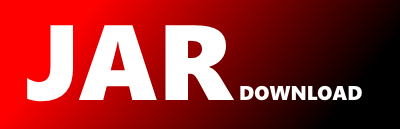
org.apache.myfaces.renderkit.html.HtmlTextareaRendererBase Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of myfaces-commons Show documentation
Show all versions of myfaces-commons Show documentation
The MyFaces Commons Subproject provides base classes for usage in both the
MyFaces implementation and the MyFaces Tomahawk components. This is also
a general set of utility classes for usage in your JSF projects independent
of the implementation you might be deciding upon.
The newest version!
/*
* Copyright 2004 The Apache Software Foundation.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.myfaces.renderkit.html;
import org.apache.myfaces.renderkit.JSFAttr;
import org.apache.myfaces.renderkit.RendererUtils;
import javax.faces.component.UIComponent;
import javax.faces.component.UIInput;
import javax.faces.component.UIOutput;
import javax.faces.component.html.HtmlInputTextarea;
import javax.faces.context.FacesContext;
import javax.faces.context.ResponseWriter;
import javax.faces.convert.ConverterException;
import java.io.IOException;
/**
* @author Manfred Geiler (latest modification by $Author: skitching $)
* @author Anton Koinov
* @version $Revision: 349370 $ $Date: 2005-11-28 05:01:41 +0000 (Mon, 28 Nov 2005) $
*/
public class HtmlTextareaRendererBase
extends HtmlRenderer
{
public void encodeEnd(FacesContext facesContext, UIComponent uiComponent)
throws IOException
{
RendererUtils.checkParamValidity(facesContext, uiComponent, UIInput.class);
encodeTextArea(facesContext, uiComponent);
}
protected void encodeTextArea(FacesContext facesContext, UIComponent uiComponent) throws IOException {
ResponseWriter writer = facesContext.getResponseWriter();
writer.startElement(HTML.TEXTAREA_ELEM, uiComponent);
String clientId = uiComponent.getClientId(facesContext);
writer.writeAttribute(HTML.NAME_ATTR, clientId, null);
HtmlRendererUtils.writeIdIfNecessary(writer, uiComponent, facesContext);
HtmlRendererUtils.renderHTMLAttributes(writer, uiComponent, HTML.TEXTAREA_PASSTHROUGH_ATTRIBUTES_WITHOUT_DISABLED);
if (isDisabled(facesContext, uiComponent))
{
writer.writeAttribute(HTML.DISABLED_ATTR, Boolean.TRUE, null);
}
String strValue = RendererUtils.getStringValue(facesContext, uiComponent);
writer.writeText(strValue, JSFAttr.VALUE_ATTR);
writer.endElement(HTML.TEXTAREA_ELEM);
}
protected boolean isDisabled(FacesContext facesContext, UIComponent uiComponent)
{
//TODO: overwrite in extended HtmlTextareaRenderer and check for enabledOnUserRole
if (uiComponent instanceof HtmlInputTextarea)
{
return ((HtmlInputTextarea)uiComponent).isDisabled();
}
else
{
return RendererUtils.getBooleanAttribute(uiComponent, HTML.DISABLED_ATTR, false);
}
}
public void decode(FacesContext facesContext, UIComponent component)
{
RendererUtils.checkParamValidity(facesContext, component, UIInput.class);
HtmlRendererUtils.decodeUIInput(facesContext, component);
}
public Object getConvertedValue(FacesContext facesContext, UIComponent uiComponent, Object submittedValue) throws ConverterException
{
RendererUtils.checkParamValidity(facesContext, uiComponent, UIOutput.class);
return RendererUtils.getConvertedUIOutputValue(facesContext,
(UIOutput)uiComponent,
submittedValue);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy