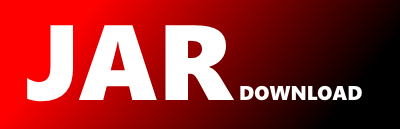
jakarta.faces.context.ExternalContext Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of myfaces-api Show documentation
Show all versions of myfaces-api Show documentation
The public API classes of the Apache MyFaces CORE JSF-2.2 project
The newest version!
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package jakarta.faces.context;
import java.io.IOException;
import java.io.OutputStream;
import java.io.Writer;
import java.util.Iterator;
import java.util.List;
import java.util.Locale;
import java.util.Map;
import java.util.Set;
import jakarta.faces.lifecycle.ClientWindow;
/**
* see Javadoc of Faces Specification
*/
public abstract class ExternalContext
{
public static final String BASIC_AUTH = "BASIC";
public static final String CLIENT_CERT_AUTH = "CLIENT_CERT";
public static final String DIGEST_AUTH = "DIGEST";
public static final String FORM_AUTH = "FORM";
/**
*
* @param name
* @param value
* @param properties
*
* @since 2.0
*/
public void addResponseCookie(String name, String value, Map properties)
{
ExternalContext ctx = _MyFacesExternalContextHelper.firstInstance.get();
if (ctx == null)
{
throw new UnsupportedOperationException();
}
ctx.addResponseCookie(name, value, properties);
}
/**
*
* @param name
* @param value
*
* @since 2.0
*/
public void addResponseHeader(String name, String value)
{
ExternalContext ctx = _MyFacesExternalContextHelper.firstInstance.get();
if (ctx == null)
{
throw new UnsupportedOperationException();
}
ctx.addResponseHeader(name, value);
}
public abstract void dispatch(String path) throws IOException;
public abstract String encodeActionURL(String url);
/**
*
* @param baseUrl
* @param parameters
*
* @since 2.0
*/
public String encodeBookmarkableURL(String baseUrl, Map> parameters)
{
ExternalContext ctx = _MyFacesExternalContextHelper.firstInstance.get();
if (ctx == null)
{
throw new UnsupportedOperationException();
}
return ctx.encodeBookmarkableURL(baseUrl, parameters);
}
public abstract String encodeNamespace(String name);
/**
* @since 2.0
*/
public String encodePartialActionURL(String url)
{
ExternalContext ctx = _MyFacesExternalContextHelper.firstInstance.get();
if (ctx == null)
{
throw new UnsupportedOperationException();
}
return ctx.encodePartialActionURL(url);
}
/**
*
* @param baseUrl
* @param parameters
*
* @since 2.0
*/
public String encodeRedirectURL(String baseUrl, Map> parameters)
{
ExternalContext ctx = _MyFacesExternalContextHelper.firstInstance.get();
if (ctx == null)
{
throw new UnsupportedOperationException();
}
return ctx.encodeRedirectURL(baseUrl, parameters);
}
public abstract String encodeResourceURL(String url);
public abstract Map getApplicationMap();
public abstract String getAuthType();
public abstract Object getContext();
/**
* Returns the name of the underlying context
*
* @return the name or null
*
* @since 2.0
*/
public String getContextName()
{
ExternalContext ctx = _MyFacesExternalContextHelper.firstInstance.get();
if (ctx == null)
{
throw new UnsupportedOperationException();
}
return ctx.getContextName();
}
/**
* @since 2.0
*/
public Flash getFlash()
{
ExternalContext ctx = _MyFacesExternalContextHelper.firstInstance.get();
if (ctx == null)
{
throw new UnsupportedOperationException();
}
return ctx.getFlash();
}
public abstract String getInitParameter(String name);
public abstract Map getInitParameterMap();
/**
* @since Faces 2.0
*/
public String getMimeType(String file)
{
ExternalContext ctx = _MyFacesExternalContextHelper.firstInstance.get();
if (ctx == null)
{
throw new UnsupportedOperationException();
}
return ctx.getMimeType(file);
}
/**
* @since Faces 2.0
*/
public String getRealPath(String path)
{
ExternalContext ctx = _MyFacesExternalContextHelper.firstInstance.get();
if (ctx == null)
{
throw new UnsupportedOperationException();
}
return ctx.getRealPath(path);
}
public abstract String getRemoteUser();
public abstract Object getRequest();
public String getRequestCharacterEncoding()
{
ExternalContext ctx = _MyFacesExternalContextHelper.firstInstance.get();
if (ctx == null)
{
throw new UnsupportedOperationException();
}
return ctx.getRequestCharacterEncoding();
}
/**
*
* @return
*
* @since 2.0
*/
public int getRequestContentLength()
{
ExternalContext ctx = _MyFacesExternalContextHelper.firstInstance.get();
if (ctx == null)
{
throw new UnsupportedOperationException();
}
return ctx.getRequestContentLength();
}
public String getRequestContentType()
{
ExternalContext ctx = _MyFacesExternalContextHelper.firstInstance.get();
if (ctx == null)
{
throw new UnsupportedOperationException();
}
return ctx.getRequestContentType();
}
public abstract String getRequestContextPath();
public abstract Map getRequestCookieMap();
public abstract Map getRequestHeaderMap();
public abstract Map getRequestHeaderValuesMap();
public abstract Locale getRequestLocale();
public abstract Iterator getRequestLocales();
public abstract Map getRequestMap();
public abstract Map getRequestParameterMap();
public abstract Iterator getRequestParameterNames();
public abstract Map getRequestParameterValuesMap();
public abstract String getRequestPathInfo();
/**
* @since Faces 2.0
*/
public String getRequestScheme()
{
ExternalContext ctx = _MyFacesExternalContextHelper.firstInstance.get();
if (ctx == null)
{
throw new UnsupportedOperationException();
}
return ctx.getRequestScheme();
}
/**
* @since Faces 2.0
*/
public String getRequestServerName()
{
ExternalContext ctx = _MyFacesExternalContextHelper.firstInstance.get();
if (ctx == null)
{
throw new UnsupportedOperationException();
}
return ctx.getRequestServerName();
}
/**
* @since Faces 2.0
*/
public int getRequestServerPort()
{
ExternalContext ctx = _MyFacesExternalContextHelper.firstInstance.get();
if (ctx == null)
{
throw new UnsupportedOperationException();
}
return ctx.getRequestServerPort();
}
public abstract String getRequestServletPath();
public abstract java.net.URL getResource(String path) throws java.net.MalformedURLException;
public abstract java.io.InputStream getResourceAsStream(String path);
public abstract Set getResourcePaths(String path);
public abstract Object getResponse();
/**
*
* @return
*
* @since 2.0
*/
public int getResponseBufferSize()
{
ExternalContext ctx = _MyFacesExternalContextHelper.firstInstance.get();
if (ctx == null)
{
throw new UnsupportedOperationException();
}
return ctx.getResponseBufferSize();
}
public String getResponseCharacterEncoding()
{
ExternalContext ctx = _MyFacesExternalContextHelper.firstInstance.get();
if (ctx == null)
{
throw new UnsupportedOperationException("Faces 1.2 : figure out how to tell if this is a Portlet request");
}
return ctx.getResponseCharacterEncoding();
}
/**
* throws UnsupportedOperationException
by default.
*
* @since Faces 1.2
*/
public String getResponseContentType()
{
ExternalContext ctx = _MyFacesExternalContextHelper.firstInstance.get();
if (ctx == null)
{
throw new UnsupportedOperationException();
}
return ctx.getResponseContentType();
}
/**
* @since Faces 2.0
*/
public OutputStream getResponseOutputStream() throws IOException
{
ExternalContext ctx = _MyFacesExternalContextHelper.firstInstance.get();
if (ctx == null)
{
throw new UnsupportedOperationException();
}
return ctx.getResponseOutputStream();
}
/**
* @since Faces 2.0
*/
public Writer getResponseOutputWriter() throws IOException
{
ExternalContext ctx = _MyFacesExternalContextHelper.firstInstance.get();
if (ctx == null)
{
throw new UnsupportedOperationException();
}
return ctx.getResponseOutputWriter();
}
public abstract Object getSession(boolean create);
public abstract Map getSessionMap();
public abstract java.security.Principal getUserPrincipal();
/**
* @since 2.0
*/
public void invalidateSession()
{
ExternalContext ctx = _MyFacesExternalContextHelper.firstInstance.get();
if (ctx == null)
{
throw new UnsupportedOperationException();
}
ctx.invalidateSession();
}
/**
* @since 2.0
*/
public boolean isResponseCommitted()
{
ExternalContext ctx = _MyFacesExternalContextHelper.firstInstance.get();
if (ctx == null)
{
throw new UnsupportedOperationException();
}
return ctx.isResponseCommitted();
}
public abstract boolean isUserInRole(String role);
/**
* @since 2.0
*/
public abstract void log(String message);
/**
* @since 2.0
*/
public abstract void log(String message, Throwable exception);
public abstract void redirect(String url) throws java.io.IOException;
/**
*
* @throws IOException
*
* @since 2.0
*/
public void responseFlushBuffer() throws IOException
{
ExternalContext ctx = _MyFacesExternalContextHelper.firstInstance.get();
if (ctx == null)
{
throw new UnsupportedOperationException();
}
ctx.responseFlushBuffer();
}
/**
*
* @since 2.0
*/
public void responseReset()
{
ExternalContext ctx = _MyFacesExternalContextHelper.firstInstance.get();
if (ctx == null)
{
throw new UnsupportedOperationException();
}
ctx.responseReset();
}
/**
*
* @param statusCode
* @param message
* @throws IOException
*
* @since 2.0
*/
public void responseSendError(int statusCode, String message) throws IOException
{
ExternalContext ctx = _MyFacesExternalContextHelper.firstInstance.get();
if (ctx == null)
{
throw new UnsupportedOperationException();
}
ctx.responseSendError(statusCode, message);
}
/**
* throws UnsupportedOperationException
by default.
*
* @since Faces 1.2
* @param request
*/
public void setRequest(Object request)
{
ExternalContext ctx = _MyFacesExternalContextHelper.firstInstance.get();
if (ctx == null)
{
throw new UnsupportedOperationException();
}
ctx.setRequest(request);
}
/**
* throws UnsupportedOperationException
by default.
*
* @since Faces 1.2
* @param encoding
* @throws java.io.UnsupportedEncodingException
*/
public void setRequestCharacterEncoding(java.lang.String encoding)
throws java.io.UnsupportedEncodingException
{
ExternalContext ctx = _MyFacesExternalContextHelper.firstInstance.get();
if (ctx == null)
{
throw new UnsupportedOperationException();
}
ctx.setRequestCharacterEncoding(encoding);
}
/**
* throws UnsupportedOperationException
by default.
*
* @since Faces 1.2
* @param response
*/
public void setResponse(Object response)
{
ExternalContext ctx = _MyFacesExternalContextHelper.firstInstance.get();
if (ctx == null)
{
throw new UnsupportedOperationException();
}
ctx.setResponse(response);
}
/**
*
* @param size
*
* @since 2.0
*/
public void setResponseBufferSize(int size)
{
ExternalContext ctx = _MyFacesExternalContextHelper.firstInstance.get();
if (ctx == null)
{
throw new UnsupportedOperationException();
}
ctx.setResponseBufferSize(size);
}
/**
* throws UnsupportedOperationException
by default.
*
* @since Faces 1.2
* @param encoding
*/
public void setResponseCharacterEncoding(String encoding)
{
ExternalContext ctx = _MyFacesExternalContextHelper.firstInstance.get();
if (ctx == null)
{
throw new UnsupportedOperationException();
}
ctx.setResponseCharacterEncoding(encoding);
}
/**
*
* @param length
*
* @since 2.0
*/
public void setResponseContentLength(int length)
{
ExternalContext ctx = _MyFacesExternalContextHelper.firstInstance.get();
if (ctx == null)
{
throw new UnsupportedOperationException();
}
ctx.setResponseContentLength(length);
}
/**
*
* @param length
*
* @since 4.1
*/
public void setResponseContentLengthLong(long length)
{
ExternalContext ctx = _MyFacesExternalContextHelper.firstInstance.get();
if (ctx == null)
{
throw new UnsupportedOperationException();
}
ctx.setResponseContentLengthLong(length);
}
/**
*
* @param contentType
*
* @since 2.0
*/
public void setResponseContentType(String contentType)
{
ExternalContext ctx = _MyFacesExternalContextHelper.firstInstance.get();
if (ctx == null)
{
throw new UnsupportedOperationException();
}
ctx.setResponseContentType(contentType);
}
/**
*
* @param name
* @param value
*
* @since 2.0
*/
public void setResponseHeader(String name, String value)
{
ExternalContext ctx = _MyFacesExternalContextHelper.firstInstance.get();
if (ctx == null)
{
throw new UnsupportedOperationException();
}
ctx.setResponseHeader(name, value);
}
/**
*
* @param statusCode
*
* @since 2.0
*/
public void setResponseStatus(int statusCode)
{
ExternalContext ctx = _MyFacesExternalContextHelper.firstInstance.get();
if (ctx == null)
{
throw new UnsupportedOperationException();
}
ctx.setResponseStatus(statusCode);
}
/**
*
* @since 2.1
* @return
*/
public boolean isSecure()
{
ExternalContext ctx = _MyFacesExternalContextHelper.firstInstance.get();
if (ctx == null)
{
throw new UnsupportedOperationException();
}
return ctx.isSecure();
}
/**
*
* @since 2.1
* @return
*/
public int getSessionMaxInactiveInterval()
{
ExternalContext ctx = _MyFacesExternalContextHelper.firstInstance.get();
if (ctx == null)
{
throw new UnsupportedOperationException();
}
return ctx.getSessionMaxInactiveInterval();
}
/**
*
* @since 2.1
* @param interval
*/
public void setSessionMaxInactiveInterval(int interval)
{
ExternalContext ctx = _MyFacesExternalContextHelper.firstInstance.get();
if (ctx == null)
{
throw new UnsupportedOperationException();
}
ctx.setSessionMaxInactiveInterval(interval);
}
/**
* @since 2.2
* @return
*/
public ClientWindow getClientWindow()
{
ExternalContext ctx = _MyFacesExternalContextHelper.firstInstance.get();
if (ctx == null)
{
throw new UnsupportedOperationException();
}
return ctx.getClientWindow();
}
/**
* @since 2.2
* @param window
*/
public void setClientWindow(ClientWindow window)
{
ExternalContext ctx = _MyFacesExternalContextHelper.firstInstance.get();
if (ctx == null)
{
throw new UnsupportedOperationException();
}
ctx.setClientWindow(window);
}
/**
* @since 2.2
* @param create
* @return
*/
public String getSessionId(boolean create)
{
ExternalContext ctx = _MyFacesExternalContextHelper.firstInstance.get();
if (ctx == null)
{
throw new UnsupportedOperationException();
}
return ctx.getSessionId(create);
}
/**
* @since 2.2
* @return
*/
public String getApplicationContextPath()
{
ExternalContext ctx = _MyFacesExternalContextHelper.firstInstance.get();
if (ctx == null)
{
throw new UnsupportedOperationException();
}
return ctx.getApplicationContextPath();
}
/**
* @since 2.3
* @param url
* @return
*/
public abstract String encodeWebsocketURL(String url);
/**
* @since 4.0
*/
public abstract void release();
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy