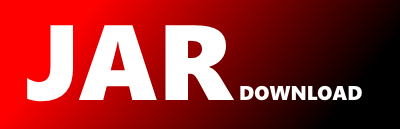
com.google.api.auth.AuthProvider.scala Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pekko-connectors-google-cloud-bigquery-storage_3 Show documentation
Show all versions of pekko-connectors-google-cloud-bigquery-storage_3 Show documentation
Apache Pekko Connectors is a Reactive Enterprise Integration library for Java and Scala, based on Reactive Streams and Pekko.
The newest version!
// Generated by the Scala Plugin for the Protocol Buffer Compiler.
// Do not edit!
//
// Protofile syntax: PROTO3
package com.google.api.auth
/** Configuration for an authentication provider, including support for
* [JSON Web Token
* (JWT)](https://tools.ietf.org/html/draft-ietf-oauth-json-web-token-32).
*
* @param id
* The unique identifier of the auth provider. It will be referred to by
* `AuthRequirement.provider_id`.
*
* Example: "bookstore_auth".
* @param issuer
* Identifies the principal that issued the JWT. See
* https://tools.ietf.org/html/draft-ietf-oauth-json-web-token-32#section-4.1.1
* Usually a URL or an email address.
*
* Example: https://securetoken.google.com
* Example: 1234567-compute@developer.gserviceaccount.com
* @param jwksUri
* URL of the provider's public key set to validate signature of the JWT. See
* [OpenID
* Discovery](https://openid.net/specs/openid-connect-discovery-1_0.html#ProviderMetadata).
* Optional if the key set document:
* - can be retrieved from
* [OpenID
* Discovery](https://openid.net/specs/openid-connect-discovery-1_0.html)
* of the issuer.
* - can be inferred from the email domain of the issuer (e.g. a Google
* service account).
*
* Example: https://www.googleapis.com/oauth2/v1/certs
* @param audiences
* The list of JWT
* [audiences](https://tools.ietf.org/html/draft-ietf-oauth-json-web-token-32#section-4.1.3).
* that are allowed to access. A JWT containing any of these audiences will
* be accepted. When this setting is absent, JWTs with audiences:
* - "https://[service.name]/[google.protobuf.Api.name]"
* - "https://[service.name]/"
* will be accepted.
* For example, if no audiences are in the setting, LibraryService API will
* accept JWTs with the following audiences:
* -
* https://library-example.googleapis.com/google.example.library.v1.LibraryService
* - https://library-example.googleapis.com/
*
* Example:
*
* audiences: bookstore_android.apps.googleusercontent.com,
* bookstore_web.apps.googleusercontent.com
* @param authorizationUrl
* Redirect URL if JWT token is required but not present or is expired.
* Implement authorizationUrl of securityDefinitions in OpenAPI spec.
* @param jwtLocations
* Defines the locations to extract the JWT. For now it is only used by the
* Cloud Endpoints to store the OpenAPI extension [x-google-jwt-locations]
* (https://cloud.google.com/endpoints/docs/openapi/openapi-extensions#x-google-jwt-locations)
*
* JWT locations can be one of HTTP headers, URL query parameters or
* cookies. The rule is that the first match wins.
*
* If not specified, default to use following 3 locations:
* 1) Authorization: Bearer
* 2) x-goog-iap-jwt-assertion
* 3) access_token query parameter
*
* Default locations can be specified as followings:
* jwt_locations:
* - header: Authorization
* value_prefix: "Bearer "
* - header: x-goog-iap-jwt-assertion
* - query: access_token
*/
@SerialVersionUID(0L)
final case class AuthProvider(
id: _root_.scala.Predef.String = "",
issuer: _root_.scala.Predef.String = "",
jwksUri: _root_.scala.Predef.String = "",
audiences: _root_.scala.Predef.String = "",
authorizationUrl: _root_.scala.Predef.String = "",
jwtLocations: _root_.scala.Seq[com.google.api.auth.JwtLocation] = _root_.scala.Seq.empty,
unknownFields: _root_.scalapb.UnknownFieldSet = _root_.scalapb.UnknownFieldSet.empty
) extends scalapb.GeneratedMessage with scalapb.lenses.Updatable[AuthProvider] {
@transient
private[this] var __serializedSizeMemoized: _root_.scala.Int = 0
private[this] def __computeSerializedSize(): _root_.scala.Int = {
var __size = 0
{
val __value = id
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeStringSize(1, __value)
}
};
{
val __value = issuer
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeStringSize(2, __value)
}
};
{
val __value = jwksUri
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeStringSize(3, __value)
}
};
{
val __value = audiences
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeStringSize(4, __value)
}
};
{
val __value = authorizationUrl
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeStringSize(5, __value)
}
};
jwtLocations.foreach { __item =>
val __value = __item
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
}
__size += unknownFields.serializedSize
__size
}
override def serializedSize: _root_.scala.Int = {
var __size = __serializedSizeMemoized
if (__size == 0) {
__size = __computeSerializedSize() + 1
__serializedSizeMemoized = __size
}
__size - 1
}
def writeTo(`_output__`: _root_.com.google.protobuf.CodedOutputStream): _root_.scala.Unit = {
{
val __v = id
if (!__v.isEmpty) {
_output__.writeString(1, __v)
}
};
{
val __v = issuer
if (!__v.isEmpty) {
_output__.writeString(2, __v)
}
};
{
val __v = jwksUri
if (!__v.isEmpty) {
_output__.writeString(3, __v)
}
};
{
val __v = audiences
if (!__v.isEmpty) {
_output__.writeString(4, __v)
}
};
{
val __v = authorizationUrl
if (!__v.isEmpty) {
_output__.writeString(5, __v)
}
};
jwtLocations.foreach { __v =>
val __m = __v
_output__.writeTag(6, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
unknownFields.writeTo(_output__)
}
def withId(__v: _root_.scala.Predef.String): AuthProvider = copy(id = __v)
def withIssuer(__v: _root_.scala.Predef.String): AuthProvider = copy(issuer = __v)
def withJwksUri(__v: _root_.scala.Predef.String): AuthProvider = copy(jwksUri = __v)
def withAudiences(__v: _root_.scala.Predef.String): AuthProvider = copy(audiences = __v)
def withAuthorizationUrl(__v: _root_.scala.Predef.String): AuthProvider = copy(authorizationUrl = __v)
def clearJwtLocations = copy(jwtLocations = _root_.scala.Seq.empty)
def addJwtLocations(__vs: com.google.api.auth.JwtLocation *): AuthProvider = addAllJwtLocations(__vs)
def addAllJwtLocations(__vs: Iterable[com.google.api.auth.JwtLocation]): AuthProvider = copy(jwtLocations = jwtLocations ++ __vs)
def withJwtLocations(__v: _root_.scala.Seq[com.google.api.auth.JwtLocation]): AuthProvider = copy(jwtLocations = __v)
def withUnknownFields(__v: _root_.scalapb.UnknownFieldSet) = copy(unknownFields = __v)
def discardUnknownFields = copy(unknownFields = _root_.scalapb.UnknownFieldSet.empty)
def getFieldByNumber(__fieldNumber: _root_.scala.Int): _root_.scala.Any = {
(__fieldNumber: @_root_.scala.unchecked) match {
case 1 => {
val __t = id
if (__t != "") __t else null
}
case 2 => {
val __t = issuer
if (__t != "") __t else null
}
case 3 => {
val __t = jwksUri
if (__t != "") __t else null
}
case 4 => {
val __t = audiences
if (__t != "") __t else null
}
case 5 => {
val __t = authorizationUrl
if (__t != "") __t else null
}
case 6 => jwtLocations
}
}
def getField(__field: _root_.scalapb.descriptors.FieldDescriptor): _root_.scalapb.descriptors.PValue = {
_root_.scala.Predef.require(__field.containingMessage eq companion.scalaDescriptor)
(__field.number: @_root_.scala.unchecked) match {
case 1 => _root_.scalapb.descriptors.PString(id)
case 2 => _root_.scalapb.descriptors.PString(issuer)
case 3 => _root_.scalapb.descriptors.PString(jwksUri)
case 4 => _root_.scalapb.descriptors.PString(audiences)
case 5 => _root_.scalapb.descriptors.PString(authorizationUrl)
case 6 => _root_.scalapb.descriptors.PRepeated(jwtLocations.iterator.map(_.toPMessage).toVector)
}
}
def toProtoString: _root_.scala.Predef.String = _root_.scalapb.TextFormat.printToUnicodeString(this)
def companion: com.google.api.auth.AuthProvider.type = com.google.api.auth.AuthProvider
// @@protoc_insertion_point(GeneratedMessage[google.api.AuthProvider])
}
object AuthProvider extends scalapb.GeneratedMessageCompanion[com.google.api.auth.AuthProvider] {
implicit def messageCompanion: scalapb.GeneratedMessageCompanion[com.google.api.auth.AuthProvider] = this
def parseFrom(`_input__`: _root_.com.google.protobuf.CodedInputStream): com.google.api.auth.AuthProvider = {
var __id: _root_.scala.Predef.String = ""
var __issuer: _root_.scala.Predef.String = ""
var __jwksUri: _root_.scala.Predef.String = ""
var __audiences: _root_.scala.Predef.String = ""
var __authorizationUrl: _root_.scala.Predef.String = ""
val __jwtLocations: _root_.scala.collection.immutable.VectorBuilder[com.google.api.auth.JwtLocation] = new _root_.scala.collection.immutable.VectorBuilder[com.google.api.auth.JwtLocation]
var `_unknownFields__`: _root_.scalapb.UnknownFieldSet.Builder = null
var _done__ = false
while (!_done__) {
val _tag__ = _input__.readTag()
_tag__ match {
case 0 => _done__ = true
case 10 =>
__id = _input__.readStringRequireUtf8()
case 18 =>
__issuer = _input__.readStringRequireUtf8()
case 26 =>
__jwksUri = _input__.readStringRequireUtf8()
case 34 =>
__audiences = _input__.readStringRequireUtf8()
case 42 =>
__authorizationUrl = _input__.readStringRequireUtf8()
case 50 =>
__jwtLocations += _root_.scalapb.LiteParser.readMessage[com.google.api.auth.JwtLocation](_input__)
case tag =>
if (_unknownFields__ == null) {
_unknownFields__ = new _root_.scalapb.UnknownFieldSet.Builder()
}
_unknownFields__.parseField(tag, _input__)
}
}
com.google.api.auth.AuthProvider(
id = __id,
issuer = __issuer,
jwksUri = __jwksUri,
audiences = __audiences,
authorizationUrl = __authorizationUrl,
jwtLocations = __jwtLocations.result(),
unknownFields = if (_unknownFields__ == null) _root_.scalapb.UnknownFieldSet.empty else _unknownFields__.result()
)
}
implicit def messageReads: _root_.scalapb.descriptors.Reads[com.google.api.auth.AuthProvider] = _root_.scalapb.descriptors.Reads{
case _root_.scalapb.descriptors.PMessage(__fieldsMap) =>
_root_.scala.Predef.require(__fieldsMap.keys.forall(_.containingMessage eq scalaDescriptor), "FieldDescriptor does not match message type.")
com.google.api.auth.AuthProvider(
id = __fieldsMap.get(scalaDescriptor.findFieldByNumber(1).get).map(_.as[_root_.scala.Predef.String]).getOrElse(""),
issuer = __fieldsMap.get(scalaDescriptor.findFieldByNumber(2).get).map(_.as[_root_.scala.Predef.String]).getOrElse(""),
jwksUri = __fieldsMap.get(scalaDescriptor.findFieldByNumber(3).get).map(_.as[_root_.scala.Predef.String]).getOrElse(""),
audiences = __fieldsMap.get(scalaDescriptor.findFieldByNumber(4).get).map(_.as[_root_.scala.Predef.String]).getOrElse(""),
authorizationUrl = __fieldsMap.get(scalaDescriptor.findFieldByNumber(5).get).map(_.as[_root_.scala.Predef.String]).getOrElse(""),
jwtLocations = __fieldsMap.get(scalaDescriptor.findFieldByNumber(6).get).map(_.as[_root_.scala.Seq[com.google.api.auth.JwtLocation]]).getOrElse(_root_.scala.Seq.empty)
)
case _ => throw new RuntimeException("Expected PMessage")
}
def javaDescriptor: _root_.com.google.protobuf.Descriptors.Descriptor = AuthProto.javaDescriptor.getMessageTypes().get(3)
def scalaDescriptor: _root_.scalapb.descriptors.Descriptor = AuthProto.scalaDescriptor.messages(3)
def messageCompanionForFieldNumber(__number: _root_.scala.Int): _root_.scalapb.GeneratedMessageCompanion[_] = {
var __out: _root_.scalapb.GeneratedMessageCompanion[_] = null
(__number: @_root_.scala.unchecked) match {
case 6 => __out = com.google.api.auth.JwtLocation
}
__out
}
lazy val nestedMessagesCompanions: Seq[_root_.scalapb.GeneratedMessageCompanion[_ <: _root_.scalapb.GeneratedMessage]] = Seq.empty
def enumCompanionForFieldNumber(__fieldNumber: _root_.scala.Int): _root_.scalapb.GeneratedEnumCompanion[_] = throw new MatchError(__fieldNumber)
lazy val defaultInstance = com.google.api.auth.AuthProvider(
id = "",
issuer = "",
jwksUri = "",
audiences = "",
authorizationUrl = "",
jwtLocations = _root_.scala.Seq.empty
)
implicit class AuthProviderLens[UpperPB](_l: _root_.scalapb.lenses.Lens[UpperPB, com.google.api.auth.AuthProvider]) extends _root_.scalapb.lenses.ObjectLens[UpperPB, com.google.api.auth.AuthProvider](_l) {
def id: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Predef.String] = field(_.id)((c_, f_) => c_.copy(id = f_))
def issuer: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Predef.String] = field(_.issuer)((c_, f_) => c_.copy(issuer = f_))
def jwksUri: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Predef.String] = field(_.jwksUri)((c_, f_) => c_.copy(jwksUri = f_))
def audiences: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Predef.String] = field(_.audiences)((c_, f_) => c_.copy(audiences = f_))
def authorizationUrl: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Predef.String] = field(_.authorizationUrl)((c_, f_) => c_.copy(authorizationUrl = f_))
def jwtLocations: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Seq[com.google.api.auth.JwtLocation]] = field(_.jwtLocations)((c_, f_) => c_.copy(jwtLocations = f_))
}
final val ID_FIELD_NUMBER = 1
final val ISSUER_FIELD_NUMBER = 2
final val JWKS_URI_FIELD_NUMBER = 3
final val AUDIENCES_FIELD_NUMBER = 4
final val AUTHORIZATION_URL_FIELD_NUMBER = 5
final val JWT_LOCATIONS_FIELD_NUMBER = 6
def of(
id: _root_.scala.Predef.String,
issuer: _root_.scala.Predef.String,
jwksUri: _root_.scala.Predef.String,
audiences: _root_.scala.Predef.String,
authorizationUrl: _root_.scala.Predef.String,
jwtLocations: _root_.scala.Seq[com.google.api.auth.JwtLocation]
): _root_.com.google.api.auth.AuthProvider = _root_.com.google.api.auth.AuthProvider(
id,
issuer,
jwksUri,
audiences,
authorizationUrl,
jwtLocations
)
// @@protoc_insertion_point(GeneratedMessageCompanion[google.api.AuthProvider])
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy