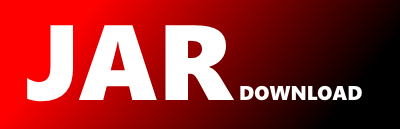
org.odftoolkit.odfdom.dom.element.style.StyleDrawingPagePropertiesElement Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of odfdom-java Show documentation
Show all versions of odfdom-java Show documentation
ODFDOM is an OpenDocument Format (ODF) framework. Its purpose
is to provide an easy common way to create, access and
manipulate ODF files, without requiring detailed knowledge of
the ODF specification. It is designed to provide the ODF
developer community with an easy lightwork programming API
portable to any object-oriented language.
The current reference implementation is written in Java.
/************************************************************************
*
* DO NOT ALTER OR REMOVE COPYRIGHT NOTICES OR THIS FILE HEADER
*
* Copyright 2008, 2010 Oracle and/or its affiliates. All rights reserved.
*
* Use is subject to license terms.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy
* of the License at http://www.apache.org/licenses/LICENSE-2.0. You can also
* obtain a copy of the License at http://odftoolkit.org/docs/license.txt
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
*
* See the License for the specific language governing permissions and
* limitations under the License.
*
************************************************************************/
/*
* This file is automatically generated.
* Don't edit manually.
*/
package org.odftoolkit.odfdom.dom.element.style;
import org.odftoolkit.odfdom.pkg.OdfElement;
import org.odftoolkit.odfdom.dom.style.props.OdfStyleProperty;
import org.odftoolkit.odfdom.dom.style.props.OdfStylePropertiesSet;
import org.odftoolkit.odfdom.pkg.ElementVisitor;
import org.odftoolkit.odfdom.pkg.OdfFileDom;
import org.odftoolkit.odfdom.pkg.OdfName;
import org.odftoolkit.odfdom.dom.OdfDocumentNamespace;
import org.odftoolkit.odfdom.dom.DefaultElementVisitor;
import org.odftoolkit.odfdom.dom.element.presentation.PresentationSoundElement;
import org.odftoolkit.odfdom.dom.attribute.draw.DrawBackgroundSizeAttribute;
import org.odftoolkit.odfdom.dom.attribute.draw.DrawFillAttribute;
import org.odftoolkit.odfdom.dom.attribute.draw.DrawFillColorAttribute;
import org.odftoolkit.odfdom.dom.attribute.draw.DrawFillGradientNameAttribute;
import org.odftoolkit.odfdom.dom.attribute.draw.DrawFillHatchNameAttribute;
import org.odftoolkit.odfdom.dom.attribute.draw.DrawFillHatchSolidAttribute;
import org.odftoolkit.odfdom.dom.attribute.draw.DrawFillImageHeightAttribute;
import org.odftoolkit.odfdom.dom.attribute.draw.DrawFillImageNameAttribute;
import org.odftoolkit.odfdom.dom.attribute.draw.DrawFillImageRefPointAttribute;
import org.odftoolkit.odfdom.dom.attribute.draw.DrawFillImageRefPointXAttribute;
import org.odftoolkit.odfdom.dom.attribute.draw.DrawFillImageRefPointYAttribute;
import org.odftoolkit.odfdom.dom.attribute.draw.DrawFillImageWidthAttribute;
import org.odftoolkit.odfdom.dom.attribute.draw.DrawGradientStepCountAttribute;
import org.odftoolkit.odfdom.dom.attribute.draw.DrawOpacityAttribute;
import org.odftoolkit.odfdom.dom.attribute.draw.DrawOpacityNameAttribute;
import org.odftoolkit.odfdom.dom.attribute.draw.DrawSecondaryFillColorAttribute;
import org.odftoolkit.odfdom.dom.attribute.draw.DrawTileRepeatOffsetAttribute;
import org.odftoolkit.odfdom.dom.attribute.presentation.PresentationBackgroundObjectsVisibleAttribute;
import org.odftoolkit.odfdom.dom.attribute.presentation.PresentationBackgroundVisibleAttribute;
import org.odftoolkit.odfdom.dom.attribute.presentation.PresentationDisplayDateTimeAttribute;
import org.odftoolkit.odfdom.dom.attribute.presentation.PresentationDisplayFooterAttribute;
import org.odftoolkit.odfdom.dom.attribute.presentation.PresentationDisplayHeaderAttribute;
import org.odftoolkit.odfdom.dom.attribute.presentation.PresentationDisplayPageNumberAttribute;
import org.odftoolkit.odfdom.dom.attribute.presentation.PresentationDurationAttribute;
import org.odftoolkit.odfdom.dom.attribute.presentation.PresentationTransitionSpeedAttribute;
import org.odftoolkit.odfdom.dom.attribute.presentation.PresentationTransitionStyleAttribute;
import org.odftoolkit.odfdom.dom.attribute.presentation.PresentationTransitionTypeAttribute;
import org.odftoolkit.odfdom.dom.attribute.presentation.PresentationVisibilityAttribute;
import org.odftoolkit.odfdom.dom.attribute.smil.SmilDirectionAttribute;
import org.odftoolkit.odfdom.dom.attribute.smil.SmilFadeColorAttribute;
import org.odftoolkit.odfdom.dom.attribute.smil.SmilSubtypeAttribute;
import org.odftoolkit.odfdom.dom.attribute.smil.SmilTypeAttribute;
import org.odftoolkit.odfdom.dom.attribute.style.StyleRepeatAttribute;
import org.odftoolkit.odfdom.dom.attribute.svg.SvgFillRuleAttribute;
import org.odftoolkit.odfdom.dom.element.OdfStylePropertiesBase;
/**
* DOM implementation of OpenDocument element {@odf.element style:drawing-page-properties}.
*
*/
public class StyleDrawingPagePropertiesElement extends OdfStylePropertiesBase {
public static final OdfName ELEMENT_NAME = OdfName.newName(OdfDocumentNamespace.STYLE, "drawing-page-properties");
/**
* Create the instance of StyleDrawingPagePropertiesElement
*
* @param ownerDoc The type is OdfFileDom
*/
public StyleDrawingPagePropertiesElement(OdfFileDom ownerDoc) {
super(ownerDoc, ELEMENT_NAME);
}
/**
* Get the element name
*
* @return return OdfName
the name of element {@odf.element style:drawing-page-properties}.
*/
public OdfName getOdfName() {
return ELEMENT_NAME;
}
public final static OdfStyleProperty BackgroundSize =
OdfStyleProperty.get(OdfStylePropertiesSet.DrawingPageProperties, OdfName.newName(OdfDocumentNamespace.DRAW, "background-size"));
public final static OdfStyleProperty Fill =
OdfStyleProperty.get(OdfStylePropertiesSet.DrawingPageProperties, OdfName.newName(OdfDocumentNamespace.DRAW, "fill"));
public final static OdfStyleProperty FillColor =
OdfStyleProperty.get(OdfStylePropertiesSet.DrawingPageProperties, OdfName.newName(OdfDocumentNamespace.DRAW, "fill-color"));
public final static OdfStyleProperty FillGradientName =
OdfStyleProperty.get(OdfStylePropertiesSet.DrawingPageProperties, OdfName.newName(OdfDocumentNamespace.DRAW, "fill-gradient-name"));
public final static OdfStyleProperty FillHatchName =
OdfStyleProperty.get(OdfStylePropertiesSet.DrawingPageProperties, OdfName.newName(OdfDocumentNamespace.DRAW, "fill-hatch-name"));
public final static OdfStyleProperty FillHatchSolid =
OdfStyleProperty.get(OdfStylePropertiesSet.DrawingPageProperties, OdfName.newName(OdfDocumentNamespace.DRAW, "fill-hatch-solid"));
public final static OdfStyleProperty FillImageHeight =
OdfStyleProperty.get(OdfStylePropertiesSet.DrawingPageProperties, OdfName.newName(OdfDocumentNamespace.DRAW, "fill-image-height"));
public final static OdfStyleProperty FillImageName =
OdfStyleProperty.get(OdfStylePropertiesSet.DrawingPageProperties, OdfName.newName(OdfDocumentNamespace.DRAW, "fill-image-name"));
public final static OdfStyleProperty FillImageRefPoint =
OdfStyleProperty.get(OdfStylePropertiesSet.DrawingPageProperties, OdfName.newName(OdfDocumentNamespace.DRAW, "fill-image-ref-point"));
public final static OdfStyleProperty FillImageRefPointX =
OdfStyleProperty.get(OdfStylePropertiesSet.DrawingPageProperties, OdfName.newName(OdfDocumentNamespace.DRAW, "fill-image-ref-point-x"));
public final static OdfStyleProperty FillImageRefPointY =
OdfStyleProperty.get(OdfStylePropertiesSet.DrawingPageProperties, OdfName.newName(OdfDocumentNamespace.DRAW, "fill-image-ref-point-y"));
public final static OdfStyleProperty FillImageWidth =
OdfStyleProperty.get(OdfStylePropertiesSet.DrawingPageProperties, OdfName.newName(OdfDocumentNamespace.DRAW, "fill-image-width"));
public final static OdfStyleProperty GradientStepCount =
OdfStyleProperty.get(OdfStylePropertiesSet.DrawingPageProperties, OdfName.newName(OdfDocumentNamespace.DRAW, "gradient-step-count"));
public final static OdfStyleProperty Opacity =
OdfStyleProperty.get(OdfStylePropertiesSet.DrawingPageProperties, OdfName.newName(OdfDocumentNamespace.DRAW, "opacity"));
public final static OdfStyleProperty OpacityName =
OdfStyleProperty.get(OdfStylePropertiesSet.DrawingPageProperties, OdfName.newName(OdfDocumentNamespace.DRAW, "opacity-name"));
public final static OdfStyleProperty SecondaryFillColor =
OdfStyleProperty.get(OdfStylePropertiesSet.DrawingPageProperties, OdfName.newName(OdfDocumentNamespace.DRAW, "secondary-fill-color"));
public final static OdfStyleProperty TileRepeatOffset =
OdfStyleProperty.get(OdfStylePropertiesSet.DrawingPageProperties, OdfName.newName(OdfDocumentNamespace.DRAW, "tile-repeat-offset"));
public final static OdfStyleProperty BackgroundObjectsVisible =
OdfStyleProperty.get(OdfStylePropertiesSet.DrawingPageProperties, OdfName.newName(OdfDocumentNamespace.PRESENTATION, "background-objects-visible"));
public final static OdfStyleProperty BackgroundVisible =
OdfStyleProperty.get(OdfStylePropertiesSet.DrawingPageProperties, OdfName.newName(OdfDocumentNamespace.PRESENTATION, "background-visible"));
public final static OdfStyleProperty DisplayDateTime =
OdfStyleProperty.get(OdfStylePropertiesSet.DrawingPageProperties, OdfName.newName(OdfDocumentNamespace.PRESENTATION, "display-date-time"));
public final static OdfStyleProperty DisplayFooter =
OdfStyleProperty.get(OdfStylePropertiesSet.DrawingPageProperties, OdfName.newName(OdfDocumentNamespace.PRESENTATION, "display-footer"));
public final static OdfStyleProperty DisplayHeader =
OdfStyleProperty.get(OdfStylePropertiesSet.DrawingPageProperties, OdfName.newName(OdfDocumentNamespace.PRESENTATION, "display-header"));
public final static OdfStyleProperty DisplayPageNumber =
OdfStyleProperty.get(OdfStylePropertiesSet.DrawingPageProperties, OdfName.newName(OdfDocumentNamespace.PRESENTATION, "display-page-number"));
public final static OdfStyleProperty Duration =
OdfStyleProperty.get(OdfStylePropertiesSet.DrawingPageProperties, OdfName.newName(OdfDocumentNamespace.PRESENTATION, "duration"));
public final static OdfStyleProperty TransitionSpeed =
OdfStyleProperty.get(OdfStylePropertiesSet.DrawingPageProperties, OdfName.newName(OdfDocumentNamespace.PRESENTATION, "transition-speed"));
public final static OdfStyleProperty TransitionStyle =
OdfStyleProperty.get(OdfStylePropertiesSet.DrawingPageProperties, OdfName.newName(OdfDocumentNamespace.PRESENTATION, "transition-style"));
public final static OdfStyleProperty TransitionType =
OdfStyleProperty.get(OdfStylePropertiesSet.DrawingPageProperties, OdfName.newName(OdfDocumentNamespace.PRESENTATION, "transition-type"));
public final static OdfStyleProperty Visibility =
OdfStyleProperty.get(OdfStylePropertiesSet.DrawingPageProperties, OdfName.newName(OdfDocumentNamespace.PRESENTATION, "visibility"));
public final static OdfStyleProperty Direction =
OdfStyleProperty.get(OdfStylePropertiesSet.DrawingPageProperties, OdfName.newName(OdfDocumentNamespace.SMIL, "direction"));
public final static OdfStyleProperty FadeColor =
OdfStyleProperty.get(OdfStylePropertiesSet.DrawingPageProperties, OdfName.newName(OdfDocumentNamespace.SMIL, "fadeColor"));
public final static OdfStyleProperty Subtype =
OdfStyleProperty.get(OdfStylePropertiesSet.DrawingPageProperties, OdfName.newName(OdfDocumentNamespace.SMIL, "subtype"));
public final static OdfStyleProperty Type =
OdfStyleProperty.get(OdfStylePropertiesSet.DrawingPageProperties, OdfName.newName(OdfDocumentNamespace.SMIL, "type"));
public final static OdfStyleProperty Repeat =
OdfStyleProperty.get(OdfStylePropertiesSet.DrawingPageProperties, OdfName.newName(OdfDocumentNamespace.STYLE, "repeat"));
public final static OdfStyleProperty FillRule =
OdfStyleProperty.get(OdfStylePropertiesSet.DrawingPageProperties, OdfName.newName(OdfDocumentNamespace.SVG, "fill-rule"));
/**
* Receives the value of the ODFDOM attribute representation DrawBackgroundSizeAttribute
, See {@odf.attribute draw:background-size}
*
* @return - the String
, the value or null
, if the attribute is not set and no default value defined.
*/
public String getDrawBackgroundSizeAttribute() {
DrawBackgroundSizeAttribute attr = (DrawBackgroundSizeAttribute) getOdfAttribute(OdfDocumentNamespace.DRAW, "background-size");
if (attr != null) {
return String.valueOf(attr.getValue());
}
return null;
}
/**
* Sets the value of ODFDOM attribute representation DrawBackgroundSizeAttribute
, See {@odf.attribute draw:background-size}
*
* @param drawBackgroundSizeValue The type is String
*/
public void setDrawBackgroundSizeAttribute(String drawBackgroundSizeValue) {
DrawBackgroundSizeAttribute attr = new DrawBackgroundSizeAttribute((OdfFileDom) this.ownerDocument);
setOdfAttribute(attr);
attr.setValue(drawBackgroundSizeValue);
}
/**
* Receives the value of the ODFDOM attribute representation DrawFillAttribute
, See {@odf.attribute draw:fill}
*
* @return - the String
, the value or null
, if the attribute is not set and no default value defined.
*/
public String getDrawFillAttribute() {
DrawFillAttribute attr = (DrawFillAttribute) getOdfAttribute(OdfDocumentNamespace.DRAW, "fill");
if (attr != null) {
return String.valueOf(attr.getValue());
}
return null;
}
/**
* Sets the value of ODFDOM attribute representation DrawFillAttribute
, See {@odf.attribute draw:fill}
*
* @param drawFillValue The type is String
*/
public void setDrawFillAttribute(String drawFillValue) {
DrawFillAttribute attr = new DrawFillAttribute((OdfFileDom) this.ownerDocument);
setOdfAttribute(attr);
attr.setValue(drawFillValue);
}
/**
* Receives the value of the ODFDOM attribute representation DrawFillColorAttribute
, See {@odf.attribute draw:fill-color}
*
* @return - the String
, the value or null
, if the attribute is not set and no default value defined.
*/
public String getDrawFillColorAttribute() {
DrawFillColorAttribute attr = (DrawFillColorAttribute) getOdfAttribute(OdfDocumentNamespace.DRAW, "fill-color");
if (attr != null) {
return String.valueOf(attr.getValue());
}
return null;
}
/**
* Sets the value of ODFDOM attribute representation DrawFillColorAttribute
, See {@odf.attribute draw:fill-color}
*
* @param drawFillColorValue The type is String
*/
public void setDrawFillColorAttribute(String drawFillColorValue) {
DrawFillColorAttribute attr = new DrawFillColorAttribute((OdfFileDom) this.ownerDocument);
setOdfAttribute(attr);
attr.setValue(drawFillColorValue);
}
/**
* Receives the value of the ODFDOM attribute representation DrawFillGradientNameAttribute
, See {@odf.attribute draw:fill-gradient-name}
*
* @return - the String
, the value or null
, if the attribute is not set and no default value defined.
*/
public String getDrawFillGradientNameAttribute() {
DrawFillGradientNameAttribute attr = (DrawFillGradientNameAttribute) getOdfAttribute(OdfDocumentNamespace.DRAW, "fill-gradient-name");
if (attr != null) {
return String.valueOf(attr.getValue());
}
return null;
}
/**
* Sets the value of ODFDOM attribute representation DrawFillGradientNameAttribute
, See {@odf.attribute draw:fill-gradient-name}
*
* @param drawFillGradientNameValue The type is String
*/
public void setDrawFillGradientNameAttribute(String drawFillGradientNameValue) {
DrawFillGradientNameAttribute attr = new DrawFillGradientNameAttribute((OdfFileDom) this.ownerDocument);
setOdfAttribute(attr);
attr.setValue(drawFillGradientNameValue);
}
/**
* Receives the value of the ODFDOM attribute representation DrawFillHatchNameAttribute
, See {@odf.attribute draw:fill-hatch-name}
*
* @return - the String
, the value or null
, if the attribute is not set and no default value defined.
*/
public String getDrawFillHatchNameAttribute() {
DrawFillHatchNameAttribute attr = (DrawFillHatchNameAttribute) getOdfAttribute(OdfDocumentNamespace.DRAW, "fill-hatch-name");
if (attr != null) {
return String.valueOf(attr.getValue());
}
return null;
}
/**
* Sets the value of ODFDOM attribute representation DrawFillHatchNameAttribute
, See {@odf.attribute draw:fill-hatch-name}
*
* @param drawFillHatchNameValue The type is String
*/
public void setDrawFillHatchNameAttribute(String drawFillHatchNameValue) {
DrawFillHatchNameAttribute attr = new DrawFillHatchNameAttribute((OdfFileDom) this.ownerDocument);
setOdfAttribute(attr);
attr.setValue(drawFillHatchNameValue);
}
/**
* Receives the value of the ODFDOM attribute representation DrawFillHatchSolidAttribute
, See {@odf.attribute draw:fill-hatch-solid}
*
* @return - the Boolean
, the value or null
, if the attribute is not set and no default value defined.
*/
public Boolean getDrawFillHatchSolidAttribute() {
DrawFillHatchSolidAttribute attr = (DrawFillHatchSolidAttribute) getOdfAttribute(OdfDocumentNamespace.DRAW, "fill-hatch-solid");
if (attr != null) {
return Boolean.valueOf(attr.booleanValue());
}
return null;
}
/**
* Sets the value of ODFDOM attribute representation DrawFillHatchSolidAttribute
, See {@odf.attribute draw:fill-hatch-solid}
*
* @param drawFillHatchSolidValue The type is Boolean
*/
public void setDrawFillHatchSolidAttribute(Boolean drawFillHatchSolidValue) {
DrawFillHatchSolidAttribute attr = new DrawFillHatchSolidAttribute((OdfFileDom) this.ownerDocument);
setOdfAttribute(attr);
attr.setBooleanValue(drawFillHatchSolidValue.booleanValue());
}
/**
* Receives the value of the ODFDOM attribute representation DrawFillImageHeightAttribute
, See {@odf.attribute draw:fill-image-height}
*
* @return - the String
, the value or null
, if the attribute is not set and no default value defined.
*/
public String getDrawFillImageHeightAttribute() {
DrawFillImageHeightAttribute attr = (DrawFillImageHeightAttribute) getOdfAttribute(OdfDocumentNamespace.DRAW, "fill-image-height");
if (attr != null) {
return String.valueOf(attr.getValue());
}
return null;
}
/**
* Sets the value of ODFDOM attribute representation DrawFillImageHeightAttribute
, See {@odf.attribute draw:fill-image-height}
*
* @param drawFillImageHeightValue The type is String
*/
public void setDrawFillImageHeightAttribute(String drawFillImageHeightValue) {
DrawFillImageHeightAttribute attr = new DrawFillImageHeightAttribute((OdfFileDom) this.ownerDocument);
setOdfAttribute(attr);
attr.setValue(drawFillImageHeightValue);
}
/**
* Receives the value of the ODFDOM attribute representation DrawFillImageNameAttribute
, See {@odf.attribute draw:fill-image-name}
*
* @return - the String
, the value or null
, if the attribute is not set and no default value defined.
*/
public String getDrawFillImageNameAttribute() {
DrawFillImageNameAttribute attr = (DrawFillImageNameAttribute) getOdfAttribute(OdfDocumentNamespace.DRAW, "fill-image-name");
if (attr != null) {
return String.valueOf(attr.getValue());
}
return null;
}
/**
* Sets the value of ODFDOM attribute representation DrawFillImageNameAttribute
, See {@odf.attribute draw:fill-image-name}
*
* @param drawFillImageNameValue The type is String
*/
public void setDrawFillImageNameAttribute(String drawFillImageNameValue) {
DrawFillImageNameAttribute attr = new DrawFillImageNameAttribute((OdfFileDom) this.ownerDocument);
setOdfAttribute(attr);
attr.setValue(drawFillImageNameValue);
}
/**
* Receives the value of the ODFDOM attribute representation DrawFillImageRefPointAttribute
, See {@odf.attribute draw:fill-image-ref-point}
*
* @return - the String
, the value or null
, if the attribute is not set and no default value defined.
*/
public String getDrawFillImageRefPointAttribute() {
DrawFillImageRefPointAttribute attr = (DrawFillImageRefPointAttribute) getOdfAttribute(OdfDocumentNamespace.DRAW, "fill-image-ref-point");
if (attr != null) {
return String.valueOf(attr.getValue());
}
return null;
}
/**
* Sets the value of ODFDOM attribute representation DrawFillImageRefPointAttribute
, See {@odf.attribute draw:fill-image-ref-point}
*
* @param drawFillImageRefPointValue The type is String
*/
public void setDrawFillImageRefPointAttribute(String drawFillImageRefPointValue) {
DrawFillImageRefPointAttribute attr = new DrawFillImageRefPointAttribute((OdfFileDom) this.ownerDocument);
setOdfAttribute(attr);
attr.setValue(drawFillImageRefPointValue);
}
/**
* Receives the value of the ODFDOM attribute representation DrawFillImageRefPointXAttribute
, See {@odf.attribute draw:fill-image-ref-point-x}
*
* @return - the String
, the value or null
, if the attribute is not set and no default value defined.
*/
public String getDrawFillImageRefPointXAttribute() {
DrawFillImageRefPointXAttribute attr = (DrawFillImageRefPointXAttribute) getOdfAttribute(OdfDocumentNamespace.DRAW, "fill-image-ref-point-x");
if (attr != null) {
return String.valueOf(attr.getValue());
}
return null;
}
/**
* Sets the value of ODFDOM attribute representation DrawFillImageRefPointXAttribute
, See {@odf.attribute draw:fill-image-ref-point-x}
*
* @param drawFillImageRefPointXValue The type is String
*/
public void setDrawFillImageRefPointXAttribute(String drawFillImageRefPointXValue) {
DrawFillImageRefPointXAttribute attr = new DrawFillImageRefPointXAttribute((OdfFileDom) this.ownerDocument);
setOdfAttribute(attr);
attr.setValue(drawFillImageRefPointXValue);
}
/**
* Receives the value of the ODFDOM attribute representation DrawFillImageRefPointYAttribute
, See {@odf.attribute draw:fill-image-ref-point-y}
*
* @return - the String
, the value or null
, if the attribute is not set and no default value defined.
*/
public String getDrawFillImageRefPointYAttribute() {
DrawFillImageRefPointYAttribute attr = (DrawFillImageRefPointYAttribute) getOdfAttribute(OdfDocumentNamespace.DRAW, "fill-image-ref-point-y");
if (attr != null) {
return String.valueOf(attr.getValue());
}
return null;
}
/**
* Sets the value of ODFDOM attribute representation DrawFillImageRefPointYAttribute
, See {@odf.attribute draw:fill-image-ref-point-y}
*
* @param drawFillImageRefPointYValue The type is String
*/
public void setDrawFillImageRefPointYAttribute(String drawFillImageRefPointYValue) {
DrawFillImageRefPointYAttribute attr = new DrawFillImageRefPointYAttribute((OdfFileDom) this.ownerDocument);
setOdfAttribute(attr);
attr.setValue(drawFillImageRefPointYValue);
}
/**
* Receives the value of the ODFDOM attribute representation DrawFillImageWidthAttribute
, See {@odf.attribute draw:fill-image-width}
*
* @return - the String
, the value or null
, if the attribute is not set and no default value defined.
*/
public String getDrawFillImageWidthAttribute() {
DrawFillImageWidthAttribute attr = (DrawFillImageWidthAttribute) getOdfAttribute(OdfDocumentNamespace.DRAW, "fill-image-width");
if (attr != null) {
return String.valueOf(attr.getValue());
}
return null;
}
/**
* Sets the value of ODFDOM attribute representation DrawFillImageWidthAttribute
, See {@odf.attribute draw:fill-image-width}
*
* @param drawFillImageWidthValue The type is String
*/
public void setDrawFillImageWidthAttribute(String drawFillImageWidthValue) {
DrawFillImageWidthAttribute attr = new DrawFillImageWidthAttribute((OdfFileDom) this.ownerDocument);
setOdfAttribute(attr);
attr.setValue(drawFillImageWidthValue);
}
/**
* Receives the value of the ODFDOM attribute representation DrawGradientStepCountAttribute
, See {@odf.attribute draw:gradient-step-count}
*
* @return - the Integer
, the value or null
, if the attribute is not set and no default value defined.
*/
public Integer getDrawGradientStepCountAttribute() {
DrawGradientStepCountAttribute attr = (DrawGradientStepCountAttribute) getOdfAttribute(OdfDocumentNamespace.DRAW, "gradient-step-count");
if (attr != null) {
return Integer.valueOf(attr.intValue());
}
return null;
}
/**
* Sets the value of ODFDOM attribute representation DrawGradientStepCountAttribute
, See {@odf.attribute draw:gradient-step-count}
*
* @param drawGradientStepCountValue The type is Integer
*/
public void setDrawGradientStepCountAttribute(Integer drawGradientStepCountValue) {
DrawGradientStepCountAttribute attr = new DrawGradientStepCountAttribute((OdfFileDom) this.ownerDocument);
setOdfAttribute(attr);
attr.setIntValue(drawGradientStepCountValue.intValue());
}
/**
* Receives the value of the ODFDOM attribute representation DrawOpacityAttribute
, See {@odf.attribute draw:opacity}
*
* @return - the String
, the value or null
, if the attribute is not set and no default value defined.
*/
public String getDrawOpacityAttribute() {
DrawOpacityAttribute attr = (DrawOpacityAttribute) getOdfAttribute(OdfDocumentNamespace.DRAW, "opacity");
if (attr != null) {
return String.valueOf(attr.getValue());
}
return null;
}
/**
* Sets the value of ODFDOM attribute representation DrawOpacityAttribute
, See {@odf.attribute draw:opacity}
*
* @param drawOpacityValue The type is String
*/
public void setDrawOpacityAttribute(String drawOpacityValue) {
DrawOpacityAttribute attr = new DrawOpacityAttribute((OdfFileDom) this.ownerDocument);
setOdfAttribute(attr);
attr.setValue(drawOpacityValue);
}
/**
* Receives the value of the ODFDOM attribute representation DrawOpacityNameAttribute
, See {@odf.attribute draw:opacity-name}
*
* @return - the String
, the value or null
, if the attribute is not set and no default value defined.
*/
public String getDrawOpacityNameAttribute() {
DrawOpacityNameAttribute attr = (DrawOpacityNameAttribute) getOdfAttribute(OdfDocumentNamespace.DRAW, "opacity-name");
if (attr != null) {
return String.valueOf(attr.getValue());
}
return null;
}
/**
* Sets the value of ODFDOM attribute representation DrawOpacityNameAttribute
, See {@odf.attribute draw:opacity-name}
*
* @param drawOpacityNameValue The type is String
*/
public void setDrawOpacityNameAttribute(String drawOpacityNameValue) {
DrawOpacityNameAttribute attr = new DrawOpacityNameAttribute((OdfFileDom) this.ownerDocument);
setOdfAttribute(attr);
attr.setValue(drawOpacityNameValue);
}
/**
* Receives the value of the ODFDOM attribute representation DrawSecondaryFillColorAttribute
, See {@odf.attribute draw:secondary-fill-color}
*
* @return - the String
, the value or null
, if the attribute is not set and no default value defined.
*/
public String getDrawSecondaryFillColorAttribute() {
DrawSecondaryFillColorAttribute attr = (DrawSecondaryFillColorAttribute) getOdfAttribute(OdfDocumentNamespace.DRAW, "secondary-fill-color");
if (attr != null) {
return String.valueOf(attr.getValue());
}
return null;
}
/**
* Sets the value of ODFDOM attribute representation DrawSecondaryFillColorAttribute
, See {@odf.attribute draw:secondary-fill-color}
*
* @param drawSecondaryFillColorValue The type is String
*/
public void setDrawSecondaryFillColorAttribute(String drawSecondaryFillColorValue) {
DrawSecondaryFillColorAttribute attr = new DrawSecondaryFillColorAttribute((OdfFileDom) this.ownerDocument);
setOdfAttribute(attr);
attr.setValue(drawSecondaryFillColorValue);
}
/**
* Receives the value of the ODFDOM attribute representation DrawTileRepeatOffsetAttribute
, See {@odf.attribute draw:tile-repeat-offset}
*
* @return - the String
, the value or null
, if the attribute is not set and no default value defined.
*/
public String getDrawTileRepeatOffsetAttribute() {
DrawTileRepeatOffsetAttribute attr = (DrawTileRepeatOffsetAttribute) getOdfAttribute(OdfDocumentNamespace.DRAW, "tile-repeat-offset");
if (attr != null) {
return String.valueOf(attr.getValue());
}
return null;
}
/**
* Sets the value of ODFDOM attribute representation DrawTileRepeatOffsetAttribute
, See {@odf.attribute draw:tile-repeat-offset}
*
* @param drawTileRepeatOffsetValue The type is String
*/
public void setDrawTileRepeatOffsetAttribute(String drawTileRepeatOffsetValue) {
DrawTileRepeatOffsetAttribute attr = new DrawTileRepeatOffsetAttribute((OdfFileDom) this.ownerDocument);
setOdfAttribute(attr);
attr.setValue(drawTileRepeatOffsetValue);
}
/**
* Receives the value of the ODFDOM attribute representation PresentationBackgroundObjectsVisibleAttribute
, See {@odf.attribute presentation:background-objects-visible}
*
* @return - the Boolean
, the value or null
, if the attribute is not set and no default value defined.
*/
public Boolean getPresentationBackgroundObjectsVisibleAttribute() {
PresentationBackgroundObjectsVisibleAttribute attr = (PresentationBackgroundObjectsVisibleAttribute) getOdfAttribute(OdfDocumentNamespace.PRESENTATION, "background-objects-visible");
if (attr != null) {
return Boolean.valueOf(attr.booleanValue());
}
return null;
}
/**
* Sets the value of ODFDOM attribute representation PresentationBackgroundObjectsVisibleAttribute
, See {@odf.attribute presentation:background-objects-visible}
*
* @param presentationBackgroundObjectsVisibleValue The type is Boolean
*/
public void setPresentationBackgroundObjectsVisibleAttribute(Boolean presentationBackgroundObjectsVisibleValue) {
PresentationBackgroundObjectsVisibleAttribute attr = new PresentationBackgroundObjectsVisibleAttribute((OdfFileDom) this.ownerDocument);
setOdfAttribute(attr);
attr.setBooleanValue(presentationBackgroundObjectsVisibleValue.booleanValue());
}
/**
* Receives the value of the ODFDOM attribute representation PresentationBackgroundVisibleAttribute
, See {@odf.attribute presentation:background-visible}
*
* @return - the Boolean
, the value or null
, if the attribute is not set and no default value defined.
*/
public Boolean getPresentationBackgroundVisibleAttribute() {
PresentationBackgroundVisibleAttribute attr = (PresentationBackgroundVisibleAttribute) getOdfAttribute(OdfDocumentNamespace.PRESENTATION, "background-visible");
if (attr != null) {
return Boolean.valueOf(attr.booleanValue());
}
return null;
}
/**
* Sets the value of ODFDOM attribute representation PresentationBackgroundVisibleAttribute
, See {@odf.attribute presentation:background-visible}
*
* @param presentationBackgroundVisibleValue The type is Boolean
*/
public void setPresentationBackgroundVisibleAttribute(Boolean presentationBackgroundVisibleValue) {
PresentationBackgroundVisibleAttribute attr = new PresentationBackgroundVisibleAttribute((OdfFileDom) this.ownerDocument);
setOdfAttribute(attr);
attr.setBooleanValue(presentationBackgroundVisibleValue.booleanValue());
}
/**
* Receives the value of the ODFDOM attribute representation PresentationDisplayDateTimeAttribute
, See {@odf.attribute presentation:display-date-time}
*
* @return - the Boolean
, the value or null
, if the attribute is not set and no default value defined.
*/
public Boolean getPresentationDisplayDateTimeAttribute() {
PresentationDisplayDateTimeAttribute attr = (PresentationDisplayDateTimeAttribute) getOdfAttribute(OdfDocumentNamespace.PRESENTATION, "display-date-time");
if (attr != null) {
return Boolean.valueOf(attr.booleanValue());
}
return null;
}
/**
* Sets the value of ODFDOM attribute representation PresentationDisplayDateTimeAttribute
, See {@odf.attribute presentation:display-date-time}
*
* @param presentationDisplayDateTimeValue The type is Boolean
*/
public void setPresentationDisplayDateTimeAttribute(Boolean presentationDisplayDateTimeValue) {
PresentationDisplayDateTimeAttribute attr = new PresentationDisplayDateTimeAttribute((OdfFileDom) this.ownerDocument);
setOdfAttribute(attr);
attr.setBooleanValue(presentationDisplayDateTimeValue.booleanValue());
}
/**
* Receives the value of the ODFDOM attribute representation PresentationDisplayFooterAttribute
, See {@odf.attribute presentation:display-footer}
*
* @return - the Boolean
, the value or null
, if the attribute is not set and no default value defined.
*/
public Boolean getPresentationDisplayFooterAttribute() {
PresentationDisplayFooterAttribute attr = (PresentationDisplayFooterAttribute) getOdfAttribute(OdfDocumentNamespace.PRESENTATION, "display-footer");
if (attr != null) {
return Boolean.valueOf(attr.booleanValue());
}
return null;
}
/**
* Sets the value of ODFDOM attribute representation PresentationDisplayFooterAttribute
, See {@odf.attribute presentation:display-footer}
*
* @param presentationDisplayFooterValue The type is Boolean
*/
public void setPresentationDisplayFooterAttribute(Boolean presentationDisplayFooterValue) {
PresentationDisplayFooterAttribute attr = new PresentationDisplayFooterAttribute((OdfFileDom) this.ownerDocument);
setOdfAttribute(attr);
attr.setBooleanValue(presentationDisplayFooterValue.booleanValue());
}
/**
* Receives the value of the ODFDOM attribute representation PresentationDisplayHeaderAttribute
, See {@odf.attribute presentation:display-header}
*
* @return - the Boolean
, the value or null
, if the attribute is not set and no default value defined.
*/
public Boolean getPresentationDisplayHeaderAttribute() {
PresentationDisplayHeaderAttribute attr = (PresentationDisplayHeaderAttribute) getOdfAttribute(OdfDocumentNamespace.PRESENTATION, "display-header");
if (attr != null) {
return Boolean.valueOf(attr.booleanValue());
}
return null;
}
/**
* Sets the value of ODFDOM attribute representation PresentationDisplayHeaderAttribute
, See {@odf.attribute presentation:display-header}
*
* @param presentationDisplayHeaderValue The type is Boolean
*/
public void setPresentationDisplayHeaderAttribute(Boolean presentationDisplayHeaderValue) {
PresentationDisplayHeaderAttribute attr = new PresentationDisplayHeaderAttribute((OdfFileDom) this.ownerDocument);
setOdfAttribute(attr);
attr.setBooleanValue(presentationDisplayHeaderValue.booleanValue());
}
/**
* Receives the value of the ODFDOM attribute representation PresentationDisplayPageNumberAttribute
, See {@odf.attribute presentation:display-page-number}
*
* @return - the Boolean
, the value or null
, if the attribute is not set and no default value defined.
*/
public Boolean getPresentationDisplayPageNumberAttribute() {
PresentationDisplayPageNumberAttribute attr = (PresentationDisplayPageNumberAttribute) getOdfAttribute(OdfDocumentNamespace.PRESENTATION, "display-page-number");
if (attr != null) {
return Boolean.valueOf(attr.booleanValue());
}
return null;
}
/**
* Sets the value of ODFDOM attribute representation PresentationDisplayPageNumberAttribute
, See {@odf.attribute presentation:display-page-number}
*
* @param presentationDisplayPageNumberValue The type is Boolean
*/
public void setPresentationDisplayPageNumberAttribute(Boolean presentationDisplayPageNumberValue) {
PresentationDisplayPageNumberAttribute attr = new PresentationDisplayPageNumberAttribute((OdfFileDom) this.ownerDocument);
setOdfAttribute(attr);
attr.setBooleanValue(presentationDisplayPageNumberValue.booleanValue());
}
/**
* Receives the value of the ODFDOM attribute representation PresentationDurationAttribute
, See {@odf.attribute presentation:duration}
*
* @return - the String
, the value or null
, if the attribute is not set and no default value defined.
*/
public String getPresentationDurationAttribute() {
PresentationDurationAttribute attr = (PresentationDurationAttribute) getOdfAttribute(OdfDocumentNamespace.PRESENTATION, "duration");
if (attr != null) {
return String.valueOf(attr.getValue());
}
return null;
}
/**
* Sets the value of ODFDOM attribute representation PresentationDurationAttribute
, See {@odf.attribute presentation:duration}
*
* @param presentationDurationValue The type is String
*/
public void setPresentationDurationAttribute(String presentationDurationValue) {
PresentationDurationAttribute attr = new PresentationDurationAttribute((OdfFileDom) this.ownerDocument);
setOdfAttribute(attr);
attr.setValue(presentationDurationValue);
}
/**
* Receives the value of the ODFDOM attribute representation PresentationTransitionSpeedAttribute
, See {@odf.attribute presentation:transition-speed}
*
* @return - the String
, the value or null
, if the attribute is not set and no default value defined.
*/
public String getPresentationTransitionSpeedAttribute() {
PresentationTransitionSpeedAttribute attr = (PresentationTransitionSpeedAttribute) getOdfAttribute(OdfDocumentNamespace.PRESENTATION, "transition-speed");
if (attr != null) {
return String.valueOf(attr.getValue());
}
return null;
}
/**
* Sets the value of ODFDOM attribute representation PresentationTransitionSpeedAttribute
, See {@odf.attribute presentation:transition-speed}
*
* @param presentationTransitionSpeedValue The type is String
*/
public void setPresentationTransitionSpeedAttribute(String presentationTransitionSpeedValue) {
PresentationTransitionSpeedAttribute attr = new PresentationTransitionSpeedAttribute((OdfFileDom) this.ownerDocument);
setOdfAttribute(attr);
attr.setValue(presentationTransitionSpeedValue);
}
/**
* Receives the value of the ODFDOM attribute representation PresentationTransitionStyleAttribute
, See {@odf.attribute presentation:transition-style}
*
* @return - the String
, the value or null
, if the attribute is not set and no default value defined.
*/
public String getPresentationTransitionStyleAttribute() {
PresentationTransitionStyleAttribute attr = (PresentationTransitionStyleAttribute) getOdfAttribute(OdfDocumentNamespace.PRESENTATION, "transition-style");
if (attr != null) {
return String.valueOf(attr.getValue());
}
return null;
}
/**
* Sets the value of ODFDOM attribute representation PresentationTransitionStyleAttribute
, See {@odf.attribute presentation:transition-style}
*
* @param presentationTransitionStyleValue The type is String
*/
public void setPresentationTransitionStyleAttribute(String presentationTransitionStyleValue) {
PresentationTransitionStyleAttribute attr = new PresentationTransitionStyleAttribute((OdfFileDom) this.ownerDocument);
setOdfAttribute(attr);
attr.setValue(presentationTransitionStyleValue);
}
/**
* Receives the value of the ODFDOM attribute representation PresentationTransitionTypeAttribute
, See {@odf.attribute presentation:transition-type}
*
* @return - the String
, the value or null
, if the attribute is not set and no default value defined.
*/
public String getPresentationTransitionTypeAttribute() {
PresentationTransitionTypeAttribute attr = (PresentationTransitionTypeAttribute) getOdfAttribute(OdfDocumentNamespace.PRESENTATION, "transition-type");
if (attr != null) {
return String.valueOf(attr.getValue());
}
return null;
}
/**
* Sets the value of ODFDOM attribute representation PresentationTransitionTypeAttribute
, See {@odf.attribute presentation:transition-type}
*
* @param presentationTransitionTypeValue The type is String
*/
public void setPresentationTransitionTypeAttribute(String presentationTransitionTypeValue) {
PresentationTransitionTypeAttribute attr = new PresentationTransitionTypeAttribute((OdfFileDom) this.ownerDocument);
setOdfAttribute(attr);
attr.setValue(presentationTransitionTypeValue);
}
/**
* Receives the value of the ODFDOM attribute representation PresentationVisibilityAttribute
, See {@odf.attribute presentation:visibility}
*
* @return - the String
, the value or null
, if the attribute is not set and no default value defined.
*/
public String getPresentationVisibilityAttribute() {
PresentationVisibilityAttribute attr = (PresentationVisibilityAttribute) getOdfAttribute(OdfDocumentNamespace.PRESENTATION, "visibility");
if (attr != null) {
return String.valueOf(attr.getValue());
}
return null;
}
/**
* Sets the value of ODFDOM attribute representation PresentationVisibilityAttribute
, See {@odf.attribute presentation:visibility}
*
* @param presentationVisibilityValue The type is String
*/
public void setPresentationVisibilityAttribute(String presentationVisibilityValue) {
PresentationVisibilityAttribute attr = new PresentationVisibilityAttribute((OdfFileDom) this.ownerDocument);
setOdfAttribute(attr);
attr.setValue(presentationVisibilityValue);
}
/**
* Receives the value of the ODFDOM attribute representation SmilDirectionAttribute
, See {@odf.attribute smil:direction}
*
* @return - the String
, the value or null
, if the attribute is not set and no default value defined.
*/
public String getSmilDirectionAttribute() {
SmilDirectionAttribute attr = (SmilDirectionAttribute) getOdfAttribute(OdfDocumentNamespace.SMIL, "direction");
if (attr != null) {
return String.valueOf(attr.getValue());
}
return null;
}
/**
* Sets the value of ODFDOM attribute representation SmilDirectionAttribute
, See {@odf.attribute smil:direction}
*
* @param smilDirectionValue The type is String
*/
public void setSmilDirectionAttribute(String smilDirectionValue) {
SmilDirectionAttribute attr = new SmilDirectionAttribute((OdfFileDom) this.ownerDocument);
setOdfAttribute(attr);
attr.setValue(smilDirectionValue);
}
/**
* Receives the value of the ODFDOM attribute representation SmilFadeColorAttribute
, See {@odf.attribute smil:fadeColor}
*
* @return - the String
, the value or null
, if the attribute is not set and no default value defined.
*/
public String getSmilFadeColorAttribute() {
SmilFadeColorAttribute attr = (SmilFadeColorAttribute) getOdfAttribute(OdfDocumentNamespace.SMIL, "fadeColor");
if (attr != null) {
return String.valueOf(attr.getValue());
}
return SmilFadeColorAttribute.DEFAULT_VALUE;
}
/**
* Sets the value of ODFDOM attribute representation SmilFadeColorAttribute
, See {@odf.attribute smil:fadeColor}
*
* @param smilFadeColorValue The type is String
*/
public void setSmilFadeColorAttribute(String smilFadeColorValue) {
SmilFadeColorAttribute attr = new SmilFadeColorAttribute((OdfFileDom) this.ownerDocument);
setOdfAttribute(attr);
attr.setValue(smilFadeColorValue);
}
/**
* Receives the value of the ODFDOM attribute representation SmilSubtypeAttribute
, See {@odf.attribute smil:subtype}
*
* @return - the String
, the value or null
, if the attribute is not set and no default value defined.
*/
public String getSmilSubtypeAttribute() {
SmilSubtypeAttribute attr = (SmilSubtypeAttribute) getOdfAttribute(OdfDocumentNamespace.SMIL, "subtype");
if (attr != null) {
return String.valueOf(attr.getValue());
}
return null;
}
/**
* Sets the value of ODFDOM attribute representation SmilSubtypeAttribute
, See {@odf.attribute smil:subtype}
*
* @param smilSubtypeValue The type is String
*/
public void setSmilSubtypeAttribute(String smilSubtypeValue) {
SmilSubtypeAttribute attr = new SmilSubtypeAttribute((OdfFileDom) this.ownerDocument);
setOdfAttribute(attr);
attr.setValue(smilSubtypeValue);
}
/**
* Receives the value of the ODFDOM attribute representation SmilTypeAttribute
, See {@odf.attribute smil:type}
*
* @return - the String
, the value or null
, if the attribute is not set and no default value defined.
*/
public String getSmilTypeAttribute() {
SmilTypeAttribute attr = (SmilTypeAttribute) getOdfAttribute(OdfDocumentNamespace.SMIL, "type");
if (attr != null) {
return String.valueOf(attr.getValue());
}
return null;
}
/**
* Sets the value of ODFDOM attribute representation SmilTypeAttribute
, See {@odf.attribute smil:type}
*
* @param smilTypeValue The type is String
*/
public void setSmilTypeAttribute(String smilTypeValue) {
SmilTypeAttribute attr = new SmilTypeAttribute((OdfFileDom) this.ownerDocument);
setOdfAttribute(attr);
attr.setValue(smilTypeValue);
}
/**
* Receives the value of the ODFDOM attribute representation StyleRepeatAttribute
, See {@odf.attribute style:repeat}
*
* @return - the String
, the value or null
, if the attribute is not set and no default value defined.
*/
public String getStyleRepeatAttribute() {
StyleRepeatAttribute attr = (StyleRepeatAttribute) getOdfAttribute(OdfDocumentNamespace.STYLE, "repeat");
if (attr != null) {
return String.valueOf(attr.getValue());
}
return null;
}
/**
* Sets the value of ODFDOM attribute representation StyleRepeatAttribute
, See {@odf.attribute style:repeat}
*
* @param styleRepeatValue The type is String
*/
public void setStyleRepeatAttribute(String styleRepeatValue) {
StyleRepeatAttribute attr = new StyleRepeatAttribute((OdfFileDom) this.ownerDocument);
setOdfAttribute(attr);
attr.setValue(styleRepeatValue);
}
/**
* Receives the value of the ODFDOM attribute representation SvgFillRuleAttribute
, See {@odf.attribute svg:fill-rule}
*
* @return - the String
, the value or null
, if the attribute is not set and no default value defined.
*/
public String getSvgFillRuleAttribute() {
SvgFillRuleAttribute attr = (SvgFillRuleAttribute) getOdfAttribute(OdfDocumentNamespace.SVG, "fill-rule");
if (attr != null) {
return String.valueOf(attr.getValue());
}
return null;
}
/**
* Sets the value of ODFDOM attribute representation SvgFillRuleAttribute
, See {@odf.attribute svg:fill-rule}
*
* @param svgFillRuleValue The type is String
*/
public void setSvgFillRuleAttribute(String svgFillRuleValue) {
SvgFillRuleAttribute attr = new SvgFillRuleAttribute((OdfFileDom) this.ownerDocument);
setOdfAttribute(attr);
attr.setValue(svgFillRuleValue);
}
/**
* Create child element {@odf.element presentation:sound}.
*
* @param xlinkHrefValue the String
value of XlinkHrefAttribute
, see {@odf.attribute xlink:href} at specification
* @param xlinkTypeValue the String
value of XlinkTypeAttribute
, see {@odf.attribute xlink:type} at specification
* Child element is new in Odf 1.2
*
* @return the element {@odf.element presentation:sound}
*/
public PresentationSoundElement newPresentationSoundElement(String xlinkHrefValue, String xlinkTypeValue) {
PresentationSoundElement presentationSound = ((OdfFileDom) this.ownerDocument).newOdfElement(PresentationSoundElement.class);
presentationSound.setXlinkHrefAttribute(xlinkHrefValue);
presentationSound.setXlinkTypeAttribute(xlinkTypeValue);
this.appendChild(presentationSound);
return presentationSound;
}
@Override
public void accept(ElementVisitor visitor) {
if (visitor instanceof DefaultElementVisitor) {
DefaultElementVisitor defaultVisitor = (DefaultElementVisitor) visitor;
defaultVisitor.visit(this);
} else {
visitor.visit(this);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy