the full grace period. For example, the executor may be
* shutdown more quickly by the agent, or failures / forcible
* terminations may occur.
*
*
* optional .mesos.v1.DurationInfo grace_period = 1;
*/
public org.apache.mesos.v1.Protos.DurationInfo.Builder getGracePeriodBuilder() {
bitField0_ |= 0x00000001;
onChanged();
return getGracePeriodFieldBuilder().getBuilder();
}
/**
*
* The grace period specifies how long to wait before forcibly
* killing the task. It is recommended to attempt to gracefully
* kill the task (and send TASK_KILLING) to indicate that the
* graceful kill is in progress. Once the grace period elapses,
* if the task has not terminated, a forcible kill should occur.
* The task should not assume that it will always be allotted
* the full grace period. For example, the executor may be
* shutdown more quickly by the agent, or failures / forcible
* terminations may occur.
*
*
* optional .mesos.v1.DurationInfo grace_period = 1;
*/
public org.apache.mesos.v1.Protos.DurationInfoOrBuilder getGracePeriodOrBuilder() {
if (gracePeriodBuilder_ != null) {
return gracePeriodBuilder_.getMessageOrBuilder();
} else {
return gracePeriod_ == null ?
org.apache.mesos.v1.Protos.DurationInfo.getDefaultInstance() : gracePeriod_;
}
}
/**
*
* The grace period specifies how long to wait before forcibly
* killing the task. It is recommended to attempt to gracefully
* kill the task (and send TASK_KILLING) to indicate that the
* graceful kill is in progress. Once the grace period elapses,
* if the task has not terminated, a forcible kill should occur.
* The task should not assume that it will always be allotted
* the full grace period. For example, the executor may be
* shutdown more quickly by the agent, or failures / forcible
* terminations may occur.
*
*
* optional .mesos.v1.DurationInfo grace_period = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.apache.mesos.v1.Protos.DurationInfo, org.apache.mesos.v1.Protos.DurationInfo.Builder, org.apache.mesos.v1.Protos.DurationInfoOrBuilder>
getGracePeriodFieldBuilder() {
if (gracePeriodBuilder_ == null) {
gracePeriodBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.apache.mesos.v1.Protos.DurationInfo, org.apache.mesos.v1.Protos.DurationInfo.Builder, org.apache.mesos.v1.Protos.DurationInfoOrBuilder>(
getGracePeriod(),
getParentForChildren(),
isClean());
gracePeriod_ = null;
}
return gracePeriodBuilder_;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:mesos.v1.KillPolicy)
}
// @@protoc_insertion_point(class_scope:mesos.v1.KillPolicy)
private static final org.apache.mesos.v1.Protos.KillPolicy DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.apache.mesos.v1.Protos.KillPolicy();
}
public static org.apache.mesos.v1.Protos.KillPolicy getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public KillPolicy parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new KillPolicy(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.apache.mesos.v1.Protos.KillPolicy getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface CommandInfoOrBuilder extends
// @@protoc_insertion_point(interface_extends:mesos.v1.CommandInfo)
com.google.protobuf.MessageOrBuilder {
/**
* repeated .mesos.v1.CommandInfo.URI uris = 1;
*/
java.util.List
getUrisList();
/**
* repeated .mesos.v1.CommandInfo.URI uris = 1;
*/
org.apache.mesos.v1.Protos.CommandInfo.URI getUris(int index);
/**
* repeated .mesos.v1.CommandInfo.URI uris = 1;
*/
int getUrisCount();
/**
* repeated .mesos.v1.CommandInfo.URI uris = 1;
*/
java.util.List extends org.apache.mesos.v1.Protos.CommandInfo.URIOrBuilder>
getUrisOrBuilderList();
/**
* repeated .mesos.v1.CommandInfo.URI uris = 1;
*/
org.apache.mesos.v1.Protos.CommandInfo.URIOrBuilder getUrisOrBuilder(
int index);
/**
* optional .mesos.v1.Environment environment = 2;
*/
boolean hasEnvironment();
/**
* optional .mesos.v1.Environment environment = 2;
*/
org.apache.mesos.v1.Protos.Environment getEnvironment();
/**
* optional .mesos.v1.Environment environment = 2;
*/
org.apache.mesos.v1.Protos.EnvironmentOrBuilder getEnvironmentOrBuilder();
/**
*
* There are two ways to specify the command:
* 1) If 'shell == true', the command will be launched via shell
* (i.e., /bin/sh -c 'value'). The 'value' specified will be
* treated as the shell command. The 'arguments' will be ignored.
* 2) If 'shell == false', the command will be launched by passing
* arguments to an executable. The 'value' specified will be
* treated as the filename of the executable. The 'arguments'
* will be treated as the arguments to the executable. This is
* similar to how POSIX exec families launch processes (i.e.,
* execlp(value, arguments(0), arguments(1), ...)).
* NOTE: The field 'value' is changed from 'required' to 'optional'
* in 0.20.0. It will only cause issues if a new framework is
* connecting to an old master.
*
*
* optional bool shell = 6 [default = true];
*/
boolean hasShell();
/**
*
* There are two ways to specify the command:
* 1) If 'shell == true', the command will be launched via shell
* (i.e., /bin/sh -c 'value'). The 'value' specified will be
* treated as the shell command. The 'arguments' will be ignored.
* 2) If 'shell == false', the command will be launched by passing
* arguments to an executable. The 'value' specified will be
* treated as the filename of the executable. The 'arguments'
* will be treated as the arguments to the executable. This is
* similar to how POSIX exec families launch processes (i.e.,
* execlp(value, arguments(0), arguments(1), ...)).
* NOTE: The field 'value' is changed from 'required' to 'optional'
* in 0.20.0. It will only cause issues if a new framework is
* connecting to an old master.
*
*
* optional bool shell = 6 [default = true];
*/
boolean getShell();
/**
* optional string value = 3;
*/
boolean hasValue();
/**
* optional string value = 3;
*/
java.lang.String getValue();
/**
* optional string value = 3;
*/
com.google.protobuf.ByteString
getValueBytes();
/**
* repeated string arguments = 7;
*/
java.util.List
getArgumentsList();
/**
* repeated string arguments = 7;
*/
int getArgumentsCount();
/**
* repeated string arguments = 7;
*/
java.lang.String getArguments(int index);
/**
* repeated string arguments = 7;
*/
com.google.protobuf.ByteString
getArgumentsBytes(int index);
/**
*
* Enables executor and tasks to run as a specific user. If the user
* field is present both in FrameworkInfo and here, the CommandInfo
* user value takes precedence.
*
*
* optional string user = 5;
*/
boolean hasUser();
/**
*
* Enables executor and tasks to run as a specific user. If the user
* field is present both in FrameworkInfo and here, the CommandInfo
* user value takes precedence.
*
*
* optional string user = 5;
*/
java.lang.String getUser();
/**
*
* Enables executor and tasks to run as a specific user. If the user
* field is present both in FrameworkInfo and here, the CommandInfo
* user value takes precedence.
*
*
* optional string user = 5;
*/
com.google.protobuf.ByteString
getUserBytes();
}
/**
*
**
* Describes a command, executed via: '/bin/sh -c value'. Any URIs specified
* are fetched before executing the command. If the executable field for an
* uri is set, executable file permission is set on the downloaded file.
* Otherwise, if the downloaded file has a recognized archive extension
* (currently [compressed] tar and zip) it is extracted into the executor's
* working directory. This extraction can be disabled by setting `extract` to
* false. In addition, any environment variables are set before executing
* the command (so they can be used to "parameterize" your command).
*
*
* Protobuf type {@code mesos.v1.CommandInfo}
*/
public static final class CommandInfo extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:mesos.v1.CommandInfo)
CommandInfoOrBuilder {
private static final long serialVersionUID = 0L;
// Use CommandInfo.newBuilder() to construct.
private CommandInfo(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private CommandInfo() {
uris_ = java.util.Collections.emptyList();
shell_ = true;
value_ = "";
arguments_ = com.google.protobuf.LazyStringArrayList.EMPTY;
user_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private CommandInfo(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
uris_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
uris_.add(
input.readMessage(org.apache.mesos.v1.Protos.CommandInfo.URI.PARSER, extensionRegistry));
break;
}
case 18: {
org.apache.mesos.v1.Protos.Environment.Builder subBuilder = null;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
subBuilder = environment_.toBuilder();
}
environment_ = input.readMessage(org.apache.mesos.v1.Protos.Environment.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(environment_);
environment_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000001;
break;
}
case 26: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000004;
value_ = bs;
break;
}
case 42: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000008;
user_ = bs;
break;
}
case 48: {
bitField0_ |= 0x00000002;
shell_ = input.readBool();
break;
}
case 58: {
com.google.protobuf.ByteString bs = input.readBytes();
if (!((mutable_bitField0_ & 0x00000010) == 0x00000010)) {
arguments_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000010;
}
arguments_.add(bs);
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
uris_ = java.util.Collections.unmodifiableList(uris_);
}
if (((mutable_bitField0_ & 0x00000010) == 0x00000010)) {
arguments_ = arguments_.getUnmodifiableView();
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_CommandInfo_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_CommandInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.mesos.v1.Protos.CommandInfo.class, org.apache.mesos.v1.Protos.CommandInfo.Builder.class);
}
public interface URIOrBuilder extends
// @@protoc_insertion_point(interface_extends:mesos.v1.CommandInfo.URI)
com.google.protobuf.MessageOrBuilder {
/**
* required string value = 1;
*/
boolean hasValue();
/**
* required string value = 1;
*/
java.lang.String getValue();
/**
* required string value = 1;
*/
com.google.protobuf.ByteString
getValueBytes();
/**
* optional bool executable = 2;
*/
boolean hasExecutable();
/**
* optional bool executable = 2;
*/
boolean getExecutable();
/**
*
* In case the fetched file is recognized as an archive, extract
* its contents into the sandbox. Note that a cached archive is
* not copied from the cache to the sandbox in case extraction
* originates from an archive in the cache.
*
*
* optional bool extract = 3 [default = true];
*/
boolean hasExtract();
/**
*
* In case the fetched file is recognized as an archive, extract
* its contents into the sandbox. Note that a cached archive is
* not copied from the cache to the sandbox in case extraction
* originates from an archive in the cache.
*
*
* optional bool extract = 3 [default = true];
*/
boolean getExtract();
/**
*
* If this field is "true", the fetcher cache will be used. If not,
* fetching bypasses the cache and downloads directly into the
* sandbox directory, no matter whether a suitable cache file is
* available or not. The former directs the fetcher to download to
* the file cache, then copy from there to the sandbox. Subsequent
* fetch attempts with the same URI will omit downloading and copy
* from the cache as long as the file is resident there. Cache files
* may get evicted at any time, which then leads to renewed
* downloading. See also "docs/fetcher.md" and
* "docs/fetcher-cache-internals.md".
*
*
* optional bool cache = 4;
*/
boolean hasCache();
/**
*
* If this field is "true", the fetcher cache will be used. If not,
* fetching bypasses the cache and downloads directly into the
* sandbox directory, no matter whether a suitable cache file is
* available or not. The former directs the fetcher to download to
* the file cache, then copy from there to the sandbox. Subsequent
* fetch attempts with the same URI will omit downloading and copy
* from the cache as long as the file is resident there. Cache files
* may get evicted at any time, which then leads to renewed
* downloading. See also "docs/fetcher.md" and
* "docs/fetcher-cache-internals.md".
*
*
* optional bool cache = 4;
*/
boolean getCache();
/**
*
* The fetcher's default behavior is to use the URI string's basename to
* name the local copy. If this field is provided, the local copy will be
* named with its value instead. If there is a directory component (which
* must be a relative path), the local copy will be stored in that
* subdirectory inside the sandbox.
*
*
* optional string output_file = 5;
*/
boolean hasOutputFile();
/**
*
* The fetcher's default behavior is to use the URI string's basename to
* name the local copy. If this field is provided, the local copy will be
* named with its value instead. If there is a directory component (which
* must be a relative path), the local copy will be stored in that
* subdirectory inside the sandbox.
*
*
* optional string output_file = 5;
*/
java.lang.String getOutputFile();
/**
*
* The fetcher's default behavior is to use the URI string's basename to
* name the local copy. If this field is provided, the local copy will be
* named with its value instead. If there is a directory component (which
* must be a relative path), the local copy will be stored in that
* subdirectory inside the sandbox.
*
*
* optional string output_file = 5;
*/
com.google.protobuf.ByteString
getOutputFileBytes();
}
/**
* Protobuf type {@code mesos.v1.CommandInfo.URI}
*/
public static final class URI extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:mesos.v1.CommandInfo.URI)
URIOrBuilder {
private static final long serialVersionUID = 0L;
// Use URI.newBuilder() to construct.
private URI(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private URI() {
value_ = "";
executable_ = false;
extract_ = true;
cache_ = false;
outputFile_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private URI(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000001;
value_ = bs;
break;
}
case 16: {
bitField0_ |= 0x00000002;
executable_ = input.readBool();
break;
}
case 24: {
bitField0_ |= 0x00000004;
extract_ = input.readBool();
break;
}
case 32: {
bitField0_ |= 0x00000008;
cache_ = input.readBool();
break;
}
case 42: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000010;
outputFile_ = bs;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_CommandInfo_URI_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_CommandInfo_URI_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.mesos.v1.Protos.CommandInfo.URI.class, org.apache.mesos.v1.Protos.CommandInfo.URI.Builder.class);
}
private int bitField0_;
public static final int VALUE_FIELD_NUMBER = 1;
private volatile java.lang.Object value_;
/**
* required string value = 1;
*/
public boolean hasValue() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required string value = 1;
*/
public java.lang.String getValue() {
java.lang.Object ref = value_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
value_ = s;
}
return s;
}
}
/**
* required string value = 1;
*/
public com.google.protobuf.ByteString
getValueBytes() {
java.lang.Object ref = value_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
value_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int EXECUTABLE_FIELD_NUMBER = 2;
private boolean executable_;
/**
* optional bool executable = 2;
*/
public boolean hasExecutable() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional bool executable = 2;
*/
public boolean getExecutable() {
return executable_;
}
public static final int EXTRACT_FIELD_NUMBER = 3;
private boolean extract_;
/**
*
* In case the fetched file is recognized as an archive, extract
* its contents into the sandbox. Note that a cached archive is
* not copied from the cache to the sandbox in case extraction
* originates from an archive in the cache.
*
*
* optional bool extract = 3 [default = true];
*/
public boolean hasExtract() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
*
* In case the fetched file is recognized as an archive, extract
* its contents into the sandbox. Note that a cached archive is
* not copied from the cache to the sandbox in case extraction
* originates from an archive in the cache.
*
*
* optional bool extract = 3 [default = true];
*/
public boolean getExtract() {
return extract_;
}
public static final int CACHE_FIELD_NUMBER = 4;
private boolean cache_;
/**
*
* If this field is "true", the fetcher cache will be used. If not,
* fetching bypasses the cache and downloads directly into the
* sandbox directory, no matter whether a suitable cache file is
* available or not. The former directs the fetcher to download to
* the file cache, then copy from there to the sandbox. Subsequent
* fetch attempts with the same URI will omit downloading and copy
* from the cache as long as the file is resident there. Cache files
* may get evicted at any time, which then leads to renewed
* downloading. See also "docs/fetcher.md" and
* "docs/fetcher-cache-internals.md".
*
*
* optional bool cache = 4;
*/
public boolean hasCache() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
*
* If this field is "true", the fetcher cache will be used. If not,
* fetching bypasses the cache and downloads directly into the
* sandbox directory, no matter whether a suitable cache file is
* available or not. The former directs the fetcher to download to
* the file cache, then copy from there to the sandbox. Subsequent
* fetch attempts with the same URI will omit downloading and copy
* from the cache as long as the file is resident there. Cache files
* may get evicted at any time, which then leads to renewed
* downloading. See also "docs/fetcher.md" and
* "docs/fetcher-cache-internals.md".
*
*
* optional bool cache = 4;
*/
public boolean getCache() {
return cache_;
}
public static final int OUTPUT_FILE_FIELD_NUMBER = 5;
private volatile java.lang.Object outputFile_;
/**
*
* The fetcher's default behavior is to use the URI string's basename to
* name the local copy. If this field is provided, the local copy will be
* named with its value instead. If there is a directory component (which
* must be a relative path), the local copy will be stored in that
* subdirectory inside the sandbox.
*
*
* optional string output_file = 5;
*/
public boolean hasOutputFile() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
*
* The fetcher's default behavior is to use the URI string's basename to
* name the local copy. If this field is provided, the local copy will be
* named with its value instead. If there is a directory component (which
* must be a relative path), the local copy will be stored in that
* subdirectory inside the sandbox.
*
*
* optional string output_file = 5;
*/
public java.lang.String getOutputFile() {
java.lang.Object ref = outputFile_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
outputFile_ = s;
}
return s;
}
}
/**
*
* The fetcher's default behavior is to use the URI string's basename to
* name the local copy. If this field is provided, the local copy will be
* named with its value instead. If there is a directory component (which
* must be a relative path), the local copy will be stored in that
* subdirectory inside the sandbox.
*
*
* optional string output_file = 5;
*/
public com.google.protobuf.ByteString
getOutputFileBytes() {
java.lang.Object ref = outputFile_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
outputFile_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasValue()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, value_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeBool(2, executable_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeBool(3, extract_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeBool(4, cache_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 5, outputFile_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, value_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(2, executable_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(3, extract_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(4, cache_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(5, outputFile_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.mesos.v1.Protos.CommandInfo.URI)) {
return super.equals(obj);
}
org.apache.mesos.v1.Protos.CommandInfo.URI other = (org.apache.mesos.v1.Protos.CommandInfo.URI) obj;
boolean result = true;
result = result && (hasValue() == other.hasValue());
if (hasValue()) {
result = result && getValue()
.equals(other.getValue());
}
result = result && (hasExecutable() == other.hasExecutable());
if (hasExecutable()) {
result = result && (getExecutable()
== other.getExecutable());
}
result = result && (hasExtract() == other.hasExtract());
if (hasExtract()) {
result = result && (getExtract()
== other.getExtract());
}
result = result && (hasCache() == other.hasCache());
if (hasCache()) {
result = result && (getCache()
== other.getCache());
}
result = result && (hasOutputFile() == other.hasOutputFile());
if (hasOutputFile()) {
result = result && getOutputFile()
.equals(other.getOutputFile());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasValue()) {
hash = (37 * hash) + VALUE_FIELD_NUMBER;
hash = (53 * hash) + getValue().hashCode();
}
if (hasExecutable()) {
hash = (37 * hash) + EXECUTABLE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getExecutable());
}
if (hasExtract()) {
hash = (37 * hash) + EXTRACT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getExtract());
}
if (hasCache()) {
hash = (37 * hash) + CACHE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getCache());
}
if (hasOutputFile()) {
hash = (37 * hash) + OUTPUT_FILE_FIELD_NUMBER;
hash = (53 * hash) + getOutputFile().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.mesos.v1.Protos.CommandInfo.URI parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.CommandInfo.URI parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.CommandInfo.URI parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.CommandInfo.URI parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.CommandInfo.URI parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.CommandInfo.URI parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.CommandInfo.URI parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.CommandInfo.URI parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.CommandInfo.URI parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.CommandInfo.URI parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.CommandInfo.URI parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.CommandInfo.URI parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.apache.mesos.v1.Protos.CommandInfo.URI prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code mesos.v1.CommandInfo.URI}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:mesos.v1.CommandInfo.URI)
org.apache.mesos.v1.Protos.CommandInfo.URIOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_CommandInfo_URI_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_CommandInfo_URI_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.mesos.v1.Protos.CommandInfo.URI.class, org.apache.mesos.v1.Protos.CommandInfo.URI.Builder.class);
}
// Construct using org.apache.mesos.v1.Protos.CommandInfo.URI.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
value_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
executable_ = false;
bitField0_ = (bitField0_ & ~0x00000002);
extract_ = true;
bitField0_ = (bitField0_ & ~0x00000004);
cache_ = false;
bitField0_ = (bitField0_ & ~0x00000008);
outputFile_ = "";
bitField0_ = (bitField0_ & ~0x00000010);
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_CommandInfo_URI_descriptor;
}
public org.apache.mesos.v1.Protos.CommandInfo.URI getDefaultInstanceForType() {
return org.apache.mesos.v1.Protos.CommandInfo.URI.getDefaultInstance();
}
public org.apache.mesos.v1.Protos.CommandInfo.URI build() {
org.apache.mesos.v1.Protos.CommandInfo.URI result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.apache.mesos.v1.Protos.CommandInfo.URI buildPartial() {
org.apache.mesos.v1.Protos.CommandInfo.URI result = new org.apache.mesos.v1.Protos.CommandInfo.URI(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.value_ = value_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.executable_ = executable_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.extract_ = extract_;
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000008;
}
result.cache_ = cache_;
if (((from_bitField0_ & 0x00000010) == 0x00000010)) {
to_bitField0_ |= 0x00000010;
}
result.outputFile_ = outputFile_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.apache.mesos.v1.Protos.CommandInfo.URI) {
return mergeFrom((org.apache.mesos.v1.Protos.CommandInfo.URI)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.mesos.v1.Protos.CommandInfo.URI other) {
if (other == org.apache.mesos.v1.Protos.CommandInfo.URI.getDefaultInstance()) return this;
if (other.hasValue()) {
bitField0_ |= 0x00000001;
value_ = other.value_;
onChanged();
}
if (other.hasExecutable()) {
setExecutable(other.getExecutable());
}
if (other.hasExtract()) {
setExtract(other.getExtract());
}
if (other.hasCache()) {
setCache(other.getCache());
}
if (other.hasOutputFile()) {
bitField0_ |= 0x00000010;
outputFile_ = other.outputFile_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
if (!hasValue()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.mesos.v1.Protos.CommandInfo.URI parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.mesos.v1.Protos.CommandInfo.URI) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object value_ = "";
/**
* required string value = 1;
*/
public boolean hasValue() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required string value = 1;
*/
public java.lang.String getValue() {
java.lang.Object ref = value_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
value_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* required string value = 1;
*/
public com.google.protobuf.ByteString
getValueBytes() {
java.lang.Object ref = value_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
value_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* required string value = 1;
*/
public Builder setValue(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
value_ = value;
onChanged();
return this;
}
/**
* required string value = 1;
*/
public Builder clearValue() {
bitField0_ = (bitField0_ & ~0x00000001);
value_ = getDefaultInstance().getValue();
onChanged();
return this;
}
/**
* required string value = 1;
*/
public Builder setValueBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
value_ = value;
onChanged();
return this;
}
private boolean executable_ ;
/**
* optional bool executable = 2;
*/
public boolean hasExecutable() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional bool executable = 2;
*/
public boolean getExecutable() {
return executable_;
}
/**
* optional bool executable = 2;
*/
public Builder setExecutable(boolean value) {
bitField0_ |= 0x00000002;
executable_ = value;
onChanged();
return this;
}
/**
* optional bool executable = 2;
*/
public Builder clearExecutable() {
bitField0_ = (bitField0_ & ~0x00000002);
executable_ = false;
onChanged();
return this;
}
private boolean extract_ = true;
/**
*
* In case the fetched file is recognized as an archive, extract
* its contents into the sandbox. Note that a cached archive is
* not copied from the cache to the sandbox in case extraction
* originates from an archive in the cache.
*
*
* optional bool extract = 3 [default = true];
*/
public boolean hasExtract() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
*
* In case the fetched file is recognized as an archive, extract
* its contents into the sandbox. Note that a cached archive is
* not copied from the cache to the sandbox in case extraction
* originates from an archive in the cache.
*
*
* optional bool extract = 3 [default = true];
*/
public boolean getExtract() {
return extract_;
}
/**
*
* In case the fetched file is recognized as an archive, extract
* its contents into the sandbox. Note that a cached archive is
* not copied from the cache to the sandbox in case extraction
* originates from an archive in the cache.
*
*
* optional bool extract = 3 [default = true];
*/
public Builder setExtract(boolean value) {
bitField0_ |= 0x00000004;
extract_ = value;
onChanged();
return this;
}
/**
*
* In case the fetched file is recognized as an archive, extract
* its contents into the sandbox. Note that a cached archive is
* not copied from the cache to the sandbox in case extraction
* originates from an archive in the cache.
*
*
* optional bool extract = 3 [default = true];
*/
public Builder clearExtract() {
bitField0_ = (bitField0_ & ~0x00000004);
extract_ = true;
onChanged();
return this;
}
private boolean cache_ ;
/**
*
* If this field is "true", the fetcher cache will be used. If not,
* fetching bypasses the cache and downloads directly into the
* sandbox directory, no matter whether a suitable cache file is
* available or not. The former directs the fetcher to download to
* the file cache, then copy from there to the sandbox. Subsequent
* fetch attempts with the same URI will omit downloading and copy
* from the cache as long as the file is resident there. Cache files
* may get evicted at any time, which then leads to renewed
* downloading. See also "docs/fetcher.md" and
* "docs/fetcher-cache-internals.md".
*
*
* optional bool cache = 4;
*/
public boolean hasCache() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
*
* If this field is "true", the fetcher cache will be used. If not,
* fetching bypasses the cache and downloads directly into the
* sandbox directory, no matter whether a suitable cache file is
* available or not. The former directs the fetcher to download to
* the file cache, then copy from there to the sandbox. Subsequent
* fetch attempts with the same URI will omit downloading and copy
* from the cache as long as the file is resident there. Cache files
* may get evicted at any time, which then leads to renewed
* downloading. See also "docs/fetcher.md" and
* "docs/fetcher-cache-internals.md".
*
*
* optional bool cache = 4;
*/
public boolean getCache() {
return cache_;
}
/**
*
* If this field is "true", the fetcher cache will be used. If not,
* fetching bypasses the cache and downloads directly into the
* sandbox directory, no matter whether a suitable cache file is
* available or not. The former directs the fetcher to download to
* the file cache, then copy from there to the sandbox. Subsequent
* fetch attempts with the same URI will omit downloading and copy
* from the cache as long as the file is resident there. Cache files
* may get evicted at any time, which then leads to renewed
* downloading. See also "docs/fetcher.md" and
* "docs/fetcher-cache-internals.md".
*
*
* optional bool cache = 4;
*/
public Builder setCache(boolean value) {
bitField0_ |= 0x00000008;
cache_ = value;
onChanged();
return this;
}
/**
*
* If this field is "true", the fetcher cache will be used. If not,
* fetching bypasses the cache and downloads directly into the
* sandbox directory, no matter whether a suitable cache file is
* available or not. The former directs the fetcher to download to
* the file cache, then copy from there to the sandbox. Subsequent
* fetch attempts with the same URI will omit downloading and copy
* from the cache as long as the file is resident there. Cache files
* may get evicted at any time, which then leads to renewed
* downloading. See also "docs/fetcher.md" and
* "docs/fetcher-cache-internals.md".
*
*
* optional bool cache = 4;
*/
public Builder clearCache() {
bitField0_ = (bitField0_ & ~0x00000008);
cache_ = false;
onChanged();
return this;
}
private java.lang.Object outputFile_ = "";
/**
*
* The fetcher's default behavior is to use the URI string's basename to
* name the local copy. If this field is provided, the local copy will be
* named with its value instead. If there is a directory component (which
* must be a relative path), the local copy will be stored in that
* subdirectory inside the sandbox.
*
*
* optional string output_file = 5;
*/
public boolean hasOutputFile() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
*
* The fetcher's default behavior is to use the URI string's basename to
* name the local copy. If this field is provided, the local copy will be
* named with its value instead. If there is a directory component (which
* must be a relative path), the local copy will be stored in that
* subdirectory inside the sandbox.
*
*
* optional string output_file = 5;
*/
public java.lang.String getOutputFile() {
java.lang.Object ref = outputFile_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
outputFile_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* The fetcher's default behavior is to use the URI string's basename to
* name the local copy. If this field is provided, the local copy will be
* named with its value instead. If there is a directory component (which
* must be a relative path), the local copy will be stored in that
* subdirectory inside the sandbox.
*
*
* optional string output_file = 5;
*/
public com.google.protobuf.ByteString
getOutputFileBytes() {
java.lang.Object ref = outputFile_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
outputFile_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* The fetcher's default behavior is to use the URI string's basename to
* name the local copy. If this field is provided, the local copy will be
* named with its value instead. If there is a directory component (which
* must be a relative path), the local copy will be stored in that
* subdirectory inside the sandbox.
*
*
* optional string output_file = 5;
*/
public Builder setOutputFile(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000010;
outputFile_ = value;
onChanged();
return this;
}
/**
*
* The fetcher's default behavior is to use the URI string's basename to
* name the local copy. If this field is provided, the local copy will be
* named with its value instead. If there is a directory component (which
* must be a relative path), the local copy will be stored in that
* subdirectory inside the sandbox.
*
*
* optional string output_file = 5;
*/
public Builder clearOutputFile() {
bitField0_ = (bitField0_ & ~0x00000010);
outputFile_ = getDefaultInstance().getOutputFile();
onChanged();
return this;
}
/**
*
* The fetcher's default behavior is to use the URI string's basename to
* name the local copy. If this field is provided, the local copy will be
* named with its value instead. If there is a directory component (which
* must be a relative path), the local copy will be stored in that
* subdirectory inside the sandbox.
*
*
* optional string output_file = 5;
*/
public Builder setOutputFileBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000010;
outputFile_ = value;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:mesos.v1.CommandInfo.URI)
}
// @@protoc_insertion_point(class_scope:mesos.v1.CommandInfo.URI)
private static final org.apache.mesos.v1.Protos.CommandInfo.URI DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.apache.mesos.v1.Protos.CommandInfo.URI();
}
public static org.apache.mesos.v1.Protos.CommandInfo.URI getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public URI parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new URI(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.apache.mesos.v1.Protos.CommandInfo.URI getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private int bitField0_;
public static final int URIS_FIELD_NUMBER = 1;
private java.util.List uris_;
/**
* repeated .mesos.v1.CommandInfo.URI uris = 1;
*/
public java.util.List getUrisList() {
return uris_;
}
/**
* repeated .mesos.v1.CommandInfo.URI uris = 1;
*/
public java.util.List extends org.apache.mesos.v1.Protos.CommandInfo.URIOrBuilder>
getUrisOrBuilderList() {
return uris_;
}
/**
* repeated .mesos.v1.CommandInfo.URI uris = 1;
*/
public int getUrisCount() {
return uris_.size();
}
/**
* repeated .mesos.v1.CommandInfo.URI uris = 1;
*/
public org.apache.mesos.v1.Protos.CommandInfo.URI getUris(int index) {
return uris_.get(index);
}
/**
* repeated .mesos.v1.CommandInfo.URI uris = 1;
*/
public org.apache.mesos.v1.Protos.CommandInfo.URIOrBuilder getUrisOrBuilder(
int index) {
return uris_.get(index);
}
public static final int ENVIRONMENT_FIELD_NUMBER = 2;
private org.apache.mesos.v1.Protos.Environment environment_;
/**
* optional .mesos.v1.Environment environment = 2;
*/
public boolean hasEnvironment() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional .mesos.v1.Environment environment = 2;
*/
public org.apache.mesos.v1.Protos.Environment getEnvironment() {
return environment_ == null ? org.apache.mesos.v1.Protos.Environment.getDefaultInstance() : environment_;
}
/**
* optional .mesos.v1.Environment environment = 2;
*/
public org.apache.mesos.v1.Protos.EnvironmentOrBuilder getEnvironmentOrBuilder() {
return environment_ == null ? org.apache.mesos.v1.Protos.Environment.getDefaultInstance() : environment_;
}
public static final int SHELL_FIELD_NUMBER = 6;
private boolean shell_;
/**
*
* There are two ways to specify the command:
* 1) If 'shell == true', the command will be launched via shell
* (i.e., /bin/sh -c 'value'). The 'value' specified will be
* treated as the shell command. The 'arguments' will be ignored.
* 2) If 'shell == false', the command will be launched by passing
* arguments to an executable. The 'value' specified will be
* treated as the filename of the executable. The 'arguments'
* will be treated as the arguments to the executable. This is
* similar to how POSIX exec families launch processes (i.e.,
* execlp(value, arguments(0), arguments(1), ...)).
* NOTE: The field 'value' is changed from 'required' to 'optional'
* in 0.20.0. It will only cause issues if a new framework is
* connecting to an old master.
*
*
* optional bool shell = 6 [default = true];
*/
public boolean hasShell() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
*
* There are two ways to specify the command:
* 1) If 'shell == true', the command will be launched via shell
* (i.e., /bin/sh -c 'value'). The 'value' specified will be
* treated as the shell command. The 'arguments' will be ignored.
* 2) If 'shell == false', the command will be launched by passing
* arguments to an executable. The 'value' specified will be
* treated as the filename of the executable. The 'arguments'
* will be treated as the arguments to the executable. This is
* similar to how POSIX exec families launch processes (i.e.,
* execlp(value, arguments(0), arguments(1), ...)).
* NOTE: The field 'value' is changed from 'required' to 'optional'
* in 0.20.0. It will only cause issues if a new framework is
* connecting to an old master.
*
*
* optional bool shell = 6 [default = true];
*/
public boolean getShell() {
return shell_;
}
public static final int VALUE_FIELD_NUMBER = 3;
private volatile java.lang.Object value_;
/**
* optional string value = 3;
*/
public boolean hasValue() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional string value = 3;
*/
public java.lang.String getValue() {
java.lang.Object ref = value_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
value_ = s;
}
return s;
}
}
/**
* optional string value = 3;
*/
public com.google.protobuf.ByteString
getValueBytes() {
java.lang.Object ref = value_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
value_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ARGUMENTS_FIELD_NUMBER = 7;
private com.google.protobuf.LazyStringList arguments_;
/**
* repeated string arguments = 7;
*/
public com.google.protobuf.ProtocolStringList
getArgumentsList() {
return arguments_;
}
/**
* repeated string arguments = 7;
*/
public int getArgumentsCount() {
return arguments_.size();
}
/**
* repeated string arguments = 7;
*/
public java.lang.String getArguments(int index) {
return arguments_.get(index);
}
/**
* repeated string arguments = 7;
*/
public com.google.protobuf.ByteString
getArgumentsBytes(int index) {
return arguments_.getByteString(index);
}
public static final int USER_FIELD_NUMBER = 5;
private volatile java.lang.Object user_;
/**
*
* Enables executor and tasks to run as a specific user. If the user
* field is present both in FrameworkInfo and here, the CommandInfo
* user value takes precedence.
*
*
* optional string user = 5;
*/
public boolean hasUser() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
*
* Enables executor and tasks to run as a specific user. If the user
* field is present both in FrameworkInfo and here, the CommandInfo
* user value takes precedence.
*
*
* optional string user = 5;
*/
public java.lang.String getUser() {
java.lang.Object ref = user_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
user_ = s;
}
return s;
}
}
/**
*
* Enables executor and tasks to run as a specific user. If the user
* field is present both in FrameworkInfo and here, the CommandInfo
* user value takes precedence.
*
*
* optional string user = 5;
*/
public com.google.protobuf.ByteString
getUserBytes() {
java.lang.Object ref = user_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
user_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
for (int i = 0; i < getUrisCount(); i++) {
if (!getUris(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
if (hasEnvironment()) {
if (!getEnvironment().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < uris_.size(); i++) {
output.writeMessage(1, uris_.get(i));
}
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeMessage(2, getEnvironment());
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, value_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 5, user_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeBool(6, shell_);
}
for (int i = 0; i < arguments_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 7, arguments_.getRaw(i));
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < uris_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, uris_.get(i));
}
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getEnvironment());
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, value_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(5, user_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(6, shell_);
}
{
int dataSize = 0;
for (int i = 0; i < arguments_.size(); i++) {
dataSize += computeStringSizeNoTag(arguments_.getRaw(i));
}
size += dataSize;
size += 1 * getArgumentsList().size();
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.mesos.v1.Protos.CommandInfo)) {
return super.equals(obj);
}
org.apache.mesos.v1.Protos.CommandInfo other = (org.apache.mesos.v1.Protos.CommandInfo) obj;
boolean result = true;
result = result && getUrisList()
.equals(other.getUrisList());
result = result && (hasEnvironment() == other.hasEnvironment());
if (hasEnvironment()) {
result = result && getEnvironment()
.equals(other.getEnvironment());
}
result = result && (hasShell() == other.hasShell());
if (hasShell()) {
result = result && (getShell()
== other.getShell());
}
result = result && (hasValue() == other.hasValue());
if (hasValue()) {
result = result && getValue()
.equals(other.getValue());
}
result = result && getArgumentsList()
.equals(other.getArgumentsList());
result = result && (hasUser() == other.hasUser());
if (hasUser()) {
result = result && getUser()
.equals(other.getUser());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getUrisCount() > 0) {
hash = (37 * hash) + URIS_FIELD_NUMBER;
hash = (53 * hash) + getUrisList().hashCode();
}
if (hasEnvironment()) {
hash = (37 * hash) + ENVIRONMENT_FIELD_NUMBER;
hash = (53 * hash) + getEnvironment().hashCode();
}
if (hasShell()) {
hash = (37 * hash) + SHELL_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getShell());
}
if (hasValue()) {
hash = (37 * hash) + VALUE_FIELD_NUMBER;
hash = (53 * hash) + getValue().hashCode();
}
if (getArgumentsCount() > 0) {
hash = (37 * hash) + ARGUMENTS_FIELD_NUMBER;
hash = (53 * hash) + getArgumentsList().hashCode();
}
if (hasUser()) {
hash = (37 * hash) + USER_FIELD_NUMBER;
hash = (53 * hash) + getUser().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.mesos.v1.Protos.CommandInfo parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.CommandInfo parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.CommandInfo parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.CommandInfo parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.CommandInfo parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.CommandInfo parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.CommandInfo parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.CommandInfo parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.CommandInfo parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.CommandInfo parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.CommandInfo parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.CommandInfo parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.apache.mesos.v1.Protos.CommandInfo prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
**
* Describes a command, executed via: '/bin/sh -c value'. Any URIs specified
* are fetched before executing the command. If the executable field for an
* uri is set, executable file permission is set on the downloaded file.
* Otherwise, if the downloaded file has a recognized archive extension
* (currently [compressed] tar and zip) it is extracted into the executor's
* working directory. This extraction can be disabled by setting `extract` to
* false. In addition, any environment variables are set before executing
* the command (so they can be used to "parameterize" your command).
*
*
* Protobuf type {@code mesos.v1.CommandInfo}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:mesos.v1.CommandInfo)
org.apache.mesos.v1.Protos.CommandInfoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_CommandInfo_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_CommandInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.mesos.v1.Protos.CommandInfo.class, org.apache.mesos.v1.Protos.CommandInfo.Builder.class);
}
// Construct using org.apache.mesos.v1.Protos.CommandInfo.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getUrisFieldBuilder();
getEnvironmentFieldBuilder();
}
}
public Builder clear() {
super.clear();
if (urisBuilder_ == null) {
uris_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
urisBuilder_.clear();
}
if (environmentBuilder_ == null) {
environment_ = null;
} else {
environmentBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
shell_ = true;
bitField0_ = (bitField0_ & ~0x00000004);
value_ = "";
bitField0_ = (bitField0_ & ~0x00000008);
arguments_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000010);
user_ = "";
bitField0_ = (bitField0_ & ~0x00000020);
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_CommandInfo_descriptor;
}
public org.apache.mesos.v1.Protos.CommandInfo getDefaultInstanceForType() {
return org.apache.mesos.v1.Protos.CommandInfo.getDefaultInstance();
}
public org.apache.mesos.v1.Protos.CommandInfo build() {
org.apache.mesos.v1.Protos.CommandInfo result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.apache.mesos.v1.Protos.CommandInfo buildPartial() {
org.apache.mesos.v1.Protos.CommandInfo result = new org.apache.mesos.v1.Protos.CommandInfo(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (urisBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
uris_ = java.util.Collections.unmodifiableList(uris_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.uris_ = uris_;
} else {
result.uris_ = urisBuilder_.build();
}
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000001;
}
if (environmentBuilder_ == null) {
result.environment_ = environment_;
} else {
result.environment_ = environmentBuilder_.build();
}
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000002;
}
result.shell_ = shell_;
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000004;
}
result.value_ = value_;
if (((bitField0_ & 0x00000010) == 0x00000010)) {
arguments_ = arguments_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x00000010);
}
result.arguments_ = arguments_;
if (((from_bitField0_ & 0x00000020) == 0x00000020)) {
to_bitField0_ |= 0x00000008;
}
result.user_ = user_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.apache.mesos.v1.Protos.CommandInfo) {
return mergeFrom((org.apache.mesos.v1.Protos.CommandInfo)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.mesos.v1.Protos.CommandInfo other) {
if (other == org.apache.mesos.v1.Protos.CommandInfo.getDefaultInstance()) return this;
if (urisBuilder_ == null) {
if (!other.uris_.isEmpty()) {
if (uris_.isEmpty()) {
uris_ = other.uris_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureUrisIsMutable();
uris_.addAll(other.uris_);
}
onChanged();
}
} else {
if (!other.uris_.isEmpty()) {
if (urisBuilder_.isEmpty()) {
urisBuilder_.dispose();
urisBuilder_ = null;
uris_ = other.uris_;
bitField0_ = (bitField0_ & ~0x00000001);
urisBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getUrisFieldBuilder() : null;
} else {
urisBuilder_.addAllMessages(other.uris_);
}
}
}
if (other.hasEnvironment()) {
mergeEnvironment(other.getEnvironment());
}
if (other.hasShell()) {
setShell(other.getShell());
}
if (other.hasValue()) {
bitField0_ |= 0x00000008;
value_ = other.value_;
onChanged();
}
if (!other.arguments_.isEmpty()) {
if (arguments_.isEmpty()) {
arguments_ = other.arguments_;
bitField0_ = (bitField0_ & ~0x00000010);
} else {
ensureArgumentsIsMutable();
arguments_.addAll(other.arguments_);
}
onChanged();
}
if (other.hasUser()) {
bitField0_ |= 0x00000020;
user_ = other.user_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
for (int i = 0; i < getUrisCount(); i++) {
if (!getUris(i).isInitialized()) {
return false;
}
}
if (hasEnvironment()) {
if (!getEnvironment().isInitialized()) {
return false;
}
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.mesos.v1.Protos.CommandInfo parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.mesos.v1.Protos.CommandInfo) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List uris_ =
java.util.Collections.emptyList();
private void ensureUrisIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
uris_ = new java.util.ArrayList(uris_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.apache.mesos.v1.Protos.CommandInfo.URI, org.apache.mesos.v1.Protos.CommandInfo.URI.Builder, org.apache.mesos.v1.Protos.CommandInfo.URIOrBuilder> urisBuilder_;
/**
* repeated .mesos.v1.CommandInfo.URI uris = 1;
*/
public java.util.List getUrisList() {
if (urisBuilder_ == null) {
return java.util.Collections.unmodifiableList(uris_);
} else {
return urisBuilder_.getMessageList();
}
}
/**
* repeated .mesos.v1.CommandInfo.URI uris = 1;
*/
public int getUrisCount() {
if (urisBuilder_ == null) {
return uris_.size();
} else {
return urisBuilder_.getCount();
}
}
/**
* repeated .mesos.v1.CommandInfo.URI uris = 1;
*/
public org.apache.mesos.v1.Protos.CommandInfo.URI getUris(int index) {
if (urisBuilder_ == null) {
return uris_.get(index);
} else {
return urisBuilder_.getMessage(index);
}
}
/**
* repeated .mesos.v1.CommandInfo.URI uris = 1;
*/
public Builder setUris(
int index, org.apache.mesos.v1.Protos.CommandInfo.URI value) {
if (urisBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureUrisIsMutable();
uris_.set(index, value);
onChanged();
} else {
urisBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .mesos.v1.CommandInfo.URI uris = 1;
*/
public Builder setUris(
int index, org.apache.mesos.v1.Protos.CommandInfo.URI.Builder builderForValue) {
if (urisBuilder_ == null) {
ensureUrisIsMutable();
uris_.set(index, builderForValue.build());
onChanged();
} else {
urisBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .mesos.v1.CommandInfo.URI uris = 1;
*/
public Builder addUris(org.apache.mesos.v1.Protos.CommandInfo.URI value) {
if (urisBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureUrisIsMutable();
uris_.add(value);
onChanged();
} else {
urisBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .mesos.v1.CommandInfo.URI uris = 1;
*/
public Builder addUris(
int index, org.apache.mesos.v1.Protos.CommandInfo.URI value) {
if (urisBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureUrisIsMutable();
uris_.add(index, value);
onChanged();
} else {
urisBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .mesos.v1.CommandInfo.URI uris = 1;
*/
public Builder addUris(
org.apache.mesos.v1.Protos.CommandInfo.URI.Builder builderForValue) {
if (urisBuilder_ == null) {
ensureUrisIsMutable();
uris_.add(builderForValue.build());
onChanged();
} else {
urisBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .mesos.v1.CommandInfo.URI uris = 1;
*/
public Builder addUris(
int index, org.apache.mesos.v1.Protos.CommandInfo.URI.Builder builderForValue) {
if (urisBuilder_ == null) {
ensureUrisIsMutable();
uris_.add(index, builderForValue.build());
onChanged();
} else {
urisBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .mesos.v1.CommandInfo.URI uris = 1;
*/
public Builder addAllUris(
java.lang.Iterable extends org.apache.mesos.v1.Protos.CommandInfo.URI> values) {
if (urisBuilder_ == null) {
ensureUrisIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, uris_);
onChanged();
} else {
urisBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .mesos.v1.CommandInfo.URI uris = 1;
*/
public Builder clearUris() {
if (urisBuilder_ == null) {
uris_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
urisBuilder_.clear();
}
return this;
}
/**
* repeated .mesos.v1.CommandInfo.URI uris = 1;
*/
public Builder removeUris(int index) {
if (urisBuilder_ == null) {
ensureUrisIsMutable();
uris_.remove(index);
onChanged();
} else {
urisBuilder_.remove(index);
}
return this;
}
/**
* repeated .mesos.v1.CommandInfo.URI uris = 1;
*/
public org.apache.mesos.v1.Protos.CommandInfo.URI.Builder getUrisBuilder(
int index) {
return getUrisFieldBuilder().getBuilder(index);
}
/**
* repeated .mesos.v1.CommandInfo.URI uris = 1;
*/
public org.apache.mesos.v1.Protos.CommandInfo.URIOrBuilder getUrisOrBuilder(
int index) {
if (urisBuilder_ == null) {
return uris_.get(index); } else {
return urisBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .mesos.v1.CommandInfo.URI uris = 1;
*/
public java.util.List extends org.apache.mesos.v1.Protos.CommandInfo.URIOrBuilder>
getUrisOrBuilderList() {
if (urisBuilder_ != null) {
return urisBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(uris_);
}
}
/**
* repeated .mesos.v1.CommandInfo.URI uris = 1;
*/
public org.apache.mesos.v1.Protos.CommandInfo.URI.Builder addUrisBuilder() {
return getUrisFieldBuilder().addBuilder(
org.apache.mesos.v1.Protos.CommandInfo.URI.getDefaultInstance());
}
/**
* repeated .mesos.v1.CommandInfo.URI uris = 1;
*/
public org.apache.mesos.v1.Protos.CommandInfo.URI.Builder addUrisBuilder(
int index) {
return getUrisFieldBuilder().addBuilder(
index, org.apache.mesos.v1.Protos.CommandInfo.URI.getDefaultInstance());
}
/**
* repeated .mesos.v1.CommandInfo.URI uris = 1;
*/
public java.util.List
getUrisBuilderList() {
return getUrisFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.apache.mesos.v1.Protos.CommandInfo.URI, org.apache.mesos.v1.Protos.CommandInfo.URI.Builder, org.apache.mesos.v1.Protos.CommandInfo.URIOrBuilder>
getUrisFieldBuilder() {
if (urisBuilder_ == null) {
urisBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
org.apache.mesos.v1.Protos.CommandInfo.URI, org.apache.mesos.v1.Protos.CommandInfo.URI.Builder, org.apache.mesos.v1.Protos.CommandInfo.URIOrBuilder>(
uris_,
((bitField0_ & 0x00000001) == 0x00000001),
getParentForChildren(),
isClean());
uris_ = null;
}
return urisBuilder_;
}
private org.apache.mesos.v1.Protos.Environment environment_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
org.apache.mesos.v1.Protos.Environment, org.apache.mesos.v1.Protos.Environment.Builder, org.apache.mesos.v1.Protos.EnvironmentOrBuilder> environmentBuilder_;
/**
* optional .mesos.v1.Environment environment = 2;
*/
public boolean hasEnvironment() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional .mesos.v1.Environment environment = 2;
*/
public org.apache.mesos.v1.Protos.Environment getEnvironment() {
if (environmentBuilder_ == null) {
return environment_ == null ? org.apache.mesos.v1.Protos.Environment.getDefaultInstance() : environment_;
} else {
return environmentBuilder_.getMessage();
}
}
/**
* optional .mesos.v1.Environment environment = 2;
*/
public Builder setEnvironment(org.apache.mesos.v1.Protos.Environment value) {
if (environmentBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
environment_ = value;
onChanged();
} else {
environmentBuilder_.setMessage(value);
}
bitField0_ |= 0x00000002;
return this;
}
/**
* optional .mesos.v1.Environment environment = 2;
*/
public Builder setEnvironment(
org.apache.mesos.v1.Protos.Environment.Builder builderForValue) {
if (environmentBuilder_ == null) {
environment_ = builderForValue.build();
onChanged();
} else {
environmentBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000002;
return this;
}
/**
* optional .mesos.v1.Environment environment = 2;
*/
public Builder mergeEnvironment(org.apache.mesos.v1.Protos.Environment value) {
if (environmentBuilder_ == null) {
if (((bitField0_ & 0x00000002) == 0x00000002) &&
environment_ != null &&
environment_ != org.apache.mesos.v1.Protos.Environment.getDefaultInstance()) {
environment_ =
org.apache.mesos.v1.Protos.Environment.newBuilder(environment_).mergeFrom(value).buildPartial();
} else {
environment_ = value;
}
onChanged();
} else {
environmentBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000002;
return this;
}
/**
* optional .mesos.v1.Environment environment = 2;
*/
public Builder clearEnvironment() {
if (environmentBuilder_ == null) {
environment_ = null;
onChanged();
} else {
environmentBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
/**
* optional .mesos.v1.Environment environment = 2;
*/
public org.apache.mesos.v1.Protos.Environment.Builder getEnvironmentBuilder() {
bitField0_ |= 0x00000002;
onChanged();
return getEnvironmentFieldBuilder().getBuilder();
}
/**
* optional .mesos.v1.Environment environment = 2;
*/
public org.apache.mesos.v1.Protos.EnvironmentOrBuilder getEnvironmentOrBuilder() {
if (environmentBuilder_ != null) {
return environmentBuilder_.getMessageOrBuilder();
} else {
return environment_ == null ?
org.apache.mesos.v1.Protos.Environment.getDefaultInstance() : environment_;
}
}
/**
* optional .mesos.v1.Environment environment = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.apache.mesos.v1.Protos.Environment, org.apache.mesos.v1.Protos.Environment.Builder, org.apache.mesos.v1.Protos.EnvironmentOrBuilder>
getEnvironmentFieldBuilder() {
if (environmentBuilder_ == null) {
environmentBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.apache.mesos.v1.Protos.Environment, org.apache.mesos.v1.Protos.Environment.Builder, org.apache.mesos.v1.Protos.EnvironmentOrBuilder>(
getEnvironment(),
getParentForChildren(),
isClean());
environment_ = null;
}
return environmentBuilder_;
}
private boolean shell_ = true;
/**
*
* There are two ways to specify the command:
* 1) If 'shell == true', the command will be launched via shell
* (i.e., /bin/sh -c 'value'). The 'value' specified will be
* treated as the shell command. The 'arguments' will be ignored.
* 2) If 'shell == false', the command will be launched by passing
* arguments to an executable. The 'value' specified will be
* treated as the filename of the executable. The 'arguments'
* will be treated as the arguments to the executable. This is
* similar to how POSIX exec families launch processes (i.e.,
* execlp(value, arguments(0), arguments(1), ...)).
* NOTE: The field 'value' is changed from 'required' to 'optional'
* in 0.20.0. It will only cause issues if a new framework is
* connecting to an old master.
*
*
* optional bool shell = 6 [default = true];
*/
public boolean hasShell() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
*
* There are two ways to specify the command:
* 1) If 'shell == true', the command will be launched via shell
* (i.e., /bin/sh -c 'value'). The 'value' specified will be
* treated as the shell command. The 'arguments' will be ignored.
* 2) If 'shell == false', the command will be launched by passing
* arguments to an executable. The 'value' specified will be
* treated as the filename of the executable. The 'arguments'
* will be treated as the arguments to the executable. This is
* similar to how POSIX exec families launch processes (i.e.,
* execlp(value, arguments(0), arguments(1), ...)).
* NOTE: The field 'value' is changed from 'required' to 'optional'
* in 0.20.0. It will only cause issues if a new framework is
* connecting to an old master.
*
*
* optional bool shell = 6 [default = true];
*/
public boolean getShell() {
return shell_;
}
/**
*
* There are two ways to specify the command:
* 1) If 'shell == true', the command will be launched via shell
* (i.e., /bin/sh -c 'value'). The 'value' specified will be
* treated as the shell command. The 'arguments' will be ignored.
* 2) If 'shell == false', the command will be launched by passing
* arguments to an executable. The 'value' specified will be
* treated as the filename of the executable. The 'arguments'
* will be treated as the arguments to the executable. This is
* similar to how POSIX exec families launch processes (i.e.,
* execlp(value, arguments(0), arguments(1), ...)).
* NOTE: The field 'value' is changed from 'required' to 'optional'
* in 0.20.0. It will only cause issues if a new framework is
* connecting to an old master.
*
*
* optional bool shell = 6 [default = true];
*/
public Builder setShell(boolean value) {
bitField0_ |= 0x00000004;
shell_ = value;
onChanged();
return this;
}
/**
*
* There are two ways to specify the command:
* 1) If 'shell == true', the command will be launched via shell
* (i.e., /bin/sh -c 'value'). The 'value' specified will be
* treated as the shell command. The 'arguments' will be ignored.
* 2) If 'shell == false', the command will be launched by passing
* arguments to an executable. The 'value' specified will be
* treated as the filename of the executable. The 'arguments'
* will be treated as the arguments to the executable. This is
* similar to how POSIX exec families launch processes (i.e.,
* execlp(value, arguments(0), arguments(1), ...)).
* NOTE: The field 'value' is changed from 'required' to 'optional'
* in 0.20.0. It will only cause issues if a new framework is
* connecting to an old master.
*
*
* optional bool shell = 6 [default = true];
*/
public Builder clearShell() {
bitField0_ = (bitField0_ & ~0x00000004);
shell_ = true;
onChanged();
return this;
}
private java.lang.Object value_ = "";
/**
* optional string value = 3;
*/
public boolean hasValue() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional string value = 3;
*/
public java.lang.String getValue() {
java.lang.Object ref = value_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
value_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string value = 3;
*/
public com.google.protobuf.ByteString
getValueBytes() {
java.lang.Object ref = value_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
value_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string value = 3;
*/
public Builder setValue(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
value_ = value;
onChanged();
return this;
}
/**
* optional string value = 3;
*/
public Builder clearValue() {
bitField0_ = (bitField0_ & ~0x00000008);
value_ = getDefaultInstance().getValue();
onChanged();
return this;
}
/**
* optional string value = 3;
*/
public Builder setValueBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
value_ = value;
onChanged();
return this;
}
private com.google.protobuf.LazyStringList arguments_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureArgumentsIsMutable() {
if (!((bitField0_ & 0x00000010) == 0x00000010)) {
arguments_ = new com.google.protobuf.LazyStringArrayList(arguments_);
bitField0_ |= 0x00000010;
}
}
/**
* repeated string arguments = 7;
*/
public com.google.protobuf.ProtocolStringList
getArgumentsList() {
return arguments_.getUnmodifiableView();
}
/**
* repeated string arguments = 7;
*/
public int getArgumentsCount() {
return arguments_.size();
}
/**
* repeated string arguments = 7;
*/
public java.lang.String getArguments(int index) {
return arguments_.get(index);
}
/**
* repeated string arguments = 7;
*/
public com.google.protobuf.ByteString
getArgumentsBytes(int index) {
return arguments_.getByteString(index);
}
/**
* repeated string arguments = 7;
*/
public Builder setArguments(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureArgumentsIsMutable();
arguments_.set(index, value);
onChanged();
return this;
}
/**
* repeated string arguments = 7;
*/
public Builder addArguments(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureArgumentsIsMutable();
arguments_.add(value);
onChanged();
return this;
}
/**
* repeated string arguments = 7;
*/
public Builder addAllArguments(
java.lang.Iterable values) {
ensureArgumentsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, arguments_);
onChanged();
return this;
}
/**
* repeated string arguments = 7;
*/
public Builder clearArguments() {
arguments_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000010);
onChanged();
return this;
}
/**
* repeated string arguments = 7;
*/
public Builder addArgumentsBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureArgumentsIsMutable();
arguments_.add(value);
onChanged();
return this;
}
private java.lang.Object user_ = "";
/**
*
* Enables executor and tasks to run as a specific user. If the user
* field is present both in FrameworkInfo and here, the CommandInfo
* user value takes precedence.
*
*
* optional string user = 5;
*/
public boolean hasUser() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
*
* Enables executor and tasks to run as a specific user. If the user
* field is present both in FrameworkInfo and here, the CommandInfo
* user value takes precedence.
*
*
* optional string user = 5;
*/
public java.lang.String getUser() {
java.lang.Object ref = user_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
user_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Enables executor and tasks to run as a specific user. If the user
* field is present both in FrameworkInfo and here, the CommandInfo
* user value takes precedence.
*
*
* optional string user = 5;
*/
public com.google.protobuf.ByteString
getUserBytes() {
java.lang.Object ref = user_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
user_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Enables executor and tasks to run as a specific user. If the user
* field is present both in FrameworkInfo and here, the CommandInfo
* user value takes precedence.
*
*
* optional string user = 5;
*/
public Builder setUser(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000020;
user_ = value;
onChanged();
return this;
}
/**
*
* Enables executor and tasks to run as a specific user. If the user
* field is present both in FrameworkInfo and here, the CommandInfo
* user value takes precedence.
*
*
* optional string user = 5;
*/
public Builder clearUser() {
bitField0_ = (bitField0_ & ~0x00000020);
user_ = getDefaultInstance().getUser();
onChanged();
return this;
}
/**
*
* Enables executor and tasks to run as a specific user. If the user
* field is present both in FrameworkInfo and here, the CommandInfo
* user value takes precedence.
*
*
* optional string user = 5;
*/
public Builder setUserBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000020;
user_ = value;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:mesos.v1.CommandInfo)
}
// @@protoc_insertion_point(class_scope:mesos.v1.CommandInfo)
private static final org.apache.mesos.v1.Protos.CommandInfo DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.apache.mesos.v1.Protos.CommandInfo();
}
public static org.apache.mesos.v1.Protos.CommandInfo getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public CommandInfo parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new CommandInfo(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.apache.mesos.v1.Protos.CommandInfo getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ExecutorInfoOrBuilder extends
// @@protoc_insertion_point(interface_extends:mesos.v1.ExecutorInfo)
com.google.protobuf.MessageOrBuilder {
/**
*
* For backwards compatibility, if this field is not set when using `LAUNCH`
* operation, Mesos will infer the type by checking if `command` is set
* (`CUSTOM`) or unset (`DEFAULT`). `type` must be set when using
* `LAUNCH_GROUP` operation.
* TODO(vinod): Add support for explicitly setting `type` to `DEFAULT` in
* `LAUNCH` operation.
*
*
* optional .mesos.v1.ExecutorInfo.Type type = 15;
*/
boolean hasType();
/**
*
* For backwards compatibility, if this field is not set when using `LAUNCH`
* operation, Mesos will infer the type by checking if `command` is set
* (`CUSTOM`) or unset (`DEFAULT`). `type` must be set when using
* `LAUNCH_GROUP` operation.
* TODO(vinod): Add support for explicitly setting `type` to `DEFAULT` in
* `LAUNCH` operation.
*
*
* optional .mesos.v1.ExecutorInfo.Type type = 15;
*/
org.apache.mesos.v1.Protos.ExecutorInfo.Type getType();
/**
* required .mesos.v1.ExecutorID executor_id = 1;
*/
boolean hasExecutorId();
/**
* required .mesos.v1.ExecutorID executor_id = 1;
*/
org.apache.mesos.v1.Protos.ExecutorID getExecutorId();
/**
* required .mesos.v1.ExecutorID executor_id = 1;
*/
org.apache.mesos.v1.Protos.ExecutorIDOrBuilder getExecutorIdOrBuilder();
/**
*
* TODO(benh): Make this required.
*
*
* optional .mesos.v1.FrameworkID framework_id = 8;
*/
boolean hasFrameworkId();
/**
*
* TODO(benh): Make this required.
*
*
* optional .mesos.v1.FrameworkID framework_id = 8;
*/
org.apache.mesos.v1.Protos.FrameworkID getFrameworkId();
/**
*
* TODO(benh): Make this required.
*
*
* optional .mesos.v1.FrameworkID framework_id = 8;
*/
org.apache.mesos.v1.Protos.FrameworkIDOrBuilder getFrameworkIdOrBuilder();
/**
* optional .mesos.v1.CommandInfo command = 7;
*/
boolean hasCommand();
/**
* optional .mesos.v1.CommandInfo command = 7;
*/
org.apache.mesos.v1.Protos.CommandInfo getCommand();
/**
* optional .mesos.v1.CommandInfo command = 7;
*/
org.apache.mesos.v1.Protos.CommandInfoOrBuilder getCommandOrBuilder();
/**
*
* Executor provided with a container will launch the container
* with the executor's CommandInfo and we expect the container to
* act as a Mesos executor.
*
*
* optional .mesos.v1.ContainerInfo container = 11;
*/
boolean hasContainer();
/**
*
* Executor provided with a container will launch the container
* with the executor's CommandInfo and we expect the container to
* act as a Mesos executor.
*
*
* optional .mesos.v1.ContainerInfo container = 11;
*/
org.apache.mesos.v1.Protos.ContainerInfo getContainer();
/**
*
* Executor provided with a container will launch the container
* with the executor's CommandInfo and we expect the container to
* act as a Mesos executor.
*
*
* optional .mesos.v1.ContainerInfo container = 11;
*/
org.apache.mesos.v1.Protos.ContainerInfoOrBuilder getContainerOrBuilder();
/**
* repeated .mesos.v1.Resource resources = 5;
*/
java.util.List
getResourcesList();
/**
* repeated .mesos.v1.Resource resources = 5;
*/
org.apache.mesos.v1.Protos.Resource getResources(int index);
/**
* repeated .mesos.v1.Resource resources = 5;
*/
int getResourcesCount();
/**
* repeated .mesos.v1.Resource resources = 5;
*/
java.util.List extends org.apache.mesos.v1.Protos.ResourceOrBuilder>
getResourcesOrBuilderList();
/**
* repeated .mesos.v1.Resource resources = 5;
*/
org.apache.mesos.v1.Protos.ResourceOrBuilder getResourcesOrBuilder(
int index);
/**
* optional string name = 9;
*/
boolean hasName();
/**
* optional string name = 9;
*/
java.lang.String getName();
/**
* optional string name = 9;
*/
com.google.protobuf.ByteString
getNameBytes();
/**
*
* 'source' is an identifier style string used by frameworks to
* track the source of an executor. This is useful when it's
* possible for different executor ids to be related semantically.
* NOTE: 'source' is exposed alongside the resource usage of the
* executor via JSON on the agent. This allows users to import usage
* information into a time series database for monitoring.
* This field is deprecated since 1.0. Please use labels for
* free-form metadata instead.
*
*
* optional string source = 10 [deprecated = true];
*/
@java.lang.Deprecated boolean hasSource();
/**
*
* 'source' is an identifier style string used by frameworks to
* track the source of an executor. This is useful when it's
* possible for different executor ids to be related semantically.
* NOTE: 'source' is exposed alongside the resource usage of the
* executor via JSON on the agent. This allows users to import usage
* information into a time series database for monitoring.
* This field is deprecated since 1.0. Please use labels for
* free-form metadata instead.
*
*
* optional string source = 10 [deprecated = true];
*/
@java.lang.Deprecated java.lang.String getSource();
/**
*
* 'source' is an identifier style string used by frameworks to
* track the source of an executor. This is useful when it's
* possible for different executor ids to be related semantically.
* NOTE: 'source' is exposed alongside the resource usage of the
* executor via JSON on the agent. This allows users to import usage
* information into a time series database for monitoring.
* This field is deprecated since 1.0. Please use labels for
* free-form metadata instead.
*
*
* optional string source = 10 [deprecated = true];
*/
@java.lang.Deprecated com.google.protobuf.ByteString
getSourceBytes();
/**
*
* This field can be used to pass arbitrary bytes to an executor.
*
*
* optional bytes data = 4;
*/
boolean hasData();
/**
*
* This field can be used to pass arbitrary bytes to an executor.
*
*
* optional bytes data = 4;
*/
com.google.protobuf.ByteString getData();
/**
*
* Service discovery information for the executor. It is not
* interpreted or acted upon by Mesos. It is up to a service
* discovery system to use this information as needed and to handle
* executors without service discovery information.
*
*
* optional .mesos.v1.DiscoveryInfo discovery = 12;
*/
boolean hasDiscovery();
/**
*
* Service discovery information for the executor. It is not
* interpreted or acted upon by Mesos. It is up to a service
* discovery system to use this information as needed and to handle
* executors without service discovery information.
*
*
* optional .mesos.v1.DiscoveryInfo discovery = 12;
*/
org.apache.mesos.v1.Protos.DiscoveryInfo getDiscovery();
/**
*
* Service discovery information for the executor. It is not
* interpreted or acted upon by Mesos. It is up to a service
* discovery system to use this information as needed and to handle
* executors without service discovery information.
*
*
* optional .mesos.v1.DiscoveryInfo discovery = 12;
*/
org.apache.mesos.v1.Protos.DiscoveryInfoOrBuilder getDiscoveryOrBuilder();
/**
*
* When shutting down an executor the agent will wait in a
* best-effort manner for the grace period specified here
* before forcibly destroying the container. The executor
* must not assume that it will always be allotted the full
* grace period, as the agent may decide to allot a shorter
* period and failures / forcible terminations may occur.
*
*
* optional .mesos.v1.DurationInfo shutdown_grace_period = 13;
*/
boolean hasShutdownGracePeriod();
/**
*
* When shutting down an executor the agent will wait in a
* best-effort manner for the grace period specified here
* before forcibly destroying the container. The executor
* must not assume that it will always be allotted the full
* grace period, as the agent may decide to allot a shorter
* period and failures / forcible terminations may occur.
*
*
* optional .mesos.v1.DurationInfo shutdown_grace_period = 13;
*/
org.apache.mesos.v1.Protos.DurationInfo getShutdownGracePeriod();
/**
*
* When shutting down an executor the agent will wait in a
* best-effort manner for the grace period specified here
* before forcibly destroying the container. The executor
* must not assume that it will always be allotted the full
* grace period, as the agent may decide to allot a shorter
* period and failures / forcible terminations may occur.
*
*
* optional .mesos.v1.DurationInfo shutdown_grace_period = 13;
*/
org.apache.mesos.v1.Protos.DurationInfoOrBuilder getShutdownGracePeriodOrBuilder();
/**
*
* Labels are free-form key value pairs which are exposed through
* master and agent endpoints. Labels will not be interpreted or
* acted upon by Mesos itself. As opposed to the data field, labels
* will be kept in memory on master and agent processes. Therefore,
* labels should be used to tag executors with lightweight metadata.
* Labels should not contain duplicate key-value pairs.
*
*
* optional .mesos.v1.Labels labels = 14;
*/
boolean hasLabels();
/**
*
* Labels are free-form key value pairs which are exposed through
* master and agent endpoints. Labels will not be interpreted or
* acted upon by Mesos itself. As opposed to the data field, labels
* will be kept in memory on master and agent processes. Therefore,
* labels should be used to tag executors with lightweight metadata.
* Labels should not contain duplicate key-value pairs.
*
*
* optional .mesos.v1.Labels labels = 14;
*/
org.apache.mesos.v1.Protos.Labels getLabels();
/**
*
* Labels are free-form key value pairs which are exposed through
* master and agent endpoints. Labels will not be interpreted or
* acted upon by Mesos itself. As opposed to the data field, labels
* will be kept in memory on master and agent processes. Therefore,
* labels should be used to tag executors with lightweight metadata.
* Labels should not contain duplicate key-value pairs.
*
*
* optional .mesos.v1.Labels labels = 14;
*/
org.apache.mesos.v1.Protos.LabelsOrBuilder getLabelsOrBuilder();
}
/**
*
**
* Describes information about an executor.
*
*
* Protobuf type {@code mesos.v1.ExecutorInfo}
*/
public static final class ExecutorInfo extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:mesos.v1.ExecutorInfo)
ExecutorInfoOrBuilder {
private static final long serialVersionUID = 0L;
// Use ExecutorInfo.newBuilder() to construct.
private ExecutorInfo(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ExecutorInfo() {
type_ = 0;
resources_ = java.util.Collections.emptyList();
name_ = "";
source_ = "";
data_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ExecutorInfo(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
org.apache.mesos.v1.Protos.ExecutorID.Builder subBuilder = null;
if (((bitField0_ & 0x00000002) == 0x00000002)) {
subBuilder = executorId_.toBuilder();
}
executorId_ = input.readMessage(org.apache.mesos.v1.Protos.ExecutorID.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(executorId_);
executorId_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000002;
break;
}
case 34: {
bitField0_ |= 0x00000080;
data_ = input.readBytes();
break;
}
case 42: {
if (!((mutable_bitField0_ & 0x00000020) == 0x00000020)) {
resources_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000020;
}
resources_.add(
input.readMessage(org.apache.mesos.v1.Protos.Resource.PARSER, extensionRegistry));
break;
}
case 58: {
org.apache.mesos.v1.Protos.CommandInfo.Builder subBuilder = null;
if (((bitField0_ & 0x00000008) == 0x00000008)) {
subBuilder = command_.toBuilder();
}
command_ = input.readMessage(org.apache.mesos.v1.Protos.CommandInfo.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(command_);
command_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000008;
break;
}
case 66: {
org.apache.mesos.v1.Protos.FrameworkID.Builder subBuilder = null;
if (((bitField0_ & 0x00000004) == 0x00000004)) {
subBuilder = frameworkId_.toBuilder();
}
frameworkId_ = input.readMessage(org.apache.mesos.v1.Protos.FrameworkID.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(frameworkId_);
frameworkId_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000004;
break;
}
case 74: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000020;
name_ = bs;
break;
}
case 82: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000040;
source_ = bs;
break;
}
case 90: {
org.apache.mesos.v1.Protos.ContainerInfo.Builder subBuilder = null;
if (((bitField0_ & 0x00000010) == 0x00000010)) {
subBuilder = container_.toBuilder();
}
container_ = input.readMessage(org.apache.mesos.v1.Protos.ContainerInfo.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(container_);
container_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000010;
break;
}
case 98: {
org.apache.mesos.v1.Protos.DiscoveryInfo.Builder subBuilder = null;
if (((bitField0_ & 0x00000100) == 0x00000100)) {
subBuilder = discovery_.toBuilder();
}
discovery_ = input.readMessage(org.apache.mesos.v1.Protos.DiscoveryInfo.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(discovery_);
discovery_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000100;
break;
}
case 106: {
org.apache.mesos.v1.Protos.DurationInfo.Builder subBuilder = null;
if (((bitField0_ & 0x00000200) == 0x00000200)) {
subBuilder = shutdownGracePeriod_.toBuilder();
}
shutdownGracePeriod_ = input.readMessage(org.apache.mesos.v1.Protos.DurationInfo.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(shutdownGracePeriod_);
shutdownGracePeriod_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000200;
break;
}
case 114: {
org.apache.mesos.v1.Protos.Labels.Builder subBuilder = null;
if (((bitField0_ & 0x00000400) == 0x00000400)) {
subBuilder = labels_.toBuilder();
}
labels_ = input.readMessage(org.apache.mesos.v1.Protos.Labels.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(labels_);
labels_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000400;
break;
}
case 120: {
int rawValue = input.readEnum();
org.apache.mesos.v1.Protos.ExecutorInfo.Type value = org.apache.mesos.v1.Protos.ExecutorInfo.Type.valueOf(rawValue);
if (value == null) {
unknownFields.mergeVarintField(15, rawValue);
} else {
bitField0_ |= 0x00000001;
type_ = rawValue;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000020) == 0x00000020)) {
resources_ = java.util.Collections.unmodifiableList(resources_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_ExecutorInfo_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_ExecutorInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.mesos.v1.Protos.ExecutorInfo.class, org.apache.mesos.v1.Protos.ExecutorInfo.Builder.class);
}
/**
* Protobuf enum {@code mesos.v1.ExecutorInfo.Type}
*/
public enum Type
implements com.google.protobuf.ProtocolMessageEnum {
/**
* UNKNOWN = 0;
*/
UNKNOWN(0),
/**
*
* Mesos provides a simple built-in default executor that frameworks can
* leverage to run shell commands and containers.
* NOTES:
* 1) `command` must not be set when using a default executor.
* 2) Default executor only accepts a *single* `LAUNCH` or `LAUNCH_GROUP`
* operation.
* 3) If `container` is set, `container.type` must be `MESOS`
* and `container.mesos.image` must not be set.
*
*
* DEFAULT = 1;
*/
DEFAULT(1),
/**
*
* For frameworks that need custom functionality to run tasks, a `CUSTOM`
* executor can be used. Note that `command` must be set when using a
* `CUSTOM` executor.
*
*
* CUSTOM = 2;
*/
CUSTOM(2),
;
/**
* UNKNOWN = 0;
*/
public static final int UNKNOWN_VALUE = 0;
/**
*
* Mesos provides a simple built-in default executor that frameworks can
* leverage to run shell commands and containers.
* NOTES:
* 1) `command` must not be set when using a default executor.
* 2) Default executor only accepts a *single* `LAUNCH` or `LAUNCH_GROUP`
* operation.
* 3) If `container` is set, `container.type` must be `MESOS`
* and `container.mesos.image` must not be set.
*
*
* DEFAULT = 1;
*/
public static final int DEFAULT_VALUE = 1;
/**
*
* For frameworks that need custom functionality to run tasks, a `CUSTOM`
* executor can be used. Note that `command` must be set when using a
* `CUSTOM` executor.
*
*
* CUSTOM = 2;
*/
public static final int CUSTOM_VALUE = 2;
public final int getNumber() {
return value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static Type valueOf(int value) {
return forNumber(value);
}
public static Type forNumber(int value) {
switch (value) {
case 0: return UNKNOWN;
case 1: return DEFAULT;
case 2: return CUSTOM;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
Type> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public Type findValueByNumber(int number) {
return Type.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return org.apache.mesos.v1.Protos.ExecutorInfo.getDescriptor().getEnumTypes().get(0);
}
private static final Type[] VALUES = values();
public static Type valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int value;
private Type(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:mesos.v1.ExecutorInfo.Type)
}
private int bitField0_;
public static final int TYPE_FIELD_NUMBER = 15;
private int type_;
/**
*
* For backwards compatibility, if this field is not set when using `LAUNCH`
* operation, Mesos will infer the type by checking if `command` is set
* (`CUSTOM`) or unset (`DEFAULT`). `type` must be set when using
* `LAUNCH_GROUP` operation.
* TODO(vinod): Add support for explicitly setting `type` to `DEFAULT` in
* `LAUNCH` operation.
*
*
* optional .mesos.v1.ExecutorInfo.Type type = 15;
*/
public boolean hasType() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
*
* For backwards compatibility, if this field is not set when using `LAUNCH`
* operation, Mesos will infer the type by checking if `command` is set
* (`CUSTOM`) or unset (`DEFAULT`). `type` must be set when using
* `LAUNCH_GROUP` operation.
* TODO(vinod): Add support for explicitly setting `type` to `DEFAULT` in
* `LAUNCH` operation.
*
*
* optional .mesos.v1.ExecutorInfo.Type type = 15;
*/
public org.apache.mesos.v1.Protos.ExecutorInfo.Type getType() {
org.apache.mesos.v1.Protos.ExecutorInfo.Type result = org.apache.mesos.v1.Protos.ExecutorInfo.Type.valueOf(type_);
return result == null ? org.apache.mesos.v1.Protos.ExecutorInfo.Type.UNKNOWN : result;
}
public static final int EXECUTOR_ID_FIELD_NUMBER = 1;
private org.apache.mesos.v1.Protos.ExecutorID executorId_;
/**
* required .mesos.v1.ExecutorID executor_id = 1;
*/
public boolean hasExecutorId() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required .mesos.v1.ExecutorID executor_id = 1;
*/
public org.apache.mesos.v1.Protos.ExecutorID getExecutorId() {
return executorId_ == null ? org.apache.mesos.v1.Protos.ExecutorID.getDefaultInstance() : executorId_;
}
/**
* required .mesos.v1.ExecutorID executor_id = 1;
*/
public org.apache.mesos.v1.Protos.ExecutorIDOrBuilder getExecutorIdOrBuilder() {
return executorId_ == null ? org.apache.mesos.v1.Protos.ExecutorID.getDefaultInstance() : executorId_;
}
public static final int FRAMEWORK_ID_FIELD_NUMBER = 8;
private org.apache.mesos.v1.Protos.FrameworkID frameworkId_;
/**
*
* TODO(benh): Make this required.
*
*
* optional .mesos.v1.FrameworkID framework_id = 8;
*/
public boolean hasFrameworkId() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
*
* TODO(benh): Make this required.
*
*
* optional .mesos.v1.FrameworkID framework_id = 8;
*/
public org.apache.mesos.v1.Protos.FrameworkID getFrameworkId() {
return frameworkId_ == null ? org.apache.mesos.v1.Protos.FrameworkID.getDefaultInstance() : frameworkId_;
}
/**
*
* TODO(benh): Make this required.
*
*
* optional .mesos.v1.FrameworkID framework_id = 8;
*/
public org.apache.mesos.v1.Protos.FrameworkIDOrBuilder getFrameworkIdOrBuilder() {
return frameworkId_ == null ? org.apache.mesos.v1.Protos.FrameworkID.getDefaultInstance() : frameworkId_;
}
public static final int COMMAND_FIELD_NUMBER = 7;
private org.apache.mesos.v1.Protos.CommandInfo command_;
/**
* optional .mesos.v1.CommandInfo command = 7;
*/
public boolean hasCommand() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional .mesos.v1.CommandInfo command = 7;
*/
public org.apache.mesos.v1.Protos.CommandInfo getCommand() {
return command_ == null ? org.apache.mesos.v1.Protos.CommandInfo.getDefaultInstance() : command_;
}
/**
* optional .mesos.v1.CommandInfo command = 7;
*/
public org.apache.mesos.v1.Protos.CommandInfoOrBuilder getCommandOrBuilder() {
return command_ == null ? org.apache.mesos.v1.Protos.CommandInfo.getDefaultInstance() : command_;
}
public static final int CONTAINER_FIELD_NUMBER = 11;
private org.apache.mesos.v1.Protos.ContainerInfo container_;
/**
*
* Executor provided with a container will launch the container
* with the executor's CommandInfo and we expect the container to
* act as a Mesos executor.
*
*
* optional .mesos.v1.ContainerInfo container = 11;
*/
public boolean hasContainer() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
*
* Executor provided with a container will launch the container
* with the executor's CommandInfo and we expect the container to
* act as a Mesos executor.
*
*
* optional .mesos.v1.ContainerInfo container = 11;
*/
public org.apache.mesos.v1.Protos.ContainerInfo getContainer() {
return container_ == null ? org.apache.mesos.v1.Protos.ContainerInfo.getDefaultInstance() : container_;
}
/**
*
* Executor provided with a container will launch the container
* with the executor's CommandInfo and we expect the container to
* act as a Mesos executor.
*
*
* optional .mesos.v1.ContainerInfo container = 11;
*/
public org.apache.mesos.v1.Protos.ContainerInfoOrBuilder getContainerOrBuilder() {
return container_ == null ? org.apache.mesos.v1.Protos.ContainerInfo.getDefaultInstance() : container_;
}
public static final int RESOURCES_FIELD_NUMBER = 5;
private java.util.List resources_;
/**
* repeated .mesos.v1.Resource resources = 5;
*/
public java.util.List getResourcesList() {
return resources_;
}
/**
* repeated .mesos.v1.Resource resources = 5;
*/
public java.util.List extends org.apache.mesos.v1.Protos.ResourceOrBuilder>
getResourcesOrBuilderList() {
return resources_;
}
/**
* repeated .mesos.v1.Resource resources = 5;
*/
public int getResourcesCount() {
return resources_.size();
}
/**
* repeated .mesos.v1.Resource resources = 5;
*/
public org.apache.mesos.v1.Protos.Resource getResources(int index) {
return resources_.get(index);
}
/**
* repeated .mesos.v1.Resource resources = 5;
*/
public org.apache.mesos.v1.Protos.ResourceOrBuilder getResourcesOrBuilder(
int index) {
return resources_.get(index);
}
public static final int NAME_FIELD_NUMBER = 9;
private volatile java.lang.Object name_;
/**
* optional string name = 9;
*/
public boolean hasName() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* optional string name = 9;
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
name_ = s;
}
return s;
}
}
/**
* optional string name = 9;
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SOURCE_FIELD_NUMBER = 10;
private volatile java.lang.Object source_;
/**
*
* 'source' is an identifier style string used by frameworks to
* track the source of an executor. This is useful when it's
* possible for different executor ids to be related semantically.
* NOTE: 'source' is exposed alongside the resource usage of the
* executor via JSON on the agent. This allows users to import usage
* information into a time series database for monitoring.
* This field is deprecated since 1.0. Please use labels for
* free-form metadata instead.
*
*
* optional string source = 10 [deprecated = true];
*/
@java.lang.Deprecated public boolean hasSource() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
*
* 'source' is an identifier style string used by frameworks to
* track the source of an executor. This is useful when it's
* possible for different executor ids to be related semantically.
* NOTE: 'source' is exposed alongside the resource usage of the
* executor via JSON on the agent. This allows users to import usage
* information into a time series database for monitoring.
* This field is deprecated since 1.0. Please use labels for
* free-form metadata instead.
*
*
* optional string source = 10 [deprecated = true];
*/
@java.lang.Deprecated public java.lang.String getSource() {
java.lang.Object ref = source_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
source_ = s;
}
return s;
}
}
/**
*
* 'source' is an identifier style string used by frameworks to
* track the source of an executor. This is useful when it's
* possible for different executor ids to be related semantically.
* NOTE: 'source' is exposed alongside the resource usage of the
* executor via JSON on the agent. This allows users to import usage
* information into a time series database for monitoring.
* This field is deprecated since 1.0. Please use labels for
* free-form metadata instead.
*
*
* optional string source = 10 [deprecated = true];
*/
@java.lang.Deprecated public com.google.protobuf.ByteString
getSourceBytes() {
java.lang.Object ref = source_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
source_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int DATA_FIELD_NUMBER = 4;
private com.google.protobuf.ByteString data_;
/**
*
* This field can be used to pass arbitrary bytes to an executor.
*
*
* optional bytes data = 4;
*/
public boolean hasData() {
return ((bitField0_ & 0x00000080) == 0x00000080);
}
/**
*
* This field can be used to pass arbitrary bytes to an executor.
*
*
* optional bytes data = 4;
*/
public com.google.protobuf.ByteString getData() {
return data_;
}
public static final int DISCOVERY_FIELD_NUMBER = 12;
private org.apache.mesos.v1.Protos.DiscoveryInfo discovery_;
/**
*
* Service discovery information for the executor. It is not
* interpreted or acted upon by Mesos. It is up to a service
* discovery system to use this information as needed and to handle
* executors without service discovery information.
*
*
* optional .mesos.v1.DiscoveryInfo discovery = 12;
*/
public boolean hasDiscovery() {
return ((bitField0_ & 0x00000100) == 0x00000100);
}
/**
*
* Service discovery information for the executor. It is not
* interpreted or acted upon by Mesos. It is up to a service
* discovery system to use this information as needed and to handle
* executors without service discovery information.
*
*
* optional .mesos.v1.DiscoveryInfo discovery = 12;
*/
public org.apache.mesos.v1.Protos.DiscoveryInfo getDiscovery() {
return discovery_ == null ? org.apache.mesos.v1.Protos.DiscoveryInfo.getDefaultInstance() : discovery_;
}
/**
*
* Service discovery information for the executor. It is not
* interpreted or acted upon by Mesos. It is up to a service
* discovery system to use this information as needed and to handle
* executors without service discovery information.
*
*
* optional .mesos.v1.DiscoveryInfo discovery = 12;
*/
public org.apache.mesos.v1.Protos.DiscoveryInfoOrBuilder getDiscoveryOrBuilder() {
return discovery_ == null ? org.apache.mesos.v1.Protos.DiscoveryInfo.getDefaultInstance() : discovery_;
}
public static final int SHUTDOWN_GRACE_PERIOD_FIELD_NUMBER = 13;
private org.apache.mesos.v1.Protos.DurationInfo shutdownGracePeriod_;
/**
*
* When shutting down an executor the agent will wait in a
* best-effort manner for the grace period specified here
* before forcibly destroying the container. The executor
* must not assume that it will always be allotted the full
* grace period, as the agent may decide to allot a shorter
* period and failures / forcible terminations may occur.
*
*
* optional .mesos.v1.DurationInfo shutdown_grace_period = 13;
*/
public boolean hasShutdownGracePeriod() {
return ((bitField0_ & 0x00000200) == 0x00000200);
}
/**
*
* When shutting down an executor the agent will wait in a
* best-effort manner for the grace period specified here
* before forcibly destroying the container. The executor
* must not assume that it will always be allotted the full
* grace period, as the agent may decide to allot a shorter
* period and failures / forcible terminations may occur.
*
*
* optional .mesos.v1.DurationInfo shutdown_grace_period = 13;
*/
public org.apache.mesos.v1.Protos.DurationInfo getShutdownGracePeriod() {
return shutdownGracePeriod_ == null ? org.apache.mesos.v1.Protos.DurationInfo.getDefaultInstance() : shutdownGracePeriod_;
}
/**
*
* When shutting down an executor the agent will wait in a
* best-effort manner for the grace period specified here
* before forcibly destroying the container. The executor
* must not assume that it will always be allotted the full
* grace period, as the agent may decide to allot a shorter
* period and failures / forcible terminations may occur.
*
*
* optional .mesos.v1.DurationInfo shutdown_grace_period = 13;
*/
public org.apache.mesos.v1.Protos.DurationInfoOrBuilder getShutdownGracePeriodOrBuilder() {
return shutdownGracePeriod_ == null ? org.apache.mesos.v1.Protos.DurationInfo.getDefaultInstance() : shutdownGracePeriod_;
}
public static final int LABELS_FIELD_NUMBER = 14;
private org.apache.mesos.v1.Protos.Labels labels_;
/**
*
* Labels are free-form key value pairs which are exposed through
* master and agent endpoints. Labels will not be interpreted or
* acted upon by Mesos itself. As opposed to the data field, labels
* will be kept in memory on master and agent processes. Therefore,
* labels should be used to tag executors with lightweight metadata.
* Labels should not contain duplicate key-value pairs.
*
*
* optional .mesos.v1.Labels labels = 14;
*/
public boolean hasLabels() {
return ((bitField0_ & 0x00000400) == 0x00000400);
}
/**
*
* Labels are free-form key value pairs which are exposed through
* master and agent endpoints. Labels will not be interpreted or
* acted upon by Mesos itself. As opposed to the data field, labels
* will be kept in memory on master and agent processes. Therefore,
* labels should be used to tag executors with lightweight metadata.
* Labels should not contain duplicate key-value pairs.
*
*
* optional .mesos.v1.Labels labels = 14;
*/
public org.apache.mesos.v1.Protos.Labels getLabels() {
return labels_ == null ? org.apache.mesos.v1.Protos.Labels.getDefaultInstance() : labels_;
}
/**
*
* Labels are free-form key value pairs which are exposed through
* master and agent endpoints. Labels will not be interpreted or
* acted upon by Mesos itself. As opposed to the data field, labels
* will be kept in memory on master and agent processes. Therefore,
* labels should be used to tag executors with lightweight metadata.
* Labels should not contain duplicate key-value pairs.
*
*
* optional .mesos.v1.Labels labels = 14;
*/
public org.apache.mesos.v1.Protos.LabelsOrBuilder getLabelsOrBuilder() {
return labels_ == null ? org.apache.mesos.v1.Protos.Labels.getDefaultInstance() : labels_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasExecutorId()) {
memoizedIsInitialized = 0;
return false;
}
if (!getExecutorId().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
if (hasFrameworkId()) {
if (!getFrameworkId().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
if (hasCommand()) {
if (!getCommand().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
if (hasContainer()) {
if (!getContainer().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
for (int i = 0; i < getResourcesCount(); i++) {
if (!getResources(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
if (hasDiscovery()) {
if (!getDiscovery().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
if (hasShutdownGracePeriod()) {
if (!getShutdownGracePeriod().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
if (hasLabels()) {
if (!getLabels().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeMessage(1, getExecutorId());
}
if (((bitField0_ & 0x00000080) == 0x00000080)) {
output.writeBytes(4, data_);
}
for (int i = 0; i < resources_.size(); i++) {
output.writeMessage(5, resources_.get(i));
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeMessage(7, getCommand());
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeMessage(8, getFrameworkId());
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 9, name_);
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 10, source_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
output.writeMessage(11, getContainer());
}
if (((bitField0_ & 0x00000100) == 0x00000100)) {
output.writeMessage(12, getDiscovery());
}
if (((bitField0_ & 0x00000200) == 0x00000200)) {
output.writeMessage(13, getShutdownGracePeriod());
}
if (((bitField0_ & 0x00000400) == 0x00000400)) {
output.writeMessage(14, getLabels());
}
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeEnum(15, type_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getExecutorId());
}
if (((bitField0_ & 0x00000080) == 0x00000080)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(4, data_);
}
for (int i = 0; i < resources_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, resources_.get(i));
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(7, getCommand());
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(8, getFrameworkId());
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(9, name_);
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(10, source_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(11, getContainer());
}
if (((bitField0_ & 0x00000100) == 0x00000100)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(12, getDiscovery());
}
if (((bitField0_ & 0x00000200) == 0x00000200)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(13, getShutdownGracePeriod());
}
if (((bitField0_ & 0x00000400) == 0x00000400)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(14, getLabels());
}
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(15, type_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.mesos.v1.Protos.ExecutorInfo)) {
return super.equals(obj);
}
org.apache.mesos.v1.Protos.ExecutorInfo other = (org.apache.mesos.v1.Protos.ExecutorInfo) obj;
boolean result = true;
result = result && (hasType() == other.hasType());
if (hasType()) {
result = result && type_ == other.type_;
}
result = result && (hasExecutorId() == other.hasExecutorId());
if (hasExecutorId()) {
result = result && getExecutorId()
.equals(other.getExecutorId());
}
result = result && (hasFrameworkId() == other.hasFrameworkId());
if (hasFrameworkId()) {
result = result && getFrameworkId()
.equals(other.getFrameworkId());
}
result = result && (hasCommand() == other.hasCommand());
if (hasCommand()) {
result = result && getCommand()
.equals(other.getCommand());
}
result = result && (hasContainer() == other.hasContainer());
if (hasContainer()) {
result = result && getContainer()
.equals(other.getContainer());
}
result = result && getResourcesList()
.equals(other.getResourcesList());
result = result && (hasName() == other.hasName());
if (hasName()) {
result = result && getName()
.equals(other.getName());
}
result = result && (hasSource() == other.hasSource());
if (hasSource()) {
result = result && getSource()
.equals(other.getSource());
}
result = result && (hasData() == other.hasData());
if (hasData()) {
result = result && getData()
.equals(other.getData());
}
result = result && (hasDiscovery() == other.hasDiscovery());
if (hasDiscovery()) {
result = result && getDiscovery()
.equals(other.getDiscovery());
}
result = result && (hasShutdownGracePeriod() == other.hasShutdownGracePeriod());
if (hasShutdownGracePeriod()) {
result = result && getShutdownGracePeriod()
.equals(other.getShutdownGracePeriod());
}
result = result && (hasLabels() == other.hasLabels());
if (hasLabels()) {
result = result && getLabels()
.equals(other.getLabels());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasType()) {
hash = (37 * hash) + TYPE_FIELD_NUMBER;
hash = (53 * hash) + type_;
}
if (hasExecutorId()) {
hash = (37 * hash) + EXECUTOR_ID_FIELD_NUMBER;
hash = (53 * hash) + getExecutorId().hashCode();
}
if (hasFrameworkId()) {
hash = (37 * hash) + FRAMEWORK_ID_FIELD_NUMBER;
hash = (53 * hash) + getFrameworkId().hashCode();
}
if (hasCommand()) {
hash = (37 * hash) + COMMAND_FIELD_NUMBER;
hash = (53 * hash) + getCommand().hashCode();
}
if (hasContainer()) {
hash = (37 * hash) + CONTAINER_FIELD_NUMBER;
hash = (53 * hash) + getContainer().hashCode();
}
if (getResourcesCount() > 0) {
hash = (37 * hash) + RESOURCES_FIELD_NUMBER;
hash = (53 * hash) + getResourcesList().hashCode();
}
if (hasName()) {
hash = (37 * hash) + NAME_FIELD_NUMBER;
hash = (53 * hash) + getName().hashCode();
}
if (hasSource()) {
hash = (37 * hash) + SOURCE_FIELD_NUMBER;
hash = (53 * hash) + getSource().hashCode();
}
if (hasData()) {
hash = (37 * hash) + DATA_FIELD_NUMBER;
hash = (53 * hash) + getData().hashCode();
}
if (hasDiscovery()) {
hash = (37 * hash) + DISCOVERY_FIELD_NUMBER;
hash = (53 * hash) + getDiscovery().hashCode();
}
if (hasShutdownGracePeriod()) {
hash = (37 * hash) + SHUTDOWN_GRACE_PERIOD_FIELD_NUMBER;
hash = (53 * hash) + getShutdownGracePeriod().hashCode();
}
if (hasLabels()) {
hash = (37 * hash) + LABELS_FIELD_NUMBER;
hash = (53 * hash) + getLabels().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.mesos.v1.Protos.ExecutorInfo parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.ExecutorInfo parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.ExecutorInfo parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.ExecutorInfo parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.ExecutorInfo parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.ExecutorInfo parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.ExecutorInfo parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.ExecutorInfo parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.ExecutorInfo parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.ExecutorInfo parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.ExecutorInfo parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.ExecutorInfo parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.apache.mesos.v1.Protos.ExecutorInfo prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
**
* Describes information about an executor.
*
*
* Protobuf type {@code mesos.v1.ExecutorInfo}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:mesos.v1.ExecutorInfo)
org.apache.mesos.v1.Protos.ExecutorInfoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_ExecutorInfo_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_ExecutorInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.mesos.v1.Protos.ExecutorInfo.class, org.apache.mesos.v1.Protos.ExecutorInfo.Builder.class);
}
// Construct using org.apache.mesos.v1.Protos.ExecutorInfo.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getExecutorIdFieldBuilder();
getFrameworkIdFieldBuilder();
getCommandFieldBuilder();
getContainerFieldBuilder();
getResourcesFieldBuilder();
getDiscoveryFieldBuilder();
getShutdownGracePeriodFieldBuilder();
getLabelsFieldBuilder();
}
}
public Builder clear() {
super.clear();
type_ = 0;
bitField0_ = (bitField0_ & ~0x00000001);
if (executorIdBuilder_ == null) {
executorId_ = null;
} else {
executorIdBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
if (frameworkIdBuilder_ == null) {
frameworkId_ = null;
} else {
frameworkIdBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000004);
if (commandBuilder_ == null) {
command_ = null;
} else {
commandBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000008);
if (containerBuilder_ == null) {
container_ = null;
} else {
containerBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000010);
if (resourcesBuilder_ == null) {
resources_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000020);
} else {
resourcesBuilder_.clear();
}
name_ = "";
bitField0_ = (bitField0_ & ~0x00000040);
source_ = "";
bitField0_ = (bitField0_ & ~0x00000080);
data_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000100);
if (discoveryBuilder_ == null) {
discovery_ = null;
} else {
discoveryBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000200);
if (shutdownGracePeriodBuilder_ == null) {
shutdownGracePeriod_ = null;
} else {
shutdownGracePeriodBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000400);
if (labelsBuilder_ == null) {
labels_ = null;
} else {
labelsBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000800);
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_ExecutorInfo_descriptor;
}
public org.apache.mesos.v1.Protos.ExecutorInfo getDefaultInstanceForType() {
return org.apache.mesos.v1.Protos.ExecutorInfo.getDefaultInstance();
}
public org.apache.mesos.v1.Protos.ExecutorInfo build() {
org.apache.mesos.v1.Protos.ExecutorInfo result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.apache.mesos.v1.Protos.ExecutorInfo buildPartial() {
org.apache.mesos.v1.Protos.ExecutorInfo result = new org.apache.mesos.v1.Protos.ExecutorInfo(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.type_ = type_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
if (executorIdBuilder_ == null) {
result.executorId_ = executorId_;
} else {
result.executorId_ = executorIdBuilder_.build();
}
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
if (frameworkIdBuilder_ == null) {
result.frameworkId_ = frameworkId_;
} else {
result.frameworkId_ = frameworkIdBuilder_.build();
}
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000008;
}
if (commandBuilder_ == null) {
result.command_ = command_;
} else {
result.command_ = commandBuilder_.build();
}
if (((from_bitField0_ & 0x00000010) == 0x00000010)) {
to_bitField0_ |= 0x00000010;
}
if (containerBuilder_ == null) {
result.container_ = container_;
} else {
result.container_ = containerBuilder_.build();
}
if (resourcesBuilder_ == null) {
if (((bitField0_ & 0x00000020) == 0x00000020)) {
resources_ = java.util.Collections.unmodifiableList(resources_);
bitField0_ = (bitField0_ & ~0x00000020);
}
result.resources_ = resources_;
} else {
result.resources_ = resourcesBuilder_.build();
}
if (((from_bitField0_ & 0x00000040) == 0x00000040)) {
to_bitField0_ |= 0x00000020;
}
result.name_ = name_;
if (((from_bitField0_ & 0x00000080) == 0x00000080)) {
to_bitField0_ |= 0x00000040;
}
result.source_ = source_;
if (((from_bitField0_ & 0x00000100) == 0x00000100)) {
to_bitField0_ |= 0x00000080;
}
result.data_ = data_;
if (((from_bitField0_ & 0x00000200) == 0x00000200)) {
to_bitField0_ |= 0x00000100;
}
if (discoveryBuilder_ == null) {
result.discovery_ = discovery_;
} else {
result.discovery_ = discoveryBuilder_.build();
}
if (((from_bitField0_ & 0x00000400) == 0x00000400)) {
to_bitField0_ |= 0x00000200;
}
if (shutdownGracePeriodBuilder_ == null) {
result.shutdownGracePeriod_ = shutdownGracePeriod_;
} else {
result.shutdownGracePeriod_ = shutdownGracePeriodBuilder_.build();
}
if (((from_bitField0_ & 0x00000800) == 0x00000800)) {
to_bitField0_ |= 0x00000400;
}
if (labelsBuilder_ == null) {
result.labels_ = labels_;
} else {
result.labels_ = labelsBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.apache.mesos.v1.Protos.ExecutorInfo) {
return mergeFrom((org.apache.mesos.v1.Protos.ExecutorInfo)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.mesos.v1.Protos.ExecutorInfo other) {
if (other == org.apache.mesos.v1.Protos.ExecutorInfo.getDefaultInstance()) return this;
if (other.hasType()) {
setType(other.getType());
}
if (other.hasExecutorId()) {
mergeExecutorId(other.getExecutorId());
}
if (other.hasFrameworkId()) {
mergeFrameworkId(other.getFrameworkId());
}
if (other.hasCommand()) {
mergeCommand(other.getCommand());
}
if (other.hasContainer()) {
mergeContainer(other.getContainer());
}
if (resourcesBuilder_ == null) {
if (!other.resources_.isEmpty()) {
if (resources_.isEmpty()) {
resources_ = other.resources_;
bitField0_ = (bitField0_ & ~0x00000020);
} else {
ensureResourcesIsMutable();
resources_.addAll(other.resources_);
}
onChanged();
}
} else {
if (!other.resources_.isEmpty()) {
if (resourcesBuilder_.isEmpty()) {
resourcesBuilder_.dispose();
resourcesBuilder_ = null;
resources_ = other.resources_;
bitField0_ = (bitField0_ & ~0x00000020);
resourcesBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getResourcesFieldBuilder() : null;
} else {
resourcesBuilder_.addAllMessages(other.resources_);
}
}
}
if (other.hasName()) {
bitField0_ |= 0x00000040;
name_ = other.name_;
onChanged();
}
if (other.hasSource()) {
bitField0_ |= 0x00000080;
source_ = other.source_;
onChanged();
}
if (other.hasData()) {
setData(other.getData());
}
if (other.hasDiscovery()) {
mergeDiscovery(other.getDiscovery());
}
if (other.hasShutdownGracePeriod()) {
mergeShutdownGracePeriod(other.getShutdownGracePeriod());
}
if (other.hasLabels()) {
mergeLabels(other.getLabels());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
if (!hasExecutorId()) {
return false;
}
if (!getExecutorId().isInitialized()) {
return false;
}
if (hasFrameworkId()) {
if (!getFrameworkId().isInitialized()) {
return false;
}
}
if (hasCommand()) {
if (!getCommand().isInitialized()) {
return false;
}
}
if (hasContainer()) {
if (!getContainer().isInitialized()) {
return false;
}
}
for (int i = 0; i < getResourcesCount(); i++) {
if (!getResources(i).isInitialized()) {
return false;
}
}
if (hasDiscovery()) {
if (!getDiscovery().isInitialized()) {
return false;
}
}
if (hasShutdownGracePeriod()) {
if (!getShutdownGracePeriod().isInitialized()) {
return false;
}
}
if (hasLabels()) {
if (!getLabels().isInitialized()) {
return false;
}
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.mesos.v1.Protos.ExecutorInfo parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.mesos.v1.Protos.ExecutorInfo) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private int type_ = 0;
/**
*
* For backwards compatibility, if this field is not set when using `LAUNCH`
* operation, Mesos will infer the type by checking if `command` is set
* (`CUSTOM`) or unset (`DEFAULT`). `type` must be set when using
* `LAUNCH_GROUP` operation.
* TODO(vinod): Add support for explicitly setting `type` to `DEFAULT` in
* `LAUNCH` operation.
*
*
* optional .mesos.v1.ExecutorInfo.Type type = 15;
*/
public boolean hasType() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
*
* For backwards compatibility, if this field is not set when using `LAUNCH`
* operation, Mesos will infer the type by checking if `command` is set
* (`CUSTOM`) or unset (`DEFAULT`). `type` must be set when using
* `LAUNCH_GROUP` operation.
* TODO(vinod): Add support for explicitly setting `type` to `DEFAULT` in
* `LAUNCH` operation.
*
*
* optional .mesos.v1.ExecutorInfo.Type type = 15;
*/
public org.apache.mesos.v1.Protos.ExecutorInfo.Type getType() {
org.apache.mesos.v1.Protos.ExecutorInfo.Type result = org.apache.mesos.v1.Protos.ExecutorInfo.Type.valueOf(type_);
return result == null ? org.apache.mesos.v1.Protos.ExecutorInfo.Type.UNKNOWN : result;
}
/**
*
* For backwards compatibility, if this field is not set when using `LAUNCH`
* operation, Mesos will infer the type by checking if `command` is set
* (`CUSTOM`) or unset (`DEFAULT`). `type` must be set when using
* `LAUNCH_GROUP` operation.
* TODO(vinod): Add support for explicitly setting `type` to `DEFAULT` in
* `LAUNCH` operation.
*
*
* optional .mesos.v1.ExecutorInfo.Type type = 15;
*/
public Builder setType(org.apache.mesos.v1.Protos.ExecutorInfo.Type value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
type_ = value.getNumber();
onChanged();
return this;
}
/**
*
* For backwards compatibility, if this field is not set when using `LAUNCH`
* operation, Mesos will infer the type by checking if `command` is set
* (`CUSTOM`) or unset (`DEFAULT`). `type` must be set when using
* `LAUNCH_GROUP` operation.
* TODO(vinod): Add support for explicitly setting `type` to `DEFAULT` in
* `LAUNCH` operation.
*
*
* optional .mesos.v1.ExecutorInfo.Type type = 15;
*/
public Builder clearType() {
bitField0_ = (bitField0_ & ~0x00000001);
type_ = 0;
onChanged();
return this;
}
private org.apache.mesos.v1.Protos.ExecutorID executorId_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
org.apache.mesos.v1.Protos.ExecutorID, org.apache.mesos.v1.Protos.ExecutorID.Builder, org.apache.mesos.v1.Protos.ExecutorIDOrBuilder> executorIdBuilder_;
/**
* required .mesos.v1.ExecutorID executor_id = 1;
*/
public boolean hasExecutorId() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required .mesos.v1.ExecutorID executor_id = 1;
*/
public org.apache.mesos.v1.Protos.ExecutorID getExecutorId() {
if (executorIdBuilder_ == null) {
return executorId_ == null ? org.apache.mesos.v1.Protos.ExecutorID.getDefaultInstance() : executorId_;
} else {
return executorIdBuilder_.getMessage();
}
}
/**
* required .mesos.v1.ExecutorID executor_id = 1;
*/
public Builder setExecutorId(org.apache.mesos.v1.Protos.ExecutorID value) {
if (executorIdBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
executorId_ = value;
onChanged();
} else {
executorIdBuilder_.setMessage(value);
}
bitField0_ |= 0x00000002;
return this;
}
/**
* required .mesos.v1.ExecutorID executor_id = 1;
*/
public Builder setExecutorId(
org.apache.mesos.v1.Protos.ExecutorID.Builder builderForValue) {
if (executorIdBuilder_ == null) {
executorId_ = builderForValue.build();
onChanged();
} else {
executorIdBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000002;
return this;
}
/**
* required .mesos.v1.ExecutorID executor_id = 1;
*/
public Builder mergeExecutorId(org.apache.mesos.v1.Protos.ExecutorID value) {
if (executorIdBuilder_ == null) {
if (((bitField0_ & 0x00000002) == 0x00000002) &&
executorId_ != null &&
executorId_ != org.apache.mesos.v1.Protos.ExecutorID.getDefaultInstance()) {
executorId_ =
org.apache.mesos.v1.Protos.ExecutorID.newBuilder(executorId_).mergeFrom(value).buildPartial();
} else {
executorId_ = value;
}
onChanged();
} else {
executorIdBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000002;
return this;
}
/**
* required .mesos.v1.ExecutorID executor_id = 1;
*/
public Builder clearExecutorId() {
if (executorIdBuilder_ == null) {
executorId_ = null;
onChanged();
} else {
executorIdBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
/**
* required .mesos.v1.ExecutorID executor_id = 1;
*/
public org.apache.mesos.v1.Protos.ExecutorID.Builder getExecutorIdBuilder() {
bitField0_ |= 0x00000002;
onChanged();
return getExecutorIdFieldBuilder().getBuilder();
}
/**
* required .mesos.v1.ExecutorID executor_id = 1;
*/
public org.apache.mesos.v1.Protos.ExecutorIDOrBuilder getExecutorIdOrBuilder() {
if (executorIdBuilder_ != null) {
return executorIdBuilder_.getMessageOrBuilder();
} else {
return executorId_ == null ?
org.apache.mesos.v1.Protos.ExecutorID.getDefaultInstance() : executorId_;
}
}
/**
* required .mesos.v1.ExecutorID executor_id = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.apache.mesos.v1.Protos.ExecutorID, org.apache.mesos.v1.Protos.ExecutorID.Builder, org.apache.mesos.v1.Protos.ExecutorIDOrBuilder>
getExecutorIdFieldBuilder() {
if (executorIdBuilder_ == null) {
executorIdBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.apache.mesos.v1.Protos.ExecutorID, org.apache.mesos.v1.Protos.ExecutorID.Builder, org.apache.mesos.v1.Protos.ExecutorIDOrBuilder>(
getExecutorId(),
getParentForChildren(),
isClean());
executorId_ = null;
}
return executorIdBuilder_;
}
private org.apache.mesos.v1.Protos.FrameworkID frameworkId_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
org.apache.mesos.v1.Protos.FrameworkID, org.apache.mesos.v1.Protos.FrameworkID.Builder, org.apache.mesos.v1.Protos.FrameworkIDOrBuilder> frameworkIdBuilder_;
/**
*
* TODO(benh): Make this required.
*
*
* optional .mesos.v1.FrameworkID framework_id = 8;
*/
public boolean hasFrameworkId() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
*
* TODO(benh): Make this required.
*
*
* optional .mesos.v1.FrameworkID framework_id = 8;
*/
public org.apache.mesos.v1.Protos.FrameworkID getFrameworkId() {
if (frameworkIdBuilder_ == null) {
return frameworkId_ == null ? org.apache.mesos.v1.Protos.FrameworkID.getDefaultInstance() : frameworkId_;
} else {
return frameworkIdBuilder_.getMessage();
}
}
/**
*
* TODO(benh): Make this required.
*
*
* optional .mesos.v1.FrameworkID framework_id = 8;
*/
public Builder setFrameworkId(org.apache.mesos.v1.Protos.FrameworkID value) {
if (frameworkIdBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
frameworkId_ = value;
onChanged();
} else {
frameworkIdBuilder_.setMessage(value);
}
bitField0_ |= 0x00000004;
return this;
}
/**
*
* TODO(benh): Make this required.
*
*
* optional .mesos.v1.FrameworkID framework_id = 8;
*/
public Builder setFrameworkId(
org.apache.mesos.v1.Protos.FrameworkID.Builder builderForValue) {
if (frameworkIdBuilder_ == null) {
frameworkId_ = builderForValue.build();
onChanged();
} else {
frameworkIdBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000004;
return this;
}
/**
*
* TODO(benh): Make this required.
*
*
* optional .mesos.v1.FrameworkID framework_id = 8;
*/
public Builder mergeFrameworkId(org.apache.mesos.v1.Protos.FrameworkID value) {
if (frameworkIdBuilder_ == null) {
if (((bitField0_ & 0x00000004) == 0x00000004) &&
frameworkId_ != null &&
frameworkId_ != org.apache.mesos.v1.Protos.FrameworkID.getDefaultInstance()) {
frameworkId_ =
org.apache.mesos.v1.Protos.FrameworkID.newBuilder(frameworkId_).mergeFrom(value).buildPartial();
} else {
frameworkId_ = value;
}
onChanged();
} else {
frameworkIdBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000004;
return this;
}
/**
*
* TODO(benh): Make this required.
*
*
* optional .mesos.v1.FrameworkID framework_id = 8;
*/
public Builder clearFrameworkId() {
if (frameworkIdBuilder_ == null) {
frameworkId_ = null;
onChanged();
} else {
frameworkIdBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000004);
return this;
}
/**
*
* TODO(benh): Make this required.
*
*
* optional .mesos.v1.FrameworkID framework_id = 8;
*/
public org.apache.mesos.v1.Protos.FrameworkID.Builder getFrameworkIdBuilder() {
bitField0_ |= 0x00000004;
onChanged();
return getFrameworkIdFieldBuilder().getBuilder();
}
/**
*
* TODO(benh): Make this required.
*
*
* optional .mesos.v1.FrameworkID framework_id = 8;
*/
public org.apache.mesos.v1.Protos.FrameworkIDOrBuilder getFrameworkIdOrBuilder() {
if (frameworkIdBuilder_ != null) {
return frameworkIdBuilder_.getMessageOrBuilder();
} else {
return frameworkId_ == null ?
org.apache.mesos.v1.Protos.FrameworkID.getDefaultInstance() : frameworkId_;
}
}
/**
*
* TODO(benh): Make this required.
*
*
* optional .mesos.v1.FrameworkID framework_id = 8;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.apache.mesos.v1.Protos.FrameworkID, org.apache.mesos.v1.Protos.FrameworkID.Builder, org.apache.mesos.v1.Protos.FrameworkIDOrBuilder>
getFrameworkIdFieldBuilder() {
if (frameworkIdBuilder_ == null) {
frameworkIdBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.apache.mesos.v1.Protos.FrameworkID, org.apache.mesos.v1.Protos.FrameworkID.Builder, org.apache.mesos.v1.Protos.FrameworkIDOrBuilder>(
getFrameworkId(),
getParentForChildren(),
isClean());
frameworkId_ = null;
}
return frameworkIdBuilder_;
}
private org.apache.mesos.v1.Protos.CommandInfo command_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
org.apache.mesos.v1.Protos.CommandInfo, org.apache.mesos.v1.Protos.CommandInfo.Builder, org.apache.mesos.v1.Protos.CommandInfoOrBuilder> commandBuilder_;
/**
* optional .mesos.v1.CommandInfo command = 7;
*/
public boolean hasCommand() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional .mesos.v1.CommandInfo command = 7;
*/
public org.apache.mesos.v1.Protos.CommandInfo getCommand() {
if (commandBuilder_ == null) {
return command_ == null ? org.apache.mesos.v1.Protos.CommandInfo.getDefaultInstance() : command_;
} else {
return commandBuilder_.getMessage();
}
}
/**
* optional .mesos.v1.CommandInfo command = 7;
*/
public Builder setCommand(org.apache.mesos.v1.Protos.CommandInfo value) {
if (commandBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
command_ = value;
onChanged();
} else {
commandBuilder_.setMessage(value);
}
bitField0_ |= 0x00000008;
return this;
}
/**
* optional .mesos.v1.CommandInfo command = 7;
*/
public Builder setCommand(
org.apache.mesos.v1.Protos.CommandInfo.Builder builderForValue) {
if (commandBuilder_ == null) {
command_ = builderForValue.build();
onChanged();
} else {
commandBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000008;
return this;
}
/**
* optional .mesos.v1.CommandInfo command = 7;
*/
public Builder mergeCommand(org.apache.mesos.v1.Protos.CommandInfo value) {
if (commandBuilder_ == null) {
if (((bitField0_ & 0x00000008) == 0x00000008) &&
command_ != null &&
command_ != org.apache.mesos.v1.Protos.CommandInfo.getDefaultInstance()) {
command_ =
org.apache.mesos.v1.Protos.CommandInfo.newBuilder(command_).mergeFrom(value).buildPartial();
} else {
command_ = value;
}
onChanged();
} else {
commandBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000008;
return this;
}
/**
* optional .mesos.v1.CommandInfo command = 7;
*/
public Builder clearCommand() {
if (commandBuilder_ == null) {
command_ = null;
onChanged();
} else {
commandBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000008);
return this;
}
/**
* optional .mesos.v1.CommandInfo command = 7;
*/
public org.apache.mesos.v1.Protos.CommandInfo.Builder getCommandBuilder() {
bitField0_ |= 0x00000008;
onChanged();
return getCommandFieldBuilder().getBuilder();
}
/**
* optional .mesos.v1.CommandInfo command = 7;
*/
public org.apache.mesos.v1.Protos.CommandInfoOrBuilder getCommandOrBuilder() {
if (commandBuilder_ != null) {
return commandBuilder_.getMessageOrBuilder();
} else {
return command_ == null ?
org.apache.mesos.v1.Protos.CommandInfo.getDefaultInstance() : command_;
}
}
/**
* optional .mesos.v1.CommandInfo command = 7;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.apache.mesos.v1.Protos.CommandInfo, org.apache.mesos.v1.Protos.CommandInfo.Builder, org.apache.mesos.v1.Protos.CommandInfoOrBuilder>
getCommandFieldBuilder() {
if (commandBuilder_ == null) {
commandBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.apache.mesos.v1.Protos.CommandInfo, org.apache.mesos.v1.Protos.CommandInfo.Builder, org.apache.mesos.v1.Protos.CommandInfoOrBuilder>(
getCommand(),
getParentForChildren(),
isClean());
command_ = null;
}
return commandBuilder_;
}
private org.apache.mesos.v1.Protos.ContainerInfo container_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
org.apache.mesos.v1.Protos.ContainerInfo, org.apache.mesos.v1.Protos.ContainerInfo.Builder, org.apache.mesos.v1.Protos.ContainerInfoOrBuilder> containerBuilder_;
/**
*
* Executor provided with a container will launch the container
* with the executor's CommandInfo and we expect the container to
* act as a Mesos executor.
*
*
* optional .mesos.v1.ContainerInfo container = 11;
*/
public boolean hasContainer() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
*
* Executor provided with a container will launch the container
* with the executor's CommandInfo and we expect the container to
* act as a Mesos executor.
*
*
* optional .mesos.v1.ContainerInfo container = 11;
*/
public org.apache.mesos.v1.Protos.ContainerInfo getContainer() {
if (containerBuilder_ == null) {
return container_ == null ? org.apache.mesos.v1.Protos.ContainerInfo.getDefaultInstance() : container_;
} else {
return containerBuilder_.getMessage();
}
}
/**
*
* Executor provided with a container will launch the container
* with the executor's CommandInfo and we expect the container to
* act as a Mesos executor.
*
*
* optional .mesos.v1.ContainerInfo container = 11;
*/
public Builder setContainer(org.apache.mesos.v1.Protos.ContainerInfo value) {
if (containerBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
container_ = value;
onChanged();
} else {
containerBuilder_.setMessage(value);
}
bitField0_ |= 0x00000010;
return this;
}
/**
*
* Executor provided with a container will launch the container
* with the executor's CommandInfo and we expect the container to
* act as a Mesos executor.
*
*
* optional .mesos.v1.ContainerInfo container = 11;
*/
public Builder setContainer(
org.apache.mesos.v1.Protos.ContainerInfo.Builder builderForValue) {
if (containerBuilder_ == null) {
container_ = builderForValue.build();
onChanged();
} else {
containerBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000010;
return this;
}
/**
*
* Executor provided with a container will launch the container
* with the executor's CommandInfo and we expect the container to
* act as a Mesos executor.
*
*
* optional .mesos.v1.ContainerInfo container = 11;
*/
public Builder mergeContainer(org.apache.mesos.v1.Protos.ContainerInfo value) {
if (containerBuilder_ == null) {
if (((bitField0_ & 0x00000010) == 0x00000010) &&
container_ != null &&
container_ != org.apache.mesos.v1.Protos.ContainerInfo.getDefaultInstance()) {
container_ =
org.apache.mesos.v1.Protos.ContainerInfo.newBuilder(container_).mergeFrom(value).buildPartial();
} else {
container_ = value;
}
onChanged();
} else {
containerBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000010;
return this;
}
/**
*
* Executor provided with a container will launch the container
* with the executor's CommandInfo and we expect the container to
* act as a Mesos executor.
*
*
* optional .mesos.v1.ContainerInfo container = 11;
*/
public Builder clearContainer() {
if (containerBuilder_ == null) {
container_ = null;
onChanged();
} else {
containerBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000010);
return this;
}
/**
*
* Executor provided with a container will launch the container
* with the executor's CommandInfo and we expect the container to
* act as a Mesos executor.
*
*
* optional .mesos.v1.ContainerInfo container = 11;
*/
public org.apache.mesos.v1.Protos.ContainerInfo.Builder getContainerBuilder() {
bitField0_ |= 0x00000010;
onChanged();
return getContainerFieldBuilder().getBuilder();
}
/**
*
* Executor provided with a container will launch the container
* with the executor's CommandInfo and we expect the container to
* act as a Mesos executor.
*
*
* optional .mesos.v1.ContainerInfo container = 11;
*/
public org.apache.mesos.v1.Protos.ContainerInfoOrBuilder getContainerOrBuilder() {
if (containerBuilder_ != null) {
return containerBuilder_.getMessageOrBuilder();
} else {
return container_ == null ?
org.apache.mesos.v1.Protos.ContainerInfo.getDefaultInstance() : container_;
}
}
/**
*
* Executor provided with a container will launch the container
* with the executor's CommandInfo and we expect the container to
* act as a Mesos executor.
*
*
* optional .mesos.v1.ContainerInfo container = 11;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.apache.mesos.v1.Protos.ContainerInfo, org.apache.mesos.v1.Protos.ContainerInfo.Builder, org.apache.mesos.v1.Protos.ContainerInfoOrBuilder>
getContainerFieldBuilder() {
if (containerBuilder_ == null) {
containerBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.apache.mesos.v1.Protos.ContainerInfo, org.apache.mesos.v1.Protos.ContainerInfo.Builder, org.apache.mesos.v1.Protos.ContainerInfoOrBuilder>(
getContainer(),
getParentForChildren(),
isClean());
container_ = null;
}
return containerBuilder_;
}
private java.util.List resources_ =
java.util.Collections.emptyList();
private void ensureResourcesIsMutable() {
if (!((bitField0_ & 0x00000020) == 0x00000020)) {
resources_ = new java.util.ArrayList(resources_);
bitField0_ |= 0x00000020;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.apache.mesos.v1.Protos.Resource, org.apache.mesos.v1.Protos.Resource.Builder, org.apache.mesos.v1.Protos.ResourceOrBuilder> resourcesBuilder_;
/**
* repeated .mesos.v1.Resource resources = 5;
*/
public java.util.List getResourcesList() {
if (resourcesBuilder_ == null) {
return java.util.Collections.unmodifiableList(resources_);
} else {
return resourcesBuilder_.getMessageList();
}
}
/**
* repeated .mesos.v1.Resource resources = 5;
*/
public int getResourcesCount() {
if (resourcesBuilder_ == null) {
return resources_.size();
} else {
return resourcesBuilder_.getCount();
}
}
/**
* repeated .mesos.v1.Resource resources = 5;
*/
public org.apache.mesos.v1.Protos.Resource getResources(int index) {
if (resourcesBuilder_ == null) {
return resources_.get(index);
} else {
return resourcesBuilder_.getMessage(index);
}
}
/**
* repeated .mesos.v1.Resource resources = 5;
*/
public Builder setResources(
int index, org.apache.mesos.v1.Protos.Resource value) {
if (resourcesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureResourcesIsMutable();
resources_.set(index, value);
onChanged();
} else {
resourcesBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .mesos.v1.Resource resources = 5;
*/
public Builder setResources(
int index, org.apache.mesos.v1.Protos.Resource.Builder builderForValue) {
if (resourcesBuilder_ == null) {
ensureResourcesIsMutable();
resources_.set(index, builderForValue.build());
onChanged();
} else {
resourcesBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .mesos.v1.Resource resources = 5;
*/
public Builder addResources(org.apache.mesos.v1.Protos.Resource value) {
if (resourcesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureResourcesIsMutable();
resources_.add(value);
onChanged();
} else {
resourcesBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .mesos.v1.Resource resources = 5;
*/
public Builder addResources(
int index, org.apache.mesos.v1.Protos.Resource value) {
if (resourcesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureResourcesIsMutable();
resources_.add(index, value);
onChanged();
} else {
resourcesBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .mesos.v1.Resource resources = 5;
*/
public Builder addResources(
org.apache.mesos.v1.Protos.Resource.Builder builderForValue) {
if (resourcesBuilder_ == null) {
ensureResourcesIsMutable();
resources_.add(builderForValue.build());
onChanged();
} else {
resourcesBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .mesos.v1.Resource resources = 5;
*/
public Builder addResources(
int index, org.apache.mesos.v1.Protos.Resource.Builder builderForValue) {
if (resourcesBuilder_ == null) {
ensureResourcesIsMutable();
resources_.add(index, builderForValue.build());
onChanged();
} else {
resourcesBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .mesos.v1.Resource resources = 5;
*/
public Builder addAllResources(
java.lang.Iterable extends org.apache.mesos.v1.Protos.Resource> values) {
if (resourcesBuilder_ == null) {
ensureResourcesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, resources_);
onChanged();
} else {
resourcesBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .mesos.v1.Resource resources = 5;
*/
public Builder clearResources() {
if (resourcesBuilder_ == null) {
resources_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000020);
onChanged();
} else {
resourcesBuilder_.clear();
}
return this;
}
/**
* repeated .mesos.v1.Resource resources = 5;
*/
public Builder removeResources(int index) {
if (resourcesBuilder_ == null) {
ensureResourcesIsMutable();
resources_.remove(index);
onChanged();
} else {
resourcesBuilder_.remove(index);
}
return this;
}
/**
* repeated .mesos.v1.Resource resources = 5;
*/
public org.apache.mesos.v1.Protos.Resource.Builder getResourcesBuilder(
int index) {
return getResourcesFieldBuilder().getBuilder(index);
}
/**
* repeated .mesos.v1.Resource resources = 5;
*/
public org.apache.mesos.v1.Protos.ResourceOrBuilder getResourcesOrBuilder(
int index) {
if (resourcesBuilder_ == null) {
return resources_.get(index); } else {
return resourcesBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .mesos.v1.Resource resources = 5;
*/
public java.util.List extends org.apache.mesos.v1.Protos.ResourceOrBuilder>
getResourcesOrBuilderList() {
if (resourcesBuilder_ != null) {
return resourcesBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(resources_);
}
}
/**
* repeated .mesos.v1.Resource resources = 5;
*/
public org.apache.mesos.v1.Protos.Resource.Builder addResourcesBuilder() {
return getResourcesFieldBuilder().addBuilder(
org.apache.mesos.v1.Protos.Resource.getDefaultInstance());
}
/**
* repeated .mesos.v1.Resource resources = 5;
*/
public org.apache.mesos.v1.Protos.Resource.Builder addResourcesBuilder(
int index) {
return getResourcesFieldBuilder().addBuilder(
index, org.apache.mesos.v1.Protos.Resource.getDefaultInstance());
}
/**
* repeated .mesos.v1.Resource resources = 5;
*/
public java.util.List
getResourcesBuilderList() {
return getResourcesFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.apache.mesos.v1.Protos.Resource, org.apache.mesos.v1.Protos.Resource.Builder, org.apache.mesos.v1.Protos.ResourceOrBuilder>
getResourcesFieldBuilder() {
if (resourcesBuilder_ == null) {
resourcesBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
org.apache.mesos.v1.Protos.Resource, org.apache.mesos.v1.Protos.Resource.Builder, org.apache.mesos.v1.Protos.ResourceOrBuilder>(
resources_,
((bitField0_ & 0x00000020) == 0x00000020),
getParentForChildren(),
isClean());
resources_ = null;
}
return resourcesBuilder_;
}
private java.lang.Object name_ = "";
/**
* optional string name = 9;
*/
public boolean hasName() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
* optional string name = 9;
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
name_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string name = 9;
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string name = 9;
*/
public Builder setName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000040;
name_ = value;
onChanged();
return this;
}
/**
* optional string name = 9;
*/
public Builder clearName() {
bitField0_ = (bitField0_ & ~0x00000040);
name_ = getDefaultInstance().getName();
onChanged();
return this;
}
/**
* optional string name = 9;
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000040;
name_ = value;
onChanged();
return this;
}
private java.lang.Object source_ = "";
/**
*
* 'source' is an identifier style string used by frameworks to
* track the source of an executor. This is useful when it's
* possible for different executor ids to be related semantically.
* NOTE: 'source' is exposed alongside the resource usage of the
* executor via JSON on the agent. This allows users to import usage
* information into a time series database for monitoring.
* This field is deprecated since 1.0. Please use labels for
* free-form metadata instead.
*
*
* optional string source = 10 [deprecated = true];
*/
@java.lang.Deprecated public boolean hasSource() {
return ((bitField0_ & 0x00000080) == 0x00000080);
}
/**
*
* 'source' is an identifier style string used by frameworks to
* track the source of an executor. This is useful when it's
* possible for different executor ids to be related semantically.
* NOTE: 'source' is exposed alongside the resource usage of the
* executor via JSON on the agent. This allows users to import usage
* information into a time series database for monitoring.
* This field is deprecated since 1.0. Please use labels for
* free-form metadata instead.
*
*
* optional string source = 10 [deprecated = true];
*/
@java.lang.Deprecated public java.lang.String getSource() {
java.lang.Object ref = source_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
source_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* 'source' is an identifier style string used by frameworks to
* track the source of an executor. This is useful when it's
* possible for different executor ids to be related semantically.
* NOTE: 'source' is exposed alongside the resource usage of the
* executor via JSON on the agent. This allows users to import usage
* information into a time series database for monitoring.
* This field is deprecated since 1.0. Please use labels for
* free-form metadata instead.
*
*
* optional string source = 10 [deprecated = true];
*/
@java.lang.Deprecated public com.google.protobuf.ByteString
getSourceBytes() {
java.lang.Object ref = source_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
source_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* 'source' is an identifier style string used by frameworks to
* track the source of an executor. This is useful when it's
* possible for different executor ids to be related semantically.
* NOTE: 'source' is exposed alongside the resource usage of the
* executor via JSON on the agent. This allows users to import usage
* information into a time series database for monitoring.
* This field is deprecated since 1.0. Please use labels for
* free-form metadata instead.
*
*
* optional string source = 10 [deprecated = true];
*/
@java.lang.Deprecated public Builder setSource(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000080;
source_ = value;
onChanged();
return this;
}
/**
*
* 'source' is an identifier style string used by frameworks to
* track the source of an executor. This is useful when it's
* possible for different executor ids to be related semantically.
* NOTE: 'source' is exposed alongside the resource usage of the
* executor via JSON on the agent. This allows users to import usage
* information into a time series database for monitoring.
* This field is deprecated since 1.0. Please use labels for
* free-form metadata instead.
*
*
* optional string source = 10 [deprecated = true];
*/
@java.lang.Deprecated public Builder clearSource() {
bitField0_ = (bitField0_ & ~0x00000080);
source_ = getDefaultInstance().getSource();
onChanged();
return this;
}
/**
*
* 'source' is an identifier style string used by frameworks to
* track the source of an executor. This is useful when it's
* possible for different executor ids to be related semantically.
* NOTE: 'source' is exposed alongside the resource usage of the
* executor via JSON on the agent. This allows users to import usage
* information into a time series database for monitoring.
* This field is deprecated since 1.0. Please use labels for
* free-form metadata instead.
*
*
* optional string source = 10 [deprecated = true];
*/
@java.lang.Deprecated public Builder setSourceBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000080;
source_ = value;
onChanged();
return this;
}
private com.google.protobuf.ByteString data_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* This field can be used to pass arbitrary bytes to an executor.
*
*
* optional bytes data = 4;
*/
public boolean hasData() {
return ((bitField0_ & 0x00000100) == 0x00000100);
}
/**
*
* This field can be used to pass arbitrary bytes to an executor.
*
*
* optional bytes data = 4;
*/
public com.google.protobuf.ByteString getData() {
return data_;
}
/**
*
* This field can be used to pass arbitrary bytes to an executor.
*
*
* optional bytes data = 4;
*/
public Builder setData(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000100;
data_ = value;
onChanged();
return this;
}
/**
*
* This field can be used to pass arbitrary bytes to an executor.
*
*
* optional bytes data = 4;
*/
public Builder clearData() {
bitField0_ = (bitField0_ & ~0x00000100);
data_ = getDefaultInstance().getData();
onChanged();
return this;
}
private org.apache.mesos.v1.Protos.DiscoveryInfo discovery_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
org.apache.mesos.v1.Protos.DiscoveryInfo, org.apache.mesos.v1.Protos.DiscoveryInfo.Builder, org.apache.mesos.v1.Protos.DiscoveryInfoOrBuilder> discoveryBuilder_;
/**
*
* Service discovery information for the executor. It is not
* interpreted or acted upon by Mesos. It is up to a service
* discovery system to use this information as needed and to handle
* executors without service discovery information.
*
*
* optional .mesos.v1.DiscoveryInfo discovery = 12;
*/
public boolean hasDiscovery() {
return ((bitField0_ & 0x00000200) == 0x00000200);
}
/**
*
* Service discovery information for the executor. It is not
* interpreted or acted upon by Mesos. It is up to a service
* discovery system to use this information as needed and to handle
* executors without service discovery information.
*
*
* optional .mesos.v1.DiscoveryInfo discovery = 12;
*/
public org.apache.mesos.v1.Protos.DiscoveryInfo getDiscovery() {
if (discoveryBuilder_ == null) {
return discovery_ == null ? org.apache.mesos.v1.Protos.DiscoveryInfo.getDefaultInstance() : discovery_;
} else {
return discoveryBuilder_.getMessage();
}
}
/**
*
* Service discovery information for the executor. It is not
* interpreted or acted upon by Mesos. It is up to a service
* discovery system to use this information as needed and to handle
* executors without service discovery information.
*
*
* optional .mesos.v1.DiscoveryInfo discovery = 12;
*/
public Builder setDiscovery(org.apache.mesos.v1.Protos.DiscoveryInfo value) {
if (discoveryBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
discovery_ = value;
onChanged();
} else {
discoveryBuilder_.setMessage(value);
}
bitField0_ |= 0x00000200;
return this;
}
/**
*
* Service discovery information for the executor. It is not
* interpreted or acted upon by Mesos. It is up to a service
* discovery system to use this information as needed and to handle
* executors without service discovery information.
*
*
* optional .mesos.v1.DiscoveryInfo discovery = 12;
*/
public Builder setDiscovery(
org.apache.mesos.v1.Protos.DiscoveryInfo.Builder builderForValue) {
if (discoveryBuilder_ == null) {
discovery_ = builderForValue.build();
onChanged();
} else {
discoveryBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000200;
return this;
}
/**
*
* Service discovery information for the executor. It is not
* interpreted or acted upon by Mesos. It is up to a service
* discovery system to use this information as needed and to handle
* executors without service discovery information.
*
*
* optional .mesos.v1.DiscoveryInfo discovery = 12;
*/
public Builder mergeDiscovery(org.apache.mesos.v1.Protos.DiscoveryInfo value) {
if (discoveryBuilder_ == null) {
if (((bitField0_ & 0x00000200) == 0x00000200) &&
discovery_ != null &&
discovery_ != org.apache.mesos.v1.Protos.DiscoveryInfo.getDefaultInstance()) {
discovery_ =
org.apache.mesos.v1.Protos.DiscoveryInfo.newBuilder(discovery_).mergeFrom(value).buildPartial();
} else {
discovery_ = value;
}
onChanged();
} else {
discoveryBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000200;
return this;
}
/**
*
* Service discovery information for the executor. It is not
* interpreted or acted upon by Mesos. It is up to a service
* discovery system to use this information as needed and to handle
* executors without service discovery information.
*
*
* optional .mesos.v1.DiscoveryInfo discovery = 12;
*/
public Builder clearDiscovery() {
if (discoveryBuilder_ == null) {
discovery_ = null;
onChanged();
} else {
discoveryBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000200);
return this;
}
/**
*
* Service discovery information for the executor. It is not
* interpreted or acted upon by Mesos. It is up to a service
* discovery system to use this information as needed and to handle
* executors without service discovery information.
*
*
* optional .mesos.v1.DiscoveryInfo discovery = 12;
*/
public org.apache.mesos.v1.Protos.DiscoveryInfo.Builder getDiscoveryBuilder() {
bitField0_ |= 0x00000200;
onChanged();
return getDiscoveryFieldBuilder().getBuilder();
}
/**
*
* Service discovery information for the executor. It is not
* interpreted or acted upon by Mesos. It is up to a service
* discovery system to use this information as needed and to handle
* executors without service discovery information.
*
*
* optional .mesos.v1.DiscoveryInfo discovery = 12;
*/
public org.apache.mesos.v1.Protos.DiscoveryInfoOrBuilder getDiscoveryOrBuilder() {
if (discoveryBuilder_ != null) {
return discoveryBuilder_.getMessageOrBuilder();
} else {
return discovery_ == null ?
org.apache.mesos.v1.Protos.DiscoveryInfo.getDefaultInstance() : discovery_;
}
}
/**
*
* Service discovery information for the executor. It is not
* interpreted or acted upon by Mesos. It is up to a service
* discovery system to use this information as needed and to handle
* executors without service discovery information.
*
*
* optional .mesos.v1.DiscoveryInfo discovery = 12;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.apache.mesos.v1.Protos.DiscoveryInfo, org.apache.mesos.v1.Protos.DiscoveryInfo.Builder, org.apache.mesos.v1.Protos.DiscoveryInfoOrBuilder>
getDiscoveryFieldBuilder() {
if (discoveryBuilder_ == null) {
discoveryBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.apache.mesos.v1.Protos.DiscoveryInfo, org.apache.mesos.v1.Protos.DiscoveryInfo.Builder, org.apache.mesos.v1.Protos.DiscoveryInfoOrBuilder>(
getDiscovery(),
getParentForChildren(),
isClean());
discovery_ = null;
}
return discoveryBuilder_;
}
private org.apache.mesos.v1.Protos.DurationInfo shutdownGracePeriod_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
org.apache.mesos.v1.Protos.DurationInfo, org.apache.mesos.v1.Protos.DurationInfo.Builder, org.apache.mesos.v1.Protos.DurationInfoOrBuilder> shutdownGracePeriodBuilder_;
/**
*
* When shutting down an executor the agent will wait in a
* best-effort manner for the grace period specified here
* before forcibly destroying the container. The executor
* must not assume that it will always be allotted the full
* grace period, as the agent may decide to allot a shorter
* period and failures / forcible terminations may occur.
*
*
* optional .mesos.v1.DurationInfo shutdown_grace_period = 13;
*/
public boolean hasShutdownGracePeriod() {
return ((bitField0_ & 0x00000400) == 0x00000400);
}
/**
*
* When shutting down an executor the agent will wait in a
* best-effort manner for the grace period specified here
* before forcibly destroying the container. The executor
* must not assume that it will always be allotted the full
* grace period, as the agent may decide to allot a shorter
* period and failures / forcible terminations may occur.
*
*
* optional .mesos.v1.DurationInfo shutdown_grace_period = 13;
*/
public org.apache.mesos.v1.Protos.DurationInfo getShutdownGracePeriod() {
if (shutdownGracePeriodBuilder_ == null) {
return shutdownGracePeriod_ == null ? org.apache.mesos.v1.Protos.DurationInfo.getDefaultInstance() : shutdownGracePeriod_;
} else {
return shutdownGracePeriodBuilder_.getMessage();
}
}
/**
*
* When shutting down an executor the agent will wait in a
* best-effort manner for the grace period specified here
* before forcibly destroying the container. The executor
* must not assume that it will always be allotted the full
* grace period, as the agent may decide to allot a shorter
* period and failures / forcible terminations may occur.
*
*
* optional .mesos.v1.DurationInfo shutdown_grace_period = 13;
*/
public Builder setShutdownGracePeriod(org.apache.mesos.v1.Protos.DurationInfo value) {
if (shutdownGracePeriodBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
shutdownGracePeriod_ = value;
onChanged();
} else {
shutdownGracePeriodBuilder_.setMessage(value);
}
bitField0_ |= 0x00000400;
return this;
}
/**
*
* When shutting down an executor the agent will wait in a
* best-effort manner for the grace period specified here
* before forcibly destroying the container. The executor
* must not assume that it will always be allotted the full
* grace period, as the agent may decide to allot a shorter
* period and failures / forcible terminations may occur.
*
*
* optional .mesos.v1.DurationInfo shutdown_grace_period = 13;
*/
public Builder setShutdownGracePeriod(
org.apache.mesos.v1.Protos.DurationInfo.Builder builderForValue) {
if (shutdownGracePeriodBuilder_ == null) {
shutdownGracePeriod_ = builderForValue.build();
onChanged();
} else {
shutdownGracePeriodBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000400;
return this;
}
/**
*
* When shutting down an executor the agent will wait in a
* best-effort manner for the grace period specified here
* before forcibly destroying the container. The executor
* must not assume that it will always be allotted the full
* grace period, as the agent may decide to allot a shorter
* period and failures / forcible terminations may occur.
*
*
* optional .mesos.v1.DurationInfo shutdown_grace_period = 13;
*/
public Builder mergeShutdownGracePeriod(org.apache.mesos.v1.Protos.DurationInfo value) {
if (shutdownGracePeriodBuilder_ == null) {
if (((bitField0_ & 0x00000400) == 0x00000400) &&
shutdownGracePeriod_ != null &&
shutdownGracePeriod_ != org.apache.mesos.v1.Protos.DurationInfo.getDefaultInstance()) {
shutdownGracePeriod_ =
org.apache.mesos.v1.Protos.DurationInfo.newBuilder(shutdownGracePeriod_).mergeFrom(value).buildPartial();
} else {
shutdownGracePeriod_ = value;
}
onChanged();
} else {
shutdownGracePeriodBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000400;
return this;
}
/**
*
* When shutting down an executor the agent will wait in a
* best-effort manner for the grace period specified here
* before forcibly destroying the container. The executor
* must not assume that it will always be allotted the full
* grace period, as the agent may decide to allot a shorter
* period and failures / forcible terminations may occur.
*
*
* optional .mesos.v1.DurationInfo shutdown_grace_period = 13;
*/
public Builder clearShutdownGracePeriod() {
if (shutdownGracePeriodBuilder_ == null) {
shutdownGracePeriod_ = null;
onChanged();
} else {
shutdownGracePeriodBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000400);
return this;
}
/**
*
* When shutting down an executor the agent will wait in a
* best-effort manner for the grace period specified here
* before forcibly destroying the container. The executor
* must not assume that it will always be allotted the full
* grace period, as the agent may decide to allot a shorter
* period and failures / forcible terminations may occur.
*
*
* optional .mesos.v1.DurationInfo shutdown_grace_period = 13;
*/
public org.apache.mesos.v1.Protos.DurationInfo.Builder getShutdownGracePeriodBuilder() {
bitField0_ |= 0x00000400;
onChanged();
return getShutdownGracePeriodFieldBuilder().getBuilder();
}
/**
*
* When shutting down an executor the agent will wait in a
* best-effort manner for the grace period specified here
* before forcibly destroying the container. The executor
* must not assume that it will always be allotted the full
* grace period, as the agent may decide to allot a shorter
* period and failures / forcible terminations may occur.
*
*
* optional .mesos.v1.DurationInfo shutdown_grace_period = 13;
*/
public org.apache.mesos.v1.Protos.DurationInfoOrBuilder getShutdownGracePeriodOrBuilder() {
if (shutdownGracePeriodBuilder_ != null) {
return shutdownGracePeriodBuilder_.getMessageOrBuilder();
} else {
return shutdownGracePeriod_ == null ?
org.apache.mesos.v1.Protos.DurationInfo.getDefaultInstance() : shutdownGracePeriod_;
}
}
/**
*
* When shutting down an executor the agent will wait in a
* best-effort manner for the grace period specified here
* before forcibly destroying the container. The executor
* must not assume that it will always be allotted the full
* grace period, as the agent may decide to allot a shorter
* period and failures / forcible terminations may occur.
*
*
* optional .mesos.v1.DurationInfo shutdown_grace_period = 13;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.apache.mesos.v1.Protos.DurationInfo, org.apache.mesos.v1.Protos.DurationInfo.Builder, org.apache.mesos.v1.Protos.DurationInfoOrBuilder>
getShutdownGracePeriodFieldBuilder() {
if (shutdownGracePeriodBuilder_ == null) {
shutdownGracePeriodBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.apache.mesos.v1.Protos.DurationInfo, org.apache.mesos.v1.Protos.DurationInfo.Builder, org.apache.mesos.v1.Protos.DurationInfoOrBuilder>(
getShutdownGracePeriod(),
getParentForChildren(),
isClean());
shutdownGracePeriod_ = null;
}
return shutdownGracePeriodBuilder_;
}
private org.apache.mesos.v1.Protos.Labels labels_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
org.apache.mesos.v1.Protos.Labels, org.apache.mesos.v1.Protos.Labels.Builder, org.apache.mesos.v1.Protos.LabelsOrBuilder> labelsBuilder_;
/**
*
* Labels are free-form key value pairs which are exposed through
* master and agent endpoints. Labels will not be interpreted or
* acted upon by Mesos itself. As opposed to the data field, labels
* will be kept in memory on master and agent processes. Therefore,
* labels should be used to tag executors with lightweight metadata.
* Labels should not contain duplicate key-value pairs.
*
*
* optional .mesos.v1.Labels labels = 14;
*/
public boolean hasLabels() {
return ((bitField0_ & 0x00000800) == 0x00000800);
}
/**
*
* Labels are free-form key value pairs which are exposed through
* master and agent endpoints. Labels will not be interpreted or
* acted upon by Mesos itself. As opposed to the data field, labels
* will be kept in memory on master and agent processes. Therefore,
* labels should be used to tag executors with lightweight metadata.
* Labels should not contain duplicate key-value pairs.
*
*
* optional .mesos.v1.Labels labels = 14;
*/
public org.apache.mesos.v1.Protos.Labels getLabels() {
if (labelsBuilder_ == null) {
return labels_ == null ? org.apache.mesos.v1.Protos.Labels.getDefaultInstance() : labels_;
} else {
return labelsBuilder_.getMessage();
}
}
/**
*
* Labels are free-form key value pairs which are exposed through
* master and agent endpoints. Labels will not be interpreted or
* acted upon by Mesos itself. As opposed to the data field, labels
* will be kept in memory on master and agent processes. Therefore,
* labels should be used to tag executors with lightweight metadata.
* Labels should not contain duplicate key-value pairs.
*
*
* optional .mesos.v1.Labels labels = 14;
*/
public Builder setLabels(org.apache.mesos.v1.Protos.Labels value) {
if (labelsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
labels_ = value;
onChanged();
} else {
labelsBuilder_.setMessage(value);
}
bitField0_ |= 0x00000800;
return this;
}
/**
*
* Labels are free-form key value pairs which are exposed through
* master and agent endpoints. Labels will not be interpreted or
* acted upon by Mesos itself. As opposed to the data field, labels
* will be kept in memory on master and agent processes. Therefore,
* labels should be used to tag executors with lightweight metadata.
* Labels should not contain duplicate key-value pairs.
*
*
* optional .mesos.v1.Labels labels = 14;
*/
public Builder setLabels(
org.apache.mesos.v1.Protos.Labels.Builder builderForValue) {
if (labelsBuilder_ == null) {
labels_ = builderForValue.build();
onChanged();
} else {
labelsBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000800;
return this;
}
/**
*
* Labels are free-form key value pairs which are exposed through
* master and agent endpoints. Labels will not be interpreted or
* acted upon by Mesos itself. As opposed to the data field, labels
* will be kept in memory on master and agent processes. Therefore,
* labels should be used to tag executors with lightweight metadata.
* Labels should not contain duplicate key-value pairs.
*
*
* optional .mesos.v1.Labels labels = 14;
*/
public Builder mergeLabels(org.apache.mesos.v1.Protos.Labels value) {
if (labelsBuilder_ == null) {
if (((bitField0_ & 0x00000800) == 0x00000800) &&
labels_ != null &&
labels_ != org.apache.mesos.v1.Protos.Labels.getDefaultInstance()) {
labels_ =
org.apache.mesos.v1.Protos.Labels.newBuilder(labels_).mergeFrom(value).buildPartial();
} else {
labels_ = value;
}
onChanged();
} else {
labelsBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000800;
return this;
}
/**
*
* Labels are free-form key value pairs which are exposed through
* master and agent endpoints. Labels will not be interpreted or
* acted upon by Mesos itself. As opposed to the data field, labels
* will be kept in memory on master and agent processes. Therefore,
* labels should be used to tag executors with lightweight metadata.
* Labels should not contain duplicate key-value pairs.
*
*
* optional .mesos.v1.Labels labels = 14;
*/
public Builder clearLabels() {
if (labelsBuilder_ == null) {
labels_ = null;
onChanged();
} else {
labelsBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000800);
return this;
}
/**
*
* Labels are free-form key value pairs which are exposed through
* master and agent endpoints. Labels will not be interpreted or
* acted upon by Mesos itself. As opposed to the data field, labels
* will be kept in memory on master and agent processes. Therefore,
* labels should be used to tag executors with lightweight metadata.
* Labels should not contain duplicate key-value pairs.
*
*
* optional .mesos.v1.Labels labels = 14;
*/
public org.apache.mesos.v1.Protos.Labels.Builder getLabelsBuilder() {
bitField0_ |= 0x00000800;
onChanged();
return getLabelsFieldBuilder().getBuilder();
}
/**
*
* Labels are free-form key value pairs which are exposed through
* master and agent endpoints. Labels will not be interpreted or
* acted upon by Mesos itself. As opposed to the data field, labels
* will be kept in memory on master and agent processes. Therefore,
* labels should be used to tag executors with lightweight metadata.
* Labels should not contain duplicate key-value pairs.
*
*
* optional .mesos.v1.Labels labels = 14;
*/
public org.apache.mesos.v1.Protos.LabelsOrBuilder getLabelsOrBuilder() {
if (labelsBuilder_ != null) {
return labelsBuilder_.getMessageOrBuilder();
} else {
return labels_ == null ?
org.apache.mesos.v1.Protos.Labels.getDefaultInstance() : labels_;
}
}
/**
*
* Labels are free-form key value pairs which are exposed through
* master and agent endpoints. Labels will not be interpreted or
* acted upon by Mesos itself. As opposed to the data field, labels
* will be kept in memory on master and agent processes. Therefore,
* labels should be used to tag executors with lightweight metadata.
* Labels should not contain duplicate key-value pairs.
*
*
* optional .mesos.v1.Labels labels = 14;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.apache.mesos.v1.Protos.Labels, org.apache.mesos.v1.Protos.Labels.Builder, org.apache.mesos.v1.Protos.LabelsOrBuilder>
getLabelsFieldBuilder() {
if (labelsBuilder_ == null) {
labelsBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.apache.mesos.v1.Protos.Labels, org.apache.mesos.v1.Protos.Labels.Builder, org.apache.mesos.v1.Protos.LabelsOrBuilder>(
getLabels(),
getParentForChildren(),
isClean());
labels_ = null;
}
return labelsBuilder_;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:mesos.v1.ExecutorInfo)
}
// @@protoc_insertion_point(class_scope:mesos.v1.ExecutorInfo)
private static final org.apache.mesos.v1.Protos.ExecutorInfo DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.apache.mesos.v1.Protos.ExecutorInfo();
}
public static org.apache.mesos.v1.Protos.ExecutorInfo getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public ExecutorInfo parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ExecutorInfo(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.apache.mesos.v1.Protos.ExecutorInfo getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface DomainInfoOrBuilder extends
// @@protoc_insertion_point(interface_extends:mesos.v1.DomainInfo)
com.google.protobuf.MessageOrBuilder {
/**
* optional .mesos.v1.DomainInfo.FaultDomain fault_domain = 1;
*/
boolean hasFaultDomain();
/**
* optional .mesos.v1.DomainInfo.FaultDomain fault_domain = 1;
*/
org.apache.mesos.v1.Protos.DomainInfo.FaultDomain getFaultDomain();
/**
* optional .mesos.v1.DomainInfo.FaultDomain fault_domain = 1;
*/
org.apache.mesos.v1.Protos.DomainInfo.FaultDomainOrBuilder getFaultDomainOrBuilder();
}
/**
*
**
* Describes a domain. A domain is a collection of hosts that have
* similar characteristics. Mesos currently only supports "fault
* domains", which identify groups of hosts with similar failure
* characteristics.
* Frameworks can generally assume that network links between hosts in
* the same fault domain have lower latency, higher bandwidth, and better
* availability than network links between hosts in different domains.
* Schedulers may prefer to place network-intensive workloads in the
* same domain, as this may improve performance. Conversely, a single
* failure that affects a host in a domain may be more likely to
* affect other hosts in the same domain; hence, schedulers may prefer
* to place workloads that require high availability in multiple
* domains. (For example, all the hosts in a single rack might lose
* power or network connectivity simultaneously.)
* There are two kinds of fault domains: regions and zones. Regions
* offer the highest degree of fault isolation, but network latency
* between regions is typically high (typically >50 ms). Zones offer a
* modest degree of fault isolation along with reasonably low network
* latency (typically <10 ms).
* The mapping from fault domains to physical infrastructure is up to
* the operator to configure. In cloud environments, regions and zones
* can be mapped to the "region" and "availability zone" concepts
* exposed by most cloud providers, respectively. In on-premise
* deployments, regions and zones can be mapped to data centers and
* racks, respectively.
* Both masters and agents can be configured with domains. Frameworks
* can compare the domains of two hosts to determine if the hosts are
* in the same zone, in different zones in the same region, or in
* different regions. Note that all masters in a given Mesos cluster
* must be in the same region.
* Complex deployments may have additional levels of hierarchy: for example,
* multiple racks might be grouped together into "halls" and multiple DCs in
* the same geographical vicinity might be grouped together. As a convention,
* the recommended way to represent additional levels of hierarchy is via dot-
* separated labels in the existing zone and region fields. For example, the
* fact that racks "abc" and "def" are in the same hall might be represented
* using the zone names "rack-abc.hall-1" and "rack-def.hall-1", for example.
* Software that is not aware of this additional structure will compare the
* zone names for equality- hence, the two zones will be treated as different
* (unrelated) zones. Software that is "hall-aware" can inspect the zone names
* and make use of the additional hierarchy.
*
*
* Protobuf type {@code mesos.v1.DomainInfo}
*/
public static final class DomainInfo extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:mesos.v1.DomainInfo)
DomainInfoOrBuilder {
private static final long serialVersionUID = 0L;
// Use DomainInfo.newBuilder() to construct.
private DomainInfo(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private DomainInfo() {
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private DomainInfo(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.Builder subBuilder = null;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
subBuilder = faultDomain_.toBuilder();
}
faultDomain_ = input.readMessage(org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(faultDomain_);
faultDomain_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000001;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_DomainInfo_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_DomainInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.mesos.v1.Protos.DomainInfo.class, org.apache.mesos.v1.Protos.DomainInfo.Builder.class);
}
public interface FaultDomainOrBuilder extends
// @@protoc_insertion_point(interface_extends:mesos.v1.DomainInfo.FaultDomain)
com.google.protobuf.MessageOrBuilder {
/**
* required .mesos.v1.DomainInfo.FaultDomain.RegionInfo region = 1;
*/
boolean hasRegion();
/**
* required .mesos.v1.DomainInfo.FaultDomain.RegionInfo region = 1;
*/
org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.RegionInfo getRegion();
/**
* required .mesos.v1.DomainInfo.FaultDomain.RegionInfo region = 1;
*/
org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.RegionInfoOrBuilder getRegionOrBuilder();
/**
* required .mesos.v1.DomainInfo.FaultDomain.ZoneInfo zone = 2;
*/
boolean hasZone();
/**
* required .mesos.v1.DomainInfo.FaultDomain.ZoneInfo zone = 2;
*/
org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.ZoneInfo getZone();
/**
* required .mesos.v1.DomainInfo.FaultDomain.ZoneInfo zone = 2;
*/
org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.ZoneInfoOrBuilder getZoneOrBuilder();
}
/**
* Protobuf type {@code mesos.v1.DomainInfo.FaultDomain}
*/
public static final class FaultDomain extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:mesos.v1.DomainInfo.FaultDomain)
FaultDomainOrBuilder {
private static final long serialVersionUID = 0L;
// Use FaultDomain.newBuilder() to construct.
private FaultDomain(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private FaultDomain() {
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private FaultDomain(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.RegionInfo.Builder subBuilder = null;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
subBuilder = region_.toBuilder();
}
region_ = input.readMessage(org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.RegionInfo.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(region_);
region_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000001;
break;
}
case 18: {
org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.ZoneInfo.Builder subBuilder = null;
if (((bitField0_ & 0x00000002) == 0x00000002)) {
subBuilder = zone_.toBuilder();
}
zone_ = input.readMessage(org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.ZoneInfo.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(zone_);
zone_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000002;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_DomainInfo_FaultDomain_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_DomainInfo_FaultDomain_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.class, org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.Builder.class);
}
public interface RegionInfoOrBuilder extends
// @@protoc_insertion_point(interface_extends:mesos.v1.DomainInfo.FaultDomain.RegionInfo)
com.google.protobuf.MessageOrBuilder {
/**
* required string name = 1;
*/
boolean hasName();
/**
* required string name = 1;
*/
java.lang.String getName();
/**
* required string name = 1;
*/
com.google.protobuf.ByteString
getNameBytes();
}
/**
* Protobuf type {@code mesos.v1.DomainInfo.FaultDomain.RegionInfo}
*/
public static final class RegionInfo extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:mesos.v1.DomainInfo.FaultDomain.RegionInfo)
RegionInfoOrBuilder {
private static final long serialVersionUID = 0L;
// Use RegionInfo.newBuilder() to construct.
private RegionInfo(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private RegionInfo() {
name_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private RegionInfo(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000001;
name_ = bs;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_DomainInfo_FaultDomain_RegionInfo_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_DomainInfo_FaultDomain_RegionInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.RegionInfo.class, org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.RegionInfo.Builder.class);
}
private int bitField0_;
public static final int NAME_FIELD_NUMBER = 1;
private volatile java.lang.Object name_;
/**
* required string name = 1;
*/
public boolean hasName() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required string name = 1;
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
name_ = s;
}
return s;
}
}
/**
* required string name = 1;
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasName()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, name_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, name_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.RegionInfo)) {
return super.equals(obj);
}
org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.RegionInfo other = (org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.RegionInfo) obj;
boolean result = true;
result = result && (hasName() == other.hasName());
if (hasName()) {
result = result && getName()
.equals(other.getName());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasName()) {
hash = (37 * hash) + NAME_FIELD_NUMBER;
hash = (53 * hash) + getName().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.RegionInfo parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.RegionInfo parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.RegionInfo parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.RegionInfo parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.RegionInfo parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.RegionInfo parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.RegionInfo parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.RegionInfo parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.RegionInfo parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.RegionInfo parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.RegionInfo parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.RegionInfo parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.RegionInfo prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code mesos.v1.DomainInfo.FaultDomain.RegionInfo}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:mesos.v1.DomainInfo.FaultDomain.RegionInfo)
org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.RegionInfoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_DomainInfo_FaultDomain_RegionInfo_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_DomainInfo_FaultDomain_RegionInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.RegionInfo.class, org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.RegionInfo.Builder.class);
}
// Construct using org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.RegionInfo.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
name_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_DomainInfo_FaultDomain_RegionInfo_descriptor;
}
public org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.RegionInfo getDefaultInstanceForType() {
return org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.RegionInfo.getDefaultInstance();
}
public org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.RegionInfo build() {
org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.RegionInfo result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.RegionInfo buildPartial() {
org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.RegionInfo result = new org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.RegionInfo(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.name_ = name_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.RegionInfo) {
return mergeFrom((org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.RegionInfo)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.RegionInfo other) {
if (other == org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.RegionInfo.getDefaultInstance()) return this;
if (other.hasName()) {
bitField0_ |= 0x00000001;
name_ = other.name_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
if (!hasName()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.RegionInfo parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.RegionInfo) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object name_ = "";
/**
* required string name = 1;
*/
public boolean hasName() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required string name = 1;
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
name_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* required string name = 1;
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* required string name = 1;
*/
public Builder setName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
name_ = value;
onChanged();
return this;
}
/**
* required string name = 1;
*/
public Builder clearName() {
bitField0_ = (bitField0_ & ~0x00000001);
name_ = getDefaultInstance().getName();
onChanged();
return this;
}
/**
* required string name = 1;
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
name_ = value;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:mesos.v1.DomainInfo.FaultDomain.RegionInfo)
}
// @@protoc_insertion_point(class_scope:mesos.v1.DomainInfo.FaultDomain.RegionInfo)
private static final org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.RegionInfo DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.RegionInfo();
}
public static org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.RegionInfo getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public RegionInfo parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new RegionInfo(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.RegionInfo getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ZoneInfoOrBuilder extends
// @@protoc_insertion_point(interface_extends:mesos.v1.DomainInfo.FaultDomain.ZoneInfo)
com.google.protobuf.MessageOrBuilder {
/**
* required string name = 1;
*/
boolean hasName();
/**
* required string name = 1;
*/
java.lang.String getName();
/**
* required string name = 1;
*/
com.google.protobuf.ByteString
getNameBytes();
}
/**
* Protobuf type {@code mesos.v1.DomainInfo.FaultDomain.ZoneInfo}
*/
public static final class ZoneInfo extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:mesos.v1.DomainInfo.FaultDomain.ZoneInfo)
ZoneInfoOrBuilder {
private static final long serialVersionUID = 0L;
// Use ZoneInfo.newBuilder() to construct.
private ZoneInfo(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ZoneInfo() {
name_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ZoneInfo(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000001;
name_ = bs;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_DomainInfo_FaultDomain_ZoneInfo_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_DomainInfo_FaultDomain_ZoneInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.ZoneInfo.class, org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.ZoneInfo.Builder.class);
}
private int bitField0_;
public static final int NAME_FIELD_NUMBER = 1;
private volatile java.lang.Object name_;
/**
* required string name = 1;
*/
public boolean hasName() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required string name = 1;
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
name_ = s;
}
return s;
}
}
/**
* required string name = 1;
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasName()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, name_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, name_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.ZoneInfo)) {
return super.equals(obj);
}
org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.ZoneInfo other = (org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.ZoneInfo) obj;
boolean result = true;
result = result && (hasName() == other.hasName());
if (hasName()) {
result = result && getName()
.equals(other.getName());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasName()) {
hash = (37 * hash) + NAME_FIELD_NUMBER;
hash = (53 * hash) + getName().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.ZoneInfo parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.ZoneInfo parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.ZoneInfo parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.ZoneInfo parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.ZoneInfo parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.ZoneInfo parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.ZoneInfo parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.ZoneInfo parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.ZoneInfo parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.ZoneInfo parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.ZoneInfo parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.ZoneInfo parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.ZoneInfo prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code mesos.v1.DomainInfo.FaultDomain.ZoneInfo}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:mesos.v1.DomainInfo.FaultDomain.ZoneInfo)
org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.ZoneInfoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_DomainInfo_FaultDomain_ZoneInfo_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_DomainInfo_FaultDomain_ZoneInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.ZoneInfo.class, org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.ZoneInfo.Builder.class);
}
// Construct using org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.ZoneInfo.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
name_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_DomainInfo_FaultDomain_ZoneInfo_descriptor;
}
public org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.ZoneInfo getDefaultInstanceForType() {
return org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.ZoneInfo.getDefaultInstance();
}
public org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.ZoneInfo build() {
org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.ZoneInfo result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.ZoneInfo buildPartial() {
org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.ZoneInfo result = new org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.ZoneInfo(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.name_ = name_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.ZoneInfo) {
return mergeFrom((org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.ZoneInfo)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.ZoneInfo other) {
if (other == org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.ZoneInfo.getDefaultInstance()) return this;
if (other.hasName()) {
bitField0_ |= 0x00000001;
name_ = other.name_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
if (!hasName()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.ZoneInfo parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.ZoneInfo) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object name_ = "";
/**
* required string name = 1;
*/
public boolean hasName() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required string name = 1;
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
name_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* required string name = 1;
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* required string name = 1;
*/
public Builder setName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
name_ = value;
onChanged();
return this;
}
/**
* required string name = 1;
*/
public Builder clearName() {
bitField0_ = (bitField0_ & ~0x00000001);
name_ = getDefaultInstance().getName();
onChanged();
return this;
}
/**
* required string name = 1;
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
name_ = value;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:mesos.v1.DomainInfo.FaultDomain.ZoneInfo)
}
// @@protoc_insertion_point(class_scope:mesos.v1.DomainInfo.FaultDomain.ZoneInfo)
private static final org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.ZoneInfo DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.ZoneInfo();
}
public static org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.ZoneInfo getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public ZoneInfo parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ZoneInfo(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.ZoneInfo getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private int bitField0_;
public static final int REGION_FIELD_NUMBER = 1;
private org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.RegionInfo region_;
/**
* required .mesos.v1.DomainInfo.FaultDomain.RegionInfo region = 1;
*/
public boolean hasRegion() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required .mesos.v1.DomainInfo.FaultDomain.RegionInfo region = 1;
*/
public org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.RegionInfo getRegion() {
return region_ == null ? org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.RegionInfo.getDefaultInstance() : region_;
}
/**
* required .mesos.v1.DomainInfo.FaultDomain.RegionInfo region = 1;
*/
public org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.RegionInfoOrBuilder getRegionOrBuilder() {
return region_ == null ? org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.RegionInfo.getDefaultInstance() : region_;
}
public static final int ZONE_FIELD_NUMBER = 2;
private org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.ZoneInfo zone_;
/**
* required .mesos.v1.DomainInfo.FaultDomain.ZoneInfo zone = 2;
*/
public boolean hasZone() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required .mesos.v1.DomainInfo.FaultDomain.ZoneInfo zone = 2;
*/
public org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.ZoneInfo getZone() {
return zone_ == null ? org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.ZoneInfo.getDefaultInstance() : zone_;
}
/**
* required .mesos.v1.DomainInfo.FaultDomain.ZoneInfo zone = 2;
*/
public org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.ZoneInfoOrBuilder getZoneOrBuilder() {
return zone_ == null ? org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.ZoneInfo.getDefaultInstance() : zone_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasRegion()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasZone()) {
memoizedIsInitialized = 0;
return false;
}
if (!getRegion().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
if (!getZone().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeMessage(1, getRegion());
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeMessage(2, getZone());
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getRegion());
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getZone());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.mesos.v1.Protos.DomainInfo.FaultDomain)) {
return super.equals(obj);
}
org.apache.mesos.v1.Protos.DomainInfo.FaultDomain other = (org.apache.mesos.v1.Protos.DomainInfo.FaultDomain) obj;
boolean result = true;
result = result && (hasRegion() == other.hasRegion());
if (hasRegion()) {
result = result && getRegion()
.equals(other.getRegion());
}
result = result && (hasZone() == other.hasZone());
if (hasZone()) {
result = result && getZone()
.equals(other.getZone());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasRegion()) {
hash = (37 * hash) + REGION_FIELD_NUMBER;
hash = (53 * hash) + getRegion().hashCode();
}
if (hasZone()) {
hash = (37 * hash) + ZONE_FIELD_NUMBER;
hash = (53 * hash) + getZone().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.mesos.v1.Protos.DomainInfo.FaultDomain parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.DomainInfo.FaultDomain parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.DomainInfo.FaultDomain parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.DomainInfo.FaultDomain parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.DomainInfo.FaultDomain parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.DomainInfo.FaultDomain parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.DomainInfo.FaultDomain parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.DomainInfo.FaultDomain parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.DomainInfo.FaultDomain parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.DomainInfo.FaultDomain parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.DomainInfo.FaultDomain parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.DomainInfo.FaultDomain parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.apache.mesos.v1.Protos.DomainInfo.FaultDomain prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code mesos.v1.DomainInfo.FaultDomain}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:mesos.v1.DomainInfo.FaultDomain)
org.apache.mesos.v1.Protos.DomainInfo.FaultDomainOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_DomainInfo_FaultDomain_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_DomainInfo_FaultDomain_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.class, org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.Builder.class);
}
// Construct using org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getRegionFieldBuilder();
getZoneFieldBuilder();
}
}
public Builder clear() {
super.clear();
if (regionBuilder_ == null) {
region_ = null;
} else {
regionBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
if (zoneBuilder_ == null) {
zone_ = null;
} else {
zoneBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_DomainInfo_FaultDomain_descriptor;
}
public org.apache.mesos.v1.Protos.DomainInfo.FaultDomain getDefaultInstanceForType() {
return org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.getDefaultInstance();
}
public org.apache.mesos.v1.Protos.DomainInfo.FaultDomain build() {
org.apache.mesos.v1.Protos.DomainInfo.FaultDomain result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.apache.mesos.v1.Protos.DomainInfo.FaultDomain buildPartial() {
org.apache.mesos.v1.Protos.DomainInfo.FaultDomain result = new org.apache.mesos.v1.Protos.DomainInfo.FaultDomain(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
if (regionBuilder_ == null) {
result.region_ = region_;
} else {
result.region_ = regionBuilder_.build();
}
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
if (zoneBuilder_ == null) {
result.zone_ = zone_;
} else {
result.zone_ = zoneBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.apache.mesos.v1.Protos.DomainInfo.FaultDomain) {
return mergeFrom((org.apache.mesos.v1.Protos.DomainInfo.FaultDomain)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.mesos.v1.Protos.DomainInfo.FaultDomain other) {
if (other == org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.getDefaultInstance()) return this;
if (other.hasRegion()) {
mergeRegion(other.getRegion());
}
if (other.hasZone()) {
mergeZone(other.getZone());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
if (!hasRegion()) {
return false;
}
if (!hasZone()) {
return false;
}
if (!getRegion().isInitialized()) {
return false;
}
if (!getZone().isInitialized()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.mesos.v1.Protos.DomainInfo.FaultDomain parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.mesos.v1.Protos.DomainInfo.FaultDomain) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.RegionInfo region_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.RegionInfo, org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.RegionInfo.Builder, org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.RegionInfoOrBuilder> regionBuilder_;
/**
* required .mesos.v1.DomainInfo.FaultDomain.RegionInfo region = 1;
*/
public boolean hasRegion() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required .mesos.v1.DomainInfo.FaultDomain.RegionInfo region = 1;
*/
public org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.RegionInfo getRegion() {
if (regionBuilder_ == null) {
return region_ == null ? org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.RegionInfo.getDefaultInstance() : region_;
} else {
return regionBuilder_.getMessage();
}
}
/**
* required .mesos.v1.DomainInfo.FaultDomain.RegionInfo region = 1;
*/
public Builder setRegion(org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.RegionInfo value) {
if (regionBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
region_ = value;
onChanged();
} else {
regionBuilder_.setMessage(value);
}
bitField0_ |= 0x00000001;
return this;
}
/**
* required .mesos.v1.DomainInfo.FaultDomain.RegionInfo region = 1;
*/
public Builder setRegion(
org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.RegionInfo.Builder builderForValue) {
if (regionBuilder_ == null) {
region_ = builderForValue.build();
onChanged();
} else {
regionBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000001;
return this;
}
/**
* required .mesos.v1.DomainInfo.FaultDomain.RegionInfo region = 1;
*/
public Builder mergeRegion(org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.RegionInfo value) {
if (regionBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001) &&
region_ != null &&
region_ != org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.RegionInfo.getDefaultInstance()) {
region_ =
org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.RegionInfo.newBuilder(region_).mergeFrom(value).buildPartial();
} else {
region_ = value;
}
onChanged();
} else {
regionBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000001;
return this;
}
/**
* required .mesos.v1.DomainInfo.FaultDomain.RegionInfo region = 1;
*/
public Builder clearRegion() {
if (regionBuilder_ == null) {
region_ = null;
onChanged();
} else {
regionBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
/**
* required .mesos.v1.DomainInfo.FaultDomain.RegionInfo region = 1;
*/
public org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.RegionInfo.Builder getRegionBuilder() {
bitField0_ |= 0x00000001;
onChanged();
return getRegionFieldBuilder().getBuilder();
}
/**
* required .mesos.v1.DomainInfo.FaultDomain.RegionInfo region = 1;
*/
public org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.RegionInfoOrBuilder getRegionOrBuilder() {
if (regionBuilder_ != null) {
return regionBuilder_.getMessageOrBuilder();
} else {
return region_ == null ?
org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.RegionInfo.getDefaultInstance() : region_;
}
}
/**
* required .mesos.v1.DomainInfo.FaultDomain.RegionInfo region = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.RegionInfo, org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.RegionInfo.Builder, org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.RegionInfoOrBuilder>
getRegionFieldBuilder() {
if (regionBuilder_ == null) {
regionBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.RegionInfo, org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.RegionInfo.Builder, org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.RegionInfoOrBuilder>(
getRegion(),
getParentForChildren(),
isClean());
region_ = null;
}
return regionBuilder_;
}
private org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.ZoneInfo zone_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.ZoneInfo, org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.ZoneInfo.Builder, org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.ZoneInfoOrBuilder> zoneBuilder_;
/**
* required .mesos.v1.DomainInfo.FaultDomain.ZoneInfo zone = 2;
*/
public boolean hasZone() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required .mesos.v1.DomainInfo.FaultDomain.ZoneInfo zone = 2;
*/
public org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.ZoneInfo getZone() {
if (zoneBuilder_ == null) {
return zone_ == null ? org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.ZoneInfo.getDefaultInstance() : zone_;
} else {
return zoneBuilder_.getMessage();
}
}
/**
* required .mesos.v1.DomainInfo.FaultDomain.ZoneInfo zone = 2;
*/
public Builder setZone(org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.ZoneInfo value) {
if (zoneBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
zone_ = value;
onChanged();
} else {
zoneBuilder_.setMessage(value);
}
bitField0_ |= 0x00000002;
return this;
}
/**
* required .mesos.v1.DomainInfo.FaultDomain.ZoneInfo zone = 2;
*/
public Builder setZone(
org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.ZoneInfo.Builder builderForValue) {
if (zoneBuilder_ == null) {
zone_ = builderForValue.build();
onChanged();
} else {
zoneBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000002;
return this;
}
/**
* required .mesos.v1.DomainInfo.FaultDomain.ZoneInfo zone = 2;
*/
public Builder mergeZone(org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.ZoneInfo value) {
if (zoneBuilder_ == null) {
if (((bitField0_ & 0x00000002) == 0x00000002) &&
zone_ != null &&
zone_ != org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.ZoneInfo.getDefaultInstance()) {
zone_ =
org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.ZoneInfo.newBuilder(zone_).mergeFrom(value).buildPartial();
} else {
zone_ = value;
}
onChanged();
} else {
zoneBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000002;
return this;
}
/**
* required .mesos.v1.DomainInfo.FaultDomain.ZoneInfo zone = 2;
*/
public Builder clearZone() {
if (zoneBuilder_ == null) {
zone_ = null;
onChanged();
} else {
zoneBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
/**
* required .mesos.v1.DomainInfo.FaultDomain.ZoneInfo zone = 2;
*/
public org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.ZoneInfo.Builder getZoneBuilder() {
bitField0_ |= 0x00000002;
onChanged();
return getZoneFieldBuilder().getBuilder();
}
/**
* required .mesos.v1.DomainInfo.FaultDomain.ZoneInfo zone = 2;
*/
public org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.ZoneInfoOrBuilder getZoneOrBuilder() {
if (zoneBuilder_ != null) {
return zoneBuilder_.getMessageOrBuilder();
} else {
return zone_ == null ?
org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.ZoneInfo.getDefaultInstance() : zone_;
}
}
/**
* required .mesos.v1.DomainInfo.FaultDomain.ZoneInfo zone = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.ZoneInfo, org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.ZoneInfo.Builder, org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.ZoneInfoOrBuilder>
getZoneFieldBuilder() {
if (zoneBuilder_ == null) {
zoneBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.ZoneInfo, org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.ZoneInfo.Builder, org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.ZoneInfoOrBuilder>(
getZone(),
getParentForChildren(),
isClean());
zone_ = null;
}
return zoneBuilder_;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:mesos.v1.DomainInfo.FaultDomain)
}
// @@protoc_insertion_point(class_scope:mesos.v1.DomainInfo.FaultDomain)
private static final org.apache.mesos.v1.Protos.DomainInfo.FaultDomain DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.apache.mesos.v1.Protos.DomainInfo.FaultDomain();
}
public static org.apache.mesos.v1.Protos.DomainInfo.FaultDomain getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public FaultDomain parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new FaultDomain(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.apache.mesos.v1.Protos.DomainInfo.FaultDomain getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private int bitField0_;
public static final int FAULT_DOMAIN_FIELD_NUMBER = 1;
private org.apache.mesos.v1.Protos.DomainInfo.FaultDomain faultDomain_;
/**
* optional .mesos.v1.DomainInfo.FaultDomain fault_domain = 1;
*/
public boolean hasFaultDomain() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional .mesos.v1.DomainInfo.FaultDomain fault_domain = 1;
*/
public org.apache.mesos.v1.Protos.DomainInfo.FaultDomain getFaultDomain() {
return faultDomain_ == null ? org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.getDefaultInstance() : faultDomain_;
}
/**
* optional .mesos.v1.DomainInfo.FaultDomain fault_domain = 1;
*/
public org.apache.mesos.v1.Protos.DomainInfo.FaultDomainOrBuilder getFaultDomainOrBuilder() {
return faultDomain_ == null ? org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.getDefaultInstance() : faultDomain_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (hasFaultDomain()) {
if (!getFaultDomain().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeMessage(1, getFaultDomain());
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getFaultDomain());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.mesos.v1.Protos.DomainInfo)) {
return super.equals(obj);
}
org.apache.mesos.v1.Protos.DomainInfo other = (org.apache.mesos.v1.Protos.DomainInfo) obj;
boolean result = true;
result = result && (hasFaultDomain() == other.hasFaultDomain());
if (hasFaultDomain()) {
result = result && getFaultDomain()
.equals(other.getFaultDomain());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasFaultDomain()) {
hash = (37 * hash) + FAULT_DOMAIN_FIELD_NUMBER;
hash = (53 * hash) + getFaultDomain().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.mesos.v1.Protos.DomainInfo parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.DomainInfo parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.DomainInfo parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.DomainInfo parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.DomainInfo parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.DomainInfo parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.DomainInfo parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.DomainInfo parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.DomainInfo parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.DomainInfo parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.DomainInfo parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.DomainInfo parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.apache.mesos.v1.Protos.DomainInfo prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
**
* Describes a domain. A domain is a collection of hosts that have
* similar characteristics. Mesos currently only supports "fault
* domains", which identify groups of hosts with similar failure
* characteristics.
* Frameworks can generally assume that network links between hosts in
* the same fault domain have lower latency, higher bandwidth, and better
* availability than network links between hosts in different domains.
* Schedulers may prefer to place network-intensive workloads in the
* same domain, as this may improve performance. Conversely, a single
* failure that affects a host in a domain may be more likely to
* affect other hosts in the same domain; hence, schedulers may prefer
* to place workloads that require high availability in multiple
* domains. (For example, all the hosts in a single rack might lose
* power or network connectivity simultaneously.)
* There are two kinds of fault domains: regions and zones. Regions
* offer the highest degree of fault isolation, but network latency
* between regions is typically high (typically >50 ms). Zones offer a
* modest degree of fault isolation along with reasonably low network
* latency (typically <10 ms).
* The mapping from fault domains to physical infrastructure is up to
* the operator to configure. In cloud environments, regions and zones
* can be mapped to the "region" and "availability zone" concepts
* exposed by most cloud providers, respectively. In on-premise
* deployments, regions and zones can be mapped to data centers and
* racks, respectively.
* Both masters and agents can be configured with domains. Frameworks
* can compare the domains of two hosts to determine if the hosts are
* in the same zone, in different zones in the same region, or in
* different regions. Note that all masters in a given Mesos cluster
* must be in the same region.
* Complex deployments may have additional levels of hierarchy: for example,
* multiple racks might be grouped together into "halls" and multiple DCs in
* the same geographical vicinity might be grouped together. As a convention,
* the recommended way to represent additional levels of hierarchy is via dot-
* separated labels in the existing zone and region fields. For example, the
* fact that racks "abc" and "def" are in the same hall might be represented
* using the zone names "rack-abc.hall-1" and "rack-def.hall-1", for example.
* Software that is not aware of this additional structure will compare the
* zone names for equality- hence, the two zones will be treated as different
* (unrelated) zones. Software that is "hall-aware" can inspect the zone names
* and make use of the additional hierarchy.
*
*
* Protobuf type {@code mesos.v1.DomainInfo}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:mesos.v1.DomainInfo)
org.apache.mesos.v1.Protos.DomainInfoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_DomainInfo_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_DomainInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.mesos.v1.Protos.DomainInfo.class, org.apache.mesos.v1.Protos.DomainInfo.Builder.class);
}
// Construct using org.apache.mesos.v1.Protos.DomainInfo.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getFaultDomainFieldBuilder();
}
}
public Builder clear() {
super.clear();
if (faultDomainBuilder_ == null) {
faultDomain_ = null;
} else {
faultDomainBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_DomainInfo_descriptor;
}
public org.apache.mesos.v1.Protos.DomainInfo getDefaultInstanceForType() {
return org.apache.mesos.v1.Protos.DomainInfo.getDefaultInstance();
}
public org.apache.mesos.v1.Protos.DomainInfo build() {
org.apache.mesos.v1.Protos.DomainInfo result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.apache.mesos.v1.Protos.DomainInfo buildPartial() {
org.apache.mesos.v1.Protos.DomainInfo result = new org.apache.mesos.v1.Protos.DomainInfo(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
if (faultDomainBuilder_ == null) {
result.faultDomain_ = faultDomain_;
} else {
result.faultDomain_ = faultDomainBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.apache.mesos.v1.Protos.DomainInfo) {
return mergeFrom((org.apache.mesos.v1.Protos.DomainInfo)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.mesos.v1.Protos.DomainInfo other) {
if (other == org.apache.mesos.v1.Protos.DomainInfo.getDefaultInstance()) return this;
if (other.hasFaultDomain()) {
mergeFaultDomain(other.getFaultDomain());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
if (hasFaultDomain()) {
if (!getFaultDomain().isInitialized()) {
return false;
}
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.mesos.v1.Protos.DomainInfo parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.mesos.v1.Protos.DomainInfo) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private org.apache.mesos.v1.Protos.DomainInfo.FaultDomain faultDomain_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
org.apache.mesos.v1.Protos.DomainInfo.FaultDomain, org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.Builder, org.apache.mesos.v1.Protos.DomainInfo.FaultDomainOrBuilder> faultDomainBuilder_;
/**
* optional .mesos.v1.DomainInfo.FaultDomain fault_domain = 1;
*/
public boolean hasFaultDomain() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional .mesos.v1.DomainInfo.FaultDomain fault_domain = 1;
*/
public org.apache.mesos.v1.Protos.DomainInfo.FaultDomain getFaultDomain() {
if (faultDomainBuilder_ == null) {
return faultDomain_ == null ? org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.getDefaultInstance() : faultDomain_;
} else {
return faultDomainBuilder_.getMessage();
}
}
/**
* optional .mesos.v1.DomainInfo.FaultDomain fault_domain = 1;
*/
public Builder setFaultDomain(org.apache.mesos.v1.Protos.DomainInfo.FaultDomain value) {
if (faultDomainBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
faultDomain_ = value;
onChanged();
} else {
faultDomainBuilder_.setMessage(value);
}
bitField0_ |= 0x00000001;
return this;
}
/**
* optional .mesos.v1.DomainInfo.FaultDomain fault_domain = 1;
*/
public Builder setFaultDomain(
org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.Builder builderForValue) {
if (faultDomainBuilder_ == null) {
faultDomain_ = builderForValue.build();
onChanged();
} else {
faultDomainBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000001;
return this;
}
/**
* optional .mesos.v1.DomainInfo.FaultDomain fault_domain = 1;
*/
public Builder mergeFaultDomain(org.apache.mesos.v1.Protos.DomainInfo.FaultDomain value) {
if (faultDomainBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001) &&
faultDomain_ != null &&
faultDomain_ != org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.getDefaultInstance()) {
faultDomain_ =
org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.newBuilder(faultDomain_).mergeFrom(value).buildPartial();
} else {
faultDomain_ = value;
}
onChanged();
} else {
faultDomainBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000001;
return this;
}
/**
* optional .mesos.v1.DomainInfo.FaultDomain fault_domain = 1;
*/
public Builder clearFaultDomain() {
if (faultDomainBuilder_ == null) {
faultDomain_ = null;
onChanged();
} else {
faultDomainBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
/**
* optional .mesos.v1.DomainInfo.FaultDomain fault_domain = 1;
*/
public org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.Builder getFaultDomainBuilder() {
bitField0_ |= 0x00000001;
onChanged();
return getFaultDomainFieldBuilder().getBuilder();
}
/**
* optional .mesos.v1.DomainInfo.FaultDomain fault_domain = 1;
*/
public org.apache.mesos.v1.Protos.DomainInfo.FaultDomainOrBuilder getFaultDomainOrBuilder() {
if (faultDomainBuilder_ != null) {
return faultDomainBuilder_.getMessageOrBuilder();
} else {
return faultDomain_ == null ?
org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.getDefaultInstance() : faultDomain_;
}
}
/**
* optional .mesos.v1.DomainInfo.FaultDomain fault_domain = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.apache.mesos.v1.Protos.DomainInfo.FaultDomain, org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.Builder, org.apache.mesos.v1.Protos.DomainInfo.FaultDomainOrBuilder>
getFaultDomainFieldBuilder() {
if (faultDomainBuilder_ == null) {
faultDomainBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.apache.mesos.v1.Protos.DomainInfo.FaultDomain, org.apache.mesos.v1.Protos.DomainInfo.FaultDomain.Builder, org.apache.mesos.v1.Protos.DomainInfo.FaultDomainOrBuilder>(
getFaultDomain(),
getParentForChildren(),
isClean());
faultDomain_ = null;
}
return faultDomainBuilder_;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:mesos.v1.DomainInfo)
}
// @@protoc_insertion_point(class_scope:mesos.v1.DomainInfo)
private static final org.apache.mesos.v1.Protos.DomainInfo DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.apache.mesos.v1.Protos.DomainInfo();
}
public static org.apache.mesos.v1.Protos.DomainInfo getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public DomainInfo parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new DomainInfo(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.apache.mesos.v1.Protos.DomainInfo getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface MasterInfoOrBuilder extends
// @@protoc_insertion_point(interface_extends:mesos.v1.MasterInfo)
com.google.protobuf.MessageOrBuilder {
/**
* required string id = 1;
*/
boolean hasId();
/**
* required string id = 1;
*/
java.lang.String getId();
/**
* required string id = 1;
*/
com.google.protobuf.ByteString
getIdBytes();
/**
*
* The IP address (only IPv4) as a packed 4-bytes integer,
* stored in network order. Deprecated, use `address.ip` instead.
*
*
* required uint32 ip = 2;
*/
boolean hasIp();
/**
*
* The IP address (only IPv4) as a packed 4-bytes integer,
* stored in network order. Deprecated, use `address.ip` instead.
*
*
* required uint32 ip = 2;
*/
int getIp();
/**
*
* The TCP port the Master is listening on for incoming
* HTTP requests; deprecated, use `address.port` instead.
*
*
* required uint32 port = 3 [default = 5050];
*/
boolean hasPort();
/**
*
* The TCP port the Master is listening on for incoming
* HTTP requests; deprecated, use `address.port` instead.
*
*
* required uint32 port = 3 [default = 5050];
*/
int getPort();
/**
*
* In the default implementation, this will contain information
* about both the IP address, port and Master name; it should really
* not be relied upon by external tooling/frameworks and be
* considered an "internal" implementation field.
*
*
* optional string pid = 4;
*/
boolean hasPid();
/**
*
* In the default implementation, this will contain information
* about both the IP address, port and Master name; it should really
* not be relied upon by external tooling/frameworks and be
* considered an "internal" implementation field.
*
*
* optional string pid = 4;
*/
java.lang.String getPid();
/**
*
* In the default implementation, this will contain information
* about both the IP address, port and Master name; it should really
* not be relied upon by external tooling/frameworks and be
* considered an "internal" implementation field.
*
*
* optional string pid = 4;
*/
com.google.protobuf.ByteString
getPidBytes();
/**
*
* The server's hostname, if available; it may be unreliable
* in environments where the DNS configuration does not resolve
* internal hostnames (eg, some public cloud providers).
* Deprecated, use `address.hostname` instead.
*
*
* optional string hostname = 5;
*/
boolean hasHostname();
/**
*
* The server's hostname, if available; it may be unreliable
* in environments where the DNS configuration does not resolve
* internal hostnames (eg, some public cloud providers).
* Deprecated, use `address.hostname` instead.
*
*
* optional string hostname = 5;
*/
java.lang.String getHostname();
/**
*
* The server's hostname, if available; it may be unreliable
* in environments where the DNS configuration does not resolve
* internal hostnames (eg, some public cloud providers).
* Deprecated, use `address.hostname` instead.
*
*
* optional string hostname = 5;
*/
com.google.protobuf.ByteString
getHostnameBytes();
/**
*
* The running Master version, as a string; taken from the
* generated "master/version.hpp".
*
*
* optional string version = 6;
*/
boolean hasVersion();
/**
*
* The running Master version, as a string; taken from the
* generated "master/version.hpp".
*
*
* optional string version = 6;
*/
java.lang.String getVersion();
/**
*
* The running Master version, as a string; taken from the
* generated "master/version.hpp".
*
*
* optional string version = 6;
*/
com.google.protobuf.ByteString
getVersionBytes();
/**
*
* The full IP address (supports both IPv4 and IPv6 formats)
* and supersedes the use of `ip`, `port` and `hostname`.
* Since Mesos 0.24.
*
*
* optional .mesos.v1.Address address = 7;
*/
boolean hasAddress();
/**
*
* The full IP address (supports both IPv4 and IPv6 formats)
* and supersedes the use of `ip`, `port` and `hostname`.
* Since Mesos 0.24.
*
*
* optional .mesos.v1.Address address = 7;
*/
org.apache.mesos.v1.Protos.Address getAddress();
/**
*
* The full IP address (supports both IPv4 and IPv6 formats)
* and supersedes the use of `ip`, `port` and `hostname`.
* Since Mesos 0.24.
*
*
* optional .mesos.v1.Address address = 7;
*/
org.apache.mesos.v1.Protos.AddressOrBuilder getAddressOrBuilder();
/**
*
* The domain that this master belongs to. All masters in a Mesos
* cluster should belong to the same region.
*
*
* optional .mesos.v1.DomainInfo domain = 8;
*/
boolean hasDomain();
/**
*
* The domain that this master belongs to. All masters in a Mesos
* cluster should belong to the same region.
*
*
* optional .mesos.v1.DomainInfo domain = 8;
*/
org.apache.mesos.v1.Protos.DomainInfo getDomain();
/**
*
* The domain that this master belongs to. All masters in a Mesos
* cluster should belong to the same region.
*
*
* optional .mesos.v1.DomainInfo domain = 8;
*/
org.apache.mesos.v1.Protos.DomainInfoOrBuilder getDomainOrBuilder();
/**
* repeated .mesos.v1.MasterInfo.Capability capabilities = 9;
*/
java.util.List
getCapabilitiesList();
/**
* repeated .mesos.v1.MasterInfo.Capability capabilities = 9;
*/
org.apache.mesos.v1.Protos.MasterInfo.Capability getCapabilities(int index);
/**
* repeated .mesos.v1.MasterInfo.Capability capabilities = 9;
*/
int getCapabilitiesCount();
/**
* repeated .mesos.v1.MasterInfo.Capability capabilities = 9;
*/
java.util.List extends org.apache.mesos.v1.Protos.MasterInfo.CapabilityOrBuilder>
getCapabilitiesOrBuilderList();
/**
* repeated .mesos.v1.MasterInfo.Capability capabilities = 9;
*/
org.apache.mesos.v1.Protos.MasterInfo.CapabilityOrBuilder getCapabilitiesOrBuilder(
int index);
}
/**
*
**
* Describes a master. This will probably have more fields in the
* future which might be used, for example, to link a framework webui
* to a master webui.
*
*
* Protobuf type {@code mesos.v1.MasterInfo}
*/
public static final class MasterInfo extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:mesos.v1.MasterInfo)
MasterInfoOrBuilder {
private static final long serialVersionUID = 0L;
// Use MasterInfo.newBuilder() to construct.
private MasterInfo(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private MasterInfo() {
id_ = "";
ip_ = 0;
port_ = 5050;
pid_ = "";
hostname_ = "";
version_ = "";
capabilities_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private MasterInfo(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000001;
id_ = bs;
break;
}
case 16: {
bitField0_ |= 0x00000002;
ip_ = input.readUInt32();
break;
}
case 24: {
bitField0_ |= 0x00000004;
port_ = input.readUInt32();
break;
}
case 34: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000008;
pid_ = bs;
break;
}
case 42: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000010;
hostname_ = bs;
break;
}
case 50: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000020;
version_ = bs;
break;
}
case 58: {
org.apache.mesos.v1.Protos.Address.Builder subBuilder = null;
if (((bitField0_ & 0x00000040) == 0x00000040)) {
subBuilder = address_.toBuilder();
}
address_ = input.readMessage(org.apache.mesos.v1.Protos.Address.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(address_);
address_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000040;
break;
}
case 66: {
org.apache.mesos.v1.Protos.DomainInfo.Builder subBuilder = null;
if (((bitField0_ & 0x00000080) == 0x00000080)) {
subBuilder = domain_.toBuilder();
}
domain_ = input.readMessage(org.apache.mesos.v1.Protos.DomainInfo.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(domain_);
domain_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000080;
break;
}
case 74: {
if (!((mutable_bitField0_ & 0x00000100) == 0x00000100)) {
capabilities_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000100;
}
capabilities_.add(
input.readMessage(org.apache.mesos.v1.Protos.MasterInfo.Capability.PARSER, extensionRegistry));
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000100) == 0x00000100)) {
capabilities_ = java.util.Collections.unmodifiableList(capabilities_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_MasterInfo_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_MasterInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.mesos.v1.Protos.MasterInfo.class, org.apache.mesos.v1.Protos.MasterInfo.Builder.class);
}
public interface CapabilityOrBuilder extends
// @@protoc_insertion_point(interface_extends:mesos.v1.MasterInfo.Capability)
com.google.protobuf.MessageOrBuilder {
/**
* optional .mesos.v1.MasterInfo.Capability.Type type = 1;
*/
boolean hasType();
/**
* optional .mesos.v1.MasterInfo.Capability.Type type = 1;
*/
org.apache.mesos.v1.Protos.MasterInfo.Capability.Type getType();
}
/**
* Protobuf type {@code mesos.v1.MasterInfo.Capability}
*/
public static final class Capability extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:mesos.v1.MasterInfo.Capability)
CapabilityOrBuilder {
private static final long serialVersionUID = 0L;
// Use Capability.newBuilder() to construct.
private Capability(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Capability() {
type_ = 0;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Capability(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
int rawValue = input.readEnum();
org.apache.mesos.v1.Protos.MasterInfo.Capability.Type value = org.apache.mesos.v1.Protos.MasterInfo.Capability.Type.valueOf(rawValue);
if (value == null) {
unknownFields.mergeVarintField(1, rawValue);
} else {
bitField0_ |= 0x00000001;
type_ = rawValue;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_MasterInfo_Capability_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_MasterInfo_Capability_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.mesos.v1.Protos.MasterInfo.Capability.class, org.apache.mesos.v1.Protos.MasterInfo.Capability.Builder.class);
}
/**
* Protobuf enum {@code mesos.v1.MasterInfo.Capability.Type}
*/
public enum Type
implements com.google.protobuf.ProtocolMessageEnum {
/**
* UNKNOWN = 0;
*/
UNKNOWN(0),
/**
*
* The master can handle slaves whose state
* changes after reregistering.
*
*
* AGENT_UPDATE = 1;
*/
AGENT_UPDATE(1),
/**
*
* The master can drain or deactivate agents when requested
* via operator APIs.
*
*
* AGENT_DRAINING = 2;
*/
AGENT_DRAINING(2),
;
/**
* UNKNOWN = 0;
*/
public static final int UNKNOWN_VALUE = 0;
/**
*
* The master can handle slaves whose state
* changes after reregistering.
*
*
* AGENT_UPDATE = 1;
*/
public static final int AGENT_UPDATE_VALUE = 1;
/**
*
* The master can drain or deactivate agents when requested
* via operator APIs.
*
*
* AGENT_DRAINING = 2;
*/
public static final int AGENT_DRAINING_VALUE = 2;
public final int getNumber() {
return value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static Type valueOf(int value) {
return forNumber(value);
}
public static Type forNumber(int value) {
switch (value) {
case 0: return UNKNOWN;
case 1: return AGENT_UPDATE;
case 2: return AGENT_DRAINING;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
Type> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public Type findValueByNumber(int number) {
return Type.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return org.apache.mesos.v1.Protos.MasterInfo.Capability.getDescriptor().getEnumTypes().get(0);
}
private static final Type[] VALUES = values();
public static Type valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int value;
private Type(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:mesos.v1.MasterInfo.Capability.Type)
}
private int bitField0_;
public static final int TYPE_FIELD_NUMBER = 1;
private int type_;
/**
* optional .mesos.v1.MasterInfo.Capability.Type type = 1;
*/
public boolean hasType() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional .mesos.v1.MasterInfo.Capability.Type type = 1;
*/
public org.apache.mesos.v1.Protos.MasterInfo.Capability.Type getType() {
org.apache.mesos.v1.Protos.MasterInfo.Capability.Type result = org.apache.mesos.v1.Protos.MasterInfo.Capability.Type.valueOf(type_);
return result == null ? org.apache.mesos.v1.Protos.MasterInfo.Capability.Type.UNKNOWN : result;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeEnum(1, type_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(1, type_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.mesos.v1.Protos.MasterInfo.Capability)) {
return super.equals(obj);
}
org.apache.mesos.v1.Protos.MasterInfo.Capability other = (org.apache.mesos.v1.Protos.MasterInfo.Capability) obj;
boolean result = true;
result = result && (hasType() == other.hasType());
if (hasType()) {
result = result && type_ == other.type_;
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasType()) {
hash = (37 * hash) + TYPE_FIELD_NUMBER;
hash = (53 * hash) + type_;
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.mesos.v1.Protos.MasterInfo.Capability parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.MasterInfo.Capability parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.MasterInfo.Capability parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.MasterInfo.Capability parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.MasterInfo.Capability parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.mesos.v1.Protos.MasterInfo.Capability parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.MasterInfo.Capability parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.MasterInfo.Capability parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.MasterInfo.Capability parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.MasterInfo.Capability parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.mesos.v1.Protos.MasterInfo.Capability parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.mesos.v1.Protos.MasterInfo.Capability parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.apache.mesos.v1.Protos.MasterInfo.Capability prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code mesos.v1.MasterInfo.Capability}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:mesos.v1.MasterInfo.Capability)
org.apache.mesos.v1.Protos.MasterInfo.CapabilityOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_MasterInfo_Capability_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_MasterInfo_Capability_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.mesos.v1.Protos.MasterInfo.Capability.class, org.apache.mesos.v1.Protos.MasterInfo.Capability.Builder.class);
}
// Construct using org.apache.mesos.v1.Protos.MasterInfo.Capability.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
type_ = 0;
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.mesos.v1.Protos.internal_static_mesos_v1_MasterInfo_Capability_descriptor;
}
public org.apache.mesos.v1.Protos.MasterInfo.Capability getDefaultInstanceForType() {
return org.apache.mesos.v1.Protos.MasterInfo.Capability.getDefaultInstance();
}
public org.apache.mesos.v1.Protos.MasterInfo.Capability build() {
org.apache.mesos.v1.Protos.MasterInfo.Capability result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.apache.mesos.v1.Protos.MasterInfo.Capability buildPartial() {
org.apache.mesos.v1.Protos.MasterInfo.Capability result = new org.apache.mesos.v1.Protos.MasterInfo.Capability(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.type_ = type_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.apache.mesos.v1.Protos.MasterInfo.Capability) {
return mergeFrom((org.apache.mesos.v1.Protos.MasterInfo.Capability)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.mesos.v1.Protos.MasterInfo.Capability other) {
if (other == org.apache.mesos.v1.Protos.MasterInfo.Capability.getDefaultInstance()) return this;
if (other.hasType()) {
setType(other.getType());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.mesos.v1.Protos.MasterInfo.Capability parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.mesos.v1.Protos.MasterInfo.Capability) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private int type_ = 0;
/**
* optional .mesos.v1.MasterInfo.Capability.Type type = 1;
*/
public boolean hasType() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional .mesos.v1.MasterInfo.Capability.Type type = 1;
*/
public org.apache.mesos.v1.Protos.MasterInfo.Capability.Type getType() {
org.apache.mesos.v1.Protos.MasterInfo.Capability.Type result = org.apache.mesos.v1.Protos.MasterInfo.Capability.Type.valueOf(type_);
return result == null ? org.apache.mesos.v1.Protos.MasterInfo.Capability.Type.UNKNOWN : result;
}
/**
* optional .mesos.v1.MasterInfo.Capability.Type type = 1;
*/
public Builder setType(org.apache.mesos.v1.Protos.MasterInfo.Capability.Type value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
type_ = value.getNumber();
onChanged();
return this;
}
/**
* optional .mesos.v1.MasterInfo.Capability.Type type = 1;
*/
public Builder clearType() {
bitField0_ = (bitField0_ & ~0x00000001);
type_ = 0;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:mesos.v1.MasterInfo.Capability)
}
// @@protoc_insertion_point(class_scope:mesos.v1.MasterInfo.Capability)
private static final org.apache.mesos.v1.Protos.MasterInfo.Capability DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.apache.mesos.v1.Protos.MasterInfo.Capability();
}
public static org.apache.mesos.v1.Protos.MasterInfo.Capability getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public Capability parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Capability(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.apache.mesos.v1.Protos.MasterInfo.Capability getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private int bitField0_;
public static final int ID_FIELD_NUMBER = 1;
private volatile java.lang.Object id_;
/**
*