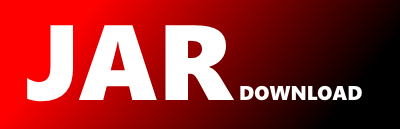
javax.faces.application.Application Maven / Gradle / Ivy
Show all versions of myfaces-api Show documentation
/* * Licensed to the Apache Software Foundation (ASF) under one * or more contributor license agreements. See the NOTICE file * distributed with this work for additional information * regarding copyright ownership. The ASF licenses this file * to you under the Apache License, Version 2.0 (the * "License"); you may not use this file except in compliance * with the License. You may obtain a copy of the License at * * http://www.apache.org/licenses/LICENSE-2.0 * * Unless required by applicable law or agreed to in writing, * software distributed under the License is distributed on an * "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY * KIND, either express or implied. See the License for the * specific language governing permissions and limitations * under the License. */ package javax.faces.application; import java.util.Collection; import java.util.Collections; import java.util.Iterator; import java.util.Locale; import java.util.Map; import java.util.ResourceBundle; import javax.el.ELContextListener; import javax.el.ELException; import javax.el.ELResolver; import javax.el.ExpressionFactory; import javax.el.ValueExpression; import javax.faces.FacesException; import javax.faces.component.UIComponent; import javax.faces.context.ExternalContext; import javax.faces.component.behavior.Behavior; import javax.faces.context.FacesContext; import javax.faces.convert.Converter; import javax.faces.el.MethodBinding; import javax.faces.el.PropertyResolver; import javax.faces.el.ReferenceSyntaxException; import javax.faces.el.ValueBinding; import javax.faces.el.VariableResolver; import javax.faces.event.ActionListener; import javax.faces.event.SystemEvent; import javax.faces.event.SystemEventListener; import javax.faces.validator.Validator; /** *
registered for interfaces that * are implemented by the target class (directly or indirectly). Locate a* Application represents a per-web-application singleton object where applications based on JavaServer Faces (or * implementations wishing to provide extended functionality) can register application-wide singletons that provide * functionality required by JavaServer Faces. Default implementations of each object are provided for cases where the * application does not choose to customize the behavior. *
* ** The instance of {@link Application} is created by calling the
* * Holds webapp-wide resources for a JSF application. There is a single one of these for a web application, accessable * via * *getApplication()
method of * {@link ApplicationFactory}. Because this instance is shared, it must be implemented in a thread-safe manner. ** FacesContext.getCurrentInstance().getApplication() ** * In particular, this provides a factory for UIComponent objects. It also provides convenience methods for creating * ValueBinding objects. * * See Javadoc of JSF Specification * * @author Manfred Geiler (latest modification by $Author: struberg $) * @author Stan Silvert * @version $Revision: 1188206 $ $Date: 2011-10-24 11:30:34 -0500 (Mon, 24 Oct 2011) $ */ @SuppressWarnings("deprecation") public abstract class Application { /** * Retrieve the current Myfaces Application Instance, lookup * on the application map. All methods introduced on jsf 1.2 * for Application interface should thrown by default * UnsupportedOperationException, but the ri scan and find the * original Application impl, and redirect the call to that * method instead throwing it, allowing application implementations * created before jsf 1.2 continue working. * * Note: every method, which uses getMyfacesApplicationInstance() to * delegate itself to the current ApplicationImpl MUST be * overriden by the current ApplicationImpl to prevent infinite loops. */ private Application getMyfacesApplicationInstance() { FacesContext facesContext = FacesContext.getCurrentInstance(); if (facesContext != null) { ExternalContext externalContext = facesContext.getExternalContext(); if (externalContext != null) { return (Application) externalContext.getApplicationMap().get( "org.apache.myfaces.application.ApplicationImpl"); } } return null; } private Application getMyfacesApplicationInstance(FacesContext facesContext) { if (facesContext != null) { ExternalContext externalContext = facesContext.getExternalContext(); if (externalContext != null) { return (Application) externalContext.getApplicationMap().get( "org.apache.myfaces.application.ApplicationImpl"); } } return null; } // The concrete methods throwing UnsupportedOperationExceptiom were added for JSF 1.2. // They supply default to allows old Application implementations to still work. /** * @since 2.0 * * FIXME: Notify EG, this should not be abstract and throw UnsupportedOperationException */ public void addBehavior(String behaviorId, String behaviorClass) { Application application = getMyfacesApplicationInstance(); if (application != null) { application.addBehavior(behaviorId, behaviorClass); return; } throw new UnsupportedOperationException(); } /** * Define a new mapping from a logical "component type" to an actual java class name. This controls what type is * created when method createComponent of this class is called. ** Param componentClass must be the fully-qualified class name of some class extending the UIComponent class. The * class must have a default constructor, as instances of it will be created using Class.newInstance. *
* It is permitted to override a previously defined mapping, ie to call this method multiple times with the same * componentType string. The createComponent method will simply use the last defined mapping. */ /** * Register a new mapping of component type to the name of the corresponding {@link UIComponent} class. This allows * subsequent calls to
createComponent()
to serve as a factory for {@link UIComponent} instances. * * @param componentType * - The component type to be registered * @param componentClass * - The fully qualified class name of the corresponding {@link UIComponent} implementation * * @throws NullPointerException * ifcomponentType
orcomponentClass
isnull
*/ public abstract void addComponent(String componentType, String componentClass); /** * Register a new converter class that is capable of performing conversions for the specified target class. * * @param targetClass * - The class for which this converter is registered * @param converterClass * - The fully qualified class name of the corresponding {@link Converter} implementation * * @throws NullPointerException * iftargetClass
orconverterClass
isnull
*/ public abstract void addConverter(Class> targetClass, String converterClass); /** * Register a new mapping of converter id to the name of the corresponding {@link Converter} class. This allows * subsequent calls to createConverter() to serve as a factory for {@link Converter} instances. * * @param converterId * - The converterId to be registered * @param converterClass * - The fully qualified class name of the corresponding {@link Converter} implementation * * @throws NullPointerException * ifcomponentType
orcomponentClass
isnull
*/ public abstract void addConverter(String converterId, String converterClass); /** * * @param validatorId * * @since 2.0 */ public void addDefaultValidatorId(String validatorId) { } /** ** Provide a way for Faces applications to register an
ELContextListener
that will be notified on * creation ofELContext
instances. ** *
* An implementation is provided that throws
* * @since 1.2 */ public void addELContextListener(ELContextListener listener) { Application application = getMyfacesApplicationInstance(); if (application != null) { application.addELContextListener(listener); return; } throw new UnsupportedOperationException(); } /** *UnsupportedOperationException
so that users that decorate * theApplication
continue to work. ** Cause an the argument
* *resolver
to be added to the resolver chain as specified in section 5.5.1 of * the JavaServer Faces Specification. ** It is not possible to remove an
* *ELResolver
registered with this method, once it has been registered. ** It is illegal to register an ELResolver after the application has received any requests from the client. If an * attempt is made to register a listener after that time, an IllegalStateException must be thrown. This restriction * is in place to allow the JSP container to optimize for the common case where no additional *
ELResolvers
are in the chain, aside from the standard ones. It is permissible to add *ELResolvers
before or after initialization to a CompositeELResolver that is already in the chain. ** *
* The default implementation throws
* * @since 1.2 */ public void addELResolver(ELResolver resolver) { // The following concrete methods were added for JSF 1.2. They supply default // implementations that throw UnsupportedOperationException. // This allows old Application implementations to still work. Application application = getMyfacesApplicationInstance(); if (application != null) { application.addELResolver(resolver); return; } throw new UnsupportedOperationException(); } /** *Register a new mapping of validator id to the name of the correspondingUnsupportedOperationException
and is provided for the sole purpose * of not breaking existing applications that extend {@link Application}. *Validator
class. This allows * subsequent calls tocreateValidator()
to serve as a factory forValidator
instances. * *@param validatorId The validator id to be registered *@param validatorClass The fully qualified class name of the corresponding Validator implementation * *@throws NullPointerException * ifvalidatorId
orvalidatorClass
isnull
*/ public abstract void addValidator(String validatorId, String validatorClass); /** * * @param behaviorId * @return * @throws FacesException * @since 2.0 * * FIXME: Notify EG, this should not be abstract and throw UnsupportedOperationException */ public Behavior createBehavior(String behaviorId) throws FacesException { Application application = getMyfacesApplicationInstance(); if (application != null) { return application.createBehavior(behaviorId); } throw new UnsupportedOperationException(); } /** * ??? * * @param context * @param componentResource * @return * * @since 2.0 */ public UIComponent createComponent(FacesContext context, Resource componentResource) { Application application = getMyfacesApplicationInstance(context); if (application != null) { return application.createComponent(context, componentResource); } throw new UnsupportedOperationException(); } /** * * @param context * @param componentType * @param rendererType * @return * * @since 2.0 */ public UIComponent createComponent(FacesContext context, String componentType, String rendererType) { Application application = getMyfacesApplicationInstance(context); if (application != null) { return application.createComponent(context, componentType, rendererType); } throw new UnsupportedOperationException(); } /** ** Create a new UIComponent subclass, using the mappings defined by previous calls to the addComponent method of * this class. *
* * @throws FacesException * if there is no mapping defined for the specified componentType, or if an instance of the specified * type could not be created for any reason. */ public abstract UIComponent createComponent(String componentType) throws FacesException; /** ** Create an object which has an associating "binding" expression tying the component to a user property. *
* ** First the specified value-binding is evaluated; if it returns a non-null value then the component * "already exists" and so the resulting value is simply returned. *
* ** Otherwise a new UIComponent instance is created using the specified componentType, and the new object stored via * the provided value-binding before being returned. *
* * @deprecated */ public abstract UIComponent createComponent(ValueBinding componentBinding, FacesContext context, String componentType) throws FacesException; /** ** Call the
* * @param componentExpression * -getValue()
method on the specifiedValueExpression
. If it returns a *{@link UIComponent}
instance, return it as the value of this method. If it does not, instantiate a * new{@linkUIComponent}
instance of the specified component type, pass the new component to the *setValue()
method of the specifiedValueExpression
, and return it. *ValueExpression
representing a component value expression (typically specified by the *component
attribute of a custom tag) * @param context * - {@link FacesContext} for the current request * @param componentType * - Component type to create if the ValueExpression does not return a component instance * * @throws FacesException * if a{@link UIComponent}
cannot be created * @throws NullPointerException * if any parameter is null ** A default implementation is provided that throws
* * @since 1.2 */ public UIComponent createComponent(ValueExpression componentExpression, FacesContext context, String componentType) throws FacesException { Application application = getMyfacesApplicationInstance(context); if (application != null) { return application.createComponent(componentExpression, context, componentType); } throw new UnsupportedOperationException(); } /** * * @param componentExpression * @param context * @param componentType * @param rendererType * @return * * @since 2.0 */ public UIComponent createComponent(ValueExpression componentExpression, FacesContext context, String componentType, String rendererType) { Application application = getMyfacesApplicationInstance(context); if (application != null) { return application.createComponent(componentExpression, context, componentType, rendererType); } throw new UnsupportedOperationException(); } /** *UnsupportedOperationException
so that * users that decorateApplication
can continue to function ** Instantiate and return a new
* *{@link Converter}
instance of the class that has registered itself as * capable of performing conversions for objects of the specified type. If no such{@link Converter}
* class can be identified, return null. ** To locate an appropriate
{@link Converter}
class, the following algorithm is performed, stopping as * soon as an appropriate{@link Converter}
class is found: Locate a{@link Converter}
* registered for the target class itself.{@link Converter} {@link Converter}
registered * for the superclass (if any) of the target class, recursively working up the inheritance hierarchy. * * ** If the
{@link Converter}
has a single argument constructor that accepts a Class, instantiate the *{@link Converter}
using that constructor, passing the argumenttargetClass
as * the sole argument. Otherwise, simply use the zero-argument constructor. * * @param targetClass * - Target class for which to return a{@link Converter}
* * @throws FacesException * if the{@link Converter}
cannot be created * @throws NullPointerException * iftargetClass
isnull
* */ public abstract Converter createConverter(Class> targetClass); /** * Instantiate and return a new{@link Converter}
instance of the class specified by a previous call to *addConverter()
for the specified converter id. If there is no such registration for this converter * id, returnnull
. * * @param converterId * - The converter id for which to create and return a new{@link Converter}
instance * * @throws FacesException * if the{@link Converter}
cannot be created * @throws NullPointerException * if converterId isnull
*/ public abstract Converter createConverter(String converterId); /** * Create an object which can be used to invoke an arbitrary method via an EL expression at a later time. This is * similar to createValueBinding except that it can invoke an arbitrary method (with parameters) rather than just * get/set a javabean property. ** This is used to invoke ActionListener method, and ValueChangeListener methods. * * @deprecated */ public abstract MethodBinding createMethodBinding(String ref, Class>[] params) throws ReferenceSyntaxException; /** * Instantiate and return a new
{@link Validator}
instance of the class specified by a previous call to *addValidator()
for the specified validator id. * * @param validatorId The{@link Validator}
id for which to create and return a new * Validator instance * * @throws FacesException * if a{@link Validator}/
, perform algorithm *of the specified id cannot be created * @throws NullPointerException * if validatorId is
{@link * #subscribeToEvent(java.lang.Class, java.lang.Class, SystemEventListener)}null
*/ public abstract Validator createValidator(String validatorId) throws FacesException; /** ** Create an object which can be used to invoke an arbitrary method via an EL expression at a later time. This is * similar to createValueBinding except that it can invoke an arbitrary method (with parameters) rather than just * get/set a javabean property. *
* This is used to invoke ActionListener method, and ValueChangeListener methods. * * @deprecated */ public abstract ValueBinding createValueBinding(String ref) throws ReferenceSyntaxException; /** ** Get a value by evaluating an expression. *
* ** Call
* *{@link #getExpressionFactory()}
then call *ExpressionFactory.createValueExpression(javax.el.ELContext, java.lang.String, java.lang.Class)
* passing the argumentexpression
andexpectedType
. Call *{@link FacesContext#getELContext()}
and pass it to *ValueExpression.getValue(javax.el.ELContext)
, returning the result. ** An implementation is provided that throws
UnsupportedOperationException
so that users that decorate * theApplication
continue to work. ** * @throws javax.el.ELException */ public
T evaluateExpressionGet(FacesContext context, String expression, Class extends T> expectedType) throws ELException { Application application = getMyfacesApplicationInstance(context); if (application != null) { return application.evaluateExpressionGet(context, expression, expectedType); } throw new UnsupportedOperationException(); } /** * * Return the default
*ActionListener
to be registered for allActionSource<3code> components * in this appication. If not explicitly set, a default implementation must be provided that performs the * following functions: *
*
*- The
* *processAction()
method must first callFacesContext.renderResponse()
in order to * bypass any intervening lifecycle phases, once the method returns.- The
* *processAction()
method must next determine the logical * outcome of this event, as follows:*
*- If the originating component has a non-
*null action
property, retrieve the* MethodBinding
from the property, and call
invoke()
* on it. Convert the returned value (if any) to a String, and use it as the logical outcome.- Otherwise, the logical outcome is null.
**
- The
*processAction()
method must finally retrieve theNavigationHandler<3code> instance * for this application and call
NavigationHandler.handleNavigation(javax.faces.context.FacesContext, * java.lang.String, java.lang.String)
passing:*
- the {@link FacesContext} for the current request
*- If there is a
*MethodBinding
instance for theaction
property of this component, the * result of calling {@link MethodBinding#getExpressionString()} on it, null otherwise- the logical outcome as determined above
**
** Note that the specification for the default
ActionListener
contiues to call for the use of a * deprecated property (action
) and class (MethodBinding
). Unfortunately, this is * necessary because the default ActionListener must continue to work with components that do not implement * {@link javax.faces.component.ActionSource2}, and only implement {@link javax.faces.component.ActionSource}. */ public abstract ActionListener getActionListener(); /** * * @return * * @since 2.0 * * FIXME: Notify EG, this should not be abstract and throw UnsupportedOperationException */ public IteratorgetBehaviorIds() { Application application = getMyfacesApplicationInstance(); if (application != null) { return application.getBehaviorIds(); } // It is better to return an empty iterator, // to keep compatiblity with previous jsf 2.0 Application // instances return Collections.EMPTY_LIST.iterator(); } /** * Return an Iterator
over the set of currently defined component types for this *Application
. */ public abstract IteratorgetComponentTypes(); /** * Return an Iterator
over the set of currently registered converter ids for this *Application
* * @return */ public abstract IteratorgetConverterIds(); /** *Return an Iterator
over the set ofClass
instances for which{@link Converter} *
classes
have been explicitly registered. * * @return */ public abstract Iterator> getConverterTypes(); /** *Return the default Locale
for this application. If not explicitly set,null
is * returned. * * @return */ public abstract Locale getDefaultLocale(); /** * Return therenderKitId
to be used for rendering this application. If not explicitly set, *null
is returned. * * @return */ public abstract String getDefaultRenderKitId(); /** * * @return * * @since 2.0 */ public MapgetDefaultValidatorInfo() { Application application = getMyfacesApplicationInstance(); if (application != null) { return application.getDefaultValidatorInfo(); } throw new UnsupportedOperationException(); } /** * * If no calls have been made to
addELContextListener(javax.el.ELContextListener)
, this method must * return an empty array ** . * *
* Otherwise, return an array representing the list of listeners added by calls to *
addELContextListener(javax.el.ELContextListener)
. ** *
* An
* * @since 1.2 */ public ELContextListener[] getELContextListeners() { Application application = getMyfacesApplicationInstance(); if (application != null) { return application.getELContextListeners(); } throw new UnsupportedOperationException(); } /** *implementation
is provided that throws UnsupportedOperationException so that users that decorate * theApplication
continue to work. ** Return the singleton
ELResolver
instance to be used for all EL resolution. This is actually an * instance ofCompositeELResolver
that must contain the following ELResolver instances in the * following order: *- * *
ELResolver
instances declared using theelement in the application configuration * resources. - An
* *implementation
that wraps the head of the legacy VariableResolver chain, as per section *VariableResolver ChainWrapper
in Chapter 5 in the spec document.- An
* *implementation
that wraps the head of the legacy PropertyResolver chain, as per section *PropertyResolver ChainWrapper
in Chapter 5 in the spec document.- Any
* *ELResolver
instances added by calls to *{@link #addELResolver(javax.el.ELResolver)}
.- The default implementation throws
*UnsupportedOperationException
and is provided for the sole * purpose of not breaking existing applications that extend{@link Application}
.* * @since 1.2 */ public ELResolver getELResolver() { Application application = getMyfacesApplicationInstance(); if (application != null) { return application.getELResolver(); } throw new UnsupportedOperationException(); } /** *
* Return the
* *ExpressionFactory
instance for this application. This instance is used by the convenience * method{@link #evaluateExpressionGet(javax.faces.context.FacesContext, java.lang.String, java.lang.Class)}. *
* The implementation must return the
* *ExpressionFactory
from the JSP container by calling* JspFactory.getDefaultFactory().getJspApplicationContext(servletContext).getExpressionFactory()
. ** An implementation is provided that throws
* * @since 1.2 */ public ExpressionFactory getExpressionFactory() { Application application = getMyfacesApplicationInstance(); if (application != null) { return application.getExpressionFactory(); } throw new UnsupportedOperationException(); } /** * Return the fully qualified class name of theUnsupportedOperationException
so that users that decorate * theApplication
continue to work. *ResourceBundle
to be used for JavaServer Faces messages * for this application. If not explicitly set,null
is returned. */ public abstract String getMessageBundle(); /** *Return the{@link NavigationHandler}
instance that will be passed the outcome returned by any * invoked application action for this web application. If not explicitly set, a default implementation must be * provided that performs the functions described in the{@link NavigationHandler}
class description. */ public abstract NavigationHandler getNavigationHandler(); /** ** Return the project stage for the currently running application instance. The default value is
* ** {@link ProjectStage#Production}
** The implementation of this method must perform the following algorithm or an equivalent with the same end result * to determine the value to return. *
* **
- If the value has already been determined by a previous call to this method, simply return that value.
*- Look for a
*JNDI
environment entry under the key given by the value of *{@link ProjectStage#PROJECT_STAGE_JNDI_NAME}
(return type of java.lang.String). If found, continue * with the algorithm below, otherwise, look for an entry in theinitParamMap
of the *ExternalContext
from the currentFacesContext
with the key *{@link ProjectStage#PROJECT_STAGE_PARAM_NAME}
- If a value is found found, see if an enum constant can be obtained by calling *
*ProjectStage.valueOf()
, passing the value from theinitParamMap
. If this succeeds * without exception, save the value and return it.- If not found, or any of the previous attempts to discover the enum constant value have failed, log a * descriptive error message, assign the value as
*ProjectStage.Production
and return it.* * @since 2.0 */ public ProjectStage getProjectStage() { Application application = getMyfacesApplicationInstance(); if (application != null) { return application.getProjectStage(); } throw new UnsupportedOperationException(); } /** * Get the object used by the VariableResolver to read and write named properties on java beans, Arrays, Lists and * Maps. This object is used by the ValueBinding implementation, and during the process of configuring * "managed bean" properties. * * @deprecated */ public abstract PropertyResolver getPropertyResolver(); /** *
* Find a
* *ResourceBundle
as defined in the application configuration resources under the specified * name. If aResourceBundle
was defined for the name, return an instance that uses the locale of the * current{@link javax.faces.component.UIViewRoot}
. ** The default implementation throws
* * @returnUnsupportedOperationException
and is provided for the sole purpose * of not breaking existing applications that extend this class. *ResourceBundle
for the current UIViewRoot, otherwise null * * @throws FacesException * if a bundle was defined, but not resolvable * @throws NullPointerException * if ctx == null || name == null */ public ResourceBundle getResourceBundle(FacesContext ctx, String name) throws FacesException, NullPointerException { Application application = getMyfacesApplicationInstance(ctx); if (application != null) { return application.getResourceBundle(ctx, name); } throw new UnsupportedOperationException(); } /** ** Return the singleton, stateless, thread-safe
* *{@link ResourceHandler}
for this application. The JSF * implementation must support the following techniques for declaring an alternate implementation of* ResourceHandler
. **
* *- The
*ResourceHandler
implementation is declared in the application configuration resources by * giving the fully qualified class name as the value of theelement within the *
application
element.- RELEASE_PENDING(edburns) It can also be declared via an annotation as * specified in [287-ConfigAnnotations].
** In all of the above cases, the runtime must employ the decorator pattern as for every other pluggable artifact in * JSF. *
* * @since 2.0 */ public ResourceHandler getResourceHandler() { Application application = getMyfacesApplicationInstance(); if (application != null) { return application.getResourceHandler(); } throw new UnsupportedOperationException(); } /** * Return theStateManager
instance that will be utilized during the Restore View and Render Response * phases of the request processing lifecycle. If not explicitly set, a default implementation must be provided that * performs the functions described in theStateManager
description in the JavaServer Faces * Specification. */ public abstract StateManager getStateManager(); /** * Return anIterator
over the supportedLocales
for this appication. */ public abstract IteratorgetSupportedLocales(); /** *Return an Iterator
over the set of currently registered validator ids for this *Application
. */ public abstract IteratorgetValidatorIds(); /** * Get the object used to resolve expressions of form "#{...}". * * @deprecated */ public abstract VariableResolver getVariableResolver(); /** * Set the {@link ViewHandler}
instance that will be utilized during the *Restore View and Render Response
phases of the request processing lifecycle. * * @return */ public abstract ViewHandler getViewHandler(); /** * * @param facesContext * @param systemEventClass * @param sourceBaseType * @param source * * @since 2.0 */ public void publishEvent(FacesContext facesContext, Class extends SystemEvent> systemEventClass, Class> sourceBaseType, Object source) { Application application = getMyfacesApplicationInstance(facesContext); if (application != null) { application.publishEvent(facesContext, systemEventClass, sourceBaseType, source); return; } throw new UnsupportedOperationException(); } /** ** If there are one or more listeners for events of the type represented by
systemEventClass
, call * those listeners,passing source as thesource
of the event. The implementation should be as fast as * possible in determining whether or not a listener for the givensystemEventClass
and *source
has been installed, and should return immediately once such a determination has been made. * The implementation ofpublishEvent
must honor the requirements stated in *{@link #subscribeToEvent(java.lang.Class, java.lang.Class, * javax.faces.event.SystemEventListener)}
**
* The default implementation must implement an algorithm semantically equivalent to the following to locate * listener instances and to invoke them. *
*
*
- If the
* *source
argument implements *{@link javax.faces.event.SystemEventListenerHolder}
, call *{@link javax.faces.event.SystemEventListenerHolder#getListenersForEventClass(java.lang.Class)}
* on it, passing the *systemEventClass
argument. If the list is not empty, perform algorithm *traverseListenerList
on the list.- If any
Application
level listeners have been installed by previous calls totraverseListenerList
on the list. * *
Application
level listeners have been installed by previous calls to
* {@link #subscribeToEvent(java.lang.Class, SystemEventListener)}
, perform algorithm
* traverseListenerList
on the list.
* If the act of invoking the processListener
method causes an
* {@link javax.faces.event.AbortProcessingException} to be thrown,
* processing of the listeners must be aborted.
*
* Algorithm traverseListenerList
: For each listener in the list,
*
* *
-
*
- Call
*
{@link SystemEventListener#isListenerForSource(java.lang.Object)}
, passing the
source
* argument. If this returnsfalse
, take no action on the listener.
*
* - Otherwise, if the event to be passed to the listener instances has not yet been constructed, construct the
* event, passing
source
as the argument to the one-argument constructor that takes an *Object
. This same event instance must be passed to all listener instances.
*
* - Call
*
{@link SystemEvent#isAppropriateListener(javax.faces.event.FacesListener)}
, passing the listener * instance as the argument. If this returns
false
, take no action on the listener.
*
* - Call
{@link SystemEvent#processListener(javax.faces.event.FacesListener)}
, passing the listener * instance.
*
* @param systemEventClass
* - The Class of event that is being published. Must be non-null.
*
* @param source
* - The
source
for the event of type systemEventClass. Must be non- null
, and
* must implement {@link javax.faces.event.SystemEventListenerHolder}
.
*
* @since 2.0
*/
public void publishEvent(FacesContext facesContext, Class extends SystemEvent> systemEventClass, Object source)
{
Application application = getMyfacesApplicationInstance(facesContext);
if (application != null)
{
application.publishEvent(facesContext, systemEventClass, source);
return;
}
throw new UnsupportedOperationException();
}
/**
*
* Remove the argument listener
from the list of ELContextListeners
. If listener
*
is null, no exception is thrown and no action is performed. If listener
is not in the list,
* no exception is thrown and no action is performed.
*
* *
* An implementation is provided that throws UnsupportedOperationException
so that users that decorate
* the Application
continue to work.
*
* @param listener
*/
public void removeELContextListener(ELContextListener listener)
{
Application application = getMyfacesApplicationInstance();
if (application != null)
{
application.removeELContextListener(listener);
return;
}
throw new UnsupportedOperationException();
}
/**
* Set the default {@link ActionListener}
to be registered for all
* {@link javax.faces.component.ActionSource}
* components.
*
* @param listener
* - The new default {@link ActionListener}
*
* @throws NullPointerException
* if listener is null
*/
public abstract void setActionListener(ActionListener listener);
/**
* Set the default Locale
for this application.
*
* @param locale
* - The new default Locale
*
* @throws NullPointerException
* if listener is null
*/
public abstract void setDefaultLocale(Locale locale);
/**
* Return the renderKitId
to be used for rendering this application. If not explicitly set, null
*
is returned.
*
* @param renderKitId
*/
public abstract void setDefaultRenderKitId(String renderKitId);
/**
* Set the fully qualified class name of the ResourceBundle
to be used for JavaServer Faces messages
* for this application. See the JavaDocs for the java.util.ResourceBundle
class for more information
* about the syntax for resource bundle names.
*
* @param bundle
* - Base name of the resource bundle to be used
*
* @throws NullPointerException
* if bundle is null
*/
public abstract void setMessageBundle(String bundle);
/**
* Set the {@link NavigationHandler} instance that will be passed the outcome returned by any invoked application
* action for this web application.
*
* @param handler
* - The new NavigationHandler instance
*/
public abstract void setNavigationHandler(NavigationHandler handler);
/**
* The recommended way to affect the execution of the EL is to provide an Restore View and Render Response
*
phases of the request processing lifecycle.
*
* @param manager The new {@link StateManager}instance
*
* @throws IllegalStateException
* if this method is called after at least one request has been processed by the Lifecycle
* instance for this application.
* @throws NullPointerException
* if manager is null
*/
public abstract void setStateManager(StateManager manager);
/**
* Set the Locale
instances representing the supported Locales
for this application.
*
* @param locales The set of supported Locales
for this application
*
* @throws NullPointerException
* if the argument newLocales is null
.
*
*/
public abstract void setSupportedLocales(CollectionRestore View and Render Response
*
phases of the request processing lifecycle.
*
* @param handler
* - The new {@link ViewHandler} instance
*
* @throws IllegalStateException
* if this method is called after at least one request has been processed by the Lifecycle
* instance for this application.
* @throws NullPointerException
* if handler
is null
*/
public abstract void setViewHandler(ViewHandler handler);
/**
*
* @param systemEventClass
* @param sourceClass
* @param listener
*
* @since 2.0
*/
public void subscribeToEvent(Class extends SystemEvent> systemEventClass, Class> sourceClass,
SystemEventListener listener)
{
Application application = getMyfacesApplicationInstance();
if (application != null)
{
application.subscribeToEvent(systemEventClass, sourceClass, listener);
return;
}
throw new UnsupportedOperationException();
}
/**
*
* @param systemEventClass
* @param listener
*
* @since 2.0
*/
public void subscribeToEvent(Class extends SystemEvent> systemEventClass, SystemEventListener listener)
{
Application application = getMyfacesApplicationInstance();
if (application != null)
{
application.subscribeToEvent(systemEventClass, listener);
return;
}
subscribeToEvent(systemEventClass, null, listener);
}
/**
*
* @param systemEventClass
* @param sourceClass
* @param listener
*
* @since 2.0
*/
public void unsubscribeFromEvent(Class extends SystemEvent> systemEventClass, Class> sourceClass,
SystemEventListener listener)
{
Application application = getMyfacesApplicationInstance();
if (application != null)
{
application.unsubscribeFromEvent(systemEventClass, sourceClass, listener);
return;
}
throw new UnsupportedOperationException();
}
/**
*
* @param systemEventClass
* @param listener
*
* @since 2.0
*/
public void unsubscribeFromEvent(Class extends SystemEvent> systemEventClass, SystemEventListener listener)
{
Application application = getMyfacesApplicationInstance();
if (application != null)
{
application.unsubscribeFromEvent(systemEventClass, listener);
return;
}
unsubscribeFromEvent(systemEventClass, null, listener);
}
}