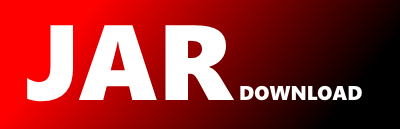
org.apache.openejb.config.ConfigurationFactory Maven / Gradle / Ivy
The newest version!
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.openejb.config;
import org.apache.openejb.Extensions;
import org.apache.openejb.OpenEJBException;
import org.apache.openejb.Vendor;
import org.apache.openejb.api.Proxy;
import org.apache.openejb.assembler.classic.AppInfo;
import org.apache.openejb.assembler.classic.Assembler;
import org.apache.openejb.assembler.classic.BmpEntityContainerInfo;
import org.apache.openejb.assembler.classic.ClientInfo;
import org.apache.openejb.assembler.classic.CmpEntityContainerInfo;
import org.apache.openejb.assembler.classic.ConnectionManagerInfo;
import org.apache.openejb.assembler.classic.ConnectorInfo;
import org.apache.openejb.assembler.classic.ContainerInfo;
import org.apache.openejb.assembler.classic.ContainerSystemInfo;
import org.apache.openejb.assembler.classic.DeploymentExceptionManager;
import org.apache.openejb.assembler.classic.EjbJarInfo;
import org.apache.openejb.assembler.classic.FacilitiesInfo;
import org.apache.openejb.assembler.classic.HandlerChainInfo;
import org.apache.openejb.assembler.classic.HandlerInfo;
import org.apache.openejb.assembler.classic.JndiContextInfo;
import org.apache.openejb.assembler.classic.ManagedContainerInfo;
import org.apache.openejb.assembler.classic.MdbContainerInfo;
import org.apache.openejb.assembler.classic.OpenEjbConfiguration;
import org.apache.openejb.assembler.classic.OpenEjbConfigurationFactory;
import org.apache.openejb.assembler.classic.ProxyFactoryInfo;
import org.apache.openejb.assembler.classic.ResourceInfo;
import org.apache.openejb.assembler.classic.SecurityServiceInfo;
import org.apache.openejb.assembler.classic.ServiceInfo;
import org.apache.openejb.assembler.classic.SingletonSessionContainerInfo;
import org.apache.openejb.assembler.classic.StatefulSessionContainerInfo;
import org.apache.openejb.assembler.classic.StatelessSessionContainerInfo;
import org.apache.openejb.assembler.classic.TransactionServiceInfo;
import org.apache.openejb.assembler.classic.WebAppInfo;
import org.apache.openejb.component.ClassLoaderEnricher;
import org.apache.openejb.config.sys.AbstractService;
import org.apache.openejb.config.sys.AdditionalDeployments;
import org.apache.openejb.config.sys.ConnectionManager;
import org.apache.openejb.config.sys.Container;
import org.apache.openejb.config.sys.Deployments;
import org.apache.openejb.config.sys.JaxbOpenejb;
import org.apache.openejb.config.sys.JndiProvider;
import org.apache.openejb.config.sys.Openejb;
import org.apache.openejb.config.sys.ProxyFactory;
import org.apache.openejb.config.sys.Resource;
import org.apache.openejb.config.sys.SecurityService;
import org.apache.openejb.config.sys.Service;
import org.apache.openejb.config.sys.ServiceProvider;
import org.apache.openejb.config.sys.TransactionManager;
import org.apache.openejb.core.ParentClassLoaderFinder;
import org.apache.openejb.jee.Application;
import org.apache.openejb.jee.EjbJar;
import org.apache.openejb.jee.EnterpriseBean;
import org.apache.openejb.jee.EnvEntry;
import org.apache.openejb.jee.Handler;
import org.apache.openejb.jee.HandlerChain;
import org.apache.openejb.jee.HandlerChains;
import org.apache.openejb.jee.ParamValue;
import org.apache.openejb.jee.SessionBean;
import org.apache.openejb.loader.FileUtils;
import org.apache.openejb.loader.Files;
import org.apache.openejb.loader.IO;
import org.apache.openejb.loader.Options;
import org.apache.openejb.loader.ProvisioningUtil;
import org.apache.openejb.loader.SystemInstance;
import org.apache.openejb.monitoring.LocalMBeanServer;
import org.apache.openejb.resource.jdbc.DataSourceFactory;
import org.apache.openejb.resource.jdbc.pool.DataSourceCreator;
import org.apache.openejb.resource.jdbc.pool.DefaultDataSourceCreator;
import org.apache.openejb.util.LogCategory;
import org.apache.openejb.util.Logger;
import org.apache.openejb.util.Messages;
import org.apache.openejb.util.PropertyPlaceHolderHelper;
import org.apache.openejb.util.References;
import org.apache.openejb.util.SuperProperties;
import org.apache.openejb.util.URISupport;
import org.apache.openejb.util.URLs;
import org.apache.openejb.util.classloader.URLClassLoaderFirst;
import org.apache.openejb.util.proxy.QueryProxy;
import org.apache.xbean.finder.MetaAnnotatedClass;
import org.apache.xbean.recipe.ObjectRecipe;
import org.apache.xbean.recipe.Option;
import javax.ejb.embeddable.EJBContainer;
import java.io.File;
import java.io.IOException;
import java.io.InputStream;
import java.net.MalformedURLException;
import java.net.URI;
import java.net.URISyntaxException;
import java.net.URL;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Properties;
import java.util.Set;
import static org.apache.openejb.config.DeploymentsResolver.DEPLOYMENTS_CLASSPATH_PROPERTY;
import static org.apache.openejb.config.ServiceUtils.implies;
@SuppressWarnings("UnusedDeclaration")
public class ConfigurationFactory implements OpenEjbConfigurationFactory {
public static final String OPENEJB_JDBC_DATASOURCE_CREATOR = "openejb.jdbc.datasource-creator";
public static final String ADDITIONAL_DEPLOYMENTS = "conf/deployments.xml";
static final String CONFIGURATION_PROPERTY = "openejb.configuration";
static final String CONF_FILE_PROPERTY = "openejb.conf.file";
private static final String DEBUGGABLE_VM_HACKERY_PROPERTY = "openejb.debuggable-vm-hackery";
protected static final String VALIDATION_SKIP_PROPERTY = "openejb.validation.skip";
private static final Logger logger = Logger.getInstance(LogCategory.OPENEJB_STARTUP_CONFIG, ConfigurationFactory.class);
private static final Messages messages = new Messages(ConfigurationFactory.class);
private static final String IGNORE_DEFAULT_VALUES_PROP = "IgnoreDefaultValues";
private static final boolean WSDL4J_AVAILABLE = exists("javax.wsdl.xml.WSDLLocator");
private String configLocation;
private OpenEjbConfiguration sys;
private Openejb openejb;
private final DynamicDeployer deployer;
private final DeploymentLoader deploymentLoader;
private final boolean offline;
private final boolean serviceTypeIsAdjustable; // offline is a bit different from this and offline could be off and this on
private static final String CLASSPATH_AS_EAR = "openejb.deployments.classpath.ear";
static final String WEBSERVICES_ENABLED = "openejb.webservices.enabled";
static final String OFFLINE_PROPERTY = "openejb.offline";
public ConfigurationFactory() {
this(!shouldAutoDeploy());
}
private static boolean exists(final String s) {
try {
ConfigurationFactory.class.getClassLoader().loadClass(s);
return true;
} catch (final ClassNotFoundException e) {
return false;
} catch (final NoClassDefFoundError e) {
return false;
}
}
private static boolean shouldAutoDeploy() {
final Options options = SystemInstance.get().getOptions();
final boolean b = options.get(ConfigurationFactory.OFFLINE_PROPERTY, false);
return options.get("tomee.autoconfig", !b);
}
public ConfigurationFactory(final boolean offline) {
this(offline, (DynamicDeployer) null);
}
public ConfigurationFactory(final boolean offline, final DynamicDeployer preAutoConfigDeployer) {
this.offline = offline;
this.serviceTypeIsAdjustable = SystemInstance.get().getOptions().get("openejb.service-type-adjustement", true);
this.deploymentLoader = new DeploymentLoader();
LocalMBeanServer.reset();
final Options options = SystemInstance.get().getOptions();
if (SystemInstance.get().getComponent(DataSourceCreator.class) == null) {
final String creator = options.get(OPENEJB_JDBC_DATASOURCE_CREATOR, (String) null);
if (creator == null) {
SystemInstance.get().setComponent(DataSourceCreator.class, new DefaultDataSourceCreator());
} else {
try {
SystemInstance.get().setComponent(DataSourceCreator.class, DataSourceFactory.creator(creator, false));
} catch (final Exception e) {
logger.error("can't load " + creator + " will use the default creator", e);
SystemInstance.get().setComponent(DataSourceCreator.class, new DefaultDataSourceCreator());
}
}
}
if (SystemInstance.get().getComponent(ClassLoaderEnricher.class) == null) {
SystemInstance.get().setComponent(ClassLoaderEnricher.class, new ClassLoaderEnricher());
}
SystemInstance.get().setComponent(ConfigurationFactory.class, this);
// annotation deployer encapsulate some logic, to be able to push to it some config
// we give the ability here to get the internal deployer to push the config values
final AnnotationDeployer annotationDeployer = new AnnotationDeployer();
final BeanProperties beanProperties = new BeanProperties();
final AppContextConfigDeployer appContextConfigDeployer = new AppContextConfigDeployer(annotationDeployer.getEnvEntriesPropertiesDeployer(), beanProperties);
final Chain chain = new Chain();
chain.add(new SystemPropertiesOverride());
chain.add(new GeneratedClientModules.Add());
chain.add(new ReadDescriptors());
chain.add(appContextConfigDeployer);
chain.add(new ApplicationProperties());
chain.add(new ModuleProperties());
chain.add(new LegacyProcessor());
chain.add(annotationDeployer);
chain.add(beanProperties);
chain.add(new ProxyBeanClassUpdate());
chain.add(new GeneratedClientModules.Prune());
chain.add(new ClearEmptyMappedName());
//START SNIPPET: code
if (!options.get(VALIDATION_SKIP_PROPERTY, false)) {
chain.add(new ValidateModules());
} else {
DeploymentLoader.logger.info("validationDisabled", VALIDATION_SKIP_PROPERTY);
}
//END SNIPPET: code
chain.add(new InitEjbDeployments());
if (options.get(DEBUGGABLE_VM_HACKERY_PROPERTY, false)) {
chain.add(new DebuggableVmHackery());
}
if (options.get(WEBSERVICES_ENABLED, true) && WSDL4J_AVAILABLE) {
chain.add(new WsDeployer());
} else {
chain.add(new RemoveWebServices());
}
chain.add(new CmpJpaConversion());
// By default all vendor support is enabled
final Set support = SystemInstance.get().getOptions().getAll("openejb.vendor.config", Vendor.values());
if (support.contains(Vendor.GERONIMO) || SystemInstance.get().hasProperty("openejb.geronimo")) {
chain.add(new OpenEjb2Conversion());
}
if (support.contains(Vendor.GLASSFISH)) {
chain.add(new SunConversion());
}
if (support.contains(Vendor.WEBLOGIC)) {
chain.add(new WlsConversion());
}
if (SystemInstance.get().hasProperty("openejb.geronimo")) {
// must be after CmpJpaConversion since it adds new persistence-context-refs
chain.add(new GeronimoMappedName());
}
if (null != preAutoConfigDeployer) {
chain.add(preAutoConfigDeployer);
}
chain.add(new ConvertDataSourceDefinitions());
chain.add(new CleanEnvEntries());
chain.add(new LinkBuiltInTypes());
if (offline) {
final AutoConfig autoConfig = new AutoConfig(this);
autoConfig.autoCreateResources(false);
autoConfig.autoCreateContainers(false);
chain.add(autoConfig);
} else {
chain.add(new AutoConfig(this));
}
chain.add(new ApplyOpenejbJar());
chain.add(new MappedNameBuilder());
chain.add(new ActivationConfigPropertyOverride());
chain.add(new OutputGeneratedDescriptors());
// chain.add(new MergeWebappJndiContext());
this.deployer = chain;
}
public ConfigurationFactory(final boolean offline, final OpenEjbConfiguration configuration) {
this(offline, (DynamicDeployer) null, configuration);
}
public ConfigurationFactory(final boolean offline,
final DynamicDeployer preAutoConfigDeployer,
final OpenEjbConfiguration configuration) {
this(offline, preAutoConfigDeployer);
sys = configuration;
}
public ConfigurationFactory(final boolean offline, final Chain deployerChain, final OpenEjbConfiguration configuration) {
this.offline = offline;
this.deploymentLoader = new DeploymentLoader();
this.deployer = deployerChain;
this.sys = configuration;
this.serviceTypeIsAdjustable = true;
}
public static List toHandlerChainInfo(final HandlerChains chains) {
final List handlerChains = new ArrayList();
if (chains == null) {
return handlerChains;
}
for (final HandlerChain handlerChain : chains.getHandlerChain()) {
final HandlerChainInfo handlerChainInfo = new HandlerChainInfo();
handlerChainInfo.serviceNamePattern = handlerChain.getServiceNamePattern();
handlerChainInfo.portNamePattern = handlerChain.getPortNamePattern();
handlerChainInfo.protocolBindings.addAll(handlerChain.getProtocolBindings());
for (final Handler handler : handlerChain.getHandler()) {
final HandlerInfo handlerInfo = new HandlerInfo();
handlerInfo.handlerName = handler.getHandlerName();
handlerInfo.handlerClass = handler.getHandlerClass();
handlerInfo.soapHeaders.addAll(handler.getSoapHeader());
handlerInfo.soapRoles.addAll(handler.getSoapRole());
for (final ParamValue param : handler.getInitParam()) {
handlerInfo.initParams.setProperty(param.getParamName(), param.getParamValue());
}
handlerChainInfo.handlers.add(handlerInfo);
}
handlerChains.add(handlerChainInfo);
}
return handlerChains;
}
public static class ProxyBeanClassUpdate implements DynamicDeployer {
@SuppressWarnings("unchecked")
@Override
public AppModule deploy(final AppModule appModule) throws OpenEJBException {
for (final EjbModule module : appModule.getEjbModules()) {
for (final EnterpriseBean eb : module.getEjbJar().getEnterpriseBeans()) {
if (!(eb instanceof SessionBean)) {
continue;
}
final SessionBean bean = (SessionBean) eb;
final Class> ejbClass;
try {
ejbClass = module.getClassLoader().loadClass(bean.getEjbClass());
} catch (final ClassNotFoundException e) {
logger.warning("can't load " + bean.getEjbClass());
continue;
}
final Class> proxyClass;
if (ejbClass.isInterface()) { // dynamic proxy implementation
bean.setLocal(ejbClass.getName());
final Proxy proxyAnnotation = (Proxy) new MetaAnnotatedClass(ejbClass).getAnnotation(Proxy.class);
if (proxyAnnotation != null) {
proxyClass = proxyAnnotation.value();
} else {
proxyClass = QueryProxy.class;
}
bean.setProxy(proxyClass.getName());
} else {
continue;
}
for (final EnvEntry entry : bean.getEnvEntry()) {
if ("java:comp/env/implementingInterfaceClass".equals(entry.getName())) {
entry.setEnvEntryValue(ejbClass.getName());
}
}
}
}
return appModule;
}
}
public static class Chain implements DynamicDeployer {
private final List chain = new ArrayList();
public boolean add(final DynamicDeployer o) {
return chain.add(o);
}
@Override
public AppModule deploy(AppModule appModule) throws OpenEJBException {
for (final DynamicDeployer deployer : chain) {
appModule = deployer.deploy(appModule);
}
return appModule;
}
}
@Override
public void init(final Properties props) throws OpenEJBException {
configLocation = props.getProperty(CONF_FILE_PROPERTY);
if (configLocation == null) {
configLocation = props.getProperty(CONFIGURATION_PROPERTY);
}
configLocation = ConfigUtils.searchForConfiguration(configLocation);
if (configLocation != null) {
logger.info("openejb configuration file is '" + configLocation + "'");
props.setProperty(CONFIGURATION_PROPERTY, configLocation);
}
}
protected void install(final ContainerInfo serviceInfo) throws OpenEJBException {
if (sys != null) {
sys.containerSystem.containers.add(serviceInfo);
} else if (!offline) {
final Assembler assembler = SystemInstance.get().getComponent(Assembler.class);
assembler.createContainer(serviceInfo);
}
}
protected void install(final ResourceInfo serviceInfo) throws OpenEJBException {
if (sys != null) {
sys.facilities.resources.add(serviceInfo);
} else if (!offline) {
final Assembler assembler = SystemInstance.get().getComponent(Assembler.class);
assembler.createResource(serviceInfo);
}
}
public OpenEjbConfiguration getOpenEjbConfiguration(final File configuartionFile) throws OpenEJBException {
if (configuartionFile != null) {
return getOpenEjbConfiguration(JaxbOpenejb.readConfig(configuartionFile.getAbsolutePath()));
} else {
return getOpenEjbConfiguration((Openejb) null);
}
}
public OpenEjbConfiguration getOpenEjbConfiguration(final Openejb providedConf) throws OpenEJBException {
if (sys != null) {
return sys;
}
if (providedConf != null) {
openejb = providedConf;
} else if (configLocation != null) {
openejb = JaxbOpenejb.readConfig(configLocation);
} else {
openejb = JaxbOpenejb.createOpenejb();
}
loadPropertiesDeclaredConfiguration(openejb);
sys = new OpenEjbConfiguration();
sys.containerSystem = new ContainerSystemInfo();
sys.facilities = new FacilitiesInfo();
// listener + some config can be defined as service
for (final Service service : openejb.getServices()) {
final ServiceInfo info = configureService(service, ServiceInfo.class);
sys.facilities.services.add(info);
}
for (final JndiProvider provider : openejb.getJndiProvider()) {
final JndiContextInfo info = configureService(provider, JndiContextInfo.class);
sys.facilities.remoteJndiContexts.add(info);
}
sys.facilities.securityService = configureService(openejb.getSecurityService(), SecurityServiceInfo.class);
sys.facilities.transactionService = configureService(openejb.getTransactionManager(), TransactionServiceInfo.class);
List resources = new ArrayList();
for (final Resource resource : openejb.getResource()) {
final ResourceInfo resourceInfo = configureService(resource, ResourceInfo.class);
resources.add(resourceInfo);
}
resources = sort(resources, null);
sys.facilities.resources.addAll(resources);
// ConnectionManagerInfo service = configureService(openejb.getConnectionManager(), ConnectionManagerInfo.class);
// sys.facilities.connectionManagers.add(service);
if (openejb.getProxyFactory() != null) {
sys.facilities.intraVmServer = configureService(openejb.getProxyFactory(), ProxyFactoryInfo.class);
}
for (final Container declaration : openejb.getContainer()) {
final ContainerInfo info = createContainerInfo(declaration);
sys.containerSystem.containers.add(info);
}
final List declaredApps = getDeclaredApps();
for (final File jarFile : declaredApps) {
try {
final AppInfo appInfo = configureApplication(jarFile);
sys.containerSystem.applications.add(appInfo);
} catch (final OpenEJBException alreadyHandled) {
final DeploymentExceptionManager exceptionManager = SystemInstance.get().getComponent(DeploymentExceptionManager.class);
if (exceptionManager != null) {
exceptionManager.pushDelpoymentException(alreadyHandled);
}
}
}
final boolean embedded = SystemInstance.get().hasProperty(EJBContainer.class.getName());
final Options options = SystemInstance.get().getOptions();
if (options.get("openejb.system.apps", false)) {
try {
final AppInfo appInfo = configureApplication(new AppModule(SystemApps.getSystemModule()));
// they doesn't use CDI so no need to activate it
// 1) will be faster
// 2) will let embedded containers (tomee-embedded mainly) not be noised by it in our singleton service
appInfo.properties.put("openejb.cdi.activated", "false");
sys.containerSystem.applications.add(appInfo);
} catch (final OpenEJBException e) {
logger.error("Unable to load the system applications.", e);
}
} else if (options.get(DEPLOYMENTS_CLASSPATH_PROPERTY, !embedded)) {
final ClassLoader classLoader = Thread.currentThread().getContextClassLoader();
final ArrayList jarFiles = getModulesFromClassPath(declaredApps, classLoader);
final String appId = "classpath.ear";
final boolean classpathAsEar = options.get(CLASSPATH_AS_EAR, true);
try {
if (classpathAsEar && !jarFiles.isEmpty()) {
final AppInfo appInfo = configureApplication(classLoader, appId, jarFiles);
sys.containerSystem.applications.add(appInfo);
} else {
for (final File jarFile : jarFiles) {
final AppInfo appInfo = configureApplication(jarFile);
sys.containerSystem.applications.add(appInfo);
}
}
if (jarFiles.size() == 0) {
logger.warning("config.noModulesFoundToDeploy");
}
} catch (final OpenEJBException alreadyHandled) {
logger.debug("config.alreadyHandled");
}
}
for (final Deployments deployments : openejb.getDeployments()) {
if (deployments.isAutoDeploy()) {
if (deployments.getDir() != null) {
sys.containerSystem.autoDeploy.add(deployments.getDir());
}
}
}
final OpenEjbConfiguration finished = sys;
sys = null;
openejb = null;
return finished;
}
/**
* Main loop that gets executed when OpenEJB starts up Reads config files and produces the basic "AST" the assembler needs to actually build the contianer system
*
* This method is called by the Assembler once at startup.
*
* @return OpenEjbConfiguration
* @throws OpenEJBException
*/
@Override
public OpenEjbConfiguration getOpenEjbConfiguration() throws OpenEJBException {
return getOpenEjbConfiguration((Openejb) null);
}
private List getDeclaredApps() {
// make a copy of the list because we update it
final List deployments = new ArrayList();
if (openejb != null) {
deployments.addAll(openejb.getDeployments());
}
Collection additionalDeploymentsList = Collections.emptyList();
try {
final File additionalDeploymentFile = SystemInstance.get().getBase().getFile(ADDITIONAL_DEPLOYMENTS, false);
if (additionalDeploymentFile.exists()) {
InputStream fis = null;
try {
fis = IO.read(additionalDeploymentFile);
final AdditionalDeployments additionalDeployments = JaxbOpenejb.unmarshal(AdditionalDeployments.class, fis);
additionalDeploymentsList = additionalDeployments.getDeployments();
} catch (final Exception e) {
logger.error("can't read " + ADDITIONAL_DEPLOYMENTS, e);
} finally {
IO.close(fis);
}
}
} catch (final Exception e) {
logger.info("No additional deployments found: " + e);
}
// resolve jar locations ////////////////////////////////////// BEGIN ///////
final FileUtils base = SystemInstance.get().getBase();
final List autoDeploy = new ArrayList();
final List declaredAppsUrls = new ArrayList();
for (final Deployments deployment : deployments) {
try {
DeploymentsResolver.loadFrom(deployment, base, declaredAppsUrls);
if (deployment.isAutoDeploy()) {
autoDeploy.add(deployment);
}
} catch (final SecurityException se) {
logger.warning("Security check failed on deployment: " + deployment.getFile(), se);
}
}
for (final Deployments additionalDep : additionalDeploymentsList) {
if (additionalDep.getFile() != null) {
declaredAppsUrls.add(Files.path(base.getDirectory().getAbsoluteFile(), additionalDep.getFile()));
} else if (additionalDep.getDir() != null) {
declaredAppsUrls.add(Files.path(base.getDirectory().getAbsoluteFile(), additionalDep.getDir()));
}
if (additionalDep.isAutoDeploy()) {
autoDeploy.add(additionalDep);
}
}
if (autoDeploy.size() > 0) {
final AutoDeployer autoDeployer = new AutoDeployer(this, autoDeploy);
SystemInstance.get().setComponent(AutoDeployer.class, autoDeployer);
SystemInstance.get().addObserver(autoDeployer);
}
return declaredAppsUrls;
}
public ArrayList getModulesFromClassPath(final List declaredApps, final ClassLoader classLoader) {
final FileUtils base = SystemInstance.get().getBase();
final List classpathAppsUrls = new ArrayList();
DeploymentsResolver.loadFromClasspath(base, classpathAppsUrls, classLoader);
final ArrayList jarFiles = new ArrayList();
for (final URL path : classpathAppsUrls) {
final File file = URLs.toFile(path);
if (declaredApps != null && declaredApps.contains(file)) {
continue;
}
jarFiles.add(file);
}
return jarFiles;
}
public ContainerInfo createContainerInfo(final Container container) throws OpenEJBException {
final Class extends ContainerInfo> infoClass = getContainerInfoType(container.getType());
if (infoClass == null) {
throw new OpenEJBException(messages.format("unrecognizedContainerType", container.getType()));
}
return configureService(container, infoClass);
}
public static void loadPropertiesDeclaredConfiguration(final Openejb openejb) {
final Properties sysProps = new Properties(System.getProperties());
sysProps.putAll(SystemInstance.get().getProperties());
fillOpenEjb(openejb, sysProps);
}
public static void fillOpenEjb(final Openejb openejb, final Properties sysProps) {
for (final Map.Entry
© 2015 - 2025 Weber Informatics LLC | Privacy Policy