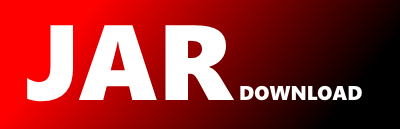
org.odftoolkit.odfdom.dom.element.style.StyleTableCellPropertiesElement Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of odfdom-java Show documentation
Show all versions of odfdom-java Show documentation
ODFDOM is an OpenDocument Format (ODF) framework. Its purpose
is to provide an easy common way to create, access and
manipulate ODF files, without requiring detailed knowledge of
the ODF specification. It is designed to provide the ODF
developer community with an easy lightwork programming API
portable to any object-oriented language.
The current reference implementation is written in Java.
/************************************************************************
*
* DO NOT ALTER OR REMOVE COPYRIGHT NOTICES OR THIS FILE HEADER
*
* Copyright 2008, 2010 Oracle and/or its affiliates. All rights reserved.
*
* Use is subject to license terms.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy
* of the License at http://www.apache.org/licenses/LICENSE-2.0. You can also
* obtain a copy of the License at http://odftoolkit.org/docs/license.txt
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
*
* See the License for the specific language governing permissions and
* limitations under the License.
*
************************************************************************/
/*
* This file is automatically generated.
* Don't edit manually.
*/
package org.odftoolkit.odfdom.dom.element.style;
import org.odftoolkit.odfdom.pkg.OdfElement;
import org.odftoolkit.odfdom.dom.style.props.OdfStyleProperty;
import org.odftoolkit.odfdom.dom.style.props.OdfStylePropertiesSet;
import org.odftoolkit.odfdom.pkg.ElementVisitor;
import org.odftoolkit.odfdom.pkg.OdfFileDom;
import org.odftoolkit.odfdom.pkg.OdfName;
import org.odftoolkit.odfdom.dom.OdfDocumentNamespace;
import org.odftoolkit.odfdom.dom.DefaultElementVisitor;
import org.odftoolkit.odfdom.dom.attribute.fo.FoBackgroundColorAttribute;
import org.odftoolkit.odfdom.dom.attribute.fo.FoBorderAttribute;
import org.odftoolkit.odfdom.dom.attribute.fo.FoBorderBottomAttribute;
import org.odftoolkit.odfdom.dom.attribute.fo.FoBorderLeftAttribute;
import org.odftoolkit.odfdom.dom.attribute.fo.FoBorderRightAttribute;
import org.odftoolkit.odfdom.dom.attribute.fo.FoBorderTopAttribute;
import org.odftoolkit.odfdom.dom.attribute.fo.FoPaddingAttribute;
import org.odftoolkit.odfdom.dom.attribute.fo.FoPaddingBottomAttribute;
import org.odftoolkit.odfdom.dom.attribute.fo.FoPaddingLeftAttribute;
import org.odftoolkit.odfdom.dom.attribute.fo.FoPaddingRightAttribute;
import org.odftoolkit.odfdom.dom.attribute.fo.FoPaddingTopAttribute;
import org.odftoolkit.odfdom.dom.attribute.fo.FoWrapOptionAttribute;
import org.odftoolkit.odfdom.dom.attribute.style.StyleBorderLineWidthAttribute;
import org.odftoolkit.odfdom.dom.attribute.style.StyleBorderLineWidthBottomAttribute;
import org.odftoolkit.odfdom.dom.attribute.style.StyleBorderLineWidthLeftAttribute;
import org.odftoolkit.odfdom.dom.attribute.style.StyleBorderLineWidthRightAttribute;
import org.odftoolkit.odfdom.dom.attribute.style.StyleBorderLineWidthTopAttribute;
import org.odftoolkit.odfdom.dom.attribute.style.StyleCellProtectAttribute;
import org.odftoolkit.odfdom.dom.attribute.style.StyleDecimalPlacesAttribute;
import org.odftoolkit.odfdom.dom.attribute.style.StyleDiagonalBlTrAttribute;
import org.odftoolkit.odfdom.dom.attribute.style.StyleDiagonalBlTrWidthsAttribute;
import org.odftoolkit.odfdom.dom.attribute.style.StyleDiagonalTlBrAttribute;
import org.odftoolkit.odfdom.dom.attribute.style.StyleDiagonalTlBrWidthsAttribute;
import org.odftoolkit.odfdom.dom.attribute.style.StyleDirectionAttribute;
import org.odftoolkit.odfdom.dom.attribute.style.StyleGlyphOrientationVerticalAttribute;
import org.odftoolkit.odfdom.dom.attribute.style.StylePrintContentAttribute;
import org.odftoolkit.odfdom.dom.attribute.style.StyleRepeatContentAttribute;
import org.odftoolkit.odfdom.dom.attribute.style.StyleRotationAlignAttribute;
import org.odftoolkit.odfdom.dom.attribute.style.StyleRotationAngleAttribute;
import org.odftoolkit.odfdom.dom.attribute.style.StyleShadowAttribute;
import org.odftoolkit.odfdom.dom.attribute.style.StyleShrinkToFitAttribute;
import org.odftoolkit.odfdom.dom.attribute.style.StyleTextAlignSourceAttribute;
import org.odftoolkit.odfdom.dom.attribute.style.StyleVerticalAlignAttribute;
import org.odftoolkit.odfdom.dom.attribute.style.StyleWritingModeAttribute;
import org.odftoolkit.odfdom.dom.element.OdfStylePropertiesBase;
/**
* DOM implementation of OpenDocument element {@odf.element style:table-cell-properties}.
*
*/
public class StyleTableCellPropertiesElement extends OdfStylePropertiesBase {
public static final OdfName ELEMENT_NAME = OdfName.newName(OdfDocumentNamespace.STYLE, "table-cell-properties");
/**
* Create the instance of StyleTableCellPropertiesElement
*
* @param ownerDoc The type is OdfFileDom
*/
public StyleTableCellPropertiesElement(OdfFileDom ownerDoc) {
super(ownerDoc, ELEMENT_NAME);
}
/**
* Get the element name
*
* @return return OdfName
the name of element {@odf.element style:table-cell-properties}.
*/
public OdfName getOdfName() {
return ELEMENT_NAME;
}
public final static OdfStyleProperty BackgroundColor =
OdfStyleProperty.get(OdfStylePropertiesSet.TableCellProperties, OdfName.newName(OdfDocumentNamespace.FO, "background-color"));
public final static OdfStyleProperty Border =
OdfStyleProperty.get(OdfStylePropertiesSet.TableCellProperties, OdfName.newName(OdfDocumentNamespace.FO, "border"));
public final static OdfStyleProperty BorderBottom =
OdfStyleProperty.get(OdfStylePropertiesSet.TableCellProperties, OdfName.newName(OdfDocumentNamespace.FO, "border-bottom"));
public final static OdfStyleProperty BorderLeft =
OdfStyleProperty.get(OdfStylePropertiesSet.TableCellProperties, OdfName.newName(OdfDocumentNamespace.FO, "border-left"));
public final static OdfStyleProperty BorderRight =
OdfStyleProperty.get(OdfStylePropertiesSet.TableCellProperties, OdfName.newName(OdfDocumentNamespace.FO, "border-right"));
public final static OdfStyleProperty BorderTop =
OdfStyleProperty.get(OdfStylePropertiesSet.TableCellProperties, OdfName.newName(OdfDocumentNamespace.FO, "border-top"));
public final static OdfStyleProperty Padding =
OdfStyleProperty.get(OdfStylePropertiesSet.TableCellProperties, OdfName.newName(OdfDocumentNamespace.FO, "padding"));
public final static OdfStyleProperty PaddingBottom =
OdfStyleProperty.get(OdfStylePropertiesSet.TableCellProperties, OdfName.newName(OdfDocumentNamespace.FO, "padding-bottom"));
public final static OdfStyleProperty PaddingLeft =
OdfStyleProperty.get(OdfStylePropertiesSet.TableCellProperties, OdfName.newName(OdfDocumentNamespace.FO, "padding-left"));
public final static OdfStyleProperty PaddingRight =
OdfStyleProperty.get(OdfStylePropertiesSet.TableCellProperties, OdfName.newName(OdfDocumentNamespace.FO, "padding-right"));
public final static OdfStyleProperty PaddingTop =
OdfStyleProperty.get(OdfStylePropertiesSet.TableCellProperties, OdfName.newName(OdfDocumentNamespace.FO, "padding-top"));
public final static OdfStyleProperty WrapOption =
OdfStyleProperty.get(OdfStylePropertiesSet.TableCellProperties, OdfName.newName(OdfDocumentNamespace.FO, "wrap-option"));
public final static OdfStyleProperty BorderLineWidth =
OdfStyleProperty.get(OdfStylePropertiesSet.TableCellProperties, OdfName.newName(OdfDocumentNamespace.STYLE, "border-line-width"));
public final static OdfStyleProperty BorderLineWidthBottom =
OdfStyleProperty.get(OdfStylePropertiesSet.TableCellProperties, OdfName.newName(OdfDocumentNamespace.STYLE, "border-line-width-bottom"));
public final static OdfStyleProperty BorderLineWidthLeft =
OdfStyleProperty.get(OdfStylePropertiesSet.TableCellProperties, OdfName.newName(OdfDocumentNamespace.STYLE, "border-line-width-left"));
public final static OdfStyleProperty BorderLineWidthRight =
OdfStyleProperty.get(OdfStylePropertiesSet.TableCellProperties, OdfName.newName(OdfDocumentNamespace.STYLE, "border-line-width-right"));
public final static OdfStyleProperty BorderLineWidthTop =
OdfStyleProperty.get(OdfStylePropertiesSet.TableCellProperties, OdfName.newName(OdfDocumentNamespace.STYLE, "border-line-width-top"));
public final static OdfStyleProperty CellProtect =
OdfStyleProperty.get(OdfStylePropertiesSet.TableCellProperties, OdfName.newName(OdfDocumentNamespace.STYLE, "cell-protect"));
public final static OdfStyleProperty DecimalPlaces =
OdfStyleProperty.get(OdfStylePropertiesSet.TableCellProperties, OdfName.newName(OdfDocumentNamespace.STYLE, "decimal-places"));
public final static OdfStyleProperty DiagonalBlTr =
OdfStyleProperty.get(OdfStylePropertiesSet.TableCellProperties, OdfName.newName(OdfDocumentNamespace.STYLE, "diagonal-bl-tr"));
public final static OdfStyleProperty DiagonalBlTrWidths =
OdfStyleProperty.get(OdfStylePropertiesSet.TableCellProperties, OdfName.newName(OdfDocumentNamespace.STYLE, "diagonal-bl-tr-widths"));
public final static OdfStyleProperty DiagonalTlBr =
OdfStyleProperty.get(OdfStylePropertiesSet.TableCellProperties, OdfName.newName(OdfDocumentNamespace.STYLE, "diagonal-tl-br"));
public final static OdfStyleProperty DiagonalTlBrWidths =
OdfStyleProperty.get(OdfStylePropertiesSet.TableCellProperties, OdfName.newName(OdfDocumentNamespace.STYLE, "diagonal-tl-br-widths"));
public final static OdfStyleProperty Direction =
OdfStyleProperty.get(OdfStylePropertiesSet.TableCellProperties, OdfName.newName(OdfDocumentNamespace.STYLE, "direction"));
public final static OdfStyleProperty GlyphOrientationVertical =
OdfStyleProperty.get(OdfStylePropertiesSet.TableCellProperties, OdfName.newName(OdfDocumentNamespace.STYLE, "glyph-orientation-vertical"));
public final static OdfStyleProperty PrintContent =
OdfStyleProperty.get(OdfStylePropertiesSet.TableCellProperties, OdfName.newName(OdfDocumentNamespace.STYLE, "print-content"));
public final static OdfStyleProperty RepeatContent =
OdfStyleProperty.get(OdfStylePropertiesSet.TableCellProperties, OdfName.newName(OdfDocumentNamespace.STYLE, "repeat-content"));
public final static OdfStyleProperty RotationAlign =
OdfStyleProperty.get(OdfStylePropertiesSet.TableCellProperties, OdfName.newName(OdfDocumentNamespace.STYLE, "rotation-align"));
public final static OdfStyleProperty RotationAngle =
OdfStyleProperty.get(OdfStylePropertiesSet.TableCellProperties, OdfName.newName(OdfDocumentNamespace.STYLE, "rotation-angle"));
public final static OdfStyleProperty Shadow =
OdfStyleProperty.get(OdfStylePropertiesSet.TableCellProperties, OdfName.newName(OdfDocumentNamespace.STYLE, "shadow"));
public final static OdfStyleProperty ShrinkToFit =
OdfStyleProperty.get(OdfStylePropertiesSet.TableCellProperties, OdfName.newName(OdfDocumentNamespace.STYLE, "shrink-to-fit"));
public final static OdfStyleProperty TextAlignSource =
OdfStyleProperty.get(OdfStylePropertiesSet.TableCellProperties, OdfName.newName(OdfDocumentNamespace.STYLE, "text-align-source"));
public final static OdfStyleProperty VerticalAlign =
OdfStyleProperty.get(OdfStylePropertiesSet.TableCellProperties, OdfName.newName(OdfDocumentNamespace.STYLE, "vertical-align"));
public final static OdfStyleProperty WritingMode =
OdfStyleProperty.get(OdfStylePropertiesSet.TableCellProperties, OdfName.newName(OdfDocumentNamespace.STYLE, "writing-mode"));
/**
* Receives the value of the ODFDOM attribute representation FoBackgroundColorAttribute
, See {@odf.attribute fo:background-color}
*
* @return - the String
, the value or null
, if the attribute is not set and no default value defined.
*/
public String getFoBackgroundColorAttribute() {
FoBackgroundColorAttribute attr = (FoBackgroundColorAttribute) getOdfAttribute(OdfDocumentNamespace.FO, "background-color");
if (attr != null) {
return String.valueOf(attr.getValue());
}
return null;
}
/**
* Sets the value of ODFDOM attribute representation FoBackgroundColorAttribute
, See {@odf.attribute fo:background-color}
*
* @param foBackgroundColorValue The type is String
*/
public void setFoBackgroundColorAttribute(String foBackgroundColorValue) {
FoBackgroundColorAttribute attr = new FoBackgroundColorAttribute((OdfFileDom) this.ownerDocument);
setOdfAttribute(attr);
attr.setValue(foBackgroundColorValue);
}
/**
* Receives the value of the ODFDOM attribute representation FoBorderAttribute
, See {@odf.attribute fo:border}
*
* @return - the String
, the value or null
, if the attribute is not set and no default value defined.
*/
public String getFoBorderAttribute() {
FoBorderAttribute attr = (FoBorderAttribute) getOdfAttribute(OdfDocumentNamespace.FO, "border");
if (attr != null) {
return String.valueOf(attr.getValue());
}
return null;
}
/**
* Sets the value of ODFDOM attribute representation FoBorderAttribute
, See {@odf.attribute fo:border}
*
* @param foBorderValue The type is String
*/
public void setFoBorderAttribute(String foBorderValue) {
FoBorderAttribute attr = new FoBorderAttribute((OdfFileDom) this.ownerDocument);
setOdfAttribute(attr);
attr.setValue(foBorderValue);
}
/**
* Receives the value of the ODFDOM attribute representation FoBorderBottomAttribute
, See {@odf.attribute fo:border-bottom}
*
* @return - the String
, the value or null
, if the attribute is not set and no default value defined.
*/
public String getFoBorderBottomAttribute() {
FoBorderBottomAttribute attr = (FoBorderBottomAttribute) getOdfAttribute(OdfDocumentNamespace.FO, "border-bottom");
if (attr != null) {
return String.valueOf(attr.getValue());
}
return null;
}
/**
* Sets the value of ODFDOM attribute representation FoBorderBottomAttribute
, See {@odf.attribute fo:border-bottom}
*
* @param foBorderBottomValue The type is String
*/
public void setFoBorderBottomAttribute(String foBorderBottomValue) {
FoBorderBottomAttribute attr = new FoBorderBottomAttribute((OdfFileDom) this.ownerDocument);
setOdfAttribute(attr);
attr.setValue(foBorderBottomValue);
}
/**
* Receives the value of the ODFDOM attribute representation FoBorderLeftAttribute
, See {@odf.attribute fo:border-left}
*
* @return - the String
, the value or null
, if the attribute is not set and no default value defined.
*/
public String getFoBorderLeftAttribute() {
FoBorderLeftAttribute attr = (FoBorderLeftAttribute) getOdfAttribute(OdfDocumentNamespace.FO, "border-left");
if (attr != null) {
return String.valueOf(attr.getValue());
}
return null;
}
/**
* Sets the value of ODFDOM attribute representation FoBorderLeftAttribute
, See {@odf.attribute fo:border-left}
*
* @param foBorderLeftValue The type is String
*/
public void setFoBorderLeftAttribute(String foBorderLeftValue) {
FoBorderLeftAttribute attr = new FoBorderLeftAttribute((OdfFileDom) this.ownerDocument);
setOdfAttribute(attr);
attr.setValue(foBorderLeftValue);
}
/**
* Receives the value of the ODFDOM attribute representation FoBorderRightAttribute
, See {@odf.attribute fo:border-right}
*
* @return - the String
, the value or null
, if the attribute is not set and no default value defined.
*/
public String getFoBorderRightAttribute() {
FoBorderRightAttribute attr = (FoBorderRightAttribute) getOdfAttribute(OdfDocumentNamespace.FO, "border-right");
if (attr != null) {
return String.valueOf(attr.getValue());
}
return null;
}
/**
* Sets the value of ODFDOM attribute representation FoBorderRightAttribute
, See {@odf.attribute fo:border-right}
*
* @param foBorderRightValue The type is String
*/
public void setFoBorderRightAttribute(String foBorderRightValue) {
FoBorderRightAttribute attr = new FoBorderRightAttribute((OdfFileDom) this.ownerDocument);
setOdfAttribute(attr);
attr.setValue(foBorderRightValue);
}
/**
* Receives the value of the ODFDOM attribute representation FoBorderTopAttribute
, See {@odf.attribute fo:border-top}
*
* @return - the String
, the value or null
, if the attribute is not set and no default value defined.
*/
public String getFoBorderTopAttribute() {
FoBorderTopAttribute attr = (FoBorderTopAttribute) getOdfAttribute(OdfDocumentNamespace.FO, "border-top");
if (attr != null) {
return String.valueOf(attr.getValue());
}
return null;
}
/**
* Sets the value of ODFDOM attribute representation FoBorderTopAttribute
, See {@odf.attribute fo:border-top}
*
* @param foBorderTopValue The type is String
*/
public void setFoBorderTopAttribute(String foBorderTopValue) {
FoBorderTopAttribute attr = new FoBorderTopAttribute((OdfFileDom) this.ownerDocument);
setOdfAttribute(attr);
attr.setValue(foBorderTopValue);
}
/**
* Receives the value of the ODFDOM attribute representation FoPaddingAttribute
, See {@odf.attribute fo:padding}
*
* @return - the String
, the value or null
, if the attribute is not set and no default value defined.
*/
public String getFoPaddingAttribute() {
FoPaddingAttribute attr = (FoPaddingAttribute) getOdfAttribute(OdfDocumentNamespace.FO, "padding");
if (attr != null) {
return String.valueOf(attr.getValue());
}
return null;
}
/**
* Sets the value of ODFDOM attribute representation FoPaddingAttribute
, See {@odf.attribute fo:padding}
*
* @param foPaddingValue The type is String
*/
public void setFoPaddingAttribute(String foPaddingValue) {
FoPaddingAttribute attr = new FoPaddingAttribute((OdfFileDom) this.ownerDocument);
setOdfAttribute(attr);
attr.setValue(foPaddingValue);
}
/**
* Receives the value of the ODFDOM attribute representation FoPaddingBottomAttribute
, See {@odf.attribute fo:padding-bottom}
*
* @return - the String
, the value or null
, if the attribute is not set and no default value defined.
*/
public String getFoPaddingBottomAttribute() {
FoPaddingBottomAttribute attr = (FoPaddingBottomAttribute) getOdfAttribute(OdfDocumentNamespace.FO, "padding-bottom");
if (attr != null) {
return String.valueOf(attr.getValue());
}
return null;
}
/**
* Sets the value of ODFDOM attribute representation FoPaddingBottomAttribute
, See {@odf.attribute fo:padding-bottom}
*
* @param foPaddingBottomValue The type is String
*/
public void setFoPaddingBottomAttribute(String foPaddingBottomValue) {
FoPaddingBottomAttribute attr = new FoPaddingBottomAttribute((OdfFileDom) this.ownerDocument);
setOdfAttribute(attr);
attr.setValue(foPaddingBottomValue);
}
/**
* Receives the value of the ODFDOM attribute representation FoPaddingLeftAttribute
, See {@odf.attribute fo:padding-left}
*
* @return - the String
, the value or null
, if the attribute is not set and no default value defined.
*/
public String getFoPaddingLeftAttribute() {
FoPaddingLeftAttribute attr = (FoPaddingLeftAttribute) getOdfAttribute(OdfDocumentNamespace.FO, "padding-left");
if (attr != null) {
return String.valueOf(attr.getValue());
}
return null;
}
/**
* Sets the value of ODFDOM attribute representation FoPaddingLeftAttribute
, See {@odf.attribute fo:padding-left}
*
* @param foPaddingLeftValue The type is String
*/
public void setFoPaddingLeftAttribute(String foPaddingLeftValue) {
FoPaddingLeftAttribute attr = new FoPaddingLeftAttribute((OdfFileDom) this.ownerDocument);
setOdfAttribute(attr);
attr.setValue(foPaddingLeftValue);
}
/**
* Receives the value of the ODFDOM attribute representation FoPaddingRightAttribute
, See {@odf.attribute fo:padding-right}
*
* @return - the String
, the value or null
, if the attribute is not set and no default value defined.
*/
public String getFoPaddingRightAttribute() {
FoPaddingRightAttribute attr = (FoPaddingRightAttribute) getOdfAttribute(OdfDocumentNamespace.FO, "padding-right");
if (attr != null) {
return String.valueOf(attr.getValue());
}
return null;
}
/**
* Sets the value of ODFDOM attribute representation FoPaddingRightAttribute
, See {@odf.attribute fo:padding-right}
*
* @param foPaddingRightValue The type is String
*/
public void setFoPaddingRightAttribute(String foPaddingRightValue) {
FoPaddingRightAttribute attr = new FoPaddingRightAttribute((OdfFileDom) this.ownerDocument);
setOdfAttribute(attr);
attr.setValue(foPaddingRightValue);
}
/**
* Receives the value of the ODFDOM attribute representation FoPaddingTopAttribute
, See {@odf.attribute fo:padding-top}
*
* @return - the String
, the value or null
, if the attribute is not set and no default value defined.
*/
public String getFoPaddingTopAttribute() {
FoPaddingTopAttribute attr = (FoPaddingTopAttribute) getOdfAttribute(OdfDocumentNamespace.FO, "padding-top");
if (attr != null) {
return String.valueOf(attr.getValue());
}
return null;
}
/**
* Sets the value of ODFDOM attribute representation FoPaddingTopAttribute
, See {@odf.attribute fo:padding-top}
*
* @param foPaddingTopValue The type is String
*/
public void setFoPaddingTopAttribute(String foPaddingTopValue) {
FoPaddingTopAttribute attr = new FoPaddingTopAttribute((OdfFileDom) this.ownerDocument);
setOdfAttribute(attr);
attr.setValue(foPaddingTopValue);
}
/**
* Receives the value of the ODFDOM attribute representation FoWrapOptionAttribute
, See {@odf.attribute fo:wrap-option}
*
* @return - the String
, the value or null
, if the attribute is not set and no default value defined.
*/
public String getFoWrapOptionAttribute() {
FoWrapOptionAttribute attr = (FoWrapOptionAttribute) getOdfAttribute(OdfDocumentNamespace.FO, "wrap-option");
if (attr != null) {
return String.valueOf(attr.getValue());
}
return null;
}
/**
* Sets the value of ODFDOM attribute representation FoWrapOptionAttribute
, See {@odf.attribute fo:wrap-option}
*
* @param foWrapOptionValue The type is String
*/
public void setFoWrapOptionAttribute(String foWrapOptionValue) {
FoWrapOptionAttribute attr = new FoWrapOptionAttribute((OdfFileDom) this.ownerDocument);
setOdfAttribute(attr);
attr.setValue(foWrapOptionValue);
}
/**
* Receives the value of the ODFDOM attribute representation StyleBorderLineWidthAttribute
, See {@odf.attribute style:border-line-width}
*
* @return - the String
, the value or null
, if the attribute is not set and no default value defined.
*/
public String getStyleBorderLineWidthAttribute() {
StyleBorderLineWidthAttribute attr = (StyleBorderLineWidthAttribute) getOdfAttribute(OdfDocumentNamespace.STYLE, "border-line-width");
if (attr != null) {
return String.valueOf(attr.getValue());
}
return null;
}
/**
* Sets the value of ODFDOM attribute representation StyleBorderLineWidthAttribute
, See {@odf.attribute style:border-line-width}
*
* @param styleBorderLineWidthValue The type is String
*/
public void setStyleBorderLineWidthAttribute(String styleBorderLineWidthValue) {
StyleBorderLineWidthAttribute attr = new StyleBorderLineWidthAttribute((OdfFileDom) this.ownerDocument);
setOdfAttribute(attr);
attr.setValue(styleBorderLineWidthValue);
}
/**
* Receives the value of the ODFDOM attribute representation StyleBorderLineWidthBottomAttribute
, See {@odf.attribute style:border-line-width-bottom}
*
* @return - the String
, the value or null
, if the attribute is not set and no default value defined.
*/
public String getStyleBorderLineWidthBottomAttribute() {
StyleBorderLineWidthBottomAttribute attr = (StyleBorderLineWidthBottomAttribute) getOdfAttribute(OdfDocumentNamespace.STYLE, "border-line-width-bottom");
if (attr != null) {
return String.valueOf(attr.getValue());
}
return null;
}
/**
* Sets the value of ODFDOM attribute representation StyleBorderLineWidthBottomAttribute
, See {@odf.attribute style:border-line-width-bottom}
*
* @param styleBorderLineWidthBottomValue The type is String
*/
public void setStyleBorderLineWidthBottomAttribute(String styleBorderLineWidthBottomValue) {
StyleBorderLineWidthBottomAttribute attr = new StyleBorderLineWidthBottomAttribute((OdfFileDom) this.ownerDocument);
setOdfAttribute(attr);
attr.setValue(styleBorderLineWidthBottomValue);
}
/**
* Receives the value of the ODFDOM attribute representation StyleBorderLineWidthLeftAttribute
, See {@odf.attribute style:border-line-width-left}
*
* @return - the String
, the value or null
, if the attribute is not set and no default value defined.
*/
public String getStyleBorderLineWidthLeftAttribute() {
StyleBorderLineWidthLeftAttribute attr = (StyleBorderLineWidthLeftAttribute) getOdfAttribute(OdfDocumentNamespace.STYLE, "border-line-width-left");
if (attr != null) {
return String.valueOf(attr.getValue());
}
return null;
}
/**
* Sets the value of ODFDOM attribute representation StyleBorderLineWidthLeftAttribute
, See {@odf.attribute style:border-line-width-left}
*
* @param styleBorderLineWidthLeftValue The type is String
*/
public void setStyleBorderLineWidthLeftAttribute(String styleBorderLineWidthLeftValue) {
StyleBorderLineWidthLeftAttribute attr = new StyleBorderLineWidthLeftAttribute((OdfFileDom) this.ownerDocument);
setOdfAttribute(attr);
attr.setValue(styleBorderLineWidthLeftValue);
}
/**
* Receives the value of the ODFDOM attribute representation StyleBorderLineWidthRightAttribute
, See {@odf.attribute style:border-line-width-right}
*
* @return - the String
, the value or null
, if the attribute is not set and no default value defined.
*/
public String getStyleBorderLineWidthRightAttribute() {
StyleBorderLineWidthRightAttribute attr = (StyleBorderLineWidthRightAttribute) getOdfAttribute(OdfDocumentNamespace.STYLE, "border-line-width-right");
if (attr != null) {
return String.valueOf(attr.getValue());
}
return null;
}
/**
* Sets the value of ODFDOM attribute representation StyleBorderLineWidthRightAttribute
, See {@odf.attribute style:border-line-width-right}
*
* @param styleBorderLineWidthRightValue The type is String
*/
public void setStyleBorderLineWidthRightAttribute(String styleBorderLineWidthRightValue) {
StyleBorderLineWidthRightAttribute attr = new StyleBorderLineWidthRightAttribute((OdfFileDom) this.ownerDocument);
setOdfAttribute(attr);
attr.setValue(styleBorderLineWidthRightValue);
}
/**
* Receives the value of the ODFDOM attribute representation StyleBorderLineWidthTopAttribute
, See {@odf.attribute style:border-line-width-top}
*
* @return - the String
, the value or null
, if the attribute is not set and no default value defined.
*/
public String getStyleBorderLineWidthTopAttribute() {
StyleBorderLineWidthTopAttribute attr = (StyleBorderLineWidthTopAttribute) getOdfAttribute(OdfDocumentNamespace.STYLE, "border-line-width-top");
if (attr != null) {
return String.valueOf(attr.getValue());
}
return null;
}
/**
* Sets the value of ODFDOM attribute representation StyleBorderLineWidthTopAttribute
, See {@odf.attribute style:border-line-width-top}
*
* @param styleBorderLineWidthTopValue The type is String
*/
public void setStyleBorderLineWidthTopAttribute(String styleBorderLineWidthTopValue) {
StyleBorderLineWidthTopAttribute attr = new StyleBorderLineWidthTopAttribute((OdfFileDom) this.ownerDocument);
setOdfAttribute(attr);
attr.setValue(styleBorderLineWidthTopValue);
}
/**
* Receives the value of the ODFDOM attribute representation StyleCellProtectAttribute
, See {@odf.attribute style:cell-protect}
*
* @return - the String
, the value or null
, if the attribute is not set and no default value defined.
*/
public String getStyleCellProtectAttribute() {
StyleCellProtectAttribute attr = (StyleCellProtectAttribute) getOdfAttribute(OdfDocumentNamespace.STYLE, "cell-protect");
if (attr != null) {
return String.valueOf(attr.getValue());
}
return null;
}
/**
* Sets the value of ODFDOM attribute representation StyleCellProtectAttribute
, See {@odf.attribute style:cell-protect}
*
* @param styleCellProtectValue The type is String
*/
public void setStyleCellProtectAttribute(String styleCellProtectValue) {
StyleCellProtectAttribute attr = new StyleCellProtectAttribute((OdfFileDom) this.ownerDocument);
setOdfAttribute(attr);
attr.setValue(styleCellProtectValue);
}
/**
* Receives the value of the ODFDOM attribute representation StyleDecimalPlacesAttribute
, See {@odf.attribute style:decimal-places}
*
* @return - the Integer
, the value or null
, if the attribute is not set and no default value defined.
*/
public Integer getStyleDecimalPlacesAttribute() {
StyleDecimalPlacesAttribute attr = (StyleDecimalPlacesAttribute) getOdfAttribute(OdfDocumentNamespace.STYLE, "decimal-places");
if (attr != null) {
return Integer.valueOf(attr.intValue());
}
return null;
}
/**
* Sets the value of ODFDOM attribute representation StyleDecimalPlacesAttribute
, See {@odf.attribute style:decimal-places}
*
* @param styleDecimalPlacesValue The type is Integer
*/
public void setStyleDecimalPlacesAttribute(Integer styleDecimalPlacesValue) {
StyleDecimalPlacesAttribute attr = new StyleDecimalPlacesAttribute((OdfFileDom) this.ownerDocument);
setOdfAttribute(attr);
attr.setIntValue(styleDecimalPlacesValue.intValue());
}
/**
* Receives the value of the ODFDOM attribute representation StyleDiagonalBlTrAttribute
, See {@odf.attribute style:diagonal-bl-tr}
*
* @return - the String
, the value or null
, if the attribute is not set and no default value defined.
*/
public String getStyleDiagonalBlTrAttribute() {
StyleDiagonalBlTrAttribute attr = (StyleDiagonalBlTrAttribute) getOdfAttribute(OdfDocumentNamespace.STYLE, "diagonal-bl-tr");
if (attr != null) {
return String.valueOf(attr.getValue());
}
return null;
}
/**
* Sets the value of ODFDOM attribute representation StyleDiagonalBlTrAttribute
, See {@odf.attribute style:diagonal-bl-tr}
*
* @param styleDiagonalBlTrValue The type is String
*/
public void setStyleDiagonalBlTrAttribute(String styleDiagonalBlTrValue) {
StyleDiagonalBlTrAttribute attr = new StyleDiagonalBlTrAttribute((OdfFileDom) this.ownerDocument);
setOdfAttribute(attr);
attr.setValue(styleDiagonalBlTrValue);
}
/**
* Receives the value of the ODFDOM attribute representation StyleDiagonalBlTrWidthsAttribute
, See {@odf.attribute style:diagonal-bl-tr-widths}
*
* @return - the String
, the value or null
, if the attribute is not set and no default value defined.
*/
public String getStyleDiagonalBlTrWidthsAttribute() {
StyleDiagonalBlTrWidthsAttribute attr = (StyleDiagonalBlTrWidthsAttribute) getOdfAttribute(OdfDocumentNamespace.STYLE, "diagonal-bl-tr-widths");
if (attr != null) {
return String.valueOf(attr.getValue());
}
return null;
}
/**
* Sets the value of ODFDOM attribute representation StyleDiagonalBlTrWidthsAttribute
, See {@odf.attribute style:diagonal-bl-tr-widths}
*
* @param styleDiagonalBlTrWidthsValue The type is String
*/
public void setStyleDiagonalBlTrWidthsAttribute(String styleDiagonalBlTrWidthsValue) {
StyleDiagonalBlTrWidthsAttribute attr = new StyleDiagonalBlTrWidthsAttribute((OdfFileDom) this.ownerDocument);
setOdfAttribute(attr);
attr.setValue(styleDiagonalBlTrWidthsValue);
}
/**
* Receives the value of the ODFDOM attribute representation StyleDiagonalTlBrAttribute
, See {@odf.attribute style:diagonal-tl-br}
*
* @return - the String
, the value or null
, if the attribute is not set and no default value defined.
*/
public String getStyleDiagonalTlBrAttribute() {
StyleDiagonalTlBrAttribute attr = (StyleDiagonalTlBrAttribute) getOdfAttribute(OdfDocumentNamespace.STYLE, "diagonal-tl-br");
if (attr != null) {
return String.valueOf(attr.getValue());
}
return null;
}
/**
* Sets the value of ODFDOM attribute representation StyleDiagonalTlBrAttribute
, See {@odf.attribute style:diagonal-tl-br}
*
* @param styleDiagonalTlBrValue The type is String
*/
public void setStyleDiagonalTlBrAttribute(String styleDiagonalTlBrValue) {
StyleDiagonalTlBrAttribute attr = new StyleDiagonalTlBrAttribute((OdfFileDom) this.ownerDocument);
setOdfAttribute(attr);
attr.setValue(styleDiagonalTlBrValue);
}
/**
* Receives the value of the ODFDOM attribute representation StyleDiagonalTlBrWidthsAttribute
, See {@odf.attribute style:diagonal-tl-br-widths}
*
* @return - the String
, the value or null
, if the attribute is not set and no default value defined.
*/
public String getStyleDiagonalTlBrWidthsAttribute() {
StyleDiagonalTlBrWidthsAttribute attr = (StyleDiagonalTlBrWidthsAttribute) getOdfAttribute(OdfDocumentNamespace.STYLE, "diagonal-tl-br-widths");
if (attr != null) {
return String.valueOf(attr.getValue());
}
return null;
}
/**
* Sets the value of ODFDOM attribute representation StyleDiagonalTlBrWidthsAttribute
, See {@odf.attribute style:diagonal-tl-br-widths}
*
* @param styleDiagonalTlBrWidthsValue The type is String
*/
public void setStyleDiagonalTlBrWidthsAttribute(String styleDiagonalTlBrWidthsValue) {
StyleDiagonalTlBrWidthsAttribute attr = new StyleDiagonalTlBrWidthsAttribute((OdfFileDom) this.ownerDocument);
setOdfAttribute(attr);
attr.setValue(styleDiagonalTlBrWidthsValue);
}
/**
* Receives the value of the ODFDOM attribute representation StyleDirectionAttribute
, See {@odf.attribute style:direction}
*
* @return - the String
, the value or null
, if the attribute is not set and no default value defined.
*/
public String getStyleDirectionAttribute() {
StyleDirectionAttribute attr = (StyleDirectionAttribute) getOdfAttribute(OdfDocumentNamespace.STYLE, "direction");
if (attr != null) {
return String.valueOf(attr.getValue());
}
return null;
}
/**
* Sets the value of ODFDOM attribute representation StyleDirectionAttribute
, See {@odf.attribute style:direction}
*
* @param styleDirectionValue The type is String
*/
public void setStyleDirectionAttribute(String styleDirectionValue) {
StyleDirectionAttribute attr = new StyleDirectionAttribute((OdfFileDom) this.ownerDocument);
setOdfAttribute(attr);
attr.setValue(styleDirectionValue);
}
/**
* Receives the value of the ODFDOM attribute representation StyleGlyphOrientationVerticalAttribute
, See {@odf.attribute style:glyph-orientation-vertical}
*
* @return - the String
, the value or null
, if the attribute is not set and no default value defined.
*/
public String getStyleGlyphOrientationVerticalAttribute() {
StyleGlyphOrientationVerticalAttribute attr = (StyleGlyphOrientationVerticalAttribute) getOdfAttribute(OdfDocumentNamespace.STYLE, "glyph-orientation-vertical");
if (attr != null) {
return String.valueOf(attr.getValue());
}
return null;
}
/**
* Sets the value of ODFDOM attribute representation StyleGlyphOrientationVerticalAttribute
, See {@odf.attribute style:glyph-orientation-vertical}
*
* @param styleGlyphOrientationVerticalValue The type is String
*/
public void setStyleGlyphOrientationVerticalAttribute(String styleGlyphOrientationVerticalValue) {
StyleGlyphOrientationVerticalAttribute attr = new StyleGlyphOrientationVerticalAttribute((OdfFileDom) this.ownerDocument);
setOdfAttribute(attr);
attr.setValue(styleGlyphOrientationVerticalValue);
}
/**
* Receives the value of the ODFDOM attribute representation StylePrintContentAttribute
, See {@odf.attribute style:print-content}
*
* @return - the Boolean
, the value or null
, if the attribute is not set and no default value defined.
*/
public Boolean getStylePrintContentAttribute() {
StylePrintContentAttribute attr = (StylePrintContentAttribute) getOdfAttribute(OdfDocumentNamespace.STYLE, "print-content");
if (attr != null) {
return Boolean.valueOf(attr.booleanValue());
}
return null;
}
/**
* Sets the value of ODFDOM attribute representation StylePrintContentAttribute
, See {@odf.attribute style:print-content}
*
* @param stylePrintContentValue The type is Boolean
*/
public void setStylePrintContentAttribute(Boolean stylePrintContentValue) {
StylePrintContentAttribute attr = new StylePrintContentAttribute((OdfFileDom) this.ownerDocument);
setOdfAttribute(attr);
attr.setBooleanValue(stylePrintContentValue.booleanValue());
}
/**
* Receives the value of the ODFDOM attribute representation StyleRepeatContentAttribute
, See {@odf.attribute style:repeat-content}
*
* @return - the Boolean
, the value or null
, if the attribute is not set and no default value defined.
*/
public Boolean getStyleRepeatContentAttribute() {
StyleRepeatContentAttribute attr = (StyleRepeatContentAttribute) getOdfAttribute(OdfDocumentNamespace.STYLE, "repeat-content");
if (attr != null) {
return Boolean.valueOf(attr.booleanValue());
}
return null;
}
/**
* Sets the value of ODFDOM attribute representation StyleRepeatContentAttribute
, See {@odf.attribute style:repeat-content}
*
* @param styleRepeatContentValue The type is Boolean
*/
public void setStyleRepeatContentAttribute(Boolean styleRepeatContentValue) {
StyleRepeatContentAttribute attr = new StyleRepeatContentAttribute((OdfFileDom) this.ownerDocument);
setOdfAttribute(attr);
attr.setBooleanValue(styleRepeatContentValue.booleanValue());
}
/**
* Receives the value of the ODFDOM attribute representation StyleRotationAlignAttribute
, See {@odf.attribute style:rotation-align}
*
* @return - the String
, the value or null
, if the attribute is not set and no default value defined.
*/
public String getStyleRotationAlignAttribute() {
StyleRotationAlignAttribute attr = (StyleRotationAlignAttribute) getOdfAttribute(OdfDocumentNamespace.STYLE, "rotation-align");
if (attr != null) {
return String.valueOf(attr.getValue());
}
return null;
}
/**
* Sets the value of ODFDOM attribute representation StyleRotationAlignAttribute
, See {@odf.attribute style:rotation-align}
*
* @param styleRotationAlignValue The type is String
*/
public void setStyleRotationAlignAttribute(String styleRotationAlignValue) {
StyleRotationAlignAttribute attr = new StyleRotationAlignAttribute((OdfFileDom) this.ownerDocument);
setOdfAttribute(attr);
attr.setValue(styleRotationAlignValue);
}
/**
* Receives the value of the ODFDOM attribute representation StyleRotationAngleAttribute
, See {@odf.attribute style:rotation-angle}
*
* @return - the String
, the value or null
, if the attribute is not set and no default value defined.
*/
public String getStyleRotationAngleAttribute() {
StyleRotationAngleAttribute attr = (StyleRotationAngleAttribute) getOdfAttribute(OdfDocumentNamespace.STYLE, "rotation-angle");
if (attr != null) {
return String.valueOf(attr.getValue());
}
return null;
}
/**
* Sets the value of ODFDOM attribute representation StyleRotationAngleAttribute
, See {@odf.attribute style:rotation-angle}
*
* @param styleRotationAngleValue The type is String
*/
public void setStyleRotationAngleAttribute(String styleRotationAngleValue) {
StyleRotationAngleAttribute attr = new StyleRotationAngleAttribute((OdfFileDom) this.ownerDocument);
setOdfAttribute(attr);
attr.setValue(styleRotationAngleValue);
}
/**
* Receives the value of the ODFDOM attribute representation StyleShadowAttribute
, See {@odf.attribute style:shadow}
*
* @return - the String
, the value or null
, if the attribute is not set and no default value defined.
*/
public String getStyleShadowAttribute() {
StyleShadowAttribute attr = (StyleShadowAttribute) getOdfAttribute(OdfDocumentNamespace.STYLE, "shadow");
if (attr != null) {
return String.valueOf(attr.getValue());
}
return null;
}
/**
* Sets the value of ODFDOM attribute representation StyleShadowAttribute
, See {@odf.attribute style:shadow}
*
* @param styleShadowValue The type is String
*/
public void setStyleShadowAttribute(String styleShadowValue) {
StyleShadowAttribute attr = new StyleShadowAttribute((OdfFileDom) this.ownerDocument);
setOdfAttribute(attr);
attr.setValue(styleShadowValue);
}
/**
* Receives the value of the ODFDOM attribute representation StyleShrinkToFitAttribute
, See {@odf.attribute style:shrink-to-fit}
*
* @return - the Boolean
, the value or null
, if the attribute is not set and no default value defined.
*/
public Boolean getStyleShrinkToFitAttribute() {
StyleShrinkToFitAttribute attr = (StyleShrinkToFitAttribute) getOdfAttribute(OdfDocumentNamespace.STYLE, "shrink-to-fit");
if (attr != null) {
return Boolean.valueOf(attr.booleanValue());
}
return null;
}
/**
* Sets the value of ODFDOM attribute representation StyleShrinkToFitAttribute
, See {@odf.attribute style:shrink-to-fit}
*
* @param styleShrinkToFitValue The type is Boolean
*/
public void setStyleShrinkToFitAttribute(Boolean styleShrinkToFitValue) {
StyleShrinkToFitAttribute attr = new StyleShrinkToFitAttribute((OdfFileDom) this.ownerDocument);
setOdfAttribute(attr);
attr.setBooleanValue(styleShrinkToFitValue.booleanValue());
}
/**
* Receives the value of the ODFDOM attribute representation StyleTextAlignSourceAttribute
, See {@odf.attribute style:text-align-source}
*
* @return - the String
, the value or null
, if the attribute is not set and no default value defined.
*/
public String getStyleTextAlignSourceAttribute() {
StyleTextAlignSourceAttribute attr = (StyleTextAlignSourceAttribute) getOdfAttribute(OdfDocumentNamespace.STYLE, "text-align-source");
if (attr != null) {
return String.valueOf(attr.getValue());
}
return null;
}
/**
* Sets the value of ODFDOM attribute representation StyleTextAlignSourceAttribute
, See {@odf.attribute style:text-align-source}
*
* @param styleTextAlignSourceValue The type is String
*/
public void setStyleTextAlignSourceAttribute(String styleTextAlignSourceValue) {
StyleTextAlignSourceAttribute attr = new StyleTextAlignSourceAttribute((OdfFileDom) this.ownerDocument);
setOdfAttribute(attr);
attr.setValue(styleTextAlignSourceValue);
}
/**
* Receives the value of the ODFDOM attribute representation StyleVerticalAlignAttribute
, See {@odf.attribute style:vertical-align}
*
* @return - the String
, the value or null
, if the attribute is not set and no default value defined.
*/
public String getStyleVerticalAlignAttribute() {
StyleVerticalAlignAttribute attr = (StyleVerticalAlignAttribute) getOdfAttribute(OdfDocumentNamespace.STYLE, "vertical-align");
if (attr != null) {
return String.valueOf(attr.getValue());
}
return null;
}
/**
* Sets the value of ODFDOM attribute representation StyleVerticalAlignAttribute
, See {@odf.attribute style:vertical-align}
*
* @param styleVerticalAlignValue The type is String
*/
public void setStyleVerticalAlignAttribute(String styleVerticalAlignValue) {
StyleVerticalAlignAttribute attr = new StyleVerticalAlignAttribute((OdfFileDom) this.ownerDocument);
setOdfAttribute(attr);
attr.setValue(styleVerticalAlignValue);
}
/**
* Receives the value of the ODFDOM attribute representation StyleWritingModeAttribute
, See {@odf.attribute style:writing-mode}
*
* @return - the String
, the value or null
, if the attribute is not set and no default value defined.
*/
public String getStyleWritingModeAttribute() {
StyleWritingModeAttribute attr = (StyleWritingModeAttribute) getOdfAttribute(OdfDocumentNamespace.STYLE, "writing-mode");
if (attr != null) {
return String.valueOf(attr.getValue());
}
return null;
}
/**
* Sets the value of ODFDOM attribute representation StyleWritingModeAttribute
, See {@odf.attribute style:writing-mode}
*
* @param styleWritingModeValue The type is String
*/
public void setStyleWritingModeAttribute(String styleWritingModeValue) {
StyleWritingModeAttribute attr = new StyleWritingModeAttribute((OdfFileDom) this.ownerDocument);
setOdfAttribute(attr);
attr.setValue(styleWritingModeValue);
}
/**
* Create child element {@odf.element style:background-image}.
*
* Child element is new in Odf 1.2
*
* @return the element {@odf.element style:background-image}
*/
public StyleBackgroundImageElement newStyleBackgroundImageElement() {
StyleBackgroundImageElement styleBackgroundImage = ((OdfFileDom) this.ownerDocument).newOdfElement(StyleBackgroundImageElement.class);
this.appendChild(styleBackgroundImage);
return styleBackgroundImage;
}
@Override
public void accept(ElementVisitor visitor) {
if (visitor instanceof DefaultElementVisitor) {
DefaultElementVisitor defaultVisitor = (DefaultElementVisitor) visitor;
defaultVisitor.visit(this);
} else {
visitor.visit(this);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy