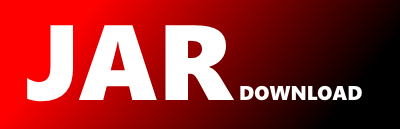
org.apache.myfaces.trinidadinternal.ui.MutableUINode Maven / Gradle / Ivy
Show all versions of trinidad-impl Show documentation
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package org.apache.myfaces.trinidadinternal.ui;
/**
* MutableUINode extends UINode to add mutability. Most WebBeans will
* inherit from this class, but Renderers only see that immutable
* UINode interface.
*
* The indexed children manipulation methods--addIndexedChild
,
* removeIndexedChild
, and clearIndexedChildren
.
* follow the same semantics as the indexed methods from the Java Collections
* List and Collection classes respectively.
*
* @version $Name: $ ($Revision: adfrt/faces/adf-faces-impl/src/main/java/oracle/adfinternal/view/faces/ui/MutableUINode.java#0 $) $Date: 10-nov-2005.18:50:14 $
* @deprecated This class comes from the old Java 1.2 UIX codebase and should not be used anymore.
*/
@Deprecated
public interface MutableUINode extends UINode
{
/**
* Inserts an indexed child to the node. Shifts the element currently at
* that position, if any, and any subsequent elements to the right
* (adds one to their indices). Children may be added to the end of the
* list of indexed children with the second version of addIndexedChild().
*
* Unlike many other APIs, adding a child does not remove it from any other
* parent nodes. In fact, the same child UINode instance is allowed to
* appear in multiple different indices of the same parent UINode.
*
* @param childIndex the zero-based index to add the child at.
* @param child the new child node
*
* @see #removeIndexedChild
* @see #clearIndexedChildren
* @see java.util.List#add
*/
public void addIndexedChild(
int childIndex,
UINode child);
/**
* Inserts an indexed child to the node, placing after all other nodes.
*
* Unlike many other APIs, adding a child does not remove it from any other
* parent nodes. In fact, the same child UINode instance is allowed to
* appear in multiple different indices of the same parent UINode.
*
* @param child the new child node
*
* @see #removeIndexedChild
* @see #clearIndexedChildren
* @see java.util.List#add
*/
public void addIndexedChild(UINode child);
/**
* Removes an indexed child from the node.
* @param childIndex the zero-based index of the child to remove
*
* @see #clearIndexedChildren
* @see java.util.List#remove
*/
public UINode removeIndexedChild(int childIndex);
/**
* Removes all of the indexed children.
*
* Although this method could be implemented in terms of
* removeIndexedChild
, it is present on this interface in
* order to allow for more efficient implementations.
*
* @see #removeIndexedChild
* @see java.util.Collection#clear
*/
public void clearIndexedChildren();
/**
* Replaces a single child.
*
* Although this method could be implemented in terms of
* addIndexedChild
and removeIndexedChild
,
* it is present on this interface in
* order to allow for more efficient implementations.
*
* @param childIndex the zero-based index to add the child at.
* @param child the new child node
*
* @see #removeIndexedChild
*/
public void replaceIndexedChild(
int childIndex,
UINode child);
/**
* Sets a named child on the node. Any node attached with
* that name will be removed.
*
* @param childName the name of the child
* @param namedChild the child; passing null will remove any existing
* UINode with that name.
*/
public void setNamedChild(
String childName,
UINode namedChild);
/**
* Sets an attribute value of the node.
* @param name the name of the attribute
* @param value the new value; passing null will remove any
* existing attribute with that name.
*/
public void setAttributeValue(
AttributeKey attrKey,
Object value);
/**
* Sets the page-wide unique client ID of this node. The string set
* must comply with the
*
* XML id specification--namely it must begin
* with a [a-z][A-z] and after that can contain as many of
* [a-z][A-Z][0-9][._-:] as desired.
*
* This property is typically only needed when writing client-side
* JavaScript.
*
*
* This method is only present on this interface for backwards compatibility
* and will be removed from this interface in a future version of UIX Components
* and moved to org.apache.myfaces.trinidadinternal.ui.beans.BaseWebBean
*
*/
public void setID(String id);
}