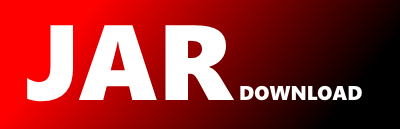
org.apache.olingo.ext.proxy.commons.InlineEntitySetInvocationHandler Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of odata-client-proxy Show documentation
Show all versions of odata-client-proxy Show documentation
Java client API for OData services: Proxy.
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package org.apache.olingo.ext.proxy.commons;
import java.io.Serializable;
import java.lang.reflect.Method;
import java.lang.reflect.Proxy;
import java.net.URI;
import java.util.Collection;
import java.util.List;
import java.util.concurrent.Callable;
import java.util.concurrent.Future;
import org.apache.olingo.client.api.uri.URIBuilder;
import org.apache.olingo.commons.api.edm.FullQualifiedName;
import org.apache.olingo.ext.proxy.AbstractService;
import org.apache.olingo.ext.proxy.api.AbstractEntitySet;
import org.apache.olingo.ext.proxy.api.AbstractSingleton;
import org.apache.olingo.ext.proxy.api.EntityCollection;
import org.apache.olingo.ext.proxy.api.EntityType;
import org.apache.olingo.ext.proxy.api.Search;
import org.apache.olingo.ext.proxy.utils.ClassUtils;
public class InlineEntitySetInvocationHandler<
T extends EntityType>, KEY extends Serializable, EC extends EntityCollection>
extends AbstractEntityCollectionInvocationHandler
implements AbstractEntitySet {
private static final long serialVersionUID = 2629912294765040027L;
@SuppressWarnings({ "rawtypes", "unchecked" })
public static InlineEntitySetInvocationHandler getInstance(final Class> ref, final AbstractService> service,
final URI uri,
final List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy