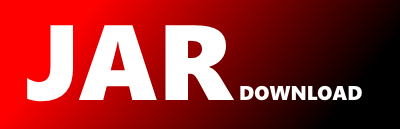
org.odftoolkit.odfdom.dom.style.OdfStyleFamily Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of odfdom-java Show documentation
Show all versions of odfdom-java Show documentation
ODFDOM is an OpenDocument Format (ODF) framework. Its purpose
is to provide an easy common way to create, access and
manipulate ODF files, without requiring detailed knowledge of
the ODF specification. It is designed to provide the ODF
developer community with an easy lightwork programming API
portable to any object-oriented language.
The current reference implementation is written in Java.
/************************************************************************
*
* DO NOT ALTER OR REMOVE COPYRIGHT NOTICES OR THIS FILE HEADER
*
* Copyright 2008, 2010 Oracle and/or its affiliates. All rights reserved.
*
* Use is subject to license terms.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy
* of the License at http://www.apache.org/licenses/LICENSE-2.0. You can also
* obtain a copy of the License at http://odftoolkit.org/docs/license.txt
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
*
* See the License for the specific language governing permissions and
* limitations under the License.
*
************************************************************************/
/*
* This file is automatically generated.
* Don't edit manually.
*/
package org.odftoolkit.odfdom.dom.style;
import java.util.Arrays;
import java.util.Collections;
import org.odftoolkit.odfdom.dom.style.props.OdfStyleProperty;
import java.util.Set;
import java.util.TreeSet;
import java.util.Map;
import java.util.HashMap;
import org.odftoolkit.odfdom.dom.element.style.StyleChartPropertiesElement;
import org.odftoolkit.odfdom.dom.element.style.StyleDrawingPagePropertiesElement;
import org.odftoolkit.odfdom.dom.element.style.StyleGraphicPropertiesElement;
import org.odftoolkit.odfdom.dom.element.style.StyleHeaderFooterPropertiesElement;
import org.odftoolkit.odfdom.dom.element.style.StyleListLevelPropertiesElement;
import org.odftoolkit.odfdom.dom.element.style.StylePageLayoutPropertiesElement;
import org.odftoolkit.odfdom.dom.element.style.StyleParagraphPropertiesElement;
import org.odftoolkit.odfdom.dom.element.style.StyleRubyPropertiesElement;
import org.odftoolkit.odfdom.dom.element.style.StyleSectionPropertiesElement;
import org.odftoolkit.odfdom.dom.element.style.StyleTableCellPropertiesElement;
import org.odftoolkit.odfdom.dom.element.style.StyleTableColumnPropertiesElement;
import org.odftoolkit.odfdom.dom.element.style.StyleTablePropertiesElement;
import org.odftoolkit.odfdom.dom.element.style.StyleTableRowPropertiesElement;
import org.odftoolkit.odfdom.dom.element.style.StyleTextPropertiesElement;
public class OdfStyleFamily implements Comparable {
private String m_name;
private Set m_properties = new TreeSet();
private static Map m_familyByName = new HashMap();
public static OdfStyleFamily getByName(String name) {
return m_familyByName.get(name);
}
private OdfStyleFamily(String name, OdfStyleProperty[] props) {
m_name = name;
m_properties.addAll(Arrays.asList(props));
m_familyByName.put(name, this);
}
private OdfStyleFamily(String name) {
m_name = name;
m_familyByName.put(name, this);
}
public String getName() {
return m_name;
}
public int compareTo(OdfStyleFamily o) {
return m_name.compareTo(o.toString());
}
public static OdfStyleFamily valueOf(String name) {
OdfStyleFamily family = getByName(name);
if (family == null) {
family = new OdfStyleFamily(name);
}
return family;
}
public static String toString(OdfStyleFamily family) {
if (family != null) {
return family.toString();
}
else {
return new String();
}
}
@Override
public String toString() {
return m_name;
}
public Set getProperties() {
return Collections.unmodifiableSet(m_properties);
}
public final static OdfStyleFamily Chart = new OdfStyleFamily("chart",
new OdfStyleProperty[]{
StyleChartPropertiesElement.AngleOffset,
StyleChartPropertiesElement.AutoPosition,
StyleChartPropertiesElement.AutoSize,
StyleChartPropertiesElement.AxisLabelPosition,
StyleChartPropertiesElement.AxisPosition,
StyleChartPropertiesElement.ConnectBars,
StyleChartPropertiesElement.DataLabelNumber,
StyleChartPropertiesElement.DataLabelSymbol,
StyleChartPropertiesElement.DataLabelText,
StyleChartPropertiesElement.Deep,
StyleChartPropertiesElement.DisplayLabel,
StyleChartPropertiesElement.ErrorCategory,
StyleChartPropertiesElement.ErrorLowerIndicator,
StyleChartPropertiesElement.ErrorLowerLimit,
StyleChartPropertiesElement.ErrorLowerRange,
StyleChartPropertiesElement.ErrorMargin,
StyleChartPropertiesElement.ErrorPercentage,
StyleChartPropertiesElement.ErrorUpperIndicator,
StyleChartPropertiesElement.ErrorUpperLimit,
StyleChartPropertiesElement.ErrorUpperRange,
StyleChartPropertiesElement.GapWidth,
StyleChartPropertiesElement.GroupBarsPerAxis,
StyleChartPropertiesElement.HoleSize,
StyleChartPropertiesElement.IncludeHiddenCells,
StyleChartPropertiesElement.Interpolation,
StyleChartPropertiesElement.IntervalMajor,
StyleChartPropertiesElement.IntervalMinorDivisor,
StyleChartPropertiesElement.JapaneseCandleStick,
StyleChartPropertiesElement.LabelArrangement,
StyleChartPropertiesElement.LabelPosition,
StyleChartPropertiesElement.LabelPositionNegative,
StyleChartPropertiesElement.Lines,
StyleChartPropertiesElement.LinkDataStyleToSource,
StyleChartPropertiesElement.Logarithmic,
StyleChartPropertiesElement.Maximum,
StyleChartPropertiesElement.MeanValue,
StyleChartPropertiesElement.Minimum,
StyleChartPropertiesElement.Origin,
StyleChartPropertiesElement.Overlap,
StyleChartPropertiesElement.Percentage,
StyleChartPropertiesElement.PieOffset,
StyleChartPropertiesElement.RegressionType,
StyleChartPropertiesElement.ReverseDirection,
StyleChartPropertiesElement.RightAngledAxes,
StyleChartPropertiesElement.ScaleText,
StyleChartPropertiesElement.SeriesSource,
StyleChartPropertiesElement.SolidType,
StyleChartPropertiesElement.SortByXValues,
StyleChartPropertiesElement.SplineOrder,
StyleChartPropertiesElement.SplineResolution,
StyleChartPropertiesElement.Stacked,
StyleChartPropertiesElement.SymbolHeight,
StyleChartPropertiesElement.SymbolName,
StyleChartPropertiesElement.SymbolType,
StyleChartPropertiesElement.SymbolType,
StyleChartPropertiesElement.SymbolType,
StyleChartPropertiesElement.SymbolType,
StyleChartPropertiesElement.SymbolWidth,
StyleChartPropertiesElement.TextOverlap,
StyleChartPropertiesElement.ThreeDimensional,
StyleChartPropertiesElement.TickMarkPosition,
StyleChartPropertiesElement.TickMarksMajorInner,
StyleChartPropertiesElement.TickMarksMajorOuter,
StyleChartPropertiesElement.TickMarksMinorInner,
StyleChartPropertiesElement.TickMarksMinorOuter,
StyleChartPropertiesElement.TreatEmptyCells,
StyleChartPropertiesElement.Vertical,
StyleChartPropertiesElement.Visible,
StyleChartPropertiesElement.Direction,
StyleChartPropertiesElement.RotationAngle,
StyleChartPropertiesElement.LineBreak,
StyleDrawingPagePropertiesElement.BackgroundSize,
StyleDrawingPagePropertiesElement.Fill,
StyleDrawingPagePropertiesElement.FillColor,
StyleDrawingPagePropertiesElement.FillGradientName,
StyleDrawingPagePropertiesElement.FillHatchName,
StyleDrawingPagePropertiesElement.FillHatchSolid,
StyleDrawingPagePropertiesElement.FillImageHeight,
StyleDrawingPagePropertiesElement.FillImageName,
StyleDrawingPagePropertiesElement.FillImageRefPoint,
StyleDrawingPagePropertiesElement.FillImageRefPointX,
StyleDrawingPagePropertiesElement.FillImageRefPointY,
StyleDrawingPagePropertiesElement.FillImageWidth,
StyleDrawingPagePropertiesElement.GradientStepCount,
StyleDrawingPagePropertiesElement.Opacity,
StyleDrawingPagePropertiesElement.OpacityName,
StyleDrawingPagePropertiesElement.SecondaryFillColor,
StyleDrawingPagePropertiesElement.TileRepeatOffset,
StyleDrawingPagePropertiesElement.BackgroundObjectsVisible,
StyleDrawingPagePropertiesElement.BackgroundVisible,
StyleDrawingPagePropertiesElement.DisplayDateTime,
StyleDrawingPagePropertiesElement.DisplayFooter,
StyleDrawingPagePropertiesElement.DisplayHeader,
StyleDrawingPagePropertiesElement.DisplayPageNumber,
StyleDrawingPagePropertiesElement.Duration,
StyleDrawingPagePropertiesElement.TransitionSpeed,
StyleDrawingPagePropertiesElement.TransitionStyle,
StyleDrawingPagePropertiesElement.TransitionType,
StyleDrawingPagePropertiesElement.Visibility,
StyleDrawingPagePropertiesElement.Direction,
StyleDrawingPagePropertiesElement.FadeColor,
StyleDrawingPagePropertiesElement.Subtype,
StyleDrawingPagePropertiesElement.Type,
StyleDrawingPagePropertiesElement.Repeat,
StyleDrawingPagePropertiesElement.FillRule,
StyleGraphicPropertiesElement.AmbientColor,
StyleGraphicPropertiesElement.BackScale,
StyleGraphicPropertiesElement.BackfaceCulling,
StyleGraphicPropertiesElement.CloseBack,
StyleGraphicPropertiesElement.CloseFront,
StyleGraphicPropertiesElement.Depth,
StyleGraphicPropertiesElement.DiffuseColor,
StyleGraphicPropertiesElement.EdgeRounding,
StyleGraphicPropertiesElement.EdgeRoundingMode,
StyleGraphicPropertiesElement.EmissiveColor,
StyleGraphicPropertiesElement.EndAngle,
StyleGraphicPropertiesElement.HorizontalSegments,
StyleGraphicPropertiesElement.LightingMode,
StyleGraphicPropertiesElement.NormalsDirection,
StyleGraphicPropertiesElement.NormalsKind,
StyleGraphicPropertiesElement.Dr3dShadow,
StyleGraphicPropertiesElement.Shininess,
StyleGraphicPropertiesElement.SpecularColor,
StyleGraphicPropertiesElement.TextureFilter,
StyleGraphicPropertiesElement.TextureGenerationModeX,
StyleGraphicPropertiesElement.TextureGenerationModeY,
StyleGraphicPropertiesElement.TextureKind,
StyleGraphicPropertiesElement.TextureMode,
StyleGraphicPropertiesElement.VerticalSegments,
StyleGraphicPropertiesElement.AutoGrowHeight,
StyleGraphicPropertiesElement.AutoGrowWidth,
StyleGraphicPropertiesElement.Blue,
StyleGraphicPropertiesElement.CaptionAngle,
StyleGraphicPropertiesElement.CaptionAngleType,
StyleGraphicPropertiesElement.CaptionEscape,
StyleGraphicPropertiesElement.CaptionEscapeDirection,
StyleGraphicPropertiesElement.CaptionFitLineLength,
StyleGraphicPropertiesElement.CaptionGap,
StyleGraphicPropertiesElement.CaptionLineLength,
StyleGraphicPropertiesElement.CaptionType,
StyleGraphicPropertiesElement.ColorInversion,
StyleGraphicPropertiesElement.ColorMode,
StyleGraphicPropertiesElement.Contrast,
StyleGraphicPropertiesElement.DecimalPlaces,
StyleGraphicPropertiesElement.DrawAspect,
StyleGraphicPropertiesElement.EndGuide,
StyleGraphicPropertiesElement.EndLineSpacingHorizontal,
StyleGraphicPropertiesElement.EndLineSpacingVertical,
StyleGraphicPropertiesElement.Fill,
StyleGraphicPropertiesElement.FillColor,
StyleGraphicPropertiesElement.FillGradientName,
StyleGraphicPropertiesElement.FillHatchName,
StyleGraphicPropertiesElement.FillHatchSolid,
StyleGraphicPropertiesElement.FillImageHeight,
StyleGraphicPropertiesElement.FillImageName,
StyleGraphicPropertiesElement.FillImageRefPoint,
StyleGraphicPropertiesElement.FillImageRefPointX,
StyleGraphicPropertiesElement.FillImageRefPointY,
StyleGraphicPropertiesElement.FillImageWidth,
StyleGraphicPropertiesElement.FitToContour,
StyleGraphicPropertiesElement.FitToSize,
StyleGraphicPropertiesElement.FrameDisplayBorder,
StyleGraphicPropertiesElement.FrameDisplayScrollbar,
StyleGraphicPropertiesElement.FrameMarginHorizontal,
StyleGraphicPropertiesElement.FrameMarginVertical,
StyleGraphicPropertiesElement.Gamma,
StyleGraphicPropertiesElement.GradientStepCount,
StyleGraphicPropertiesElement.Green,
StyleGraphicPropertiesElement.GuideDistance,
StyleGraphicPropertiesElement.GuideOverhang,
StyleGraphicPropertiesElement.ImageOpacity,
StyleGraphicPropertiesElement.LineDistance,
StyleGraphicPropertiesElement.Luminance,
StyleGraphicPropertiesElement.MarkerEnd,
StyleGraphicPropertiesElement.MarkerEndCenter,
StyleGraphicPropertiesElement.MarkerEndWidth,
StyleGraphicPropertiesElement.MarkerStart,
StyleGraphicPropertiesElement.MarkerStartCenter,
StyleGraphicPropertiesElement.MarkerStartWidth,
StyleGraphicPropertiesElement.MeasureAlign,
StyleGraphicPropertiesElement.MeasureVerticalAlign,
StyleGraphicPropertiesElement.OleDrawAspect,
StyleGraphicPropertiesElement.Opacity,
StyleGraphicPropertiesElement.OpacityName,
StyleGraphicPropertiesElement.Parallel,
StyleGraphicPropertiesElement.Placing,
StyleGraphicPropertiesElement.Red,
StyleGraphicPropertiesElement.SecondaryFillColor,
StyleGraphicPropertiesElement.DrawShadow,
StyleGraphicPropertiesElement.ShadowColor,
StyleGraphicPropertiesElement.ShadowOffsetX,
StyleGraphicPropertiesElement.ShadowOffsetY,
StyleGraphicPropertiesElement.ShadowOpacity,
StyleGraphicPropertiesElement.ShowUnit,
StyleGraphicPropertiesElement.StartGuide,
StyleGraphicPropertiesElement.StartLineSpacingHorizontal,
StyleGraphicPropertiesElement.StartLineSpacingVertical,
StyleGraphicPropertiesElement.Stroke,
StyleGraphicPropertiesElement.StrokeDash,
StyleGraphicPropertiesElement.StrokeDashNames,
StyleGraphicPropertiesElement.StrokeLinejoin,
StyleGraphicPropertiesElement.SymbolColor,
StyleGraphicPropertiesElement.TextareaHorizontalAlign,
StyleGraphicPropertiesElement.TextareaVerticalAlign,
StyleGraphicPropertiesElement.TileRepeatOffset,
StyleGraphicPropertiesElement.Unit,
StyleGraphicPropertiesElement.VisibleAreaHeight,
StyleGraphicPropertiesElement.VisibleAreaLeft,
StyleGraphicPropertiesElement.VisibleAreaTop,
StyleGraphicPropertiesElement.VisibleAreaWidth,
StyleGraphicPropertiesElement.WrapInfluenceOnPosition,
StyleGraphicPropertiesElement.BackgroundColor,
StyleGraphicPropertiesElement.Border,
StyleGraphicPropertiesElement.BorderBottom,
StyleGraphicPropertiesElement.BorderLeft,
StyleGraphicPropertiesElement.BorderRight,
StyleGraphicPropertiesElement.BorderTop,
StyleGraphicPropertiesElement.Clip,
StyleGraphicPropertiesElement.Margin,
StyleGraphicPropertiesElement.MarginBottom,
StyleGraphicPropertiesElement.MarginLeft,
StyleGraphicPropertiesElement.MarginRight,
StyleGraphicPropertiesElement.MarginTop,
StyleGraphicPropertiesElement.MaxHeight,
StyleGraphicPropertiesElement.MaxWidth,
StyleGraphicPropertiesElement.MinHeight,
StyleGraphicPropertiesElement.MinWidth,
StyleGraphicPropertiesElement.Padding,
StyleGraphicPropertiesElement.PaddingBottom,
StyleGraphicPropertiesElement.PaddingLeft,
StyleGraphicPropertiesElement.PaddingRight,
StyleGraphicPropertiesElement.PaddingTop,
StyleGraphicPropertiesElement.WrapOption,
StyleGraphicPropertiesElement.BackgroundTransparency,
StyleGraphicPropertiesElement.BorderLineWidth,
StyleGraphicPropertiesElement.BorderLineWidthBottom,
StyleGraphicPropertiesElement.BorderLineWidthLeft,
StyleGraphicPropertiesElement.BorderLineWidthRight,
StyleGraphicPropertiesElement.BorderLineWidthTop,
StyleGraphicPropertiesElement.Editable,
StyleGraphicPropertiesElement.FlowWithText,
StyleGraphicPropertiesElement.HorizontalPos,
StyleGraphicPropertiesElement.HorizontalRel,
StyleGraphicPropertiesElement.Mirror,
StyleGraphicPropertiesElement.NumberWrappedParagraphs,
StyleGraphicPropertiesElement.OverflowBehavior,
StyleGraphicPropertiesElement.PrintContent,
StyleGraphicPropertiesElement.Protect,
StyleGraphicPropertiesElement.RelHeight,
StyleGraphicPropertiesElement.RelWidth,
StyleGraphicPropertiesElement.Repeat,
StyleGraphicPropertiesElement.RunThrough,
StyleGraphicPropertiesElement.StyleShadow,
StyleGraphicPropertiesElement.ShrinkToFit,
StyleGraphicPropertiesElement.VerticalPos,
StyleGraphicPropertiesElement.VerticalRel,
StyleGraphicPropertiesElement.Wrap,
StyleGraphicPropertiesElement.WrapContour,
StyleGraphicPropertiesElement.WrapContourMode,
StyleGraphicPropertiesElement.WrapDynamicThreshold,
StyleGraphicPropertiesElement.WritingMode,
StyleGraphicPropertiesElement.FillRule,
StyleGraphicPropertiesElement.Height,
StyleGraphicPropertiesElement.StrokeColor,
StyleGraphicPropertiesElement.StrokeLinecap,
StyleGraphicPropertiesElement.StrokeOpacity,
StyleGraphicPropertiesElement.StrokeWidth,
StyleGraphicPropertiesElement.Width,
StyleGraphicPropertiesElement.X,
StyleGraphicPropertiesElement.Y,
StyleGraphicPropertiesElement.AnchorPageNumber,
StyleGraphicPropertiesElement.AnchorType,
StyleGraphicPropertiesElement.Animation,
StyleGraphicPropertiesElement.AnimationDelay,
StyleGraphicPropertiesElement.AnimationDirection,
StyleGraphicPropertiesElement.AnimationRepeat,
StyleGraphicPropertiesElement.AnimationStartInside,
StyleGraphicPropertiesElement.AnimationSteps,
StyleGraphicPropertiesElement.AnimationStopInside,
StyleHeaderFooterPropertiesElement.BackgroundColor,
StyleHeaderFooterPropertiesElement.Border,
StyleHeaderFooterPropertiesElement.BorderBottom,
StyleHeaderFooterPropertiesElement.BorderLeft,
StyleHeaderFooterPropertiesElement.BorderRight,
StyleHeaderFooterPropertiesElement.BorderTop,
StyleHeaderFooterPropertiesElement.Margin,
StyleHeaderFooterPropertiesElement.MarginBottom,
StyleHeaderFooterPropertiesElement.MarginLeft,
StyleHeaderFooterPropertiesElement.MarginRight,
StyleHeaderFooterPropertiesElement.MarginTop,
StyleHeaderFooterPropertiesElement.MinHeight,
StyleHeaderFooterPropertiesElement.Padding,
StyleHeaderFooterPropertiesElement.PaddingBottom,
StyleHeaderFooterPropertiesElement.PaddingLeft,
StyleHeaderFooterPropertiesElement.PaddingRight,
StyleHeaderFooterPropertiesElement.PaddingTop,
StyleHeaderFooterPropertiesElement.BorderLineWidth,
StyleHeaderFooterPropertiesElement.BorderLineWidthBottom,
StyleHeaderFooterPropertiesElement.BorderLineWidthLeft,
StyleHeaderFooterPropertiesElement.BorderLineWidthRight,
StyleHeaderFooterPropertiesElement.BorderLineWidthTop,
StyleHeaderFooterPropertiesElement.DynamicSpacing,
StyleHeaderFooterPropertiesElement.Shadow,
StyleHeaderFooterPropertiesElement.Height,
StyleListLevelPropertiesElement.Height,
StyleListLevelPropertiesElement.TextAlign,
StyleListLevelPropertiesElement.Width,
StyleListLevelPropertiesElement.FontName,
StyleListLevelPropertiesElement.VerticalPos,
StyleListLevelPropertiesElement.VerticalRel,
StyleListLevelPropertiesElement.Y,
StyleListLevelPropertiesElement.ListLevelPositionAndSpaceMode,
StyleListLevelPropertiesElement.MinLabelDistance,
StyleListLevelPropertiesElement.MinLabelWidth,
StyleListLevelPropertiesElement.SpaceBefore,
StylePageLayoutPropertiesElement.BackgroundColor,
StylePageLayoutPropertiesElement.Border,
StylePageLayoutPropertiesElement.BorderBottom,
StylePageLayoutPropertiesElement.BorderLeft,
StylePageLayoutPropertiesElement.BorderRight,
StylePageLayoutPropertiesElement.BorderTop,
StylePageLayoutPropertiesElement.Margin,
StylePageLayoutPropertiesElement.MarginBottom,
StylePageLayoutPropertiesElement.MarginLeft,
StylePageLayoutPropertiesElement.MarginRight,
StylePageLayoutPropertiesElement.MarginTop,
StylePageLayoutPropertiesElement.Padding,
StylePageLayoutPropertiesElement.PaddingBottom,
StylePageLayoutPropertiesElement.PaddingLeft,
StylePageLayoutPropertiesElement.PaddingRight,
StylePageLayoutPropertiesElement.PaddingTop,
StylePageLayoutPropertiesElement.PageHeight,
StylePageLayoutPropertiesElement.PageWidth,
StylePageLayoutPropertiesElement.BorderLineWidth,
StylePageLayoutPropertiesElement.BorderLineWidthBottom,
StylePageLayoutPropertiesElement.BorderLineWidthLeft,
StylePageLayoutPropertiesElement.BorderLineWidthRight,
StylePageLayoutPropertiesElement.BorderLineWidthTop,
StylePageLayoutPropertiesElement.FirstPageNumber,
StylePageLayoutPropertiesElement.FootnoteMaxHeight,
StylePageLayoutPropertiesElement.LayoutGridBaseHeight,
StylePageLayoutPropertiesElement.LayoutGridBaseWidth,
StylePageLayoutPropertiesElement.LayoutGridColor,
StylePageLayoutPropertiesElement.LayoutGridDisplay,
StylePageLayoutPropertiesElement.LayoutGridLines,
StylePageLayoutPropertiesElement.LayoutGridMode,
StylePageLayoutPropertiesElement.LayoutGridPrint,
StylePageLayoutPropertiesElement.LayoutGridRubyBelow,
StylePageLayoutPropertiesElement.LayoutGridRubyHeight,
StylePageLayoutPropertiesElement.LayoutGridSnapTo,
StylePageLayoutPropertiesElement.LayoutGridStandardMode,
StylePageLayoutPropertiesElement.NumFormat,
StylePageLayoutPropertiesElement.NumFormat,
StylePageLayoutPropertiesElement.NumLetterSync,
StylePageLayoutPropertiesElement.NumPrefix,
StylePageLayoutPropertiesElement.NumSuffix,
StylePageLayoutPropertiesElement.PaperTrayName,
StylePageLayoutPropertiesElement.Print,
StylePageLayoutPropertiesElement.PrintOrientation,
StylePageLayoutPropertiesElement.PrintPageOrder,
StylePageLayoutPropertiesElement.RegisterTruthRefStyleName,
StylePageLayoutPropertiesElement.ScaleTo,
StylePageLayoutPropertiesElement.ScaleToPages,
StylePageLayoutPropertiesElement.Shadow,
StylePageLayoutPropertiesElement.TableCentering,
StylePageLayoutPropertiesElement.WritingMode,
StyleParagraphPropertiesElement.BackgroundColor,
StyleParagraphPropertiesElement.Border,
StyleParagraphPropertiesElement.BorderBottom,
StyleParagraphPropertiesElement.BorderLeft,
StyleParagraphPropertiesElement.BorderRight,
StyleParagraphPropertiesElement.BorderTop,
StyleParagraphPropertiesElement.BreakAfter,
StyleParagraphPropertiesElement.BreakBefore,
StyleParagraphPropertiesElement.HyphenationKeep,
StyleParagraphPropertiesElement.HyphenationLadderCount,
StyleParagraphPropertiesElement.KeepTogether,
StyleParagraphPropertiesElement.KeepWithNext,
StyleParagraphPropertiesElement.LineHeight,
StyleParagraphPropertiesElement.Margin,
StyleParagraphPropertiesElement.MarginBottom,
StyleParagraphPropertiesElement.MarginLeft,
StyleParagraphPropertiesElement.MarginRight,
StyleParagraphPropertiesElement.MarginTop,
StyleParagraphPropertiesElement.Orphans,
StyleParagraphPropertiesElement.Padding,
StyleParagraphPropertiesElement.PaddingBottom,
StyleParagraphPropertiesElement.PaddingLeft,
StyleParagraphPropertiesElement.PaddingRight,
StyleParagraphPropertiesElement.PaddingTop,
StyleParagraphPropertiesElement.TextAlign,
StyleParagraphPropertiesElement.TextAlignLast,
StyleParagraphPropertiesElement.TextIndent,
StyleParagraphPropertiesElement.Widows,
StyleParagraphPropertiesElement.AutoTextIndent,
StyleParagraphPropertiesElement.BackgroundTransparency,
StyleParagraphPropertiesElement.BorderLineWidth,
StyleParagraphPropertiesElement.BorderLineWidthBottom,
StyleParagraphPropertiesElement.BorderLineWidthLeft,
StyleParagraphPropertiesElement.BorderLineWidthRight,
StyleParagraphPropertiesElement.BorderLineWidthTop,
StyleParagraphPropertiesElement.FontIndependentLineSpacing,
StyleParagraphPropertiesElement.JoinBorder,
StyleParagraphPropertiesElement.JustifySingleWord,
StyleParagraphPropertiesElement.LineBreak,
StyleParagraphPropertiesElement.LineHeightAtLeast,
StyleParagraphPropertiesElement.LineSpacing,
StyleParagraphPropertiesElement.PageNumber,
StyleParagraphPropertiesElement.PunctuationWrap,
StyleParagraphPropertiesElement.RegisterTrue,
StyleParagraphPropertiesElement.Shadow,
StyleParagraphPropertiesElement.SnapToLayoutGrid,
StyleParagraphPropertiesElement.TabStopDistance,
StyleParagraphPropertiesElement.TextAutospace,
StyleParagraphPropertiesElement.VerticalAlign,
StyleParagraphPropertiesElement.WritingMode,
StyleParagraphPropertiesElement.WritingModeAutomatic,
StyleParagraphPropertiesElement.LineNumber,
StyleParagraphPropertiesElement.NumberLines,
StyleRubyPropertiesElement.RubyAlign,
StyleRubyPropertiesElement.RubyPosition,
StyleSectionPropertiesElement.BackgroundColor,
StyleSectionPropertiesElement.MarginLeft,
StyleSectionPropertiesElement.MarginRight,
StyleSectionPropertiesElement.Editable,
StyleSectionPropertiesElement.Protect,
StyleSectionPropertiesElement.WritingMode,
StyleSectionPropertiesElement.DontBalanceTextColumns,
StyleTableCellPropertiesElement.BackgroundColor,
StyleTableCellPropertiesElement.Border,
StyleTableCellPropertiesElement.BorderBottom,
StyleTableCellPropertiesElement.BorderLeft,
StyleTableCellPropertiesElement.BorderRight,
StyleTableCellPropertiesElement.BorderTop,
StyleTableCellPropertiesElement.Padding,
StyleTableCellPropertiesElement.PaddingBottom,
StyleTableCellPropertiesElement.PaddingLeft,
StyleTableCellPropertiesElement.PaddingRight,
StyleTableCellPropertiesElement.PaddingTop,
StyleTableCellPropertiesElement.WrapOption,
StyleTableCellPropertiesElement.BorderLineWidth,
StyleTableCellPropertiesElement.BorderLineWidthBottom,
StyleTableCellPropertiesElement.BorderLineWidthLeft,
StyleTableCellPropertiesElement.BorderLineWidthRight,
StyleTableCellPropertiesElement.BorderLineWidthTop,
StyleTableCellPropertiesElement.CellProtect,
StyleTableCellPropertiesElement.DecimalPlaces,
StyleTableCellPropertiesElement.DiagonalBlTr,
StyleTableCellPropertiesElement.DiagonalBlTrWidths,
StyleTableCellPropertiesElement.DiagonalTlBr,
StyleTableCellPropertiesElement.DiagonalTlBrWidths,
StyleTableCellPropertiesElement.Direction,
StyleTableCellPropertiesElement.GlyphOrientationVertical,
StyleTableCellPropertiesElement.PrintContent,
StyleTableCellPropertiesElement.RepeatContent,
StyleTableCellPropertiesElement.RotationAlign,
StyleTableCellPropertiesElement.RotationAngle,
StyleTableCellPropertiesElement.Shadow,
StyleTableCellPropertiesElement.ShrinkToFit,
StyleTableCellPropertiesElement.TextAlignSource,
StyleTableCellPropertiesElement.VerticalAlign,
StyleTableCellPropertiesElement.WritingMode,
StyleTableColumnPropertiesElement.BreakAfter,
StyleTableColumnPropertiesElement.BreakBefore,
StyleTableColumnPropertiesElement.ColumnWidth,
StyleTableColumnPropertiesElement.RelColumnWidth,
StyleTableColumnPropertiesElement.UseOptimalColumnWidth,
StyleTablePropertiesElement.BackgroundColor,
StyleTablePropertiesElement.BreakAfter,
StyleTablePropertiesElement.BreakBefore,
StyleTablePropertiesElement.KeepWithNext,
StyleTablePropertiesElement.Margin,
StyleTablePropertiesElement.MarginBottom,
StyleTablePropertiesElement.MarginLeft,
StyleTablePropertiesElement.MarginRight,
StyleTablePropertiesElement.MarginTop,
StyleTablePropertiesElement.MayBreakBetweenRows,
StyleTablePropertiesElement.PageNumber,
StyleTablePropertiesElement.RelWidth,
StyleTablePropertiesElement.Shadow,
StyleTablePropertiesElement.Width,
StyleTablePropertiesElement.WritingMode,
StyleTablePropertiesElement.Align,
StyleTablePropertiesElement.BorderModel,
StyleTablePropertiesElement.Display,
StyleTableRowPropertiesElement.BackgroundColor,
StyleTableRowPropertiesElement.BreakAfter,
StyleTableRowPropertiesElement.BreakBefore,
StyleTableRowPropertiesElement.KeepTogether,
StyleTableRowPropertiesElement.MinRowHeight,
StyleTableRowPropertiesElement.RowHeight,
StyleTableRowPropertiesElement.UseOptimalRowHeight,
StyleTextPropertiesElement.BackgroundColor,
StyleTextPropertiesElement.Color,
StyleTextPropertiesElement.Country,
StyleTextPropertiesElement.FontFamily,
StyleTextPropertiesElement.FontSize,
StyleTextPropertiesElement.FontStyle,
StyleTextPropertiesElement.FontVariant,
StyleTextPropertiesElement.FontWeight,
StyleTextPropertiesElement.Hyphenate,
StyleTextPropertiesElement.HyphenationPushCharCount,
StyleTextPropertiesElement.HyphenationRemainCharCount,
StyleTextPropertiesElement.Language,
StyleTextPropertiesElement.LetterSpacing,
StyleTextPropertiesElement.Script,
StyleTextPropertiesElement.TextShadow,
StyleTextPropertiesElement.TextTransform,
StyleTextPropertiesElement.CountryAsian,
StyleTextPropertiesElement.CountryComplex,
StyleTextPropertiesElement.FontCharset,
StyleTextPropertiesElement.FontCharsetAsian,
StyleTextPropertiesElement.FontCharsetComplex,
StyleTextPropertiesElement.FontFamilyAsian,
StyleTextPropertiesElement.FontFamilyComplex,
StyleTextPropertiesElement.FontFamilyGeneric,
StyleTextPropertiesElement.FontFamilyGenericAsian,
StyleTextPropertiesElement.FontFamilyGenericComplex,
StyleTextPropertiesElement.FontName,
StyleTextPropertiesElement.FontNameAsian,
StyleTextPropertiesElement.FontNameComplex,
StyleTextPropertiesElement.FontPitch,
StyleTextPropertiesElement.FontPitchAsian,
StyleTextPropertiesElement.FontPitchComplex,
StyleTextPropertiesElement.FontRelief,
StyleTextPropertiesElement.FontSizeAsian,
StyleTextPropertiesElement.FontSizeComplex,
StyleTextPropertiesElement.FontSizeRel,
StyleTextPropertiesElement.FontSizeRelAsian,
StyleTextPropertiesElement.FontSizeRelComplex,
StyleTextPropertiesElement.FontStyleAsian,
StyleTextPropertiesElement.FontStyleComplex,
StyleTextPropertiesElement.FontStyleName,
StyleTextPropertiesElement.FontStyleNameAsian,
StyleTextPropertiesElement.FontStyleNameComplex,
StyleTextPropertiesElement.FontWeightAsian,
StyleTextPropertiesElement.FontWeightComplex,
StyleTextPropertiesElement.LanguageAsian,
StyleTextPropertiesElement.LanguageComplex,
StyleTextPropertiesElement.LetterKerning,
StyleTextPropertiesElement.RfcLanguageTag,
StyleTextPropertiesElement.RfcLanguageTagAsian,
StyleTextPropertiesElement.RfcLanguageTagComplex,
StyleTextPropertiesElement.ScriptAsian,
StyleTextPropertiesElement.ScriptComplex,
StyleTextPropertiesElement.ScriptType,
StyleTextPropertiesElement.TextBlinking,
StyleTextPropertiesElement.TextCombine,
StyleTextPropertiesElement.TextCombineEndChar,
StyleTextPropertiesElement.TextCombineStartChar,
StyleTextPropertiesElement.TextEmphasize,
StyleTextPropertiesElement.TextLineThroughColor,
StyleTextPropertiesElement.TextLineThroughMode,
StyleTextPropertiesElement.TextLineThroughStyle,
StyleTextPropertiesElement.TextLineThroughText,
StyleTextPropertiesElement.TextLineThroughTextStyle,
StyleTextPropertiesElement.TextLineThroughType,
StyleTextPropertiesElement.TextLineThroughWidth,
StyleTextPropertiesElement.TextOutline,
StyleTextPropertiesElement.TextOverlineColor,
StyleTextPropertiesElement.TextOverlineMode,
StyleTextPropertiesElement.TextOverlineStyle,
StyleTextPropertiesElement.TextOverlineType,
StyleTextPropertiesElement.TextOverlineWidth,
StyleTextPropertiesElement.TextPosition,
StyleTextPropertiesElement.TextRotationAngle,
StyleTextPropertiesElement.TextRotationScale,
StyleTextPropertiesElement.TextScale,
StyleTextPropertiesElement.TextUnderlineColor,
StyleTextPropertiesElement.TextUnderlineMode,
StyleTextPropertiesElement.TextUnderlineStyle,
StyleTextPropertiesElement.TextUnderlineType,
StyleTextPropertiesElement.TextUnderlineWidth,
StyleTextPropertiesElement.UseWindowFontColor,
StyleTextPropertiesElement.Condition,
StyleTextPropertiesElement.Display,
StyleTextPropertiesElement.Display,
StyleTextPropertiesElement.Display,
});
public final static OdfStyleFamily DrawingPage = new OdfStyleFamily("drawing-page",
new OdfStyleProperty[]{
StyleChartPropertiesElement.AngleOffset,
StyleChartPropertiesElement.AutoPosition,
StyleChartPropertiesElement.AutoSize,
StyleChartPropertiesElement.AxisLabelPosition,
StyleChartPropertiesElement.AxisPosition,
StyleChartPropertiesElement.ConnectBars,
StyleChartPropertiesElement.DataLabelNumber,
StyleChartPropertiesElement.DataLabelSymbol,
StyleChartPropertiesElement.DataLabelText,
StyleChartPropertiesElement.Deep,
StyleChartPropertiesElement.DisplayLabel,
StyleChartPropertiesElement.ErrorCategory,
StyleChartPropertiesElement.ErrorLowerIndicator,
StyleChartPropertiesElement.ErrorLowerLimit,
StyleChartPropertiesElement.ErrorLowerRange,
StyleChartPropertiesElement.ErrorMargin,
StyleChartPropertiesElement.ErrorPercentage,
StyleChartPropertiesElement.ErrorUpperIndicator,
StyleChartPropertiesElement.ErrorUpperLimit,
StyleChartPropertiesElement.ErrorUpperRange,
StyleChartPropertiesElement.GapWidth,
StyleChartPropertiesElement.GroupBarsPerAxis,
StyleChartPropertiesElement.HoleSize,
StyleChartPropertiesElement.IncludeHiddenCells,
StyleChartPropertiesElement.Interpolation,
StyleChartPropertiesElement.IntervalMajor,
StyleChartPropertiesElement.IntervalMinorDivisor,
StyleChartPropertiesElement.JapaneseCandleStick,
StyleChartPropertiesElement.LabelArrangement,
StyleChartPropertiesElement.LabelPosition,
StyleChartPropertiesElement.LabelPositionNegative,
StyleChartPropertiesElement.Lines,
StyleChartPropertiesElement.LinkDataStyleToSource,
StyleChartPropertiesElement.Logarithmic,
StyleChartPropertiesElement.Maximum,
StyleChartPropertiesElement.MeanValue,
StyleChartPropertiesElement.Minimum,
StyleChartPropertiesElement.Origin,
StyleChartPropertiesElement.Overlap,
StyleChartPropertiesElement.Percentage,
StyleChartPropertiesElement.PieOffset,
StyleChartPropertiesElement.RegressionType,
StyleChartPropertiesElement.ReverseDirection,
StyleChartPropertiesElement.RightAngledAxes,
StyleChartPropertiesElement.ScaleText,
StyleChartPropertiesElement.SeriesSource,
StyleChartPropertiesElement.SolidType,
StyleChartPropertiesElement.SortByXValues,
StyleChartPropertiesElement.SplineOrder,
StyleChartPropertiesElement.SplineResolution,
StyleChartPropertiesElement.Stacked,
StyleChartPropertiesElement.SymbolHeight,
StyleChartPropertiesElement.SymbolName,
StyleChartPropertiesElement.SymbolType,
StyleChartPropertiesElement.SymbolType,
StyleChartPropertiesElement.SymbolType,
StyleChartPropertiesElement.SymbolType,
StyleChartPropertiesElement.SymbolWidth,
StyleChartPropertiesElement.TextOverlap,
StyleChartPropertiesElement.ThreeDimensional,
StyleChartPropertiesElement.TickMarkPosition,
StyleChartPropertiesElement.TickMarksMajorInner,
StyleChartPropertiesElement.TickMarksMajorOuter,
StyleChartPropertiesElement.TickMarksMinorInner,
StyleChartPropertiesElement.TickMarksMinorOuter,
StyleChartPropertiesElement.TreatEmptyCells,
StyleChartPropertiesElement.Vertical,
StyleChartPropertiesElement.Visible,
StyleChartPropertiesElement.Direction,
StyleChartPropertiesElement.RotationAngle,
StyleChartPropertiesElement.LineBreak,
StyleDrawingPagePropertiesElement.BackgroundSize,
StyleDrawingPagePropertiesElement.Fill,
StyleDrawingPagePropertiesElement.FillColor,
StyleDrawingPagePropertiesElement.FillGradientName,
StyleDrawingPagePropertiesElement.FillHatchName,
StyleDrawingPagePropertiesElement.FillHatchSolid,
StyleDrawingPagePropertiesElement.FillImageHeight,
StyleDrawingPagePropertiesElement.FillImageName,
StyleDrawingPagePropertiesElement.FillImageRefPoint,
StyleDrawingPagePropertiesElement.FillImageRefPointX,
StyleDrawingPagePropertiesElement.FillImageRefPointY,
StyleDrawingPagePropertiesElement.FillImageWidth,
StyleDrawingPagePropertiesElement.GradientStepCount,
StyleDrawingPagePropertiesElement.Opacity,
StyleDrawingPagePropertiesElement.OpacityName,
StyleDrawingPagePropertiesElement.SecondaryFillColor,
StyleDrawingPagePropertiesElement.TileRepeatOffset,
StyleDrawingPagePropertiesElement.BackgroundObjectsVisible,
StyleDrawingPagePropertiesElement.BackgroundVisible,
StyleDrawingPagePropertiesElement.DisplayDateTime,
StyleDrawingPagePropertiesElement.DisplayFooter,
StyleDrawingPagePropertiesElement.DisplayHeader,
StyleDrawingPagePropertiesElement.DisplayPageNumber,
StyleDrawingPagePropertiesElement.Duration,
StyleDrawingPagePropertiesElement.TransitionSpeed,
StyleDrawingPagePropertiesElement.TransitionStyle,
StyleDrawingPagePropertiesElement.TransitionType,
StyleDrawingPagePropertiesElement.Visibility,
StyleDrawingPagePropertiesElement.Direction,
StyleDrawingPagePropertiesElement.FadeColor,
StyleDrawingPagePropertiesElement.Subtype,
StyleDrawingPagePropertiesElement.Type,
StyleDrawingPagePropertiesElement.Repeat,
StyleDrawingPagePropertiesElement.FillRule,
StyleGraphicPropertiesElement.AmbientColor,
StyleGraphicPropertiesElement.BackScale,
StyleGraphicPropertiesElement.BackfaceCulling,
StyleGraphicPropertiesElement.CloseBack,
StyleGraphicPropertiesElement.CloseFront,
StyleGraphicPropertiesElement.Depth,
StyleGraphicPropertiesElement.DiffuseColor,
StyleGraphicPropertiesElement.EdgeRounding,
StyleGraphicPropertiesElement.EdgeRoundingMode,
StyleGraphicPropertiesElement.EmissiveColor,
StyleGraphicPropertiesElement.EndAngle,
StyleGraphicPropertiesElement.HorizontalSegments,
StyleGraphicPropertiesElement.LightingMode,
StyleGraphicPropertiesElement.NormalsDirection,
StyleGraphicPropertiesElement.NormalsKind,
StyleGraphicPropertiesElement.Dr3dShadow,
StyleGraphicPropertiesElement.Shininess,
StyleGraphicPropertiesElement.SpecularColor,
StyleGraphicPropertiesElement.TextureFilter,
StyleGraphicPropertiesElement.TextureGenerationModeX,
StyleGraphicPropertiesElement.TextureGenerationModeY,
StyleGraphicPropertiesElement.TextureKind,
StyleGraphicPropertiesElement.TextureMode,
StyleGraphicPropertiesElement.VerticalSegments,
StyleGraphicPropertiesElement.AutoGrowHeight,
StyleGraphicPropertiesElement.AutoGrowWidth,
StyleGraphicPropertiesElement.Blue,
StyleGraphicPropertiesElement.CaptionAngle,
StyleGraphicPropertiesElement.CaptionAngleType,
StyleGraphicPropertiesElement.CaptionEscape,
StyleGraphicPropertiesElement.CaptionEscapeDirection,
StyleGraphicPropertiesElement.CaptionFitLineLength,
StyleGraphicPropertiesElement.CaptionGap,
StyleGraphicPropertiesElement.CaptionLineLength,
StyleGraphicPropertiesElement.CaptionType,
StyleGraphicPropertiesElement.ColorInversion,
StyleGraphicPropertiesElement.ColorMode,
StyleGraphicPropertiesElement.Contrast,
StyleGraphicPropertiesElement.DecimalPlaces,
StyleGraphicPropertiesElement.DrawAspect,
StyleGraphicPropertiesElement.EndGuide,
StyleGraphicPropertiesElement.EndLineSpacingHorizontal,
StyleGraphicPropertiesElement.EndLineSpacingVertical,
StyleGraphicPropertiesElement.Fill,
StyleGraphicPropertiesElement.FillColor,
StyleGraphicPropertiesElement.FillGradientName,
StyleGraphicPropertiesElement.FillHatchName,
StyleGraphicPropertiesElement.FillHatchSolid,
StyleGraphicPropertiesElement.FillImageHeight,
StyleGraphicPropertiesElement.FillImageName,
StyleGraphicPropertiesElement.FillImageRefPoint,
StyleGraphicPropertiesElement.FillImageRefPointX,
StyleGraphicPropertiesElement.FillImageRefPointY,
StyleGraphicPropertiesElement.FillImageWidth,
StyleGraphicPropertiesElement.FitToContour,
StyleGraphicPropertiesElement.FitToSize,
StyleGraphicPropertiesElement.FrameDisplayBorder,
StyleGraphicPropertiesElement.FrameDisplayScrollbar,
StyleGraphicPropertiesElement.FrameMarginHorizontal,
StyleGraphicPropertiesElement.FrameMarginVertical,
StyleGraphicPropertiesElement.Gamma,
StyleGraphicPropertiesElement.GradientStepCount,
StyleGraphicPropertiesElement.Green,
StyleGraphicPropertiesElement.GuideDistance,
StyleGraphicPropertiesElement.GuideOverhang,
StyleGraphicPropertiesElement.ImageOpacity,
StyleGraphicPropertiesElement.LineDistance,
StyleGraphicPropertiesElement.Luminance,
StyleGraphicPropertiesElement.MarkerEnd,
StyleGraphicPropertiesElement.MarkerEndCenter,
StyleGraphicPropertiesElement.MarkerEndWidth,
StyleGraphicPropertiesElement.MarkerStart,
StyleGraphicPropertiesElement.MarkerStartCenter,
StyleGraphicPropertiesElement.MarkerStartWidth,
StyleGraphicPropertiesElement.MeasureAlign,
StyleGraphicPropertiesElement.MeasureVerticalAlign,
StyleGraphicPropertiesElement.OleDrawAspect,
StyleGraphicPropertiesElement.Opacity,
StyleGraphicPropertiesElement.OpacityName,
StyleGraphicPropertiesElement.Parallel,
StyleGraphicPropertiesElement.Placing,
StyleGraphicPropertiesElement.Red,
StyleGraphicPropertiesElement.SecondaryFillColor,
StyleGraphicPropertiesElement.DrawShadow,
StyleGraphicPropertiesElement.ShadowColor,
StyleGraphicPropertiesElement.ShadowOffsetX,
StyleGraphicPropertiesElement.ShadowOffsetY,
StyleGraphicPropertiesElement.ShadowOpacity,
StyleGraphicPropertiesElement.ShowUnit,
StyleGraphicPropertiesElement.StartGuide,
StyleGraphicPropertiesElement.StartLineSpacingHorizontal,
StyleGraphicPropertiesElement.StartLineSpacingVertical,
StyleGraphicPropertiesElement.Stroke,
StyleGraphicPropertiesElement.StrokeDash,
StyleGraphicPropertiesElement.StrokeDashNames,
StyleGraphicPropertiesElement.StrokeLinejoin,
StyleGraphicPropertiesElement.SymbolColor,
StyleGraphicPropertiesElement.TextareaHorizontalAlign,
StyleGraphicPropertiesElement.TextareaVerticalAlign,
StyleGraphicPropertiesElement.TileRepeatOffset,
StyleGraphicPropertiesElement.Unit,
StyleGraphicPropertiesElement.VisibleAreaHeight,
StyleGraphicPropertiesElement.VisibleAreaLeft,
StyleGraphicPropertiesElement.VisibleAreaTop,
StyleGraphicPropertiesElement.VisibleAreaWidth,
StyleGraphicPropertiesElement.WrapInfluenceOnPosition,
StyleGraphicPropertiesElement.BackgroundColor,
StyleGraphicPropertiesElement.Border,
StyleGraphicPropertiesElement.BorderBottom,
StyleGraphicPropertiesElement.BorderLeft,
StyleGraphicPropertiesElement.BorderRight,
StyleGraphicPropertiesElement.BorderTop,
StyleGraphicPropertiesElement.Clip,
StyleGraphicPropertiesElement.Margin,
StyleGraphicPropertiesElement.MarginBottom,
StyleGraphicPropertiesElement.MarginLeft,
StyleGraphicPropertiesElement.MarginRight,
StyleGraphicPropertiesElement.MarginTop,
StyleGraphicPropertiesElement.MaxHeight,
StyleGraphicPropertiesElement.MaxWidth,
StyleGraphicPropertiesElement.MinHeight,
StyleGraphicPropertiesElement.MinWidth,
StyleGraphicPropertiesElement.Padding,
StyleGraphicPropertiesElement.PaddingBottom,
StyleGraphicPropertiesElement.PaddingLeft,
StyleGraphicPropertiesElement.PaddingRight,
StyleGraphicPropertiesElement.PaddingTop,
StyleGraphicPropertiesElement.WrapOption,
StyleGraphicPropertiesElement.BackgroundTransparency,
StyleGraphicPropertiesElement.BorderLineWidth,
StyleGraphicPropertiesElement.BorderLineWidthBottom,
StyleGraphicPropertiesElement.BorderLineWidthLeft,
StyleGraphicPropertiesElement.BorderLineWidthRight,
StyleGraphicPropertiesElement.BorderLineWidthTop,
StyleGraphicPropertiesElement.Editable,
StyleGraphicPropertiesElement.FlowWithText,
StyleGraphicPropertiesElement.HorizontalPos,
StyleGraphicPropertiesElement.HorizontalRel,
StyleGraphicPropertiesElement.Mirror,
StyleGraphicPropertiesElement.NumberWrappedParagraphs,
StyleGraphicPropertiesElement.OverflowBehavior,
StyleGraphicPropertiesElement.PrintContent,
StyleGraphicPropertiesElement.Protect,
StyleGraphicPropertiesElement.RelHeight,
StyleGraphicPropertiesElement.RelWidth,
StyleGraphicPropertiesElement.Repeat,
StyleGraphicPropertiesElement.RunThrough,
StyleGraphicPropertiesElement.StyleShadow,
StyleGraphicPropertiesElement.ShrinkToFit,
StyleGraphicPropertiesElement.VerticalPos,
StyleGraphicPropertiesElement.VerticalRel,
StyleGraphicPropertiesElement.Wrap,
StyleGraphicPropertiesElement.WrapContour,
StyleGraphicPropertiesElement.WrapContourMode,
StyleGraphicPropertiesElement.WrapDynamicThreshold,
StyleGraphicPropertiesElement.WritingMode,
StyleGraphicPropertiesElement.FillRule,
StyleGraphicPropertiesElement.Height,
StyleGraphicPropertiesElement.StrokeColor,
StyleGraphicPropertiesElement.StrokeLinecap,
StyleGraphicPropertiesElement.StrokeOpacity,
StyleGraphicPropertiesElement.StrokeWidth,
StyleGraphicPropertiesElement.Width,
StyleGraphicPropertiesElement.X,
StyleGraphicPropertiesElement.Y,
StyleGraphicPropertiesElement.AnchorPageNumber,
StyleGraphicPropertiesElement.AnchorType,
StyleGraphicPropertiesElement.Animation,
StyleGraphicPropertiesElement.AnimationDelay,
StyleGraphicPropertiesElement.AnimationDirection,
StyleGraphicPropertiesElement.AnimationRepeat,
StyleGraphicPropertiesElement.AnimationStartInside,
StyleGraphicPropertiesElement.AnimationSteps,
StyleGraphicPropertiesElement.AnimationStopInside,
StyleHeaderFooterPropertiesElement.BackgroundColor,
StyleHeaderFooterPropertiesElement.Border,
StyleHeaderFooterPropertiesElement.BorderBottom,
StyleHeaderFooterPropertiesElement.BorderLeft,
StyleHeaderFooterPropertiesElement.BorderRight,
StyleHeaderFooterPropertiesElement.BorderTop,
StyleHeaderFooterPropertiesElement.Margin,
StyleHeaderFooterPropertiesElement.MarginBottom,
StyleHeaderFooterPropertiesElement.MarginLeft,
StyleHeaderFooterPropertiesElement.MarginRight,
StyleHeaderFooterPropertiesElement.MarginTop,
StyleHeaderFooterPropertiesElement.MinHeight,
StyleHeaderFooterPropertiesElement.Padding,
StyleHeaderFooterPropertiesElement.PaddingBottom,
StyleHeaderFooterPropertiesElement.PaddingLeft,
StyleHeaderFooterPropertiesElement.PaddingRight,
StyleHeaderFooterPropertiesElement.PaddingTop,
StyleHeaderFooterPropertiesElement.BorderLineWidth,
StyleHeaderFooterPropertiesElement.BorderLineWidthBottom,
StyleHeaderFooterPropertiesElement.BorderLineWidthLeft,
StyleHeaderFooterPropertiesElement.BorderLineWidthRight,
StyleHeaderFooterPropertiesElement.BorderLineWidthTop,
StyleHeaderFooterPropertiesElement.DynamicSpacing,
StyleHeaderFooterPropertiesElement.Shadow,
StyleHeaderFooterPropertiesElement.Height,
StyleListLevelPropertiesElement.Height,
StyleListLevelPropertiesElement.TextAlign,
StyleListLevelPropertiesElement.Width,
StyleListLevelPropertiesElement.FontName,
StyleListLevelPropertiesElement.VerticalPos,
StyleListLevelPropertiesElement.VerticalRel,
StyleListLevelPropertiesElement.Y,
StyleListLevelPropertiesElement.ListLevelPositionAndSpaceMode,
StyleListLevelPropertiesElement.MinLabelDistance,
StyleListLevelPropertiesElement.MinLabelWidth,
StyleListLevelPropertiesElement.SpaceBefore,
StylePageLayoutPropertiesElement.BackgroundColor,
StylePageLayoutPropertiesElement.Border,
StylePageLayoutPropertiesElement.BorderBottom,
StylePageLayoutPropertiesElement.BorderLeft,
StylePageLayoutPropertiesElement.BorderRight,
StylePageLayoutPropertiesElement.BorderTop,
StylePageLayoutPropertiesElement.Margin,
StylePageLayoutPropertiesElement.MarginBottom,
StylePageLayoutPropertiesElement.MarginLeft,
StylePageLayoutPropertiesElement.MarginRight,
StylePageLayoutPropertiesElement.MarginTop,
StylePageLayoutPropertiesElement.Padding,
StylePageLayoutPropertiesElement.PaddingBottom,
StylePageLayoutPropertiesElement.PaddingLeft,
StylePageLayoutPropertiesElement.PaddingRight,
StylePageLayoutPropertiesElement.PaddingTop,
StylePageLayoutPropertiesElement.PageHeight,
StylePageLayoutPropertiesElement.PageWidth,
StylePageLayoutPropertiesElement.BorderLineWidth,
StylePageLayoutPropertiesElement.BorderLineWidthBottom,
StylePageLayoutPropertiesElement.BorderLineWidthLeft,
StylePageLayoutPropertiesElement.BorderLineWidthRight,
StylePageLayoutPropertiesElement.BorderLineWidthTop,
StylePageLayoutPropertiesElement.FirstPageNumber,
StylePageLayoutPropertiesElement.FootnoteMaxHeight,
StylePageLayoutPropertiesElement.LayoutGridBaseHeight,
StylePageLayoutPropertiesElement.LayoutGridBaseWidth,
StylePageLayoutPropertiesElement.LayoutGridColor,
StylePageLayoutPropertiesElement.LayoutGridDisplay,
StylePageLayoutPropertiesElement.LayoutGridLines,
StylePageLayoutPropertiesElement.LayoutGridMode,
StylePageLayoutPropertiesElement.LayoutGridPrint,
StylePageLayoutPropertiesElement.LayoutGridRubyBelow,
StylePageLayoutPropertiesElement.LayoutGridRubyHeight,
StylePageLayoutPropertiesElement.LayoutGridSnapTo,
StylePageLayoutPropertiesElement.LayoutGridStandardMode,
StylePageLayoutPropertiesElement.NumFormat,
StylePageLayoutPropertiesElement.NumFormat,
StylePageLayoutPropertiesElement.NumLetterSync,
StylePageLayoutPropertiesElement.NumPrefix,
StylePageLayoutPropertiesElement.NumSuffix,
StylePageLayoutPropertiesElement.PaperTrayName,
StylePageLayoutPropertiesElement.Print,
StylePageLayoutPropertiesElement.PrintOrientation,
StylePageLayoutPropertiesElement.PrintPageOrder,
StylePageLayoutPropertiesElement.RegisterTruthRefStyleName,
StylePageLayoutPropertiesElement.ScaleTo,
StylePageLayoutPropertiesElement.ScaleToPages,
StylePageLayoutPropertiesElement.Shadow,
StylePageLayoutPropertiesElement.TableCentering,
StylePageLayoutPropertiesElement.WritingMode,
StyleParagraphPropertiesElement.BackgroundColor,
StyleParagraphPropertiesElement.Border,
StyleParagraphPropertiesElement.BorderBottom,
StyleParagraphPropertiesElement.BorderLeft,
StyleParagraphPropertiesElement.BorderRight,
StyleParagraphPropertiesElement.BorderTop,
StyleParagraphPropertiesElement.BreakAfter,
StyleParagraphPropertiesElement.BreakBefore,
StyleParagraphPropertiesElement.HyphenationKeep,
StyleParagraphPropertiesElement.HyphenationLadderCount,
StyleParagraphPropertiesElement.KeepTogether,
StyleParagraphPropertiesElement.KeepWithNext,
StyleParagraphPropertiesElement.LineHeight,
StyleParagraphPropertiesElement.Margin,
StyleParagraphPropertiesElement.MarginBottom,
StyleParagraphPropertiesElement.MarginLeft,
StyleParagraphPropertiesElement.MarginRight,
StyleParagraphPropertiesElement.MarginTop,
StyleParagraphPropertiesElement.Orphans,
StyleParagraphPropertiesElement.Padding,
StyleParagraphPropertiesElement.PaddingBottom,
StyleParagraphPropertiesElement.PaddingLeft,
StyleParagraphPropertiesElement.PaddingRight,
StyleParagraphPropertiesElement.PaddingTop,
StyleParagraphPropertiesElement.TextAlign,
StyleParagraphPropertiesElement.TextAlignLast,
StyleParagraphPropertiesElement.TextIndent,
StyleParagraphPropertiesElement.Widows,
StyleParagraphPropertiesElement.AutoTextIndent,
StyleParagraphPropertiesElement.BackgroundTransparency,
StyleParagraphPropertiesElement.BorderLineWidth,
StyleParagraphPropertiesElement.BorderLineWidthBottom,
StyleParagraphPropertiesElement.BorderLineWidthLeft,
StyleParagraphPropertiesElement.BorderLineWidthRight,
StyleParagraphPropertiesElement.BorderLineWidthTop,
StyleParagraphPropertiesElement.FontIndependentLineSpacing,
StyleParagraphPropertiesElement.JoinBorder,
StyleParagraphPropertiesElement.JustifySingleWord,
StyleParagraphPropertiesElement.LineBreak,
StyleParagraphPropertiesElement.LineHeightAtLeast,
StyleParagraphPropertiesElement.LineSpacing,
StyleParagraphPropertiesElement.PageNumber,
StyleParagraphPropertiesElement.PunctuationWrap,
StyleParagraphPropertiesElement.RegisterTrue,
StyleParagraphPropertiesElement.Shadow,
StyleParagraphPropertiesElement.SnapToLayoutGrid,
StyleParagraphPropertiesElement.TabStopDistance,
StyleParagraphPropertiesElement.TextAutospace,
StyleParagraphPropertiesElement.VerticalAlign,
StyleParagraphPropertiesElement.WritingMode,
StyleParagraphPropertiesElement.WritingModeAutomatic,
StyleParagraphPropertiesElement.LineNumber,
StyleParagraphPropertiesElement.NumberLines,
StyleRubyPropertiesElement.RubyAlign,
StyleRubyPropertiesElement.RubyPosition,
StyleSectionPropertiesElement.BackgroundColor,
StyleSectionPropertiesElement.MarginLeft,
StyleSectionPropertiesElement.MarginRight,
StyleSectionPropertiesElement.Editable,
StyleSectionPropertiesElement.Protect,
StyleSectionPropertiesElement.WritingMode,
StyleSectionPropertiesElement.DontBalanceTextColumns,
StyleTableCellPropertiesElement.BackgroundColor,
StyleTableCellPropertiesElement.Border,
StyleTableCellPropertiesElement.BorderBottom,
StyleTableCellPropertiesElement.BorderLeft,
StyleTableCellPropertiesElement.BorderRight,
StyleTableCellPropertiesElement.BorderTop,
StyleTableCellPropertiesElement.Padding,
StyleTableCellPropertiesElement.PaddingBottom,
StyleTableCellPropertiesElement.PaddingLeft,
StyleTableCellPropertiesElement.PaddingRight,
StyleTableCellPropertiesElement.PaddingTop,
StyleTableCellPropertiesElement.WrapOption,
StyleTableCellPropertiesElement.BorderLineWidth,
StyleTableCellPropertiesElement.BorderLineWidthBottom,
StyleTableCellPropertiesElement.BorderLineWidthLeft,
StyleTableCellPropertiesElement.BorderLineWidthRight,
StyleTableCellPropertiesElement.BorderLineWidthTop,
StyleTableCellPropertiesElement.CellProtect,
StyleTableCellPropertiesElement.DecimalPlaces,
StyleTableCellPropertiesElement.DiagonalBlTr,
StyleTableCellPropertiesElement.DiagonalBlTrWidths,
StyleTableCellPropertiesElement.DiagonalTlBr,
StyleTableCellPropertiesElement.DiagonalTlBrWidths,
StyleTableCellPropertiesElement.Direction,
StyleTableCellPropertiesElement.GlyphOrientationVertical,
StyleTableCellPropertiesElement.PrintContent,
StyleTableCellPropertiesElement.RepeatContent,
StyleTableCellPropertiesElement.RotationAlign,
StyleTableCellPropertiesElement.RotationAngle,
StyleTableCellPropertiesElement.Shadow,
StyleTableCellPropertiesElement.ShrinkToFit,
StyleTableCellPropertiesElement.TextAlignSource,
StyleTableCellPropertiesElement.VerticalAlign,
StyleTableCellPropertiesElement.WritingMode,
StyleTableColumnPropertiesElement.BreakAfter,
StyleTableColumnPropertiesElement.BreakBefore,
StyleTableColumnPropertiesElement.ColumnWidth,
StyleTableColumnPropertiesElement.RelColumnWidth,
StyleTableColumnPropertiesElement.UseOptimalColumnWidth,
StyleTablePropertiesElement.BackgroundColor,
StyleTablePropertiesElement.BreakAfter,
StyleTablePropertiesElement.BreakBefore,
StyleTablePropertiesElement.KeepWithNext,
StyleTablePropertiesElement.Margin,
StyleTablePropertiesElement.MarginBottom,
StyleTablePropertiesElement.MarginLeft,
StyleTablePropertiesElement.MarginRight,
StyleTablePropertiesElement.MarginTop,
StyleTablePropertiesElement.MayBreakBetweenRows,
StyleTablePropertiesElement.PageNumber,
StyleTablePropertiesElement.RelWidth,
StyleTablePropertiesElement.Shadow,
StyleTablePropertiesElement.Width,
StyleTablePropertiesElement.WritingMode,
StyleTablePropertiesElement.Align,
StyleTablePropertiesElement.BorderModel,
StyleTablePropertiesElement.Display,
StyleTableRowPropertiesElement.BackgroundColor,
StyleTableRowPropertiesElement.BreakAfter,
StyleTableRowPropertiesElement.BreakBefore,
StyleTableRowPropertiesElement.KeepTogether,
StyleTableRowPropertiesElement.MinRowHeight,
StyleTableRowPropertiesElement.RowHeight,
StyleTableRowPropertiesElement.UseOptimalRowHeight,
StyleTextPropertiesElement.BackgroundColor,
StyleTextPropertiesElement.Color,
StyleTextPropertiesElement.Country,
StyleTextPropertiesElement.FontFamily,
StyleTextPropertiesElement.FontSize,
StyleTextPropertiesElement.FontStyle,
StyleTextPropertiesElement.FontVariant,
StyleTextPropertiesElement.FontWeight,
StyleTextPropertiesElement.Hyphenate,
StyleTextPropertiesElement.HyphenationPushCharCount,
StyleTextPropertiesElement.HyphenationRemainCharCount,
StyleTextPropertiesElement.Language,
StyleTextPropertiesElement.LetterSpacing,
StyleTextPropertiesElement.Script,
StyleTextPropertiesElement.TextShadow,
StyleTextPropertiesElement.TextTransform,
StyleTextPropertiesElement.CountryAsian,
StyleTextPropertiesElement.CountryComplex,
StyleTextPropertiesElement.FontCharset,
StyleTextPropertiesElement.FontCharsetAsian,
StyleTextPropertiesElement.FontCharsetComplex,
StyleTextPropertiesElement.FontFamilyAsian,
StyleTextPropertiesElement.FontFamilyComplex,
StyleTextPropertiesElement.FontFamilyGeneric,
StyleTextPropertiesElement.FontFamilyGenericAsian,
StyleTextPropertiesElement.FontFamilyGenericComplex,
StyleTextPropertiesElement.FontName,
StyleTextPropertiesElement.FontNameAsian,
StyleTextPropertiesElement.FontNameComplex,
StyleTextPropertiesElement.FontPitch,
StyleTextPropertiesElement.FontPitchAsian,
StyleTextPropertiesElement.FontPitchComplex,
StyleTextPropertiesElement.FontRelief,
StyleTextPropertiesElement.FontSizeAsian,
StyleTextPropertiesElement.FontSizeComplex,
StyleTextPropertiesElement.FontSizeRel,
StyleTextPropertiesElement.FontSizeRelAsian,
StyleTextPropertiesElement.FontSizeRelComplex,
StyleTextPropertiesElement.FontStyleAsian,
StyleTextPropertiesElement.FontStyleComplex,
StyleTextPropertiesElement.FontStyleName,
StyleTextPropertiesElement.FontStyleNameAsian,
StyleTextPropertiesElement.FontStyleNameComplex,
StyleTextPropertiesElement.FontWeightAsian,
StyleTextPropertiesElement.FontWeightComplex,
StyleTextPropertiesElement.LanguageAsian,
StyleTextPropertiesElement.LanguageComplex,
StyleTextPropertiesElement.LetterKerning,
StyleTextPropertiesElement.RfcLanguageTag,
StyleTextPropertiesElement.RfcLanguageTagAsian,
StyleTextPropertiesElement.RfcLanguageTagComplex,
StyleTextPropertiesElement.ScriptAsian,
StyleTextPropertiesElement.ScriptComplex,
StyleTextPropertiesElement.ScriptType,
StyleTextPropertiesElement.TextBlinking,
StyleTextPropertiesElement.TextCombine,
StyleTextPropertiesElement.TextCombineEndChar,
StyleTextPropertiesElement.TextCombineStartChar,
StyleTextPropertiesElement.TextEmphasize,
StyleTextPropertiesElement.TextLineThroughColor,
StyleTextPropertiesElement.TextLineThroughMode,
StyleTextPropertiesElement.TextLineThroughStyle,
StyleTextPropertiesElement.TextLineThroughText,
StyleTextPropertiesElement.TextLineThroughTextStyle,
StyleTextPropertiesElement.TextLineThroughType,
StyleTextPropertiesElement.TextLineThroughWidth,
StyleTextPropertiesElement.TextOutline,
StyleTextPropertiesElement.TextOverlineColor,
StyleTextPropertiesElement.TextOverlineMode,
StyleTextPropertiesElement.TextOverlineStyle,
StyleTextPropertiesElement.TextOverlineType,
StyleTextPropertiesElement.TextOverlineWidth,
StyleTextPropertiesElement.TextPosition,
StyleTextPropertiesElement.TextRotationAngle,
StyleTextPropertiesElement.TextRotationScale,
StyleTextPropertiesElement.TextScale,
StyleTextPropertiesElement.TextUnderlineColor,
StyleTextPropertiesElement.TextUnderlineMode,
StyleTextPropertiesElement.TextUnderlineStyle,
StyleTextPropertiesElement.TextUnderlineType,
StyleTextPropertiesElement.TextUnderlineWidth,
StyleTextPropertiesElement.UseWindowFontColor,
StyleTextPropertiesElement.Condition,
StyleTextPropertiesElement.Display,
StyleTextPropertiesElement.Display,
StyleTextPropertiesElement.Display,
});
public final static OdfStyleFamily Graphic = new OdfStyleFamily("graphic",
new OdfStyleProperty[]{
StyleChartPropertiesElement.AngleOffset,
StyleChartPropertiesElement.AutoPosition,
StyleChartPropertiesElement.AutoSize,
StyleChartPropertiesElement.AxisLabelPosition,
StyleChartPropertiesElement.AxisPosition,
StyleChartPropertiesElement.ConnectBars,
StyleChartPropertiesElement.DataLabelNumber,
StyleChartPropertiesElement.DataLabelSymbol,
StyleChartPropertiesElement.DataLabelText,
StyleChartPropertiesElement.Deep,
StyleChartPropertiesElement.DisplayLabel,
StyleChartPropertiesElement.ErrorCategory,
StyleChartPropertiesElement.ErrorLowerIndicator,
StyleChartPropertiesElement.ErrorLowerLimit,
StyleChartPropertiesElement.ErrorLowerRange,
StyleChartPropertiesElement.ErrorMargin,
StyleChartPropertiesElement.ErrorPercentage,
StyleChartPropertiesElement.ErrorUpperIndicator,
StyleChartPropertiesElement.ErrorUpperLimit,
StyleChartPropertiesElement.ErrorUpperRange,
StyleChartPropertiesElement.GapWidth,
StyleChartPropertiesElement.GroupBarsPerAxis,
StyleChartPropertiesElement.HoleSize,
StyleChartPropertiesElement.IncludeHiddenCells,
StyleChartPropertiesElement.Interpolation,
StyleChartPropertiesElement.IntervalMajor,
StyleChartPropertiesElement.IntervalMinorDivisor,
StyleChartPropertiesElement.JapaneseCandleStick,
StyleChartPropertiesElement.LabelArrangement,
StyleChartPropertiesElement.LabelPosition,
StyleChartPropertiesElement.LabelPositionNegative,
StyleChartPropertiesElement.Lines,
StyleChartPropertiesElement.LinkDataStyleToSource,
StyleChartPropertiesElement.Logarithmic,
StyleChartPropertiesElement.Maximum,
StyleChartPropertiesElement.MeanValue,
StyleChartPropertiesElement.Minimum,
StyleChartPropertiesElement.Origin,
StyleChartPropertiesElement.Overlap,
StyleChartPropertiesElement.Percentage,
StyleChartPropertiesElement.PieOffset,
StyleChartPropertiesElement.RegressionType,
StyleChartPropertiesElement.ReverseDirection,
StyleChartPropertiesElement.RightAngledAxes,
StyleChartPropertiesElement.ScaleText,
StyleChartPropertiesElement.SeriesSource,
StyleChartPropertiesElement.SolidType,
StyleChartPropertiesElement.SortByXValues,
StyleChartPropertiesElement.SplineOrder,
StyleChartPropertiesElement.SplineResolution,
StyleChartPropertiesElement.Stacked,
StyleChartPropertiesElement.SymbolHeight,
StyleChartPropertiesElement.SymbolName,
StyleChartPropertiesElement.SymbolType,
StyleChartPropertiesElement.SymbolType,
StyleChartPropertiesElement.SymbolType,
StyleChartPropertiesElement.SymbolType,
StyleChartPropertiesElement.SymbolWidth,
StyleChartPropertiesElement.TextOverlap,
StyleChartPropertiesElement.ThreeDimensional,
StyleChartPropertiesElement.TickMarkPosition,
StyleChartPropertiesElement.TickMarksMajorInner,
StyleChartPropertiesElement.TickMarksMajorOuter,
StyleChartPropertiesElement.TickMarksMinorInner,
StyleChartPropertiesElement.TickMarksMinorOuter,
StyleChartPropertiesElement.TreatEmptyCells,
StyleChartPropertiesElement.Vertical,
StyleChartPropertiesElement.Visible,
StyleChartPropertiesElement.Direction,
StyleChartPropertiesElement.RotationAngle,
StyleChartPropertiesElement.LineBreak,
StyleDrawingPagePropertiesElement.BackgroundSize,
StyleDrawingPagePropertiesElement.Fill,
StyleDrawingPagePropertiesElement.FillColor,
StyleDrawingPagePropertiesElement.FillGradientName,
StyleDrawingPagePropertiesElement.FillHatchName,
StyleDrawingPagePropertiesElement.FillHatchSolid,
StyleDrawingPagePropertiesElement.FillImageHeight,
StyleDrawingPagePropertiesElement.FillImageName,
StyleDrawingPagePropertiesElement.FillImageRefPoint,
StyleDrawingPagePropertiesElement.FillImageRefPointX,
StyleDrawingPagePropertiesElement.FillImageRefPointY,
StyleDrawingPagePropertiesElement.FillImageWidth,
StyleDrawingPagePropertiesElement.GradientStepCount,
StyleDrawingPagePropertiesElement.Opacity,
StyleDrawingPagePropertiesElement.OpacityName,
StyleDrawingPagePropertiesElement.SecondaryFillColor,
StyleDrawingPagePropertiesElement.TileRepeatOffset,
StyleDrawingPagePropertiesElement.BackgroundObjectsVisible,
StyleDrawingPagePropertiesElement.BackgroundVisible,
StyleDrawingPagePropertiesElement.DisplayDateTime,
StyleDrawingPagePropertiesElement.DisplayFooter,
StyleDrawingPagePropertiesElement.DisplayHeader,
StyleDrawingPagePropertiesElement.DisplayPageNumber,
StyleDrawingPagePropertiesElement.Duration,
StyleDrawingPagePropertiesElement.TransitionSpeed,
StyleDrawingPagePropertiesElement.TransitionStyle,
StyleDrawingPagePropertiesElement.TransitionType,
StyleDrawingPagePropertiesElement.Visibility,
StyleDrawingPagePropertiesElement.Direction,
StyleDrawingPagePropertiesElement.FadeColor,
StyleDrawingPagePropertiesElement.Subtype,
StyleDrawingPagePropertiesElement.Type,
StyleDrawingPagePropertiesElement.Repeat,
StyleDrawingPagePropertiesElement.FillRule,
StyleGraphicPropertiesElement.AmbientColor,
StyleGraphicPropertiesElement.BackScale,
StyleGraphicPropertiesElement.BackfaceCulling,
StyleGraphicPropertiesElement.CloseBack,
StyleGraphicPropertiesElement.CloseFront,
StyleGraphicPropertiesElement.Depth,
StyleGraphicPropertiesElement.DiffuseColor,
StyleGraphicPropertiesElement.EdgeRounding,
StyleGraphicPropertiesElement.EdgeRoundingMode,
StyleGraphicPropertiesElement.EmissiveColor,
StyleGraphicPropertiesElement.EndAngle,
StyleGraphicPropertiesElement.HorizontalSegments,
StyleGraphicPropertiesElement.LightingMode,
StyleGraphicPropertiesElement.NormalsDirection,
StyleGraphicPropertiesElement.NormalsKind,
StyleGraphicPropertiesElement.Dr3dShadow,
StyleGraphicPropertiesElement.Shininess,
StyleGraphicPropertiesElement.SpecularColor,
StyleGraphicPropertiesElement.TextureFilter,
StyleGraphicPropertiesElement.TextureGenerationModeX,
StyleGraphicPropertiesElement.TextureGenerationModeY,
StyleGraphicPropertiesElement.TextureKind,
StyleGraphicPropertiesElement.TextureMode,
StyleGraphicPropertiesElement.VerticalSegments,
StyleGraphicPropertiesElement.AutoGrowHeight,
StyleGraphicPropertiesElement.AutoGrowWidth,
StyleGraphicPropertiesElement.Blue,
StyleGraphicPropertiesElement.CaptionAngle,
StyleGraphicPropertiesElement.CaptionAngleType,
StyleGraphicPropertiesElement.CaptionEscape,
StyleGraphicPropertiesElement.CaptionEscapeDirection,
StyleGraphicPropertiesElement.CaptionFitLineLength,
StyleGraphicPropertiesElement.CaptionGap,
StyleGraphicPropertiesElement.CaptionLineLength,
StyleGraphicPropertiesElement.CaptionType,
StyleGraphicPropertiesElement.ColorInversion,
StyleGraphicPropertiesElement.ColorMode,
StyleGraphicPropertiesElement.Contrast,
StyleGraphicPropertiesElement.DecimalPlaces,
StyleGraphicPropertiesElement.DrawAspect,
StyleGraphicPropertiesElement.EndGuide,
StyleGraphicPropertiesElement.EndLineSpacingHorizontal,
StyleGraphicPropertiesElement.EndLineSpacingVertical,
StyleGraphicPropertiesElement.Fill,
StyleGraphicPropertiesElement.FillColor,
StyleGraphicPropertiesElement.FillGradientName,
StyleGraphicPropertiesElement.FillHatchName,
StyleGraphicPropertiesElement.FillHatchSolid,
StyleGraphicPropertiesElement.FillImageHeight,
StyleGraphicPropertiesElement.FillImageName,
StyleGraphicPropertiesElement.FillImageRefPoint,
StyleGraphicPropertiesElement.FillImageRefPointX,
StyleGraphicPropertiesElement.FillImageRefPointY,
StyleGraphicPropertiesElement.FillImageWidth,
StyleGraphicPropertiesElement.FitToContour,
StyleGraphicPropertiesElement.FitToSize,
StyleGraphicPropertiesElement.FrameDisplayBorder,
StyleGraphicPropertiesElement.FrameDisplayScrollbar,
StyleGraphicPropertiesElement.FrameMarginHorizontal,
StyleGraphicPropertiesElement.FrameMarginVertical,
StyleGraphicPropertiesElement.Gamma,
StyleGraphicPropertiesElement.GradientStepCount,
StyleGraphicPropertiesElement.Green,
StyleGraphicPropertiesElement.GuideDistance,
StyleGraphicPropertiesElement.GuideOverhang,
StyleGraphicPropertiesElement.ImageOpacity,
StyleGraphicPropertiesElement.LineDistance,
StyleGraphicPropertiesElement.Luminance,
StyleGraphicPropertiesElement.MarkerEnd,
StyleGraphicPropertiesElement.MarkerEndCenter,
StyleGraphicPropertiesElement.MarkerEndWidth,
StyleGraphicPropertiesElement.MarkerStart,
StyleGraphicPropertiesElement.MarkerStartCenter,
StyleGraphicPropertiesElement.MarkerStartWidth,
StyleGraphicPropertiesElement.MeasureAlign,
StyleGraphicPropertiesElement.MeasureVerticalAlign,
StyleGraphicPropertiesElement.OleDrawAspect,
StyleGraphicPropertiesElement.Opacity,
StyleGraphicPropertiesElement.OpacityName,
StyleGraphicPropertiesElement.Parallel,
StyleGraphicPropertiesElement.Placing,
StyleGraphicPropertiesElement.Red,
StyleGraphicPropertiesElement.SecondaryFillColor,
StyleGraphicPropertiesElement.DrawShadow,
StyleGraphicPropertiesElement.ShadowColor,
StyleGraphicPropertiesElement.ShadowOffsetX,
StyleGraphicPropertiesElement.ShadowOffsetY,
StyleGraphicPropertiesElement.ShadowOpacity,
StyleGraphicPropertiesElement.ShowUnit,
StyleGraphicPropertiesElement.StartGuide,
StyleGraphicPropertiesElement.StartLineSpacingHorizontal,
StyleGraphicPropertiesElement.StartLineSpacingVertical,
StyleGraphicPropertiesElement.Stroke,
StyleGraphicPropertiesElement.StrokeDash,
StyleGraphicPropertiesElement.StrokeDashNames,
StyleGraphicPropertiesElement.StrokeLinejoin,
StyleGraphicPropertiesElement.SymbolColor,
StyleGraphicPropertiesElement.TextareaHorizontalAlign,
StyleGraphicPropertiesElement.TextareaVerticalAlign,
StyleGraphicPropertiesElement.TileRepeatOffset,
StyleGraphicPropertiesElement.Unit,
StyleGraphicPropertiesElement.VisibleAreaHeight,
StyleGraphicPropertiesElement.VisibleAreaLeft,
StyleGraphicPropertiesElement.VisibleAreaTop,
StyleGraphicPropertiesElement.VisibleAreaWidth,
StyleGraphicPropertiesElement.WrapInfluenceOnPosition,
StyleGraphicPropertiesElement.BackgroundColor,
StyleGraphicPropertiesElement.Border,
StyleGraphicPropertiesElement.BorderBottom,
StyleGraphicPropertiesElement.BorderLeft,
StyleGraphicPropertiesElement.BorderRight,
StyleGraphicPropertiesElement.BorderTop,
StyleGraphicPropertiesElement.Clip,
StyleGraphicPropertiesElement.Margin,
StyleGraphicPropertiesElement.MarginBottom,
StyleGraphicPropertiesElement.MarginLeft,
StyleGraphicPropertiesElement.MarginRight,
StyleGraphicPropertiesElement.MarginTop,
StyleGraphicPropertiesElement.MaxHeight,
StyleGraphicPropertiesElement.MaxWidth,
StyleGraphicPropertiesElement.MinHeight,
StyleGraphicPropertiesElement.MinWidth,
StyleGraphicPropertiesElement.Padding,
StyleGraphicPropertiesElement.PaddingBottom,
StyleGraphicPropertiesElement.PaddingLeft,
StyleGraphicPropertiesElement.PaddingRight,
StyleGraphicPropertiesElement.PaddingTop,
StyleGraphicPropertiesElement.WrapOption,
StyleGraphicPropertiesElement.BackgroundTransparency,
StyleGraphicPropertiesElement.BorderLineWidth,
StyleGraphicPropertiesElement.BorderLineWidthBottom,
StyleGraphicPropertiesElement.BorderLineWidthLeft,
StyleGraphicPropertiesElement.BorderLineWidthRight,
StyleGraphicPropertiesElement.BorderLineWidthTop,
StyleGraphicPropertiesElement.Editable,
StyleGraphicPropertiesElement.FlowWithText,
StyleGraphicPropertiesElement.HorizontalPos,
StyleGraphicPropertiesElement.HorizontalRel,
StyleGraphicPropertiesElement.Mirror,
StyleGraphicPropertiesElement.NumberWrappedParagraphs,
StyleGraphicPropertiesElement.OverflowBehavior,
StyleGraphicPropertiesElement.PrintContent,
StyleGraphicPropertiesElement.Protect,
StyleGraphicPropertiesElement.RelHeight,
StyleGraphicPropertiesElement.RelWidth,
StyleGraphicPropertiesElement.Repeat,
StyleGraphicPropertiesElement.RunThrough,
StyleGraphicPropertiesElement.StyleShadow,
StyleGraphicPropertiesElement.ShrinkToFit,
StyleGraphicPropertiesElement.VerticalPos,
StyleGraphicPropertiesElement.VerticalRel,
StyleGraphicPropertiesElement.Wrap,
StyleGraphicPropertiesElement.WrapContour,
StyleGraphicPropertiesElement.WrapContourMode,
StyleGraphicPropertiesElement.WrapDynamicThreshold,
StyleGraphicPropertiesElement.WritingMode,
StyleGraphicPropertiesElement.FillRule,
StyleGraphicPropertiesElement.Height,
StyleGraphicPropertiesElement.StrokeColor,
StyleGraphicPropertiesElement.StrokeLinecap,
StyleGraphicPropertiesElement.StrokeOpacity,
StyleGraphicPropertiesElement.StrokeWidth,
StyleGraphicPropertiesElement.Width,
StyleGraphicPropertiesElement.X,
StyleGraphicPropertiesElement.Y,
StyleGraphicPropertiesElement.AnchorPageNumber,
StyleGraphicPropertiesElement.AnchorType,
StyleGraphicPropertiesElement.Animation,
StyleGraphicPropertiesElement.AnimationDelay,
StyleGraphicPropertiesElement.AnimationDirection,
StyleGraphicPropertiesElement.AnimationRepeat,
StyleGraphicPropertiesElement.AnimationStartInside,
StyleGraphicPropertiesElement.AnimationSteps,
StyleGraphicPropertiesElement.AnimationStopInside,
StyleHeaderFooterPropertiesElement.BackgroundColor,
StyleHeaderFooterPropertiesElement.Border,
StyleHeaderFooterPropertiesElement.BorderBottom,
StyleHeaderFooterPropertiesElement.BorderLeft,
StyleHeaderFooterPropertiesElement.BorderRight,
StyleHeaderFooterPropertiesElement.BorderTop,
StyleHeaderFooterPropertiesElement.Margin,
StyleHeaderFooterPropertiesElement.MarginBottom,
StyleHeaderFooterPropertiesElement.MarginLeft,
StyleHeaderFooterPropertiesElement.MarginRight,
StyleHeaderFooterPropertiesElement.MarginTop,
StyleHeaderFooterPropertiesElement.MinHeight,
StyleHeaderFooterPropertiesElement.Padding,
StyleHeaderFooterPropertiesElement.PaddingBottom,
StyleHeaderFooterPropertiesElement.PaddingLeft,
StyleHeaderFooterPropertiesElement.PaddingRight,
StyleHeaderFooterPropertiesElement.PaddingTop,
StyleHeaderFooterPropertiesElement.BorderLineWidth,
StyleHeaderFooterPropertiesElement.BorderLineWidthBottom,
StyleHeaderFooterPropertiesElement.BorderLineWidthLeft,
StyleHeaderFooterPropertiesElement.BorderLineWidthRight,
StyleHeaderFooterPropertiesElement.BorderLineWidthTop,
StyleHeaderFooterPropertiesElement.DynamicSpacing,
StyleHeaderFooterPropertiesElement.Shadow,
StyleHeaderFooterPropertiesElement.Height,
StyleListLevelPropertiesElement.Height,
StyleListLevelPropertiesElement.TextAlign,
StyleListLevelPropertiesElement.Width,
StyleListLevelPropertiesElement.FontName,
StyleListLevelPropertiesElement.VerticalPos,
StyleListLevelPropertiesElement.VerticalRel,
StyleListLevelPropertiesElement.Y,
StyleListLevelPropertiesElement.ListLevelPositionAndSpaceMode,
StyleListLevelPropertiesElement.MinLabelDistance,
StyleListLevelPropertiesElement.MinLabelWidth,
StyleListLevelPropertiesElement.SpaceBefore,
StylePageLayoutPropertiesElement.BackgroundColor,
StylePageLayoutPropertiesElement.Border,
StylePageLayoutPropertiesElement.BorderBottom,
StylePageLayoutPropertiesElement.BorderLeft,
StylePageLayoutPropertiesElement.BorderRight,
StylePageLayoutPropertiesElement.BorderTop,
StylePageLayoutPropertiesElement.Margin,
StylePageLayoutPropertiesElement.MarginBottom,
StylePageLayoutPropertiesElement.MarginLeft,
StylePageLayoutPropertiesElement.MarginRight,
StylePageLayoutPropertiesElement.MarginTop,
StylePageLayoutPropertiesElement.Padding,
StylePageLayoutPropertiesElement.PaddingBottom,
StylePageLayoutPropertiesElement.PaddingLeft,
StylePageLayoutPropertiesElement.PaddingRight,
StylePageLayoutPropertiesElement.PaddingTop,
StylePageLayoutPropertiesElement.PageHeight,
StylePageLayoutPropertiesElement.PageWidth,
StylePageLayoutPropertiesElement.BorderLineWidth,
StylePageLayoutPropertiesElement.BorderLineWidthBottom,
StylePageLayoutPropertiesElement.BorderLineWidthLeft,
StylePageLayoutPropertiesElement.BorderLineWidthRight,
StylePageLayoutPropertiesElement.BorderLineWidthTop,
StylePageLayoutPropertiesElement.FirstPageNumber,
StylePageLayoutPropertiesElement.FootnoteMaxHeight,
StylePageLayoutPropertiesElement.LayoutGridBaseHeight,
StylePageLayoutPropertiesElement.LayoutGridBaseWidth,
StylePageLayoutPropertiesElement.LayoutGridColor,
StylePageLayoutPropertiesElement.LayoutGridDisplay,
StylePageLayoutPropertiesElement.LayoutGridLines,
StylePageLayoutPropertiesElement.LayoutGridMode,
StylePageLayoutPropertiesElement.LayoutGridPrint,
StylePageLayoutPropertiesElement.LayoutGridRubyBelow,
StylePageLayoutPropertiesElement.LayoutGridRubyHeight,
StylePageLayoutPropertiesElement.LayoutGridSnapTo,
StylePageLayoutPropertiesElement.LayoutGridStandardMode,
StylePageLayoutPropertiesElement.NumFormat,
StylePageLayoutPropertiesElement.NumFormat,
StylePageLayoutPropertiesElement.NumLetterSync,
StylePageLayoutPropertiesElement.NumPrefix,
StylePageLayoutPropertiesElement.NumSuffix,
StylePageLayoutPropertiesElement.PaperTrayName,
StylePageLayoutPropertiesElement.Print,
StylePageLayoutPropertiesElement.PrintOrientation,
StylePageLayoutPropertiesElement.PrintPageOrder,
StylePageLayoutPropertiesElement.RegisterTruthRefStyleName,
StylePageLayoutPropertiesElement.ScaleTo,
StylePageLayoutPropertiesElement.ScaleToPages,
StylePageLayoutPropertiesElement.Shadow,
StylePageLayoutPropertiesElement.TableCentering,
StylePageLayoutPropertiesElement.WritingMode,
StyleParagraphPropertiesElement.BackgroundColor,
StyleParagraphPropertiesElement.Border,
StyleParagraphPropertiesElement.BorderBottom,
StyleParagraphPropertiesElement.BorderLeft,
StyleParagraphPropertiesElement.BorderRight,
StyleParagraphPropertiesElement.BorderTop,
StyleParagraphPropertiesElement.BreakAfter,
StyleParagraphPropertiesElement.BreakBefore,
StyleParagraphPropertiesElement.HyphenationKeep,
StyleParagraphPropertiesElement.HyphenationLadderCount,
StyleParagraphPropertiesElement.KeepTogether,
StyleParagraphPropertiesElement.KeepWithNext,
StyleParagraphPropertiesElement.LineHeight,
StyleParagraphPropertiesElement.Margin,
StyleParagraphPropertiesElement.MarginBottom,
StyleParagraphPropertiesElement.MarginLeft,
StyleParagraphPropertiesElement.MarginRight,
StyleParagraphPropertiesElement.MarginTop,
StyleParagraphPropertiesElement.Orphans,
StyleParagraphPropertiesElement.Padding,
StyleParagraphPropertiesElement.PaddingBottom,
StyleParagraphPropertiesElement.PaddingLeft,
StyleParagraphPropertiesElement.PaddingRight,
StyleParagraphPropertiesElement.PaddingTop,
StyleParagraphPropertiesElement.TextAlign,
StyleParagraphPropertiesElement.TextAlignLast,
StyleParagraphPropertiesElement.TextIndent,
StyleParagraphPropertiesElement.Widows,
StyleParagraphPropertiesElement.AutoTextIndent,
StyleParagraphPropertiesElement.BackgroundTransparency,
StyleParagraphPropertiesElement.BorderLineWidth,
StyleParagraphPropertiesElement.BorderLineWidthBottom,
StyleParagraphPropertiesElement.BorderLineWidthLeft,
StyleParagraphPropertiesElement.BorderLineWidthRight,
StyleParagraphPropertiesElement.BorderLineWidthTop,
StyleParagraphPropertiesElement.FontIndependentLineSpacing,
StyleParagraphPropertiesElement.JoinBorder,
StyleParagraphPropertiesElement.JustifySingleWord,
StyleParagraphPropertiesElement.LineBreak,
StyleParagraphPropertiesElement.LineHeightAtLeast,
StyleParagraphPropertiesElement.LineSpacing,
StyleParagraphPropertiesElement.PageNumber,
StyleParagraphPropertiesElement.PunctuationWrap,
StyleParagraphPropertiesElement.RegisterTrue,
StyleParagraphPropertiesElement.Shadow,
StyleParagraphPropertiesElement.SnapToLayoutGrid,
StyleParagraphPropertiesElement.TabStopDistance,
StyleParagraphPropertiesElement.TextAutospace,
StyleParagraphPropertiesElement.VerticalAlign,
StyleParagraphPropertiesElement.WritingMode,
StyleParagraphPropertiesElement.WritingModeAutomatic,
StyleParagraphPropertiesElement.LineNumber,
StyleParagraphPropertiesElement.NumberLines,
StyleRubyPropertiesElement.RubyAlign,
StyleRubyPropertiesElement.RubyPosition,
StyleSectionPropertiesElement.BackgroundColor,
StyleSectionPropertiesElement.MarginLeft,
StyleSectionPropertiesElement.MarginRight,
StyleSectionPropertiesElement.Editable,
StyleSectionPropertiesElement.Protect,
StyleSectionPropertiesElement.WritingMode,
StyleSectionPropertiesElement.DontBalanceTextColumns,
StyleTableCellPropertiesElement.BackgroundColor,
StyleTableCellPropertiesElement.Border,
StyleTableCellPropertiesElement.BorderBottom,
StyleTableCellPropertiesElement.BorderLeft,
StyleTableCellPropertiesElement.BorderRight,
StyleTableCellPropertiesElement.BorderTop,
StyleTableCellPropertiesElement.Padding,
StyleTableCellPropertiesElement.PaddingBottom,
StyleTableCellPropertiesElement.PaddingLeft,
StyleTableCellPropertiesElement.PaddingRight,
StyleTableCellPropertiesElement.PaddingTop,
StyleTableCellPropertiesElement.WrapOption,
StyleTableCellPropertiesElement.BorderLineWidth,
StyleTableCellPropertiesElement.BorderLineWidthBottom,
StyleTableCellPropertiesElement.BorderLineWidthLeft,
StyleTableCellPropertiesElement.BorderLineWidthRight,
StyleTableCellPropertiesElement.BorderLineWidthTop,
StyleTableCellPropertiesElement.CellProtect,
StyleTableCellPropertiesElement.DecimalPlaces,
StyleTableCellPropertiesElement.DiagonalBlTr,
StyleTableCellPropertiesElement.DiagonalBlTrWidths,
StyleTableCellPropertiesElement.DiagonalTlBr,
StyleTableCellPropertiesElement.DiagonalTlBrWidths,
StyleTableCellPropertiesElement.Direction,
StyleTableCellPropertiesElement.GlyphOrientationVertical,
StyleTableCellPropertiesElement.PrintContent,
StyleTableCellPropertiesElement.RepeatContent,
StyleTableCellPropertiesElement.RotationAlign,
StyleTableCellPropertiesElement.RotationAngle,
StyleTableCellPropertiesElement.Shadow,
StyleTableCellPropertiesElement.ShrinkToFit,
StyleTableCellPropertiesElement.TextAlignSource,
StyleTableCellPropertiesElement.VerticalAlign,
StyleTableCellPropertiesElement.WritingMode,
StyleTableColumnPropertiesElement.BreakAfter,
StyleTableColumnPropertiesElement.BreakBefore,
StyleTableColumnPropertiesElement.ColumnWidth,
StyleTableColumnPropertiesElement.RelColumnWidth,
StyleTableColumnPropertiesElement.UseOptimalColumnWidth,
StyleTablePropertiesElement.BackgroundColor,
StyleTablePropertiesElement.BreakAfter,
StyleTablePropertiesElement.BreakBefore,
StyleTablePropertiesElement.KeepWithNext,
StyleTablePropertiesElement.Margin,
StyleTablePropertiesElement.MarginBottom,
StyleTablePropertiesElement.MarginLeft,
StyleTablePropertiesElement.MarginRight,
StyleTablePropertiesElement.MarginTop,
StyleTablePropertiesElement.MayBreakBetweenRows,
StyleTablePropertiesElement.PageNumber,
StyleTablePropertiesElement.RelWidth,
StyleTablePropertiesElement.Shadow,
StyleTablePropertiesElement.Width,
StyleTablePropertiesElement.WritingMode,
StyleTablePropertiesElement.Align,
StyleTablePropertiesElement.BorderModel,
StyleTablePropertiesElement.Display,
StyleTableRowPropertiesElement.BackgroundColor,
StyleTableRowPropertiesElement.BreakAfter,
StyleTableRowPropertiesElement.BreakBefore,
StyleTableRowPropertiesElement.KeepTogether,
StyleTableRowPropertiesElement.MinRowHeight,
StyleTableRowPropertiesElement.RowHeight,
StyleTableRowPropertiesElement.UseOptimalRowHeight,
StyleTextPropertiesElement.BackgroundColor,
StyleTextPropertiesElement.Color,
StyleTextPropertiesElement.Country,
StyleTextPropertiesElement.FontFamily,
StyleTextPropertiesElement.FontSize,
StyleTextPropertiesElement.FontStyle,
StyleTextPropertiesElement.FontVariant,
StyleTextPropertiesElement.FontWeight,
StyleTextPropertiesElement.Hyphenate,
StyleTextPropertiesElement.HyphenationPushCharCount,
StyleTextPropertiesElement.HyphenationRemainCharCount,
StyleTextPropertiesElement.Language,
StyleTextPropertiesElement.LetterSpacing,
StyleTextPropertiesElement.Script,
StyleTextPropertiesElement.TextShadow,
StyleTextPropertiesElement.TextTransform,
StyleTextPropertiesElement.CountryAsian,
StyleTextPropertiesElement.CountryComplex,
StyleTextPropertiesElement.FontCharset,
StyleTextPropertiesElement.FontCharsetAsian,
StyleTextPropertiesElement.FontCharsetComplex,
StyleTextPropertiesElement.FontFamilyAsian,
StyleTextPropertiesElement.FontFamilyComplex,
StyleTextPropertiesElement.FontFamilyGeneric,
StyleTextPropertiesElement.FontFamilyGenericAsian,
StyleTextPropertiesElement.FontFamilyGenericComplex,
StyleTextPropertiesElement.FontName,
StyleTextPropertiesElement.FontNameAsian,
StyleTextPropertiesElement.FontNameComplex,
StyleTextPropertiesElement.FontPitch,
StyleTextPropertiesElement.FontPitchAsian,
StyleTextPropertiesElement.FontPitchComplex,
StyleTextPropertiesElement.FontRelief,
StyleTextPropertiesElement.FontSizeAsian,
StyleTextPropertiesElement.FontSizeComplex,
StyleTextPropertiesElement.FontSizeRel,
StyleTextPropertiesElement.FontSizeRelAsian,
StyleTextPropertiesElement.FontSizeRelComplex,
StyleTextPropertiesElement.FontStyleAsian,
StyleTextPropertiesElement.FontStyleComplex,
StyleTextPropertiesElement.FontStyleName,
StyleTextPropertiesElement.FontStyleNameAsian,
StyleTextPropertiesElement.FontStyleNameComplex,
StyleTextPropertiesElement.FontWeightAsian,
StyleTextPropertiesElement.FontWeightComplex,
StyleTextPropertiesElement.LanguageAsian,
StyleTextPropertiesElement.LanguageComplex,
StyleTextPropertiesElement.LetterKerning,
StyleTextPropertiesElement.RfcLanguageTag,
StyleTextPropertiesElement.RfcLanguageTagAsian,
StyleTextPropertiesElement.RfcLanguageTagComplex,
StyleTextPropertiesElement.ScriptAsian,
StyleTextPropertiesElement.ScriptComplex,
StyleTextPropertiesElement.ScriptType,
StyleTextPropertiesElement.TextBlinking,
StyleTextPropertiesElement.TextCombine,
StyleTextPropertiesElement.TextCombineEndChar,
StyleTextPropertiesElement.TextCombineStartChar,
StyleTextPropertiesElement.TextEmphasize,
StyleTextPropertiesElement.TextLineThroughColor,
StyleTextPropertiesElement.TextLineThroughMode,
StyleTextPropertiesElement.TextLineThroughStyle,
StyleTextPropertiesElement.TextLineThroughText,
StyleTextPropertiesElement.TextLineThroughTextStyle,
StyleTextPropertiesElement.TextLineThroughType,
StyleTextPropertiesElement.TextLineThroughWidth,
StyleTextPropertiesElement.TextOutline,
StyleTextPropertiesElement.TextOverlineColor,
StyleTextPropertiesElement.TextOverlineMode,
StyleTextPropertiesElement.TextOverlineStyle,
StyleTextPropertiesElement.TextOverlineType,
StyleTextPropertiesElement.TextOverlineWidth,
StyleTextPropertiesElement.TextPosition,
StyleTextPropertiesElement.TextRotationAngle,
StyleTextPropertiesElement.TextRotationScale,
StyleTextPropertiesElement.TextScale,
StyleTextPropertiesElement.TextUnderlineColor,
StyleTextPropertiesElement.TextUnderlineMode,
StyleTextPropertiesElement.TextUnderlineStyle,
StyleTextPropertiesElement.TextUnderlineType,
StyleTextPropertiesElement.TextUnderlineWidth,
StyleTextPropertiesElement.UseWindowFontColor,
StyleTextPropertiesElement.Condition,
StyleTextPropertiesElement.Display,
StyleTextPropertiesElement.Display,
StyleTextPropertiesElement.Display,
});
public final static OdfStyleFamily Presentation = new OdfStyleFamily("presentation",
new OdfStyleProperty[]{
StyleChartPropertiesElement.AngleOffset,
StyleChartPropertiesElement.AutoPosition,
StyleChartPropertiesElement.AutoSize,
StyleChartPropertiesElement.AxisLabelPosition,
StyleChartPropertiesElement.AxisPosition,
StyleChartPropertiesElement.ConnectBars,
StyleChartPropertiesElement.DataLabelNumber,
StyleChartPropertiesElement.DataLabelSymbol,
StyleChartPropertiesElement.DataLabelText,
StyleChartPropertiesElement.Deep,
StyleChartPropertiesElement.DisplayLabel,
StyleChartPropertiesElement.ErrorCategory,
StyleChartPropertiesElement.ErrorLowerIndicator,
StyleChartPropertiesElement.ErrorLowerLimit,
StyleChartPropertiesElement.ErrorLowerRange,
StyleChartPropertiesElement.ErrorMargin,
StyleChartPropertiesElement.ErrorPercentage,
StyleChartPropertiesElement.ErrorUpperIndicator,
StyleChartPropertiesElement.ErrorUpperLimit,
StyleChartPropertiesElement.ErrorUpperRange,
StyleChartPropertiesElement.GapWidth,
StyleChartPropertiesElement.GroupBarsPerAxis,
StyleChartPropertiesElement.HoleSize,
StyleChartPropertiesElement.IncludeHiddenCells,
StyleChartPropertiesElement.Interpolation,
StyleChartPropertiesElement.IntervalMajor,
StyleChartPropertiesElement.IntervalMinorDivisor,
StyleChartPropertiesElement.JapaneseCandleStick,
StyleChartPropertiesElement.LabelArrangement,
StyleChartPropertiesElement.LabelPosition,
StyleChartPropertiesElement.LabelPositionNegative,
StyleChartPropertiesElement.Lines,
StyleChartPropertiesElement.LinkDataStyleToSource,
StyleChartPropertiesElement.Logarithmic,
StyleChartPropertiesElement.Maximum,
StyleChartPropertiesElement.MeanValue,
StyleChartPropertiesElement.Minimum,
StyleChartPropertiesElement.Origin,
StyleChartPropertiesElement.Overlap,
StyleChartPropertiesElement.Percentage,
StyleChartPropertiesElement.PieOffset,
StyleChartPropertiesElement.RegressionType,
StyleChartPropertiesElement.ReverseDirection,
StyleChartPropertiesElement.RightAngledAxes,
StyleChartPropertiesElement.ScaleText,
StyleChartPropertiesElement.SeriesSource,
StyleChartPropertiesElement.SolidType,
StyleChartPropertiesElement.SortByXValues,
StyleChartPropertiesElement.SplineOrder,
StyleChartPropertiesElement.SplineResolution,
StyleChartPropertiesElement.Stacked,
StyleChartPropertiesElement.SymbolHeight,
StyleChartPropertiesElement.SymbolName,
StyleChartPropertiesElement.SymbolType,
StyleChartPropertiesElement.SymbolType,
StyleChartPropertiesElement.SymbolType,
StyleChartPropertiesElement.SymbolType,
StyleChartPropertiesElement.SymbolWidth,
StyleChartPropertiesElement.TextOverlap,
StyleChartPropertiesElement.ThreeDimensional,
StyleChartPropertiesElement.TickMarkPosition,
StyleChartPropertiesElement.TickMarksMajorInner,
StyleChartPropertiesElement.TickMarksMajorOuter,
StyleChartPropertiesElement.TickMarksMinorInner,
StyleChartPropertiesElement.TickMarksMinorOuter,
StyleChartPropertiesElement.TreatEmptyCells,
StyleChartPropertiesElement.Vertical,
StyleChartPropertiesElement.Visible,
StyleChartPropertiesElement.Direction,
StyleChartPropertiesElement.RotationAngle,
StyleChartPropertiesElement.LineBreak,
StyleDrawingPagePropertiesElement.BackgroundSize,
StyleDrawingPagePropertiesElement.Fill,
StyleDrawingPagePropertiesElement.FillColor,
StyleDrawingPagePropertiesElement.FillGradientName,
StyleDrawingPagePropertiesElement.FillHatchName,
StyleDrawingPagePropertiesElement.FillHatchSolid,
StyleDrawingPagePropertiesElement.FillImageHeight,
StyleDrawingPagePropertiesElement.FillImageName,
StyleDrawingPagePropertiesElement.FillImageRefPoint,
StyleDrawingPagePropertiesElement.FillImageRefPointX,
StyleDrawingPagePropertiesElement.FillImageRefPointY,
StyleDrawingPagePropertiesElement.FillImageWidth,
StyleDrawingPagePropertiesElement.GradientStepCount,
StyleDrawingPagePropertiesElement.Opacity,
StyleDrawingPagePropertiesElement.OpacityName,
StyleDrawingPagePropertiesElement.SecondaryFillColor,
StyleDrawingPagePropertiesElement.TileRepeatOffset,
StyleDrawingPagePropertiesElement.BackgroundObjectsVisible,
StyleDrawingPagePropertiesElement.BackgroundVisible,
StyleDrawingPagePropertiesElement.DisplayDateTime,
StyleDrawingPagePropertiesElement.DisplayFooter,
StyleDrawingPagePropertiesElement.DisplayHeader,
StyleDrawingPagePropertiesElement.DisplayPageNumber,
StyleDrawingPagePropertiesElement.Duration,
StyleDrawingPagePropertiesElement.TransitionSpeed,
StyleDrawingPagePropertiesElement.TransitionStyle,
StyleDrawingPagePropertiesElement.TransitionType,
StyleDrawingPagePropertiesElement.Visibility,
StyleDrawingPagePropertiesElement.Direction,
StyleDrawingPagePropertiesElement.FadeColor,
StyleDrawingPagePropertiesElement.Subtype,
StyleDrawingPagePropertiesElement.Type,
StyleDrawingPagePropertiesElement.Repeat,
StyleDrawingPagePropertiesElement.FillRule,
StyleGraphicPropertiesElement.AmbientColor,
StyleGraphicPropertiesElement.BackScale,
StyleGraphicPropertiesElement.BackfaceCulling,
StyleGraphicPropertiesElement.CloseBack,
StyleGraphicPropertiesElement.CloseFront,
StyleGraphicPropertiesElement.Depth,
StyleGraphicPropertiesElement.DiffuseColor,
StyleGraphicPropertiesElement.EdgeRounding,
StyleGraphicPropertiesElement.EdgeRoundingMode,
StyleGraphicPropertiesElement.EmissiveColor,
StyleGraphicPropertiesElement.EndAngle,
StyleGraphicPropertiesElement.HorizontalSegments,
StyleGraphicPropertiesElement.LightingMode,
StyleGraphicPropertiesElement.NormalsDirection,
StyleGraphicPropertiesElement.NormalsKind,
StyleGraphicPropertiesElement.Dr3dShadow,
StyleGraphicPropertiesElement.Shininess,
StyleGraphicPropertiesElement.SpecularColor,
StyleGraphicPropertiesElement.TextureFilter,
StyleGraphicPropertiesElement.TextureGenerationModeX,
StyleGraphicPropertiesElement.TextureGenerationModeY,
StyleGraphicPropertiesElement.TextureKind,
StyleGraphicPropertiesElement.TextureMode,
StyleGraphicPropertiesElement.VerticalSegments,
StyleGraphicPropertiesElement.AutoGrowHeight,
StyleGraphicPropertiesElement.AutoGrowWidth,
StyleGraphicPropertiesElement.Blue,
StyleGraphicPropertiesElement.CaptionAngle,
StyleGraphicPropertiesElement.CaptionAngleType,
StyleGraphicPropertiesElement.CaptionEscape,
StyleGraphicPropertiesElement.CaptionEscapeDirection,
StyleGraphicPropertiesElement.CaptionFitLineLength,
StyleGraphicPropertiesElement.CaptionGap,
StyleGraphicPropertiesElement.CaptionLineLength,
StyleGraphicPropertiesElement.CaptionType,
StyleGraphicPropertiesElement.ColorInversion,
StyleGraphicPropertiesElement.ColorMode,
StyleGraphicPropertiesElement.Contrast,
StyleGraphicPropertiesElement.DecimalPlaces,
StyleGraphicPropertiesElement.DrawAspect,
StyleGraphicPropertiesElement.EndGuide,
StyleGraphicPropertiesElement.EndLineSpacingHorizontal,
StyleGraphicPropertiesElement.EndLineSpacingVertical,
StyleGraphicPropertiesElement.Fill,
StyleGraphicPropertiesElement.FillColor,
StyleGraphicPropertiesElement.FillGradientName,
StyleGraphicPropertiesElement.FillHatchName,
StyleGraphicPropertiesElement.FillHatchSolid,
StyleGraphicPropertiesElement.FillImageHeight,
StyleGraphicPropertiesElement.FillImageName,
StyleGraphicPropertiesElement.FillImageRefPoint,
StyleGraphicPropertiesElement.FillImageRefPointX,
StyleGraphicPropertiesElement.FillImageRefPointY,
StyleGraphicPropertiesElement.FillImageWidth,
StyleGraphicPropertiesElement.FitToContour,
StyleGraphicPropertiesElement.FitToSize,
StyleGraphicPropertiesElement.FrameDisplayBorder,
StyleGraphicPropertiesElement.FrameDisplayScrollbar,
StyleGraphicPropertiesElement.FrameMarginHorizontal,
StyleGraphicPropertiesElement.FrameMarginVertical,
StyleGraphicPropertiesElement.Gamma,
StyleGraphicPropertiesElement.GradientStepCount,
StyleGraphicPropertiesElement.Green,
StyleGraphicPropertiesElement.GuideDistance,
StyleGraphicPropertiesElement.GuideOverhang,
StyleGraphicPropertiesElement.ImageOpacity,
StyleGraphicPropertiesElement.LineDistance,
StyleGraphicPropertiesElement.Luminance,
StyleGraphicPropertiesElement.MarkerEnd,
StyleGraphicPropertiesElement.MarkerEndCenter,
StyleGraphicPropertiesElement.MarkerEndWidth,
StyleGraphicPropertiesElement.MarkerStart,
StyleGraphicPropertiesElement.MarkerStartCenter,
StyleGraphicPropertiesElement.MarkerStartWidth,
StyleGraphicPropertiesElement.MeasureAlign,
StyleGraphicPropertiesElement.MeasureVerticalAlign,
StyleGraphicPropertiesElement.OleDrawAspect,
StyleGraphicPropertiesElement.Opacity,
StyleGraphicPropertiesElement.OpacityName,
StyleGraphicPropertiesElement.Parallel,
StyleGraphicPropertiesElement.Placing,
StyleGraphicPropertiesElement.Red,
StyleGraphicPropertiesElement.SecondaryFillColor,
StyleGraphicPropertiesElement.DrawShadow,
StyleGraphicPropertiesElement.ShadowColor,
StyleGraphicPropertiesElement.ShadowOffsetX,
StyleGraphicPropertiesElement.ShadowOffsetY,
StyleGraphicPropertiesElement.ShadowOpacity,
StyleGraphicPropertiesElement.ShowUnit,
StyleGraphicPropertiesElement.StartGuide,
StyleGraphicPropertiesElement.StartLineSpacingHorizontal,
StyleGraphicPropertiesElement.StartLineSpacingVertical,
StyleGraphicPropertiesElement.Stroke,
StyleGraphicPropertiesElement.StrokeDash,
StyleGraphicPropertiesElement.StrokeDashNames,
StyleGraphicPropertiesElement.StrokeLinejoin,
StyleGraphicPropertiesElement.SymbolColor,
StyleGraphicPropertiesElement.TextareaHorizontalAlign,
StyleGraphicPropertiesElement.TextareaVerticalAlign,
StyleGraphicPropertiesElement.TileRepeatOffset,
StyleGraphicPropertiesElement.Unit,
StyleGraphicPropertiesElement.VisibleAreaHeight,
StyleGraphicPropertiesElement.VisibleAreaLeft,
StyleGraphicPropertiesElement.VisibleAreaTop,
StyleGraphicPropertiesElement.VisibleAreaWidth,
StyleGraphicPropertiesElement.WrapInfluenceOnPosition,
StyleGraphicPropertiesElement.BackgroundColor,
StyleGraphicPropertiesElement.Border,
StyleGraphicPropertiesElement.BorderBottom,
StyleGraphicPropertiesElement.BorderLeft,
StyleGraphicPropertiesElement.BorderRight,
StyleGraphicPropertiesElement.BorderTop,
StyleGraphicPropertiesElement.Clip,
StyleGraphicPropertiesElement.Margin,
StyleGraphicPropertiesElement.MarginBottom,
StyleGraphicPropertiesElement.MarginLeft,
StyleGraphicPropertiesElement.MarginRight,
StyleGraphicPropertiesElement.MarginTop,
StyleGraphicPropertiesElement.MaxHeight,
StyleGraphicPropertiesElement.MaxWidth,
StyleGraphicPropertiesElement.MinHeight,
StyleGraphicPropertiesElement.MinWidth,
StyleGraphicPropertiesElement.Padding,
StyleGraphicPropertiesElement.PaddingBottom,
StyleGraphicPropertiesElement.PaddingLeft,
StyleGraphicPropertiesElement.PaddingRight,
StyleGraphicPropertiesElement.PaddingTop,
StyleGraphicPropertiesElement.WrapOption,
StyleGraphicPropertiesElement.BackgroundTransparency,
StyleGraphicPropertiesElement.BorderLineWidth,
StyleGraphicPropertiesElement.BorderLineWidthBottom,
StyleGraphicPropertiesElement.BorderLineWidthLeft,
StyleGraphicPropertiesElement.BorderLineWidthRight,
StyleGraphicPropertiesElement.BorderLineWidthTop,
StyleGraphicPropertiesElement.Editable,
StyleGraphicPropertiesElement.FlowWithText,
StyleGraphicPropertiesElement.HorizontalPos,
StyleGraphicPropertiesElement.HorizontalRel,
StyleGraphicPropertiesElement.Mirror,
StyleGraphicPropertiesElement.NumberWrappedParagraphs,
StyleGraphicPropertiesElement.OverflowBehavior,
StyleGraphicPropertiesElement.PrintContent,
StyleGraphicPropertiesElement.Protect,
StyleGraphicPropertiesElement.RelHeight,
StyleGraphicPropertiesElement.RelWidth,
StyleGraphicPropertiesElement.Repeat,
StyleGraphicPropertiesElement.RunThrough,
StyleGraphicPropertiesElement.StyleShadow,
StyleGraphicPropertiesElement.ShrinkToFit,
StyleGraphicPropertiesElement.VerticalPos,
StyleGraphicPropertiesElement.VerticalRel,
StyleGraphicPropertiesElement.Wrap,
StyleGraphicPropertiesElement.WrapContour,
StyleGraphicPropertiesElement.WrapContourMode,
StyleGraphicPropertiesElement.WrapDynamicThreshold,
StyleGraphicPropertiesElement.WritingMode,
StyleGraphicPropertiesElement.FillRule,
StyleGraphicPropertiesElement.Height,
StyleGraphicPropertiesElement.StrokeColor,
StyleGraphicPropertiesElement.StrokeLinecap,
StyleGraphicPropertiesElement.StrokeOpacity,
StyleGraphicPropertiesElement.StrokeWidth,
StyleGraphicPropertiesElement.Width,
StyleGraphicPropertiesElement.X,
StyleGraphicPropertiesElement.Y,
StyleGraphicPropertiesElement.AnchorPageNumber,
StyleGraphicPropertiesElement.AnchorType,
StyleGraphicPropertiesElement.Animation,
StyleGraphicPropertiesElement.AnimationDelay,
StyleGraphicPropertiesElement.AnimationDirection,
StyleGraphicPropertiesElement.AnimationRepeat,
StyleGraphicPropertiesElement.AnimationStartInside,
StyleGraphicPropertiesElement.AnimationSteps,
StyleGraphicPropertiesElement.AnimationStopInside,
StyleHeaderFooterPropertiesElement.BackgroundColor,
StyleHeaderFooterPropertiesElement.Border,
StyleHeaderFooterPropertiesElement.BorderBottom,
StyleHeaderFooterPropertiesElement.BorderLeft,
StyleHeaderFooterPropertiesElement.BorderRight,
StyleHeaderFooterPropertiesElement.BorderTop,
StyleHeaderFooterPropertiesElement.Margin,
StyleHeaderFooterPropertiesElement.MarginBottom,
StyleHeaderFooterPropertiesElement.MarginLeft,
StyleHeaderFooterPropertiesElement.MarginRight,
StyleHeaderFooterPropertiesElement.MarginTop,
StyleHeaderFooterPropertiesElement.MinHeight,
StyleHeaderFooterPropertiesElement.Padding,
StyleHeaderFooterPropertiesElement.PaddingBottom,
StyleHeaderFooterPropertiesElement.PaddingLeft,
StyleHeaderFooterPropertiesElement.PaddingRight,
StyleHeaderFooterPropertiesElement.PaddingTop,
StyleHeaderFooterPropertiesElement.BorderLineWidth,
StyleHeaderFooterPropertiesElement.BorderLineWidthBottom,
StyleHeaderFooterPropertiesElement.BorderLineWidthLeft,
StyleHeaderFooterPropertiesElement.BorderLineWidthRight,
StyleHeaderFooterPropertiesElement.BorderLineWidthTop,
StyleHeaderFooterPropertiesElement.DynamicSpacing,
StyleHeaderFooterPropertiesElement.Shadow,
StyleHeaderFooterPropertiesElement.Height,
StyleListLevelPropertiesElement.Height,
StyleListLevelPropertiesElement.TextAlign,
StyleListLevelPropertiesElement.Width,
StyleListLevelPropertiesElement.FontName,
StyleListLevelPropertiesElement.VerticalPos,
StyleListLevelPropertiesElement.VerticalRel,
StyleListLevelPropertiesElement.Y,
StyleListLevelPropertiesElement.ListLevelPositionAndSpaceMode,
StyleListLevelPropertiesElement.MinLabelDistance,
StyleListLevelPropertiesElement.MinLabelWidth,
StyleListLevelPropertiesElement.SpaceBefore,
StylePageLayoutPropertiesElement.BackgroundColor,
StylePageLayoutPropertiesElement.Border,
StylePageLayoutPropertiesElement.BorderBottom,
StylePageLayoutPropertiesElement.BorderLeft,
StylePageLayoutPropertiesElement.BorderRight,
StylePageLayoutPropertiesElement.BorderTop,
StylePageLayoutPropertiesElement.Margin,
StylePageLayoutPropertiesElement.MarginBottom,
StylePageLayoutPropertiesElement.MarginLeft,
StylePageLayoutPropertiesElement.MarginRight,
StylePageLayoutPropertiesElement.MarginTop,
StylePageLayoutPropertiesElement.Padding,
StylePageLayoutPropertiesElement.PaddingBottom,
StylePageLayoutPropertiesElement.PaddingLeft,
StylePageLayoutPropertiesElement.PaddingRight,
StylePageLayoutPropertiesElement.PaddingTop,
StylePageLayoutPropertiesElement.PageHeight,
StylePageLayoutPropertiesElement.PageWidth,
StylePageLayoutPropertiesElement.BorderLineWidth,
StylePageLayoutPropertiesElement.BorderLineWidthBottom,
StylePageLayoutPropertiesElement.BorderLineWidthLeft,
StylePageLayoutPropertiesElement.BorderLineWidthRight,
StylePageLayoutPropertiesElement.BorderLineWidthTop,
StylePageLayoutPropertiesElement.FirstPageNumber,
StylePageLayoutPropertiesElement.FootnoteMaxHeight,
StylePageLayoutPropertiesElement.LayoutGridBaseHeight,
StylePageLayoutPropertiesElement.LayoutGridBaseWidth,
StylePageLayoutPropertiesElement.LayoutGridColor,
StylePageLayoutPropertiesElement.LayoutGridDisplay,
StylePageLayoutPropertiesElement.LayoutGridLines,
StylePageLayoutPropertiesElement.LayoutGridMode,
StylePageLayoutPropertiesElement.LayoutGridPrint,
StylePageLayoutPropertiesElement.LayoutGridRubyBelow,
StylePageLayoutPropertiesElement.LayoutGridRubyHeight,
StylePageLayoutPropertiesElement.LayoutGridSnapTo,
StylePageLayoutPropertiesElement.LayoutGridStandardMode,
StylePageLayoutPropertiesElement.NumFormat,
StylePageLayoutPropertiesElement.NumFormat,
StylePageLayoutPropertiesElement.NumLetterSync,
StylePageLayoutPropertiesElement.NumPrefix,
StylePageLayoutPropertiesElement.NumSuffix,
StylePageLayoutPropertiesElement.PaperTrayName,
StylePageLayoutPropertiesElement.Print,
StylePageLayoutPropertiesElement.PrintOrientation,
StylePageLayoutPropertiesElement.PrintPageOrder,
StylePageLayoutPropertiesElement.RegisterTruthRefStyleName,
StylePageLayoutPropertiesElement.ScaleTo,
StylePageLayoutPropertiesElement.ScaleToPages,
StylePageLayoutPropertiesElement.Shadow,
StylePageLayoutPropertiesElement.TableCentering,
StylePageLayoutPropertiesElement.WritingMode,
StyleParagraphPropertiesElement.BackgroundColor,
StyleParagraphPropertiesElement.Border,
StyleParagraphPropertiesElement.BorderBottom,
StyleParagraphPropertiesElement.BorderLeft,
StyleParagraphPropertiesElement.BorderRight,
StyleParagraphPropertiesElement.BorderTop,
StyleParagraphPropertiesElement.BreakAfter,
StyleParagraphPropertiesElement.BreakBefore,
StyleParagraphPropertiesElement.HyphenationKeep,
StyleParagraphPropertiesElement.HyphenationLadderCount,
StyleParagraphPropertiesElement.KeepTogether,
StyleParagraphPropertiesElement.KeepWithNext,
StyleParagraphPropertiesElement.LineHeight,
StyleParagraphPropertiesElement.Margin,
StyleParagraphPropertiesElement.MarginBottom,
StyleParagraphPropertiesElement.MarginLeft,
StyleParagraphPropertiesElement.MarginRight,
StyleParagraphPropertiesElement.MarginTop,
StyleParagraphPropertiesElement.Orphans,
StyleParagraphPropertiesElement.Padding,
StyleParagraphPropertiesElement.PaddingBottom,
StyleParagraphPropertiesElement.PaddingLeft,
StyleParagraphPropertiesElement.PaddingRight,
StyleParagraphPropertiesElement.PaddingTop,
StyleParagraphPropertiesElement.TextAlign,
StyleParagraphPropertiesElement.TextAlignLast,
StyleParagraphPropertiesElement.TextIndent,
StyleParagraphPropertiesElement.Widows,
StyleParagraphPropertiesElement.AutoTextIndent,
StyleParagraphPropertiesElement.BackgroundTransparency,
StyleParagraphPropertiesElement.BorderLineWidth,
StyleParagraphPropertiesElement.BorderLineWidthBottom,
StyleParagraphPropertiesElement.BorderLineWidthLeft,
StyleParagraphPropertiesElement.BorderLineWidthRight,
StyleParagraphPropertiesElement.BorderLineWidthTop,
StyleParagraphPropertiesElement.FontIndependentLineSpacing,
StyleParagraphPropertiesElement.JoinBorder,
StyleParagraphPropertiesElement.JustifySingleWord,
StyleParagraphPropertiesElement.LineBreak,
StyleParagraphPropertiesElement.LineHeightAtLeast,
StyleParagraphPropertiesElement.LineSpacing,
StyleParagraphPropertiesElement.PageNumber,
StyleParagraphPropertiesElement.PunctuationWrap,
StyleParagraphPropertiesElement.RegisterTrue,
StyleParagraphPropertiesElement.Shadow,
StyleParagraphPropertiesElement.SnapToLayoutGrid,
StyleParagraphPropertiesElement.TabStopDistance,
StyleParagraphPropertiesElement.TextAutospace,
StyleParagraphPropertiesElement.VerticalAlign,
StyleParagraphPropertiesElement.WritingMode,
StyleParagraphPropertiesElement.WritingModeAutomatic,
StyleParagraphPropertiesElement.LineNumber,
StyleParagraphPropertiesElement.NumberLines,
StyleRubyPropertiesElement.RubyAlign,
StyleRubyPropertiesElement.RubyPosition,
StyleSectionPropertiesElement.BackgroundColor,
StyleSectionPropertiesElement.MarginLeft,
StyleSectionPropertiesElement.MarginRight,
StyleSectionPropertiesElement.Editable,
StyleSectionPropertiesElement.Protect,
StyleSectionPropertiesElement.WritingMode,
StyleSectionPropertiesElement.DontBalanceTextColumns,
StyleTableCellPropertiesElement.BackgroundColor,
StyleTableCellPropertiesElement.Border,
StyleTableCellPropertiesElement.BorderBottom,
StyleTableCellPropertiesElement.BorderLeft,
StyleTableCellPropertiesElement.BorderRight,
StyleTableCellPropertiesElement.BorderTop,
StyleTableCellPropertiesElement.Padding,
StyleTableCellPropertiesElement.PaddingBottom,
StyleTableCellPropertiesElement.PaddingLeft,
StyleTableCellPropertiesElement.PaddingRight,
StyleTableCellPropertiesElement.PaddingTop,
StyleTableCellPropertiesElement.WrapOption,
StyleTableCellPropertiesElement.BorderLineWidth,
StyleTableCellPropertiesElement.BorderLineWidthBottom,
StyleTableCellPropertiesElement.BorderLineWidthLeft,
StyleTableCellPropertiesElement.BorderLineWidthRight,
StyleTableCellPropertiesElement.BorderLineWidthTop,
StyleTableCellPropertiesElement.CellProtect,
StyleTableCellPropertiesElement.DecimalPlaces,
StyleTableCellPropertiesElement.DiagonalBlTr,
StyleTableCellPropertiesElement.DiagonalBlTrWidths,
StyleTableCellPropertiesElement.DiagonalTlBr,
StyleTableCellPropertiesElement.DiagonalTlBrWidths,
StyleTableCellPropertiesElement.Direction,
StyleTableCellPropertiesElement.GlyphOrientationVertical,
StyleTableCellPropertiesElement.PrintContent,
StyleTableCellPropertiesElement.RepeatContent,
StyleTableCellPropertiesElement.RotationAlign,
StyleTableCellPropertiesElement.RotationAngle,
StyleTableCellPropertiesElement.Shadow,
StyleTableCellPropertiesElement.ShrinkToFit,
StyleTableCellPropertiesElement.TextAlignSource,
StyleTableCellPropertiesElement.VerticalAlign,
StyleTableCellPropertiesElement.WritingMode,
StyleTableColumnPropertiesElement.BreakAfter,
StyleTableColumnPropertiesElement.BreakBefore,
StyleTableColumnPropertiesElement.ColumnWidth,
StyleTableColumnPropertiesElement.RelColumnWidth,
StyleTableColumnPropertiesElement.UseOptimalColumnWidth,
StyleTablePropertiesElement.BackgroundColor,
StyleTablePropertiesElement.BreakAfter,
StyleTablePropertiesElement.BreakBefore,
StyleTablePropertiesElement.KeepWithNext,
StyleTablePropertiesElement.Margin,
StyleTablePropertiesElement.MarginBottom,
StyleTablePropertiesElement.MarginLeft,
StyleTablePropertiesElement.MarginRight,
StyleTablePropertiesElement.MarginTop,
StyleTablePropertiesElement.MayBreakBetweenRows,
StyleTablePropertiesElement.PageNumber,
StyleTablePropertiesElement.RelWidth,
StyleTablePropertiesElement.Shadow,
StyleTablePropertiesElement.Width,
StyleTablePropertiesElement.WritingMode,
StyleTablePropertiesElement.Align,
StyleTablePropertiesElement.BorderModel,
StyleTablePropertiesElement.Display,
StyleTableRowPropertiesElement.BackgroundColor,
StyleTableRowPropertiesElement.BreakAfter,
StyleTableRowPropertiesElement.BreakBefore,
StyleTableRowPropertiesElement.KeepTogether,
StyleTableRowPropertiesElement.MinRowHeight,
StyleTableRowPropertiesElement.RowHeight,
StyleTableRowPropertiesElement.UseOptimalRowHeight,
StyleTextPropertiesElement.BackgroundColor,
StyleTextPropertiesElement.Color,
StyleTextPropertiesElement.Country,
StyleTextPropertiesElement.FontFamily,
StyleTextPropertiesElement.FontSize,
StyleTextPropertiesElement.FontStyle,
StyleTextPropertiesElement.FontVariant,
StyleTextPropertiesElement.FontWeight,
StyleTextPropertiesElement.Hyphenate,
StyleTextPropertiesElement.HyphenationPushCharCount,
StyleTextPropertiesElement.HyphenationRemainCharCount,
StyleTextPropertiesElement.Language,
StyleTextPropertiesElement.LetterSpacing,
StyleTextPropertiesElement.Script,
StyleTextPropertiesElement.TextShadow,
StyleTextPropertiesElement.TextTransform,
StyleTextPropertiesElement.CountryAsian,
StyleTextPropertiesElement.CountryComplex,
StyleTextPropertiesElement.FontCharset,
StyleTextPropertiesElement.FontCharsetAsian,
StyleTextPropertiesElement.FontCharsetComplex,
StyleTextPropertiesElement.FontFamilyAsian,
StyleTextPropertiesElement.FontFamilyComplex,
StyleTextPropertiesElement.FontFamilyGeneric,
StyleTextPropertiesElement.FontFamilyGenericAsian,
StyleTextPropertiesElement.FontFamilyGenericComplex,
StyleTextPropertiesElement.FontName,
StyleTextPropertiesElement.FontNameAsian,
StyleTextPropertiesElement.FontNameComplex,
StyleTextPropertiesElement.FontPitch,
StyleTextPropertiesElement.FontPitchAsian,
StyleTextPropertiesElement.FontPitchComplex,
StyleTextPropertiesElement.FontRelief,
StyleTextPropertiesElement.FontSizeAsian,
StyleTextPropertiesElement.FontSizeComplex,
StyleTextPropertiesElement.FontSizeRel,
StyleTextPropertiesElement.FontSizeRelAsian,
StyleTextPropertiesElement.FontSizeRelComplex,
StyleTextPropertiesElement.FontStyleAsian,
StyleTextPropertiesElement.FontStyleComplex,
StyleTextPropertiesElement.FontStyleName,
StyleTextPropertiesElement.FontStyleNameAsian,
StyleTextPropertiesElement.FontStyleNameComplex,
StyleTextPropertiesElement.FontWeightAsian,
StyleTextPropertiesElement.FontWeightComplex,
StyleTextPropertiesElement.LanguageAsian,
StyleTextPropertiesElement.LanguageComplex,
StyleTextPropertiesElement.LetterKerning,
StyleTextPropertiesElement.RfcLanguageTag,
StyleTextPropertiesElement.RfcLanguageTagAsian,
StyleTextPropertiesElement.RfcLanguageTagComplex,
StyleTextPropertiesElement.ScriptAsian,
StyleTextPropertiesElement.ScriptComplex,
StyleTextPropertiesElement.ScriptType,
StyleTextPropertiesElement.TextBlinking,
StyleTextPropertiesElement.TextCombine,
StyleTextPropertiesElement.TextCombineEndChar,
StyleTextPropertiesElement.TextCombineStartChar,
StyleTextPropertiesElement.TextEmphasize,
StyleTextPropertiesElement.TextLineThroughColor,
StyleTextPropertiesElement.TextLineThroughMode,
StyleTextPropertiesElement.TextLineThroughStyle,
StyleTextPropertiesElement.TextLineThroughText,
StyleTextPropertiesElement.TextLineThroughTextStyle,
StyleTextPropertiesElement.TextLineThroughType,
StyleTextPropertiesElement.TextLineThroughWidth,
StyleTextPropertiesElement.TextOutline,
StyleTextPropertiesElement.TextOverlineColor,
StyleTextPropertiesElement.TextOverlineMode,
StyleTextPropertiesElement.TextOverlineStyle,
StyleTextPropertiesElement.TextOverlineType,
StyleTextPropertiesElement.TextOverlineWidth,
StyleTextPropertiesElement.TextPosition,
StyleTextPropertiesElement.TextRotationAngle,
StyleTextPropertiesElement.TextRotationScale,
StyleTextPropertiesElement.TextScale,
StyleTextPropertiesElement.TextUnderlineColor,
StyleTextPropertiesElement.TextUnderlineMode,
StyleTextPropertiesElement.TextUnderlineStyle,
StyleTextPropertiesElement.TextUnderlineType,
StyleTextPropertiesElement.TextUnderlineWidth,
StyleTextPropertiesElement.UseWindowFontColor,
StyleTextPropertiesElement.Condition,
StyleTextPropertiesElement.Display,
StyleTextPropertiesElement.Display,
StyleTextPropertiesElement.Display,
});
public final static OdfStyleFamily Table = new OdfStyleFamily("table",
new OdfStyleProperty[]{
StyleChartPropertiesElement.AngleOffset,
StyleChartPropertiesElement.AutoPosition,
StyleChartPropertiesElement.AutoSize,
StyleChartPropertiesElement.AxisLabelPosition,
StyleChartPropertiesElement.AxisPosition,
StyleChartPropertiesElement.ConnectBars,
StyleChartPropertiesElement.DataLabelNumber,
StyleChartPropertiesElement.DataLabelSymbol,
StyleChartPropertiesElement.DataLabelText,
StyleChartPropertiesElement.Deep,
StyleChartPropertiesElement.DisplayLabel,
StyleChartPropertiesElement.ErrorCategory,
StyleChartPropertiesElement.ErrorLowerIndicator,
StyleChartPropertiesElement.ErrorLowerLimit,
StyleChartPropertiesElement.ErrorLowerRange,
StyleChartPropertiesElement.ErrorMargin,
StyleChartPropertiesElement.ErrorPercentage,
StyleChartPropertiesElement.ErrorUpperIndicator,
StyleChartPropertiesElement.ErrorUpperLimit,
StyleChartPropertiesElement.ErrorUpperRange,
StyleChartPropertiesElement.GapWidth,
StyleChartPropertiesElement.GroupBarsPerAxis,
StyleChartPropertiesElement.HoleSize,
StyleChartPropertiesElement.IncludeHiddenCells,
StyleChartPropertiesElement.Interpolation,
StyleChartPropertiesElement.IntervalMajor,
StyleChartPropertiesElement.IntervalMinorDivisor,
StyleChartPropertiesElement.JapaneseCandleStick,
StyleChartPropertiesElement.LabelArrangement,
StyleChartPropertiesElement.LabelPosition,
StyleChartPropertiesElement.LabelPositionNegative,
StyleChartPropertiesElement.Lines,
StyleChartPropertiesElement.LinkDataStyleToSource,
StyleChartPropertiesElement.Logarithmic,
StyleChartPropertiesElement.Maximum,
StyleChartPropertiesElement.MeanValue,
StyleChartPropertiesElement.Minimum,
StyleChartPropertiesElement.Origin,
StyleChartPropertiesElement.Overlap,
StyleChartPropertiesElement.Percentage,
StyleChartPropertiesElement.PieOffset,
StyleChartPropertiesElement.RegressionType,
StyleChartPropertiesElement.ReverseDirection,
StyleChartPropertiesElement.RightAngledAxes,
StyleChartPropertiesElement.ScaleText,
StyleChartPropertiesElement.SeriesSource,
StyleChartPropertiesElement.SolidType,
StyleChartPropertiesElement.SortByXValues,
StyleChartPropertiesElement.SplineOrder,
StyleChartPropertiesElement.SplineResolution,
StyleChartPropertiesElement.Stacked,
StyleChartPropertiesElement.SymbolHeight,
StyleChartPropertiesElement.SymbolName,
StyleChartPropertiesElement.SymbolType,
StyleChartPropertiesElement.SymbolType,
StyleChartPropertiesElement.SymbolType,
StyleChartPropertiesElement.SymbolType,
StyleChartPropertiesElement.SymbolWidth,
StyleChartPropertiesElement.TextOverlap,
StyleChartPropertiesElement.ThreeDimensional,
StyleChartPropertiesElement.TickMarkPosition,
StyleChartPropertiesElement.TickMarksMajorInner,
StyleChartPropertiesElement.TickMarksMajorOuter,
StyleChartPropertiesElement.TickMarksMinorInner,
StyleChartPropertiesElement.TickMarksMinorOuter,
StyleChartPropertiesElement.TreatEmptyCells,
StyleChartPropertiesElement.Vertical,
StyleChartPropertiesElement.Visible,
StyleChartPropertiesElement.Direction,
StyleChartPropertiesElement.RotationAngle,
StyleChartPropertiesElement.LineBreak,
StyleDrawingPagePropertiesElement.BackgroundSize,
StyleDrawingPagePropertiesElement.Fill,
StyleDrawingPagePropertiesElement.FillColor,
StyleDrawingPagePropertiesElement.FillGradientName,
StyleDrawingPagePropertiesElement.FillHatchName,
StyleDrawingPagePropertiesElement.FillHatchSolid,
StyleDrawingPagePropertiesElement.FillImageHeight,
StyleDrawingPagePropertiesElement.FillImageName,
StyleDrawingPagePropertiesElement.FillImageRefPoint,
StyleDrawingPagePropertiesElement.FillImageRefPointX,
StyleDrawingPagePropertiesElement.FillImageRefPointY,
StyleDrawingPagePropertiesElement.FillImageWidth,
StyleDrawingPagePropertiesElement.GradientStepCount,
StyleDrawingPagePropertiesElement.Opacity,
StyleDrawingPagePropertiesElement.OpacityName,
StyleDrawingPagePropertiesElement.SecondaryFillColor,
StyleDrawingPagePropertiesElement.TileRepeatOffset,
StyleDrawingPagePropertiesElement.BackgroundObjectsVisible,
StyleDrawingPagePropertiesElement.BackgroundVisible,
StyleDrawingPagePropertiesElement.DisplayDateTime,
StyleDrawingPagePropertiesElement.DisplayFooter,
StyleDrawingPagePropertiesElement.DisplayHeader,
StyleDrawingPagePropertiesElement.DisplayPageNumber,
StyleDrawingPagePropertiesElement.Duration,
StyleDrawingPagePropertiesElement.TransitionSpeed,
StyleDrawingPagePropertiesElement.TransitionStyle,
StyleDrawingPagePropertiesElement.TransitionType,
StyleDrawingPagePropertiesElement.Visibility,
StyleDrawingPagePropertiesElement.Direction,
StyleDrawingPagePropertiesElement.FadeColor,
StyleDrawingPagePropertiesElement.Subtype,
StyleDrawingPagePropertiesElement.Type,
StyleDrawingPagePropertiesElement.Repeat,
StyleDrawingPagePropertiesElement.FillRule,
StyleGraphicPropertiesElement.AmbientColor,
StyleGraphicPropertiesElement.BackScale,
StyleGraphicPropertiesElement.BackfaceCulling,
StyleGraphicPropertiesElement.CloseBack,
StyleGraphicPropertiesElement.CloseFront,
StyleGraphicPropertiesElement.Depth,
StyleGraphicPropertiesElement.DiffuseColor,
StyleGraphicPropertiesElement.EdgeRounding,
StyleGraphicPropertiesElement.EdgeRoundingMode,
StyleGraphicPropertiesElement.EmissiveColor,
StyleGraphicPropertiesElement.EndAngle,
StyleGraphicPropertiesElement.HorizontalSegments,
StyleGraphicPropertiesElement.LightingMode,
StyleGraphicPropertiesElement.NormalsDirection,
StyleGraphicPropertiesElement.NormalsKind,
StyleGraphicPropertiesElement.Dr3dShadow,
StyleGraphicPropertiesElement.Shininess,
StyleGraphicPropertiesElement.SpecularColor,
StyleGraphicPropertiesElement.TextureFilter,
StyleGraphicPropertiesElement.TextureGenerationModeX,
StyleGraphicPropertiesElement.TextureGenerationModeY,
StyleGraphicPropertiesElement.TextureKind,
StyleGraphicPropertiesElement.TextureMode,
StyleGraphicPropertiesElement.VerticalSegments,
StyleGraphicPropertiesElement.AutoGrowHeight,
StyleGraphicPropertiesElement.AutoGrowWidth,
StyleGraphicPropertiesElement.Blue,
StyleGraphicPropertiesElement.CaptionAngle,
StyleGraphicPropertiesElement.CaptionAngleType,
StyleGraphicPropertiesElement.CaptionEscape,
StyleGraphicPropertiesElement.CaptionEscapeDirection,
StyleGraphicPropertiesElement.CaptionFitLineLength,
StyleGraphicPropertiesElement.CaptionGap,
StyleGraphicPropertiesElement.CaptionLineLength,
StyleGraphicPropertiesElement.CaptionType,
StyleGraphicPropertiesElement.ColorInversion,
StyleGraphicPropertiesElement.ColorMode,
StyleGraphicPropertiesElement.Contrast,
StyleGraphicPropertiesElement.DecimalPlaces,
StyleGraphicPropertiesElement.DrawAspect,
StyleGraphicPropertiesElement.EndGuide,
StyleGraphicPropertiesElement.EndLineSpacingHorizontal,
StyleGraphicPropertiesElement.EndLineSpacingVertical,
StyleGraphicPropertiesElement.Fill,
StyleGraphicPropertiesElement.FillColor,
StyleGraphicPropertiesElement.FillGradientName,
StyleGraphicPropertiesElement.FillHatchName,
StyleGraphicPropertiesElement.FillHatchSolid,
StyleGraphicPropertiesElement.FillImageHeight,
StyleGraphicPropertiesElement.FillImageName,
StyleGraphicPropertiesElement.FillImageRefPoint,
StyleGraphicPropertiesElement.FillImageRefPointX,
StyleGraphicPropertiesElement.FillImageRefPointY,
StyleGraphicPropertiesElement.FillImageWidth,
StyleGraphicPropertiesElement.FitToContour,
StyleGraphicPropertiesElement.FitToSize,
StyleGraphicPropertiesElement.FrameDisplayBorder,
StyleGraphicPropertiesElement.FrameDisplayScrollbar,
StyleGraphicPropertiesElement.FrameMarginHorizontal,
StyleGraphicPropertiesElement.FrameMarginVertical,
StyleGraphicPropertiesElement.Gamma,
StyleGraphicPropertiesElement.GradientStepCount,
StyleGraphicPropertiesElement.Green,
StyleGraphicPropertiesElement.GuideDistance,
StyleGraphicPropertiesElement.GuideOverhang,
StyleGraphicPropertiesElement.ImageOpacity,
StyleGraphicPropertiesElement.LineDistance,
StyleGraphicPropertiesElement.Luminance,
StyleGraphicPropertiesElement.MarkerEnd,
StyleGraphicPropertiesElement.MarkerEndCenter,
StyleGraphicPropertiesElement.MarkerEndWidth,
StyleGraphicPropertiesElement.MarkerStart,
StyleGraphicPropertiesElement.MarkerStartCenter,
StyleGraphicPropertiesElement.MarkerStartWidth,
StyleGraphicPropertiesElement.MeasureAlign,
StyleGraphicPropertiesElement.MeasureVerticalAlign,
StyleGraphicPropertiesElement.OleDrawAspect,
StyleGraphicPropertiesElement.Opacity,
StyleGraphicPropertiesElement.OpacityName,
StyleGraphicPropertiesElement.Parallel,
StyleGraphicPropertiesElement.Placing,
StyleGraphicPropertiesElement.Red,
StyleGraphicPropertiesElement.SecondaryFillColor,
StyleGraphicPropertiesElement.DrawShadow,
StyleGraphicPropertiesElement.ShadowColor,
StyleGraphicPropertiesElement.ShadowOffsetX,
StyleGraphicPropertiesElement.ShadowOffsetY,
StyleGraphicPropertiesElement.ShadowOpacity,
StyleGraphicPropertiesElement.ShowUnit,
StyleGraphicPropertiesElement.StartGuide,
StyleGraphicPropertiesElement.StartLineSpacingHorizontal,
StyleGraphicPropertiesElement.StartLineSpacingVertical,
StyleGraphicPropertiesElement.Stroke,
StyleGraphicPropertiesElement.StrokeDash,
StyleGraphicPropertiesElement.StrokeDashNames,
StyleGraphicPropertiesElement.StrokeLinejoin,
StyleGraphicPropertiesElement.SymbolColor,
StyleGraphicPropertiesElement.TextareaHorizontalAlign,
StyleGraphicPropertiesElement.TextareaVerticalAlign,
StyleGraphicPropertiesElement.TileRepeatOffset,
StyleGraphicPropertiesElement.Unit,
StyleGraphicPropertiesElement.VisibleAreaHeight,
StyleGraphicPropertiesElement.VisibleAreaLeft,
StyleGraphicPropertiesElement.VisibleAreaTop,
StyleGraphicPropertiesElement.VisibleAreaWidth,
StyleGraphicPropertiesElement.WrapInfluenceOnPosition,
StyleGraphicPropertiesElement.BackgroundColor,
StyleGraphicPropertiesElement.Border,
StyleGraphicPropertiesElement.BorderBottom,
StyleGraphicPropertiesElement.BorderLeft,
StyleGraphicPropertiesElement.BorderRight,
StyleGraphicPropertiesElement.BorderTop,
StyleGraphicPropertiesElement.Clip,
StyleGraphicPropertiesElement.Margin,
StyleGraphicPropertiesElement.MarginBottom,
StyleGraphicPropertiesElement.MarginLeft,
StyleGraphicPropertiesElement.MarginRight,
StyleGraphicPropertiesElement.MarginTop,
StyleGraphicPropertiesElement.MaxHeight,
StyleGraphicPropertiesElement.MaxWidth,
StyleGraphicPropertiesElement.MinHeight,
StyleGraphicPropertiesElement.MinWidth,
StyleGraphicPropertiesElement.Padding,
StyleGraphicPropertiesElement.PaddingBottom,
StyleGraphicPropertiesElement.PaddingLeft,
StyleGraphicPropertiesElement.PaddingRight,
StyleGraphicPropertiesElement.PaddingTop,
StyleGraphicPropertiesElement.WrapOption,
StyleGraphicPropertiesElement.BackgroundTransparency,
StyleGraphicPropertiesElement.BorderLineWidth,
StyleGraphicPropertiesElement.BorderLineWidthBottom,
StyleGraphicPropertiesElement.BorderLineWidthLeft,
StyleGraphicPropertiesElement.BorderLineWidthRight,
StyleGraphicPropertiesElement.BorderLineWidthTop,
StyleGraphicPropertiesElement.Editable,
StyleGraphicPropertiesElement.FlowWithText,
StyleGraphicPropertiesElement.HorizontalPos,
StyleGraphicPropertiesElement.HorizontalRel,
StyleGraphicPropertiesElement.Mirror,
StyleGraphicPropertiesElement.NumberWrappedParagraphs,
StyleGraphicPropertiesElement.OverflowBehavior,
StyleGraphicPropertiesElement.PrintContent,
StyleGraphicPropertiesElement.Protect,
StyleGraphicPropertiesElement.RelHeight,
StyleGraphicPropertiesElement.RelWidth,
StyleGraphicPropertiesElement.Repeat,
StyleGraphicPropertiesElement.RunThrough,
StyleGraphicPropertiesElement.StyleShadow,
StyleGraphicPropertiesElement.ShrinkToFit,
StyleGraphicPropertiesElement.VerticalPos,
StyleGraphicPropertiesElement.VerticalRel,
StyleGraphicPropertiesElement.Wrap,
StyleGraphicPropertiesElement.WrapContour,
StyleGraphicPropertiesElement.WrapContourMode,
StyleGraphicPropertiesElement.WrapDynamicThreshold,
StyleGraphicPropertiesElement.WritingMode,
StyleGraphicPropertiesElement.FillRule,
StyleGraphicPropertiesElement.Height,
StyleGraphicPropertiesElement.StrokeColor,
StyleGraphicPropertiesElement.StrokeLinecap,
StyleGraphicPropertiesElement.StrokeOpacity,
StyleGraphicPropertiesElement.StrokeWidth,
StyleGraphicPropertiesElement.Width,
StyleGraphicPropertiesElement.X,
StyleGraphicPropertiesElement.Y,
StyleGraphicPropertiesElement.AnchorPageNumber,
StyleGraphicPropertiesElement.AnchorType,
StyleGraphicPropertiesElement.Animation,
StyleGraphicPropertiesElement.AnimationDelay,
StyleGraphicPropertiesElement.AnimationDirection,
StyleGraphicPropertiesElement.AnimationRepeat,
StyleGraphicPropertiesElement.AnimationStartInside,
StyleGraphicPropertiesElement.AnimationSteps,
StyleGraphicPropertiesElement.AnimationStopInside,
StyleHeaderFooterPropertiesElement.BackgroundColor,
StyleHeaderFooterPropertiesElement.Border,
StyleHeaderFooterPropertiesElement.BorderBottom,
StyleHeaderFooterPropertiesElement.BorderLeft,
StyleHeaderFooterPropertiesElement.BorderRight,
StyleHeaderFooterPropertiesElement.BorderTop,
StyleHeaderFooterPropertiesElement.Margin,
StyleHeaderFooterPropertiesElement.MarginBottom,
StyleHeaderFooterPropertiesElement.MarginLeft,
StyleHeaderFooterPropertiesElement.MarginRight,
StyleHeaderFooterPropertiesElement.MarginTop,
StyleHeaderFooterPropertiesElement.MinHeight,
StyleHeaderFooterPropertiesElement.Padding,
StyleHeaderFooterPropertiesElement.PaddingBottom,
StyleHeaderFooterPropertiesElement.PaddingLeft,
StyleHeaderFooterPropertiesElement.PaddingRight,
StyleHeaderFooterPropertiesElement.PaddingTop,
StyleHeaderFooterPropertiesElement.BorderLineWidth,
StyleHeaderFooterPropertiesElement.BorderLineWidthBottom,
StyleHeaderFooterPropertiesElement.BorderLineWidthLeft,
StyleHeaderFooterPropertiesElement.BorderLineWidthRight,
StyleHeaderFooterPropertiesElement.BorderLineWidthTop,
StyleHeaderFooterPropertiesElement.DynamicSpacing,
StyleHeaderFooterPropertiesElement.Shadow,
StyleHeaderFooterPropertiesElement.Height,
StyleListLevelPropertiesElement.Height,
StyleListLevelPropertiesElement.TextAlign,
StyleListLevelPropertiesElement.Width,
StyleListLevelPropertiesElement.FontName,
StyleListLevelPropertiesElement.VerticalPos,
StyleListLevelPropertiesElement.VerticalRel,
StyleListLevelPropertiesElement.Y,
StyleListLevelPropertiesElement.ListLevelPositionAndSpaceMode,
StyleListLevelPropertiesElement.MinLabelDistance,
StyleListLevelPropertiesElement.MinLabelWidth,
StyleListLevelPropertiesElement.SpaceBefore,
StylePageLayoutPropertiesElement.BackgroundColor,
StylePageLayoutPropertiesElement.Border,
StylePageLayoutPropertiesElement.BorderBottom,
StylePageLayoutPropertiesElement.BorderLeft,
StylePageLayoutPropertiesElement.BorderRight,
StylePageLayoutPropertiesElement.BorderTop,
StylePageLayoutPropertiesElement.Margin,
StylePageLayoutPropertiesElement.MarginBottom,
StylePageLayoutPropertiesElement.MarginLeft,
StylePageLayoutPropertiesElement.MarginRight,
StylePageLayoutPropertiesElement.MarginTop,
StylePageLayoutPropertiesElement.Padding,
StylePageLayoutPropertiesElement.PaddingBottom,
StylePageLayoutPropertiesElement.PaddingLeft,
StylePageLayoutPropertiesElement.PaddingRight,
StylePageLayoutPropertiesElement.PaddingTop,
StylePageLayoutPropertiesElement.PageHeight,
StylePageLayoutPropertiesElement.PageWidth,
StylePageLayoutPropertiesElement.BorderLineWidth,
StylePageLayoutPropertiesElement.BorderLineWidthBottom,
StylePageLayoutPropertiesElement.BorderLineWidthLeft,
StylePageLayoutPropertiesElement.BorderLineWidthRight,
StylePageLayoutPropertiesElement.BorderLineWidthTop,
StylePageLayoutPropertiesElement.FirstPageNumber,
StylePageLayoutPropertiesElement.FootnoteMaxHeight,
StylePageLayoutPropertiesElement.LayoutGridBaseHeight,
StylePageLayoutPropertiesElement.LayoutGridBaseWidth,
StylePageLayoutPropertiesElement.LayoutGridColor,
StylePageLayoutPropertiesElement.LayoutGridDisplay,
StylePageLayoutPropertiesElement.LayoutGridLines,
StylePageLayoutPropertiesElement.LayoutGridMode,
StylePageLayoutPropertiesElement.LayoutGridPrint,
StylePageLayoutPropertiesElement.LayoutGridRubyBelow,
StylePageLayoutPropertiesElement.LayoutGridRubyHeight,
StylePageLayoutPropertiesElement.LayoutGridSnapTo,
StylePageLayoutPropertiesElement.LayoutGridStandardMode,
StylePageLayoutPropertiesElement.NumFormat,
StylePageLayoutPropertiesElement.NumFormat,
StylePageLayoutPropertiesElement.NumLetterSync,
StylePageLayoutPropertiesElement.NumPrefix,
StylePageLayoutPropertiesElement.NumSuffix,
StylePageLayoutPropertiesElement.PaperTrayName,
StylePageLayoutPropertiesElement.Print,
StylePageLayoutPropertiesElement.PrintOrientation,
StylePageLayoutPropertiesElement.PrintPageOrder,
StylePageLayoutPropertiesElement.RegisterTruthRefStyleName,
StylePageLayoutPropertiesElement.ScaleTo,
StylePageLayoutPropertiesElement.ScaleToPages,
StylePageLayoutPropertiesElement.Shadow,
StylePageLayoutPropertiesElement.TableCentering,
StylePageLayoutPropertiesElement.WritingMode,
StyleParagraphPropertiesElement.BackgroundColor,
StyleParagraphPropertiesElement.Border,
StyleParagraphPropertiesElement.BorderBottom,
StyleParagraphPropertiesElement.BorderLeft,
StyleParagraphPropertiesElement.BorderRight,
StyleParagraphPropertiesElement.BorderTop,
StyleParagraphPropertiesElement.BreakAfter,
StyleParagraphPropertiesElement.BreakBefore,
StyleParagraphPropertiesElement.HyphenationKeep,
StyleParagraphPropertiesElement.HyphenationLadderCount,
StyleParagraphPropertiesElement.KeepTogether,
StyleParagraphPropertiesElement.KeepWithNext,
StyleParagraphPropertiesElement.LineHeight,
StyleParagraphPropertiesElement.Margin,
StyleParagraphPropertiesElement.MarginBottom,
StyleParagraphPropertiesElement.MarginLeft,
StyleParagraphPropertiesElement.MarginRight,
StyleParagraphPropertiesElement.MarginTop,
StyleParagraphPropertiesElement.Orphans,
StyleParagraphPropertiesElement.Padding,
StyleParagraphPropertiesElement.PaddingBottom,
StyleParagraphPropertiesElement.PaddingLeft,
StyleParagraphPropertiesElement.PaddingRight,
StyleParagraphPropertiesElement.PaddingTop,
StyleParagraphPropertiesElement.TextAlign,
StyleParagraphPropertiesElement.TextAlignLast,
StyleParagraphPropertiesElement.TextIndent,
StyleParagraphPropertiesElement.Widows,
StyleParagraphPropertiesElement.AutoTextIndent,
StyleParagraphPropertiesElement.BackgroundTransparency,
StyleParagraphPropertiesElement.BorderLineWidth,
StyleParagraphPropertiesElement.BorderLineWidthBottom,
StyleParagraphPropertiesElement.BorderLineWidthLeft,
StyleParagraphPropertiesElement.BorderLineWidthRight,
StyleParagraphPropertiesElement.BorderLineWidthTop,
StyleParagraphPropertiesElement.FontIndependentLineSpacing,
StyleParagraphPropertiesElement.JoinBorder,
StyleParagraphPropertiesElement.JustifySingleWord,
StyleParagraphPropertiesElement.LineBreak,
StyleParagraphPropertiesElement.LineHeightAtLeast,
StyleParagraphPropertiesElement.LineSpacing,
StyleParagraphPropertiesElement.PageNumber,
StyleParagraphPropertiesElement.PunctuationWrap,
StyleParagraphPropertiesElement.RegisterTrue,
StyleParagraphPropertiesElement.Shadow,
StyleParagraphPropertiesElement.SnapToLayoutGrid,
StyleParagraphPropertiesElement.TabStopDistance,
StyleParagraphPropertiesElement.TextAutospace,
StyleParagraphPropertiesElement.VerticalAlign,
StyleParagraphPropertiesElement.WritingMode,
StyleParagraphPropertiesElement.WritingModeAutomatic,
StyleParagraphPropertiesElement.LineNumber,
StyleParagraphPropertiesElement.NumberLines,
StyleRubyPropertiesElement.RubyAlign,
StyleRubyPropertiesElement.RubyPosition,
StyleSectionPropertiesElement.BackgroundColor,
StyleSectionPropertiesElement.MarginLeft,
StyleSectionPropertiesElement.MarginRight,
StyleSectionPropertiesElement.Editable,
StyleSectionPropertiesElement.Protect,
StyleSectionPropertiesElement.WritingMode,
StyleSectionPropertiesElement.DontBalanceTextColumns,
StyleTableCellPropertiesElement.BackgroundColor,
StyleTableCellPropertiesElement.Border,
StyleTableCellPropertiesElement.BorderBottom,
StyleTableCellPropertiesElement.BorderLeft,
StyleTableCellPropertiesElement.BorderRight,
StyleTableCellPropertiesElement.BorderTop,
StyleTableCellPropertiesElement.Padding,
StyleTableCellPropertiesElement.PaddingBottom,
StyleTableCellPropertiesElement.PaddingLeft,
StyleTableCellPropertiesElement.PaddingRight,
StyleTableCellPropertiesElement.PaddingTop,
StyleTableCellPropertiesElement.WrapOption,
StyleTableCellPropertiesElement.BorderLineWidth,
StyleTableCellPropertiesElement.BorderLineWidthBottom,
StyleTableCellPropertiesElement.BorderLineWidthLeft,
StyleTableCellPropertiesElement.BorderLineWidthRight,
StyleTableCellPropertiesElement.BorderLineWidthTop,
StyleTableCellPropertiesElement.CellProtect,
StyleTableCellPropertiesElement.DecimalPlaces,
StyleTableCellPropertiesElement.DiagonalBlTr,
StyleTableCellPropertiesElement.DiagonalBlTrWidths,
StyleTableCellPropertiesElement.DiagonalTlBr,
StyleTableCellPropertiesElement.DiagonalTlBrWidths,
StyleTableCellPropertiesElement.Direction,
StyleTableCellPropertiesElement.GlyphOrientationVertical,
StyleTableCellPropertiesElement.PrintContent,
StyleTableCellPropertiesElement.RepeatContent,
StyleTableCellPropertiesElement.RotationAlign,
StyleTableCellPropertiesElement.RotationAngle,
StyleTableCellPropertiesElement.Shadow,
StyleTableCellPropertiesElement.ShrinkToFit,
StyleTableCellPropertiesElement.TextAlignSource,
StyleTableCellPropertiesElement.VerticalAlign,
StyleTableCellPropertiesElement.WritingMode,
StyleTableColumnPropertiesElement.BreakAfter,
StyleTableColumnPropertiesElement.BreakBefore,
StyleTableColumnPropertiesElement.ColumnWidth,
StyleTableColumnPropertiesElement.RelColumnWidth,
StyleTableColumnPropertiesElement.UseOptimalColumnWidth,
StyleTablePropertiesElement.BackgroundColor,
StyleTablePropertiesElement.BreakAfter,
StyleTablePropertiesElement.BreakBefore,
StyleTablePropertiesElement.KeepWithNext,
StyleTablePropertiesElement.Margin,
StyleTablePropertiesElement.MarginBottom,
StyleTablePropertiesElement.MarginLeft,
StyleTablePropertiesElement.MarginRight,
StyleTablePropertiesElement.MarginTop,
StyleTablePropertiesElement.MayBreakBetweenRows,
StyleTablePropertiesElement.PageNumber,
StyleTablePropertiesElement.RelWidth,
StyleTablePropertiesElement.Shadow,
StyleTablePropertiesElement.Width,
StyleTablePropertiesElement.WritingMode,
StyleTablePropertiesElement.Align,
StyleTablePropertiesElement.BorderModel,
StyleTablePropertiesElement.Display,
StyleTableRowPropertiesElement.BackgroundColor,
StyleTableRowPropertiesElement.BreakAfter,
StyleTableRowPropertiesElement.BreakBefore,
StyleTableRowPropertiesElement.KeepTogether,
StyleTableRowPropertiesElement.MinRowHeight,
StyleTableRowPropertiesElement.RowHeight,
StyleTableRowPropertiesElement.UseOptimalRowHeight,
StyleTextPropertiesElement.BackgroundColor,
StyleTextPropertiesElement.Color,
StyleTextPropertiesElement.Country,
StyleTextPropertiesElement.FontFamily,
StyleTextPropertiesElement.FontSize,
StyleTextPropertiesElement.FontStyle,
StyleTextPropertiesElement.FontVariant,
StyleTextPropertiesElement.FontWeight,
StyleTextPropertiesElement.Hyphenate,
StyleTextPropertiesElement.HyphenationPushCharCount,
StyleTextPropertiesElement.HyphenationRemainCharCount,
StyleTextPropertiesElement.Language,
StyleTextPropertiesElement.LetterSpacing,
StyleTextPropertiesElement.Script,
StyleTextPropertiesElement.TextShadow,
StyleTextPropertiesElement.TextTransform,
StyleTextPropertiesElement.CountryAsian,
StyleTextPropertiesElement.CountryComplex,
StyleTextPropertiesElement.FontCharset,
StyleTextPropertiesElement.FontCharsetAsian,
StyleTextPropertiesElement.FontCharsetComplex,
StyleTextPropertiesElement.FontFamilyAsian,
StyleTextPropertiesElement.FontFamilyComplex,
StyleTextPropertiesElement.FontFamilyGeneric,
StyleTextPropertiesElement.FontFamilyGenericAsian,
StyleTextPropertiesElement.FontFamilyGenericComplex,
StyleTextPropertiesElement.FontName,
StyleTextPropertiesElement.FontNameAsian,
StyleTextPropertiesElement.FontNameComplex,
StyleTextPropertiesElement.FontPitch,
StyleTextPropertiesElement.FontPitchAsian,
StyleTextPropertiesElement.FontPitchComplex,
StyleTextPropertiesElement.FontRelief,
StyleTextPropertiesElement.FontSizeAsian,
StyleTextPropertiesElement.FontSizeComplex,
StyleTextPropertiesElement.FontSizeRel,
StyleTextPropertiesElement.FontSizeRelAsian,
StyleTextPropertiesElement.FontSizeRelComplex,
StyleTextPropertiesElement.FontStyleAsian,
StyleTextPropertiesElement.FontStyleComplex,
StyleTextPropertiesElement.FontStyleName,
StyleTextPropertiesElement.FontStyleNameAsian,
StyleTextPropertiesElement.FontStyleNameComplex,
StyleTextPropertiesElement.FontWeightAsian,
StyleTextPropertiesElement.FontWeightComplex,
StyleTextPropertiesElement.LanguageAsian,
StyleTextPropertiesElement.LanguageComplex,
StyleTextPropertiesElement.LetterKerning,
StyleTextPropertiesElement.RfcLanguageTag,
StyleTextPropertiesElement.RfcLanguageTagAsian,
StyleTextPropertiesElement.RfcLanguageTagComplex,
StyleTextPropertiesElement.ScriptAsian,
StyleTextPropertiesElement.ScriptComplex,
StyleTextPropertiesElement.ScriptType,
StyleTextPropertiesElement.TextBlinking,
StyleTextPropertiesElement.TextCombine,
StyleTextPropertiesElement.TextCombineEndChar,
StyleTextPropertiesElement.TextCombineStartChar,
StyleTextPropertiesElement.TextEmphasize,
StyleTextPropertiesElement.TextLineThroughColor,
StyleTextPropertiesElement.TextLineThroughMode,
StyleTextPropertiesElement.TextLineThroughStyle,
StyleTextPropertiesElement.TextLineThroughText,
StyleTextPropertiesElement.TextLineThroughTextStyle,
StyleTextPropertiesElement.TextLineThroughType,
StyleTextPropertiesElement.TextLineThroughWidth,
StyleTextPropertiesElement.TextOutline,
StyleTextPropertiesElement.TextOverlineColor,
StyleTextPropertiesElement.TextOverlineMode,
StyleTextPropertiesElement.TextOverlineStyle,
StyleTextPropertiesElement.TextOverlineType,
StyleTextPropertiesElement.TextOverlineWidth,
StyleTextPropertiesElement.TextPosition,
StyleTextPropertiesElement.TextRotationAngle,
StyleTextPropertiesElement.TextRotationScale,
StyleTextPropertiesElement.TextScale,
StyleTextPropertiesElement.TextUnderlineColor,
StyleTextPropertiesElement.TextUnderlineMode,
StyleTextPropertiesElement.TextUnderlineStyle,
StyleTextPropertiesElement.TextUnderlineType,
StyleTextPropertiesElement.TextUnderlineWidth,
StyleTextPropertiesElement.UseWindowFontColor,
StyleTextPropertiesElement.Condition,
StyleTextPropertiesElement.Display,
StyleTextPropertiesElement.Display,
StyleTextPropertiesElement.Display,
});
public final static OdfStyleFamily TableCell = new OdfStyleFamily("table-cell",
new OdfStyleProperty[]{
StyleChartPropertiesElement.AngleOffset,
StyleChartPropertiesElement.AutoPosition,
StyleChartPropertiesElement.AutoSize,
StyleChartPropertiesElement.AxisLabelPosition,
StyleChartPropertiesElement.AxisPosition,
StyleChartPropertiesElement.ConnectBars,
StyleChartPropertiesElement.DataLabelNumber,
StyleChartPropertiesElement.DataLabelSymbol,
StyleChartPropertiesElement.DataLabelText,
StyleChartPropertiesElement.Deep,
StyleChartPropertiesElement.DisplayLabel,
StyleChartPropertiesElement.ErrorCategory,
StyleChartPropertiesElement.ErrorLowerIndicator,
StyleChartPropertiesElement.ErrorLowerLimit,
StyleChartPropertiesElement.ErrorLowerRange,
StyleChartPropertiesElement.ErrorMargin,
StyleChartPropertiesElement.ErrorPercentage,
StyleChartPropertiesElement.ErrorUpperIndicator,
StyleChartPropertiesElement.ErrorUpperLimit,
StyleChartPropertiesElement.ErrorUpperRange,
StyleChartPropertiesElement.GapWidth,
StyleChartPropertiesElement.GroupBarsPerAxis,
StyleChartPropertiesElement.HoleSize,
StyleChartPropertiesElement.IncludeHiddenCells,
StyleChartPropertiesElement.Interpolation,
StyleChartPropertiesElement.IntervalMajor,
StyleChartPropertiesElement.IntervalMinorDivisor,
StyleChartPropertiesElement.JapaneseCandleStick,
StyleChartPropertiesElement.LabelArrangement,
StyleChartPropertiesElement.LabelPosition,
StyleChartPropertiesElement.LabelPositionNegative,
StyleChartPropertiesElement.Lines,
StyleChartPropertiesElement.LinkDataStyleToSource,
StyleChartPropertiesElement.Logarithmic,
StyleChartPropertiesElement.Maximum,
StyleChartPropertiesElement.MeanValue,
StyleChartPropertiesElement.Minimum,
StyleChartPropertiesElement.Origin,
StyleChartPropertiesElement.Overlap,
StyleChartPropertiesElement.Percentage,
StyleChartPropertiesElement.PieOffset,
StyleChartPropertiesElement.RegressionType,
StyleChartPropertiesElement.ReverseDirection,
StyleChartPropertiesElement.RightAngledAxes,
StyleChartPropertiesElement.ScaleText,
StyleChartPropertiesElement.SeriesSource,
StyleChartPropertiesElement.SolidType,
StyleChartPropertiesElement.SortByXValues,
StyleChartPropertiesElement.SplineOrder,
StyleChartPropertiesElement.SplineResolution,
StyleChartPropertiesElement.Stacked,
StyleChartPropertiesElement.SymbolHeight,
StyleChartPropertiesElement.SymbolName,
StyleChartPropertiesElement.SymbolType,
StyleChartPropertiesElement.SymbolType,
StyleChartPropertiesElement.SymbolType,
StyleChartPropertiesElement.SymbolType,
StyleChartPropertiesElement.SymbolWidth,
StyleChartPropertiesElement.TextOverlap,
StyleChartPropertiesElement.ThreeDimensional,
StyleChartPropertiesElement.TickMarkPosition,
StyleChartPropertiesElement.TickMarksMajorInner,
StyleChartPropertiesElement.TickMarksMajorOuter,
StyleChartPropertiesElement.TickMarksMinorInner,
StyleChartPropertiesElement.TickMarksMinorOuter,
StyleChartPropertiesElement.TreatEmptyCells,
StyleChartPropertiesElement.Vertical,
StyleChartPropertiesElement.Visible,
StyleChartPropertiesElement.Direction,
StyleChartPropertiesElement.RotationAngle,
StyleChartPropertiesElement.LineBreak,
StyleDrawingPagePropertiesElement.BackgroundSize,
StyleDrawingPagePropertiesElement.Fill,
StyleDrawingPagePropertiesElement.FillColor,
StyleDrawingPagePropertiesElement.FillGradientName,
StyleDrawingPagePropertiesElement.FillHatchName,
StyleDrawingPagePropertiesElement.FillHatchSolid,
StyleDrawingPagePropertiesElement.FillImageHeight,
StyleDrawingPagePropertiesElement.FillImageName,
StyleDrawingPagePropertiesElement.FillImageRefPoint,
StyleDrawingPagePropertiesElement.FillImageRefPointX,
StyleDrawingPagePropertiesElement.FillImageRefPointY,
StyleDrawingPagePropertiesElement.FillImageWidth,
StyleDrawingPagePropertiesElement.GradientStepCount,
StyleDrawingPagePropertiesElement.Opacity,
StyleDrawingPagePropertiesElement.OpacityName,
StyleDrawingPagePropertiesElement.SecondaryFillColor,
StyleDrawingPagePropertiesElement.TileRepeatOffset,
StyleDrawingPagePropertiesElement.BackgroundObjectsVisible,
StyleDrawingPagePropertiesElement.BackgroundVisible,
StyleDrawingPagePropertiesElement.DisplayDateTime,
StyleDrawingPagePropertiesElement.DisplayFooter,
StyleDrawingPagePropertiesElement.DisplayHeader,
StyleDrawingPagePropertiesElement.DisplayPageNumber,
StyleDrawingPagePropertiesElement.Duration,
StyleDrawingPagePropertiesElement.TransitionSpeed,
StyleDrawingPagePropertiesElement.TransitionStyle,
StyleDrawingPagePropertiesElement.TransitionType,
StyleDrawingPagePropertiesElement.Visibility,
StyleDrawingPagePropertiesElement.Direction,
StyleDrawingPagePropertiesElement.FadeColor,
StyleDrawingPagePropertiesElement.Subtype,
StyleDrawingPagePropertiesElement.Type,
StyleDrawingPagePropertiesElement.Repeat,
StyleDrawingPagePropertiesElement.FillRule,
StyleGraphicPropertiesElement.AmbientColor,
StyleGraphicPropertiesElement.BackScale,
StyleGraphicPropertiesElement.BackfaceCulling,
StyleGraphicPropertiesElement.CloseBack,
StyleGraphicPropertiesElement.CloseFront,
StyleGraphicPropertiesElement.Depth,
StyleGraphicPropertiesElement.DiffuseColor,
StyleGraphicPropertiesElement.EdgeRounding,
StyleGraphicPropertiesElement.EdgeRoundingMode,
StyleGraphicPropertiesElement.EmissiveColor,
StyleGraphicPropertiesElement.EndAngle,
StyleGraphicPropertiesElement.HorizontalSegments,
StyleGraphicPropertiesElement.LightingMode,
StyleGraphicPropertiesElement.NormalsDirection,
StyleGraphicPropertiesElement.NormalsKind,
StyleGraphicPropertiesElement.Dr3dShadow,
StyleGraphicPropertiesElement.Shininess,
StyleGraphicPropertiesElement.SpecularColor,
StyleGraphicPropertiesElement.TextureFilter,
StyleGraphicPropertiesElement.TextureGenerationModeX,
StyleGraphicPropertiesElement.TextureGenerationModeY,
StyleGraphicPropertiesElement.TextureKind,
StyleGraphicPropertiesElement.TextureMode,
StyleGraphicPropertiesElement.VerticalSegments,
StyleGraphicPropertiesElement.AutoGrowHeight,
StyleGraphicPropertiesElement.AutoGrowWidth,
StyleGraphicPropertiesElement.Blue,
StyleGraphicPropertiesElement.CaptionAngle,
StyleGraphicPropertiesElement.CaptionAngleType,
StyleGraphicPropertiesElement.CaptionEscape,
StyleGraphicPropertiesElement.CaptionEscapeDirection,
StyleGraphicPropertiesElement.CaptionFitLineLength,
StyleGraphicPropertiesElement.CaptionGap,
StyleGraphicPropertiesElement.CaptionLineLength,
StyleGraphicPropertiesElement.CaptionType,
StyleGraphicPropertiesElement.ColorInversion,
StyleGraphicPropertiesElement.ColorMode,
StyleGraphicPropertiesElement.Contrast,
StyleGraphicPropertiesElement.DecimalPlaces,
StyleGraphicPropertiesElement.DrawAspect,
StyleGraphicPropertiesElement.EndGuide,
StyleGraphicPropertiesElement.EndLineSpacingHorizontal,
StyleGraphicPropertiesElement.EndLineSpacingVertical,
StyleGraphicPropertiesElement.Fill,
StyleGraphicPropertiesElement.FillColor,
StyleGraphicPropertiesElement.FillGradientName,
StyleGraphicPropertiesElement.FillHatchName,
StyleGraphicPropertiesElement.FillHatchSolid,
StyleGraphicPropertiesElement.FillImageHeight,
StyleGraphicPropertiesElement.FillImageName,
StyleGraphicPropertiesElement.FillImageRefPoint,
StyleGraphicPropertiesElement.FillImageRefPointX,
StyleGraphicPropertiesElement.FillImageRefPointY,
StyleGraphicPropertiesElement.FillImageWidth,
StyleGraphicPropertiesElement.FitToContour,
StyleGraphicPropertiesElement.FitToSize,
StyleGraphicPropertiesElement.FrameDisplayBorder,
StyleGraphicPropertiesElement.FrameDisplayScrollbar,
StyleGraphicPropertiesElement.FrameMarginHorizontal,
StyleGraphicPropertiesElement.FrameMarginVertical,
StyleGraphicPropertiesElement.Gamma,
StyleGraphicPropertiesElement.GradientStepCount,
StyleGraphicPropertiesElement.Green,
StyleGraphicPropertiesElement.GuideDistance,
StyleGraphicPropertiesElement.GuideOverhang,
StyleGraphicPropertiesElement.ImageOpacity,
StyleGraphicPropertiesElement.LineDistance,
StyleGraphicPropertiesElement.Luminance,
StyleGraphicPropertiesElement.MarkerEnd,
StyleGraphicPropertiesElement.MarkerEndCenter,
StyleGraphicPropertiesElement.MarkerEndWidth,
StyleGraphicPropertiesElement.MarkerStart,
StyleGraphicPropertiesElement.MarkerStartCenter,
StyleGraphicPropertiesElement.MarkerStartWidth,
StyleGraphicPropertiesElement.MeasureAlign,
StyleGraphicPropertiesElement.MeasureVerticalAlign,
StyleGraphicPropertiesElement.OleDrawAspect,
StyleGraphicPropertiesElement.Opacity,
StyleGraphicPropertiesElement.OpacityName,
StyleGraphicPropertiesElement.Parallel,
StyleGraphicPropertiesElement.Placing,
StyleGraphicPropertiesElement.Red,
StyleGraphicPropertiesElement.SecondaryFillColor,
StyleGraphicPropertiesElement.DrawShadow,
StyleGraphicPropertiesElement.ShadowColor,
StyleGraphicPropertiesElement.ShadowOffsetX,
StyleGraphicPropertiesElement.ShadowOffsetY,
StyleGraphicPropertiesElement.ShadowOpacity,
StyleGraphicPropertiesElement.ShowUnit,
StyleGraphicPropertiesElement.StartGuide,
StyleGraphicPropertiesElement.StartLineSpacingHorizontal,
StyleGraphicPropertiesElement.StartLineSpacingVertical,
StyleGraphicPropertiesElement.Stroke,
StyleGraphicPropertiesElement.StrokeDash,
StyleGraphicPropertiesElement.StrokeDashNames,
StyleGraphicPropertiesElement.StrokeLinejoin,
StyleGraphicPropertiesElement.SymbolColor,
StyleGraphicPropertiesElement.TextareaHorizontalAlign,
StyleGraphicPropertiesElement.TextareaVerticalAlign,
StyleGraphicPropertiesElement.TileRepeatOffset,
StyleGraphicPropertiesElement.Unit,
StyleGraphicPropertiesElement.VisibleAreaHeight,
StyleGraphicPropertiesElement.VisibleAreaLeft,
StyleGraphicPropertiesElement.VisibleAreaTop,
StyleGraphicPropertiesElement.VisibleAreaWidth,
StyleGraphicPropertiesElement.WrapInfluenceOnPosition,
StyleGraphicPropertiesElement.BackgroundColor,
StyleGraphicPropertiesElement.Border,
StyleGraphicPropertiesElement.BorderBottom,
StyleGraphicPropertiesElement.BorderLeft,
StyleGraphicPropertiesElement.BorderRight,
StyleGraphicPropertiesElement.BorderTop,
StyleGraphicPropertiesElement.Clip,
StyleGraphicPropertiesElement.Margin,
StyleGraphicPropertiesElement.MarginBottom,
StyleGraphicPropertiesElement.MarginLeft,
StyleGraphicPropertiesElement.MarginRight,
StyleGraphicPropertiesElement.MarginTop,
StyleGraphicPropertiesElement.MaxHeight,
StyleGraphicPropertiesElement.MaxWidth,
StyleGraphicPropertiesElement.MinHeight,
StyleGraphicPropertiesElement.MinWidth,
StyleGraphicPropertiesElement.Padding,
StyleGraphicPropertiesElement.PaddingBottom,
StyleGraphicPropertiesElement.PaddingLeft,
StyleGraphicPropertiesElement.PaddingRight,
StyleGraphicPropertiesElement.PaddingTop,
StyleGraphicPropertiesElement.WrapOption,
StyleGraphicPropertiesElement.BackgroundTransparency,
StyleGraphicPropertiesElement.BorderLineWidth,
StyleGraphicPropertiesElement.BorderLineWidthBottom,
StyleGraphicPropertiesElement.BorderLineWidthLeft,
StyleGraphicPropertiesElement.BorderLineWidthRight,
StyleGraphicPropertiesElement.BorderLineWidthTop,
StyleGraphicPropertiesElement.Editable,
StyleGraphicPropertiesElement.FlowWithText,
StyleGraphicPropertiesElement.HorizontalPos,
StyleGraphicPropertiesElement.HorizontalRel,
StyleGraphicPropertiesElement.Mirror,
StyleGraphicPropertiesElement.NumberWrappedParagraphs,
StyleGraphicPropertiesElement.OverflowBehavior,
StyleGraphicPropertiesElement.PrintContent,
StyleGraphicPropertiesElement.Protect,
StyleGraphicPropertiesElement.RelHeight,
StyleGraphicPropertiesElement.RelWidth,
StyleGraphicPropertiesElement.Repeat,
StyleGraphicPropertiesElement.RunThrough,
StyleGraphicPropertiesElement.StyleShadow,
StyleGraphicPropertiesElement.ShrinkToFit,
StyleGraphicPropertiesElement.VerticalPos,
StyleGraphicPropertiesElement.VerticalRel,
StyleGraphicPropertiesElement.Wrap,
StyleGraphicPropertiesElement.WrapContour,
StyleGraphicPropertiesElement.WrapContourMode,
StyleGraphicPropertiesElement.WrapDynamicThreshold,
StyleGraphicPropertiesElement.WritingMode,
StyleGraphicPropertiesElement.FillRule,
StyleGraphicPropertiesElement.Height,
StyleGraphicPropertiesElement.StrokeColor,
StyleGraphicPropertiesElement.StrokeLinecap,
StyleGraphicPropertiesElement.StrokeOpacity,
StyleGraphicPropertiesElement.StrokeWidth,
StyleGraphicPropertiesElement.Width,
StyleGraphicPropertiesElement.X,
StyleGraphicPropertiesElement.Y,
StyleGraphicPropertiesElement.AnchorPageNumber,
StyleGraphicPropertiesElement.AnchorType,
StyleGraphicPropertiesElement.Animation,
StyleGraphicPropertiesElement.AnimationDelay,
StyleGraphicPropertiesElement.AnimationDirection,
StyleGraphicPropertiesElement.AnimationRepeat,
StyleGraphicPropertiesElement.AnimationStartInside,
StyleGraphicPropertiesElement.AnimationSteps,
StyleGraphicPropertiesElement.AnimationStopInside,
StyleHeaderFooterPropertiesElement.BackgroundColor,
StyleHeaderFooterPropertiesElement.Border,
StyleHeaderFooterPropertiesElement.BorderBottom,
StyleHeaderFooterPropertiesElement.BorderLeft,
StyleHeaderFooterPropertiesElement.BorderRight,
StyleHeaderFooterPropertiesElement.BorderTop,
StyleHeaderFooterPropertiesElement.Margin,
StyleHeaderFooterPropertiesElement.MarginBottom,
StyleHeaderFooterPropertiesElement.MarginLeft,
StyleHeaderFooterPropertiesElement.MarginRight,
StyleHeaderFooterPropertiesElement.MarginTop,
StyleHeaderFooterPropertiesElement.MinHeight,
StyleHeaderFooterPropertiesElement.Padding,
StyleHeaderFooterPropertiesElement.PaddingBottom,
StyleHeaderFooterPropertiesElement.PaddingLeft,
StyleHeaderFooterPropertiesElement.PaddingRight,
StyleHeaderFooterPropertiesElement.PaddingTop,
StyleHeaderFooterPropertiesElement.BorderLineWidth,
StyleHeaderFooterPropertiesElement.BorderLineWidthBottom,
StyleHeaderFooterPropertiesElement.BorderLineWidthLeft,
StyleHeaderFooterPropertiesElement.BorderLineWidthRight,
StyleHeaderFooterPropertiesElement.BorderLineWidthTop,
StyleHeaderFooterPropertiesElement.DynamicSpacing,
StyleHeaderFooterPropertiesElement.Shadow,
StyleHeaderFooterPropertiesElement.Height,
StyleListLevelPropertiesElement.Height,
StyleListLevelPropertiesElement.TextAlign,
StyleListLevelPropertiesElement.Width,
StyleListLevelPropertiesElement.FontName,
StyleListLevelPropertiesElement.VerticalPos,
StyleListLevelPropertiesElement.VerticalRel,
StyleListLevelPropertiesElement.Y,
StyleListLevelPropertiesElement.ListLevelPositionAndSpaceMode,
StyleListLevelPropertiesElement.MinLabelDistance,
StyleListLevelPropertiesElement.MinLabelWidth,
StyleListLevelPropertiesElement.SpaceBefore,
StylePageLayoutPropertiesElement.BackgroundColor,
StylePageLayoutPropertiesElement.Border,
StylePageLayoutPropertiesElement.BorderBottom,
StylePageLayoutPropertiesElement.BorderLeft,
StylePageLayoutPropertiesElement.BorderRight,
StylePageLayoutPropertiesElement.BorderTop,
StylePageLayoutPropertiesElement.Margin,
StylePageLayoutPropertiesElement.MarginBottom,
StylePageLayoutPropertiesElement.MarginLeft,
StylePageLayoutPropertiesElement.MarginRight,
StylePageLayoutPropertiesElement.MarginTop,
StylePageLayoutPropertiesElement.Padding,
StylePageLayoutPropertiesElement.PaddingBottom,
StylePageLayoutPropertiesElement.PaddingLeft,
StylePageLayoutPropertiesElement.PaddingRight,
StylePageLayoutPropertiesElement.PaddingTop,
StylePageLayoutPropertiesElement.PageHeight,
StylePageLayoutPropertiesElement.PageWidth,
StylePageLayoutPropertiesElement.BorderLineWidth,
StylePageLayoutPropertiesElement.BorderLineWidthBottom,
StylePageLayoutPropertiesElement.BorderLineWidthLeft,
StylePageLayoutPropertiesElement.BorderLineWidthRight,
StylePageLayoutPropertiesElement.BorderLineWidthTop,
StylePageLayoutPropertiesElement.FirstPageNumber,
StylePageLayoutPropertiesElement.FootnoteMaxHeight,
StylePageLayoutPropertiesElement.LayoutGridBaseHeight,
StylePageLayoutPropertiesElement.LayoutGridBaseWidth,
StylePageLayoutPropertiesElement.LayoutGridColor,
StylePageLayoutPropertiesElement.LayoutGridDisplay,
StylePageLayoutPropertiesElement.LayoutGridLines,
StylePageLayoutPropertiesElement.LayoutGridMode,
StylePageLayoutPropertiesElement.LayoutGridPrint,
StylePageLayoutPropertiesElement.LayoutGridRubyBelow,
StylePageLayoutPropertiesElement.LayoutGridRubyHeight,
StylePageLayoutPropertiesElement.LayoutGridSnapTo,
StylePageLayoutPropertiesElement.LayoutGridStandardMode,
StylePageLayoutPropertiesElement.NumFormat,
StylePageLayoutPropertiesElement.NumFormat,
StylePageLayoutPropertiesElement.NumLetterSync,
StylePageLayoutPropertiesElement.NumPrefix,
StylePageLayoutPropertiesElement.NumSuffix,
StylePageLayoutPropertiesElement.PaperTrayName,
StylePageLayoutPropertiesElement.Print,
StylePageLayoutPropertiesElement.PrintOrientation,
StylePageLayoutPropertiesElement.PrintPageOrder,
StylePageLayoutPropertiesElement.RegisterTruthRefStyleName,
StylePageLayoutPropertiesElement.ScaleTo,
StylePageLayoutPropertiesElement.ScaleToPages,
StylePageLayoutPropertiesElement.Shadow,
StylePageLayoutPropertiesElement.TableCentering,
StylePageLayoutPropertiesElement.WritingMode,
StyleParagraphPropertiesElement.BackgroundColor,
StyleParagraphPropertiesElement.Border,
StyleParagraphPropertiesElement.BorderBottom,
StyleParagraphPropertiesElement.BorderLeft,
StyleParagraphPropertiesElement.BorderRight,
StyleParagraphPropertiesElement.BorderTop,
StyleParagraphPropertiesElement.BreakAfter,
StyleParagraphPropertiesElement.BreakBefore,
StyleParagraphPropertiesElement.HyphenationKeep,
StyleParagraphPropertiesElement.HyphenationLadderCount,
StyleParagraphPropertiesElement.KeepTogether,
StyleParagraphPropertiesElement.KeepWithNext,
StyleParagraphPropertiesElement.LineHeight,
StyleParagraphPropertiesElement.Margin,
StyleParagraphPropertiesElement.MarginBottom,
StyleParagraphPropertiesElement.MarginLeft,
StyleParagraphPropertiesElement.MarginRight,
StyleParagraphPropertiesElement.MarginTop,
StyleParagraphPropertiesElement.Orphans,
StyleParagraphPropertiesElement.Padding,
StyleParagraphPropertiesElement.PaddingBottom,
StyleParagraphPropertiesElement.PaddingLeft,
StyleParagraphPropertiesElement.PaddingRight,
StyleParagraphPropertiesElement.PaddingTop,
StyleParagraphPropertiesElement.TextAlign,
StyleParagraphPropertiesElement.TextAlignLast,
StyleParagraphPropertiesElement.TextIndent,
StyleParagraphPropertiesElement.Widows,
StyleParagraphPropertiesElement.AutoTextIndent,
StyleParagraphPropertiesElement.BackgroundTransparency,
StyleParagraphPropertiesElement.BorderLineWidth,
StyleParagraphPropertiesElement.BorderLineWidthBottom,
StyleParagraphPropertiesElement.BorderLineWidthLeft,
StyleParagraphPropertiesElement.BorderLineWidthRight,
StyleParagraphPropertiesElement.BorderLineWidthTop,
StyleParagraphPropertiesElement.FontIndependentLineSpacing,
StyleParagraphPropertiesElement.JoinBorder,
StyleParagraphPropertiesElement.JustifySingleWord,
StyleParagraphPropertiesElement.LineBreak,
StyleParagraphPropertiesElement.LineHeightAtLeast,
StyleParagraphPropertiesElement.LineSpacing,
StyleParagraphPropertiesElement.PageNumber,
StyleParagraphPropertiesElement.PunctuationWrap,
StyleParagraphPropertiesElement.RegisterTrue,
StyleParagraphPropertiesElement.Shadow,
StyleParagraphPropertiesElement.SnapToLayoutGrid,
StyleParagraphPropertiesElement.TabStopDistance,
StyleParagraphPropertiesElement.TextAutospace,
StyleParagraphPropertiesElement.VerticalAlign,
StyleParagraphPropertiesElement.WritingMode,
StyleParagraphPropertiesElement.WritingModeAutomatic,
StyleParagraphPropertiesElement.LineNumber,
StyleParagraphPropertiesElement.NumberLines,
StyleRubyPropertiesElement.RubyAlign,
StyleRubyPropertiesElement.RubyPosition,
StyleSectionPropertiesElement.BackgroundColor,
StyleSectionPropertiesElement.MarginLeft,
StyleSectionPropertiesElement.MarginRight,
StyleSectionPropertiesElement.Editable,
StyleSectionPropertiesElement.Protect,
StyleSectionPropertiesElement.WritingMode,
StyleSectionPropertiesElement.DontBalanceTextColumns,
StyleTableCellPropertiesElement.BackgroundColor,
StyleTableCellPropertiesElement.Border,
StyleTableCellPropertiesElement.BorderBottom,
StyleTableCellPropertiesElement.BorderLeft,
StyleTableCellPropertiesElement.BorderRight,
StyleTableCellPropertiesElement.BorderTop,
StyleTableCellPropertiesElement.Padding,
StyleTableCellPropertiesElement.PaddingBottom,
StyleTableCellPropertiesElement.PaddingLeft,
StyleTableCellPropertiesElement.PaddingRight,
StyleTableCellPropertiesElement.PaddingTop,
StyleTableCellPropertiesElement.WrapOption,
StyleTableCellPropertiesElement.BorderLineWidth,
StyleTableCellPropertiesElement.BorderLineWidthBottom,
StyleTableCellPropertiesElement.BorderLineWidthLeft,
StyleTableCellPropertiesElement.BorderLineWidthRight,
StyleTableCellPropertiesElement.BorderLineWidthTop,
StyleTableCellPropertiesElement.CellProtect,
StyleTableCellPropertiesElement.DecimalPlaces,
StyleTableCellPropertiesElement.DiagonalBlTr,
StyleTableCellPropertiesElement.DiagonalBlTrWidths,
StyleTableCellPropertiesElement.DiagonalTlBr,
StyleTableCellPropertiesElement.DiagonalTlBrWidths,
StyleTableCellPropertiesElement.Direction,
StyleTableCellPropertiesElement.GlyphOrientationVertical,
StyleTableCellPropertiesElement.PrintContent,
StyleTableCellPropertiesElement.RepeatContent,
StyleTableCellPropertiesElement.RotationAlign,
StyleTableCellPropertiesElement.RotationAngle,
StyleTableCellPropertiesElement.Shadow,
StyleTableCellPropertiesElement.ShrinkToFit,
StyleTableCellPropertiesElement.TextAlignSource,
StyleTableCellPropertiesElement.VerticalAlign,
StyleTableCellPropertiesElement.WritingMode,
StyleTableColumnPropertiesElement.BreakAfter,
StyleTableColumnPropertiesElement.BreakBefore,
StyleTableColumnPropertiesElement.ColumnWidth,
StyleTableColumnPropertiesElement.RelColumnWidth,
StyleTableColumnPropertiesElement.UseOptimalColumnWidth,
StyleTablePropertiesElement.BackgroundColor,
StyleTablePropertiesElement.BreakAfter,
StyleTablePropertiesElement.BreakBefore,
StyleTablePropertiesElement.KeepWithNext,
StyleTablePropertiesElement.Margin,
StyleTablePropertiesElement.MarginBottom,
StyleTablePropertiesElement.MarginLeft,
StyleTablePropertiesElement.MarginRight,
StyleTablePropertiesElement.MarginTop,
StyleTablePropertiesElement.MayBreakBetweenRows,
StyleTablePropertiesElement.PageNumber,
StyleTablePropertiesElement.RelWidth,
StyleTablePropertiesElement.Shadow,
StyleTablePropertiesElement.Width,
StyleTablePropertiesElement.WritingMode,
StyleTablePropertiesElement.Align,
StyleTablePropertiesElement.BorderModel,
StyleTablePropertiesElement.Display,
StyleTableRowPropertiesElement.BackgroundColor,
StyleTableRowPropertiesElement.BreakAfter,
StyleTableRowPropertiesElement.BreakBefore,
StyleTableRowPropertiesElement.KeepTogether,
StyleTableRowPropertiesElement.MinRowHeight,
StyleTableRowPropertiesElement.RowHeight,
StyleTableRowPropertiesElement.UseOptimalRowHeight,
StyleTextPropertiesElement.BackgroundColor,
StyleTextPropertiesElement.Color,
StyleTextPropertiesElement.Country,
StyleTextPropertiesElement.FontFamily,
StyleTextPropertiesElement.FontSize,
StyleTextPropertiesElement.FontStyle,
StyleTextPropertiesElement.FontVariant,
StyleTextPropertiesElement.FontWeight,
StyleTextPropertiesElement.Hyphenate,
StyleTextPropertiesElement.HyphenationPushCharCount,
StyleTextPropertiesElement.HyphenationRemainCharCount,
StyleTextPropertiesElement.Language,
StyleTextPropertiesElement.LetterSpacing,
StyleTextPropertiesElement.Script,
StyleTextPropertiesElement.TextShadow,
StyleTextPropertiesElement.TextTransform,
StyleTextPropertiesElement.CountryAsian,
StyleTextPropertiesElement.CountryComplex,
StyleTextPropertiesElement.FontCharset,
StyleTextPropertiesElement.FontCharsetAsian,
StyleTextPropertiesElement.FontCharsetComplex,
StyleTextPropertiesElement.FontFamilyAsian,
StyleTextPropertiesElement.FontFamilyComplex,
StyleTextPropertiesElement.FontFamilyGeneric,
StyleTextPropertiesElement.FontFamilyGenericAsian,
StyleTextPropertiesElement.FontFamilyGenericComplex,
StyleTextPropertiesElement.FontName,
StyleTextPropertiesElement.FontNameAsian,
StyleTextPropertiesElement.FontNameComplex,
StyleTextPropertiesElement.FontPitch,
StyleTextPropertiesElement.FontPitchAsian,
StyleTextPropertiesElement.FontPitchComplex,
StyleTextPropertiesElement.FontRelief,
StyleTextPropertiesElement.FontSizeAsian,
StyleTextPropertiesElement.FontSizeComplex,
StyleTextPropertiesElement.FontSizeRel,
StyleTextPropertiesElement.FontSizeRelAsian,
StyleTextPropertiesElement.FontSizeRelComplex,
StyleTextPropertiesElement.FontStyleAsian,
StyleTextPropertiesElement.FontStyleComplex,
StyleTextPropertiesElement.FontStyleName,
StyleTextPropertiesElement.FontStyleNameAsian,
StyleTextPropertiesElement.FontStyleNameComplex,
StyleTextPropertiesElement.FontWeightAsian,
StyleTextPropertiesElement.FontWeightComplex,
StyleTextPropertiesElement.LanguageAsian,
StyleTextPropertiesElement.LanguageComplex,
StyleTextPropertiesElement.LetterKerning,
StyleTextPropertiesElement.RfcLanguageTag,
StyleTextPropertiesElement.RfcLanguageTagAsian,
StyleTextPropertiesElement.RfcLanguageTagComplex,
StyleTextPropertiesElement.ScriptAsian,
StyleTextPropertiesElement.ScriptComplex,
StyleTextPropertiesElement.ScriptType,
StyleTextPropertiesElement.TextBlinking,
StyleTextPropertiesElement.TextCombine,
StyleTextPropertiesElement.TextCombineEndChar,
StyleTextPropertiesElement.TextCombineStartChar,
StyleTextPropertiesElement.TextEmphasize,
StyleTextPropertiesElement.TextLineThroughColor,
StyleTextPropertiesElement.TextLineThroughMode,
StyleTextPropertiesElement.TextLineThroughStyle,
StyleTextPropertiesElement.TextLineThroughText,
StyleTextPropertiesElement.TextLineThroughTextStyle,
StyleTextPropertiesElement.TextLineThroughType,
StyleTextPropertiesElement.TextLineThroughWidth,
StyleTextPropertiesElement.TextOutline,
StyleTextPropertiesElement.TextOverlineColor,
StyleTextPropertiesElement.TextOverlineMode,
StyleTextPropertiesElement.TextOverlineStyle,
StyleTextPropertiesElement.TextOverlineType,
StyleTextPropertiesElement.TextOverlineWidth,
StyleTextPropertiesElement.TextPosition,
StyleTextPropertiesElement.TextRotationAngle,
StyleTextPropertiesElement.TextRotationScale,
StyleTextPropertiesElement.TextScale,
StyleTextPropertiesElement.TextUnderlineColor,
StyleTextPropertiesElement.TextUnderlineMode,
StyleTextPropertiesElement.TextUnderlineStyle,
StyleTextPropertiesElement.TextUnderlineType,
StyleTextPropertiesElement.TextUnderlineWidth,
StyleTextPropertiesElement.UseWindowFontColor,
StyleTextPropertiesElement.Condition,
StyleTextPropertiesElement.Display,
StyleTextPropertiesElement.Display,
StyleTextPropertiesElement.Display,
});
public final static OdfStyleFamily TableColumn = new OdfStyleFamily("table-column",
new OdfStyleProperty[]{
StyleChartPropertiesElement.AngleOffset,
StyleChartPropertiesElement.AutoPosition,
StyleChartPropertiesElement.AutoSize,
StyleChartPropertiesElement.AxisLabelPosition,
StyleChartPropertiesElement.AxisPosition,
StyleChartPropertiesElement.ConnectBars,
StyleChartPropertiesElement.DataLabelNumber,
StyleChartPropertiesElement.DataLabelSymbol,
StyleChartPropertiesElement.DataLabelText,
StyleChartPropertiesElement.Deep,
StyleChartPropertiesElement.DisplayLabel,
StyleChartPropertiesElement.ErrorCategory,
StyleChartPropertiesElement.ErrorLowerIndicator,
StyleChartPropertiesElement.ErrorLowerLimit,
StyleChartPropertiesElement.ErrorLowerRange,
StyleChartPropertiesElement.ErrorMargin,
StyleChartPropertiesElement.ErrorPercentage,
StyleChartPropertiesElement.ErrorUpperIndicator,
StyleChartPropertiesElement.ErrorUpperLimit,
StyleChartPropertiesElement.ErrorUpperRange,
StyleChartPropertiesElement.GapWidth,
StyleChartPropertiesElement.GroupBarsPerAxis,
StyleChartPropertiesElement.HoleSize,
StyleChartPropertiesElement.IncludeHiddenCells,
StyleChartPropertiesElement.Interpolation,
StyleChartPropertiesElement.IntervalMajor,
StyleChartPropertiesElement.IntervalMinorDivisor,
StyleChartPropertiesElement.JapaneseCandleStick,
StyleChartPropertiesElement.LabelArrangement,
StyleChartPropertiesElement.LabelPosition,
StyleChartPropertiesElement.LabelPositionNegative,
StyleChartPropertiesElement.Lines,
StyleChartPropertiesElement.LinkDataStyleToSource,
StyleChartPropertiesElement.Logarithmic,
StyleChartPropertiesElement.Maximum,
StyleChartPropertiesElement.MeanValue,
StyleChartPropertiesElement.Minimum,
StyleChartPropertiesElement.Origin,
StyleChartPropertiesElement.Overlap,
StyleChartPropertiesElement.Percentage,
StyleChartPropertiesElement.PieOffset,
StyleChartPropertiesElement.RegressionType,
StyleChartPropertiesElement.ReverseDirection,
StyleChartPropertiesElement.RightAngledAxes,
StyleChartPropertiesElement.ScaleText,
StyleChartPropertiesElement.SeriesSource,
StyleChartPropertiesElement.SolidType,
StyleChartPropertiesElement.SortByXValues,
StyleChartPropertiesElement.SplineOrder,
StyleChartPropertiesElement.SplineResolution,
StyleChartPropertiesElement.Stacked,
StyleChartPropertiesElement.SymbolHeight,
StyleChartPropertiesElement.SymbolName,
StyleChartPropertiesElement.SymbolType,
StyleChartPropertiesElement.SymbolType,
StyleChartPropertiesElement.SymbolType,
StyleChartPropertiesElement.SymbolType,
StyleChartPropertiesElement.SymbolWidth,
StyleChartPropertiesElement.TextOverlap,
StyleChartPropertiesElement.ThreeDimensional,
StyleChartPropertiesElement.TickMarkPosition,
StyleChartPropertiesElement.TickMarksMajorInner,
StyleChartPropertiesElement.TickMarksMajorOuter,
StyleChartPropertiesElement.TickMarksMinorInner,
StyleChartPropertiesElement.TickMarksMinorOuter,
StyleChartPropertiesElement.TreatEmptyCells,
StyleChartPropertiesElement.Vertical,
StyleChartPropertiesElement.Visible,
StyleChartPropertiesElement.Direction,
StyleChartPropertiesElement.RotationAngle,
StyleChartPropertiesElement.LineBreak,
StyleDrawingPagePropertiesElement.BackgroundSize,
StyleDrawingPagePropertiesElement.Fill,
StyleDrawingPagePropertiesElement.FillColor,
StyleDrawingPagePropertiesElement.FillGradientName,
StyleDrawingPagePropertiesElement.FillHatchName,
StyleDrawingPagePropertiesElement.FillHatchSolid,
StyleDrawingPagePropertiesElement.FillImageHeight,
StyleDrawingPagePropertiesElement.FillImageName,
StyleDrawingPagePropertiesElement.FillImageRefPoint,
StyleDrawingPagePropertiesElement.FillImageRefPointX,
StyleDrawingPagePropertiesElement.FillImageRefPointY,
StyleDrawingPagePropertiesElement.FillImageWidth,
StyleDrawingPagePropertiesElement.GradientStepCount,
StyleDrawingPagePropertiesElement.Opacity,
StyleDrawingPagePropertiesElement.OpacityName,
StyleDrawingPagePropertiesElement.SecondaryFillColor,
StyleDrawingPagePropertiesElement.TileRepeatOffset,
StyleDrawingPagePropertiesElement.BackgroundObjectsVisible,
StyleDrawingPagePropertiesElement.BackgroundVisible,
StyleDrawingPagePropertiesElement.DisplayDateTime,
StyleDrawingPagePropertiesElement.DisplayFooter,
StyleDrawingPagePropertiesElement.DisplayHeader,
StyleDrawingPagePropertiesElement.DisplayPageNumber,
StyleDrawingPagePropertiesElement.Duration,
StyleDrawingPagePropertiesElement.TransitionSpeed,
StyleDrawingPagePropertiesElement.TransitionStyle,
StyleDrawingPagePropertiesElement.TransitionType,
StyleDrawingPagePropertiesElement.Visibility,
StyleDrawingPagePropertiesElement.Direction,
StyleDrawingPagePropertiesElement.FadeColor,
StyleDrawingPagePropertiesElement.Subtype,
StyleDrawingPagePropertiesElement.Type,
StyleDrawingPagePropertiesElement.Repeat,
StyleDrawingPagePropertiesElement.FillRule,
StyleGraphicPropertiesElement.AmbientColor,
StyleGraphicPropertiesElement.BackScale,
StyleGraphicPropertiesElement.BackfaceCulling,
StyleGraphicPropertiesElement.CloseBack,
StyleGraphicPropertiesElement.CloseFront,
StyleGraphicPropertiesElement.Depth,
StyleGraphicPropertiesElement.DiffuseColor,
StyleGraphicPropertiesElement.EdgeRounding,
StyleGraphicPropertiesElement.EdgeRoundingMode,
StyleGraphicPropertiesElement.EmissiveColor,
StyleGraphicPropertiesElement.EndAngle,
StyleGraphicPropertiesElement.HorizontalSegments,
StyleGraphicPropertiesElement.LightingMode,
StyleGraphicPropertiesElement.NormalsDirection,
StyleGraphicPropertiesElement.NormalsKind,
StyleGraphicPropertiesElement.Dr3dShadow,
StyleGraphicPropertiesElement.Shininess,
StyleGraphicPropertiesElement.SpecularColor,
StyleGraphicPropertiesElement.TextureFilter,
StyleGraphicPropertiesElement.TextureGenerationModeX,
StyleGraphicPropertiesElement.TextureGenerationModeY,
StyleGraphicPropertiesElement.TextureKind,
StyleGraphicPropertiesElement.TextureMode,
StyleGraphicPropertiesElement.VerticalSegments,
StyleGraphicPropertiesElement.AutoGrowHeight,
StyleGraphicPropertiesElement.AutoGrowWidth,
StyleGraphicPropertiesElement.Blue,
StyleGraphicPropertiesElement.CaptionAngle,
StyleGraphicPropertiesElement.CaptionAngleType,
StyleGraphicPropertiesElement.CaptionEscape,
StyleGraphicPropertiesElement.CaptionEscapeDirection,
StyleGraphicPropertiesElement.CaptionFitLineLength,
StyleGraphicPropertiesElement.CaptionGap,
StyleGraphicPropertiesElement.CaptionLineLength,
StyleGraphicPropertiesElement.CaptionType,
StyleGraphicPropertiesElement.ColorInversion,
StyleGraphicPropertiesElement.ColorMode,
StyleGraphicPropertiesElement.Contrast,
StyleGraphicPropertiesElement.DecimalPlaces,
StyleGraphicPropertiesElement.DrawAspect,
StyleGraphicPropertiesElement.EndGuide,
StyleGraphicPropertiesElement.EndLineSpacingHorizontal,
StyleGraphicPropertiesElement.EndLineSpacingVertical,
StyleGraphicPropertiesElement.Fill,
StyleGraphicPropertiesElement.FillColor,
StyleGraphicPropertiesElement.FillGradientName,
StyleGraphicPropertiesElement.FillHatchName,
StyleGraphicPropertiesElement.FillHatchSolid,
StyleGraphicPropertiesElement.FillImageHeight,
StyleGraphicPropertiesElement.FillImageName,
StyleGraphicPropertiesElement.FillImageRefPoint,
StyleGraphicPropertiesElement.FillImageRefPointX,
StyleGraphicPropertiesElement.FillImageRefPointY,
StyleGraphicPropertiesElement.FillImageWidth,
StyleGraphicPropertiesElement.FitToContour,
StyleGraphicPropertiesElement.FitToSize,
StyleGraphicPropertiesElement.FrameDisplayBorder,
StyleGraphicPropertiesElement.FrameDisplayScrollbar,
StyleGraphicPropertiesElement.FrameMarginHorizontal,
StyleGraphicPropertiesElement.FrameMarginVertical,
StyleGraphicPropertiesElement.Gamma,
StyleGraphicPropertiesElement.GradientStepCount,
StyleGraphicPropertiesElement.Green,
StyleGraphicPropertiesElement.GuideDistance,
StyleGraphicPropertiesElement.GuideOverhang,
StyleGraphicPropertiesElement.ImageOpacity,
StyleGraphicPropertiesElement.LineDistance,
StyleGraphicPropertiesElement.Luminance,
StyleGraphicPropertiesElement.MarkerEnd,
StyleGraphicPropertiesElement.MarkerEndCenter,
StyleGraphicPropertiesElement.MarkerEndWidth,
StyleGraphicPropertiesElement.MarkerStart,
StyleGraphicPropertiesElement.MarkerStartCenter,
StyleGraphicPropertiesElement.MarkerStartWidth,
StyleGraphicPropertiesElement.MeasureAlign,
StyleGraphicPropertiesElement.MeasureVerticalAlign,
StyleGraphicPropertiesElement.OleDrawAspect,
StyleGraphicPropertiesElement.Opacity,
StyleGraphicPropertiesElement.OpacityName,
StyleGraphicPropertiesElement.Parallel,
StyleGraphicPropertiesElement.Placing,
StyleGraphicPropertiesElement.Red,
StyleGraphicPropertiesElement.SecondaryFillColor,
StyleGraphicPropertiesElement.DrawShadow,
StyleGraphicPropertiesElement.ShadowColor,
StyleGraphicPropertiesElement.ShadowOffsetX,
StyleGraphicPropertiesElement.ShadowOffsetY,
StyleGraphicPropertiesElement.ShadowOpacity,
StyleGraphicPropertiesElement.ShowUnit,
StyleGraphicPropertiesElement.StartGuide,
StyleGraphicPropertiesElement.StartLineSpacingHorizontal,
StyleGraphicPropertiesElement.StartLineSpacingVertical,
StyleGraphicPropertiesElement.Stroke,
StyleGraphicPropertiesElement.StrokeDash,
StyleGraphicPropertiesElement.StrokeDashNames,
StyleGraphicPropertiesElement.StrokeLinejoin,
StyleGraphicPropertiesElement.SymbolColor,
StyleGraphicPropertiesElement.TextareaHorizontalAlign,
StyleGraphicPropertiesElement.TextareaVerticalAlign,
StyleGraphicPropertiesElement.TileRepeatOffset,
StyleGraphicPropertiesElement.Unit,
StyleGraphicPropertiesElement.VisibleAreaHeight,
StyleGraphicPropertiesElement.VisibleAreaLeft,
StyleGraphicPropertiesElement.VisibleAreaTop,
StyleGraphicPropertiesElement.VisibleAreaWidth,
StyleGraphicPropertiesElement.WrapInfluenceOnPosition,
StyleGraphicPropertiesElement.BackgroundColor,
StyleGraphicPropertiesElement.Border,
StyleGraphicPropertiesElement.BorderBottom,
StyleGraphicPropertiesElement.BorderLeft,
StyleGraphicPropertiesElement.BorderRight,
StyleGraphicPropertiesElement.BorderTop,
StyleGraphicPropertiesElement.Clip,
StyleGraphicPropertiesElement.Margin,
StyleGraphicPropertiesElement.MarginBottom,
StyleGraphicPropertiesElement.MarginLeft,
StyleGraphicPropertiesElement.MarginRight,
StyleGraphicPropertiesElement.MarginTop,
StyleGraphicPropertiesElement.MaxHeight,
StyleGraphicPropertiesElement.MaxWidth,
StyleGraphicPropertiesElement.MinHeight,
StyleGraphicPropertiesElement.MinWidth,
StyleGraphicPropertiesElement.Padding,
StyleGraphicPropertiesElement.PaddingBottom,
StyleGraphicPropertiesElement.PaddingLeft,
StyleGraphicPropertiesElement.PaddingRight,
StyleGraphicPropertiesElement.PaddingTop,
StyleGraphicPropertiesElement.WrapOption,
StyleGraphicPropertiesElement.BackgroundTransparency,
StyleGraphicPropertiesElement.BorderLineWidth,
StyleGraphicPropertiesElement.BorderLineWidthBottom,
StyleGraphicPropertiesElement.BorderLineWidthLeft,
StyleGraphicPropertiesElement.BorderLineWidthRight,
StyleGraphicPropertiesElement.BorderLineWidthTop,
StyleGraphicPropertiesElement.Editable,
StyleGraphicPropertiesElement.FlowWithText,
StyleGraphicPropertiesElement.HorizontalPos,
StyleGraphicPropertiesElement.HorizontalRel,
StyleGraphicPropertiesElement.Mirror,
StyleGraphicPropertiesElement.NumberWrappedParagraphs,
StyleGraphicPropertiesElement.OverflowBehavior,
StyleGraphicPropertiesElement.PrintContent,
StyleGraphicPropertiesElement.Protect,
StyleGraphicPropertiesElement.RelHeight,
StyleGraphicPropertiesElement.RelWidth,
StyleGraphicPropertiesElement.Repeat,
StyleGraphicPropertiesElement.RunThrough,
StyleGraphicPropertiesElement.StyleShadow,
StyleGraphicPropertiesElement.ShrinkToFit,
StyleGraphicPropertiesElement.VerticalPos,
StyleGraphicPropertiesElement.VerticalRel,
StyleGraphicPropertiesElement.Wrap,
StyleGraphicPropertiesElement.WrapContour,
StyleGraphicPropertiesElement.WrapContourMode,
StyleGraphicPropertiesElement.WrapDynamicThreshold,
StyleGraphicPropertiesElement.WritingMode,
StyleGraphicPropertiesElement.FillRule,
StyleGraphicPropertiesElement.Height,
StyleGraphicPropertiesElement.StrokeColor,
StyleGraphicPropertiesElement.StrokeLinecap,
StyleGraphicPropertiesElement.StrokeOpacity,
StyleGraphicPropertiesElement.StrokeWidth,
StyleGraphicPropertiesElement.Width,
StyleGraphicPropertiesElement.X,
StyleGraphicPropertiesElement.Y,
StyleGraphicPropertiesElement.AnchorPageNumber,
StyleGraphicPropertiesElement.AnchorType,
StyleGraphicPropertiesElement.Animation,
StyleGraphicPropertiesElement.AnimationDelay,
StyleGraphicPropertiesElement.AnimationDirection,
StyleGraphicPropertiesElement.AnimationRepeat,
StyleGraphicPropertiesElement.AnimationStartInside,
StyleGraphicPropertiesElement.AnimationSteps,
StyleGraphicPropertiesElement.AnimationStopInside,
StyleHeaderFooterPropertiesElement.BackgroundColor,
StyleHeaderFooterPropertiesElement.Border,
StyleHeaderFooterPropertiesElement.BorderBottom,
StyleHeaderFooterPropertiesElement.BorderLeft,
StyleHeaderFooterPropertiesElement.BorderRight,
StyleHeaderFooterPropertiesElement.BorderTop,
StyleHeaderFooterPropertiesElement.Margin,
StyleHeaderFooterPropertiesElement.MarginBottom,
StyleHeaderFooterPropertiesElement.MarginLeft,
StyleHeaderFooterPropertiesElement.MarginRight,
StyleHeaderFooterPropertiesElement.MarginTop,
StyleHeaderFooterPropertiesElement.MinHeight,
StyleHeaderFooterPropertiesElement.Padding,
StyleHeaderFooterPropertiesElement.PaddingBottom,
StyleHeaderFooterPropertiesElement.PaddingLeft,
StyleHeaderFooterPropertiesElement.PaddingRight,
StyleHeaderFooterPropertiesElement.PaddingTop,
StyleHeaderFooterPropertiesElement.BorderLineWidth,
StyleHeaderFooterPropertiesElement.BorderLineWidthBottom,
StyleHeaderFooterPropertiesElement.BorderLineWidthLeft,
StyleHeaderFooterPropertiesElement.BorderLineWidthRight,
StyleHeaderFooterPropertiesElement.BorderLineWidthTop,
StyleHeaderFooterPropertiesElement.DynamicSpacing,
StyleHeaderFooterPropertiesElement.Shadow,
StyleHeaderFooterPropertiesElement.Height,
StyleListLevelPropertiesElement.Height,
StyleListLevelPropertiesElement.TextAlign,
StyleListLevelPropertiesElement.Width,
StyleListLevelPropertiesElement.FontName,
StyleListLevelPropertiesElement.VerticalPos,
StyleListLevelPropertiesElement.VerticalRel,
StyleListLevelPropertiesElement.Y,
StyleListLevelPropertiesElement.ListLevelPositionAndSpaceMode,
StyleListLevelPropertiesElement.MinLabelDistance,
StyleListLevelPropertiesElement.MinLabelWidth,
StyleListLevelPropertiesElement.SpaceBefore,
StylePageLayoutPropertiesElement.BackgroundColor,
StylePageLayoutPropertiesElement.Border,
StylePageLayoutPropertiesElement.BorderBottom,
StylePageLayoutPropertiesElement.BorderLeft,
StylePageLayoutPropertiesElement.BorderRight,
StylePageLayoutPropertiesElement.BorderTop,
StylePageLayoutPropertiesElement.Margin,
StylePageLayoutPropertiesElement.MarginBottom,
StylePageLayoutPropertiesElement.MarginLeft,
StylePageLayoutPropertiesElement.MarginRight,
StylePageLayoutPropertiesElement.MarginTop,
StylePageLayoutPropertiesElement.Padding,
StylePageLayoutPropertiesElement.PaddingBottom,
StylePageLayoutPropertiesElement.PaddingLeft,
StylePageLayoutPropertiesElement.PaddingRight,
StylePageLayoutPropertiesElement.PaddingTop,
StylePageLayoutPropertiesElement.PageHeight,
StylePageLayoutPropertiesElement.PageWidth,
StylePageLayoutPropertiesElement.BorderLineWidth,
StylePageLayoutPropertiesElement.BorderLineWidthBottom,
StylePageLayoutPropertiesElement.BorderLineWidthLeft,
StylePageLayoutPropertiesElement.BorderLineWidthRight,
StylePageLayoutPropertiesElement.BorderLineWidthTop,
StylePageLayoutPropertiesElement.FirstPageNumber,
StylePageLayoutPropertiesElement.FootnoteMaxHeight,
StylePageLayoutPropertiesElement.LayoutGridBaseHeight,
StylePageLayoutPropertiesElement.LayoutGridBaseWidth,
StylePageLayoutPropertiesElement.LayoutGridColor,
StylePageLayoutPropertiesElement.LayoutGridDisplay,
StylePageLayoutPropertiesElement.LayoutGridLines,
StylePageLayoutPropertiesElement.LayoutGridMode,
StylePageLayoutPropertiesElement.LayoutGridPrint,
StylePageLayoutPropertiesElement.LayoutGridRubyBelow,
StylePageLayoutPropertiesElement.LayoutGridRubyHeight,
StylePageLayoutPropertiesElement.LayoutGridSnapTo,
StylePageLayoutPropertiesElement.LayoutGridStandardMode,
StylePageLayoutPropertiesElement.NumFormat,
StylePageLayoutPropertiesElement.NumFormat,
StylePageLayoutPropertiesElement.NumLetterSync,
StylePageLayoutPropertiesElement.NumPrefix,
StylePageLayoutPropertiesElement.NumSuffix,
StylePageLayoutPropertiesElement.PaperTrayName,
StylePageLayoutPropertiesElement.Print,
StylePageLayoutPropertiesElement.PrintOrientation,
StylePageLayoutPropertiesElement.PrintPageOrder,
StylePageLayoutPropertiesElement.RegisterTruthRefStyleName,
StylePageLayoutPropertiesElement.ScaleTo,
StylePageLayoutPropertiesElement.ScaleToPages,
StylePageLayoutPropertiesElement.Shadow,
StylePageLayoutPropertiesElement.TableCentering,
StylePageLayoutPropertiesElement.WritingMode,
StyleParagraphPropertiesElement.BackgroundColor,
StyleParagraphPropertiesElement.Border,
StyleParagraphPropertiesElement.BorderBottom,
StyleParagraphPropertiesElement.BorderLeft,
StyleParagraphPropertiesElement.BorderRight,
StyleParagraphPropertiesElement.BorderTop,
StyleParagraphPropertiesElement.BreakAfter,
StyleParagraphPropertiesElement.BreakBefore,
StyleParagraphPropertiesElement.HyphenationKeep,
StyleParagraphPropertiesElement.HyphenationLadderCount,
StyleParagraphPropertiesElement.KeepTogether,
StyleParagraphPropertiesElement.KeepWithNext,
StyleParagraphPropertiesElement.LineHeight,
StyleParagraphPropertiesElement.Margin,
StyleParagraphPropertiesElement.MarginBottom,
StyleParagraphPropertiesElement.MarginLeft,
StyleParagraphPropertiesElement.MarginRight,
StyleParagraphPropertiesElement.MarginTop,
StyleParagraphPropertiesElement.Orphans,
StyleParagraphPropertiesElement.Padding,
StyleParagraphPropertiesElement.PaddingBottom,
StyleParagraphPropertiesElement.PaddingLeft,
StyleParagraphPropertiesElement.PaddingRight,
StyleParagraphPropertiesElement.PaddingTop,
StyleParagraphPropertiesElement.TextAlign,
StyleParagraphPropertiesElement.TextAlignLast,
StyleParagraphPropertiesElement.TextIndent,
StyleParagraphPropertiesElement.Widows,
StyleParagraphPropertiesElement.AutoTextIndent,
StyleParagraphPropertiesElement.BackgroundTransparency,
StyleParagraphPropertiesElement.BorderLineWidth,
StyleParagraphPropertiesElement.BorderLineWidthBottom,
StyleParagraphPropertiesElement.BorderLineWidthLeft,
StyleParagraphPropertiesElement.BorderLineWidthRight,
StyleParagraphPropertiesElement.BorderLineWidthTop,
StyleParagraphPropertiesElement.FontIndependentLineSpacing,
StyleParagraphPropertiesElement.JoinBorder,
StyleParagraphPropertiesElement.JustifySingleWord,
StyleParagraphPropertiesElement.LineBreak,
StyleParagraphPropertiesElement.LineHeightAtLeast,
StyleParagraphPropertiesElement.LineSpacing,
StyleParagraphPropertiesElement.PageNumber,
StyleParagraphPropertiesElement.PunctuationWrap,
StyleParagraphPropertiesElement.RegisterTrue,
StyleParagraphPropertiesElement.Shadow,
StyleParagraphPropertiesElement.SnapToLayoutGrid,
StyleParagraphPropertiesElement.TabStopDistance,
StyleParagraphPropertiesElement.TextAutospace,
StyleParagraphPropertiesElement.VerticalAlign,
StyleParagraphPropertiesElement.WritingMode,
StyleParagraphPropertiesElement.WritingModeAutomatic,
StyleParagraphPropertiesElement.LineNumber,
StyleParagraphPropertiesElement.NumberLines,
StyleRubyPropertiesElement.RubyAlign,
StyleRubyPropertiesElement.RubyPosition,
StyleSectionPropertiesElement.BackgroundColor,
StyleSectionPropertiesElement.MarginLeft,
StyleSectionPropertiesElement.MarginRight,
StyleSectionPropertiesElement.Editable,
StyleSectionPropertiesElement.Protect,
StyleSectionPropertiesElement.WritingMode,
StyleSectionPropertiesElement.DontBalanceTextColumns,
StyleTableCellPropertiesElement.BackgroundColor,
StyleTableCellPropertiesElement.Border,
StyleTableCellPropertiesElement.BorderBottom,
StyleTableCellPropertiesElement.BorderLeft,
StyleTableCellPropertiesElement.BorderRight,
StyleTableCellPropertiesElement.BorderTop,
StyleTableCellPropertiesElement.Padding,
StyleTableCellPropertiesElement.PaddingBottom,
StyleTableCellPropertiesElement.PaddingLeft,
StyleTableCellPropertiesElement.PaddingRight,
StyleTableCellPropertiesElement.PaddingTop,
StyleTableCellPropertiesElement.WrapOption,
StyleTableCellPropertiesElement.BorderLineWidth,
StyleTableCellPropertiesElement.BorderLineWidthBottom,
StyleTableCellPropertiesElement.BorderLineWidthLeft,
StyleTableCellPropertiesElement.BorderLineWidthRight,
StyleTableCellPropertiesElement.BorderLineWidthTop,
StyleTableCellPropertiesElement.CellProtect,
StyleTableCellPropertiesElement.DecimalPlaces,
StyleTableCellPropertiesElement.DiagonalBlTr,
StyleTableCellPropertiesElement.DiagonalBlTrWidths,
StyleTableCellPropertiesElement.DiagonalTlBr,
StyleTableCellPropertiesElement.DiagonalTlBrWidths,
StyleTableCellPropertiesElement.Direction,
StyleTableCellPropertiesElement.GlyphOrientationVertical,
StyleTableCellPropertiesElement.PrintContent,
StyleTableCellPropertiesElement.RepeatContent,
StyleTableCellPropertiesElement.RotationAlign,
StyleTableCellPropertiesElement.RotationAngle,
StyleTableCellPropertiesElement.Shadow,
StyleTableCellPropertiesElement.ShrinkToFit,
StyleTableCellPropertiesElement.TextAlignSource,
StyleTableCellPropertiesElement.VerticalAlign,
StyleTableCellPropertiesElement.WritingMode,
StyleTableColumnPropertiesElement.BreakAfter,
StyleTableColumnPropertiesElement.BreakBefore,
StyleTableColumnPropertiesElement.ColumnWidth,
StyleTableColumnPropertiesElement.RelColumnWidth,
StyleTableColumnPropertiesElement.UseOptimalColumnWidth,
StyleTablePropertiesElement.BackgroundColor,
StyleTablePropertiesElement.BreakAfter,
StyleTablePropertiesElement.BreakBefore,
StyleTablePropertiesElement.KeepWithNext,
StyleTablePropertiesElement.Margin,
StyleTablePropertiesElement.MarginBottom,
StyleTablePropertiesElement.MarginLeft,
StyleTablePropertiesElement.MarginRight,
StyleTablePropertiesElement.MarginTop,
StyleTablePropertiesElement.MayBreakBetweenRows,
StyleTablePropertiesElement.PageNumber,
StyleTablePropertiesElement.RelWidth,
StyleTablePropertiesElement.Shadow,
StyleTablePropertiesElement.Width,
StyleTablePropertiesElement.WritingMode,
StyleTablePropertiesElement.Align,
StyleTablePropertiesElement.BorderModel,
StyleTablePropertiesElement.Display,
StyleTableRowPropertiesElement.BackgroundColor,
StyleTableRowPropertiesElement.BreakAfter,
StyleTableRowPropertiesElement.BreakBefore,
StyleTableRowPropertiesElement.KeepTogether,
StyleTableRowPropertiesElement.MinRowHeight,
StyleTableRowPropertiesElement.RowHeight,
StyleTableRowPropertiesElement.UseOptimalRowHeight,
StyleTextPropertiesElement.BackgroundColor,
StyleTextPropertiesElement.Color,
StyleTextPropertiesElement.Country,
StyleTextPropertiesElement.FontFamily,
StyleTextPropertiesElement.FontSize,
StyleTextPropertiesElement.FontStyle,
StyleTextPropertiesElement.FontVariant,
StyleTextPropertiesElement.FontWeight,
StyleTextPropertiesElement.Hyphenate,
StyleTextPropertiesElement.HyphenationPushCharCount,
StyleTextPropertiesElement.HyphenationRemainCharCount,
StyleTextPropertiesElement.Language,
StyleTextPropertiesElement.LetterSpacing,
StyleTextPropertiesElement.Script,
StyleTextPropertiesElement.TextShadow,
StyleTextPropertiesElement.TextTransform,
StyleTextPropertiesElement.CountryAsian,
StyleTextPropertiesElement.CountryComplex,
StyleTextPropertiesElement.FontCharset,
StyleTextPropertiesElement.FontCharsetAsian,
StyleTextPropertiesElement.FontCharsetComplex,
StyleTextPropertiesElement.FontFamilyAsian,
StyleTextPropertiesElement.FontFamilyComplex,
StyleTextPropertiesElement.FontFamilyGeneric,
StyleTextPropertiesElement.FontFamilyGenericAsian,
StyleTextPropertiesElement.FontFamilyGenericComplex,
StyleTextPropertiesElement.FontName,
StyleTextPropertiesElement.FontNameAsian,
StyleTextPropertiesElement.FontNameComplex,
StyleTextPropertiesElement.FontPitch,
StyleTextPropertiesElement.FontPitchAsian,
StyleTextPropertiesElement.FontPitchComplex,
StyleTextPropertiesElement.FontRelief,
StyleTextPropertiesElement.FontSizeAsian,
StyleTextPropertiesElement.FontSizeComplex,
StyleTextPropertiesElement.FontSizeRel,
StyleTextPropertiesElement.FontSizeRelAsian,
StyleTextPropertiesElement.FontSizeRelComplex,
StyleTextPropertiesElement.FontStyleAsian,
StyleTextPropertiesElement.FontStyleComplex,
StyleTextPropertiesElement.FontStyleName,
StyleTextPropertiesElement.FontStyleNameAsian,
StyleTextPropertiesElement.FontStyleNameComplex,
StyleTextPropertiesElement.FontWeightAsian,
StyleTextPropertiesElement.FontWeightComplex,
StyleTextPropertiesElement.LanguageAsian,
StyleTextPropertiesElement.LanguageComplex,
StyleTextPropertiesElement.LetterKerning,
StyleTextPropertiesElement.RfcLanguageTag,
StyleTextPropertiesElement.RfcLanguageTagAsian,
StyleTextPropertiesElement.RfcLanguageTagComplex,
StyleTextPropertiesElement.ScriptAsian,
StyleTextPropertiesElement.ScriptComplex,
StyleTextPropertiesElement.ScriptType,
StyleTextPropertiesElement.TextBlinking,
StyleTextPropertiesElement.TextCombine,
StyleTextPropertiesElement.TextCombineEndChar,
StyleTextPropertiesElement.TextCombineStartChar,
StyleTextPropertiesElement.TextEmphasize,
StyleTextPropertiesElement.TextLineThroughColor,
StyleTextPropertiesElement.TextLineThroughMode,
StyleTextPropertiesElement.TextLineThroughStyle,
StyleTextPropertiesElement.TextLineThroughText,
StyleTextPropertiesElement.TextLineThroughTextStyle,
StyleTextPropertiesElement.TextLineThroughType,
StyleTextPropertiesElement.TextLineThroughWidth,
StyleTextPropertiesElement.TextOutline,
StyleTextPropertiesElement.TextOverlineColor,
StyleTextPropertiesElement.TextOverlineMode,
StyleTextPropertiesElement.TextOverlineStyle,
StyleTextPropertiesElement.TextOverlineType,
StyleTextPropertiesElement.TextOverlineWidth,
StyleTextPropertiesElement.TextPosition,
StyleTextPropertiesElement.TextRotationAngle,
StyleTextPropertiesElement.TextRotationScale,
StyleTextPropertiesElement.TextScale,
StyleTextPropertiesElement.TextUnderlineColor,
StyleTextPropertiesElement.TextUnderlineMode,
StyleTextPropertiesElement.TextUnderlineStyle,
StyleTextPropertiesElement.TextUnderlineType,
StyleTextPropertiesElement.TextUnderlineWidth,
StyleTextPropertiesElement.UseWindowFontColor,
StyleTextPropertiesElement.Condition,
StyleTextPropertiesElement.Display,
StyleTextPropertiesElement.Display,
StyleTextPropertiesElement.Display,
});
public final static OdfStyleFamily TableRow = new OdfStyleFamily("table-row",
new OdfStyleProperty[]{
StyleChartPropertiesElement.AngleOffset,
StyleChartPropertiesElement.AutoPosition,
StyleChartPropertiesElement.AutoSize,
StyleChartPropertiesElement.AxisLabelPosition,
StyleChartPropertiesElement.AxisPosition,
StyleChartPropertiesElement.ConnectBars,
StyleChartPropertiesElement.DataLabelNumber,
StyleChartPropertiesElement.DataLabelSymbol,
StyleChartPropertiesElement.DataLabelText,
StyleChartPropertiesElement.Deep,
StyleChartPropertiesElement.DisplayLabel,
StyleChartPropertiesElement.ErrorCategory,
StyleChartPropertiesElement.ErrorLowerIndicator,
StyleChartPropertiesElement.ErrorLowerLimit,
StyleChartPropertiesElement.ErrorLowerRange,
StyleChartPropertiesElement.ErrorMargin,
StyleChartPropertiesElement.ErrorPercentage,
StyleChartPropertiesElement.ErrorUpperIndicator,
StyleChartPropertiesElement.ErrorUpperLimit,
StyleChartPropertiesElement.ErrorUpperRange,
StyleChartPropertiesElement.GapWidth,
StyleChartPropertiesElement.GroupBarsPerAxis,
StyleChartPropertiesElement.HoleSize,
StyleChartPropertiesElement.IncludeHiddenCells,
StyleChartPropertiesElement.Interpolation,
StyleChartPropertiesElement.IntervalMajor,
StyleChartPropertiesElement.IntervalMinorDivisor,
StyleChartPropertiesElement.JapaneseCandleStick,
StyleChartPropertiesElement.LabelArrangement,
StyleChartPropertiesElement.LabelPosition,
StyleChartPropertiesElement.LabelPositionNegative,
StyleChartPropertiesElement.Lines,
StyleChartPropertiesElement.LinkDataStyleToSource,
StyleChartPropertiesElement.Logarithmic,
StyleChartPropertiesElement.Maximum,
StyleChartPropertiesElement.MeanValue,
StyleChartPropertiesElement.Minimum,
StyleChartPropertiesElement.Origin,
StyleChartPropertiesElement.Overlap,
StyleChartPropertiesElement.Percentage,
StyleChartPropertiesElement.PieOffset,
StyleChartPropertiesElement.RegressionType,
StyleChartPropertiesElement.ReverseDirection,
StyleChartPropertiesElement.RightAngledAxes,
StyleChartPropertiesElement.ScaleText,
StyleChartPropertiesElement.SeriesSource,
StyleChartPropertiesElement.SolidType,
StyleChartPropertiesElement.SortByXValues,
StyleChartPropertiesElement.SplineOrder,
StyleChartPropertiesElement.SplineResolution,
StyleChartPropertiesElement.Stacked,
StyleChartPropertiesElement.SymbolHeight,
StyleChartPropertiesElement.SymbolName,
StyleChartPropertiesElement.SymbolType,
StyleChartPropertiesElement.SymbolType,
StyleChartPropertiesElement.SymbolType,
StyleChartPropertiesElement.SymbolType,
StyleChartPropertiesElement.SymbolWidth,
StyleChartPropertiesElement.TextOverlap,
StyleChartPropertiesElement.ThreeDimensional,
StyleChartPropertiesElement.TickMarkPosition,
StyleChartPropertiesElement.TickMarksMajorInner,
StyleChartPropertiesElement.TickMarksMajorOuter,
StyleChartPropertiesElement.TickMarksMinorInner,
StyleChartPropertiesElement.TickMarksMinorOuter,
StyleChartPropertiesElement.TreatEmptyCells,
StyleChartPropertiesElement.Vertical,
StyleChartPropertiesElement.Visible,
StyleChartPropertiesElement.Direction,
StyleChartPropertiesElement.RotationAngle,
StyleChartPropertiesElement.LineBreak,
StyleDrawingPagePropertiesElement.BackgroundSize,
StyleDrawingPagePropertiesElement.Fill,
StyleDrawingPagePropertiesElement.FillColor,
StyleDrawingPagePropertiesElement.FillGradientName,
StyleDrawingPagePropertiesElement.FillHatchName,
StyleDrawingPagePropertiesElement.FillHatchSolid,
StyleDrawingPagePropertiesElement.FillImageHeight,
StyleDrawingPagePropertiesElement.FillImageName,
StyleDrawingPagePropertiesElement.FillImageRefPoint,
StyleDrawingPagePropertiesElement.FillImageRefPointX,
StyleDrawingPagePropertiesElement.FillImageRefPointY,
StyleDrawingPagePropertiesElement.FillImageWidth,
StyleDrawingPagePropertiesElement.GradientStepCount,
StyleDrawingPagePropertiesElement.Opacity,
StyleDrawingPagePropertiesElement.OpacityName,
StyleDrawingPagePropertiesElement.SecondaryFillColor,
StyleDrawingPagePropertiesElement.TileRepeatOffset,
StyleDrawingPagePropertiesElement.BackgroundObjectsVisible,
StyleDrawingPagePropertiesElement.BackgroundVisible,
StyleDrawingPagePropertiesElement.DisplayDateTime,
StyleDrawingPagePropertiesElement.DisplayFooter,
StyleDrawingPagePropertiesElement.DisplayHeader,
StyleDrawingPagePropertiesElement.DisplayPageNumber,
StyleDrawingPagePropertiesElement.Duration,
StyleDrawingPagePropertiesElement.TransitionSpeed,
StyleDrawingPagePropertiesElement.TransitionStyle,
StyleDrawingPagePropertiesElement.TransitionType,
StyleDrawingPagePropertiesElement.Visibility,
StyleDrawingPagePropertiesElement.Direction,
StyleDrawingPagePropertiesElement.FadeColor,
StyleDrawingPagePropertiesElement.Subtype,
StyleDrawingPagePropertiesElement.Type,
StyleDrawingPagePropertiesElement.Repeat,
StyleDrawingPagePropertiesElement.FillRule,
StyleGraphicPropertiesElement.AmbientColor,
StyleGraphicPropertiesElement.BackScale,
StyleGraphicPropertiesElement.BackfaceCulling,
StyleGraphicPropertiesElement.CloseBack,
StyleGraphicPropertiesElement.CloseFront,
StyleGraphicPropertiesElement.Depth,
StyleGraphicPropertiesElement.DiffuseColor,
StyleGraphicPropertiesElement.EdgeRounding,
StyleGraphicPropertiesElement.EdgeRoundingMode,
StyleGraphicPropertiesElement.EmissiveColor,
StyleGraphicPropertiesElement.EndAngle,
StyleGraphicPropertiesElement.HorizontalSegments,
StyleGraphicPropertiesElement.LightingMode,
StyleGraphicPropertiesElement.NormalsDirection,
StyleGraphicPropertiesElement.NormalsKind,
StyleGraphicPropertiesElement.Dr3dShadow,
StyleGraphicPropertiesElement.Shininess,
StyleGraphicPropertiesElement.SpecularColor,
StyleGraphicPropertiesElement.TextureFilter,
StyleGraphicPropertiesElement.TextureGenerationModeX,
StyleGraphicPropertiesElement.TextureGenerationModeY,
StyleGraphicPropertiesElement.TextureKind,
StyleGraphicPropertiesElement.TextureMode,
StyleGraphicPropertiesElement.VerticalSegments,
StyleGraphicPropertiesElement.AutoGrowHeight,
StyleGraphicPropertiesElement.AutoGrowWidth,
StyleGraphicPropertiesElement.Blue,
StyleGraphicPropertiesElement.CaptionAngle,
StyleGraphicPropertiesElement.CaptionAngleType,
StyleGraphicPropertiesElement.CaptionEscape,
StyleGraphicPropertiesElement.CaptionEscapeDirection,
StyleGraphicPropertiesElement.CaptionFitLineLength,
StyleGraphicPropertiesElement.CaptionGap,
StyleGraphicPropertiesElement.CaptionLineLength,
StyleGraphicPropertiesElement.CaptionType,
StyleGraphicPropertiesElement.ColorInversion,
StyleGraphicPropertiesElement.ColorMode,
StyleGraphicPropertiesElement.Contrast,
StyleGraphicPropertiesElement.DecimalPlaces,
StyleGraphicPropertiesElement.DrawAspect,
StyleGraphicPropertiesElement.EndGuide,
StyleGraphicPropertiesElement.EndLineSpacingHorizontal,
StyleGraphicPropertiesElement.EndLineSpacingVertical,
StyleGraphicPropertiesElement.Fill,
StyleGraphicPropertiesElement.FillColor,
StyleGraphicPropertiesElement.FillGradientName,
StyleGraphicPropertiesElement.FillHatchName,
StyleGraphicPropertiesElement.FillHatchSolid,
StyleGraphicPropertiesElement.FillImageHeight,
StyleGraphicPropertiesElement.FillImageName,
StyleGraphicPropertiesElement.FillImageRefPoint,
StyleGraphicPropertiesElement.FillImageRefPointX,
StyleGraphicPropertiesElement.FillImageRefPointY,
StyleGraphicPropertiesElement.FillImageWidth,
StyleGraphicPropertiesElement.FitToContour,
StyleGraphicPropertiesElement.FitToSize,
StyleGraphicPropertiesElement.FrameDisplayBorder,
StyleGraphicPropertiesElement.FrameDisplayScrollbar,
StyleGraphicPropertiesElement.FrameMarginHorizontal,
StyleGraphicPropertiesElement.FrameMarginVertical,
StyleGraphicPropertiesElement.Gamma,
StyleGraphicPropertiesElement.GradientStepCount,
StyleGraphicPropertiesElement.Green,
StyleGraphicPropertiesElement.GuideDistance,
StyleGraphicPropertiesElement.GuideOverhang,
StyleGraphicPropertiesElement.ImageOpacity,
StyleGraphicPropertiesElement.LineDistance,
StyleGraphicPropertiesElement.Luminance,
StyleGraphicPropertiesElement.MarkerEnd,
StyleGraphicPropertiesElement.MarkerEndCenter,
StyleGraphicPropertiesElement.MarkerEndWidth,
StyleGraphicPropertiesElement.MarkerStart,
StyleGraphicPropertiesElement.MarkerStartCenter,
StyleGraphicPropertiesElement.MarkerStartWidth,
StyleGraphicPropertiesElement.MeasureAlign,
StyleGraphicPropertiesElement.MeasureVerticalAlign,
StyleGraphicPropertiesElement.OleDrawAspect,
StyleGraphicPropertiesElement.Opacity,
StyleGraphicPropertiesElement.OpacityName,
StyleGraphicPropertiesElement.Parallel,
StyleGraphicPropertiesElement.Placing,
StyleGraphicPropertiesElement.Red,
StyleGraphicPropertiesElement.SecondaryFillColor,
StyleGraphicPropertiesElement.DrawShadow,
StyleGraphicPropertiesElement.ShadowColor,
StyleGraphicPropertiesElement.ShadowOffsetX,
StyleGraphicPropertiesElement.ShadowOffsetY,
StyleGraphicPropertiesElement.ShadowOpacity,
StyleGraphicPropertiesElement.ShowUnit,
StyleGraphicPropertiesElement.StartGuide,
StyleGraphicPropertiesElement.StartLineSpacingHorizontal,
StyleGraphicPropertiesElement.StartLineSpacingVertical,
StyleGraphicPropertiesElement.Stroke,
StyleGraphicPropertiesElement.StrokeDash,
StyleGraphicPropertiesElement.StrokeDashNames,
StyleGraphicPropertiesElement.StrokeLinejoin,
StyleGraphicPropertiesElement.SymbolColor,
StyleGraphicPropertiesElement.TextareaHorizontalAlign,
StyleGraphicPropertiesElement.TextareaVerticalAlign,
StyleGraphicPropertiesElement.TileRepeatOffset,
StyleGraphicPropertiesElement.Unit,
StyleGraphicPropertiesElement.VisibleAreaHeight,
StyleGraphicPropertiesElement.VisibleAreaLeft,
StyleGraphicPropertiesElement.VisibleAreaTop,
StyleGraphicPropertiesElement.VisibleAreaWidth,
StyleGraphicPropertiesElement.WrapInfluenceOnPosition,
StyleGraphicPropertiesElement.BackgroundColor,
StyleGraphicPropertiesElement.Border,
StyleGraphicPropertiesElement.BorderBottom,
StyleGraphicPropertiesElement.BorderLeft,
StyleGraphicPropertiesElement.BorderRight,
StyleGraphicPropertiesElement.BorderTop,
StyleGraphicPropertiesElement.Clip,
StyleGraphicPropertiesElement.Margin,
StyleGraphicPropertiesElement.MarginBottom,
StyleGraphicPropertiesElement.MarginLeft,
StyleGraphicPropertiesElement.MarginRight,
StyleGraphicPropertiesElement.MarginTop,
StyleGraphicPropertiesElement.MaxHeight,
StyleGraphicPropertiesElement.MaxWidth,
StyleGraphicPropertiesElement.MinHeight,
StyleGraphicPropertiesElement.MinWidth,
StyleGraphicPropertiesElement.Padding,
StyleGraphicPropertiesElement.PaddingBottom,
StyleGraphicPropertiesElement.PaddingLeft,
StyleGraphicPropertiesElement.PaddingRight,
StyleGraphicPropertiesElement.PaddingTop,
StyleGraphicPropertiesElement.WrapOption,
StyleGraphicPropertiesElement.BackgroundTransparency,
StyleGraphicPropertiesElement.BorderLineWidth,
StyleGraphicPropertiesElement.BorderLineWidthBottom,
StyleGraphicPropertiesElement.BorderLineWidthLeft,
StyleGraphicPropertiesElement.BorderLineWidthRight,
StyleGraphicPropertiesElement.BorderLineWidthTop,
StyleGraphicPropertiesElement.Editable,
StyleGraphicPropertiesElement.FlowWithText,
StyleGraphicPropertiesElement.HorizontalPos,
StyleGraphicPropertiesElement.HorizontalRel,
StyleGraphicPropertiesElement.Mirror,
StyleGraphicPropertiesElement.NumberWrappedParagraphs,
StyleGraphicPropertiesElement.OverflowBehavior,
StyleGraphicPropertiesElement.PrintContent,
StyleGraphicPropertiesElement.Protect,
StyleGraphicPropertiesElement.RelHeight,
StyleGraphicPropertiesElement.RelWidth,
StyleGraphicPropertiesElement.Repeat,
StyleGraphicPropertiesElement.RunThrough,
StyleGraphicPropertiesElement.StyleShadow,
StyleGraphicPropertiesElement.ShrinkToFit,
StyleGraphicPropertiesElement.VerticalPos,
StyleGraphicPropertiesElement.VerticalRel,
StyleGraphicPropertiesElement.Wrap,
StyleGraphicPropertiesElement.WrapContour,
StyleGraphicPropertiesElement.WrapContourMode,
StyleGraphicPropertiesElement.WrapDynamicThreshold,
StyleGraphicPropertiesElement.WritingMode,
StyleGraphicPropertiesElement.FillRule,
StyleGraphicPropertiesElement.Height,
StyleGraphicPropertiesElement.StrokeColor,
StyleGraphicPropertiesElement.StrokeLinecap,
StyleGraphicPropertiesElement.StrokeOpacity,
StyleGraphicPropertiesElement.StrokeWidth,
StyleGraphicPropertiesElement.Width,
StyleGraphicPropertiesElement.X,
StyleGraphicPropertiesElement.Y,
StyleGraphicPropertiesElement.AnchorPageNumber,
StyleGraphicPropertiesElement.AnchorType,
StyleGraphicPropertiesElement.Animation,
StyleGraphicPropertiesElement.AnimationDelay,
StyleGraphicPropertiesElement.AnimationDirection,
StyleGraphicPropertiesElement.AnimationRepeat,
StyleGraphicPropertiesElement.AnimationStartInside,
StyleGraphicPropertiesElement.AnimationSteps,
StyleGraphicPropertiesElement.AnimationStopInside,
StyleHeaderFooterPropertiesElement.BackgroundColor,
StyleHeaderFooterPropertiesElement.Border,
StyleHeaderFooterPropertiesElement.BorderBottom,
StyleHeaderFooterPropertiesElement.BorderLeft,
StyleHeaderFooterPropertiesElement.BorderRight,
StyleHeaderFooterPropertiesElement.BorderTop,
StyleHeaderFooterPropertiesElement.Margin,
StyleHeaderFooterPropertiesElement.MarginBottom,
StyleHeaderFooterPropertiesElement.MarginLeft,
StyleHeaderFooterPropertiesElement.MarginRight,
StyleHeaderFooterPropertiesElement.MarginTop,
StyleHeaderFooterPropertiesElement.MinHeight,
StyleHeaderFooterPropertiesElement.Padding,
StyleHeaderFooterPropertiesElement.PaddingBottom,
StyleHeaderFooterPropertiesElement.PaddingLeft,
StyleHeaderFooterPropertiesElement.PaddingRight,
StyleHeaderFooterPropertiesElement.PaddingTop,
StyleHeaderFooterPropertiesElement.BorderLineWidth,
StyleHeaderFooterPropertiesElement.BorderLineWidthBottom,
StyleHeaderFooterPropertiesElement.BorderLineWidthLeft,
StyleHeaderFooterPropertiesElement.BorderLineWidthRight,
StyleHeaderFooterPropertiesElement.BorderLineWidthTop,
StyleHeaderFooterPropertiesElement.DynamicSpacing,
StyleHeaderFooterPropertiesElement.Shadow,
StyleHeaderFooterPropertiesElement.Height,
StyleListLevelPropertiesElement.Height,
StyleListLevelPropertiesElement.TextAlign,
StyleListLevelPropertiesElement.Width,
StyleListLevelPropertiesElement.FontName,
StyleListLevelPropertiesElement.VerticalPos,
StyleListLevelPropertiesElement.VerticalRel,
StyleListLevelPropertiesElement.Y,
StyleListLevelPropertiesElement.ListLevelPositionAndSpaceMode,
StyleListLevelPropertiesElement.MinLabelDistance,
StyleListLevelPropertiesElement.MinLabelWidth,
StyleListLevelPropertiesElement.SpaceBefore,
StylePageLayoutPropertiesElement.BackgroundColor,
StylePageLayoutPropertiesElement.Border,
StylePageLayoutPropertiesElement.BorderBottom,
StylePageLayoutPropertiesElement.BorderLeft,
StylePageLayoutPropertiesElement.BorderRight,
StylePageLayoutPropertiesElement.BorderTop,
StylePageLayoutPropertiesElement.Margin,
StylePageLayoutPropertiesElement.MarginBottom,
StylePageLayoutPropertiesElement.MarginLeft,
StylePageLayoutPropertiesElement.MarginRight,
StylePageLayoutPropertiesElement.MarginTop,
StylePageLayoutPropertiesElement.Padding,
StylePageLayoutPropertiesElement.PaddingBottom,
StylePageLayoutPropertiesElement.PaddingLeft,
StylePageLayoutPropertiesElement.PaddingRight,
StylePageLayoutPropertiesElement.PaddingTop,
StylePageLayoutPropertiesElement.PageHeight,
StylePageLayoutPropertiesElement.PageWidth,
StylePageLayoutPropertiesElement.BorderLineWidth,
StylePageLayoutPropertiesElement.BorderLineWidthBottom,
StylePageLayoutPropertiesElement.BorderLineWidthLeft,
StylePageLayoutPropertiesElement.BorderLineWidthRight,
StylePageLayoutPropertiesElement.BorderLineWidthTop,
StylePageLayoutPropertiesElement.FirstPageNumber,
StylePageLayoutPropertiesElement.FootnoteMaxHeight,
StylePageLayoutPropertiesElement.LayoutGridBaseHeight,
StylePageLayoutPropertiesElement.LayoutGridBaseWidth,
StylePageLayoutPropertiesElement.LayoutGridColor,
StylePageLayoutPropertiesElement.LayoutGridDisplay,
StylePageLayoutPropertiesElement.LayoutGridLines,
StylePageLayoutPropertiesElement.LayoutGridMode,
StylePageLayoutPropertiesElement.LayoutGridPrint,
StylePageLayoutPropertiesElement.LayoutGridRubyBelow,
StylePageLayoutPropertiesElement.LayoutGridRubyHeight,
StylePageLayoutPropertiesElement.LayoutGridSnapTo,
StylePageLayoutPropertiesElement.LayoutGridStandardMode,
StylePageLayoutPropertiesElement.NumFormat,
StylePageLayoutPropertiesElement.NumFormat,
StylePageLayoutPropertiesElement.NumLetterSync,
StylePageLayoutPropertiesElement.NumPrefix,
StylePageLayoutPropertiesElement.NumSuffix,
StylePageLayoutPropertiesElement.PaperTrayName,
StylePageLayoutPropertiesElement.Print,
StylePageLayoutPropertiesElement.PrintOrientation,
StylePageLayoutPropertiesElement.PrintPageOrder,
StylePageLayoutPropertiesElement.RegisterTruthRefStyleName,
StylePageLayoutPropertiesElement.ScaleTo,
StylePageLayoutPropertiesElement.ScaleToPages,
StylePageLayoutPropertiesElement.Shadow,
StylePageLayoutPropertiesElement.TableCentering,
StylePageLayoutPropertiesElement.WritingMode,
StyleParagraphPropertiesElement.BackgroundColor,
StyleParagraphPropertiesElement.Border,
StyleParagraphPropertiesElement.BorderBottom,
StyleParagraphPropertiesElement.BorderLeft,
StyleParagraphPropertiesElement.BorderRight,
StyleParagraphPropertiesElement.BorderTop,
StyleParagraphPropertiesElement.BreakAfter,
StyleParagraphPropertiesElement.BreakBefore,
StyleParagraphPropertiesElement.HyphenationKeep,
StyleParagraphPropertiesElement.HyphenationLadderCount,
StyleParagraphPropertiesElement.KeepTogether,
StyleParagraphPropertiesElement.KeepWithNext,
StyleParagraphPropertiesElement.LineHeight,
StyleParagraphPropertiesElement.Margin,
StyleParagraphPropertiesElement.MarginBottom,
StyleParagraphPropertiesElement.MarginLeft,
StyleParagraphPropertiesElement.MarginRight,
StyleParagraphPropertiesElement.MarginTop,
StyleParagraphPropertiesElement.Orphans,
StyleParagraphPropertiesElement.Padding,
StyleParagraphPropertiesElement.PaddingBottom,
StyleParagraphPropertiesElement.PaddingLeft,
StyleParagraphPropertiesElement.PaddingRight,
StyleParagraphPropertiesElement.PaddingTop,
StyleParagraphPropertiesElement.TextAlign,
StyleParagraphPropertiesElement.TextAlignLast,
StyleParagraphPropertiesElement.TextIndent,
StyleParagraphPropertiesElement.Widows,
StyleParagraphPropertiesElement.AutoTextIndent,
StyleParagraphPropertiesElement.BackgroundTransparency,
StyleParagraphPropertiesElement.BorderLineWidth,
StyleParagraphPropertiesElement.BorderLineWidthBottom,
StyleParagraphPropertiesElement.BorderLineWidthLeft,
StyleParagraphPropertiesElement.BorderLineWidthRight,
StyleParagraphPropertiesElement.BorderLineWidthTop,
StyleParagraphPropertiesElement.FontIndependentLineSpacing,
StyleParagraphPropertiesElement.JoinBorder,
StyleParagraphPropertiesElement.JustifySingleWord,
StyleParagraphPropertiesElement.LineBreak,
StyleParagraphPropertiesElement.LineHeightAtLeast,
StyleParagraphPropertiesElement.LineSpacing,
StyleParagraphPropertiesElement.PageNumber,
StyleParagraphPropertiesElement.PunctuationWrap,
StyleParagraphPropertiesElement.RegisterTrue,
StyleParagraphPropertiesElement.Shadow,
StyleParagraphPropertiesElement.SnapToLayoutGrid,
StyleParagraphPropertiesElement.TabStopDistance,
StyleParagraphPropertiesElement.TextAutospace,
StyleParagraphPropertiesElement.VerticalAlign,
StyleParagraphPropertiesElement.WritingMode,
StyleParagraphPropertiesElement.WritingModeAutomatic,
StyleParagraphPropertiesElement.LineNumber,
StyleParagraphPropertiesElement.NumberLines,
StyleRubyPropertiesElement.RubyAlign,
StyleRubyPropertiesElement.RubyPosition,
StyleSectionPropertiesElement.BackgroundColor,
StyleSectionPropertiesElement.MarginLeft,
StyleSectionPropertiesElement.MarginRight,
StyleSectionPropertiesElement.Editable,
StyleSectionPropertiesElement.Protect,
StyleSectionPropertiesElement.WritingMode,
StyleSectionPropertiesElement.DontBalanceTextColumns,
StyleTableCellPropertiesElement.BackgroundColor,
StyleTableCellPropertiesElement.Border,
StyleTableCellPropertiesElement.BorderBottom,
StyleTableCellPropertiesElement.BorderLeft,
StyleTableCellPropertiesElement.BorderRight,
StyleTableCellPropertiesElement.BorderTop,
StyleTableCellPropertiesElement.Padding,
StyleTableCellPropertiesElement.PaddingBottom,
StyleTableCellPropertiesElement.PaddingLeft,
StyleTableCellPropertiesElement.PaddingRight,
StyleTableCellPropertiesElement.PaddingTop,
StyleTableCellPropertiesElement.WrapOption,
StyleTableCellPropertiesElement.BorderLineWidth,
StyleTableCellPropertiesElement.BorderLineWidthBottom,
StyleTableCellPropertiesElement.BorderLineWidthLeft,
StyleTableCellPropertiesElement.BorderLineWidthRight,
StyleTableCellPropertiesElement.BorderLineWidthTop,
StyleTableCellPropertiesElement.CellProtect,
StyleTableCellPropertiesElement.DecimalPlaces,
StyleTableCellPropertiesElement.DiagonalBlTr,
StyleTableCellPropertiesElement.DiagonalBlTrWidths,
StyleTableCellPropertiesElement.DiagonalTlBr,
StyleTableCellPropertiesElement.DiagonalTlBrWidths,
StyleTableCellPropertiesElement.Direction,
StyleTableCellPropertiesElement.GlyphOrientationVertical,
StyleTableCellPropertiesElement.PrintContent,
StyleTableCellPropertiesElement.RepeatContent,
StyleTableCellPropertiesElement.RotationAlign,
StyleTableCellPropertiesElement.RotationAngle,
StyleTableCellPropertiesElement.Shadow,
StyleTableCellPropertiesElement.ShrinkToFit,
StyleTableCellPropertiesElement.TextAlignSource,
StyleTableCellPropertiesElement.VerticalAlign,
StyleTableCellPropertiesElement.WritingMode,
StyleTableColumnPropertiesElement.BreakAfter,
StyleTableColumnPropertiesElement.BreakBefore,
StyleTableColumnPropertiesElement.ColumnWidth,
StyleTableColumnPropertiesElement.RelColumnWidth,
StyleTableColumnPropertiesElement.UseOptimalColumnWidth,
StyleTablePropertiesElement.BackgroundColor,
StyleTablePropertiesElement.BreakAfter,
StyleTablePropertiesElement.BreakBefore,
StyleTablePropertiesElement.KeepWithNext,
StyleTablePropertiesElement.Margin,
StyleTablePropertiesElement.MarginBottom,
StyleTablePropertiesElement.MarginLeft,
StyleTablePropertiesElement.MarginRight,
StyleTablePropertiesElement.MarginTop,
StyleTablePropertiesElement.MayBreakBetweenRows,
StyleTablePropertiesElement.PageNumber,
StyleTablePropertiesElement.RelWidth,
StyleTablePropertiesElement.Shadow,
StyleTablePropertiesElement.Width,
StyleTablePropertiesElement.WritingMode,
StyleTablePropertiesElement.Align,
StyleTablePropertiesElement.BorderModel,
StyleTablePropertiesElement.Display,
StyleTableRowPropertiesElement.BackgroundColor,
StyleTableRowPropertiesElement.BreakAfter,
StyleTableRowPropertiesElement.BreakBefore,
StyleTableRowPropertiesElement.KeepTogether,
StyleTableRowPropertiesElement.MinRowHeight,
StyleTableRowPropertiesElement.RowHeight,
StyleTableRowPropertiesElement.UseOptimalRowHeight,
StyleTextPropertiesElement.BackgroundColor,
StyleTextPropertiesElement.Color,
StyleTextPropertiesElement.Country,
StyleTextPropertiesElement.FontFamily,
StyleTextPropertiesElement.FontSize,
StyleTextPropertiesElement.FontStyle,
StyleTextPropertiesElement.FontVariant,
StyleTextPropertiesElement.FontWeight,
StyleTextPropertiesElement.Hyphenate,
StyleTextPropertiesElement.HyphenationPushCharCount,
StyleTextPropertiesElement.HyphenationRemainCharCount,
StyleTextPropertiesElement.Language,
StyleTextPropertiesElement.LetterSpacing,
StyleTextPropertiesElement.Script,
StyleTextPropertiesElement.TextShadow,
StyleTextPropertiesElement.TextTransform,
StyleTextPropertiesElement.CountryAsian,
StyleTextPropertiesElement.CountryComplex,
StyleTextPropertiesElement.FontCharset,
StyleTextPropertiesElement.FontCharsetAsian,
StyleTextPropertiesElement.FontCharsetComplex,
StyleTextPropertiesElement.FontFamilyAsian,
StyleTextPropertiesElement.FontFamilyComplex,
StyleTextPropertiesElement.FontFamilyGeneric,
StyleTextPropertiesElement.FontFamilyGenericAsian,
StyleTextPropertiesElement.FontFamilyGenericComplex,
StyleTextPropertiesElement.FontName,
StyleTextPropertiesElement.FontNameAsian,
StyleTextPropertiesElement.FontNameComplex,
StyleTextPropertiesElement.FontPitch,
StyleTextPropertiesElement.FontPitchAsian,
StyleTextPropertiesElement.FontPitchComplex,
StyleTextPropertiesElement.FontRelief,
StyleTextPropertiesElement.FontSizeAsian,
StyleTextPropertiesElement.FontSizeComplex,
StyleTextPropertiesElement.FontSizeRel,
StyleTextPropertiesElement.FontSizeRelAsian,
StyleTextPropertiesElement.FontSizeRelComplex,
StyleTextPropertiesElement.FontStyleAsian,
StyleTextPropertiesElement.FontStyleComplex,
StyleTextPropertiesElement.FontStyleName,
StyleTextPropertiesElement.FontStyleNameAsian,
StyleTextPropertiesElement.FontStyleNameComplex,
StyleTextPropertiesElement.FontWeightAsian,
StyleTextPropertiesElement.FontWeightComplex,
StyleTextPropertiesElement.LanguageAsian,
StyleTextPropertiesElement.LanguageComplex,
StyleTextPropertiesElement.LetterKerning,
StyleTextPropertiesElement.RfcLanguageTag,
StyleTextPropertiesElement.RfcLanguageTagAsian,
StyleTextPropertiesElement.RfcLanguageTagComplex,
StyleTextPropertiesElement.ScriptAsian,
StyleTextPropertiesElement.ScriptComplex,
StyleTextPropertiesElement.ScriptType,
StyleTextPropertiesElement.TextBlinking,
StyleTextPropertiesElement.TextCombine,
StyleTextPropertiesElement.TextCombineEndChar,
StyleTextPropertiesElement.TextCombineStartChar,
StyleTextPropertiesElement.TextEmphasize,
StyleTextPropertiesElement.TextLineThroughColor,
StyleTextPropertiesElement.TextLineThroughMode,
StyleTextPropertiesElement.TextLineThroughStyle,
StyleTextPropertiesElement.TextLineThroughText,
StyleTextPropertiesElement.TextLineThroughTextStyle,
StyleTextPropertiesElement.TextLineThroughType,
StyleTextPropertiesElement.TextLineThroughWidth,
StyleTextPropertiesElement.TextOutline,
StyleTextPropertiesElement.TextOverlineColor,
StyleTextPropertiesElement.TextOverlineMode,
StyleTextPropertiesElement.TextOverlineStyle,
StyleTextPropertiesElement.TextOverlineType,
StyleTextPropertiesElement.TextOverlineWidth,
StyleTextPropertiesElement.TextPosition,
StyleTextPropertiesElement.TextRotationAngle,
StyleTextPropertiesElement.TextRotationScale,
StyleTextPropertiesElement.TextScale,
StyleTextPropertiesElement.TextUnderlineColor,
StyleTextPropertiesElement.TextUnderlineMode,
StyleTextPropertiesElement.TextUnderlineStyle,
StyleTextPropertiesElement.TextUnderlineType,
StyleTextPropertiesElement.TextUnderlineWidth,
StyleTextPropertiesElement.UseWindowFontColor,
StyleTextPropertiesElement.Condition,
StyleTextPropertiesElement.Display,
StyleTextPropertiesElement.Display,
StyleTextPropertiesElement.Display,
});
public final static OdfStyleFamily List = new OdfStyleFamily("list",
new OdfStyleProperty[]{
StyleChartPropertiesElement.AngleOffset,
StyleChartPropertiesElement.AutoPosition,
StyleChartPropertiesElement.AutoSize,
StyleChartPropertiesElement.AxisLabelPosition,
StyleChartPropertiesElement.AxisPosition,
StyleChartPropertiesElement.ConnectBars,
StyleChartPropertiesElement.DataLabelNumber,
StyleChartPropertiesElement.DataLabelSymbol,
StyleChartPropertiesElement.DataLabelText,
StyleChartPropertiesElement.Deep,
StyleChartPropertiesElement.DisplayLabel,
StyleChartPropertiesElement.ErrorCategory,
StyleChartPropertiesElement.ErrorLowerIndicator,
StyleChartPropertiesElement.ErrorLowerLimit,
StyleChartPropertiesElement.ErrorLowerRange,
StyleChartPropertiesElement.ErrorMargin,
StyleChartPropertiesElement.ErrorPercentage,
StyleChartPropertiesElement.ErrorUpperIndicator,
StyleChartPropertiesElement.ErrorUpperLimit,
StyleChartPropertiesElement.ErrorUpperRange,
StyleChartPropertiesElement.GapWidth,
StyleChartPropertiesElement.GroupBarsPerAxis,
StyleChartPropertiesElement.HoleSize,
StyleChartPropertiesElement.IncludeHiddenCells,
StyleChartPropertiesElement.Interpolation,
StyleChartPropertiesElement.IntervalMajor,
StyleChartPropertiesElement.IntervalMinorDivisor,
StyleChartPropertiesElement.JapaneseCandleStick,
StyleChartPropertiesElement.LabelArrangement,
StyleChartPropertiesElement.LabelPosition,
StyleChartPropertiesElement.LabelPositionNegative,
StyleChartPropertiesElement.Lines,
StyleChartPropertiesElement.LinkDataStyleToSource,
StyleChartPropertiesElement.Logarithmic,
StyleChartPropertiesElement.Maximum,
StyleChartPropertiesElement.MeanValue,
StyleChartPropertiesElement.Minimum,
StyleChartPropertiesElement.Origin,
StyleChartPropertiesElement.Overlap,
StyleChartPropertiesElement.Percentage,
StyleChartPropertiesElement.PieOffset,
StyleChartPropertiesElement.RegressionType,
StyleChartPropertiesElement.ReverseDirection,
StyleChartPropertiesElement.RightAngledAxes,
StyleChartPropertiesElement.ScaleText,
StyleChartPropertiesElement.SeriesSource,
StyleChartPropertiesElement.SolidType,
StyleChartPropertiesElement.SortByXValues,
StyleChartPropertiesElement.SplineOrder,
StyleChartPropertiesElement.SplineResolution,
StyleChartPropertiesElement.Stacked,
StyleChartPropertiesElement.SymbolHeight,
StyleChartPropertiesElement.SymbolName,
StyleChartPropertiesElement.SymbolType,
StyleChartPropertiesElement.SymbolType,
StyleChartPropertiesElement.SymbolType,
StyleChartPropertiesElement.SymbolType,
StyleChartPropertiesElement.SymbolWidth,
StyleChartPropertiesElement.TextOverlap,
StyleChartPropertiesElement.ThreeDimensional,
StyleChartPropertiesElement.TickMarkPosition,
StyleChartPropertiesElement.TickMarksMajorInner,
StyleChartPropertiesElement.TickMarksMajorOuter,
StyleChartPropertiesElement.TickMarksMinorInner,
StyleChartPropertiesElement.TickMarksMinorOuter,
StyleChartPropertiesElement.TreatEmptyCells,
StyleChartPropertiesElement.Vertical,
StyleChartPropertiesElement.Visible,
StyleChartPropertiesElement.Direction,
StyleChartPropertiesElement.RotationAngle,
StyleChartPropertiesElement.LineBreak,
StyleDrawingPagePropertiesElement.BackgroundSize,
StyleDrawingPagePropertiesElement.Fill,
StyleDrawingPagePropertiesElement.FillColor,
StyleDrawingPagePropertiesElement.FillGradientName,
StyleDrawingPagePropertiesElement.FillHatchName,
StyleDrawingPagePropertiesElement.FillHatchSolid,
StyleDrawingPagePropertiesElement.FillImageHeight,
StyleDrawingPagePropertiesElement.FillImageName,
StyleDrawingPagePropertiesElement.FillImageRefPoint,
StyleDrawingPagePropertiesElement.FillImageRefPointX,
StyleDrawingPagePropertiesElement.FillImageRefPointY,
StyleDrawingPagePropertiesElement.FillImageWidth,
StyleDrawingPagePropertiesElement.GradientStepCount,
StyleDrawingPagePropertiesElement.Opacity,
StyleDrawingPagePropertiesElement.OpacityName,
StyleDrawingPagePropertiesElement.SecondaryFillColor,
StyleDrawingPagePropertiesElement.TileRepeatOffset,
StyleDrawingPagePropertiesElement.BackgroundObjectsVisible,
StyleDrawingPagePropertiesElement.BackgroundVisible,
StyleDrawingPagePropertiesElement.DisplayDateTime,
StyleDrawingPagePropertiesElement.DisplayFooter,
StyleDrawingPagePropertiesElement.DisplayHeader,
StyleDrawingPagePropertiesElement.DisplayPageNumber,
StyleDrawingPagePropertiesElement.Duration,
StyleDrawingPagePropertiesElement.TransitionSpeed,
StyleDrawingPagePropertiesElement.TransitionStyle,
StyleDrawingPagePropertiesElement.TransitionType,
StyleDrawingPagePropertiesElement.Visibility,
StyleDrawingPagePropertiesElement.Direction,
StyleDrawingPagePropertiesElement.FadeColor,
StyleDrawingPagePropertiesElement.Subtype,
StyleDrawingPagePropertiesElement.Type,
StyleDrawingPagePropertiesElement.Repeat,
StyleDrawingPagePropertiesElement.FillRule,
StyleGraphicPropertiesElement.AmbientColor,
StyleGraphicPropertiesElement.BackScale,
StyleGraphicPropertiesElement.BackfaceCulling,
StyleGraphicPropertiesElement.CloseBack,
StyleGraphicPropertiesElement.CloseFront,
StyleGraphicPropertiesElement.Depth,
StyleGraphicPropertiesElement.DiffuseColor,
StyleGraphicPropertiesElement.EdgeRounding,
StyleGraphicPropertiesElement.EdgeRoundingMode,
StyleGraphicPropertiesElement.EmissiveColor,
StyleGraphicPropertiesElement.EndAngle,
StyleGraphicPropertiesElement.HorizontalSegments,
StyleGraphicPropertiesElement.LightingMode,
StyleGraphicPropertiesElement.NormalsDirection,
StyleGraphicPropertiesElement.NormalsKind,
StyleGraphicPropertiesElement.Dr3dShadow,
StyleGraphicPropertiesElement.Shininess,
StyleGraphicPropertiesElement.SpecularColor,
StyleGraphicPropertiesElement.TextureFilter,
StyleGraphicPropertiesElement.TextureGenerationModeX,
StyleGraphicPropertiesElement.TextureGenerationModeY,
StyleGraphicPropertiesElement.TextureKind,
StyleGraphicPropertiesElement.TextureMode,
StyleGraphicPropertiesElement.VerticalSegments,
StyleGraphicPropertiesElement.AutoGrowHeight,
StyleGraphicPropertiesElement.AutoGrowWidth,
StyleGraphicPropertiesElement.Blue,
StyleGraphicPropertiesElement.CaptionAngle,
StyleGraphicPropertiesElement.CaptionAngleType,
StyleGraphicPropertiesElement.CaptionEscape,
StyleGraphicPropertiesElement.CaptionEscapeDirection,
StyleGraphicPropertiesElement.CaptionFitLineLength,
StyleGraphicPropertiesElement.CaptionGap,
StyleGraphicPropertiesElement.CaptionLineLength,
StyleGraphicPropertiesElement.CaptionType,
StyleGraphicPropertiesElement.ColorInversion,
StyleGraphicPropertiesElement.ColorMode,
StyleGraphicPropertiesElement.Contrast,
StyleGraphicPropertiesElement.DecimalPlaces,
StyleGraphicPropertiesElement.DrawAspect,
StyleGraphicPropertiesElement.EndGuide,
StyleGraphicPropertiesElement.EndLineSpacingHorizontal,
StyleGraphicPropertiesElement.EndLineSpacingVertical,
StyleGraphicPropertiesElement.Fill,
StyleGraphicPropertiesElement.FillColor,
StyleGraphicPropertiesElement.FillGradientName,
StyleGraphicPropertiesElement.FillHatchName,
StyleGraphicPropertiesElement.FillHatchSolid,
StyleGraphicPropertiesElement.FillImageHeight,
StyleGraphicPropertiesElement.FillImageName,
StyleGraphicPropertiesElement.FillImageRefPoint,
StyleGraphicPropertiesElement.FillImageRefPointX,
StyleGraphicPropertiesElement.FillImageRefPointY,
StyleGraphicPropertiesElement.FillImageWidth,
StyleGraphicPropertiesElement.FitToContour,
StyleGraphicPropertiesElement.FitToSize,
StyleGraphicPropertiesElement.FrameDisplayBorder,
StyleGraphicPropertiesElement.FrameDisplayScrollbar,
StyleGraphicPropertiesElement.FrameMarginHorizontal,
StyleGraphicPropertiesElement.FrameMarginVertical,
StyleGraphicPropertiesElement.Gamma,
StyleGraphicPropertiesElement.GradientStepCount,
StyleGraphicPropertiesElement.Green,
StyleGraphicPropertiesElement.GuideDistance,
StyleGraphicPropertiesElement.GuideOverhang,
StyleGraphicPropertiesElement.ImageOpacity,
StyleGraphicPropertiesElement.LineDistance,
StyleGraphicPropertiesElement.Luminance,
StyleGraphicPropertiesElement.MarkerEnd,
StyleGraphicPropertiesElement.MarkerEndCenter,
StyleGraphicPropertiesElement.MarkerEndWidth,
StyleGraphicPropertiesElement.MarkerStart,
StyleGraphicPropertiesElement.MarkerStartCenter,
StyleGraphicPropertiesElement.MarkerStartWidth,
StyleGraphicPropertiesElement.MeasureAlign,
StyleGraphicPropertiesElement.MeasureVerticalAlign,
StyleGraphicPropertiesElement.OleDrawAspect,
StyleGraphicPropertiesElement.Opacity,
StyleGraphicPropertiesElement.OpacityName,
StyleGraphicPropertiesElement.Parallel,
StyleGraphicPropertiesElement.Placing,
StyleGraphicPropertiesElement.Red,
StyleGraphicPropertiesElement.SecondaryFillColor,
StyleGraphicPropertiesElement.DrawShadow,
StyleGraphicPropertiesElement.ShadowColor,
StyleGraphicPropertiesElement.ShadowOffsetX,
StyleGraphicPropertiesElement.ShadowOffsetY,
StyleGraphicPropertiesElement.ShadowOpacity,
StyleGraphicPropertiesElement.ShowUnit,
StyleGraphicPropertiesElement.StartGuide,
StyleGraphicPropertiesElement.StartLineSpacingHorizontal,
StyleGraphicPropertiesElement.StartLineSpacingVertical,
StyleGraphicPropertiesElement.Stroke,
StyleGraphicPropertiesElement.StrokeDash,
StyleGraphicPropertiesElement.StrokeDashNames,
StyleGraphicPropertiesElement.StrokeLinejoin,
StyleGraphicPropertiesElement.SymbolColor,
StyleGraphicPropertiesElement.TextareaHorizontalAlign,
StyleGraphicPropertiesElement.TextareaVerticalAlign,
StyleGraphicPropertiesElement.TileRepeatOffset,
StyleGraphicPropertiesElement.Unit,
StyleGraphicPropertiesElement.VisibleAreaHeight,
StyleGraphicPropertiesElement.VisibleAreaLeft,
StyleGraphicPropertiesElement.VisibleAreaTop,
StyleGraphicPropertiesElement.VisibleAreaWidth,
StyleGraphicPropertiesElement.WrapInfluenceOnPosition,
StyleGraphicPropertiesElement.BackgroundColor,
StyleGraphicPropertiesElement.Border,
StyleGraphicPropertiesElement.BorderBottom,
StyleGraphicPropertiesElement.BorderLeft,
StyleGraphicPropertiesElement.BorderRight,
StyleGraphicPropertiesElement.BorderTop,
StyleGraphicPropertiesElement.Clip,
StyleGraphicPropertiesElement.Margin,
StyleGraphicPropertiesElement.MarginBottom,
StyleGraphicPropertiesElement.MarginLeft,
StyleGraphicPropertiesElement.MarginRight,
StyleGraphicPropertiesElement.MarginTop,
StyleGraphicPropertiesElement.MaxHeight,
StyleGraphicPropertiesElement.MaxWidth,
StyleGraphicPropertiesElement.MinHeight,
StyleGraphicPropertiesElement.MinWidth,
StyleGraphicPropertiesElement.Padding,
StyleGraphicPropertiesElement.PaddingBottom,
StyleGraphicPropertiesElement.PaddingLeft,
StyleGraphicPropertiesElement.PaddingRight,
StyleGraphicPropertiesElement.PaddingTop,
StyleGraphicPropertiesElement.WrapOption,
StyleGraphicPropertiesElement.BackgroundTransparency,
StyleGraphicPropertiesElement.BorderLineWidth,
StyleGraphicPropertiesElement.BorderLineWidthBottom,
StyleGraphicPropertiesElement.BorderLineWidthLeft,
StyleGraphicPropertiesElement.BorderLineWidthRight,
StyleGraphicPropertiesElement.BorderLineWidthTop,
StyleGraphicPropertiesElement.Editable,
StyleGraphicPropertiesElement.FlowWithText,
StyleGraphicPropertiesElement.HorizontalPos,
StyleGraphicPropertiesElement.HorizontalRel,
StyleGraphicPropertiesElement.Mirror,
StyleGraphicPropertiesElement.NumberWrappedParagraphs,
StyleGraphicPropertiesElement.OverflowBehavior,
StyleGraphicPropertiesElement.PrintContent,
StyleGraphicPropertiesElement.Protect,
StyleGraphicPropertiesElement.RelHeight,
StyleGraphicPropertiesElement.RelWidth,
StyleGraphicPropertiesElement.Repeat,
StyleGraphicPropertiesElement.RunThrough,
StyleGraphicPropertiesElement.StyleShadow,
StyleGraphicPropertiesElement.ShrinkToFit,
StyleGraphicPropertiesElement.VerticalPos,
StyleGraphicPropertiesElement.VerticalRel,
StyleGraphicPropertiesElement.Wrap,
StyleGraphicPropertiesElement.WrapContour,
StyleGraphicPropertiesElement.WrapContourMode,
StyleGraphicPropertiesElement.WrapDynamicThreshold,
StyleGraphicPropertiesElement.WritingMode,
StyleGraphicPropertiesElement.FillRule,
StyleGraphicPropertiesElement.Height,
StyleGraphicPropertiesElement.StrokeColor,
StyleGraphicPropertiesElement.StrokeLinecap,
StyleGraphicPropertiesElement.StrokeOpacity,
StyleGraphicPropertiesElement.StrokeWidth,
StyleGraphicPropertiesElement.Width,
StyleGraphicPropertiesElement.X,
StyleGraphicPropertiesElement.Y,
StyleGraphicPropertiesElement.AnchorPageNumber,
StyleGraphicPropertiesElement.AnchorType,
StyleGraphicPropertiesElement.Animation,
StyleGraphicPropertiesElement.AnimationDelay,
StyleGraphicPropertiesElement.AnimationDirection,
StyleGraphicPropertiesElement.AnimationRepeat,
StyleGraphicPropertiesElement.AnimationStartInside,
StyleGraphicPropertiesElement.AnimationSteps,
StyleGraphicPropertiesElement.AnimationStopInside,
StyleHeaderFooterPropertiesElement.BackgroundColor,
StyleHeaderFooterPropertiesElement.Border,
StyleHeaderFooterPropertiesElement.BorderBottom,
StyleHeaderFooterPropertiesElement.BorderLeft,
StyleHeaderFooterPropertiesElement.BorderRight,
StyleHeaderFooterPropertiesElement.BorderTop,
StyleHeaderFooterPropertiesElement.Margin,
StyleHeaderFooterPropertiesElement.MarginBottom,
StyleHeaderFooterPropertiesElement.MarginLeft,
StyleHeaderFooterPropertiesElement.MarginRight,
StyleHeaderFooterPropertiesElement.MarginTop,
StyleHeaderFooterPropertiesElement.MinHeight,
StyleHeaderFooterPropertiesElement.Padding,
StyleHeaderFooterPropertiesElement.PaddingBottom,
StyleHeaderFooterPropertiesElement.PaddingLeft,
StyleHeaderFooterPropertiesElement.PaddingRight,
StyleHeaderFooterPropertiesElement.PaddingTop,
StyleHeaderFooterPropertiesElement.BorderLineWidth,
StyleHeaderFooterPropertiesElement.BorderLineWidthBottom,
StyleHeaderFooterPropertiesElement.BorderLineWidthLeft,
StyleHeaderFooterPropertiesElement.BorderLineWidthRight,
StyleHeaderFooterPropertiesElement.BorderLineWidthTop,
StyleHeaderFooterPropertiesElement.DynamicSpacing,
StyleHeaderFooterPropertiesElement.Shadow,
StyleHeaderFooterPropertiesElement.Height,
StyleListLevelPropertiesElement.Height,
StyleListLevelPropertiesElement.TextAlign,
StyleListLevelPropertiesElement.Width,
StyleListLevelPropertiesElement.FontName,
StyleListLevelPropertiesElement.VerticalPos,
StyleListLevelPropertiesElement.VerticalRel,
StyleListLevelPropertiesElement.Y,
StyleListLevelPropertiesElement.ListLevelPositionAndSpaceMode,
StyleListLevelPropertiesElement.MinLabelDistance,
StyleListLevelPropertiesElement.MinLabelWidth,
StyleListLevelPropertiesElement.SpaceBefore,
StylePageLayoutPropertiesElement.BackgroundColor,
StylePageLayoutPropertiesElement.Border,
StylePageLayoutPropertiesElement.BorderBottom,
StylePageLayoutPropertiesElement.BorderLeft,
StylePageLayoutPropertiesElement.BorderRight,
StylePageLayoutPropertiesElement.BorderTop,
StylePageLayoutPropertiesElement.Margin,
StylePageLayoutPropertiesElement.MarginBottom,
StylePageLayoutPropertiesElement.MarginLeft,
StylePageLayoutPropertiesElement.MarginRight,
StylePageLayoutPropertiesElement.MarginTop,
StylePageLayoutPropertiesElement.Padding,
StylePageLayoutPropertiesElement.PaddingBottom,
StylePageLayoutPropertiesElement.PaddingLeft,
StylePageLayoutPropertiesElement.PaddingRight,
StylePageLayoutPropertiesElement.PaddingTop,
StylePageLayoutPropertiesElement.PageHeight,
StylePageLayoutPropertiesElement.PageWidth,
StylePageLayoutPropertiesElement.BorderLineWidth,
StylePageLayoutPropertiesElement.BorderLineWidthBottom,
StylePageLayoutPropertiesElement.BorderLineWidthLeft,
StylePageLayoutPropertiesElement.BorderLineWidthRight,
StylePageLayoutPropertiesElement.BorderLineWidthTop,
StylePageLayoutPropertiesElement.FirstPageNumber,
StylePageLayoutPropertiesElement.FootnoteMaxHeight,
StylePageLayoutPropertiesElement.LayoutGridBaseHeight,
StylePageLayoutPropertiesElement.LayoutGridBaseWidth,
StylePageLayoutPropertiesElement.LayoutGridColor,
StylePageLayoutPropertiesElement.LayoutGridDisplay,
StylePageLayoutPropertiesElement.LayoutGridLines,
StylePageLayoutPropertiesElement.LayoutGridMode,
StylePageLayoutPropertiesElement.LayoutGridPrint,
StylePageLayoutPropertiesElement.LayoutGridRubyBelow,
StylePageLayoutPropertiesElement.LayoutGridRubyHeight,
StylePageLayoutPropertiesElement.LayoutGridSnapTo,
StylePageLayoutPropertiesElement.LayoutGridStandardMode,
StylePageLayoutPropertiesElement.NumFormat,
StylePageLayoutPropertiesElement.NumFormat,
StylePageLayoutPropertiesElement.NumLetterSync,
StylePageLayoutPropertiesElement.NumPrefix,
StylePageLayoutPropertiesElement.NumSuffix,
StylePageLayoutPropertiesElement.PaperTrayName,
StylePageLayoutPropertiesElement.Print,
StylePageLayoutPropertiesElement.PrintOrientation,
StylePageLayoutPropertiesElement.PrintPageOrder,
StylePageLayoutPropertiesElement.RegisterTruthRefStyleName,
StylePageLayoutPropertiesElement.ScaleTo,
StylePageLayoutPropertiesElement.ScaleToPages,
StylePageLayoutPropertiesElement.Shadow,
StylePageLayoutPropertiesElement.TableCentering,
StylePageLayoutPropertiesElement.WritingMode,
StyleParagraphPropertiesElement.BackgroundColor,
StyleParagraphPropertiesElement.Border,
StyleParagraphPropertiesElement.BorderBottom,
StyleParagraphPropertiesElement.BorderLeft,
StyleParagraphPropertiesElement.BorderRight,
StyleParagraphPropertiesElement.BorderTop,
StyleParagraphPropertiesElement.BreakAfter,
StyleParagraphPropertiesElement.BreakBefore,
StyleParagraphPropertiesElement.HyphenationKeep,
StyleParagraphPropertiesElement.HyphenationLadderCount,
StyleParagraphPropertiesElement.KeepTogether,
StyleParagraphPropertiesElement.KeepWithNext,
StyleParagraphPropertiesElement.LineHeight,
StyleParagraphPropertiesElement.Margin,
StyleParagraphPropertiesElement.MarginBottom,
StyleParagraphPropertiesElement.MarginLeft,
StyleParagraphPropertiesElement.MarginRight,
StyleParagraphPropertiesElement.MarginTop,
StyleParagraphPropertiesElement.Orphans,
StyleParagraphPropertiesElement.Padding,
StyleParagraphPropertiesElement.PaddingBottom,
StyleParagraphPropertiesElement.PaddingLeft,
StyleParagraphPropertiesElement.PaddingRight,
StyleParagraphPropertiesElement.PaddingTop,
StyleParagraphPropertiesElement.TextAlign,
StyleParagraphPropertiesElement.TextAlignLast,
StyleParagraphPropertiesElement.TextIndent,
StyleParagraphPropertiesElement.Widows,
StyleParagraphPropertiesElement.AutoTextIndent,
StyleParagraphPropertiesElement.BackgroundTransparency,
StyleParagraphPropertiesElement.BorderLineWidth,
StyleParagraphPropertiesElement.BorderLineWidthBottom,
StyleParagraphPropertiesElement.BorderLineWidthLeft,
StyleParagraphPropertiesElement.BorderLineWidthRight,
StyleParagraphPropertiesElement.BorderLineWidthTop,
StyleParagraphPropertiesElement.FontIndependentLineSpacing,
StyleParagraphPropertiesElement.JoinBorder,
StyleParagraphPropertiesElement.JustifySingleWord,
StyleParagraphPropertiesElement.LineBreak,
StyleParagraphPropertiesElement.LineHeightAtLeast,
StyleParagraphPropertiesElement.LineSpacing,
StyleParagraphPropertiesElement.PageNumber,
StyleParagraphPropertiesElement.PunctuationWrap,
StyleParagraphPropertiesElement.RegisterTrue,
StyleParagraphPropertiesElement.Shadow,
StyleParagraphPropertiesElement.SnapToLayoutGrid,
StyleParagraphPropertiesElement.TabStopDistance,
StyleParagraphPropertiesElement.TextAutospace,
StyleParagraphPropertiesElement.VerticalAlign,
StyleParagraphPropertiesElement.WritingMode,
StyleParagraphPropertiesElement.WritingModeAutomatic,
StyleParagraphPropertiesElement.LineNumber,
StyleParagraphPropertiesElement.NumberLines,
StyleRubyPropertiesElement.RubyAlign,
StyleRubyPropertiesElement.RubyPosition,
StyleSectionPropertiesElement.BackgroundColor,
StyleSectionPropertiesElement.MarginLeft,
StyleSectionPropertiesElement.MarginRight,
StyleSectionPropertiesElement.Editable,
StyleSectionPropertiesElement.Protect,
StyleSectionPropertiesElement.WritingMode,
StyleSectionPropertiesElement.DontBalanceTextColumns,
StyleTableCellPropertiesElement.BackgroundColor,
StyleTableCellPropertiesElement.Border,
StyleTableCellPropertiesElement.BorderBottom,
StyleTableCellPropertiesElement.BorderLeft,
StyleTableCellPropertiesElement.BorderRight,
StyleTableCellPropertiesElement.BorderTop,
StyleTableCellPropertiesElement.Padding,
StyleTableCellPropertiesElement.PaddingBottom,
StyleTableCellPropertiesElement.PaddingLeft,
StyleTableCellPropertiesElement.PaddingRight,
StyleTableCellPropertiesElement.PaddingTop,
StyleTableCellPropertiesElement.WrapOption,
StyleTableCellPropertiesElement.BorderLineWidth,
StyleTableCellPropertiesElement.BorderLineWidthBottom,
StyleTableCellPropertiesElement.BorderLineWidthLeft,
StyleTableCellPropertiesElement.BorderLineWidthRight,
StyleTableCellPropertiesElement.BorderLineWidthTop,
StyleTableCellPropertiesElement.CellProtect,
StyleTableCellPropertiesElement.DecimalPlaces,
StyleTableCellPropertiesElement.DiagonalBlTr,
StyleTableCellPropertiesElement.DiagonalBlTrWidths,
StyleTableCellPropertiesElement.DiagonalTlBr,
StyleTableCellPropertiesElement.DiagonalTlBrWidths,
StyleTableCellPropertiesElement.Direction,
StyleTableCellPropertiesElement.GlyphOrientationVertical,
StyleTableCellPropertiesElement.PrintContent,
StyleTableCellPropertiesElement.RepeatContent,
StyleTableCellPropertiesElement.RotationAlign,
StyleTableCellPropertiesElement.RotationAngle,
StyleTableCellPropertiesElement.Shadow,
StyleTableCellPropertiesElement.ShrinkToFit,
StyleTableCellPropertiesElement.TextAlignSource,
StyleTableCellPropertiesElement.VerticalAlign,
StyleTableCellPropertiesElement.WritingMode,
StyleTableColumnPropertiesElement.BreakAfter,
StyleTableColumnPropertiesElement.BreakBefore,
StyleTableColumnPropertiesElement.ColumnWidth,
StyleTableColumnPropertiesElement.RelColumnWidth,
StyleTableColumnPropertiesElement.UseOptimalColumnWidth,
StyleTablePropertiesElement.BackgroundColor,
StyleTablePropertiesElement.BreakAfter,
StyleTablePropertiesElement.BreakBefore,
StyleTablePropertiesElement.KeepWithNext,
StyleTablePropertiesElement.Margin,
StyleTablePropertiesElement.MarginBottom,
StyleTablePropertiesElement.MarginLeft,
StyleTablePropertiesElement.MarginRight,
StyleTablePropertiesElement.MarginTop,
StyleTablePropertiesElement.MayBreakBetweenRows,
StyleTablePropertiesElement.PageNumber,
StyleTablePropertiesElement.RelWidth,
StyleTablePropertiesElement.Shadow,
StyleTablePropertiesElement.Width,
StyleTablePropertiesElement.WritingMode,
StyleTablePropertiesElement.Align,
StyleTablePropertiesElement.BorderModel,
StyleTablePropertiesElement.Display,
StyleTableRowPropertiesElement.BackgroundColor,
StyleTableRowPropertiesElement.BreakAfter,
StyleTableRowPropertiesElement.BreakBefore,
StyleTableRowPropertiesElement.KeepTogether,
StyleTableRowPropertiesElement.MinRowHeight,
StyleTableRowPropertiesElement.RowHeight,
StyleTableRowPropertiesElement.UseOptimalRowHeight,
StyleTextPropertiesElement.BackgroundColor,
StyleTextPropertiesElement.Color,
StyleTextPropertiesElement.Country,
StyleTextPropertiesElement.FontFamily,
StyleTextPropertiesElement.FontSize,
StyleTextPropertiesElement.FontStyle,
StyleTextPropertiesElement.FontVariant,
StyleTextPropertiesElement.FontWeight,
StyleTextPropertiesElement.Hyphenate,
StyleTextPropertiesElement.HyphenationPushCharCount,
StyleTextPropertiesElement.HyphenationRemainCharCount,
StyleTextPropertiesElement.Language,
StyleTextPropertiesElement.LetterSpacing,
StyleTextPropertiesElement.Script,
StyleTextPropertiesElement.TextShadow,
StyleTextPropertiesElement.TextTransform,
StyleTextPropertiesElement.CountryAsian,
StyleTextPropertiesElement.CountryComplex,
StyleTextPropertiesElement.FontCharset,
StyleTextPropertiesElement.FontCharsetAsian,
StyleTextPropertiesElement.FontCharsetComplex,
StyleTextPropertiesElement.FontFamilyAsian,
StyleTextPropertiesElement.FontFamilyComplex,
StyleTextPropertiesElement.FontFamilyGeneric,
StyleTextPropertiesElement.FontFamilyGenericAsian,
StyleTextPropertiesElement.FontFamilyGenericComplex,
StyleTextPropertiesElement.FontName,
StyleTextPropertiesElement.FontNameAsian,
StyleTextPropertiesElement.FontNameComplex,
StyleTextPropertiesElement.FontPitch,
StyleTextPropertiesElement.FontPitchAsian,
StyleTextPropertiesElement.FontPitchComplex,
StyleTextPropertiesElement.FontRelief,
StyleTextPropertiesElement.FontSizeAsian,
StyleTextPropertiesElement.FontSizeComplex,
StyleTextPropertiesElement.FontSizeRel,
StyleTextPropertiesElement.FontSizeRelAsian,
StyleTextPropertiesElement.FontSizeRelComplex,
StyleTextPropertiesElement.FontStyleAsian,
StyleTextPropertiesElement.FontStyleComplex,
StyleTextPropertiesElement.FontStyleName,
StyleTextPropertiesElement.FontStyleNameAsian,
StyleTextPropertiesElement.FontStyleNameComplex,
StyleTextPropertiesElement.FontWeightAsian,
StyleTextPropertiesElement.FontWeightComplex,
StyleTextPropertiesElement.LanguageAsian,
StyleTextPropertiesElement.LanguageComplex,
StyleTextPropertiesElement.LetterKerning,
StyleTextPropertiesElement.RfcLanguageTag,
StyleTextPropertiesElement.RfcLanguageTagAsian,
StyleTextPropertiesElement.RfcLanguageTagComplex,
StyleTextPropertiesElement.ScriptAsian,
StyleTextPropertiesElement.ScriptComplex,
StyleTextPropertiesElement.ScriptType,
StyleTextPropertiesElement.TextBlinking,
StyleTextPropertiesElement.TextCombine,
StyleTextPropertiesElement.TextCombineEndChar,
StyleTextPropertiesElement.TextCombineStartChar,
StyleTextPropertiesElement.TextEmphasize,
StyleTextPropertiesElement.TextLineThroughColor,
StyleTextPropertiesElement.TextLineThroughMode,
StyleTextPropertiesElement.TextLineThroughStyle,
StyleTextPropertiesElement.TextLineThroughText,
StyleTextPropertiesElement.TextLineThroughTextStyle,
StyleTextPropertiesElement.TextLineThroughType,
StyleTextPropertiesElement.TextLineThroughWidth,
StyleTextPropertiesElement.TextOutline,
StyleTextPropertiesElement.TextOverlineColor,
StyleTextPropertiesElement.TextOverlineMode,
StyleTextPropertiesElement.TextOverlineStyle,
StyleTextPropertiesElement.TextOverlineType,
StyleTextPropertiesElement.TextOverlineWidth,
StyleTextPropertiesElement.TextPosition,
StyleTextPropertiesElement.TextRotationAngle,
StyleTextPropertiesElement.TextRotationScale,
StyleTextPropertiesElement.TextScale,
StyleTextPropertiesElement.TextUnderlineColor,
StyleTextPropertiesElement.TextUnderlineMode,
StyleTextPropertiesElement.TextUnderlineStyle,
StyleTextPropertiesElement.TextUnderlineType,
StyleTextPropertiesElement.TextUnderlineWidth,
StyleTextPropertiesElement.UseWindowFontColor,
StyleTextPropertiesElement.Condition,
StyleTextPropertiesElement.Display,
StyleTextPropertiesElement.Display,
StyleTextPropertiesElement.Display,
});
public final static OdfStyleFamily Paragraph = new OdfStyleFamily("paragraph",
new OdfStyleProperty[]{
StyleChartPropertiesElement.AngleOffset,
StyleChartPropertiesElement.AutoPosition,
StyleChartPropertiesElement.AutoSize,
StyleChartPropertiesElement.AxisLabelPosition,
StyleChartPropertiesElement.AxisPosition,
StyleChartPropertiesElement.ConnectBars,
StyleChartPropertiesElement.DataLabelNumber,
StyleChartPropertiesElement.DataLabelSymbol,
StyleChartPropertiesElement.DataLabelText,
StyleChartPropertiesElement.Deep,
StyleChartPropertiesElement.DisplayLabel,
StyleChartPropertiesElement.ErrorCategory,
StyleChartPropertiesElement.ErrorLowerIndicator,
StyleChartPropertiesElement.ErrorLowerLimit,
StyleChartPropertiesElement.ErrorLowerRange,
StyleChartPropertiesElement.ErrorMargin,
StyleChartPropertiesElement.ErrorPercentage,
StyleChartPropertiesElement.ErrorUpperIndicator,
StyleChartPropertiesElement.ErrorUpperLimit,
StyleChartPropertiesElement.ErrorUpperRange,
StyleChartPropertiesElement.GapWidth,
StyleChartPropertiesElement.GroupBarsPerAxis,
StyleChartPropertiesElement.HoleSize,
StyleChartPropertiesElement.IncludeHiddenCells,
StyleChartPropertiesElement.Interpolation,
StyleChartPropertiesElement.IntervalMajor,
StyleChartPropertiesElement.IntervalMinorDivisor,
StyleChartPropertiesElement.JapaneseCandleStick,
StyleChartPropertiesElement.LabelArrangement,
StyleChartPropertiesElement.LabelPosition,
StyleChartPropertiesElement.LabelPositionNegative,
StyleChartPropertiesElement.Lines,
StyleChartPropertiesElement.LinkDataStyleToSource,
StyleChartPropertiesElement.Logarithmic,
StyleChartPropertiesElement.Maximum,
StyleChartPropertiesElement.MeanValue,
StyleChartPropertiesElement.Minimum,
StyleChartPropertiesElement.Origin,
StyleChartPropertiesElement.Overlap,
StyleChartPropertiesElement.Percentage,
StyleChartPropertiesElement.PieOffset,
StyleChartPropertiesElement.RegressionType,
StyleChartPropertiesElement.ReverseDirection,
StyleChartPropertiesElement.RightAngledAxes,
StyleChartPropertiesElement.ScaleText,
StyleChartPropertiesElement.SeriesSource,
StyleChartPropertiesElement.SolidType,
StyleChartPropertiesElement.SortByXValues,
StyleChartPropertiesElement.SplineOrder,
StyleChartPropertiesElement.SplineResolution,
StyleChartPropertiesElement.Stacked,
StyleChartPropertiesElement.SymbolHeight,
StyleChartPropertiesElement.SymbolName,
StyleChartPropertiesElement.SymbolType,
StyleChartPropertiesElement.SymbolType,
StyleChartPropertiesElement.SymbolType,
StyleChartPropertiesElement.SymbolType,
StyleChartPropertiesElement.SymbolWidth,
StyleChartPropertiesElement.TextOverlap,
StyleChartPropertiesElement.ThreeDimensional,
StyleChartPropertiesElement.TickMarkPosition,
StyleChartPropertiesElement.TickMarksMajorInner,
StyleChartPropertiesElement.TickMarksMajorOuter,
StyleChartPropertiesElement.TickMarksMinorInner,
StyleChartPropertiesElement.TickMarksMinorOuter,
StyleChartPropertiesElement.TreatEmptyCells,
StyleChartPropertiesElement.Vertical,
StyleChartPropertiesElement.Visible,
StyleChartPropertiesElement.Direction,
StyleChartPropertiesElement.RotationAngle,
StyleChartPropertiesElement.LineBreak,
StyleDrawingPagePropertiesElement.BackgroundSize,
StyleDrawingPagePropertiesElement.Fill,
StyleDrawingPagePropertiesElement.FillColor,
StyleDrawingPagePropertiesElement.FillGradientName,
StyleDrawingPagePropertiesElement.FillHatchName,
StyleDrawingPagePropertiesElement.FillHatchSolid,
StyleDrawingPagePropertiesElement.FillImageHeight,
StyleDrawingPagePropertiesElement.FillImageName,
StyleDrawingPagePropertiesElement.FillImageRefPoint,
StyleDrawingPagePropertiesElement.FillImageRefPointX,
StyleDrawingPagePropertiesElement.FillImageRefPointY,
StyleDrawingPagePropertiesElement.FillImageWidth,
StyleDrawingPagePropertiesElement.GradientStepCount,
StyleDrawingPagePropertiesElement.Opacity,
StyleDrawingPagePropertiesElement.OpacityName,
StyleDrawingPagePropertiesElement.SecondaryFillColor,
StyleDrawingPagePropertiesElement.TileRepeatOffset,
StyleDrawingPagePropertiesElement.BackgroundObjectsVisible,
StyleDrawingPagePropertiesElement.BackgroundVisible,
StyleDrawingPagePropertiesElement.DisplayDateTime,
StyleDrawingPagePropertiesElement.DisplayFooter,
StyleDrawingPagePropertiesElement.DisplayHeader,
StyleDrawingPagePropertiesElement.DisplayPageNumber,
StyleDrawingPagePropertiesElement.Duration,
StyleDrawingPagePropertiesElement.TransitionSpeed,
StyleDrawingPagePropertiesElement.TransitionStyle,
StyleDrawingPagePropertiesElement.TransitionType,
StyleDrawingPagePropertiesElement.Visibility,
StyleDrawingPagePropertiesElement.Direction,
StyleDrawingPagePropertiesElement.FadeColor,
StyleDrawingPagePropertiesElement.Subtype,
StyleDrawingPagePropertiesElement.Type,
StyleDrawingPagePropertiesElement.Repeat,
StyleDrawingPagePropertiesElement.FillRule,
StyleGraphicPropertiesElement.AmbientColor,
StyleGraphicPropertiesElement.BackScale,
StyleGraphicPropertiesElement.BackfaceCulling,
StyleGraphicPropertiesElement.CloseBack,
StyleGraphicPropertiesElement.CloseFront,
StyleGraphicPropertiesElement.Depth,
StyleGraphicPropertiesElement.DiffuseColor,
StyleGraphicPropertiesElement.EdgeRounding,
StyleGraphicPropertiesElement.EdgeRoundingMode,
StyleGraphicPropertiesElement.EmissiveColor,
StyleGraphicPropertiesElement.EndAngle,
StyleGraphicPropertiesElement.HorizontalSegments,
StyleGraphicPropertiesElement.LightingMode,
StyleGraphicPropertiesElement.NormalsDirection,
StyleGraphicPropertiesElement.NormalsKind,
StyleGraphicPropertiesElement.Dr3dShadow,
StyleGraphicPropertiesElement.Shininess,
StyleGraphicPropertiesElement.SpecularColor,
StyleGraphicPropertiesElement.TextureFilter,
StyleGraphicPropertiesElement.TextureGenerationModeX,
StyleGraphicPropertiesElement.TextureGenerationModeY,
StyleGraphicPropertiesElement.TextureKind,
StyleGraphicPropertiesElement.TextureMode,
StyleGraphicPropertiesElement.VerticalSegments,
StyleGraphicPropertiesElement.AutoGrowHeight,
StyleGraphicPropertiesElement.AutoGrowWidth,
StyleGraphicPropertiesElement.Blue,
StyleGraphicPropertiesElement.CaptionAngle,
StyleGraphicPropertiesElement.CaptionAngleType,
StyleGraphicPropertiesElement.CaptionEscape,
StyleGraphicPropertiesElement.CaptionEscapeDirection,
StyleGraphicPropertiesElement.CaptionFitLineLength,
StyleGraphicPropertiesElement.CaptionGap,
StyleGraphicPropertiesElement.CaptionLineLength,
StyleGraphicPropertiesElement.CaptionType,
StyleGraphicPropertiesElement.ColorInversion,
StyleGraphicPropertiesElement.ColorMode,
StyleGraphicPropertiesElement.Contrast,
StyleGraphicPropertiesElement.DecimalPlaces,
StyleGraphicPropertiesElement.DrawAspect,
StyleGraphicPropertiesElement.EndGuide,
StyleGraphicPropertiesElement.EndLineSpacingHorizontal,
StyleGraphicPropertiesElement.EndLineSpacingVertical,
StyleGraphicPropertiesElement.Fill,
StyleGraphicPropertiesElement.FillColor,
StyleGraphicPropertiesElement.FillGradientName,
StyleGraphicPropertiesElement.FillHatchName,
StyleGraphicPropertiesElement.FillHatchSolid,
StyleGraphicPropertiesElement.FillImageHeight,
StyleGraphicPropertiesElement.FillImageName,
StyleGraphicPropertiesElement.FillImageRefPoint,
StyleGraphicPropertiesElement.FillImageRefPointX,
StyleGraphicPropertiesElement.FillImageRefPointY,
StyleGraphicPropertiesElement.FillImageWidth,
StyleGraphicPropertiesElement.FitToContour,
StyleGraphicPropertiesElement.FitToSize,
StyleGraphicPropertiesElement.FrameDisplayBorder,
StyleGraphicPropertiesElement.FrameDisplayScrollbar,
StyleGraphicPropertiesElement.FrameMarginHorizontal,
StyleGraphicPropertiesElement.FrameMarginVertical,
StyleGraphicPropertiesElement.Gamma,
StyleGraphicPropertiesElement.GradientStepCount,
StyleGraphicPropertiesElement.Green,
StyleGraphicPropertiesElement.GuideDistance,
StyleGraphicPropertiesElement.GuideOverhang,
StyleGraphicPropertiesElement.ImageOpacity,
StyleGraphicPropertiesElement.LineDistance,
StyleGraphicPropertiesElement.Luminance,
StyleGraphicPropertiesElement.MarkerEnd,
StyleGraphicPropertiesElement.MarkerEndCenter,
StyleGraphicPropertiesElement.MarkerEndWidth,
StyleGraphicPropertiesElement.MarkerStart,
StyleGraphicPropertiesElement.MarkerStartCenter,
StyleGraphicPropertiesElement.MarkerStartWidth,
StyleGraphicPropertiesElement.MeasureAlign,
StyleGraphicPropertiesElement.MeasureVerticalAlign,
StyleGraphicPropertiesElement.OleDrawAspect,
StyleGraphicPropertiesElement.Opacity,
StyleGraphicPropertiesElement.OpacityName,
StyleGraphicPropertiesElement.Parallel,
StyleGraphicPropertiesElement.Placing,
StyleGraphicPropertiesElement.Red,
StyleGraphicPropertiesElement.SecondaryFillColor,
StyleGraphicPropertiesElement.DrawShadow,
StyleGraphicPropertiesElement.ShadowColor,
StyleGraphicPropertiesElement.ShadowOffsetX,
StyleGraphicPropertiesElement.ShadowOffsetY,
StyleGraphicPropertiesElement.ShadowOpacity,
StyleGraphicPropertiesElement.ShowUnit,
StyleGraphicPropertiesElement.StartGuide,
StyleGraphicPropertiesElement.StartLineSpacingHorizontal,
StyleGraphicPropertiesElement.StartLineSpacingVertical,
StyleGraphicPropertiesElement.Stroke,
StyleGraphicPropertiesElement.StrokeDash,
StyleGraphicPropertiesElement.StrokeDashNames,
StyleGraphicPropertiesElement.StrokeLinejoin,
StyleGraphicPropertiesElement.SymbolColor,
StyleGraphicPropertiesElement.TextareaHorizontalAlign,
StyleGraphicPropertiesElement.TextareaVerticalAlign,
StyleGraphicPropertiesElement.TileRepeatOffset,
StyleGraphicPropertiesElement.Unit,
StyleGraphicPropertiesElement.VisibleAreaHeight,
StyleGraphicPropertiesElement.VisibleAreaLeft,
StyleGraphicPropertiesElement.VisibleAreaTop,
StyleGraphicPropertiesElement.VisibleAreaWidth,
StyleGraphicPropertiesElement.WrapInfluenceOnPosition,
StyleGraphicPropertiesElement.BackgroundColor,
StyleGraphicPropertiesElement.Border,
StyleGraphicPropertiesElement.BorderBottom,
StyleGraphicPropertiesElement.BorderLeft,
StyleGraphicPropertiesElement.BorderRight,
StyleGraphicPropertiesElement.BorderTop,
StyleGraphicPropertiesElement.Clip,
StyleGraphicPropertiesElement.Margin,
StyleGraphicPropertiesElement.MarginBottom,
StyleGraphicPropertiesElement.MarginLeft,
StyleGraphicPropertiesElement.MarginRight,
StyleGraphicPropertiesElement.MarginTop,
StyleGraphicPropertiesElement.MaxHeight,
StyleGraphicPropertiesElement.MaxWidth,
StyleGraphicPropertiesElement.MinHeight,
StyleGraphicPropertiesElement.MinWidth,
StyleGraphicPropertiesElement.Padding,
StyleGraphicPropertiesElement.PaddingBottom,
StyleGraphicPropertiesElement.PaddingLeft,
StyleGraphicPropertiesElement.PaddingRight,
StyleGraphicPropertiesElement.PaddingTop,
StyleGraphicPropertiesElement.WrapOption,
StyleGraphicPropertiesElement.BackgroundTransparency,
StyleGraphicPropertiesElement.BorderLineWidth,
StyleGraphicPropertiesElement.BorderLineWidthBottom,
StyleGraphicPropertiesElement.BorderLineWidthLeft,
StyleGraphicPropertiesElement.BorderLineWidthRight,
StyleGraphicPropertiesElement.BorderLineWidthTop,
StyleGraphicPropertiesElement.Editable,
StyleGraphicPropertiesElement.FlowWithText,
StyleGraphicPropertiesElement.HorizontalPos,
StyleGraphicPropertiesElement.HorizontalRel,
StyleGraphicPropertiesElement.Mirror,
StyleGraphicPropertiesElement.NumberWrappedParagraphs,
StyleGraphicPropertiesElement.OverflowBehavior,
StyleGraphicPropertiesElement.PrintContent,
StyleGraphicPropertiesElement.Protect,
StyleGraphicPropertiesElement.RelHeight,
StyleGraphicPropertiesElement.RelWidth,
StyleGraphicPropertiesElement.Repeat,
StyleGraphicPropertiesElement.RunThrough,
StyleGraphicPropertiesElement.StyleShadow,
StyleGraphicPropertiesElement.ShrinkToFit,
StyleGraphicPropertiesElement.VerticalPos,
StyleGraphicPropertiesElement.VerticalRel,
StyleGraphicPropertiesElement.Wrap,
StyleGraphicPropertiesElement.WrapContour,
StyleGraphicPropertiesElement.WrapContourMode,
StyleGraphicPropertiesElement.WrapDynamicThreshold,
StyleGraphicPropertiesElement.WritingMode,
StyleGraphicPropertiesElement.FillRule,
StyleGraphicPropertiesElement.Height,
StyleGraphicPropertiesElement.StrokeColor,
StyleGraphicPropertiesElement.StrokeLinecap,
StyleGraphicPropertiesElement.StrokeOpacity,
StyleGraphicPropertiesElement.StrokeWidth,
StyleGraphicPropertiesElement.Width,
StyleGraphicPropertiesElement.X,
StyleGraphicPropertiesElement.Y,
StyleGraphicPropertiesElement.AnchorPageNumber,
StyleGraphicPropertiesElement.AnchorType,
StyleGraphicPropertiesElement.Animation,
StyleGraphicPropertiesElement.AnimationDelay,
StyleGraphicPropertiesElement.AnimationDirection,
StyleGraphicPropertiesElement.AnimationRepeat,
StyleGraphicPropertiesElement.AnimationStartInside,
StyleGraphicPropertiesElement.AnimationSteps,
StyleGraphicPropertiesElement.AnimationStopInside,
StyleHeaderFooterPropertiesElement.BackgroundColor,
StyleHeaderFooterPropertiesElement.Border,
StyleHeaderFooterPropertiesElement.BorderBottom,
StyleHeaderFooterPropertiesElement.BorderLeft,
StyleHeaderFooterPropertiesElement.BorderRight,
StyleHeaderFooterPropertiesElement.BorderTop,
StyleHeaderFooterPropertiesElement.Margin,
StyleHeaderFooterPropertiesElement.MarginBottom,
StyleHeaderFooterPropertiesElement.MarginLeft,
StyleHeaderFooterPropertiesElement.MarginRight,
StyleHeaderFooterPropertiesElement.MarginTop,
StyleHeaderFooterPropertiesElement.MinHeight,
StyleHeaderFooterPropertiesElement.Padding,
StyleHeaderFooterPropertiesElement.PaddingBottom,
StyleHeaderFooterPropertiesElement.PaddingLeft,
StyleHeaderFooterPropertiesElement.PaddingRight,
StyleHeaderFooterPropertiesElement.PaddingTop,
StyleHeaderFooterPropertiesElement.BorderLineWidth,
StyleHeaderFooterPropertiesElement.BorderLineWidthBottom,
StyleHeaderFooterPropertiesElement.BorderLineWidthLeft,
StyleHeaderFooterPropertiesElement.BorderLineWidthRight,
StyleHeaderFooterPropertiesElement.BorderLineWidthTop,
StyleHeaderFooterPropertiesElement.DynamicSpacing,
StyleHeaderFooterPropertiesElement.Shadow,
StyleHeaderFooterPropertiesElement.Height,
StyleListLevelPropertiesElement.Height,
StyleListLevelPropertiesElement.TextAlign,
StyleListLevelPropertiesElement.Width,
StyleListLevelPropertiesElement.FontName,
StyleListLevelPropertiesElement.VerticalPos,
StyleListLevelPropertiesElement.VerticalRel,
StyleListLevelPropertiesElement.Y,
StyleListLevelPropertiesElement.ListLevelPositionAndSpaceMode,
StyleListLevelPropertiesElement.MinLabelDistance,
StyleListLevelPropertiesElement.MinLabelWidth,
StyleListLevelPropertiesElement.SpaceBefore,
StylePageLayoutPropertiesElement.BackgroundColor,
StylePageLayoutPropertiesElement.Border,
StylePageLayoutPropertiesElement.BorderBottom,
StylePageLayoutPropertiesElement.BorderLeft,
StylePageLayoutPropertiesElement.BorderRight,
StylePageLayoutPropertiesElement.BorderTop,
StylePageLayoutPropertiesElement.Margin,
StylePageLayoutPropertiesElement.MarginBottom,
StylePageLayoutPropertiesElement.MarginLeft,
StylePageLayoutPropertiesElement.MarginRight,
StylePageLayoutPropertiesElement.MarginTop,
StylePageLayoutPropertiesElement.Padding,
StylePageLayoutPropertiesElement.PaddingBottom,
StylePageLayoutPropertiesElement.PaddingLeft,
StylePageLayoutPropertiesElement.PaddingRight,
StylePageLayoutPropertiesElement.PaddingTop,
StylePageLayoutPropertiesElement.PageHeight,
StylePageLayoutPropertiesElement.PageWidth,
StylePageLayoutPropertiesElement.BorderLineWidth,
StylePageLayoutPropertiesElement.BorderLineWidthBottom,
StylePageLayoutPropertiesElement.BorderLineWidthLeft,
StylePageLayoutPropertiesElement.BorderLineWidthRight,
StylePageLayoutPropertiesElement.BorderLineWidthTop,
StylePageLayoutPropertiesElement.FirstPageNumber,
StylePageLayoutPropertiesElement.FootnoteMaxHeight,
StylePageLayoutPropertiesElement.LayoutGridBaseHeight,
StylePageLayoutPropertiesElement.LayoutGridBaseWidth,
StylePageLayoutPropertiesElement.LayoutGridColor,
StylePageLayoutPropertiesElement.LayoutGridDisplay,
StylePageLayoutPropertiesElement.LayoutGridLines,
StylePageLayoutPropertiesElement.LayoutGridMode,
StylePageLayoutPropertiesElement.LayoutGridPrint,
StylePageLayoutPropertiesElement.LayoutGridRubyBelow,
StylePageLayoutPropertiesElement.LayoutGridRubyHeight,
StylePageLayoutPropertiesElement.LayoutGridSnapTo,
StylePageLayoutPropertiesElement.LayoutGridStandardMode,
StylePageLayoutPropertiesElement.NumFormat,
StylePageLayoutPropertiesElement.NumFormat,
StylePageLayoutPropertiesElement.NumLetterSync,
StylePageLayoutPropertiesElement.NumPrefix,
StylePageLayoutPropertiesElement.NumSuffix,
StylePageLayoutPropertiesElement.PaperTrayName,
StylePageLayoutPropertiesElement.Print,
StylePageLayoutPropertiesElement.PrintOrientation,
StylePageLayoutPropertiesElement.PrintPageOrder,
StylePageLayoutPropertiesElement.RegisterTruthRefStyleName,
StylePageLayoutPropertiesElement.ScaleTo,
StylePageLayoutPropertiesElement.ScaleToPages,
StylePageLayoutPropertiesElement.Shadow,
StylePageLayoutPropertiesElement.TableCentering,
StylePageLayoutPropertiesElement.WritingMode,
StyleParagraphPropertiesElement.BackgroundColor,
StyleParagraphPropertiesElement.Border,
StyleParagraphPropertiesElement.BorderBottom,
StyleParagraphPropertiesElement.BorderLeft,
StyleParagraphPropertiesElement.BorderRight,
StyleParagraphPropertiesElement.BorderTop,
StyleParagraphPropertiesElement.BreakAfter,
StyleParagraphPropertiesElement.BreakBefore,
StyleParagraphPropertiesElement.HyphenationKeep,
StyleParagraphPropertiesElement.HyphenationLadderCount,
StyleParagraphPropertiesElement.KeepTogether,
StyleParagraphPropertiesElement.KeepWithNext,
StyleParagraphPropertiesElement.LineHeight,
StyleParagraphPropertiesElement.Margin,
StyleParagraphPropertiesElement.MarginBottom,
StyleParagraphPropertiesElement.MarginLeft,
StyleParagraphPropertiesElement.MarginRight,
StyleParagraphPropertiesElement.MarginTop,
StyleParagraphPropertiesElement.Orphans,
StyleParagraphPropertiesElement.Padding,
StyleParagraphPropertiesElement.PaddingBottom,
StyleParagraphPropertiesElement.PaddingLeft,
StyleParagraphPropertiesElement.PaddingRight,
StyleParagraphPropertiesElement.PaddingTop,
StyleParagraphPropertiesElement.TextAlign,
StyleParagraphPropertiesElement.TextAlignLast,
StyleParagraphPropertiesElement.TextIndent,
StyleParagraphPropertiesElement.Widows,
StyleParagraphPropertiesElement.AutoTextIndent,
StyleParagraphPropertiesElement.BackgroundTransparency,
StyleParagraphPropertiesElement.BorderLineWidth,
StyleParagraphPropertiesElement.BorderLineWidthBottom,
StyleParagraphPropertiesElement.BorderLineWidthLeft,
StyleParagraphPropertiesElement.BorderLineWidthRight,
StyleParagraphPropertiesElement.BorderLineWidthTop,
StyleParagraphPropertiesElement.FontIndependentLineSpacing,
StyleParagraphPropertiesElement.JoinBorder,
StyleParagraphPropertiesElement.JustifySingleWord,
StyleParagraphPropertiesElement.LineBreak,
StyleParagraphPropertiesElement.LineHeightAtLeast,
StyleParagraphPropertiesElement.LineSpacing,
StyleParagraphPropertiesElement.PageNumber,
StyleParagraphPropertiesElement.PunctuationWrap,
StyleParagraphPropertiesElement.RegisterTrue,
StyleParagraphPropertiesElement.Shadow,
StyleParagraphPropertiesElement.SnapToLayoutGrid,
StyleParagraphPropertiesElement.TabStopDistance,
StyleParagraphPropertiesElement.TextAutospace,
StyleParagraphPropertiesElement.VerticalAlign,
StyleParagraphPropertiesElement.WritingMode,
StyleParagraphPropertiesElement.WritingModeAutomatic,
StyleParagraphPropertiesElement.LineNumber,
StyleParagraphPropertiesElement.NumberLines,
StyleRubyPropertiesElement.RubyAlign,
StyleRubyPropertiesElement.RubyPosition,
StyleSectionPropertiesElement.BackgroundColor,
StyleSectionPropertiesElement.MarginLeft,
StyleSectionPropertiesElement.MarginRight,
StyleSectionPropertiesElement.Editable,
StyleSectionPropertiesElement.Protect,
StyleSectionPropertiesElement.WritingMode,
StyleSectionPropertiesElement.DontBalanceTextColumns,
StyleTableCellPropertiesElement.BackgroundColor,
StyleTableCellPropertiesElement.Border,
StyleTableCellPropertiesElement.BorderBottom,
StyleTableCellPropertiesElement.BorderLeft,
StyleTableCellPropertiesElement.BorderRight,
StyleTableCellPropertiesElement.BorderTop,
StyleTableCellPropertiesElement.Padding,
StyleTableCellPropertiesElement.PaddingBottom,
StyleTableCellPropertiesElement.PaddingLeft,
StyleTableCellPropertiesElement.PaddingRight,
StyleTableCellPropertiesElement.PaddingTop,
StyleTableCellPropertiesElement.WrapOption,
StyleTableCellPropertiesElement.BorderLineWidth,
StyleTableCellPropertiesElement.BorderLineWidthBottom,
StyleTableCellPropertiesElement.BorderLineWidthLeft,
StyleTableCellPropertiesElement.BorderLineWidthRight,
StyleTableCellPropertiesElement.BorderLineWidthTop,
StyleTableCellPropertiesElement.CellProtect,
StyleTableCellPropertiesElement.DecimalPlaces,
StyleTableCellPropertiesElement.DiagonalBlTr,
StyleTableCellPropertiesElement.DiagonalBlTrWidths,
StyleTableCellPropertiesElement.DiagonalTlBr,
StyleTableCellPropertiesElement.DiagonalTlBrWidths,
StyleTableCellPropertiesElement.Direction,
StyleTableCellPropertiesElement.GlyphOrientationVertical,
StyleTableCellPropertiesElement.PrintContent,
StyleTableCellPropertiesElement.RepeatContent,
StyleTableCellPropertiesElement.RotationAlign,
StyleTableCellPropertiesElement.RotationAngle,
StyleTableCellPropertiesElement.Shadow,
StyleTableCellPropertiesElement.ShrinkToFit,
StyleTableCellPropertiesElement.TextAlignSource,
StyleTableCellPropertiesElement.VerticalAlign,
StyleTableCellPropertiesElement.WritingMode,
StyleTableColumnPropertiesElement.BreakAfter,
StyleTableColumnPropertiesElement.BreakBefore,
StyleTableColumnPropertiesElement.ColumnWidth,
StyleTableColumnPropertiesElement.RelColumnWidth,
StyleTableColumnPropertiesElement.UseOptimalColumnWidth,
StyleTablePropertiesElement.BackgroundColor,
StyleTablePropertiesElement.BreakAfter,
StyleTablePropertiesElement.BreakBefore,
StyleTablePropertiesElement.KeepWithNext,
StyleTablePropertiesElement.Margin,
StyleTablePropertiesElement.MarginBottom,
StyleTablePropertiesElement.MarginLeft,
StyleTablePropertiesElement.MarginRight,
StyleTablePropertiesElement.MarginTop,
StyleTablePropertiesElement.MayBreakBetweenRows,
StyleTablePropertiesElement.PageNumber,
StyleTablePropertiesElement.RelWidth,
StyleTablePropertiesElement.Shadow,
StyleTablePropertiesElement.Width,
StyleTablePropertiesElement.WritingMode,
StyleTablePropertiesElement.Align,
StyleTablePropertiesElement.BorderModel,
StyleTablePropertiesElement.Display,
StyleTableRowPropertiesElement.BackgroundColor,
StyleTableRowPropertiesElement.BreakAfter,
StyleTableRowPropertiesElement.BreakBefore,
StyleTableRowPropertiesElement.KeepTogether,
StyleTableRowPropertiesElement.MinRowHeight,
StyleTableRowPropertiesElement.RowHeight,
StyleTableRowPropertiesElement.UseOptimalRowHeight,
StyleTextPropertiesElement.BackgroundColor,
StyleTextPropertiesElement.Color,
StyleTextPropertiesElement.Country,
StyleTextPropertiesElement.FontFamily,
StyleTextPropertiesElement.FontSize,
StyleTextPropertiesElement.FontStyle,
StyleTextPropertiesElement.FontVariant,
StyleTextPropertiesElement.FontWeight,
StyleTextPropertiesElement.Hyphenate,
StyleTextPropertiesElement.HyphenationPushCharCount,
StyleTextPropertiesElement.HyphenationRemainCharCount,
StyleTextPropertiesElement.Language,
StyleTextPropertiesElement.LetterSpacing,
StyleTextPropertiesElement.Script,
StyleTextPropertiesElement.TextShadow,
StyleTextPropertiesElement.TextTransform,
StyleTextPropertiesElement.CountryAsian,
StyleTextPropertiesElement.CountryComplex,
StyleTextPropertiesElement.FontCharset,
StyleTextPropertiesElement.FontCharsetAsian,
StyleTextPropertiesElement.FontCharsetComplex,
StyleTextPropertiesElement.FontFamilyAsian,
StyleTextPropertiesElement.FontFamilyComplex,
StyleTextPropertiesElement.FontFamilyGeneric,
StyleTextPropertiesElement.FontFamilyGenericAsian,
StyleTextPropertiesElement.FontFamilyGenericComplex,
StyleTextPropertiesElement.FontName,
StyleTextPropertiesElement.FontNameAsian,
StyleTextPropertiesElement.FontNameComplex,
StyleTextPropertiesElement.FontPitch,
StyleTextPropertiesElement.FontPitchAsian,
StyleTextPropertiesElement.FontPitchComplex,
StyleTextPropertiesElement.FontRelief,
StyleTextPropertiesElement.FontSizeAsian,
StyleTextPropertiesElement.FontSizeComplex,
StyleTextPropertiesElement.FontSizeRel,
StyleTextPropertiesElement.FontSizeRelAsian,
StyleTextPropertiesElement.FontSizeRelComplex,
StyleTextPropertiesElement.FontStyleAsian,
StyleTextPropertiesElement.FontStyleComplex,
StyleTextPropertiesElement.FontStyleName,
StyleTextPropertiesElement.FontStyleNameAsian,
StyleTextPropertiesElement.FontStyleNameComplex,
StyleTextPropertiesElement.FontWeightAsian,
StyleTextPropertiesElement.FontWeightComplex,
StyleTextPropertiesElement.LanguageAsian,
StyleTextPropertiesElement.LanguageComplex,
StyleTextPropertiesElement.LetterKerning,
StyleTextPropertiesElement.RfcLanguageTag,
StyleTextPropertiesElement.RfcLanguageTagAsian,
StyleTextPropertiesElement.RfcLanguageTagComplex,
StyleTextPropertiesElement.ScriptAsian,
StyleTextPropertiesElement.ScriptComplex,
StyleTextPropertiesElement.ScriptType,
StyleTextPropertiesElement.TextBlinking,
StyleTextPropertiesElement.TextCombine,
StyleTextPropertiesElement.TextCombineEndChar,
StyleTextPropertiesElement.TextCombineStartChar,
StyleTextPropertiesElement.TextEmphasize,
StyleTextPropertiesElement.TextLineThroughColor,
StyleTextPropertiesElement.TextLineThroughMode,
StyleTextPropertiesElement.TextLineThroughStyle,
StyleTextPropertiesElement.TextLineThroughText,
StyleTextPropertiesElement.TextLineThroughTextStyle,
StyleTextPropertiesElement.TextLineThroughType,
StyleTextPropertiesElement.TextLineThroughWidth,
StyleTextPropertiesElement.TextOutline,
StyleTextPropertiesElement.TextOverlineColor,
StyleTextPropertiesElement.TextOverlineMode,
StyleTextPropertiesElement.TextOverlineStyle,
StyleTextPropertiesElement.TextOverlineType,
StyleTextPropertiesElement.TextOverlineWidth,
StyleTextPropertiesElement.TextPosition,
StyleTextPropertiesElement.TextRotationAngle,
StyleTextPropertiesElement.TextRotationScale,
StyleTextPropertiesElement.TextScale,
StyleTextPropertiesElement.TextUnderlineColor,
StyleTextPropertiesElement.TextUnderlineMode,
StyleTextPropertiesElement.TextUnderlineStyle,
StyleTextPropertiesElement.TextUnderlineType,
StyleTextPropertiesElement.TextUnderlineWidth,
StyleTextPropertiesElement.UseWindowFontColor,
StyleTextPropertiesElement.Condition,
StyleTextPropertiesElement.Display,
StyleTextPropertiesElement.Display,
StyleTextPropertiesElement.Display,
});
public final static OdfStyleFamily Ruby = new OdfStyleFamily("ruby",
new OdfStyleProperty[]{
StyleChartPropertiesElement.AngleOffset,
StyleChartPropertiesElement.AutoPosition,
StyleChartPropertiesElement.AutoSize,
StyleChartPropertiesElement.AxisLabelPosition,
StyleChartPropertiesElement.AxisPosition,
StyleChartPropertiesElement.ConnectBars,
StyleChartPropertiesElement.DataLabelNumber,
StyleChartPropertiesElement.DataLabelSymbol,
StyleChartPropertiesElement.DataLabelText,
StyleChartPropertiesElement.Deep,
StyleChartPropertiesElement.DisplayLabel,
StyleChartPropertiesElement.ErrorCategory,
StyleChartPropertiesElement.ErrorLowerIndicator,
StyleChartPropertiesElement.ErrorLowerLimit,
StyleChartPropertiesElement.ErrorLowerRange,
StyleChartPropertiesElement.ErrorMargin,
StyleChartPropertiesElement.ErrorPercentage,
StyleChartPropertiesElement.ErrorUpperIndicator,
StyleChartPropertiesElement.ErrorUpperLimit,
StyleChartPropertiesElement.ErrorUpperRange,
StyleChartPropertiesElement.GapWidth,
StyleChartPropertiesElement.GroupBarsPerAxis,
StyleChartPropertiesElement.HoleSize,
StyleChartPropertiesElement.IncludeHiddenCells,
StyleChartPropertiesElement.Interpolation,
StyleChartPropertiesElement.IntervalMajor,
StyleChartPropertiesElement.IntervalMinorDivisor,
StyleChartPropertiesElement.JapaneseCandleStick,
StyleChartPropertiesElement.LabelArrangement,
StyleChartPropertiesElement.LabelPosition,
StyleChartPropertiesElement.LabelPositionNegative,
StyleChartPropertiesElement.Lines,
StyleChartPropertiesElement.LinkDataStyleToSource,
StyleChartPropertiesElement.Logarithmic,
StyleChartPropertiesElement.Maximum,
StyleChartPropertiesElement.MeanValue,
StyleChartPropertiesElement.Minimum,
StyleChartPropertiesElement.Origin,
StyleChartPropertiesElement.Overlap,
StyleChartPropertiesElement.Percentage,
StyleChartPropertiesElement.PieOffset,
StyleChartPropertiesElement.RegressionType,
StyleChartPropertiesElement.ReverseDirection,
StyleChartPropertiesElement.RightAngledAxes,
StyleChartPropertiesElement.ScaleText,
StyleChartPropertiesElement.SeriesSource,
StyleChartPropertiesElement.SolidType,
StyleChartPropertiesElement.SortByXValues,
StyleChartPropertiesElement.SplineOrder,
StyleChartPropertiesElement.SplineResolution,
StyleChartPropertiesElement.Stacked,
StyleChartPropertiesElement.SymbolHeight,
StyleChartPropertiesElement.SymbolName,
StyleChartPropertiesElement.SymbolType,
StyleChartPropertiesElement.SymbolType,
StyleChartPropertiesElement.SymbolType,
StyleChartPropertiesElement.SymbolType,
StyleChartPropertiesElement.SymbolWidth,
StyleChartPropertiesElement.TextOverlap,
StyleChartPropertiesElement.ThreeDimensional,
StyleChartPropertiesElement.TickMarkPosition,
StyleChartPropertiesElement.TickMarksMajorInner,
StyleChartPropertiesElement.TickMarksMajorOuter,
StyleChartPropertiesElement.TickMarksMinorInner,
StyleChartPropertiesElement.TickMarksMinorOuter,
StyleChartPropertiesElement.TreatEmptyCells,
StyleChartPropertiesElement.Vertical,
StyleChartPropertiesElement.Visible,
StyleChartPropertiesElement.Direction,
StyleChartPropertiesElement.RotationAngle,
StyleChartPropertiesElement.LineBreak,
StyleDrawingPagePropertiesElement.BackgroundSize,
StyleDrawingPagePropertiesElement.Fill,
StyleDrawingPagePropertiesElement.FillColor,
StyleDrawingPagePropertiesElement.FillGradientName,
StyleDrawingPagePropertiesElement.FillHatchName,
StyleDrawingPagePropertiesElement.FillHatchSolid,
StyleDrawingPagePropertiesElement.FillImageHeight,
StyleDrawingPagePropertiesElement.FillImageName,
StyleDrawingPagePropertiesElement.FillImageRefPoint,
StyleDrawingPagePropertiesElement.FillImageRefPointX,
StyleDrawingPagePropertiesElement.FillImageRefPointY,
StyleDrawingPagePropertiesElement.FillImageWidth,
StyleDrawingPagePropertiesElement.GradientStepCount,
StyleDrawingPagePropertiesElement.Opacity,
StyleDrawingPagePropertiesElement.OpacityName,
StyleDrawingPagePropertiesElement.SecondaryFillColor,
StyleDrawingPagePropertiesElement.TileRepeatOffset,
StyleDrawingPagePropertiesElement.BackgroundObjectsVisible,
StyleDrawingPagePropertiesElement.BackgroundVisible,
StyleDrawingPagePropertiesElement.DisplayDateTime,
StyleDrawingPagePropertiesElement.DisplayFooter,
StyleDrawingPagePropertiesElement.DisplayHeader,
StyleDrawingPagePropertiesElement.DisplayPageNumber,
StyleDrawingPagePropertiesElement.Duration,
StyleDrawingPagePropertiesElement.TransitionSpeed,
StyleDrawingPagePropertiesElement.TransitionStyle,
StyleDrawingPagePropertiesElement.TransitionType,
StyleDrawingPagePropertiesElement.Visibility,
StyleDrawingPagePropertiesElement.Direction,
StyleDrawingPagePropertiesElement.FadeColor,
StyleDrawingPagePropertiesElement.Subtype,
StyleDrawingPagePropertiesElement.Type,
StyleDrawingPagePropertiesElement.Repeat,
StyleDrawingPagePropertiesElement.FillRule,
StyleGraphicPropertiesElement.AmbientColor,
StyleGraphicPropertiesElement.BackScale,
StyleGraphicPropertiesElement.BackfaceCulling,
StyleGraphicPropertiesElement.CloseBack,
StyleGraphicPropertiesElement.CloseFront,
StyleGraphicPropertiesElement.Depth,
StyleGraphicPropertiesElement.DiffuseColor,
StyleGraphicPropertiesElement.EdgeRounding,
StyleGraphicPropertiesElement.EdgeRoundingMode,
StyleGraphicPropertiesElement.EmissiveColor,
StyleGraphicPropertiesElement.EndAngle,
StyleGraphicPropertiesElement.HorizontalSegments,
StyleGraphicPropertiesElement.LightingMode,
StyleGraphicPropertiesElement.NormalsDirection,
StyleGraphicPropertiesElement.NormalsKind,
StyleGraphicPropertiesElement.Dr3dShadow,
StyleGraphicPropertiesElement.Shininess,
StyleGraphicPropertiesElement.SpecularColor,
StyleGraphicPropertiesElement.TextureFilter,
StyleGraphicPropertiesElement.TextureGenerationModeX,
StyleGraphicPropertiesElement.TextureGenerationModeY,
StyleGraphicPropertiesElement.TextureKind,
StyleGraphicPropertiesElement.TextureMode,
StyleGraphicPropertiesElement.VerticalSegments,
StyleGraphicPropertiesElement.AutoGrowHeight,
StyleGraphicPropertiesElement.AutoGrowWidth,
StyleGraphicPropertiesElement.Blue,
StyleGraphicPropertiesElement.CaptionAngle,
StyleGraphicPropertiesElement.CaptionAngleType,
StyleGraphicPropertiesElement.CaptionEscape,
StyleGraphicPropertiesElement.CaptionEscapeDirection,
StyleGraphicPropertiesElement.CaptionFitLineLength,
StyleGraphicPropertiesElement.CaptionGap,
StyleGraphicPropertiesElement.CaptionLineLength,
StyleGraphicPropertiesElement.CaptionType,
StyleGraphicPropertiesElement.ColorInversion,
StyleGraphicPropertiesElement.ColorMode,
StyleGraphicPropertiesElement.Contrast,
StyleGraphicPropertiesElement.DecimalPlaces,
StyleGraphicPropertiesElement.DrawAspect,
StyleGraphicPropertiesElement.EndGuide,
StyleGraphicPropertiesElement.EndLineSpacingHorizontal,
StyleGraphicPropertiesElement.EndLineSpacingVertical,
StyleGraphicPropertiesElement.Fill,
StyleGraphicPropertiesElement.FillColor,
StyleGraphicPropertiesElement.FillGradientName,
StyleGraphicPropertiesElement.FillHatchName,
StyleGraphicPropertiesElement.FillHatchSolid,
StyleGraphicPropertiesElement.FillImageHeight,
StyleGraphicPropertiesElement.FillImageName,
StyleGraphicPropertiesElement.FillImageRefPoint,
StyleGraphicPropertiesElement.FillImageRefPointX,
StyleGraphicPropertiesElement.FillImageRefPointY,
StyleGraphicPropertiesElement.FillImageWidth,
StyleGraphicPropertiesElement.FitToContour,
StyleGraphicPropertiesElement.FitToSize,
StyleGraphicPropertiesElement.FrameDisplayBorder,
StyleGraphicPropertiesElement.FrameDisplayScrollbar,
StyleGraphicPropertiesElement.FrameMarginHorizontal,
StyleGraphicPropertiesElement.FrameMarginVertical,
StyleGraphicPropertiesElement.Gamma,
StyleGraphicPropertiesElement.GradientStepCount,
StyleGraphicPropertiesElement.Green,
StyleGraphicPropertiesElement.GuideDistance,
StyleGraphicPropertiesElement.GuideOverhang,
StyleGraphicPropertiesElement.ImageOpacity,
StyleGraphicPropertiesElement.LineDistance,
StyleGraphicPropertiesElement.Luminance,
StyleGraphicPropertiesElement.MarkerEnd,
StyleGraphicPropertiesElement.MarkerEndCenter,
StyleGraphicPropertiesElement.MarkerEndWidth,
StyleGraphicPropertiesElement.MarkerStart,
StyleGraphicPropertiesElement.MarkerStartCenter,
StyleGraphicPropertiesElement.MarkerStartWidth,
StyleGraphicPropertiesElement.MeasureAlign,
StyleGraphicPropertiesElement.MeasureVerticalAlign,
StyleGraphicPropertiesElement.OleDrawAspect,
StyleGraphicPropertiesElement.Opacity,
StyleGraphicPropertiesElement.OpacityName,
StyleGraphicPropertiesElement.Parallel,
StyleGraphicPropertiesElement.Placing,
StyleGraphicPropertiesElement.Red,
StyleGraphicPropertiesElement.SecondaryFillColor,
StyleGraphicPropertiesElement.DrawShadow,
StyleGraphicPropertiesElement.ShadowColor,
StyleGraphicPropertiesElement.ShadowOffsetX,
StyleGraphicPropertiesElement.ShadowOffsetY,
StyleGraphicPropertiesElement.ShadowOpacity,
StyleGraphicPropertiesElement.ShowUnit,
StyleGraphicPropertiesElement.StartGuide,
StyleGraphicPropertiesElement.StartLineSpacingHorizontal,
StyleGraphicPropertiesElement.StartLineSpacingVertical,
StyleGraphicPropertiesElement.Stroke,
StyleGraphicPropertiesElement.StrokeDash,
StyleGraphicPropertiesElement.StrokeDashNames,
StyleGraphicPropertiesElement.StrokeLinejoin,
StyleGraphicPropertiesElement.SymbolColor,
StyleGraphicPropertiesElement.TextareaHorizontalAlign,
StyleGraphicPropertiesElement.TextareaVerticalAlign,
StyleGraphicPropertiesElement.TileRepeatOffset,
StyleGraphicPropertiesElement.Unit,
StyleGraphicPropertiesElement.VisibleAreaHeight,
StyleGraphicPropertiesElement.VisibleAreaLeft,
StyleGraphicPropertiesElement.VisibleAreaTop,
StyleGraphicPropertiesElement.VisibleAreaWidth,
StyleGraphicPropertiesElement.WrapInfluenceOnPosition,
StyleGraphicPropertiesElement.BackgroundColor,
StyleGraphicPropertiesElement.Border,
StyleGraphicPropertiesElement.BorderBottom,
StyleGraphicPropertiesElement.BorderLeft,
StyleGraphicPropertiesElement.BorderRight,
StyleGraphicPropertiesElement.BorderTop,
StyleGraphicPropertiesElement.Clip,
StyleGraphicPropertiesElement.Margin,
StyleGraphicPropertiesElement.MarginBottom,
StyleGraphicPropertiesElement.MarginLeft,
StyleGraphicPropertiesElement.MarginRight,
StyleGraphicPropertiesElement.MarginTop,
StyleGraphicPropertiesElement.MaxHeight,
StyleGraphicPropertiesElement.MaxWidth,
StyleGraphicPropertiesElement.MinHeight,
StyleGraphicPropertiesElement.MinWidth,
StyleGraphicPropertiesElement.Padding,
StyleGraphicPropertiesElement.PaddingBottom,
StyleGraphicPropertiesElement.PaddingLeft,
StyleGraphicPropertiesElement.PaddingRight,
StyleGraphicPropertiesElement.PaddingTop,
StyleGraphicPropertiesElement.WrapOption,
StyleGraphicPropertiesElement.BackgroundTransparency,
StyleGraphicPropertiesElement.BorderLineWidth,
StyleGraphicPropertiesElement.BorderLineWidthBottom,
StyleGraphicPropertiesElement.BorderLineWidthLeft,
StyleGraphicPropertiesElement.BorderLineWidthRight,
StyleGraphicPropertiesElement.BorderLineWidthTop,
StyleGraphicPropertiesElement.Editable,
StyleGraphicPropertiesElement.FlowWithText,
StyleGraphicPropertiesElement.HorizontalPos,
StyleGraphicPropertiesElement.HorizontalRel,
StyleGraphicPropertiesElement.Mirror,
StyleGraphicPropertiesElement.NumberWrappedParagraphs,
StyleGraphicPropertiesElement.OverflowBehavior,
StyleGraphicPropertiesElement.PrintContent,
StyleGraphicPropertiesElement.Protect,
StyleGraphicPropertiesElement.RelHeight,
StyleGraphicPropertiesElement.RelWidth,
StyleGraphicPropertiesElement.Repeat,
StyleGraphicPropertiesElement.RunThrough,
StyleGraphicPropertiesElement.StyleShadow,
StyleGraphicPropertiesElement.ShrinkToFit,
StyleGraphicPropertiesElement.VerticalPos,
StyleGraphicPropertiesElement.VerticalRel,
StyleGraphicPropertiesElement.Wrap,
StyleGraphicPropertiesElement.WrapContour,
StyleGraphicPropertiesElement.WrapContourMode,
StyleGraphicPropertiesElement.WrapDynamicThreshold,
StyleGraphicPropertiesElement.WritingMode,
StyleGraphicPropertiesElement.FillRule,
StyleGraphicPropertiesElement.Height,
StyleGraphicPropertiesElement.StrokeColor,
StyleGraphicPropertiesElement.StrokeLinecap,
StyleGraphicPropertiesElement.StrokeOpacity,
StyleGraphicPropertiesElement.StrokeWidth,
StyleGraphicPropertiesElement.Width,
StyleGraphicPropertiesElement.X,
StyleGraphicPropertiesElement.Y,
StyleGraphicPropertiesElement.AnchorPageNumber,
StyleGraphicPropertiesElement.AnchorType,
StyleGraphicPropertiesElement.Animation,
StyleGraphicPropertiesElement.AnimationDelay,
StyleGraphicPropertiesElement.AnimationDirection,
StyleGraphicPropertiesElement.AnimationRepeat,
StyleGraphicPropertiesElement.AnimationStartInside,
StyleGraphicPropertiesElement.AnimationSteps,
StyleGraphicPropertiesElement.AnimationStopInside,
StyleHeaderFooterPropertiesElement.BackgroundColor,
StyleHeaderFooterPropertiesElement.Border,
StyleHeaderFooterPropertiesElement.BorderBottom,
StyleHeaderFooterPropertiesElement.BorderLeft,
StyleHeaderFooterPropertiesElement.BorderRight,
StyleHeaderFooterPropertiesElement.BorderTop,
StyleHeaderFooterPropertiesElement.Margin,
StyleHeaderFooterPropertiesElement.MarginBottom,
StyleHeaderFooterPropertiesElement.MarginLeft,
StyleHeaderFooterPropertiesElement.MarginRight,
StyleHeaderFooterPropertiesElement.MarginTop,
StyleHeaderFooterPropertiesElement.MinHeight,
StyleHeaderFooterPropertiesElement.Padding,
StyleHeaderFooterPropertiesElement.PaddingBottom,
StyleHeaderFooterPropertiesElement.PaddingLeft,
StyleHeaderFooterPropertiesElement.PaddingRight,
StyleHeaderFooterPropertiesElement.PaddingTop,
StyleHeaderFooterPropertiesElement.BorderLineWidth,
StyleHeaderFooterPropertiesElement.BorderLineWidthBottom,
StyleHeaderFooterPropertiesElement.BorderLineWidthLeft,
StyleHeaderFooterPropertiesElement.BorderLineWidthRight,
StyleHeaderFooterPropertiesElement.BorderLineWidthTop,
StyleHeaderFooterPropertiesElement.DynamicSpacing,
StyleHeaderFooterPropertiesElement.Shadow,
StyleHeaderFooterPropertiesElement.Height,
StyleListLevelPropertiesElement.Height,
StyleListLevelPropertiesElement.TextAlign,
StyleListLevelPropertiesElement.Width,
StyleListLevelPropertiesElement.FontName,
StyleListLevelPropertiesElement.VerticalPos,
StyleListLevelPropertiesElement.VerticalRel,
StyleListLevelPropertiesElement.Y,
StyleListLevelPropertiesElement.ListLevelPositionAndSpaceMode,
StyleListLevelPropertiesElement.MinLabelDistance,
StyleListLevelPropertiesElement.MinLabelWidth,
StyleListLevelPropertiesElement.SpaceBefore,
StylePageLayoutPropertiesElement.BackgroundColor,
StylePageLayoutPropertiesElement.Border,
StylePageLayoutPropertiesElement.BorderBottom,
StylePageLayoutPropertiesElement.BorderLeft,
StylePageLayoutPropertiesElement.BorderRight,
StylePageLayoutPropertiesElement.BorderTop,
StylePageLayoutPropertiesElement.Margin,
StylePageLayoutPropertiesElement.MarginBottom,
StylePageLayoutPropertiesElement.MarginLeft,
StylePageLayoutPropertiesElement.MarginRight,
StylePageLayoutPropertiesElement.MarginTop,
StylePageLayoutPropertiesElement.Padding,
StylePageLayoutPropertiesElement.PaddingBottom,
StylePageLayoutPropertiesElement.PaddingLeft,
StylePageLayoutPropertiesElement.PaddingRight,
StylePageLayoutPropertiesElement.PaddingTop,
StylePageLayoutPropertiesElement.PageHeight,
StylePageLayoutPropertiesElement.PageWidth,
StylePageLayoutPropertiesElement.BorderLineWidth,
StylePageLayoutPropertiesElement.BorderLineWidthBottom,
StylePageLayoutPropertiesElement.BorderLineWidthLeft,
StylePageLayoutPropertiesElement.BorderLineWidthRight,
StylePageLayoutPropertiesElement.BorderLineWidthTop,
StylePageLayoutPropertiesElement.FirstPageNumber,
StylePageLayoutPropertiesElement.FootnoteMaxHeight,
StylePageLayoutPropertiesElement.LayoutGridBaseHeight,
StylePageLayoutPropertiesElement.LayoutGridBaseWidth,
StylePageLayoutPropertiesElement.LayoutGridColor,
StylePageLayoutPropertiesElement.LayoutGridDisplay,
StylePageLayoutPropertiesElement.LayoutGridLines,
StylePageLayoutPropertiesElement.LayoutGridMode,
StylePageLayoutPropertiesElement.LayoutGridPrint,
StylePageLayoutPropertiesElement.LayoutGridRubyBelow,
StylePageLayoutPropertiesElement.LayoutGridRubyHeight,
StylePageLayoutPropertiesElement.LayoutGridSnapTo,
StylePageLayoutPropertiesElement.LayoutGridStandardMode,
StylePageLayoutPropertiesElement.NumFormat,
StylePageLayoutPropertiesElement.NumFormat,
StylePageLayoutPropertiesElement.NumLetterSync,
StylePageLayoutPropertiesElement.NumPrefix,
StylePageLayoutPropertiesElement.NumSuffix,
StylePageLayoutPropertiesElement.PaperTrayName,
StylePageLayoutPropertiesElement.Print,
StylePageLayoutPropertiesElement.PrintOrientation,
StylePageLayoutPropertiesElement.PrintPageOrder,
StylePageLayoutPropertiesElement.RegisterTruthRefStyleName,
StylePageLayoutPropertiesElement.ScaleTo,
StylePageLayoutPropertiesElement.ScaleToPages,
StylePageLayoutPropertiesElement.Shadow,
StylePageLayoutPropertiesElement.TableCentering,
StylePageLayoutPropertiesElement.WritingMode,
StyleParagraphPropertiesElement.BackgroundColor,
StyleParagraphPropertiesElement.Border,
StyleParagraphPropertiesElement.BorderBottom,
StyleParagraphPropertiesElement.BorderLeft,
StyleParagraphPropertiesElement.BorderRight,
StyleParagraphPropertiesElement.BorderTop,
StyleParagraphPropertiesElement.BreakAfter,
StyleParagraphPropertiesElement.BreakBefore,
StyleParagraphPropertiesElement.HyphenationKeep,
StyleParagraphPropertiesElement.HyphenationLadderCount,
StyleParagraphPropertiesElement.KeepTogether,
StyleParagraphPropertiesElement.KeepWithNext,
StyleParagraphPropertiesElement.LineHeight,
StyleParagraphPropertiesElement.Margin,
StyleParagraphPropertiesElement.MarginBottom,
StyleParagraphPropertiesElement.MarginLeft,
StyleParagraphPropertiesElement.MarginRight,
StyleParagraphPropertiesElement.MarginTop,
StyleParagraphPropertiesElement.Orphans,
StyleParagraphPropertiesElement.Padding,
StyleParagraphPropertiesElement.PaddingBottom,
StyleParagraphPropertiesElement.PaddingLeft,
StyleParagraphPropertiesElement.PaddingRight,
StyleParagraphPropertiesElement.PaddingTop,
StyleParagraphPropertiesElement.TextAlign,
StyleParagraphPropertiesElement.TextAlignLast,
StyleParagraphPropertiesElement.TextIndent,
StyleParagraphPropertiesElement.Widows,
StyleParagraphPropertiesElement.AutoTextIndent,
StyleParagraphPropertiesElement.BackgroundTransparency,
StyleParagraphPropertiesElement.BorderLineWidth,
StyleParagraphPropertiesElement.BorderLineWidthBottom,
StyleParagraphPropertiesElement.BorderLineWidthLeft,
StyleParagraphPropertiesElement.BorderLineWidthRight,
StyleParagraphPropertiesElement.BorderLineWidthTop,
StyleParagraphPropertiesElement.FontIndependentLineSpacing,
StyleParagraphPropertiesElement.JoinBorder,
StyleParagraphPropertiesElement.JustifySingleWord,
StyleParagraphPropertiesElement.LineBreak,
StyleParagraphPropertiesElement.LineHeightAtLeast,
StyleParagraphPropertiesElement.LineSpacing,
StyleParagraphPropertiesElement.PageNumber,
StyleParagraphPropertiesElement.PunctuationWrap,
StyleParagraphPropertiesElement.RegisterTrue,
StyleParagraphPropertiesElement.Shadow,
StyleParagraphPropertiesElement.SnapToLayoutGrid,
StyleParagraphPropertiesElement.TabStopDistance,
StyleParagraphPropertiesElement.TextAutospace,
StyleParagraphPropertiesElement.VerticalAlign,
StyleParagraphPropertiesElement.WritingMode,
StyleParagraphPropertiesElement.WritingModeAutomatic,
StyleParagraphPropertiesElement.LineNumber,
StyleParagraphPropertiesElement.NumberLines,
StyleRubyPropertiesElement.RubyAlign,
StyleRubyPropertiesElement.RubyPosition,
StyleSectionPropertiesElement.BackgroundColor,
StyleSectionPropertiesElement.MarginLeft,
StyleSectionPropertiesElement.MarginRight,
StyleSectionPropertiesElement.Editable,
StyleSectionPropertiesElement.Protect,
StyleSectionPropertiesElement.WritingMode,
StyleSectionPropertiesElement.DontBalanceTextColumns,
StyleTableCellPropertiesElement.BackgroundColor,
StyleTableCellPropertiesElement.Border,
StyleTableCellPropertiesElement.BorderBottom,
StyleTableCellPropertiesElement.BorderLeft,
StyleTableCellPropertiesElement.BorderRight,
StyleTableCellPropertiesElement.BorderTop,
StyleTableCellPropertiesElement.Padding,
StyleTableCellPropertiesElement.PaddingBottom,
StyleTableCellPropertiesElement.PaddingLeft,
StyleTableCellPropertiesElement.PaddingRight,
StyleTableCellPropertiesElement.PaddingTop,
StyleTableCellPropertiesElement.WrapOption,
StyleTableCellPropertiesElement.BorderLineWidth,
StyleTableCellPropertiesElement.BorderLineWidthBottom,
StyleTableCellPropertiesElement.BorderLineWidthLeft,
StyleTableCellPropertiesElement.BorderLineWidthRight,
StyleTableCellPropertiesElement.BorderLineWidthTop,
StyleTableCellPropertiesElement.CellProtect,
StyleTableCellPropertiesElement.DecimalPlaces,
StyleTableCellPropertiesElement.DiagonalBlTr,
StyleTableCellPropertiesElement.DiagonalBlTrWidths,
StyleTableCellPropertiesElement.DiagonalTlBr,
StyleTableCellPropertiesElement.DiagonalTlBrWidths,
StyleTableCellPropertiesElement.Direction,
StyleTableCellPropertiesElement.GlyphOrientationVertical,
StyleTableCellPropertiesElement.PrintContent,
StyleTableCellPropertiesElement.RepeatContent,
StyleTableCellPropertiesElement.RotationAlign,
StyleTableCellPropertiesElement.RotationAngle,
StyleTableCellPropertiesElement.Shadow,
StyleTableCellPropertiesElement.ShrinkToFit,
StyleTableCellPropertiesElement.TextAlignSource,
StyleTableCellPropertiesElement.VerticalAlign,
StyleTableCellPropertiesElement.WritingMode,
StyleTableColumnPropertiesElement.BreakAfter,
StyleTableColumnPropertiesElement.BreakBefore,
StyleTableColumnPropertiesElement.ColumnWidth,
StyleTableColumnPropertiesElement.RelColumnWidth,
StyleTableColumnPropertiesElement.UseOptimalColumnWidth,
StyleTablePropertiesElement.BackgroundColor,
StyleTablePropertiesElement.BreakAfter,
StyleTablePropertiesElement.BreakBefore,
StyleTablePropertiesElement.KeepWithNext,
StyleTablePropertiesElement.Margin,
StyleTablePropertiesElement.MarginBottom,
StyleTablePropertiesElement.MarginLeft,
StyleTablePropertiesElement.MarginRight,
StyleTablePropertiesElement.MarginTop,
StyleTablePropertiesElement.MayBreakBetweenRows,
StyleTablePropertiesElement.PageNumber,
StyleTablePropertiesElement.RelWidth,
StyleTablePropertiesElement.Shadow,
StyleTablePropertiesElement.Width,
StyleTablePropertiesElement.WritingMode,
StyleTablePropertiesElement.Align,
StyleTablePropertiesElement.BorderModel,
StyleTablePropertiesElement.Display,
StyleTableRowPropertiesElement.BackgroundColor,
StyleTableRowPropertiesElement.BreakAfter,
StyleTableRowPropertiesElement.BreakBefore,
StyleTableRowPropertiesElement.KeepTogether,
StyleTableRowPropertiesElement.MinRowHeight,
StyleTableRowPropertiesElement.RowHeight,
StyleTableRowPropertiesElement.UseOptimalRowHeight,
StyleTextPropertiesElement.BackgroundColor,
StyleTextPropertiesElement.Color,
StyleTextPropertiesElement.Country,
StyleTextPropertiesElement.FontFamily,
StyleTextPropertiesElement.FontSize,
StyleTextPropertiesElement.FontStyle,
StyleTextPropertiesElement.FontVariant,
StyleTextPropertiesElement.FontWeight,
StyleTextPropertiesElement.Hyphenate,
StyleTextPropertiesElement.HyphenationPushCharCount,
StyleTextPropertiesElement.HyphenationRemainCharCount,
StyleTextPropertiesElement.Language,
StyleTextPropertiesElement.LetterSpacing,
StyleTextPropertiesElement.Script,
StyleTextPropertiesElement.TextShadow,
StyleTextPropertiesElement.TextTransform,
StyleTextPropertiesElement.CountryAsian,
StyleTextPropertiesElement.CountryComplex,
StyleTextPropertiesElement.FontCharset,
StyleTextPropertiesElement.FontCharsetAsian,
StyleTextPropertiesElement.FontCharsetComplex,
StyleTextPropertiesElement.FontFamilyAsian,
StyleTextPropertiesElement.FontFamilyComplex,
StyleTextPropertiesElement.FontFamilyGeneric,
StyleTextPropertiesElement.FontFamilyGenericAsian,
StyleTextPropertiesElement.FontFamilyGenericComplex,
StyleTextPropertiesElement.FontName,
StyleTextPropertiesElement.FontNameAsian,
StyleTextPropertiesElement.FontNameComplex,
StyleTextPropertiesElement.FontPitch,
StyleTextPropertiesElement.FontPitchAsian,
StyleTextPropertiesElement.FontPitchComplex,
StyleTextPropertiesElement.FontRelief,
StyleTextPropertiesElement.FontSizeAsian,
StyleTextPropertiesElement.FontSizeComplex,
StyleTextPropertiesElement.FontSizeRel,
StyleTextPropertiesElement.FontSizeRelAsian,
StyleTextPropertiesElement.FontSizeRelComplex,
StyleTextPropertiesElement.FontStyleAsian,
StyleTextPropertiesElement.FontStyleComplex,
StyleTextPropertiesElement.FontStyleName,
StyleTextPropertiesElement.FontStyleNameAsian,
StyleTextPropertiesElement.FontStyleNameComplex,
StyleTextPropertiesElement.FontWeightAsian,
StyleTextPropertiesElement.FontWeightComplex,
StyleTextPropertiesElement.LanguageAsian,
StyleTextPropertiesElement.LanguageComplex,
StyleTextPropertiesElement.LetterKerning,
StyleTextPropertiesElement.RfcLanguageTag,
StyleTextPropertiesElement.RfcLanguageTagAsian,
StyleTextPropertiesElement.RfcLanguageTagComplex,
StyleTextPropertiesElement.ScriptAsian,
StyleTextPropertiesElement.ScriptComplex,
StyleTextPropertiesElement.ScriptType,
StyleTextPropertiesElement.TextBlinking,
StyleTextPropertiesElement.TextCombine,
StyleTextPropertiesElement.TextCombineEndChar,
StyleTextPropertiesElement.TextCombineStartChar,
StyleTextPropertiesElement.TextEmphasize,
StyleTextPropertiesElement.TextLineThroughColor,
StyleTextPropertiesElement.TextLineThroughMode,
StyleTextPropertiesElement.TextLineThroughStyle,
StyleTextPropertiesElement.TextLineThroughText,
StyleTextPropertiesElement.TextLineThroughTextStyle,
StyleTextPropertiesElement.TextLineThroughType,
StyleTextPropertiesElement.TextLineThroughWidth,
StyleTextPropertiesElement.TextOutline,
StyleTextPropertiesElement.TextOverlineColor,
StyleTextPropertiesElement.TextOverlineMode,
StyleTextPropertiesElement.TextOverlineStyle,
StyleTextPropertiesElement.TextOverlineType,
StyleTextPropertiesElement.TextOverlineWidth,
StyleTextPropertiesElement.TextPosition,
StyleTextPropertiesElement.TextRotationAngle,
StyleTextPropertiesElement.TextRotationScale,
StyleTextPropertiesElement.TextScale,
StyleTextPropertiesElement.TextUnderlineColor,
StyleTextPropertiesElement.TextUnderlineMode,
StyleTextPropertiesElement.TextUnderlineStyle,
StyleTextPropertiesElement.TextUnderlineType,
StyleTextPropertiesElement.TextUnderlineWidth,
StyleTextPropertiesElement.UseWindowFontColor,
StyleTextPropertiesElement.Condition,
StyleTextPropertiesElement.Display,
StyleTextPropertiesElement.Display,
StyleTextPropertiesElement.Display,
});
public final static OdfStyleFamily Section = new OdfStyleFamily("section",
new OdfStyleProperty[]{
StyleChartPropertiesElement.AngleOffset,
StyleChartPropertiesElement.AutoPosition,
StyleChartPropertiesElement.AutoSize,
StyleChartPropertiesElement.AxisLabelPosition,
StyleChartPropertiesElement.AxisPosition,
StyleChartPropertiesElement.ConnectBars,
StyleChartPropertiesElement.DataLabelNumber,
StyleChartPropertiesElement.DataLabelSymbol,
StyleChartPropertiesElement.DataLabelText,
StyleChartPropertiesElement.Deep,
StyleChartPropertiesElement.DisplayLabel,
StyleChartPropertiesElement.ErrorCategory,
StyleChartPropertiesElement.ErrorLowerIndicator,
StyleChartPropertiesElement.ErrorLowerLimit,
StyleChartPropertiesElement.ErrorLowerRange,
StyleChartPropertiesElement.ErrorMargin,
StyleChartPropertiesElement.ErrorPercentage,
StyleChartPropertiesElement.ErrorUpperIndicator,
StyleChartPropertiesElement.ErrorUpperLimit,
StyleChartPropertiesElement.ErrorUpperRange,
StyleChartPropertiesElement.GapWidth,
StyleChartPropertiesElement.GroupBarsPerAxis,
StyleChartPropertiesElement.HoleSize,
StyleChartPropertiesElement.IncludeHiddenCells,
StyleChartPropertiesElement.Interpolation,
StyleChartPropertiesElement.IntervalMajor,
StyleChartPropertiesElement.IntervalMinorDivisor,
StyleChartPropertiesElement.JapaneseCandleStick,
StyleChartPropertiesElement.LabelArrangement,
StyleChartPropertiesElement.LabelPosition,
StyleChartPropertiesElement.LabelPositionNegative,
StyleChartPropertiesElement.Lines,
StyleChartPropertiesElement.LinkDataStyleToSource,
StyleChartPropertiesElement.Logarithmic,
StyleChartPropertiesElement.Maximum,
StyleChartPropertiesElement.MeanValue,
StyleChartPropertiesElement.Minimum,
StyleChartPropertiesElement.Origin,
StyleChartPropertiesElement.Overlap,
StyleChartPropertiesElement.Percentage,
StyleChartPropertiesElement.PieOffset,
StyleChartPropertiesElement.RegressionType,
StyleChartPropertiesElement.ReverseDirection,
StyleChartPropertiesElement.RightAngledAxes,
StyleChartPropertiesElement.ScaleText,
StyleChartPropertiesElement.SeriesSource,
StyleChartPropertiesElement.SolidType,
StyleChartPropertiesElement.SortByXValues,
StyleChartPropertiesElement.SplineOrder,
StyleChartPropertiesElement.SplineResolution,
StyleChartPropertiesElement.Stacked,
StyleChartPropertiesElement.SymbolHeight,
StyleChartPropertiesElement.SymbolName,
StyleChartPropertiesElement.SymbolType,
StyleChartPropertiesElement.SymbolType,
StyleChartPropertiesElement.SymbolType,
StyleChartPropertiesElement.SymbolType,
StyleChartPropertiesElement.SymbolWidth,
StyleChartPropertiesElement.TextOverlap,
StyleChartPropertiesElement.ThreeDimensional,
StyleChartPropertiesElement.TickMarkPosition,
StyleChartPropertiesElement.TickMarksMajorInner,
StyleChartPropertiesElement.TickMarksMajorOuter,
StyleChartPropertiesElement.TickMarksMinorInner,
StyleChartPropertiesElement.TickMarksMinorOuter,
StyleChartPropertiesElement.TreatEmptyCells,
StyleChartPropertiesElement.Vertical,
StyleChartPropertiesElement.Visible,
StyleChartPropertiesElement.Direction,
StyleChartPropertiesElement.RotationAngle,
StyleChartPropertiesElement.LineBreak,
StyleDrawingPagePropertiesElement.BackgroundSize,
StyleDrawingPagePropertiesElement.Fill,
StyleDrawingPagePropertiesElement.FillColor,
StyleDrawingPagePropertiesElement.FillGradientName,
StyleDrawingPagePropertiesElement.FillHatchName,
StyleDrawingPagePropertiesElement.FillHatchSolid,
StyleDrawingPagePropertiesElement.FillImageHeight,
StyleDrawingPagePropertiesElement.FillImageName,
StyleDrawingPagePropertiesElement.FillImageRefPoint,
StyleDrawingPagePropertiesElement.FillImageRefPointX,
StyleDrawingPagePropertiesElement.FillImageRefPointY,
StyleDrawingPagePropertiesElement.FillImageWidth,
StyleDrawingPagePropertiesElement.GradientStepCount,
StyleDrawingPagePropertiesElement.Opacity,
StyleDrawingPagePropertiesElement.OpacityName,
StyleDrawingPagePropertiesElement.SecondaryFillColor,
StyleDrawingPagePropertiesElement.TileRepeatOffset,
StyleDrawingPagePropertiesElement.BackgroundObjectsVisible,
StyleDrawingPagePropertiesElement.BackgroundVisible,
StyleDrawingPagePropertiesElement.DisplayDateTime,
StyleDrawingPagePropertiesElement.DisplayFooter,
StyleDrawingPagePropertiesElement.DisplayHeader,
StyleDrawingPagePropertiesElement.DisplayPageNumber,
StyleDrawingPagePropertiesElement.Duration,
StyleDrawingPagePropertiesElement.TransitionSpeed,
StyleDrawingPagePropertiesElement.TransitionStyle,
StyleDrawingPagePropertiesElement.TransitionType,
StyleDrawingPagePropertiesElement.Visibility,
StyleDrawingPagePropertiesElement.Direction,
StyleDrawingPagePropertiesElement.FadeColor,
StyleDrawingPagePropertiesElement.Subtype,
StyleDrawingPagePropertiesElement.Type,
StyleDrawingPagePropertiesElement.Repeat,
StyleDrawingPagePropertiesElement.FillRule,
StyleGraphicPropertiesElement.AmbientColor,
StyleGraphicPropertiesElement.BackScale,
StyleGraphicPropertiesElement.BackfaceCulling,
StyleGraphicPropertiesElement.CloseBack,
StyleGraphicPropertiesElement.CloseFront,
StyleGraphicPropertiesElement.Depth,
StyleGraphicPropertiesElement.DiffuseColor,
StyleGraphicPropertiesElement.EdgeRounding,
StyleGraphicPropertiesElement.EdgeRoundingMode,
StyleGraphicPropertiesElement.EmissiveColor,
StyleGraphicPropertiesElement.EndAngle,
StyleGraphicPropertiesElement.HorizontalSegments,
StyleGraphicPropertiesElement.LightingMode,
StyleGraphicPropertiesElement.NormalsDirection,
StyleGraphicPropertiesElement.NormalsKind,
StyleGraphicPropertiesElement.Dr3dShadow,
StyleGraphicPropertiesElement.Shininess,
StyleGraphicPropertiesElement.SpecularColor,
StyleGraphicPropertiesElement.TextureFilter,
StyleGraphicPropertiesElement.TextureGenerationModeX,
StyleGraphicPropertiesElement.TextureGenerationModeY,
StyleGraphicPropertiesElement.TextureKind,
StyleGraphicPropertiesElement.TextureMode,
StyleGraphicPropertiesElement.VerticalSegments,
StyleGraphicPropertiesElement.AutoGrowHeight,
StyleGraphicPropertiesElement.AutoGrowWidth,
StyleGraphicPropertiesElement.Blue,
StyleGraphicPropertiesElement.CaptionAngle,
StyleGraphicPropertiesElement.CaptionAngleType,
StyleGraphicPropertiesElement.CaptionEscape,
StyleGraphicPropertiesElement.CaptionEscapeDirection,
StyleGraphicPropertiesElement.CaptionFitLineLength,
StyleGraphicPropertiesElement.CaptionGap,
StyleGraphicPropertiesElement.CaptionLineLength,
StyleGraphicPropertiesElement.CaptionType,
StyleGraphicPropertiesElement.ColorInversion,
StyleGraphicPropertiesElement.ColorMode,
StyleGraphicPropertiesElement.Contrast,
StyleGraphicPropertiesElement.DecimalPlaces,
StyleGraphicPropertiesElement.DrawAspect,
StyleGraphicPropertiesElement.EndGuide,
StyleGraphicPropertiesElement.EndLineSpacingHorizontal,
StyleGraphicPropertiesElement.EndLineSpacingVertical,
StyleGraphicPropertiesElement.Fill,
StyleGraphicPropertiesElement.FillColor,
StyleGraphicPropertiesElement.FillGradientName,
StyleGraphicPropertiesElement.FillHatchName,
StyleGraphicPropertiesElement.FillHatchSolid,
StyleGraphicPropertiesElement.FillImageHeight,
StyleGraphicPropertiesElement.FillImageName,
StyleGraphicPropertiesElement.FillImageRefPoint,
StyleGraphicPropertiesElement.FillImageRefPointX,
StyleGraphicPropertiesElement.FillImageRefPointY,
StyleGraphicPropertiesElement.FillImageWidth,
StyleGraphicPropertiesElement.FitToContour,
StyleGraphicPropertiesElement.FitToSize,
StyleGraphicPropertiesElement.FrameDisplayBorder,
StyleGraphicPropertiesElement.FrameDisplayScrollbar,
StyleGraphicPropertiesElement.FrameMarginHorizontal,
StyleGraphicPropertiesElement.FrameMarginVertical,
StyleGraphicPropertiesElement.Gamma,
StyleGraphicPropertiesElement.GradientStepCount,
StyleGraphicPropertiesElement.Green,
StyleGraphicPropertiesElement.GuideDistance,
StyleGraphicPropertiesElement.GuideOverhang,
StyleGraphicPropertiesElement.ImageOpacity,
StyleGraphicPropertiesElement.LineDistance,
StyleGraphicPropertiesElement.Luminance,
StyleGraphicPropertiesElement.MarkerEnd,
StyleGraphicPropertiesElement.MarkerEndCenter,
StyleGraphicPropertiesElement.MarkerEndWidth,
StyleGraphicPropertiesElement.MarkerStart,
StyleGraphicPropertiesElement.MarkerStartCenter,
StyleGraphicPropertiesElement.MarkerStartWidth,
StyleGraphicPropertiesElement.MeasureAlign,
StyleGraphicPropertiesElement.MeasureVerticalAlign,
StyleGraphicPropertiesElement.OleDrawAspect,
StyleGraphicPropertiesElement.Opacity,
StyleGraphicPropertiesElement.OpacityName,
StyleGraphicPropertiesElement.Parallel,
StyleGraphicPropertiesElement.Placing,
StyleGraphicPropertiesElement.Red,
StyleGraphicPropertiesElement.SecondaryFillColor,
StyleGraphicPropertiesElement.DrawShadow,
StyleGraphicPropertiesElement.ShadowColor,
StyleGraphicPropertiesElement.ShadowOffsetX,
StyleGraphicPropertiesElement.ShadowOffsetY,
StyleGraphicPropertiesElement.ShadowOpacity,
StyleGraphicPropertiesElement.ShowUnit,
StyleGraphicPropertiesElement.StartGuide,
StyleGraphicPropertiesElement.StartLineSpacingHorizontal,
StyleGraphicPropertiesElement.StartLineSpacingVertical,
StyleGraphicPropertiesElement.Stroke,
StyleGraphicPropertiesElement.StrokeDash,
StyleGraphicPropertiesElement.StrokeDashNames,
StyleGraphicPropertiesElement.StrokeLinejoin,
StyleGraphicPropertiesElement.SymbolColor,
StyleGraphicPropertiesElement.TextareaHorizontalAlign,
StyleGraphicPropertiesElement.TextareaVerticalAlign,
StyleGraphicPropertiesElement.TileRepeatOffset,
StyleGraphicPropertiesElement.Unit,
StyleGraphicPropertiesElement.VisibleAreaHeight,
StyleGraphicPropertiesElement.VisibleAreaLeft,
StyleGraphicPropertiesElement.VisibleAreaTop,
StyleGraphicPropertiesElement.VisibleAreaWidth,
StyleGraphicPropertiesElement.WrapInfluenceOnPosition,
StyleGraphicPropertiesElement.BackgroundColor,
StyleGraphicPropertiesElement.Border,
StyleGraphicPropertiesElement.BorderBottom,
StyleGraphicPropertiesElement.BorderLeft,
StyleGraphicPropertiesElement.BorderRight,
StyleGraphicPropertiesElement.BorderTop,
StyleGraphicPropertiesElement.Clip,
StyleGraphicPropertiesElement.Margin,
StyleGraphicPropertiesElement.MarginBottom,
StyleGraphicPropertiesElement.MarginLeft,
StyleGraphicPropertiesElement.MarginRight,
StyleGraphicPropertiesElement.MarginTop,
StyleGraphicPropertiesElement.MaxHeight,
StyleGraphicPropertiesElement.MaxWidth,
StyleGraphicPropertiesElement.MinHeight,
StyleGraphicPropertiesElement.MinWidth,
StyleGraphicPropertiesElement.Padding,
StyleGraphicPropertiesElement.PaddingBottom,
StyleGraphicPropertiesElement.PaddingLeft,
StyleGraphicPropertiesElement.PaddingRight,
StyleGraphicPropertiesElement.PaddingTop,
StyleGraphicPropertiesElement.WrapOption,
StyleGraphicPropertiesElement.BackgroundTransparency,
StyleGraphicPropertiesElement.BorderLineWidth,
StyleGraphicPropertiesElement.BorderLineWidthBottom,
StyleGraphicPropertiesElement.BorderLineWidthLeft,
StyleGraphicPropertiesElement.BorderLineWidthRight,
StyleGraphicPropertiesElement.BorderLineWidthTop,
StyleGraphicPropertiesElement.Editable,
StyleGraphicPropertiesElement.FlowWithText,
StyleGraphicPropertiesElement.HorizontalPos,
StyleGraphicPropertiesElement.HorizontalRel,
StyleGraphicPropertiesElement.Mirror,
StyleGraphicPropertiesElement.NumberWrappedParagraphs,
StyleGraphicPropertiesElement.OverflowBehavior,
StyleGraphicPropertiesElement.PrintContent,
StyleGraphicPropertiesElement.Protect,
StyleGraphicPropertiesElement.RelHeight,
StyleGraphicPropertiesElement.RelWidth,
StyleGraphicPropertiesElement.Repeat,
StyleGraphicPropertiesElement.RunThrough,
StyleGraphicPropertiesElement.StyleShadow,
StyleGraphicPropertiesElement.ShrinkToFit,
StyleGraphicPropertiesElement.VerticalPos,
StyleGraphicPropertiesElement.VerticalRel,
StyleGraphicPropertiesElement.Wrap,
StyleGraphicPropertiesElement.WrapContour,
StyleGraphicPropertiesElement.WrapContourMode,
StyleGraphicPropertiesElement.WrapDynamicThreshold,
StyleGraphicPropertiesElement.WritingMode,
StyleGraphicPropertiesElement.FillRule,
StyleGraphicPropertiesElement.Height,
StyleGraphicPropertiesElement.StrokeColor,
StyleGraphicPropertiesElement.StrokeLinecap,
StyleGraphicPropertiesElement.StrokeOpacity,
StyleGraphicPropertiesElement.StrokeWidth,
StyleGraphicPropertiesElement.Width,
StyleGraphicPropertiesElement.X,
StyleGraphicPropertiesElement.Y,
StyleGraphicPropertiesElement.AnchorPageNumber,
StyleGraphicPropertiesElement.AnchorType,
StyleGraphicPropertiesElement.Animation,
StyleGraphicPropertiesElement.AnimationDelay,
StyleGraphicPropertiesElement.AnimationDirection,
StyleGraphicPropertiesElement.AnimationRepeat,
StyleGraphicPropertiesElement.AnimationStartInside,
StyleGraphicPropertiesElement.AnimationSteps,
StyleGraphicPropertiesElement.AnimationStopInside,
StyleHeaderFooterPropertiesElement.BackgroundColor,
StyleHeaderFooterPropertiesElement.Border,
StyleHeaderFooterPropertiesElement.BorderBottom,
StyleHeaderFooterPropertiesElement.BorderLeft,
StyleHeaderFooterPropertiesElement.BorderRight,
StyleHeaderFooterPropertiesElement.BorderTop,
StyleHeaderFooterPropertiesElement.Margin,
StyleHeaderFooterPropertiesElement.MarginBottom,
StyleHeaderFooterPropertiesElement.MarginLeft,
StyleHeaderFooterPropertiesElement.MarginRight,
StyleHeaderFooterPropertiesElement.MarginTop,
StyleHeaderFooterPropertiesElement.MinHeight,
StyleHeaderFooterPropertiesElement.Padding,
StyleHeaderFooterPropertiesElement.PaddingBottom,
StyleHeaderFooterPropertiesElement.PaddingLeft,
StyleHeaderFooterPropertiesElement.PaddingRight,
StyleHeaderFooterPropertiesElement.PaddingTop,
StyleHeaderFooterPropertiesElement.BorderLineWidth,
StyleHeaderFooterPropertiesElement.BorderLineWidthBottom,
StyleHeaderFooterPropertiesElement.BorderLineWidthLeft,
StyleHeaderFooterPropertiesElement.BorderLineWidthRight,
StyleHeaderFooterPropertiesElement.BorderLineWidthTop,
StyleHeaderFooterPropertiesElement.DynamicSpacing,
StyleHeaderFooterPropertiesElement.Shadow,
StyleHeaderFooterPropertiesElement.Height,
StyleListLevelPropertiesElement.Height,
StyleListLevelPropertiesElement.TextAlign,
StyleListLevelPropertiesElement.Width,
StyleListLevelPropertiesElement.FontName,
StyleListLevelPropertiesElement.VerticalPos,
StyleListLevelPropertiesElement.VerticalRel,
StyleListLevelPropertiesElement.Y,
StyleListLevelPropertiesElement.ListLevelPositionAndSpaceMode,
StyleListLevelPropertiesElement.MinLabelDistance,
StyleListLevelPropertiesElement.MinLabelWidth,
StyleListLevelPropertiesElement.SpaceBefore,
StylePageLayoutPropertiesElement.BackgroundColor,
StylePageLayoutPropertiesElement.Border,
StylePageLayoutPropertiesElement.BorderBottom,
StylePageLayoutPropertiesElement.BorderLeft,
StylePageLayoutPropertiesElement.BorderRight,
StylePageLayoutPropertiesElement.BorderTop,
StylePageLayoutPropertiesElement.Margin,
StylePageLayoutPropertiesElement.MarginBottom,
StylePageLayoutPropertiesElement.MarginLeft,
StylePageLayoutPropertiesElement.MarginRight,
StylePageLayoutPropertiesElement.MarginTop,
StylePageLayoutPropertiesElement.Padding,
StylePageLayoutPropertiesElement.PaddingBottom,
StylePageLayoutPropertiesElement.PaddingLeft,
StylePageLayoutPropertiesElement.PaddingRight,
StylePageLayoutPropertiesElement.PaddingTop,
StylePageLayoutPropertiesElement.PageHeight,
StylePageLayoutPropertiesElement.PageWidth,
StylePageLayoutPropertiesElement.BorderLineWidth,
StylePageLayoutPropertiesElement.BorderLineWidthBottom,
StylePageLayoutPropertiesElement.BorderLineWidthLeft,
StylePageLayoutPropertiesElement.BorderLineWidthRight,
StylePageLayoutPropertiesElement.BorderLineWidthTop,
StylePageLayoutPropertiesElement.FirstPageNumber,
StylePageLayoutPropertiesElement.FootnoteMaxHeight,
StylePageLayoutPropertiesElement.LayoutGridBaseHeight,
StylePageLayoutPropertiesElement.LayoutGridBaseWidth,
StylePageLayoutPropertiesElement.LayoutGridColor,
StylePageLayoutPropertiesElement.LayoutGridDisplay,
StylePageLayoutPropertiesElement.LayoutGridLines,
StylePageLayoutPropertiesElement.LayoutGridMode,
StylePageLayoutPropertiesElement.LayoutGridPrint,
StylePageLayoutPropertiesElement.LayoutGridRubyBelow,
StylePageLayoutPropertiesElement.LayoutGridRubyHeight,
StylePageLayoutPropertiesElement.LayoutGridSnapTo,
StylePageLayoutPropertiesElement.LayoutGridStandardMode,
StylePageLayoutPropertiesElement.NumFormat,
StylePageLayoutPropertiesElement.NumFormat,
StylePageLayoutPropertiesElement.NumLetterSync,
StylePageLayoutPropertiesElement.NumPrefix,
StylePageLayoutPropertiesElement.NumSuffix,
StylePageLayoutPropertiesElement.PaperTrayName,
StylePageLayoutPropertiesElement.Print,
StylePageLayoutPropertiesElement.PrintOrientation,
StylePageLayoutPropertiesElement.PrintPageOrder,
StylePageLayoutPropertiesElement.RegisterTruthRefStyleName,
StylePageLayoutPropertiesElement.ScaleTo,
StylePageLayoutPropertiesElement.ScaleToPages,
StylePageLayoutPropertiesElement.Shadow,
StylePageLayoutPropertiesElement.TableCentering,
StylePageLayoutPropertiesElement.WritingMode,
StyleParagraphPropertiesElement.BackgroundColor,
StyleParagraphPropertiesElement.Border,
StyleParagraphPropertiesElement.BorderBottom,
StyleParagraphPropertiesElement.BorderLeft,
StyleParagraphPropertiesElement.BorderRight,
StyleParagraphPropertiesElement.BorderTop,
StyleParagraphPropertiesElement.BreakAfter,
StyleParagraphPropertiesElement.BreakBefore,
StyleParagraphPropertiesElement.HyphenationKeep,
StyleParagraphPropertiesElement.HyphenationLadderCount,
StyleParagraphPropertiesElement.KeepTogether,
StyleParagraphPropertiesElement.KeepWithNext,
StyleParagraphPropertiesElement.LineHeight,
StyleParagraphPropertiesElement.Margin,
StyleParagraphPropertiesElement.MarginBottom,
StyleParagraphPropertiesElement.MarginLeft,
StyleParagraphPropertiesElement.MarginRight,
StyleParagraphPropertiesElement.MarginTop,
StyleParagraphPropertiesElement.Orphans,
StyleParagraphPropertiesElement.Padding,
StyleParagraphPropertiesElement.PaddingBottom,
StyleParagraphPropertiesElement.PaddingLeft,
StyleParagraphPropertiesElement.PaddingRight,
StyleParagraphPropertiesElement.PaddingTop,
StyleParagraphPropertiesElement.TextAlign,
StyleParagraphPropertiesElement.TextAlignLast,
StyleParagraphPropertiesElement.TextIndent,
StyleParagraphPropertiesElement.Widows,
StyleParagraphPropertiesElement.AutoTextIndent,
StyleParagraphPropertiesElement.BackgroundTransparency,
StyleParagraphPropertiesElement.BorderLineWidth,
StyleParagraphPropertiesElement.BorderLineWidthBottom,
StyleParagraphPropertiesElement.BorderLineWidthLeft,
StyleParagraphPropertiesElement.BorderLineWidthRight,
StyleParagraphPropertiesElement.BorderLineWidthTop,
StyleParagraphPropertiesElement.FontIndependentLineSpacing,
StyleParagraphPropertiesElement.JoinBorder,
StyleParagraphPropertiesElement.JustifySingleWord,
StyleParagraphPropertiesElement.LineBreak,
StyleParagraphPropertiesElement.LineHeightAtLeast,
StyleParagraphPropertiesElement.LineSpacing,
StyleParagraphPropertiesElement.PageNumber,
StyleParagraphPropertiesElement.PunctuationWrap,
StyleParagraphPropertiesElement.RegisterTrue,
StyleParagraphPropertiesElement.Shadow,
StyleParagraphPropertiesElement.SnapToLayoutGrid,
StyleParagraphPropertiesElement.TabStopDistance,
StyleParagraphPropertiesElement.TextAutospace,
StyleParagraphPropertiesElement.VerticalAlign,
StyleParagraphPropertiesElement.WritingMode,
StyleParagraphPropertiesElement.WritingModeAutomatic,
StyleParagraphPropertiesElement.LineNumber,
StyleParagraphPropertiesElement.NumberLines,
StyleRubyPropertiesElement.RubyAlign,
StyleRubyPropertiesElement.RubyPosition,
StyleSectionPropertiesElement.BackgroundColor,
StyleSectionPropertiesElement.MarginLeft,
StyleSectionPropertiesElement.MarginRight,
StyleSectionPropertiesElement.Editable,
StyleSectionPropertiesElement.Protect,
StyleSectionPropertiesElement.WritingMode,
StyleSectionPropertiesElement.DontBalanceTextColumns,
StyleTableCellPropertiesElement.BackgroundColor,
StyleTableCellPropertiesElement.Border,
StyleTableCellPropertiesElement.BorderBottom,
StyleTableCellPropertiesElement.BorderLeft,
StyleTableCellPropertiesElement.BorderRight,
StyleTableCellPropertiesElement.BorderTop,
StyleTableCellPropertiesElement.Padding,
StyleTableCellPropertiesElement.PaddingBottom,
StyleTableCellPropertiesElement.PaddingLeft,
StyleTableCellPropertiesElement.PaddingRight,
StyleTableCellPropertiesElement.PaddingTop,
StyleTableCellPropertiesElement.WrapOption,
StyleTableCellPropertiesElement.BorderLineWidth,
StyleTableCellPropertiesElement.BorderLineWidthBottom,
StyleTableCellPropertiesElement.BorderLineWidthLeft,
StyleTableCellPropertiesElement.BorderLineWidthRight,
StyleTableCellPropertiesElement.BorderLineWidthTop,
StyleTableCellPropertiesElement.CellProtect,
StyleTableCellPropertiesElement.DecimalPlaces,
StyleTableCellPropertiesElement.DiagonalBlTr,
StyleTableCellPropertiesElement.DiagonalBlTrWidths,
StyleTableCellPropertiesElement.DiagonalTlBr,
StyleTableCellPropertiesElement.DiagonalTlBrWidths,
StyleTableCellPropertiesElement.Direction,
StyleTableCellPropertiesElement.GlyphOrientationVertical,
StyleTableCellPropertiesElement.PrintContent,
StyleTableCellPropertiesElement.RepeatContent,
StyleTableCellPropertiesElement.RotationAlign,
StyleTableCellPropertiesElement.RotationAngle,
StyleTableCellPropertiesElement.Shadow,
StyleTableCellPropertiesElement.ShrinkToFit,
StyleTableCellPropertiesElement.TextAlignSource,
StyleTableCellPropertiesElement.VerticalAlign,
StyleTableCellPropertiesElement.WritingMode,
StyleTableColumnPropertiesElement.BreakAfter,
StyleTableColumnPropertiesElement.BreakBefore,
StyleTableColumnPropertiesElement.ColumnWidth,
StyleTableColumnPropertiesElement.RelColumnWidth,
StyleTableColumnPropertiesElement.UseOptimalColumnWidth,
StyleTablePropertiesElement.BackgroundColor,
StyleTablePropertiesElement.BreakAfter,
StyleTablePropertiesElement.BreakBefore,
StyleTablePropertiesElement.KeepWithNext,
StyleTablePropertiesElement.Margin,
StyleTablePropertiesElement.MarginBottom,
StyleTablePropertiesElement.MarginLeft,
StyleTablePropertiesElement.MarginRight,
StyleTablePropertiesElement.MarginTop,
StyleTablePropertiesElement.MayBreakBetweenRows,
StyleTablePropertiesElement.PageNumber,
StyleTablePropertiesElement.RelWidth,
StyleTablePropertiesElement.Shadow,
StyleTablePropertiesElement.Width,
StyleTablePropertiesElement.WritingMode,
StyleTablePropertiesElement.Align,
StyleTablePropertiesElement.BorderModel,
StyleTablePropertiesElement.Display,
StyleTableRowPropertiesElement.BackgroundColor,
StyleTableRowPropertiesElement.BreakAfter,
StyleTableRowPropertiesElement.BreakBefore,
StyleTableRowPropertiesElement.KeepTogether,
StyleTableRowPropertiesElement.MinRowHeight,
StyleTableRowPropertiesElement.RowHeight,
StyleTableRowPropertiesElement.UseOptimalRowHeight,
StyleTextPropertiesElement.BackgroundColor,
StyleTextPropertiesElement.Color,
StyleTextPropertiesElement.Country,
StyleTextPropertiesElement.FontFamily,
StyleTextPropertiesElement.FontSize,
StyleTextPropertiesElement.FontStyle,
StyleTextPropertiesElement.FontVariant,
StyleTextPropertiesElement.FontWeight,
StyleTextPropertiesElement.Hyphenate,
StyleTextPropertiesElement.HyphenationPushCharCount,
StyleTextPropertiesElement.HyphenationRemainCharCount,
StyleTextPropertiesElement.Language,
StyleTextPropertiesElement.LetterSpacing,
StyleTextPropertiesElement.Script,
StyleTextPropertiesElement.TextShadow,
StyleTextPropertiesElement.TextTransform,
StyleTextPropertiesElement.CountryAsian,
StyleTextPropertiesElement.CountryComplex,
StyleTextPropertiesElement.FontCharset,
StyleTextPropertiesElement.FontCharsetAsian,
StyleTextPropertiesElement.FontCharsetComplex,
StyleTextPropertiesElement.FontFamilyAsian,
StyleTextPropertiesElement.FontFamilyComplex,
StyleTextPropertiesElement.FontFamilyGeneric,
StyleTextPropertiesElement.FontFamilyGenericAsian,
StyleTextPropertiesElement.FontFamilyGenericComplex,
StyleTextPropertiesElement.FontName,
StyleTextPropertiesElement.FontNameAsian,
StyleTextPropertiesElement.FontNameComplex,
StyleTextPropertiesElement.FontPitch,
StyleTextPropertiesElement.FontPitchAsian,
StyleTextPropertiesElement.FontPitchComplex,
StyleTextPropertiesElement.FontRelief,
StyleTextPropertiesElement.FontSizeAsian,
StyleTextPropertiesElement.FontSizeComplex,
StyleTextPropertiesElement.FontSizeRel,
StyleTextPropertiesElement.FontSizeRelAsian,
StyleTextPropertiesElement.FontSizeRelComplex,
StyleTextPropertiesElement.FontStyleAsian,
StyleTextPropertiesElement.FontStyleComplex,
StyleTextPropertiesElement.FontStyleName,
StyleTextPropertiesElement.FontStyleNameAsian,
StyleTextPropertiesElement.FontStyleNameComplex,
StyleTextPropertiesElement.FontWeightAsian,
StyleTextPropertiesElement.FontWeightComplex,
StyleTextPropertiesElement.LanguageAsian,
StyleTextPropertiesElement.LanguageComplex,
StyleTextPropertiesElement.LetterKerning,
StyleTextPropertiesElement.RfcLanguageTag,
StyleTextPropertiesElement.RfcLanguageTagAsian,
StyleTextPropertiesElement.RfcLanguageTagComplex,
StyleTextPropertiesElement.ScriptAsian,
StyleTextPropertiesElement.ScriptComplex,
StyleTextPropertiesElement.ScriptType,
StyleTextPropertiesElement.TextBlinking,
StyleTextPropertiesElement.TextCombine,
StyleTextPropertiesElement.TextCombineEndChar,
StyleTextPropertiesElement.TextCombineStartChar,
StyleTextPropertiesElement.TextEmphasize,
StyleTextPropertiesElement.TextLineThroughColor,
StyleTextPropertiesElement.TextLineThroughMode,
StyleTextPropertiesElement.TextLineThroughStyle,
StyleTextPropertiesElement.TextLineThroughText,
StyleTextPropertiesElement.TextLineThroughTextStyle,
StyleTextPropertiesElement.TextLineThroughType,
StyleTextPropertiesElement.TextLineThroughWidth,
StyleTextPropertiesElement.TextOutline,
StyleTextPropertiesElement.TextOverlineColor,
StyleTextPropertiesElement.TextOverlineMode,
StyleTextPropertiesElement.TextOverlineStyle,
StyleTextPropertiesElement.TextOverlineType,
StyleTextPropertiesElement.TextOverlineWidth,
StyleTextPropertiesElement.TextPosition,
StyleTextPropertiesElement.TextRotationAngle,
StyleTextPropertiesElement.TextRotationScale,
StyleTextPropertiesElement.TextScale,
StyleTextPropertiesElement.TextUnderlineColor,
StyleTextPropertiesElement.TextUnderlineMode,
StyleTextPropertiesElement.TextUnderlineStyle,
StyleTextPropertiesElement.TextUnderlineType,
StyleTextPropertiesElement.TextUnderlineWidth,
StyleTextPropertiesElement.UseWindowFontColor,
StyleTextPropertiesElement.Condition,
StyleTextPropertiesElement.Display,
StyleTextPropertiesElement.Display,
StyleTextPropertiesElement.Display,
});
public final static OdfStyleFamily Text = new OdfStyleFamily("text",
new OdfStyleProperty[]{
StyleChartPropertiesElement.AngleOffset,
StyleChartPropertiesElement.AutoPosition,
StyleChartPropertiesElement.AutoSize,
StyleChartPropertiesElement.AxisLabelPosition,
StyleChartPropertiesElement.AxisPosition,
StyleChartPropertiesElement.ConnectBars,
StyleChartPropertiesElement.DataLabelNumber,
StyleChartPropertiesElement.DataLabelSymbol,
StyleChartPropertiesElement.DataLabelText,
StyleChartPropertiesElement.Deep,
StyleChartPropertiesElement.DisplayLabel,
StyleChartPropertiesElement.ErrorCategory,
StyleChartPropertiesElement.ErrorLowerIndicator,
StyleChartPropertiesElement.ErrorLowerLimit,
StyleChartPropertiesElement.ErrorLowerRange,
StyleChartPropertiesElement.ErrorMargin,
StyleChartPropertiesElement.ErrorPercentage,
StyleChartPropertiesElement.ErrorUpperIndicator,
StyleChartPropertiesElement.ErrorUpperLimit,
StyleChartPropertiesElement.ErrorUpperRange,
StyleChartPropertiesElement.GapWidth,
StyleChartPropertiesElement.GroupBarsPerAxis,
StyleChartPropertiesElement.HoleSize,
StyleChartPropertiesElement.IncludeHiddenCells,
StyleChartPropertiesElement.Interpolation,
StyleChartPropertiesElement.IntervalMajor,
StyleChartPropertiesElement.IntervalMinorDivisor,
StyleChartPropertiesElement.JapaneseCandleStick,
StyleChartPropertiesElement.LabelArrangement,
StyleChartPropertiesElement.LabelPosition,
StyleChartPropertiesElement.LabelPositionNegative,
StyleChartPropertiesElement.Lines,
StyleChartPropertiesElement.LinkDataStyleToSource,
StyleChartPropertiesElement.Logarithmic,
StyleChartPropertiesElement.Maximum,
StyleChartPropertiesElement.MeanValue,
StyleChartPropertiesElement.Minimum,
StyleChartPropertiesElement.Origin,
StyleChartPropertiesElement.Overlap,
StyleChartPropertiesElement.Percentage,
StyleChartPropertiesElement.PieOffset,
StyleChartPropertiesElement.RegressionType,
StyleChartPropertiesElement.ReverseDirection,
StyleChartPropertiesElement.RightAngledAxes,
StyleChartPropertiesElement.ScaleText,
StyleChartPropertiesElement.SeriesSource,
StyleChartPropertiesElement.SolidType,
StyleChartPropertiesElement.SortByXValues,
StyleChartPropertiesElement.SplineOrder,
StyleChartPropertiesElement.SplineResolution,
StyleChartPropertiesElement.Stacked,
StyleChartPropertiesElement.SymbolHeight,
StyleChartPropertiesElement.SymbolName,
StyleChartPropertiesElement.SymbolType,
StyleChartPropertiesElement.SymbolType,
StyleChartPropertiesElement.SymbolType,
StyleChartPropertiesElement.SymbolType,
StyleChartPropertiesElement.SymbolWidth,
StyleChartPropertiesElement.TextOverlap,
StyleChartPropertiesElement.ThreeDimensional,
StyleChartPropertiesElement.TickMarkPosition,
StyleChartPropertiesElement.TickMarksMajorInner,
StyleChartPropertiesElement.TickMarksMajorOuter,
StyleChartPropertiesElement.TickMarksMinorInner,
StyleChartPropertiesElement.TickMarksMinorOuter,
StyleChartPropertiesElement.TreatEmptyCells,
StyleChartPropertiesElement.Vertical,
StyleChartPropertiesElement.Visible,
StyleChartPropertiesElement.Direction,
StyleChartPropertiesElement.RotationAngle,
StyleChartPropertiesElement.LineBreak,
StyleDrawingPagePropertiesElement.BackgroundSize,
StyleDrawingPagePropertiesElement.Fill,
StyleDrawingPagePropertiesElement.FillColor,
StyleDrawingPagePropertiesElement.FillGradientName,
StyleDrawingPagePropertiesElement.FillHatchName,
StyleDrawingPagePropertiesElement.FillHatchSolid,
StyleDrawingPagePropertiesElement.FillImageHeight,
StyleDrawingPagePropertiesElement.FillImageName,
StyleDrawingPagePropertiesElement.FillImageRefPoint,
StyleDrawingPagePropertiesElement.FillImageRefPointX,
StyleDrawingPagePropertiesElement.FillImageRefPointY,
StyleDrawingPagePropertiesElement.FillImageWidth,
StyleDrawingPagePropertiesElement.GradientStepCount,
StyleDrawingPagePropertiesElement.Opacity,
StyleDrawingPagePropertiesElement.OpacityName,
StyleDrawingPagePropertiesElement.SecondaryFillColor,
StyleDrawingPagePropertiesElement.TileRepeatOffset,
StyleDrawingPagePropertiesElement.BackgroundObjectsVisible,
StyleDrawingPagePropertiesElement.BackgroundVisible,
StyleDrawingPagePropertiesElement.DisplayDateTime,
StyleDrawingPagePropertiesElement.DisplayFooter,
StyleDrawingPagePropertiesElement.DisplayHeader,
StyleDrawingPagePropertiesElement.DisplayPageNumber,
StyleDrawingPagePropertiesElement.Duration,
StyleDrawingPagePropertiesElement.TransitionSpeed,
StyleDrawingPagePropertiesElement.TransitionStyle,
StyleDrawingPagePropertiesElement.TransitionType,
StyleDrawingPagePropertiesElement.Visibility,
StyleDrawingPagePropertiesElement.Direction,
StyleDrawingPagePropertiesElement.FadeColor,
StyleDrawingPagePropertiesElement.Subtype,
StyleDrawingPagePropertiesElement.Type,
StyleDrawingPagePropertiesElement.Repeat,
StyleDrawingPagePropertiesElement.FillRule,
StyleGraphicPropertiesElement.AmbientColor,
StyleGraphicPropertiesElement.BackScale,
StyleGraphicPropertiesElement.BackfaceCulling,
StyleGraphicPropertiesElement.CloseBack,
StyleGraphicPropertiesElement.CloseFront,
StyleGraphicPropertiesElement.Depth,
StyleGraphicPropertiesElement.DiffuseColor,
StyleGraphicPropertiesElement.EdgeRounding,
StyleGraphicPropertiesElement.EdgeRoundingMode,
StyleGraphicPropertiesElement.EmissiveColor,
StyleGraphicPropertiesElement.EndAngle,
StyleGraphicPropertiesElement.HorizontalSegments,
StyleGraphicPropertiesElement.LightingMode,
StyleGraphicPropertiesElement.NormalsDirection,
StyleGraphicPropertiesElement.NormalsKind,
StyleGraphicPropertiesElement.Dr3dShadow,
StyleGraphicPropertiesElement.Shininess,
StyleGraphicPropertiesElement.SpecularColor,
StyleGraphicPropertiesElement.TextureFilter,
StyleGraphicPropertiesElement.TextureGenerationModeX,
StyleGraphicPropertiesElement.TextureGenerationModeY,
StyleGraphicPropertiesElement.TextureKind,
StyleGraphicPropertiesElement.TextureMode,
StyleGraphicPropertiesElement.VerticalSegments,
StyleGraphicPropertiesElement.AutoGrowHeight,
StyleGraphicPropertiesElement.AutoGrowWidth,
StyleGraphicPropertiesElement.Blue,
StyleGraphicPropertiesElement.CaptionAngle,
StyleGraphicPropertiesElement.CaptionAngleType,
StyleGraphicPropertiesElement.CaptionEscape,
StyleGraphicPropertiesElement.CaptionEscapeDirection,
StyleGraphicPropertiesElement.CaptionFitLineLength,
StyleGraphicPropertiesElement.CaptionGap,
StyleGraphicPropertiesElement.CaptionLineLength,
StyleGraphicPropertiesElement.CaptionType,
StyleGraphicPropertiesElement.ColorInversion,
StyleGraphicPropertiesElement.ColorMode,
StyleGraphicPropertiesElement.Contrast,
StyleGraphicPropertiesElement.DecimalPlaces,
StyleGraphicPropertiesElement.DrawAspect,
StyleGraphicPropertiesElement.EndGuide,
StyleGraphicPropertiesElement.EndLineSpacingHorizontal,
StyleGraphicPropertiesElement.EndLineSpacingVertical,
StyleGraphicPropertiesElement.Fill,
StyleGraphicPropertiesElement.FillColor,
StyleGraphicPropertiesElement.FillGradientName,
StyleGraphicPropertiesElement.FillHatchName,
StyleGraphicPropertiesElement.FillHatchSolid,
StyleGraphicPropertiesElement.FillImageHeight,
StyleGraphicPropertiesElement.FillImageName,
StyleGraphicPropertiesElement.FillImageRefPoint,
StyleGraphicPropertiesElement.FillImageRefPointX,
StyleGraphicPropertiesElement.FillImageRefPointY,
StyleGraphicPropertiesElement.FillImageWidth,
StyleGraphicPropertiesElement.FitToContour,
StyleGraphicPropertiesElement.FitToSize,
StyleGraphicPropertiesElement.FrameDisplayBorder,
StyleGraphicPropertiesElement.FrameDisplayScrollbar,
StyleGraphicPropertiesElement.FrameMarginHorizontal,
StyleGraphicPropertiesElement.FrameMarginVertical,
StyleGraphicPropertiesElement.Gamma,
StyleGraphicPropertiesElement.GradientStepCount,
StyleGraphicPropertiesElement.Green,
StyleGraphicPropertiesElement.GuideDistance,
StyleGraphicPropertiesElement.GuideOverhang,
StyleGraphicPropertiesElement.ImageOpacity,
StyleGraphicPropertiesElement.LineDistance,
StyleGraphicPropertiesElement.Luminance,
StyleGraphicPropertiesElement.MarkerEnd,
StyleGraphicPropertiesElement.MarkerEndCenter,
StyleGraphicPropertiesElement.MarkerEndWidth,
StyleGraphicPropertiesElement.MarkerStart,
StyleGraphicPropertiesElement.MarkerStartCenter,
StyleGraphicPropertiesElement.MarkerStartWidth,
StyleGraphicPropertiesElement.MeasureAlign,
StyleGraphicPropertiesElement.MeasureVerticalAlign,
StyleGraphicPropertiesElement.OleDrawAspect,
StyleGraphicPropertiesElement.Opacity,
StyleGraphicPropertiesElement.OpacityName,
StyleGraphicPropertiesElement.Parallel,
StyleGraphicPropertiesElement.Placing,
StyleGraphicPropertiesElement.Red,
StyleGraphicPropertiesElement.SecondaryFillColor,
StyleGraphicPropertiesElement.DrawShadow,
StyleGraphicPropertiesElement.ShadowColor,
StyleGraphicPropertiesElement.ShadowOffsetX,
StyleGraphicPropertiesElement.ShadowOffsetY,
StyleGraphicPropertiesElement.ShadowOpacity,
StyleGraphicPropertiesElement.ShowUnit,
StyleGraphicPropertiesElement.StartGuide,
StyleGraphicPropertiesElement.StartLineSpacingHorizontal,
StyleGraphicPropertiesElement.StartLineSpacingVertical,
StyleGraphicPropertiesElement.Stroke,
StyleGraphicPropertiesElement.StrokeDash,
StyleGraphicPropertiesElement.StrokeDashNames,
StyleGraphicPropertiesElement.StrokeLinejoin,
StyleGraphicPropertiesElement.SymbolColor,
StyleGraphicPropertiesElement.TextareaHorizontalAlign,
StyleGraphicPropertiesElement.TextareaVerticalAlign,
StyleGraphicPropertiesElement.TileRepeatOffset,
StyleGraphicPropertiesElement.Unit,
StyleGraphicPropertiesElement.VisibleAreaHeight,
StyleGraphicPropertiesElement.VisibleAreaLeft,
StyleGraphicPropertiesElement.VisibleAreaTop,
StyleGraphicPropertiesElement.VisibleAreaWidth,
StyleGraphicPropertiesElement.WrapInfluenceOnPosition,
StyleGraphicPropertiesElement.BackgroundColor,
StyleGraphicPropertiesElement.Border,
StyleGraphicPropertiesElement.BorderBottom,
StyleGraphicPropertiesElement.BorderLeft,
StyleGraphicPropertiesElement.BorderRight,
StyleGraphicPropertiesElement.BorderTop,
StyleGraphicPropertiesElement.Clip,
StyleGraphicPropertiesElement.Margin,
StyleGraphicPropertiesElement.MarginBottom,
StyleGraphicPropertiesElement.MarginLeft,
StyleGraphicPropertiesElement.MarginRight,
StyleGraphicPropertiesElement.MarginTop,
StyleGraphicPropertiesElement.MaxHeight,
StyleGraphicPropertiesElement.MaxWidth,
StyleGraphicPropertiesElement.MinHeight,
StyleGraphicPropertiesElement.MinWidth,
StyleGraphicPropertiesElement.Padding,
StyleGraphicPropertiesElement.PaddingBottom,
StyleGraphicPropertiesElement.PaddingLeft,
StyleGraphicPropertiesElement.PaddingRight,
StyleGraphicPropertiesElement.PaddingTop,
StyleGraphicPropertiesElement.WrapOption,
StyleGraphicPropertiesElement.BackgroundTransparency,
StyleGraphicPropertiesElement.BorderLineWidth,
StyleGraphicPropertiesElement.BorderLineWidthBottom,
StyleGraphicPropertiesElement.BorderLineWidthLeft,
StyleGraphicPropertiesElement.BorderLineWidthRight,
StyleGraphicPropertiesElement.BorderLineWidthTop,
StyleGraphicPropertiesElement.Editable,
StyleGraphicPropertiesElement.FlowWithText,
StyleGraphicPropertiesElement.HorizontalPos,
StyleGraphicPropertiesElement.HorizontalRel,
StyleGraphicPropertiesElement.Mirror,
StyleGraphicPropertiesElement.NumberWrappedParagraphs,
StyleGraphicPropertiesElement.OverflowBehavior,
StyleGraphicPropertiesElement.PrintContent,
StyleGraphicPropertiesElement.Protect,
StyleGraphicPropertiesElement.RelHeight,
StyleGraphicPropertiesElement.RelWidth,
StyleGraphicPropertiesElement.Repeat,
StyleGraphicPropertiesElement.RunThrough,
StyleGraphicPropertiesElement.StyleShadow,
StyleGraphicPropertiesElement.ShrinkToFit,
StyleGraphicPropertiesElement.VerticalPos,
StyleGraphicPropertiesElement.VerticalRel,
StyleGraphicPropertiesElement.Wrap,
StyleGraphicPropertiesElement.WrapContour,
StyleGraphicPropertiesElement.WrapContourMode,
StyleGraphicPropertiesElement.WrapDynamicThreshold,
StyleGraphicPropertiesElement.WritingMode,
StyleGraphicPropertiesElement.FillRule,
StyleGraphicPropertiesElement.Height,
StyleGraphicPropertiesElement.StrokeColor,
StyleGraphicPropertiesElement.StrokeLinecap,
StyleGraphicPropertiesElement.StrokeOpacity,
StyleGraphicPropertiesElement.StrokeWidth,
StyleGraphicPropertiesElement.Width,
StyleGraphicPropertiesElement.X,
StyleGraphicPropertiesElement.Y,
StyleGraphicPropertiesElement.AnchorPageNumber,
StyleGraphicPropertiesElement.AnchorType,
StyleGraphicPropertiesElement.Animation,
StyleGraphicPropertiesElement.AnimationDelay,
StyleGraphicPropertiesElement.AnimationDirection,
StyleGraphicPropertiesElement.AnimationRepeat,
StyleGraphicPropertiesElement.AnimationStartInside,
StyleGraphicPropertiesElement.AnimationSteps,
StyleGraphicPropertiesElement.AnimationStopInside,
StyleHeaderFooterPropertiesElement.BackgroundColor,
StyleHeaderFooterPropertiesElement.Border,
StyleHeaderFooterPropertiesElement.BorderBottom,
StyleHeaderFooterPropertiesElement.BorderLeft,
StyleHeaderFooterPropertiesElement.BorderRight,
StyleHeaderFooterPropertiesElement.BorderTop,
StyleHeaderFooterPropertiesElement.Margin,
StyleHeaderFooterPropertiesElement.MarginBottom,
StyleHeaderFooterPropertiesElement.MarginLeft,
StyleHeaderFooterPropertiesElement.MarginRight,
StyleHeaderFooterPropertiesElement.MarginTop,
StyleHeaderFooterPropertiesElement.MinHeight,
StyleHeaderFooterPropertiesElement.Padding,
StyleHeaderFooterPropertiesElement.PaddingBottom,
StyleHeaderFooterPropertiesElement.PaddingLeft,
StyleHeaderFooterPropertiesElement.PaddingRight,
StyleHeaderFooterPropertiesElement.PaddingTop,
StyleHeaderFooterPropertiesElement.BorderLineWidth,
StyleHeaderFooterPropertiesElement.BorderLineWidthBottom,
StyleHeaderFooterPropertiesElement.BorderLineWidthLeft,
StyleHeaderFooterPropertiesElement.BorderLineWidthRight,
StyleHeaderFooterPropertiesElement.BorderLineWidthTop,
StyleHeaderFooterPropertiesElement.DynamicSpacing,
StyleHeaderFooterPropertiesElement.Shadow,
StyleHeaderFooterPropertiesElement.Height,
StyleListLevelPropertiesElement.Height,
StyleListLevelPropertiesElement.TextAlign,
StyleListLevelPropertiesElement.Width,
StyleListLevelPropertiesElement.FontName,
StyleListLevelPropertiesElement.VerticalPos,
StyleListLevelPropertiesElement.VerticalRel,
StyleListLevelPropertiesElement.Y,
StyleListLevelPropertiesElement.ListLevelPositionAndSpaceMode,
StyleListLevelPropertiesElement.MinLabelDistance,
StyleListLevelPropertiesElement.MinLabelWidth,
StyleListLevelPropertiesElement.SpaceBefore,
StylePageLayoutPropertiesElement.BackgroundColor,
StylePageLayoutPropertiesElement.Border,
StylePageLayoutPropertiesElement.BorderBottom,
StylePageLayoutPropertiesElement.BorderLeft,
StylePageLayoutPropertiesElement.BorderRight,
StylePageLayoutPropertiesElement.BorderTop,
StylePageLayoutPropertiesElement.Margin,
StylePageLayoutPropertiesElement.MarginBottom,
StylePageLayoutPropertiesElement.MarginLeft,
StylePageLayoutPropertiesElement.MarginRight,
StylePageLayoutPropertiesElement.MarginTop,
StylePageLayoutPropertiesElement.Padding,
StylePageLayoutPropertiesElement.PaddingBottom,
StylePageLayoutPropertiesElement.PaddingLeft,
StylePageLayoutPropertiesElement.PaddingRight,
StylePageLayoutPropertiesElement.PaddingTop,
StylePageLayoutPropertiesElement.PageHeight,
StylePageLayoutPropertiesElement.PageWidth,
StylePageLayoutPropertiesElement.BorderLineWidth,
StylePageLayoutPropertiesElement.BorderLineWidthBottom,
StylePageLayoutPropertiesElement.BorderLineWidthLeft,
StylePageLayoutPropertiesElement.BorderLineWidthRight,
StylePageLayoutPropertiesElement.BorderLineWidthTop,
StylePageLayoutPropertiesElement.FirstPageNumber,
StylePageLayoutPropertiesElement.FootnoteMaxHeight,
StylePageLayoutPropertiesElement.LayoutGridBaseHeight,
StylePageLayoutPropertiesElement.LayoutGridBaseWidth,
StylePageLayoutPropertiesElement.LayoutGridColor,
StylePageLayoutPropertiesElement.LayoutGridDisplay,
StylePageLayoutPropertiesElement.LayoutGridLines,
StylePageLayoutPropertiesElement.LayoutGridMode,
StylePageLayoutPropertiesElement.LayoutGridPrint,
StylePageLayoutPropertiesElement.LayoutGridRubyBelow,
StylePageLayoutPropertiesElement.LayoutGridRubyHeight,
StylePageLayoutPropertiesElement.LayoutGridSnapTo,
StylePageLayoutPropertiesElement.LayoutGridStandardMode,
StylePageLayoutPropertiesElement.NumFormat,
StylePageLayoutPropertiesElement.NumFormat,
StylePageLayoutPropertiesElement.NumLetterSync,
StylePageLayoutPropertiesElement.NumPrefix,
StylePageLayoutPropertiesElement.NumSuffix,
StylePageLayoutPropertiesElement.PaperTrayName,
StylePageLayoutPropertiesElement.Print,
StylePageLayoutPropertiesElement.PrintOrientation,
StylePageLayoutPropertiesElement.PrintPageOrder,
StylePageLayoutPropertiesElement.RegisterTruthRefStyleName,
StylePageLayoutPropertiesElement.ScaleTo,
StylePageLayoutPropertiesElement.ScaleToPages,
StylePageLayoutPropertiesElement.Shadow,
StylePageLayoutPropertiesElement.TableCentering,
StylePageLayoutPropertiesElement.WritingMode,
StyleParagraphPropertiesElement.BackgroundColor,
StyleParagraphPropertiesElement.Border,
StyleParagraphPropertiesElement.BorderBottom,
StyleParagraphPropertiesElement.BorderLeft,
StyleParagraphPropertiesElement.BorderRight,
StyleParagraphPropertiesElement.BorderTop,
StyleParagraphPropertiesElement.BreakAfter,
StyleParagraphPropertiesElement.BreakBefore,
StyleParagraphPropertiesElement.HyphenationKeep,
StyleParagraphPropertiesElement.HyphenationLadderCount,
StyleParagraphPropertiesElement.KeepTogether,
StyleParagraphPropertiesElement.KeepWithNext,
StyleParagraphPropertiesElement.LineHeight,
StyleParagraphPropertiesElement.Margin,
StyleParagraphPropertiesElement.MarginBottom,
StyleParagraphPropertiesElement.MarginLeft,
StyleParagraphPropertiesElement.MarginRight,
StyleParagraphPropertiesElement.MarginTop,
StyleParagraphPropertiesElement.Orphans,
StyleParagraphPropertiesElement.Padding,
StyleParagraphPropertiesElement.PaddingBottom,
StyleParagraphPropertiesElement.PaddingLeft,
StyleParagraphPropertiesElement.PaddingRight,
StyleParagraphPropertiesElement.PaddingTop,
StyleParagraphPropertiesElement.TextAlign,
StyleParagraphPropertiesElement.TextAlignLast,
StyleParagraphPropertiesElement.TextIndent,
StyleParagraphPropertiesElement.Widows,
StyleParagraphPropertiesElement.AutoTextIndent,
StyleParagraphPropertiesElement.BackgroundTransparency,
StyleParagraphPropertiesElement.BorderLineWidth,
StyleParagraphPropertiesElement.BorderLineWidthBottom,
StyleParagraphPropertiesElement.BorderLineWidthLeft,
StyleParagraphPropertiesElement.BorderLineWidthRight,
StyleParagraphPropertiesElement.BorderLineWidthTop,
StyleParagraphPropertiesElement.FontIndependentLineSpacing,
StyleParagraphPropertiesElement.JoinBorder,
StyleParagraphPropertiesElement.JustifySingleWord,
StyleParagraphPropertiesElement.LineBreak,
StyleParagraphPropertiesElement.LineHeightAtLeast,
StyleParagraphPropertiesElement.LineSpacing,
StyleParagraphPropertiesElement.PageNumber,
StyleParagraphPropertiesElement.PunctuationWrap,
StyleParagraphPropertiesElement.RegisterTrue,
StyleParagraphPropertiesElement.Shadow,
StyleParagraphPropertiesElement.SnapToLayoutGrid,
StyleParagraphPropertiesElement.TabStopDistance,
StyleParagraphPropertiesElement.TextAutospace,
StyleParagraphPropertiesElement.VerticalAlign,
StyleParagraphPropertiesElement.WritingMode,
StyleParagraphPropertiesElement.WritingModeAutomatic,
StyleParagraphPropertiesElement.LineNumber,
StyleParagraphPropertiesElement.NumberLines,
StyleRubyPropertiesElement.RubyAlign,
StyleRubyPropertiesElement.RubyPosition,
StyleSectionPropertiesElement.BackgroundColor,
StyleSectionPropertiesElement.MarginLeft,
StyleSectionPropertiesElement.MarginRight,
StyleSectionPropertiesElement.Editable,
StyleSectionPropertiesElement.Protect,
StyleSectionPropertiesElement.WritingMode,
StyleSectionPropertiesElement.DontBalanceTextColumns,
StyleTableCellPropertiesElement.BackgroundColor,
StyleTableCellPropertiesElement.Border,
StyleTableCellPropertiesElement.BorderBottom,
StyleTableCellPropertiesElement.BorderLeft,
StyleTableCellPropertiesElement.BorderRight,
StyleTableCellPropertiesElement.BorderTop,
StyleTableCellPropertiesElement.Padding,
StyleTableCellPropertiesElement.PaddingBottom,
StyleTableCellPropertiesElement.PaddingLeft,
StyleTableCellPropertiesElement.PaddingRight,
StyleTableCellPropertiesElement.PaddingTop,
StyleTableCellPropertiesElement.WrapOption,
StyleTableCellPropertiesElement.BorderLineWidth,
StyleTableCellPropertiesElement.BorderLineWidthBottom,
StyleTableCellPropertiesElement.BorderLineWidthLeft,
StyleTableCellPropertiesElement.BorderLineWidthRight,
StyleTableCellPropertiesElement.BorderLineWidthTop,
StyleTableCellPropertiesElement.CellProtect,
StyleTableCellPropertiesElement.DecimalPlaces,
StyleTableCellPropertiesElement.DiagonalBlTr,
StyleTableCellPropertiesElement.DiagonalBlTrWidths,
StyleTableCellPropertiesElement.DiagonalTlBr,
StyleTableCellPropertiesElement.DiagonalTlBrWidths,
StyleTableCellPropertiesElement.Direction,
StyleTableCellPropertiesElement.GlyphOrientationVertical,
StyleTableCellPropertiesElement.PrintContent,
StyleTableCellPropertiesElement.RepeatContent,
StyleTableCellPropertiesElement.RotationAlign,
StyleTableCellPropertiesElement.RotationAngle,
StyleTableCellPropertiesElement.Shadow,
StyleTableCellPropertiesElement.ShrinkToFit,
StyleTableCellPropertiesElement.TextAlignSource,
StyleTableCellPropertiesElement.VerticalAlign,
StyleTableCellPropertiesElement.WritingMode,
StyleTableColumnPropertiesElement.BreakAfter,
StyleTableColumnPropertiesElement.BreakBefore,
StyleTableColumnPropertiesElement.ColumnWidth,
StyleTableColumnPropertiesElement.RelColumnWidth,
StyleTableColumnPropertiesElement.UseOptimalColumnWidth,
StyleTablePropertiesElement.BackgroundColor,
StyleTablePropertiesElement.BreakAfter,
StyleTablePropertiesElement.BreakBefore,
StyleTablePropertiesElement.KeepWithNext,
StyleTablePropertiesElement.Margin,
StyleTablePropertiesElement.MarginBottom,
StyleTablePropertiesElement.MarginLeft,
StyleTablePropertiesElement.MarginRight,
StyleTablePropertiesElement.MarginTop,
StyleTablePropertiesElement.MayBreakBetweenRows,
StyleTablePropertiesElement.PageNumber,
StyleTablePropertiesElement.RelWidth,
StyleTablePropertiesElement.Shadow,
StyleTablePropertiesElement.Width,
StyleTablePropertiesElement.WritingMode,
StyleTablePropertiesElement.Align,
StyleTablePropertiesElement.BorderModel,
StyleTablePropertiesElement.Display,
StyleTableRowPropertiesElement.BackgroundColor,
StyleTableRowPropertiesElement.BreakAfter,
StyleTableRowPropertiesElement.BreakBefore,
StyleTableRowPropertiesElement.KeepTogether,
StyleTableRowPropertiesElement.MinRowHeight,
StyleTableRowPropertiesElement.RowHeight,
StyleTableRowPropertiesElement.UseOptimalRowHeight,
StyleTextPropertiesElement.BackgroundColor,
StyleTextPropertiesElement.Color,
StyleTextPropertiesElement.Country,
StyleTextPropertiesElement.FontFamily,
StyleTextPropertiesElement.FontSize,
StyleTextPropertiesElement.FontStyle,
StyleTextPropertiesElement.FontVariant,
StyleTextPropertiesElement.FontWeight,
StyleTextPropertiesElement.Hyphenate,
StyleTextPropertiesElement.HyphenationPushCharCount,
StyleTextPropertiesElement.HyphenationRemainCharCount,
StyleTextPropertiesElement.Language,
StyleTextPropertiesElement.LetterSpacing,
StyleTextPropertiesElement.Script,
StyleTextPropertiesElement.TextShadow,
StyleTextPropertiesElement.TextTransform,
StyleTextPropertiesElement.CountryAsian,
StyleTextPropertiesElement.CountryComplex,
StyleTextPropertiesElement.FontCharset,
StyleTextPropertiesElement.FontCharsetAsian,
StyleTextPropertiesElement.FontCharsetComplex,
StyleTextPropertiesElement.FontFamilyAsian,
StyleTextPropertiesElement.FontFamilyComplex,
StyleTextPropertiesElement.FontFamilyGeneric,
StyleTextPropertiesElement.FontFamilyGenericAsian,
StyleTextPropertiesElement.FontFamilyGenericComplex,
StyleTextPropertiesElement.FontName,
StyleTextPropertiesElement.FontNameAsian,
StyleTextPropertiesElement.FontNameComplex,
StyleTextPropertiesElement.FontPitch,
StyleTextPropertiesElement.FontPitchAsian,
StyleTextPropertiesElement.FontPitchComplex,
StyleTextPropertiesElement.FontRelief,
StyleTextPropertiesElement.FontSizeAsian,
StyleTextPropertiesElement.FontSizeComplex,
StyleTextPropertiesElement.FontSizeRel,
StyleTextPropertiesElement.FontSizeRelAsian,
StyleTextPropertiesElement.FontSizeRelComplex,
StyleTextPropertiesElement.FontStyleAsian,
StyleTextPropertiesElement.FontStyleComplex,
StyleTextPropertiesElement.FontStyleName,
StyleTextPropertiesElement.FontStyleNameAsian,
StyleTextPropertiesElement.FontStyleNameComplex,
StyleTextPropertiesElement.FontWeightAsian,
StyleTextPropertiesElement.FontWeightComplex,
StyleTextPropertiesElement.LanguageAsian,
StyleTextPropertiesElement.LanguageComplex,
StyleTextPropertiesElement.LetterKerning,
StyleTextPropertiesElement.RfcLanguageTag,
StyleTextPropertiesElement.RfcLanguageTagAsian,
StyleTextPropertiesElement.RfcLanguageTagComplex,
StyleTextPropertiesElement.ScriptAsian,
StyleTextPropertiesElement.ScriptComplex,
StyleTextPropertiesElement.ScriptType,
StyleTextPropertiesElement.TextBlinking,
StyleTextPropertiesElement.TextCombine,
StyleTextPropertiesElement.TextCombineEndChar,
StyleTextPropertiesElement.TextCombineStartChar,
StyleTextPropertiesElement.TextEmphasize,
StyleTextPropertiesElement.TextLineThroughColor,
StyleTextPropertiesElement.TextLineThroughMode,
StyleTextPropertiesElement.TextLineThroughStyle,
StyleTextPropertiesElement.TextLineThroughText,
StyleTextPropertiesElement.TextLineThroughTextStyle,
StyleTextPropertiesElement.TextLineThroughType,
StyleTextPropertiesElement.TextLineThroughWidth,
StyleTextPropertiesElement.TextOutline,
StyleTextPropertiesElement.TextOverlineColor,
StyleTextPropertiesElement.TextOverlineMode,
StyleTextPropertiesElement.TextOverlineStyle,
StyleTextPropertiesElement.TextOverlineType,
StyleTextPropertiesElement.TextOverlineWidth,
StyleTextPropertiesElement.TextPosition,
StyleTextPropertiesElement.TextRotationAngle,
StyleTextPropertiesElement.TextRotationScale,
StyleTextPropertiesElement.TextScale,
StyleTextPropertiesElement.TextUnderlineColor,
StyleTextPropertiesElement.TextUnderlineMode,
StyleTextPropertiesElement.TextUnderlineStyle,
StyleTextPropertiesElement.TextUnderlineType,
StyleTextPropertiesElement.TextUnderlineWidth,
StyleTextPropertiesElement.UseWindowFontColor,
StyleTextPropertiesElement.Condition,
StyleTextPropertiesElement.Display,
StyleTextPropertiesElement.Display,
StyleTextPropertiesElement.Display,
});
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy