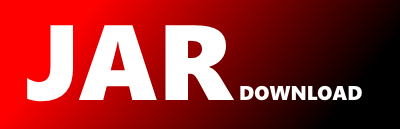
org.apache.myfaces.commons.converter.DateTimeConverter Maven / Gradle / Ivy
The newest version!
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package org.apache.myfaces.commons.converter;
import java.text.DateFormat;
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.Date;
import java.util.HashMap;
import java.util.Iterator;
import java.util.Locale;
import java.util.Map;
import java.util.TimeZone;
import javax.el.ValueExpression;
import javax.faces.application.FacesMessage;
import javax.faces.component.UIComponent;
import javax.faces.context.FacesContext;
import javax.faces.convert.ConverterException;
import org.apache.myfaces.buildtools.maven2.plugin.builder.annotation.JSFConverter;
import org.apache.myfaces.buildtools.maven2.plugin.builder.annotation.JSFProperty;
import org.apache.myfaces.commons.util.MessageUtils;
/**
* Simple convert that overrides the spec DateTimeConverter and uses TimeZone.getDefault() as the
* base timezone, rather than GMT.
*
* Convert date time using normal system timezone like it should
*
* User: treeder
* Date: Oct 28, 2005
* Time: 7:19:01 PM
*/
@JSFConverter(
name = "mcc:convertDateTime",
tagClass = "org.apache.myfaces.commons.converter.ConvertDateTimeTag",
tagSuperclass = "org.apache.myfaces.commons.converter.ConverterTag",
tagHandler = "org.apache.myfaces.commons.converter.ConvertDateTimeTagHandler",
serialuidtag = "1542071733367150635L",
evaluateELOnExecution=true)
public class DateTimeConverter extends javax.faces.convert.DateTimeConverter
{
public static final String CONVERTER_ID = "org.apache.myfaces.custom.convertDateTime.DateTimeConverter";
// internal constants
private static final String TYPE_DATE = "date";
private static final String TYPE_TIME = "time";
private static final String TYPE_BOTH = "both";
private static final String STYLE_DEFAULT = "default";
private static final String STYLE_MEDIUM = "medium";
private static final String STYLE_SHORT = "short";
private static final String STYLE_LONG = "long";
private static final String STYLE_FULL = "full";
private static final TimeZone TIMEZONE_DEFAULT = TimeZone.getTimeZone("GMT");
// CONSTRUCTORS
public DateTimeConverter()
{
//setTimeZone(TimeZone.getDefault());
}
private String _dateStyle;
private Locale _locale;
private String _pattern;
private String _timeStyle;
private TimeZone _timeZone;
private String _type;
private boolean _transient;
private transient FacesContext _facesContext;
// METHODS
public Object getAsObject(FacesContext facesContext, UIComponent uiComponent, String value)
{
if (facesContext == null) throw new NullPointerException("facesContext");
if (uiComponent == null) throw new NullPointerException("uiComponent");
if (value != null)
{
value = value.trim();
if (value.length() > 0)
{
DateFormat format = getDateFormat();
TimeZone tz = getTimeZone();
if( tz != null )
format.setTimeZone( tz );
try
{
return format.parse(value);
}
catch (ParseException e)
{
String type = getType();
Object[] args = new Object[]{value,format.format(new Date()),MessageUtils.getLabel(facesContext, uiComponent)};
if(type.equals(TYPE_DATE))
throw new ConverterException(MessageUtils.getMessage(FacesMessage.FACES_MESSAGES, FacesMessage.SEVERITY_ERROR, DATE_ID,args, facesContext));
else if (type.equals(TYPE_TIME))
throw new ConverterException(MessageUtils.getMessage(FacesMessage.FACES_MESSAGES, FacesMessage.SEVERITY_ERROR,TIME_ID,args, facesContext));
else if (type.equals(TYPE_BOTH))
throw new ConverterException(MessageUtils.getMessage(FacesMessage.FACES_MESSAGES, FacesMessage.SEVERITY_ERROR,DATETIME_ID,args, facesContext));
else
throw new ConverterException("invalid type '" + _type + "'");
}
}
}
return null;
}
public String getAsString(FacesContext facesContext, UIComponent uiComponent, Object value)
{
if (facesContext == null) throw new NullPointerException("facesContext");
if (uiComponent == null) throw new NullPointerException("uiComponent");
if (value == null)
{
return "";
}
if (value instanceof String)
{
return (String)value;
}
DateFormat format = getDateFormat();
TimeZone tz = getTimeZone();
if (tz != null)
{
format.setTimeZone(tz);
}
try
{
return format.format(value);
}
catch (Exception e)
{
throw new ConverterException(MessageUtils.getMessage(FacesMessage.FACES_MESSAGES, FacesMessage.SEVERITY_ERROR, STRING_ID,
new Object[]{value,MessageUtils.getLabel(facesContext, uiComponent)}, facesContext)
,e);
}
}
private DateFormat getDateFormat()
{
String type = getType();
DateFormat format;
if (_pattern != null)
{
try
{
format = new SimpleDateFormat(_pattern, getLocale());
}
catch (IllegalArgumentException iae)
{
throw new ConverterException("Invalid pattern", iae);
}
}
else if (type.equals(TYPE_DATE))
{
format = DateFormat.getDateInstance(calcStyle(getDateStyle()), getLocale());
}
else if (type.equals(TYPE_TIME))
{
format = DateFormat.getTimeInstance(calcStyle(getTimeStyle()), getLocale());
}
else if (type.equals(TYPE_BOTH))
{
format = DateFormat.getDateTimeInstance(calcStyle(getDateStyle()),
calcStyle(getTimeStyle()),
getLocale());
}
else
{
throw new ConverterException("invalid type '" + _type + "'");
}
// format cannot be lenient (JSR-127)
format.setLenient(false);
return format;
}
private int calcStyle(String name)
{
if (name.equals(STYLE_DEFAULT))
{
return DateFormat.DEFAULT;
}
if (name.equals(STYLE_MEDIUM))
{
return DateFormat.MEDIUM;
}
if (name.equals(STYLE_SHORT))
{
return DateFormat.SHORT;
}
if (name.equals(STYLE_LONG))
{
return DateFormat.LONG;
}
if (name.equals(STYLE_FULL))
{
return DateFormat.FULL;
}
throw new ConverterException("invalid style '" + name + "'");
}
// STATE SAVE/RESTORE
public void restoreState(FacesContext facesContext, Object state)
{
Object[] values = (Object[])state;
_dateStyle = (String)values[0];
_locale = (Locale)values[1];
_pattern = (String)values[2];
_timeStyle = (String)values[3];
_timeZone = (TimeZone)values[4];
_type = (String)values[5];
restoreValueExpressionMap(facesContext, values[6]);
}
public Object saveState(FacesContext facesContext)
{
Object[] values = new Object[7];
values[0] = _dateStyle;
values[1] = _locale;
values[2] = _pattern;
values[3] = _timeStyle;
values[4] = _timeZone;
values[5] = _type;
values[6] = saveValueExpressionMap(facesContext);
return values;
}
// --------------------- borrowed from UIComponentBase ------------
private Map _valueExpressionMap = null;
public ValueExpression getValueExpression(String name)
{
if (name == null) throw new NullPointerException("name");
if (_valueExpressionMap == null)
{
return null;
}
else
{
return (ValueExpression)_valueExpressionMap.get(name);
}
}
public void setValueExpression(String name,
ValueExpression binding)
{
if (name == null) throw new NullPointerException("name");
if (_valueExpressionMap == null)
{
_valueExpressionMap = new HashMap();
}
_valueExpressionMap.put(name, binding);
}
private Object saveValueExpressionMap(FacesContext context)
{
if (_valueExpressionMap != null)
{
int initCapacity = (_valueExpressionMap.size() * 4 + 3) / 3;
HashMap stateMap = new HashMap(initCapacity);
for (Iterator it = _valueExpressionMap.entrySet().iterator(); it.hasNext(); )
{
Map.Entry entry = (Map.Entry)it.next();
stateMap.put(entry.getKey(),
ConverterBase.saveAttachedState(context, entry.getValue()));
}
return stateMap;
}
else
{
return null;
}
}
private void restoreValueExpressionMap(FacesContext context, Object stateObj)
{
if (stateObj != null)
{
Map stateMap = (Map)stateObj;
int initCapacity = (stateMap.size() * 4 + 3) / 3;
_valueExpressionMap = new HashMap(initCapacity);
for (Iterator it = stateMap.entrySet().iterator(); it.hasNext(); )
{
Map.Entry entry = (Map.Entry)it.next();
_valueExpressionMap.put(entry.getKey(),
ConverterBase.restoreAttachedState(context, entry.getValue()));
}
}
else
{
_valueExpressionMap = null;
}
}
protected FacesContext getFacesContext()
{
if (_facesContext == null)
{
return FacesContext.getCurrentInstance();
}
else
{
return _facesContext;
}
}
boolean isCachedFacesContext()
{
return _facesContext != null;
}
void setCachedFacesContext(FacesContext facesContext)
{
_facesContext = facesContext;
}
// GETTER & SETTER
/**
* The style of the date. Values include: default, short, medium,
* long, and full.
*
*/
@JSFProperty(inheritedTag = false)
public String getDateStyle()
{
if (_dateStyle != null)
{
return _dateStyle;
}
ValueExpression vb = getValueExpression("dateStyle");
if (vb != null)
{
return (String) vb.getValue(getFacesContext().getELContext());
}
return STYLE_DEFAULT;
}
public void setDateStyle(String dateStyle)
{
_dateStyle = dateStyle;
}
/**
* The name of the locale to be used, instead of the default.
*
*/
@JSFProperty(inheritedTag = false,deferredValueType="java.lang.Object")
public Locale getLocale()
{
if (_locale != null)
{
return _locale;
}
ValueExpression vb = getValueExpression("locale");
if (vb != null)
{
Object _localeValue = vb.getValue(getFacesContext().getELContext());
if (_localeValue instanceof String)
{
_localeValue = org.apache.myfaces.commons.util.TagUtils.getLocale((String)_localeValue);
}
return (java.util.Locale)_localeValue;
}
FacesContext context = FacesContext.getCurrentInstance();
return context.getViewRoot().getLocale();
}
public void setLocale(Locale locale)
{
_locale = locale;
}
/**
* A custom Date formatting pattern, in the format used by java.text.SimpleDateFormat.
*
*/
@JSFProperty(inheritedTag = false)
public String getPattern()
{
if (_pattern != null)
{
return _pattern;
}
ValueExpression vb = getValueExpression("pattern");
if (vb != null)
{
return (String) vb.getValue(getFacesContext().getELContext()).toString();
}
return null;
}
public void setPattern(String pattern)
{
_pattern = pattern;
}
/**
* The style of the time. Values include: default, short, medium, long,
* and full.
*
*/
@JSFProperty(inheritedTag = false)
public String getTimeStyle()
{
if (_timeStyle != null)
{
return _timeStyle;
}
ValueExpression vb = getValueExpression("timeStyle");
if (vb != null)
{
return (String) vb.getValue(getFacesContext().getELContext()).toString();
}
return STYLE_DEFAULT;
}
public void setTimeStyle(String timeStyle)
{
_timeStyle = timeStyle;
}
/**
* The time zone to use instead of GMT (the default timezone). When
* this value is a value-binding to a TimeZone instance, that
* timezone is used. Otherwise this value is treated as a String
* containing a timezone id, ie as the ID parameter of method
* java.util.TimeZone.getTimeZone(String).
*
*/
@JSFProperty(inheritedTag = false,deferredValueType="java.lang.Object")
public TimeZone getTimeZone()
{
//return _timeZone != null ? _timeZone : TIMEZONE_DEFAULT;
if (_timeZone != null)
{
return _timeZone;
}
ValueExpression vb = getValueExpression("timeZone");
if (vb != null)
{
Object _timeZoneValue = vb.getValue(getFacesContext().getELContext());
if(_timeZoneValue instanceof java.util.TimeZone)
{
return (java.util.TimeZone) _timeZoneValue;
}
else
{
return java.util.TimeZone.getTimeZone(_timeZoneValue.toString());
}
}
return TimeZone.getDefault(); //TIMEZONE_DEFAULT
}
public void setTimeZone(TimeZone timeZone)
{
_timeZone = timeZone;
}
/**
* Specifies whether the date, time, or both should be
* parsed/formatted. Values include: date, time, and both.
* Default based on setting of timeStyle and dateStyle.
*
*/
@JSFProperty(inheritedTag = false)
public String getType()
{
if (_type != null)
{
return _type;
}
ValueExpression vb = getValueExpression("type");
if (vb != null)
{
return (String) vb.getValue(getFacesContext().getELContext()).toString();
}
return TYPE_DATE;
}
public void setType(String type)
{
_type = type;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy