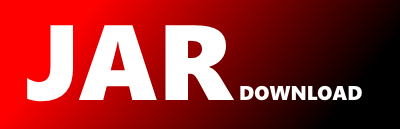
javax.faces.component.UISelectItem Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of myfaces-api Show documentation
Show all versions of myfaces-api Show documentation
The public API classes of the Apache MyFaces CORE JSF-2.2 project
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package javax.faces.component;
import javax.el.ValueExpression;
import javax.faces.context.FacesContext;
import javax.faces.component.UIComponent;
// Generated from class javax.faces.component._UISelectItem.
//
// WARNING: This file was automatically generated. Do not edit it directly,
// or you will lose your changes.
public class UISelectItem extends javax.faces.component.UIComponentBase
{
static public final String COMPONENT_FAMILY =
"javax.faces.SelectItem";
static public final String COMPONENT_TYPE =
"javax.faces.SelectItem";
//BEGIN CODE COPIED FROM javax.faces.component._UISelectItem
public void setRendered(boolean state)
{
super.setRendered(state);
//call parent method due TCK problems
//throw new UnsupportedOperationException();
}
//END CODE COPIED FROM javax.faces.component._UISelectItem
public UISelectItem()
{
setRendererType(null);
}
@Override
public String getFamily()
{
return COMPONENT_FAMILY;
}
// Property: value
private Object _value;
public Object getValue()
{
if (_value != null)
{
return _value;
}
ValueExpression vb = getValueExpression("value");
if (vb != null)
{
return vb.getValue(getFacesContext().getELContext());
}
return null;
}
public void setValue(Object value)
{
this._value = value;
}
// Property: itemDisabled
private boolean _itemDisabled;
private boolean _itemDisabledSet;
public boolean isItemDisabled()
{
if (_itemDisabledSet)
{
return _itemDisabled;
}
ValueExpression vb = getValueExpression("itemDisabled");
if (vb != null)
{
return ((Boolean) vb.getValue(getFacesContext().getELContext())).booleanValue();
}
return false;
}
public void setItemDisabled(boolean itemDisabled)
{
this._itemDisabled = itemDisabled;
this._itemDisabledSet = true;
}
// Property: itemEscaped
private boolean _itemEscaped;
private boolean _itemEscapedSet;
public boolean isItemEscaped()
{
if (_itemEscapedSet)
{
return _itemEscaped;
}
ValueExpression vb = getValueExpression("itemEscaped");
if (vb != null)
{
return ((Boolean) vb.getValue(getFacesContext().getELContext())).booleanValue();
}
return false;
}
public void setItemEscaped(boolean itemEscaped)
{
this._itemEscaped = itemEscaped;
this._itemEscapedSet = true;
}
// Property: itemDescription
private String _itemDescription;
public String getItemDescription()
{
if (_itemDescription != null)
{
return _itemDescription;
}
ValueExpression vb = getValueExpression("itemDescription");
if (vb != null)
{
return (String) vb.getValue(getFacesContext().getELContext());
}
return null;
}
public void setItemDescription(String itemDescription)
{
this._itemDescription = itemDescription;
}
// Property: itemLabel
private String _itemLabel;
public String getItemLabel()
{
if (_itemLabel != null)
{
return _itemLabel;
}
ValueExpression vb = getValueExpression("itemLabel");
if (vb != null)
{
return (String) vb.getValue(getFacesContext().getELContext());
}
return null;
}
public void setItemLabel(String itemLabel)
{
this._itemLabel = itemLabel;
}
// Property: itemValue
private Object _itemValue;
public Object getItemValue()
{
if (_itemValue != null)
{
return _itemValue;
}
ValueExpression vb = getValueExpression("itemValue");
if (vb != null)
{
return vb.getValue(getFacesContext().getELContext());
}
return null;
}
public void setItemValue(Object itemValue)
{
this._itemValue = itemValue;
}
@Override
public Object saveState(FacesContext facesContext)
{
Object[] values = new Object[9];
values[0] = super.saveState(facesContext);
values[1] = _value;
values[2] = Boolean.valueOf(_itemDisabled);
values[3] = Boolean.valueOf(_itemDisabledSet);
values[4] = Boolean.valueOf(_itemEscaped);
values[5] = Boolean.valueOf(_itemEscapedSet);
values[6] = _itemDescription;
values[7] = _itemLabel;
values[8] = _itemValue;
return values;
}
@Override
public void restoreState(FacesContext facesContext, Object state)
{
Object[] values = (Object[])state;
super.restoreState(facesContext,values[0]);
_value = values[1];
_itemDisabled = ((Boolean) values[2]).booleanValue();
_itemDisabledSet = ((Boolean) values[3]).booleanValue();
_itemEscaped = ((Boolean) values[4]).booleanValue();
_itemEscapedSet = ((Boolean) values[5]).booleanValue();
_itemDescription = (java.lang.String) values[6];
_itemLabel = (java.lang.String) values[7];
_itemValue = values[8];
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy