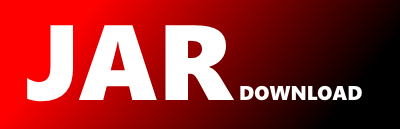
org.apache.openejb.jee.FacesApplication Maven / Gradle / Ivy
The newest version!
/**
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.openejb.jee;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlAttribute;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlID;
import javax.xml.bind.annotation.XmlSchemaType;
import javax.xml.bind.annotation.XmlType;
import javax.xml.bind.annotation.adapters.CollapsedStringAdapter;
import javax.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
import java.util.ArrayList;
import java.util.List;
/**
* web-facesconfig_2_0.xsd
*
* Java class for faces-config-applicationType complex type.
*
* The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="faces-config-applicationType">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <choice maxOccurs="unbounded" minOccurs="0">
* <element name="action-listener" type="{http://java.sun.com/xml/ns/javaee}fully-qualified-classType"/>
* <element name="default-render-kit-id" type="{http://java.sun.com/xml/ns/javaee}string"/>
* <element name="message-bundle" type="{http://java.sun.com/xml/ns/javaee}string"/>
* <element name="navigation-handler" type="{http://java.sun.com/xml/ns/javaee}fully-qualified-classType"/>
* <element name="view-handler" type="{http://java.sun.com/xml/ns/javaee}fully-qualified-classType"/>
* <element name="state-manager" type="{http://java.sun.com/xml/ns/javaee}fully-qualified-classType"/>
* <element name="el-resolver" type="{http://java.sun.com/xml/ns/javaee}fully-qualified-classType"/>
* <element name="property-resolver" type="{http://java.sun.com/xml/ns/javaee}fully-qualified-classType"/>
* <element name="variable-resolver" type="{http://java.sun.com/xml/ns/javaee}fully-qualified-classType"/>
* <element name="resource-handler" type="{http://java.sun.com/xml/ns/javaee}fully-qualified-classType"/>
* <element name="system-event-listener" type="{http://java.sun.com/xml/ns/javaee}faces-config-system-event-listenerType" maxOccurs="unbounded" minOccurs="0"/>
* <element name="locale-config" type="{http://java.sun.com/xml/ns/javaee}faces-config-locale-configType"/>
* <element name="resource-bundle" type="{http://java.sun.com/xml/ns/javaee}faces-config-application-resource-bundleType"/>
* <element name="application-extension" type="{http://java.sun.com/xml/ns/javaee}faces-config-application-extensionType" maxOccurs="unbounded" minOccurs="0"/>
* <element name="default-validators" type="{http://java.sun.com/xml/ns/javaee}faces-config-default-validatorsType"/>
* </choice>
* <attribute name="id" type="{http://www.w3.org/2001/XMLSchema}ID" />
* </restriction>
* </complexContent>
* </complexType>
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "faces-config-applicationType", propOrder = {
"actionListener",
"defaultRenderKitId",
"messageBundle",
"navigationHandler",
"viewHandler",
"stateManager",
"elResolver",
"propertyResolver",
"variableResolver",
"resourceHandler",
"systemEventListener",
"localeConfig",
"resourceBundle",
"applicationExtension",
"defaultValidators"
})
public class FacesApplication {
@XmlElement(name = "action-listener")
protected List actionListener;
@XmlElement(name = "default-render-kit-id")
protected List defaultRenderKitId;
@XmlElement(name = "message-bundle")
protected List messageBundle;
@XmlElement(name = "navigation-handler")
protected List navigationHandler;
@XmlElement(name = "view-handler")
protected List viewHandler;
@XmlElement(name = "state-manager")
protected List stateManager;
@XmlElement(name = "el-resolver")
protected List elResolver;
@XmlElement(name = "property-resolver")
protected List propertyResolver;
@XmlElement(name = "variable-resolver")
protected List variableResolver;
@XmlElement(name = "resource-handler")
protected List resourceHandler;
@XmlElement(name = "system-event-listener")
protected List systemEventListener;
@XmlElement(name = "locale-config")
protected List localeConfig;
@XmlElement(name = "resource-bundle", required = true)
protected FacesApplicationResourceBundle resourceBundle;
@XmlElement(name = "application-extension")
protected List applicationExtension;
@XmlElement(name = "default-validators")
protected List defaultValidators;
@XmlAttribute
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
@XmlID
@XmlSchemaType(name = "ID")
protected java.lang.String id;
/**
* Gets the value of the actionListener property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the actionListener property.
*
*
* For example, to add a new item, do as follows:
*
* getActionListener().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link java.lang.String }
*/
public List getActionListener() {
if (actionListener == null) {
actionListener = new ArrayList();
}
return this.actionListener;
}
/**
* Gets the value of the defaultRenderKitId property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the defaultRenderKitId property.
*
*
* For example, to add a new item, do as follows:
*
* getDefaultRenderKitId().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link java.lang.String }
*/
public List getDefaultRenderKitId() {
if (defaultRenderKitId == null) {
defaultRenderKitId = new ArrayList();
}
return this.defaultRenderKitId;
}
/**
* Gets the value of the messageBundle property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the messageBundle property.
*
*
* For example, to add a new item, do as follows:
*
* getMessageBundle().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link java.lang.String }
*/
public List getMessageBundle() {
if (messageBundle == null) {
messageBundle = new ArrayList();
}
return this.messageBundle;
}
/**
* Gets the value of the navigationHandler property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the navigationHandler property.
*
*
* For example, to add a new item, do as follows:
*
* getNavigationHandler().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link java.lang.String }
*/
public List getNavigationHandler() {
if (navigationHandler == null) {
navigationHandler = new ArrayList();
}
return this.navigationHandler;
}
/**
* Gets the value of the viewHandler property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the viewHandler property.
*
*
* For example, to add a new item, do as follows:
*
* getViewHandler().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link java.lang.String }
*/
public List getViewHandler() {
if (viewHandler == null) {
viewHandler = new ArrayList();
}
return this.viewHandler;
}
/**
* Gets the value of the stateManager property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the stateManager property.
*
*
* For example, to add a new item, do as follows:
*
* getStateManager().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link java.lang.String }
*/
public List getStateManager() {
if (stateManager == null) {
stateManager = new ArrayList();
}
return this.stateManager;
}
/**
* Gets the value of the elResolver property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the elResolver property.
*
*
* For example, to add a new item, do as follows:
*
* getElResolver().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link java.lang.String }
*/
public List getElResolver() {
if (elResolver == null) {
elResolver = new ArrayList();
}
return this.elResolver;
}
/**
* Gets the value of the propertyResolver property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the propertyResolver property.
*
*
* For example, to add a new item, do as follows:
*
* getPropertyResolver().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link java.lang.String }
*/
public List getPropertyResolver() {
if (propertyResolver == null) {
propertyResolver = new ArrayList();
}
return this.propertyResolver;
}
/**
* Gets the value of the variableResolver property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the variableResolver property.
*
*
* For example, to add a new item, do as follows:
*
* getVariableResolver().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link java.lang.String }
*/
public List getVariableResolver() {
if (variableResolver == null) {
variableResolver = new ArrayList();
}
return this.variableResolver;
}
public List getResourceHandler() {
if (resourceHandler == null) {
resourceHandler = new ArrayList();
}
return resourceHandler;
}
public List getSystemEventListener() {
if (systemEventListener == null) {
systemEventListener = new ArrayList();
}
return systemEventListener;
}
/**
* Gets the value of the localeConfig property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the localeConfig property.
*
*
* For example, to add a new item, do as follows:
*
* getLocaleConfig().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link FacesLocaleConfig }
*/
public List getLocaleConfig() {
if (localeConfig == null) {
localeConfig = new ArrayList();
}
return this.localeConfig;
}
/**
* Gets the value of the resourceBundle property.
*
* @return possible object is
* {@link FacesApplicationResourceBundle }
*/
public FacesApplicationResourceBundle getResourceBundle() {
return resourceBundle;
}
/**
* Sets the value of the resourceBundle property.
*
* @param value allowed object is
* {@link FacesApplicationResourceBundle }
*/
public void setResourceBundle(final FacesApplicationResourceBundle value) {
this.resourceBundle = value;
}
/**
* Gets the value of the applicationExtension property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the applicationExtension property.
*
*
* For example, to add a new item, do as follows:
*
* getApplicationExtension().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link FacesApplicationExtension }
*/
public List getApplicationExtension() {
if (applicationExtension == null) {
applicationExtension = new ArrayList();
}
return this.applicationExtension;
}
public List getDefaultValidators() {
if (defaultValidators == null) {
defaultValidators = new ArrayList();
}
return defaultValidators;
}
/**
* Gets the value of the id property.
*
* @return possible object is
* {@link java.lang.String }
*/
public java.lang.String getId() {
return id;
}
/**
* Sets the value of the id property.
*
* @param value allowed object is
* {@link java.lang.String }
*/
public void setId(final java.lang.String value) {
this.id = value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy