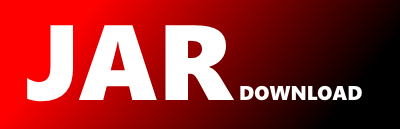
org.apache.parquet.format.ColumnIndex Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of parquet-format Show documentation
Show all versions of parquet-format Show documentation
Parquet is a columnar storage format that supports nested data. This provides all generated metadata code.
/**
* Autogenerated by Thrift Compiler (0.9.3)
*
* DO NOT EDIT UNLESS YOU ARE SURE THAT YOU KNOW WHAT YOU ARE DOING
* @generated
*/
package org.apache.parquet.format;
import org.apache.thrift.scheme.IScheme;
import org.apache.thrift.scheme.SchemeFactory;
import org.apache.thrift.scheme.StandardScheme;
import org.apache.thrift.scheme.TupleScheme;
import org.apache.thrift.protocol.TTupleProtocol;
import org.apache.thrift.protocol.TProtocolException;
import org.apache.thrift.EncodingUtils;
import org.apache.thrift.TException;
import org.apache.thrift.async.AsyncMethodCallback;
import org.apache.thrift.server.AbstractNonblockingServer.*;
import java.util.List;
import java.util.ArrayList;
import java.util.Map;
import java.util.HashMap;
import java.util.EnumMap;
import java.util.Set;
import java.util.HashSet;
import java.util.EnumSet;
import java.util.Collections;
import java.util.BitSet;
import java.nio.ByteBuffer;
import java.util.Arrays;
import javax.annotation.Generated;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
@SuppressWarnings({"cast", "rawtypes", "serial", "unchecked"})
/**
* Description for ColumnIndex.
* Each [i] refers to the page at OffsetIndex.page_locations[i]
*/
@Generated(value = "Autogenerated by Thrift Compiler (0.9.3)", date = "2018-09-27")
public class ColumnIndex implements org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final org.apache.thrift.protocol.TStruct STRUCT_DESC = new org.apache.thrift.protocol.TStruct("ColumnIndex");
private static final org.apache.thrift.protocol.TField NULL_PAGES_FIELD_DESC = new org.apache.thrift.protocol.TField("null_pages", org.apache.thrift.protocol.TType.LIST, (short)1);
private static final org.apache.thrift.protocol.TField MIN_VALUES_FIELD_DESC = new org.apache.thrift.protocol.TField("min_values", org.apache.thrift.protocol.TType.LIST, (short)2);
private static final org.apache.thrift.protocol.TField MAX_VALUES_FIELD_DESC = new org.apache.thrift.protocol.TField("max_values", org.apache.thrift.protocol.TType.LIST, (short)3);
private static final org.apache.thrift.protocol.TField BOUNDARY_ORDER_FIELD_DESC = new org.apache.thrift.protocol.TField("boundary_order", org.apache.thrift.protocol.TType.I32, (short)4);
private static final org.apache.thrift.protocol.TField NULL_COUNTS_FIELD_DESC = new org.apache.thrift.protocol.TField("null_counts", org.apache.thrift.protocol.TType.LIST, (short)5);
private static final Map, SchemeFactory> schemes = new HashMap, SchemeFactory>();
static {
schemes.put(StandardScheme.class, new ColumnIndexStandardSchemeFactory());
schemes.put(TupleScheme.class, new ColumnIndexTupleSchemeFactory());
}
/**
* A list of Boolean values to determine the validity of the corresponding
* min and max values. If true, a page contains only null values, and writers
* have to set the corresponding entries in min_values and max_values to
* byte[0], so that all lists have the same length. If false, the
* corresponding entries in min_values and max_values must be valid.
*/
public List null_pages; // required
/**
* Two lists containing lower and upper bounds for the values of each page.
* These may be the actual minimum and maximum values found on a page, but
* can also be (more compact) values that do not exist on a page. For
* example, instead of storing ""Blart Versenwald III", a writer may set
* min_values[i]="B", max_values[i]="C". Such more compact values must still
* be valid values within the column's logical type. Readers must make sure
* that list entries are populated before using them by inspecting null_pages.
*/
public List min_values; // required
public List max_values; // required
/**
* Stores whether both min_values and max_values are orderd and if so, in
* which direction. This allows readers to perform binary searches in both
* lists. Readers cannot assume that max_values[i] <= min_values[i+1], even
* if the lists are ordered.
*
* @see BoundaryOrder
*/
public BoundaryOrder boundary_order; // required
/**
* A list containing the number of null values for each page *
*/
public List null_counts; // optional
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements org.apache.thrift.TFieldIdEnum {
/**
* A list of Boolean values to determine the validity of the corresponding
* min and max values. If true, a page contains only null values, and writers
* have to set the corresponding entries in min_values and max_values to
* byte[0], so that all lists have the same length. If false, the
* corresponding entries in min_values and max_values must be valid.
*/
NULL_PAGES((short)1, "null_pages"),
/**
* Two lists containing lower and upper bounds for the values of each page.
* These may be the actual minimum and maximum values found on a page, but
* can also be (more compact) values that do not exist on a page. For
* example, instead of storing ""Blart Versenwald III", a writer may set
* min_values[i]="B", max_values[i]="C". Such more compact values must still
* be valid values within the column's logical type. Readers must make sure
* that list entries are populated before using them by inspecting null_pages.
*/
MIN_VALUES((short)2, "min_values"),
MAX_VALUES((short)3, "max_values"),
/**
* Stores whether both min_values and max_values are orderd and if so, in
* which direction. This allows readers to perform binary searches in both
* lists. Readers cannot assume that max_values[i] <= min_values[i+1], even
* if the lists are ordered.
*
* @see BoundaryOrder
*/
BOUNDARY_ORDER((short)4, "boundary_order"),
/**
* A list containing the number of null values for each page *
*/
NULL_COUNTS((short)5, "null_counts");
private static final Map byName = new HashMap();
static {
for (_Fields field : EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case 1: // NULL_PAGES
return NULL_PAGES;
case 2: // MIN_VALUES
return MIN_VALUES;
case 3: // MAX_VALUES
return MAX_VALUES;
case 4: // BOUNDARY_ORDER
return BOUNDARY_ORDER;
case 5: // NULL_COUNTS
return NULL_COUNTS;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
public static _Fields findByName(String name) {
return byName.get(name);
}
private final short _thriftId;
private final String _fieldName;
_Fields(short thriftId, String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public String getFieldName() {
return _fieldName;
}
}
// isset id assignments
private static final _Fields optionals[] = {_Fields.NULL_COUNTS};
public static final Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> tmpMap = new EnumMap<_Fields, org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.NULL_PAGES, new org.apache.thrift.meta_data.FieldMetaData("null_pages", org.apache.thrift.TFieldRequirementType.REQUIRED,
new org.apache.thrift.meta_data.ListMetaData(org.apache.thrift.protocol.TType.LIST,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.BOOL))));
tmpMap.put(_Fields.MIN_VALUES, new org.apache.thrift.meta_data.FieldMetaData("min_values", org.apache.thrift.TFieldRequirementType.REQUIRED,
new org.apache.thrift.meta_data.ListMetaData(org.apache.thrift.protocol.TType.LIST,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING , true))));
tmpMap.put(_Fields.MAX_VALUES, new org.apache.thrift.meta_data.FieldMetaData("max_values", org.apache.thrift.TFieldRequirementType.REQUIRED,
new org.apache.thrift.meta_data.ListMetaData(org.apache.thrift.protocol.TType.LIST,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING , true))));
tmpMap.put(_Fields.BOUNDARY_ORDER, new org.apache.thrift.meta_data.FieldMetaData("boundary_order", org.apache.thrift.TFieldRequirementType.REQUIRED,
new org.apache.thrift.meta_data.EnumMetaData(org.apache.thrift.protocol.TType.ENUM, BoundaryOrder.class)));
tmpMap.put(_Fields.NULL_COUNTS, new org.apache.thrift.meta_data.FieldMetaData("null_counts", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.ListMetaData(org.apache.thrift.protocol.TType.LIST,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.I64))));
metaDataMap = Collections.unmodifiableMap(tmpMap);
org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(ColumnIndex.class, metaDataMap);
}
public ColumnIndex() {
}
public ColumnIndex(
List null_pages,
List min_values,
List max_values,
BoundaryOrder boundary_order)
{
this();
this.null_pages = null_pages;
this.min_values = min_values;
this.max_values = max_values;
this.boundary_order = boundary_order;
}
/**
* Performs a deep copy on other.
*/
public ColumnIndex(ColumnIndex other) {
if (other.isSetNull_pages()) {
List __this__null_pages = new ArrayList(other.null_pages);
this.null_pages = __this__null_pages;
}
if (other.isSetMin_values()) {
List __this__min_values = new ArrayList(other.min_values);
this.min_values = __this__min_values;
}
if (other.isSetMax_values()) {
List __this__max_values = new ArrayList(other.max_values);
this.max_values = __this__max_values;
}
if (other.isSetBoundary_order()) {
this.boundary_order = other.boundary_order;
}
if (other.isSetNull_counts()) {
List __this__null_counts = new ArrayList(other.null_counts);
this.null_counts = __this__null_counts;
}
}
public ColumnIndex deepCopy() {
return new ColumnIndex(this);
}
@Override
public void clear() {
this.null_pages = null;
this.min_values = null;
this.max_values = null;
this.boundary_order = null;
this.null_counts = null;
}
public int getNull_pagesSize() {
return (this.null_pages == null) ? 0 : this.null_pages.size();
}
public java.util.Iterator getNull_pagesIterator() {
return (this.null_pages == null) ? null : this.null_pages.iterator();
}
public void addToNull_pages(boolean elem) {
if (this.null_pages == null) {
this.null_pages = new ArrayList();
}
this.null_pages.add(elem);
}
/**
* A list of Boolean values to determine the validity of the corresponding
* min and max values. If true, a page contains only null values, and writers
* have to set the corresponding entries in min_values and max_values to
* byte[0], so that all lists have the same length. If false, the
* corresponding entries in min_values and max_values must be valid.
*/
public List getNull_pages() {
return this.null_pages;
}
/**
* A list of Boolean values to determine the validity of the corresponding
* min and max values. If true, a page contains only null values, and writers
* have to set the corresponding entries in min_values and max_values to
* byte[0], so that all lists have the same length. If false, the
* corresponding entries in min_values and max_values must be valid.
*/
public ColumnIndex setNull_pages(List null_pages) {
this.null_pages = null_pages;
return this;
}
public void unsetNull_pages() {
this.null_pages = null;
}
/** Returns true if field null_pages is set (has been assigned a value) and false otherwise */
public boolean isSetNull_pages() {
return this.null_pages != null;
}
public void setNull_pagesIsSet(boolean value) {
if (!value) {
this.null_pages = null;
}
}
public int getMin_valuesSize() {
return (this.min_values == null) ? 0 : this.min_values.size();
}
public java.util.Iterator getMin_valuesIterator() {
return (this.min_values == null) ? null : this.min_values.iterator();
}
public void addToMin_values(ByteBuffer elem) {
if (this.min_values == null) {
this.min_values = new ArrayList();
}
this.min_values.add(elem);
}
/**
* Two lists containing lower and upper bounds for the values of each page.
* These may be the actual minimum and maximum values found on a page, but
* can also be (more compact) values that do not exist on a page. For
* example, instead of storing ""Blart Versenwald III", a writer may set
* min_values[i]="B", max_values[i]="C". Such more compact values must still
* be valid values within the column's logical type. Readers must make sure
* that list entries are populated before using them by inspecting null_pages.
*/
public List getMin_values() {
return this.min_values;
}
/**
* Two lists containing lower and upper bounds for the values of each page.
* These may be the actual minimum and maximum values found on a page, but
* can also be (more compact) values that do not exist on a page. For
* example, instead of storing ""Blart Versenwald III", a writer may set
* min_values[i]="B", max_values[i]="C". Such more compact values must still
* be valid values within the column's logical type. Readers must make sure
* that list entries are populated before using them by inspecting null_pages.
*/
public ColumnIndex setMin_values(List min_values) {
this.min_values = min_values;
return this;
}
public void unsetMin_values() {
this.min_values = null;
}
/** Returns true if field min_values is set (has been assigned a value) and false otherwise */
public boolean isSetMin_values() {
return this.min_values != null;
}
public void setMin_valuesIsSet(boolean value) {
if (!value) {
this.min_values = null;
}
}
public int getMax_valuesSize() {
return (this.max_values == null) ? 0 : this.max_values.size();
}
public java.util.Iterator getMax_valuesIterator() {
return (this.max_values == null) ? null : this.max_values.iterator();
}
public void addToMax_values(ByteBuffer elem) {
if (this.max_values == null) {
this.max_values = new ArrayList();
}
this.max_values.add(elem);
}
public List getMax_values() {
return this.max_values;
}
public ColumnIndex setMax_values(List max_values) {
this.max_values = max_values;
return this;
}
public void unsetMax_values() {
this.max_values = null;
}
/** Returns true if field max_values is set (has been assigned a value) and false otherwise */
public boolean isSetMax_values() {
return this.max_values != null;
}
public void setMax_valuesIsSet(boolean value) {
if (!value) {
this.max_values = null;
}
}
/**
* Stores whether both min_values and max_values are orderd and if so, in
* which direction. This allows readers to perform binary searches in both
* lists. Readers cannot assume that max_values[i] <= min_values[i+1], even
* if the lists are ordered.
*
* @see BoundaryOrder
*/
public BoundaryOrder getBoundary_order() {
return this.boundary_order;
}
/**
* Stores whether both min_values and max_values are orderd and if so, in
* which direction. This allows readers to perform binary searches in both
* lists. Readers cannot assume that max_values[i] <= min_values[i+1], even
* if the lists are ordered.
*
* @see BoundaryOrder
*/
public ColumnIndex setBoundary_order(BoundaryOrder boundary_order) {
this.boundary_order = boundary_order;
return this;
}
public void unsetBoundary_order() {
this.boundary_order = null;
}
/** Returns true if field boundary_order is set (has been assigned a value) and false otherwise */
public boolean isSetBoundary_order() {
return this.boundary_order != null;
}
public void setBoundary_orderIsSet(boolean value) {
if (!value) {
this.boundary_order = null;
}
}
public int getNull_countsSize() {
return (this.null_counts == null) ? 0 : this.null_counts.size();
}
public java.util.Iterator getNull_countsIterator() {
return (this.null_counts == null) ? null : this.null_counts.iterator();
}
public void addToNull_counts(long elem) {
if (this.null_counts == null) {
this.null_counts = new ArrayList();
}
this.null_counts.add(elem);
}
/**
* A list containing the number of null values for each page *
*/
public List getNull_counts() {
return this.null_counts;
}
/**
* A list containing the number of null values for each page *
*/
public ColumnIndex setNull_counts(List null_counts) {
this.null_counts = null_counts;
return this;
}
public void unsetNull_counts() {
this.null_counts = null;
}
/** Returns true if field null_counts is set (has been assigned a value) and false otherwise */
public boolean isSetNull_counts() {
return this.null_counts != null;
}
public void setNull_countsIsSet(boolean value) {
if (!value) {
this.null_counts = null;
}
}
public void setFieldValue(_Fields field, Object value) {
switch (field) {
case NULL_PAGES:
if (value == null) {
unsetNull_pages();
} else {
setNull_pages((List)value);
}
break;
case MIN_VALUES:
if (value == null) {
unsetMin_values();
} else {
setMin_values((List)value);
}
break;
case MAX_VALUES:
if (value == null) {
unsetMax_values();
} else {
setMax_values((List)value);
}
break;
case BOUNDARY_ORDER:
if (value == null) {
unsetBoundary_order();
} else {
setBoundary_order((BoundaryOrder)value);
}
break;
case NULL_COUNTS:
if (value == null) {
unsetNull_counts();
} else {
setNull_counts((List)value);
}
break;
}
}
public Object getFieldValue(_Fields field) {
switch (field) {
case NULL_PAGES:
return getNull_pages();
case MIN_VALUES:
return getMin_values();
case MAX_VALUES:
return getMax_values();
case BOUNDARY_ORDER:
return getBoundary_order();
case NULL_COUNTS:
return getNull_counts();
}
throw new IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new IllegalArgumentException();
}
switch (field) {
case NULL_PAGES:
return isSetNull_pages();
case MIN_VALUES:
return isSetMin_values();
case MAX_VALUES:
return isSetMax_values();
case BOUNDARY_ORDER:
return isSetBoundary_order();
case NULL_COUNTS:
return isSetNull_counts();
}
throw new IllegalStateException();
}
@Override
public boolean equals(Object that) {
if (that == null)
return false;
if (that instanceof ColumnIndex)
return this.equals((ColumnIndex)that);
return false;
}
public boolean equals(ColumnIndex that) {
if (that == null)
return false;
boolean this_present_null_pages = true && this.isSetNull_pages();
boolean that_present_null_pages = true && that.isSetNull_pages();
if (this_present_null_pages || that_present_null_pages) {
if (!(this_present_null_pages && that_present_null_pages))
return false;
if (!this.null_pages.equals(that.null_pages))
return false;
}
boolean this_present_min_values = true && this.isSetMin_values();
boolean that_present_min_values = true && that.isSetMin_values();
if (this_present_min_values || that_present_min_values) {
if (!(this_present_min_values && that_present_min_values))
return false;
if (!this.min_values.equals(that.min_values))
return false;
}
boolean this_present_max_values = true && this.isSetMax_values();
boolean that_present_max_values = true && that.isSetMax_values();
if (this_present_max_values || that_present_max_values) {
if (!(this_present_max_values && that_present_max_values))
return false;
if (!this.max_values.equals(that.max_values))
return false;
}
boolean this_present_boundary_order = true && this.isSetBoundary_order();
boolean that_present_boundary_order = true && that.isSetBoundary_order();
if (this_present_boundary_order || that_present_boundary_order) {
if (!(this_present_boundary_order && that_present_boundary_order))
return false;
if (!this.boundary_order.equals(that.boundary_order))
return false;
}
boolean this_present_null_counts = true && this.isSetNull_counts();
boolean that_present_null_counts = true && that.isSetNull_counts();
if (this_present_null_counts || that_present_null_counts) {
if (!(this_present_null_counts && that_present_null_counts))
return false;
if (!this.null_counts.equals(that.null_counts))
return false;
}
return true;
}
@Override
public int hashCode() {
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy