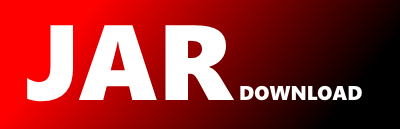
org.apache.ode.utils.stl.CollectionsX Maven / Gradle / Ivy
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package org.apache.ode.utils.stl;
import java.util.*;
/**
* Useful extensions to the java.util.Collections class.
*/
public class CollectionsX {
public static UnaryFunction ufnMapEntry_getKey = new UnaryFunction() {
public Object apply(Map.Entry x) {
return x.getKey();
}
};
public static UnaryFunction ufnMapEntry_getValue = new UnaryFunction() {
public Object apply(Map.Entry x) {
return x.getValue();
}
};
public static void apply(Collection coll, UnaryFunction f) {
apply(coll.iterator(), f);
}
public static void apply(Iterator i, UnaryFunction f) {
while (i.hasNext()) {
f.apply(i.next());
}
}
public static void apply(Collection coll, UnaryFunctionEx f) throws Exception {
apply(coll.iterator(), f);
}
public static void apply(Iterator i, UnaryFunctionEx f) throws Exception {
while (i.hasNext()) {
f.apply(i.next());
}
}
/**
* Find an element in a colletion satisfying a condition. The condition is
* given in the form of a unary function which returns a non-false
* value when the condition is satisfied. The first object in the collection
* matching the condition is returned.
*
* @param coll
* the collection to search through
* @param f
* the test to apply to the collection elements
*
* @return the first object in the collection (coll) which, satisfies the
* condition (f)
*/
public static T find_if(Collection coll, MemberOfFunction super T> f) {
return find_if(coll.iterator(), f);
}
/**
* Find an element in a collection satisfying a condition.
*
* @param i
* the iterator to iterate with
* @param f
* the test to apply to the elements
*
* @return the first object enumerated by the iterator (i) which satisfies
* the condition (f)
*
* @see #find_if(java.util.Collection,
* org.apache.ode.utils.stl.MemberOfFunction)
*/
public static T find_if(Iterator i, MemberOfFunction super T> f) {
while (i.hasNext()) {
T x = i.next();
if (f.isMember(x)) {
return x;
}
}
return null;
}
public static Collection insert(Collection coll, Enumeration extends T> e) {
while (e.hasMoreElements()) {
coll.add(e.nextElement());
}
return coll;
}
public static Collection insert(Collection coll, Iterator extends T> i) {
while (i.hasNext()) {
coll.add(i.next());
}
return coll;
}
public static Collection insert(Collection coll, Collection extends T> src) {
return insert(coll, src.iterator());
}
/**
* Remove elements from collection based on the results of specified unary
* function. An element will be deleted if f.isMember(element)
*
* returns true
. So: coll' = { x : x el-of coll
* AND f(x) == false }
*
* @param coll
* the collection from which to remove elements
* @param f
* the function to apply
*
* @return coll, for convenience
*/
public static Collection remove_if(Collection coll, MemberOfFunction f) {
Iterator i = coll.iterator();
while (i.hasNext()) {
if (f.isMember(i.next())) {
i.remove();
}
}
return coll;
}
/**
* Transform a collection with a unary function. Roughly speaking dest = {
* f(a) : a el-of src }
*
* @param dest
* the empty (mutable) collection to transform into
* @param src
* the collection to transform from
* @param f
* the unary function to apply
*
* @return dest, for convenience
*/
public static , T, V extends T, E> C transform(C dest, Collection src,
UnaryFunction f) {
Iterator i = src.iterator();
while (i.hasNext()) {
dest.add(f.apply(i.next()));
}
return dest;
}
public static , T, V extends T, E> C transformEx(C dest, Collection src,
UnaryFunctionEx f) throws Exception {
Iterator i = src.iterator();
while (i.hasNext()) {
dest.add(f.apply(i.next()));
}
return dest;
}
public static , T, V extends T, E> C transform(C dest, Enumeration i,
UnaryFunction f) {
while (i.hasMoreElements()) {
dest.add(f.apply(i.nextElement()));
}
return dest;
}
public static , T, S extends T> C filter(C dest, Collection source,
MemberOfFunction function) {
return filter(dest, source.iterator(), function);
}
public static , T, S extends T> C filter(C dest, Iterator source,
MemberOfFunction function) {
while (source.hasNext()) {
S next = source.next();
if (function.isMember(next)) {
dest.add(next);
}
}
return dest;
}
public static , S, T extends S> C filter(C dest, Collection src, Class t) {
return filter(dest, src.iterator(), t);
}
public static , S, T extends S> C filter(C newList, Iterator iterator, Class t) {
while (iterator.hasNext()) {
S next = iterator.next();
if (t.isAssignableFrom(next.getClass())) {
newList.add((T) next);
}
}
return newList;
}
/**
* Filter a collection by member class.
*
* @param src
* source collection
* @param aClass
* requested class
* @return collection consisting of the members of the input that are
* assignable to the given class
*/
@SuppressWarnings("unchecked")
public static Collection filter(Collection src, final Class aClass) {
return filter(new ArrayList(src.size()), src.iterator(), aClass);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy