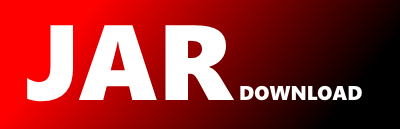
org.odftoolkit.odfdom.dom.element.text.TextBibliographyConfigurationElement Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of odfdom-java Show documentation
Show all versions of odfdom-java Show documentation
ODFDOM is an OpenDocument Format (ODF) framework. Its purpose
is to provide an easy common way to create, access and
manipulate ODF files, without requiring detailed knowledge of
the ODF specification. It is designed to provide the ODF
developer community with an easy lightwork programming API
portable to any object-oriented language.
The current reference implementation is written in Java.
/************************************************************************
*
* DO NOT ALTER OR REMOVE COPYRIGHT NOTICES OR THIS FILE HEADER
*
* Copyright 2008, 2010 Oracle and/or its affiliates. All rights reserved.
*
* Use is subject to license terms.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy
* of the License at http://www.apache.org/licenses/LICENSE-2.0. You can also
* obtain a copy of the License at http://odftoolkit.org/docs/license.txt
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
*
* See the License for the specific language governing permissions and
* limitations under the License.
*
************************************************************************/
/*
* This file is automatically generated.
* Don't edit manually.
*/
package org.odftoolkit.odfdom.dom.element.text;
import org.odftoolkit.odfdom.pkg.OdfElement;
import org.odftoolkit.odfdom.pkg.ElementVisitor;
import org.odftoolkit.odfdom.pkg.OdfFileDom;
import org.odftoolkit.odfdom.pkg.OdfName;
import org.odftoolkit.odfdom.dom.OdfDocumentNamespace;
import org.odftoolkit.odfdom.dom.DefaultElementVisitor;
import org.odftoolkit.odfdom.dom.attribute.fo.FoCountryAttribute;
import org.odftoolkit.odfdom.dom.attribute.fo.FoLanguageAttribute;
import org.odftoolkit.odfdom.dom.attribute.fo.FoScriptAttribute;
import org.odftoolkit.odfdom.dom.attribute.style.StyleRfcLanguageTagAttribute;
import org.odftoolkit.odfdom.dom.attribute.text.TextNumberedEntriesAttribute;
import org.odftoolkit.odfdom.dom.attribute.text.TextPrefixAttribute;
import org.odftoolkit.odfdom.dom.attribute.text.TextSortAlgorithmAttribute;
import org.odftoolkit.odfdom.dom.attribute.text.TextSortByPositionAttribute;
import org.odftoolkit.odfdom.dom.attribute.text.TextSuffixAttribute;
/**
* DOM implementation of OpenDocument element {@odf.element text:bibliography-configuration}.
*
*/
public class TextBibliographyConfigurationElement extends OdfElement {
public static final OdfName ELEMENT_NAME = OdfName.newName(OdfDocumentNamespace.TEXT, "bibliography-configuration");
/**
* Create the instance of TextBibliographyConfigurationElement
*
* @param ownerDoc The type is OdfFileDom
*/
public TextBibliographyConfigurationElement(OdfFileDom ownerDoc) {
super(ownerDoc, ELEMENT_NAME);
}
/**
* Get the element name
*
* @return return OdfName
the name of element {@odf.element text:bibliography-configuration}.
*/
public OdfName getOdfName() {
return ELEMENT_NAME;
}
/**
* Receives the value of the ODFDOM attribute representation FoCountryAttribute
, See {@odf.attribute fo:country}
*
* @return - the String
, the value or null
, if the attribute is not set and no default value defined.
*/
public String getFoCountryAttribute() {
FoCountryAttribute attr = (FoCountryAttribute) getOdfAttribute(OdfDocumentNamespace.FO, "country");
if (attr != null) {
return String.valueOf(attr.getValue());
}
return null;
}
/**
* Sets the value of ODFDOM attribute representation FoCountryAttribute
, See {@odf.attribute fo:country}
*
* @param foCountryValue The type is String
*/
public void setFoCountryAttribute(String foCountryValue) {
FoCountryAttribute attr = new FoCountryAttribute((OdfFileDom) this.ownerDocument);
setOdfAttribute(attr);
attr.setValue(foCountryValue);
}
/**
* Receives the value of the ODFDOM attribute representation FoLanguageAttribute
, See {@odf.attribute fo:language}
*
* @return - the String
, the value or null
, if the attribute is not set and no default value defined.
*/
public String getFoLanguageAttribute() {
FoLanguageAttribute attr = (FoLanguageAttribute) getOdfAttribute(OdfDocumentNamespace.FO, "language");
if (attr != null) {
return String.valueOf(attr.getValue());
}
return null;
}
/**
* Sets the value of ODFDOM attribute representation FoLanguageAttribute
, See {@odf.attribute fo:language}
*
* @param foLanguageValue The type is String
*/
public void setFoLanguageAttribute(String foLanguageValue) {
FoLanguageAttribute attr = new FoLanguageAttribute((OdfFileDom) this.ownerDocument);
setOdfAttribute(attr);
attr.setValue(foLanguageValue);
}
/**
* Receives the value of the ODFDOM attribute representation FoScriptAttribute
, See {@odf.attribute fo:script}
*
* @return - the String
, the value or null
, if the attribute is not set and no default value defined.
*/
public String getFoScriptAttribute() {
FoScriptAttribute attr = (FoScriptAttribute) getOdfAttribute(OdfDocumentNamespace.FO, "script");
if (attr != null) {
return String.valueOf(attr.getValue());
}
return null;
}
/**
* Sets the value of ODFDOM attribute representation FoScriptAttribute
, See {@odf.attribute fo:script}
*
* @param foScriptValue The type is String
*/
public void setFoScriptAttribute(String foScriptValue) {
FoScriptAttribute attr = new FoScriptAttribute((OdfFileDom) this.ownerDocument);
setOdfAttribute(attr);
attr.setValue(foScriptValue);
}
/**
* Receives the value of the ODFDOM attribute representation StyleRfcLanguageTagAttribute
, See {@odf.attribute style:rfc-language-tag}
*
* @return - the String
, the value or null
, if the attribute is not set and no default value defined.
*/
public String getStyleRfcLanguageTagAttribute() {
StyleRfcLanguageTagAttribute attr = (StyleRfcLanguageTagAttribute) getOdfAttribute(OdfDocumentNamespace.STYLE, "rfc-language-tag");
if (attr != null) {
return String.valueOf(attr.getValue());
}
return null;
}
/**
* Sets the value of ODFDOM attribute representation StyleRfcLanguageTagAttribute
, See {@odf.attribute style:rfc-language-tag}
*
* @param styleRfcLanguageTagValue The type is String
*/
public void setStyleRfcLanguageTagAttribute(String styleRfcLanguageTagValue) {
StyleRfcLanguageTagAttribute attr = new StyleRfcLanguageTagAttribute((OdfFileDom) this.ownerDocument);
setOdfAttribute(attr);
attr.setValue(styleRfcLanguageTagValue);
}
/**
* Receives the value of the ODFDOM attribute representation TextNumberedEntriesAttribute
, See {@odf.attribute text:numbered-entries}
*
* @return - the Boolean
, the value or null
, if the attribute is not set and no default value defined.
*/
public Boolean getTextNumberedEntriesAttribute() {
TextNumberedEntriesAttribute attr = (TextNumberedEntriesAttribute) getOdfAttribute(OdfDocumentNamespace.TEXT, "numbered-entries");
if (attr != null) {
return Boolean.valueOf(attr.booleanValue());
}
return Boolean.valueOf(TextNumberedEntriesAttribute.DEFAULT_VALUE);
}
/**
* Sets the value of ODFDOM attribute representation TextNumberedEntriesAttribute
, See {@odf.attribute text:numbered-entries}
*
* @param textNumberedEntriesValue The type is Boolean
*/
public void setTextNumberedEntriesAttribute(Boolean textNumberedEntriesValue) {
TextNumberedEntriesAttribute attr = new TextNumberedEntriesAttribute((OdfFileDom) this.ownerDocument);
setOdfAttribute(attr);
attr.setBooleanValue(textNumberedEntriesValue.booleanValue());
}
/**
* Receives the value of the ODFDOM attribute representation TextPrefixAttribute
, See {@odf.attribute text:prefix}
*
* @return - the String
, the value or null
, if the attribute is not set and no default value defined.
*/
public String getTextPrefixAttribute() {
TextPrefixAttribute attr = (TextPrefixAttribute) getOdfAttribute(OdfDocumentNamespace.TEXT, "prefix");
if (attr != null) {
return String.valueOf(attr.getValue());
}
return null;
}
/**
* Sets the value of ODFDOM attribute representation TextPrefixAttribute
, See {@odf.attribute text:prefix}
*
* @param textPrefixValue The type is String
*/
public void setTextPrefixAttribute(String textPrefixValue) {
TextPrefixAttribute attr = new TextPrefixAttribute((OdfFileDom) this.ownerDocument);
setOdfAttribute(attr);
attr.setValue(textPrefixValue);
}
/**
* Receives the value of the ODFDOM attribute representation TextSortAlgorithmAttribute
, See {@odf.attribute text:sort-algorithm}
*
* @return - the String
, the value or null
, if the attribute is not set and no default value defined.
*/
public String getTextSortAlgorithmAttribute() {
TextSortAlgorithmAttribute attr = (TextSortAlgorithmAttribute) getOdfAttribute(OdfDocumentNamespace.TEXT, "sort-algorithm");
if (attr != null) {
return String.valueOf(attr.getValue());
}
return null;
}
/**
* Sets the value of ODFDOM attribute representation TextSortAlgorithmAttribute
, See {@odf.attribute text:sort-algorithm}
*
* @param textSortAlgorithmValue The type is String
*/
public void setTextSortAlgorithmAttribute(String textSortAlgorithmValue) {
TextSortAlgorithmAttribute attr = new TextSortAlgorithmAttribute((OdfFileDom) this.ownerDocument);
setOdfAttribute(attr);
attr.setValue(textSortAlgorithmValue);
}
/**
* Receives the value of the ODFDOM attribute representation TextSortByPositionAttribute
, See {@odf.attribute text:sort-by-position}
*
* @return - the Boolean
, the value or null
, if the attribute is not set and no default value defined.
*/
public Boolean getTextSortByPositionAttribute() {
TextSortByPositionAttribute attr = (TextSortByPositionAttribute) getOdfAttribute(OdfDocumentNamespace.TEXT, "sort-by-position");
if (attr != null) {
return Boolean.valueOf(attr.booleanValue());
}
return Boolean.valueOf(TextSortByPositionAttribute.DEFAULT_VALUE);
}
/**
* Sets the value of ODFDOM attribute representation TextSortByPositionAttribute
, See {@odf.attribute text:sort-by-position}
*
* @param textSortByPositionValue The type is Boolean
*/
public void setTextSortByPositionAttribute(Boolean textSortByPositionValue) {
TextSortByPositionAttribute attr = new TextSortByPositionAttribute((OdfFileDom) this.ownerDocument);
setOdfAttribute(attr);
attr.setBooleanValue(textSortByPositionValue.booleanValue());
}
/**
* Receives the value of the ODFDOM attribute representation TextSuffixAttribute
, See {@odf.attribute text:suffix}
*
* @return - the String
, the value or null
, if the attribute is not set and no default value defined.
*/
public String getTextSuffixAttribute() {
TextSuffixAttribute attr = (TextSuffixAttribute) getOdfAttribute(OdfDocumentNamespace.TEXT, "suffix");
if (attr != null) {
return String.valueOf(attr.getValue());
}
return null;
}
/**
* Sets the value of ODFDOM attribute representation TextSuffixAttribute
, See {@odf.attribute text:suffix}
*
* @param textSuffixValue The type is String
*/
public void setTextSuffixAttribute(String textSuffixValue) {
TextSuffixAttribute attr = new TextSuffixAttribute((OdfFileDom) this.ownerDocument);
setOdfAttribute(attr);
attr.setValue(textSuffixValue);
}
/**
* Create child element {@odf.element text:sort-key}.
*
* @param textKeyValue the String
value of TextKeyAttribute
, see {@odf.attribute text:key} at specification
* @return the element {@odf.element text:sort-key}
*/
public TextSortKeyElement newTextSortKeyElement(String textKeyValue) {
TextSortKeyElement textSortKey = ((OdfFileDom) this.ownerDocument).newOdfElement(TextSortKeyElement.class);
textSortKey.setTextKeyAttribute(textKeyValue);
this.appendChild(textSortKey);
return textSortKey;
}
@Override
public void accept(ElementVisitor visitor) {
if (visitor instanceof DefaultElementVisitor) {
DefaultElementVisitor defaultVisitor = (DefaultElementVisitor) visitor;
defaultVisitor.visit(this);
} else {
visitor.visit(this);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy