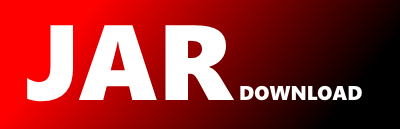
org.apache.myfaces.trinidad.component.UIXGroup Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of trinidad-api Show documentation
Show all versions of trinidad-api Show documentation
Public API for the Apache MyFaces Trinidad project
The newest version!
// WARNING: This file was automatically generated. Do not edit it directly,
// or you will lose your changes.
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package org.apache.myfaces.trinidad.component;
import java.io.IOException;
import java.util.List;
import javax.faces.component.UIComponent;
import javax.faces.context.FacesContext;
import org.apache.myfaces.trinidad.bean.FacesBean;
import org.apache.myfaces.trinidad.bean.PropertyKey;
import org.apache.myfaces.trinidad.util.ComponentUtils;
/**
*
* The group component is an invisible control that aggregates semantically-related children; the group itself has no associated client representation (visual or API).
* Some parent components may have special representation for groups like adding separators around the group but this is a special case and is not always rendered this way.
* In most cases, only the children of the group will be rendered directly to the page.
* There will be no layout applied to the children so the natural layout behavior of the underlying HTML elements will apply.
* If you require a more predictable layout, you should use a layout component such as panelGroupLayout.
*
* Events:
*
*
* Type
* Phases
* Description
*
*
* org.apache.myfaces.trinidad.event.AttributeChangeEvent
* Invoke
Application
Apply
Request
Values
* Event delivered to describe an attribute change. Attribute change events are not delivered for any programmatic change to a property. They are only delivered when a renderer changes a property without the application's specific request. An example of an attribute change event might include the width of a column that supported client-side resizing.
*
*
*/
public class UIXGroup extends UIXComponentBase
implements FlattenedComponent
{
static public final String START_BOUNDARY_SHOW = "show";
static public final String START_BOUNDARY_HIDE = "hide";
static public final String START_BOUNDARY_DONT_CARE = "dontCare";
static public final String END_BOUNDARY_SHOW = "show";
static public final String END_BOUNDARY_HIDE = "hide";
static public final String END_BOUNDARY_DONT_CARE = "dontCare";
static public final FacesBean.Type TYPE = new FacesBean.Type(
UIXComponentBase.TYPE);
static public final PropertyKey START_BOUNDARY_KEY =
TYPE.registerKey("startBoundary", String.class, "dontCare");
static public final PropertyKey END_BOUNDARY_KEY =
TYPE.registerKey("endBoundary", String.class, "dontCare");
static public final PropertyKey TITLE_KEY =
TYPE.registerKey("title", String.class);
static public final String COMPONENT_FAMILY =
"org.apache.myfaces.trinidad.Group";
static public final String COMPONENT_TYPE =
"org.apache.myfaces.trinidad.Group";
/**
* Construct an instance of the UIXGroup.
*/
public UIXGroup()
{
super(null);
}
/**
* Overridden to return true.
* @return true because the children are rendered by this component
*/
@Override
public boolean getRendersChildren()
{
return true;
}
/**
* Sets up the grouping context and processes all of the
* UIXGroup's children
*/
public boolean processFlattenedChildren(
FacesContext context,
ComponentProcessingContext cpContext,
ComponentProcessor childProcessor,
S callBackContext
) throws IOException
{
cpContext.pushGroup();
try
{
setupFlattenedContext(context, cpContext);
try
{
setupFlattenedChildrenContext(context, cpContext);
try
{
// bump up the group depth and render all of the children
return UIXComponent.processFlattenedChildren(context,
cpContext,
childProcessor,
this.getChildren(),
callBackContext);
}
finally
{
tearDownFlattenedChildrenContext(context, cpContext);
}
}
finally
{
tearDownFlattenedContext(context, cpContext);
}
}
finally
{
cpContext.popGroup();
}
}
/**
* Returns true
if this FlattenedComponent is currently flattening its children
* @param context FacesContext
* @return true
if this FlattenedComponent is currently flattening its children
*/
public boolean isFlatteningChildren(FacesContext context)
{
return true;
}
/**
* Renders the children in their raw form.
* There is no Renderer for this component because it has no
* visual representation or any sort of layout for its children.
* @param context the FacesContext
* @throws IOException if there is an error encoding the children
*/
@Override
public void encodeChildren(FacesContext context) throws IOException
{
if (context == null)
throw new NullPointerException();
if (!isRendered())
return;
if (getChildCount() > 0)
{
for(UIComponent child : (List)getChildren())
{
child.encodeAll(context);
}
}
}
/**
* Gets
* indicates if a visual group start boundary is desired. The default value of 'dontCare'
* indicates no preference. A value of 'show' indicates a preference to show a start boundary.
* A value of 'hide' indicates a preference to not show a start boundary. Regardless of the
* start boundary value, whether a visual boundary will be displayed is up to the group's
* parent component.
*
*
* @return the new startBoundary value
*/
final public String getStartBoundary()
{
return ComponentUtils.resolveString(getProperty(START_BOUNDARY_KEY), "dontCare");
}
/**
* Sets
* indicates if a visual group start boundary is desired. The default value of 'dontCare'
* indicates no preference. A value of 'show' indicates a preference to show a start boundary.
* A value of 'hide' indicates a preference to not show a start boundary. Regardless of the
* start boundary value, whether a visual boundary will be displayed is up to the group's
* parent component.
*
*
* @param startBoundary the new startBoundary value
*/
final public void setStartBoundary(String startBoundary)
{
setProperty(START_BOUNDARY_KEY, (startBoundary));
}
/**
* Gets
* indicates if a visual group end boundary is desired. The default value of 'dontCare'
* indicates no preference. A value of 'show' indicates a preference to show an end boundary.
* A value of 'hide' indicates a preference to not show an end boundary. Regardless of the
* end boundary value, whether a visual boundary will be displayed is up to the group's
* parent component.
*
*
* @return the new endBoundary value
*/
final public String getEndBoundary()
{
return ComponentUtils.resolveString(getProperty(END_BOUNDARY_KEY), "dontCare");
}
/**
* Sets
* indicates if a visual group end boundary is desired. The default value of 'dontCare'
* indicates no preference. A value of 'show' indicates a preference to show an end boundary.
* A value of 'hide' indicates a preference to not show an end boundary. Regardless of the
* end boundary value, whether a visual boundary will be displayed is up to the group's
* parent component.
*
*
* @param endBoundary the new endBoundary value
*/
final public void setEndBoundary(String endBoundary)
{
setProperty(END_BOUNDARY_KEY, (endBoundary));
}
/**
* Gets
* a title value for the group. Whether anything is done with this title value is up to the
* group's parent component.
*
*
* @return the new title value
*/
final public String getTitle()
{
return ComponentUtils.resolveString(getProperty(TITLE_KEY));
}
/**
* Sets
* a title value for the group. Whether anything is done with this title value is up to the
* group's parent component.
*
*
* @param title the new title value
*/
final public void setTitle(String title)
{
setProperty(TITLE_KEY, (title));
}
@Override
public String getFamily()
{
return COMPONENT_FAMILY;
}
@Override
protected FacesBean.Type getBeanType()
{
return TYPE;
}
/**
* Construct an instance of the UIXGroup.
*/
protected UIXGroup(
String rendererType
)
{
super(rendererType);
}
static
{
TYPE.lock();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy