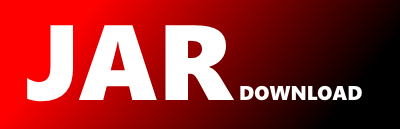
org.apache.myfaces.renderkit.html.util.DefaultAddResource Maven / Gradle / Ivy
Show all versions of tomahawk Show documentation
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package org.apache.myfaces.renderkit.html.util;
import org.apache.commons.lang.builder.EqualsBuilder;
import org.apache.commons.lang.builder.HashCodeBuilder;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import org.apache.myfaces.shared_tomahawk.config.MyfacesConfig;
import org.apache.myfaces.shared_tomahawk.renderkit.html.HTML;
import org.apache.myfaces.shared_tomahawk.renderkit.html.HtmlRendererUtils;
import org.apache.myfaces.shared_tomahawk.renderkit.html.HtmlResponseWriterImpl;
import org.apache.myfaces.shared_tomahawk.util.ClassUtils;
import javax.faces.context.FacesContext;
import javax.faces.context.ResponseWriter;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
import java.util.Iterator;
import java.util.LinkedHashSet;
import java.util.Set;
/**
* This is a utility class to render link to resources used by custom components.
* Mostly used to avoid having to include [script src="..."][/script]
* in the head of the pages before using a component.
*
* When used together with the ExtensionsFilter, this class can allow components
* in the body of a page to emit script and stylesheet references into the page
* head section. The relevant methods on this object simply queue the changes,
* and when the page is complete the ExtensionsFilter calls back into this
* class to allow it to insert the commands into the buffered response.
*
* This class also works with the ExtensionsFilter to allow components to
* emit references to javascript/css/etc which are bundled in the component's
* jar file. Special URLs are generated which the ExtensionsFilter will later
* handle by retrieving the specified resource from the classpath.
*
* The special URL format is:
*
* {contextPath}/faces/myFacesExtensionResource/
* {resourceLoaderName}/{cacheKey}/{resourceURI}
*
* Where:
*
* - {contextPath} is the context path of the current webapp
*
- {resourceLoaderName} is the fully-qualified name of a class which
* implements the ResourceLoader interface. When a browser app sends a request
* for the specified resource, an instance of the specified ResourceLoader class
* will be created and passed the resourceURI part of the URL for resolving to the
* actual resource to be served back. The standard MyFaces ResourceLoader
* implementation only serves resources for files stored beneath path
* org/apache/myfaces/custom in the classpath but non-myfaces code can provide their
* own ResourceLoader implementations.
*
*
* @author Sylvain Vieujot (latest modification by $Author: mmarinschek $)
* @version $Revision: 371739 373827 $ $Date: 2006-01-31 14:50:35 +0000 (Tue, 31 Jan 2006) $
*/
public class DefaultAddResource extends NonBufferingAddResource
{
protected Log log = LogFactory.getLog(DefaultAddResource.class);
protected StringBuffer originalResponse;
private Set headerBeginInfo;
private Set bodyEndInfo;
private Set bodyOnloadInfo;
protected boolean parserCalled = false;
protected int headerInsertPosition = -1;
protected int bodyInsertPosition = -1;
protected int beforeBodyPosition = -1;
protected int afterBodyContentInsertPosition = -1;
protected int beforeBodyEndPosition = -1;
protected DefaultAddResource()
{
}
// Methods to add resources
/**
* Adds the given Javascript resource to the document header at the specified
* document positioy by supplying a resourcehandler instance.
*
* Use this method to have full control about building the reference url
* to identify the resource and to customize how the resource is
* written to the response. In most cases, however, one of the convenience
* methods on this class can be used without requiring a custom ResourceHandler
* to be provided.
*
* If the script has already been referenced, it's added only once.
*
* Note that this method queues the javascript for insertion, and that
* the script is inserted into the buffered response by the ExtensionsFilter
* after the page is complete.
*/
public void addJavaScriptAtPosition(FacesContext context, ResourcePosition position,
ResourceHandler resourceHandler)
{
addJavaScriptAtPosition(context, position, resourceHandler, false);
}
/**
* Insert a [script src="url"] entry into the document header at the
* specified document position. If the script has already been
* referenced, it's added only once.
*
* The resource is expected to be in the classpath, at the same location as the
* specified component + "/resource".
*
* Example: when customComponent is class example.Widget, and
* resourceName is script.js, the resource will be retrieved from
* "example/Widget/resource/script.js" in the classpath.
*/
public void addJavaScriptAtPosition(FacesContext context, ResourcePosition position,
Class myfacesCustomComponent, String resourceName)
{
addJavaScriptAtPosition(context, position, new MyFacesResourceHandler(
myfacesCustomComponent, resourceName));
}
public void addJavaScriptAtPositionPlain(FacesContext context, ResourcePosition position, Class myfacesCustomComponent, String resourceName)
{
addJavaScriptAtPosition(context, position,
new MyFacesResourceHandler(myfacesCustomComponent, resourceName),
false, false);
}
/**
* Insert a [script src="url"] entry into the document header at the
* specified document position. If the script has already been
* referenced, it's added only once.
*
* @param defer specifies whether the html attribute "defer" is set on the
* generated script tag. If this is true then the browser will continue
* processing the html page without waiting for the specified script to
* load and be run.
*/
public void addJavaScriptAtPosition(FacesContext context, ResourcePosition position,
Class myfacesCustomComponent, String resourceName, boolean defer)
{
addJavaScriptAtPosition(context, position, new MyFacesResourceHandler(
myfacesCustomComponent, resourceName), defer);
}
/**
* Insert a [script src="url"] entry into the document header at the
* specified document position. If the script has already been
* referenced, it's added only once.
*
* @param uri is the location of the desired resource, relative to the base
* directory of the webapp (ie its contextPath).
*/
public void addJavaScriptAtPosition(FacesContext context, ResourcePosition position, String uri)
{
addJavaScriptAtPosition(context, position, uri, false);
}
/**
* Adds the given Javascript resource at the specified document position.
* If the script has already been referenced, it's added only once.
*/
public void addJavaScriptAtPosition(FacesContext context, ResourcePosition position, String uri,
boolean defer)
{
addPositionedInfo(position, getScriptInstance(context, uri, defer));
}
public void addJavaScriptToBodyTag(FacesContext context, String javascriptEventName,
String addedJavaScript)
{
AttributeInfo info = new AttributeInfo();
info.setAttributeName(javascriptEventName);
info.setAttributeValue(addedJavaScript);
addPositionedInfo(BODY_ONLOAD, info);
}
/**
* Adds the given Javascript resource at the specified document position.
* If the script has already been referenced, it's added only once.
*/
public void addJavaScriptAtPosition(FacesContext context, ResourcePosition position,
ResourceHandler resourceHandler, boolean defer)
{
validateResourceHandler(resourceHandler);
addPositionedInfo(position, getScriptInstance(context, resourceHandler, defer));
}
private void addJavaScriptAtPosition(FacesContext context, ResourcePosition position,
ResourceHandler resourceHandler, boolean defer, boolean encodeUrl)
{
validateResourceHandler(resourceHandler);
addPositionedInfo(position, getScriptInstance(context, resourceHandler, defer, encodeUrl));
}
/**
* Adds the given Style Sheet at the specified document position.
* If the style sheet has already been referenced, it's added only once.
*/
public void addStyleSheet(FacesContext context, ResourcePosition position,
Class myfacesCustomComponent, String resourceName)
{
addStyleSheet(context, position, new MyFacesResourceHandler(myfacesCustomComponent,
resourceName));
}
/**
* Adds the given Style Sheet at the specified document position.
* If the style sheet has already been referenced, it's added only once.
*/
public void addStyleSheet(FacesContext context, ResourcePosition position, String uri)
{
addPositionedInfo(position, getStyleInstance(context, uri));
}
/**
* Adds the given Style Sheet at the specified document position.
* If the style sheet has already been referenced, it's added only once.
*/
public void addStyleSheet(FacesContext context, ResourcePosition position,
ResourceHandler resourceHandler)
{
validateResourceHandler(resourceHandler);
addPositionedInfo(position, getStyleInstance(context, resourceHandler));
}
/**
* Adds the given Inline Style at the specified document position.
*/
public void addInlineStyleAtPosition(FacesContext context, ResourcePosition position, String inlineStyle)
{
addPositionedInfo(position, getInlineStyleInstance(inlineStyle));
}
/**
* Adds the given Inline Script at the specified document position.
*/
public void addInlineScriptAtPosition(FacesContext context, ResourcePosition position,
String inlineScript)
{
addPositionedInfo(position, getInlineScriptInstance(inlineScript));
}
// Positioned stuffs
protected Set getHeaderBeginInfos()
{
if (headerBeginInfo == null)
{
headerBeginInfo = new LinkedHashSet();
}
return headerBeginInfo;
}
protected Set getBodyEndInfos()
{
if (bodyEndInfo == null)
{
bodyEndInfo = new LinkedHashSet();
}
return bodyEndInfo;
}
protected Set getBodyOnloadInfos()
{
if (bodyOnloadInfo == null)
{
bodyOnloadInfo = new LinkedHashSet();
}
return bodyOnloadInfo;
}
private void addPositionedInfo(ResourcePosition position, PositionedInfo info)
{
if (HEADER_BEGIN.equals(position))
{
Set set = getHeaderBeginInfos();
set.add(info);
}
else if (BODY_END.equals(position))
{
Set set = getBodyEndInfos();
set.add(info);
}
else if (BODY_ONLOAD.equals(position))
{
Set set = getBodyOnloadInfos();
set.add(info);
}
}
public boolean hasHeaderBeginInfos()
{
return headerBeginInfo != null;
}
/**
* Parses the response to mark the positions where code will be inserted
*/
public void parseResponse(HttpServletRequest request, String bufferedResponse,
HttpServletResponse response)
{
originalResponse = new StringBuffer(bufferedResponse);
ParseCallbackListener l = new ParseCallbackListener();
ReducedHTMLParser.parse(originalResponse, l);
headerInsertPosition = l.getHeaderInsertPosition();
bodyInsertPosition = l.getBodyInsertPosition();
beforeBodyPosition = l.getBeforeBodyPosition();
afterBodyContentInsertPosition = l.getAfterBodyContentInsertPosition();
beforeBodyEndPosition = l.getAfterBodyEndPosition() - 7; // 7, which is the length of