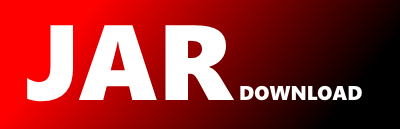
org.apache.nifi.nar.ExtensionMapping Maven / Gradle / Ivy
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.nifi.nar;
import org.apache.nifi.bundle.BundleCoordinate;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Map;
import java.util.Set;
import java.util.function.BiFunction;
public class ExtensionMapping {
private final Map> processorNames = new HashMap<>();
private final Map> controllerServiceNames = new HashMap<>();
private final Map> reportingTaskNames = new HashMap<>();
private final Map> flowAnalysisRuleNames = new HashMap<>();
private final Map> parameterProviderNames = new HashMap<>();
private final Map> flowRegistryClientNames = new HashMap<>();
private final BiFunction, Set, Set> merger = (oldValue, newValue) -> {
final Set merged = new HashSet<>();
merged.addAll(oldValue);
merged.addAll(newValue);
return merged;
};
void addProcessor(final BundleCoordinate coordinate, final String processorName) {
processorNames.computeIfAbsent(processorName, name -> new HashSet<>()).add(coordinate);
}
void addAllProcessors(final BundleCoordinate coordinate, final Collection processorNames) {
processorNames.forEach(name -> {
addProcessor(coordinate, name);
});
}
void addControllerService(final BundleCoordinate coordinate, final String controllerServiceName) {
controllerServiceNames.computeIfAbsent(controllerServiceName, name -> new HashSet<>()).add(coordinate);
}
void addAllControllerServices(final BundleCoordinate coordinate, final Collection controllerServiceNames) {
controllerServiceNames.forEach(name -> {
addControllerService(coordinate, name);
});
}
void addReportingTask(final BundleCoordinate coordinate, final String reportingTaskName) {
reportingTaskNames.computeIfAbsent(reportingTaskName, name -> new HashSet<>()).add(coordinate);
}
void addAllReportingTasks(final BundleCoordinate coordinate, final Collection reportingTaskNames) {
reportingTaskNames.forEach(name -> {
addReportingTask(coordinate, name);
});
}
void addFlowAnalysisRule(final BundleCoordinate coordinate, final String flowAnalysisRuleName) {
flowAnalysisRuleNames.computeIfAbsent(flowAnalysisRuleName, name -> new HashSet<>()).add(coordinate);
}
void addAllFlowAnalysisRules(final BundleCoordinate coordinate, final Collection flowAnalysisRuleNames) {
flowAnalysisRuleNames.forEach(name -> {
addFlowAnalysisRule(coordinate, name);
});
}
void addParameterProvider(final BundleCoordinate coordinate, final String parameterProviderName) {
parameterProviderNames.computeIfAbsent(parameterProviderName, name -> new HashSet<>()).add(coordinate);
}
void addAllParameterProviders(final BundleCoordinate coordinate, final Collection parameterProviderNames) {
parameterProviderNames.forEach(name -> {
addParameterProvider(coordinate, name);
});
}
void addFlowRegistryClient(final BundleCoordinate coordinate, final String flowRegistryClientName) {
parameterProviderNames.computeIfAbsent(flowRegistryClientName, name -> new HashSet<>()).add(coordinate);
}
void addAllFlowRegistryClients(final BundleCoordinate coordinate, final Collection flowRegistryClientNames) {
flowRegistryClientNames.forEach(name -> {
addFlowRegistryClient(coordinate, name);
});
}
void merge(final ExtensionMapping other) {
other.getProcessorNames().forEach((name, otherCoordinates) -> {
processorNames.merge(name, otherCoordinates, merger);
});
other.getControllerServiceNames().forEach((name, otherCoordinates) -> {
controllerServiceNames.merge(name, otherCoordinates, merger);
});
other.getReportingTaskNames().forEach((name, otherCoordinates) -> {
reportingTaskNames.merge(name, otherCoordinates, merger);
});
other.getFlowAnalysisRuleNames().forEach((name, otherCoordinates) -> {
flowAnalysisRuleNames.merge(name, otherCoordinates, merger);
});
other.getParameterProviderNames().forEach((name, otherCoordinates) -> {
parameterProviderNames.merge(name, otherCoordinates, merger);
});
other.getFlowRegistryClientNames().forEach((name, otherCoordinates) -> {
flowRegistryClientNames.merge(name, otherCoordinates, merger);
});
}
public Map> getProcessorNames() {
return Collections.unmodifiableMap(processorNames);
}
public Map> getControllerServiceNames() {
return Collections.unmodifiableMap(controllerServiceNames);
}
public Map> getReportingTaskNames() {
return Collections.unmodifiableMap(reportingTaskNames);
}
public Map> getFlowAnalysisRuleNames() {
return Collections.unmodifiableMap(flowAnalysisRuleNames);
}
public Map> getParameterProviderNames() {
return Collections.unmodifiableMap(parameterProviderNames);
}
public Map> getFlowRegistryClientNames() {
return Collections.unmodifiableMap(flowRegistryClientNames);
}
public Map> getAllExtensionNames() {
final Map> extensionNames = new HashMap<>();
extensionNames.putAll(processorNames);
extensionNames.putAll(controllerServiceNames);
extensionNames.putAll(reportingTaskNames);
extensionNames.putAll(flowAnalysisRuleNames);
extensionNames.putAll(parameterProviderNames);
extensionNames.putAll(flowRegistryClientNames);
return extensionNames;
}
public int size() {
int size = 0;
for (final Set coordinates : processorNames.values()) {
size += coordinates.size();
}
for (final Set coordinates : controllerServiceNames.values()) {
size += coordinates.size();
}
for (final Set coordinates : reportingTaskNames.values()) {
size += coordinates.size();
}
for (final Set coordinates : flowAnalysisRuleNames.values()) {
size += coordinates.size();
}
for (final Set coordinates : parameterProviderNames.values()) {
size += coordinates.size();
}
for (final Set coordinates : flowRegistryClientNames.values()) {
size += coordinates.size();
}
return size;
}
public boolean isEmpty() {
return processorNames.isEmpty()
&& controllerServiceNames.isEmpty()
&& reportingTaskNames.isEmpty()
&& flowAnalysisRuleNames.isEmpty()
&& parameterProviderNames.isEmpty()
&& flowRegistryClientNames.isEmpty();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy