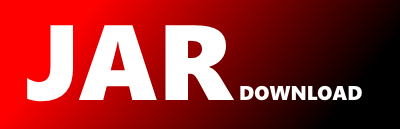
org.apache.openejb.jee.WebApp Maven / Gradle / Ivy
/**
*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.openejb.jee;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlAttribute;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlID;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlTransient;
import javax.xml.bind.annotation.XmlType;
import javax.xml.bind.annotation.adapters.CollapsedStringAdapter;
import javax.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
import java.util.ArrayList;
import java.util.List;
import java.util.Collection;
import java.util.Map;
@XmlRootElement(name = "web-app")
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "web-appType", propOrder = {
"descriptions",
"displayNames",
"icon",
"distributable",
"contextParam",
"filter",
"filterMapping",
"listener",
"servlet",
"servletMapping",
"sessionConfig",
"mimeMapping",
"welcomeFileList",
"errorPage",
"jspConfig",
"securityConstraint",
"loginConfig",
"securityRole",
"localeEncodingMappingList",
"envEntry",
"ejbRef",
"ejbLocalRef",
"serviceRef",
"resourceRef",
"resourceEnvRef",
"messageDestinationRef",
"persistenceContextRef",
"persistenceUnitRef",
"postConstruct",
"preDestroy",
"messageDestination"
})
public class WebApp implements JndiConsumer {
@XmlTransient
private String contextRoot;
@XmlTransient
protected TextMap description = new TextMap();
@XmlTransient
protected TextMap displayName = new TextMap();
@XmlElement(name = "icon", required = true)
protected LocalCollection icon = new LocalCollection();
protected List distributable;
@XmlElement(name = "context-param")
protected List contextParam;
protected List filter;
@XmlElement(name = "filter-mapping")
protected List filterMapping;
protected List listener;
protected List servlet;
@XmlElement(name = "servlet-mapping")
protected List servletMapping;
@XmlElement(name = "session-config")
protected List sessionConfig;
@XmlElement(name = "mime-mapping")
protected List mimeMapping;
@XmlElement(name = "welcome-file-list")
protected List welcomeFileList;
@XmlElement(name = "error-page")
protected List errorPage;
@XmlElement(name = "jsp-config")
protected List jspConfig;
@XmlElement(name = "security-constraint")
protected List securityConstraint;
@XmlElement(name = "login-config")
protected List loginConfig;
@XmlElement(name = "security-role")
protected List securityRole;
@XmlElement(name = "locale-encoding-mapping-list")
protected List localeEncodingMappingList;
@XmlElement(name = "env-entry", required = true)
protected KeyedCollection envEntry;
@XmlElement(name = "ejb-ref", required = true)
protected KeyedCollection ejbRef;
@XmlElement(name = "ejb-local-ref", required = true)
protected KeyedCollection ejbLocalRef;
@XmlElement(name = "service-ref", required = true)
protected KeyedCollection serviceRef;
@XmlElement(name = "resource-ref", required = true)
protected KeyedCollection resourceRef;
@XmlElement(name = "resource-env-ref", required = true)
protected KeyedCollection resourceEnvRef;
@XmlElement(name = "message-destination-ref", required = true)
protected KeyedCollection messageDestinationRef;
@XmlElement(name = "persistence-context-ref", required = true)
protected KeyedCollection persistenceContextRef;
@XmlElement(name = "persistence-unit-ref", required = true)
protected KeyedCollection persistenceUnitRef;
@XmlElement(name = "post-construct", required = true)
protected List postConstruct;
@XmlElement(name = "pre-destroy", required = true)
protected List preDestroy;
@XmlElement(name = "message-destination", required = true)
protected List messageDestination;
@XmlAttribute
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
@XmlID
protected String id;
@XmlAttribute(name = "metadata-complete")
protected Boolean metadataComplete;
@XmlAttribute(required = true)
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
protected String version;
public String getJndiConsumerName() {
return contextRoot;
}
public String getContextRoot() {
return contextRoot;
}
public void setContextRoot(String contextRoot) {
this.contextRoot = contextRoot;
}
@XmlElement(name = "description", required = true)
public Text[] getDescriptions() {
return description.toArray();
}
public void setDescriptions(Text[] text) {
description.set(text);
}
public String getDescription() {
return description.get();
}
@XmlElement(name = "display-name", required = true)
public Text[] getDisplayNames() {
return displayName.toArray();
}
public void setDisplayNames(Text[] text) {
displayName.set(text);
}
public String getDisplayName() {
return displayName.get();
}
public Collection getIcons() {
if (icon == null) {
icon = new LocalCollection();
}
return icon;
}
public Map getIconMap() {
if (icon == null) {
icon = new LocalCollection();
}
return icon.toMap();
}
public Icon getIcon() {
return icon.getLocal();
}
public List getDistributable() {
if (distributable == null) {
distributable = new ArrayList();
}
return this.distributable;
}
public List getContextParam() {
if (contextParam == null) {
contextParam = new ArrayList();
}
return this.contextParam;
}
public List getFilter() {
if (filter == null) {
filter = new ArrayList();
}
return this.filter;
}
public List getFilterMapping() {
if (filterMapping == null) {
filterMapping = new ArrayList();
}
return this.filterMapping;
}
public List getListener() {
if (listener == null) {
listener = new ArrayList();
}
return this.listener;
}
public List getServlet() {
if (servlet == null) {
servlet = new ArrayList();
}
return this.servlet;
}
public List getServletMapping() {
if (servletMapping == null) {
servletMapping = new ArrayList();
}
return this.servletMapping;
}
public List getSessionConfig() {
if (sessionConfig == null) {
sessionConfig = new ArrayList();
}
return this.sessionConfig;
}
public List getMimeMapping() {
if (mimeMapping == null) {
mimeMapping = new ArrayList();
}
return this.mimeMapping;
}
public List getWelcomeFileList() {
if (welcomeFileList == null) {
welcomeFileList = new ArrayList();
}
return this.welcomeFileList;
}
public List getErrorPage() {
if (errorPage == null) {
errorPage = new ArrayList();
}
return this.errorPage;
}
public List getJspConfig() {
if (jspConfig == null) {
jspConfig = new ArrayList();
}
return this.jspConfig;
}
public List getSecurityConstraint() {
if (securityConstraint == null) {
securityConstraint = new ArrayList();
}
return this.securityConstraint;
}
public List getLoginConfig() {
if (loginConfig == null) {
loginConfig = new ArrayList();
}
return this.loginConfig;
}
public List getSecurityRole() {
if (securityRole == null) {
securityRole = new ArrayList();
}
return this.securityRole;
}
public List getLocaleEncodingMappingList() {
if (localeEncodingMappingList == null) {
localeEncodingMappingList = new ArrayList();
}
return this.localeEncodingMappingList;
}
public Collection getEnvEntry() {
if (envEntry == null) {
envEntry = new KeyedCollection();
}
return this.envEntry;
}
public Map getEnvEntryMap() {
if (envEntry == null) {
envEntry = new KeyedCollection();
}
return this.envEntry.toMap();
}
public Collection getEjbRef() {
if (ejbRef == null) {
ejbRef = new KeyedCollection();
}
return this.ejbRef;
}
public Map getEjbRefMap() {
if (ejbRef == null) {
ejbRef = new KeyedCollection();
}
return this.ejbRef.toMap();
}
public Collection getEjbLocalRef() {
if (ejbLocalRef == null) {
ejbLocalRef = new KeyedCollection();
}
return this.ejbLocalRef;
}
public Map getEjbLocalRefMap() {
if (ejbLocalRef == null) {
ejbLocalRef = new KeyedCollection();
}
return this.ejbLocalRef.toMap();
}
public Collection getServiceRef() {
if (serviceRef == null) {
serviceRef = new KeyedCollection();
}
return this.serviceRef;
}
public Map getServiceRefMap() {
if (serviceRef == null) {
serviceRef = new KeyedCollection();
}
return this.serviceRef.toMap();
}
public Collection getResourceRef() {
if (resourceRef == null) {
resourceRef = new KeyedCollection();
}
return this.resourceRef;
}
public Map getResourceRefMap() {
if (resourceRef == null) {
resourceRef = new KeyedCollection();
}
return this.resourceRef.toMap();
}
public Collection getResourceEnvRef() {
if (resourceEnvRef == null) {
resourceEnvRef = new KeyedCollection();
}
return this.resourceEnvRef;
}
public Map getResourceEnvRefMap() {
if (resourceEnvRef == null) {
resourceEnvRef = new KeyedCollection();
}
return this.resourceEnvRef.toMap();
}
public Collection getMessageDestinationRef() {
if (messageDestinationRef == null) {
messageDestinationRef = new KeyedCollection();
}
return this.messageDestinationRef;
}
public Map getMessageDestinationRefMap() {
if (messageDestinationRef == null) {
messageDestinationRef = new KeyedCollection();
}
return this.messageDestinationRef.toMap();
}
public Collection getPersistenceContextRef() {
if (persistenceContextRef == null) {
persistenceContextRef = new KeyedCollection();
}
return this.persistenceContextRef;
}
public Map getPersistenceContextRefMap() {
if (persistenceContextRef == null) {
persistenceContextRef = new KeyedCollection();
}
return this.persistenceContextRef.toMap();
}
public Collection getPersistenceUnitRef() {
if (persistenceUnitRef == null) {
persistenceUnitRef = new KeyedCollection();
}
return this.persistenceUnitRef;
}
public Map getPersistenceUnitRefMap() {
if (persistenceUnitRef == null) {
persistenceUnitRef = new KeyedCollection();
}
return this.persistenceUnitRef.toMap();
}
public List getPostConstruct() {
if (postConstruct == null) {
postConstruct = new ArrayList();
}
return this.postConstruct;
}
public List getPreDestroy() {
if (preDestroy == null) {
preDestroy = new ArrayList();
}
return this.preDestroy;
}
public List getMessageDestination() {
if (messageDestination == null) {
messageDestination = new ArrayList();
}
return this.messageDestination;
}
public String getId() {
return id;
}
public void setId(String value) {
this.id = value;
}
public Boolean isMetadataComplete() {
return metadataComplete != null && metadataComplete;
}
public void setMetadataComplete(Boolean value) {
this.metadataComplete = value;
}
public String getVersion() {
return version;
}
public void setVersion(String value) {
this.version = value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy