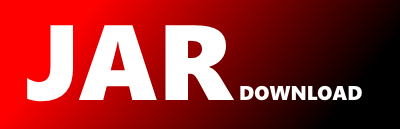
com.google.api.service.Service.scala Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pekko-connectors-google-cloud-bigquery-storage_3 Show documentation
Show all versions of pekko-connectors-google-cloud-bigquery-storage_3 Show documentation
Apache Pekko Connectors is a Reactive Enterprise Integration library for Java and Scala, based on Reactive Streams and Pekko.
The newest version!
// Generated by the Scala Plugin for the Protocol Buffer Compiler.
// Do not edit!
//
// Protofile syntax: PROTO3
package com.google.api.service
/** `Service` is the root object of Google API service configuration (service
* config). It describes the basic information about a logical service,
* such as the service name and the user-facing title, and delegates other
* aspects to sub-sections. Each sub-section is either a proto message or a
* repeated proto message that configures a specific aspect, such as auth.
* For more information, see each proto message definition.
*
* Example:
*
* type: google.api.Service
* name: calendar.googleapis.com
* title: Google Calendar API
* apis:
* - name: google.calendar.v3.Calendar
*
* visibility:
* rules:
* - selector: "google.calendar.v3.*"
* restriction: PREVIEW
* backend:
* rules:
* - selector: "google.calendar.v3.*"
* address: calendar.example.com
*
* authentication:
* providers:
* - id: google_calendar_auth
* jwks_uri: https://www.googleapis.com/oauth2/v1/certs
* issuer: https://securetoken.google.com
* rules:
* - selector: "*"
* requirements:
* provider_id: google_calendar_auth
*
* @param name
* The service name, which is a DNS-like logical identifier for the
* service, such as `calendar.googleapis.com`. The service name
* typically goes through DNS verification to make sure the owner
* of the service also owns the DNS name.
* @param title
* The product title for this service, it is the name displayed in Google
* Cloud Console.
* @param producerProjectId
* The Google project that owns this service.
* @param id
* A unique ID for a specific instance of this message, typically assigned
* by the client for tracking purpose. Must be no longer than 63 characters
* and only lower case letters, digits, '.', '_' and '-' are allowed. If
* empty, the server may choose to generate one instead.
* @param apis
* A list of API interfaces exported by this service. Only the `name` field
* of the [google.protobuf.Api][google.protobuf.Api] needs to be provided by
* the configuration author, as the remaining fields will be derived from the
* IDL during the normalization process. It is an error to specify an API
* interface here which cannot be resolved against the associated IDL files.
* @param types
* A list of all proto message types included in this API service.
* Types referenced directly or indirectly by the `apis` are automatically
* included. Messages which are not referenced but shall be included, such as
* types used by the `google.protobuf.Any` type, should be listed here by
* name by the configuration author. Example:
*
* types:
* - name: google.protobuf.Int32
* @param enums
* A list of all enum types included in this API service. Enums referenced
* directly or indirectly by the `apis` are automatically included. Enums
* which are not referenced but shall be included should be listed here by
* name by the configuration author. Example:
*
* enums:
* - name: google.someapi.v1.SomeEnum
* @param documentation
* Additional API documentation.
* @param backend
* API backend configuration.
* @param http
* HTTP configuration.
* @param quota
* Quota configuration.
* @param authentication
* Auth configuration.
* @param context
* Context configuration.
* @param usage
* Configuration controlling usage of this service.
* @param endpoints
* Configuration for network endpoints. If this is empty, then an endpoint
* with the same name as the service is automatically generated to service all
* defined APIs.
* @param control
* Configuration for the service control plane.
* @param logs
* Defines the logs used by this service.
* @param metrics
* Defines the metrics used by this service.
* @param monitoredResources
* Defines the monitored resources used by this service. This is required
* by the [Service.monitoring][google.api.Service.monitoring] and
* [Service.logging][google.api.Service.logging] configurations.
* @param billing
* Billing configuration.
* @param logging
* Logging configuration.
* @param monitoring
* Monitoring configuration.
* @param systemParameters
* System parameter configuration.
* @param sourceInfo
* Output only. The source information for this configuration if available.
* @param publishing
* Settings for [Google Cloud Client
* libraries](https://cloud.google.com/apis/docs/cloud-client-libraries)
* generated from APIs defined as protocol buffers.
* @param configVersion
* Obsolete. Do not use.
*
* This field has no semantic meaning. The service config compiler always
* sets this field to `3`.
*/
@SerialVersionUID(0L)
final case class Service(
name: _root_.scala.Predef.String = "",
title: _root_.scala.Predef.String = "",
producerProjectId: _root_.scala.Predef.String = "",
id: _root_.scala.Predef.String = "",
apis: _root_.scala.Seq[com.google.protobuf.api.Api] = _root_.scala.Seq.empty,
types: _root_.scala.Seq[com.google.protobuf.`type`.Type] = _root_.scala.Seq.empty,
enums: _root_.scala.Seq[com.google.protobuf.`type`.Enum] = _root_.scala.Seq.empty,
documentation: _root_.scala.Option[com.google.api.documentation.Documentation] = _root_.scala.None,
backend: _root_.scala.Option[com.google.api.backend.Backend] = _root_.scala.None,
http: _root_.scala.Option[com.google.api.http.Http] = _root_.scala.None,
quota: _root_.scala.Option[com.google.api.quota.Quota] = _root_.scala.None,
authentication: _root_.scala.Option[com.google.api.auth.Authentication] = _root_.scala.None,
context: _root_.scala.Option[com.google.api.context.Context] = _root_.scala.None,
usage: _root_.scala.Option[com.google.api.usage.Usage] = _root_.scala.None,
endpoints: _root_.scala.Seq[com.google.api.endpoint.Endpoint] = _root_.scala.Seq.empty,
control: _root_.scala.Option[com.google.api.control.Control] = _root_.scala.None,
logs: _root_.scala.Seq[com.google.api.log.LogDescriptor] = _root_.scala.Seq.empty,
metrics: _root_.scala.Seq[com.google.api.metric.MetricDescriptor] = _root_.scala.Seq.empty,
monitoredResources: _root_.scala.Seq[com.google.api.monitored_resource.MonitoredResourceDescriptor] = _root_.scala.Seq.empty,
billing: _root_.scala.Option[com.google.api.billing.Billing] = _root_.scala.None,
logging: _root_.scala.Option[com.google.api.logging.Logging] = _root_.scala.None,
monitoring: _root_.scala.Option[com.google.api.monitoring.Monitoring] = _root_.scala.None,
systemParameters: _root_.scala.Option[com.google.api.system_parameter.SystemParameters] = _root_.scala.None,
sourceInfo: _root_.scala.Option[com.google.api.source_info.SourceInfo] = _root_.scala.None,
publishing: _root_.scala.Option[com.google.api.client.Publishing] = _root_.scala.None,
configVersion: _root_.scala.Option[_root_.scala.Int] = _root_.scala.None,
unknownFields: _root_.scalapb.UnknownFieldSet = _root_.scalapb.UnknownFieldSet.empty
) extends scalapb.GeneratedMessage with scalapb.lenses.Updatable[Service] {
@transient
private[this] var __serializedSizeMemoized: _root_.scala.Int = 0
private[this] def __computeSerializedSize(): _root_.scala.Int = {
var __size = 0
{
val __value = name
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeStringSize(1, __value)
}
};
{
val __value = title
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeStringSize(2, __value)
}
};
{
val __value = producerProjectId
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeStringSize(22, __value)
}
};
{
val __value = id
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeStringSize(33, __value)
}
};
apis.foreach { __item =>
val __value = __item
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
}
types.foreach { __item =>
val __value = __item
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
}
enums.foreach { __item =>
val __value = __item
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
}
if (documentation.isDefined) {
val __value = documentation.get
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
};
if (backend.isDefined) {
val __value = backend.get
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
};
if (http.isDefined) {
val __value = http.get
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
};
if (quota.isDefined) {
val __value = quota.get
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
};
if (authentication.isDefined) {
val __value = authentication.get
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
};
if (context.isDefined) {
val __value = context.get
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
};
if (usage.isDefined) {
val __value = usage.get
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
};
endpoints.foreach { __item =>
val __value = __item
__size += 2 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
}
if (control.isDefined) {
val __value = control.get
__size += 2 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
};
logs.foreach { __item =>
val __value = __item
__size += 2 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
}
metrics.foreach { __item =>
val __value = __item
__size += 2 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
}
monitoredResources.foreach { __item =>
val __value = __item
__size += 2 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
}
if (billing.isDefined) {
val __value = billing.get
__size += 2 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
};
if (logging.isDefined) {
val __value = logging.get
__size += 2 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
};
if (monitoring.isDefined) {
val __value = monitoring.get
__size += 2 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
};
if (systemParameters.isDefined) {
val __value = systemParameters.get
__size += 2 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
};
if (sourceInfo.isDefined) {
val __value = sourceInfo.get
__size += 2 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
};
if (publishing.isDefined) {
val __value = publishing.get
__size += 2 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
};
if (configVersion.isDefined) {
val __value = com.google.api.service.Service._typemapper_configVersion.toBase(configVersion.get)
__size += 2 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
};
__size += unknownFields.serializedSize
__size
}
override def serializedSize: _root_.scala.Int = {
var __size = __serializedSizeMemoized
if (__size == 0) {
__size = __computeSerializedSize() + 1
__serializedSizeMemoized = __size
}
__size - 1
}
def writeTo(`_output__`: _root_.com.google.protobuf.CodedOutputStream): _root_.scala.Unit = {
{
val __v = name
if (!__v.isEmpty) {
_output__.writeString(1, __v)
}
};
{
val __v = title
if (!__v.isEmpty) {
_output__.writeString(2, __v)
}
};
apis.foreach { __v =>
val __m = __v
_output__.writeTag(3, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
types.foreach { __v =>
val __m = __v
_output__.writeTag(4, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
enums.foreach { __v =>
val __m = __v
_output__.writeTag(5, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
documentation.foreach { __v =>
val __m = __v
_output__.writeTag(6, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
backend.foreach { __v =>
val __m = __v
_output__.writeTag(8, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
http.foreach { __v =>
val __m = __v
_output__.writeTag(9, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
quota.foreach { __v =>
val __m = __v
_output__.writeTag(10, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
authentication.foreach { __v =>
val __m = __v
_output__.writeTag(11, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
context.foreach { __v =>
val __m = __v
_output__.writeTag(12, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
usage.foreach { __v =>
val __m = __v
_output__.writeTag(15, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
endpoints.foreach { __v =>
val __m = __v
_output__.writeTag(18, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
configVersion.foreach { __v =>
val __m = com.google.api.service.Service._typemapper_configVersion.toBase(__v)
_output__.writeTag(20, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
control.foreach { __v =>
val __m = __v
_output__.writeTag(21, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
{
val __v = producerProjectId
if (!__v.isEmpty) {
_output__.writeString(22, __v)
}
};
logs.foreach { __v =>
val __m = __v
_output__.writeTag(23, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
metrics.foreach { __v =>
val __m = __v
_output__.writeTag(24, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
monitoredResources.foreach { __v =>
val __m = __v
_output__.writeTag(25, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
billing.foreach { __v =>
val __m = __v
_output__.writeTag(26, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
logging.foreach { __v =>
val __m = __v
_output__.writeTag(27, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
monitoring.foreach { __v =>
val __m = __v
_output__.writeTag(28, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
systemParameters.foreach { __v =>
val __m = __v
_output__.writeTag(29, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
{
val __v = id
if (!__v.isEmpty) {
_output__.writeString(33, __v)
}
};
sourceInfo.foreach { __v =>
val __m = __v
_output__.writeTag(37, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
publishing.foreach { __v =>
val __m = __v
_output__.writeTag(45, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
unknownFields.writeTo(_output__)
}
def withName(__v: _root_.scala.Predef.String): Service = copy(name = __v)
def withTitle(__v: _root_.scala.Predef.String): Service = copy(title = __v)
def withProducerProjectId(__v: _root_.scala.Predef.String): Service = copy(producerProjectId = __v)
def withId(__v: _root_.scala.Predef.String): Service = copy(id = __v)
def clearApis = copy(apis = _root_.scala.Seq.empty)
def addApis(__vs: com.google.protobuf.api.Api *): Service = addAllApis(__vs)
def addAllApis(__vs: Iterable[com.google.protobuf.api.Api]): Service = copy(apis = apis ++ __vs)
def withApis(__v: _root_.scala.Seq[com.google.protobuf.api.Api]): Service = copy(apis = __v)
def clearTypes = copy(types = _root_.scala.Seq.empty)
def addTypes(__vs: com.google.protobuf.`type`.Type *): Service = addAllTypes(__vs)
def addAllTypes(__vs: Iterable[com.google.protobuf.`type`.Type]): Service = copy(types = types ++ __vs)
def withTypes(__v: _root_.scala.Seq[com.google.protobuf.`type`.Type]): Service = copy(types = __v)
def clearEnums = copy(enums = _root_.scala.Seq.empty)
def addEnums(__vs: com.google.protobuf.`type`.Enum *): Service = addAllEnums(__vs)
def addAllEnums(__vs: Iterable[com.google.protobuf.`type`.Enum]): Service = copy(enums = enums ++ __vs)
def withEnums(__v: _root_.scala.Seq[com.google.protobuf.`type`.Enum]): Service = copy(enums = __v)
def getDocumentation: com.google.api.documentation.Documentation = documentation.getOrElse(com.google.api.documentation.Documentation.defaultInstance)
def clearDocumentation: Service = copy(documentation = _root_.scala.None)
def withDocumentation(__v: com.google.api.documentation.Documentation): Service = copy(documentation = Option(__v))
def getBackend: com.google.api.backend.Backend = backend.getOrElse(com.google.api.backend.Backend.defaultInstance)
def clearBackend: Service = copy(backend = _root_.scala.None)
def withBackend(__v: com.google.api.backend.Backend): Service = copy(backend = Option(__v))
def getHttp: com.google.api.http.Http = http.getOrElse(com.google.api.http.Http.defaultInstance)
def clearHttp: Service = copy(http = _root_.scala.None)
def withHttp(__v: com.google.api.http.Http): Service = copy(http = Option(__v))
def getQuota: com.google.api.quota.Quota = quota.getOrElse(com.google.api.quota.Quota.defaultInstance)
def clearQuota: Service = copy(quota = _root_.scala.None)
def withQuota(__v: com.google.api.quota.Quota): Service = copy(quota = Option(__v))
def getAuthentication: com.google.api.auth.Authentication = authentication.getOrElse(com.google.api.auth.Authentication.defaultInstance)
def clearAuthentication: Service = copy(authentication = _root_.scala.None)
def withAuthentication(__v: com.google.api.auth.Authentication): Service = copy(authentication = Option(__v))
def getContext: com.google.api.context.Context = context.getOrElse(com.google.api.context.Context.defaultInstance)
def clearContext: Service = copy(context = _root_.scala.None)
def withContext(__v: com.google.api.context.Context): Service = copy(context = Option(__v))
def getUsage: com.google.api.usage.Usage = usage.getOrElse(com.google.api.usage.Usage.defaultInstance)
def clearUsage: Service = copy(usage = _root_.scala.None)
def withUsage(__v: com.google.api.usage.Usage): Service = copy(usage = Option(__v))
def clearEndpoints = copy(endpoints = _root_.scala.Seq.empty)
def addEndpoints(__vs: com.google.api.endpoint.Endpoint *): Service = addAllEndpoints(__vs)
def addAllEndpoints(__vs: Iterable[com.google.api.endpoint.Endpoint]): Service = copy(endpoints = endpoints ++ __vs)
def withEndpoints(__v: _root_.scala.Seq[com.google.api.endpoint.Endpoint]): Service = copy(endpoints = __v)
def getControl: com.google.api.control.Control = control.getOrElse(com.google.api.control.Control.defaultInstance)
def clearControl: Service = copy(control = _root_.scala.None)
def withControl(__v: com.google.api.control.Control): Service = copy(control = Option(__v))
def clearLogs = copy(logs = _root_.scala.Seq.empty)
def addLogs(__vs: com.google.api.log.LogDescriptor *): Service = addAllLogs(__vs)
def addAllLogs(__vs: Iterable[com.google.api.log.LogDescriptor]): Service = copy(logs = logs ++ __vs)
def withLogs(__v: _root_.scala.Seq[com.google.api.log.LogDescriptor]): Service = copy(logs = __v)
def clearMetrics = copy(metrics = _root_.scala.Seq.empty)
def addMetrics(__vs: com.google.api.metric.MetricDescriptor *): Service = addAllMetrics(__vs)
def addAllMetrics(__vs: Iterable[com.google.api.metric.MetricDescriptor]): Service = copy(metrics = metrics ++ __vs)
def withMetrics(__v: _root_.scala.Seq[com.google.api.metric.MetricDescriptor]): Service = copy(metrics = __v)
def clearMonitoredResources = copy(monitoredResources = _root_.scala.Seq.empty)
def addMonitoredResources(__vs: com.google.api.monitored_resource.MonitoredResourceDescriptor *): Service = addAllMonitoredResources(__vs)
def addAllMonitoredResources(__vs: Iterable[com.google.api.monitored_resource.MonitoredResourceDescriptor]): Service = copy(monitoredResources = monitoredResources ++ __vs)
def withMonitoredResources(__v: _root_.scala.Seq[com.google.api.monitored_resource.MonitoredResourceDescriptor]): Service = copy(monitoredResources = __v)
def getBilling: com.google.api.billing.Billing = billing.getOrElse(com.google.api.billing.Billing.defaultInstance)
def clearBilling: Service = copy(billing = _root_.scala.None)
def withBilling(__v: com.google.api.billing.Billing): Service = copy(billing = Option(__v))
def getLogging: com.google.api.logging.Logging = logging.getOrElse(com.google.api.logging.Logging.defaultInstance)
def clearLogging: Service = copy(logging = _root_.scala.None)
def withLogging(__v: com.google.api.logging.Logging): Service = copy(logging = Option(__v))
def getMonitoring: com.google.api.monitoring.Monitoring = monitoring.getOrElse(com.google.api.monitoring.Monitoring.defaultInstance)
def clearMonitoring: Service = copy(monitoring = _root_.scala.None)
def withMonitoring(__v: com.google.api.monitoring.Monitoring): Service = copy(monitoring = Option(__v))
def getSystemParameters: com.google.api.system_parameter.SystemParameters = systemParameters.getOrElse(com.google.api.system_parameter.SystemParameters.defaultInstance)
def clearSystemParameters: Service = copy(systemParameters = _root_.scala.None)
def withSystemParameters(__v: com.google.api.system_parameter.SystemParameters): Service = copy(systemParameters = Option(__v))
def getSourceInfo: com.google.api.source_info.SourceInfo = sourceInfo.getOrElse(com.google.api.source_info.SourceInfo.defaultInstance)
def clearSourceInfo: Service = copy(sourceInfo = _root_.scala.None)
def withSourceInfo(__v: com.google.api.source_info.SourceInfo): Service = copy(sourceInfo = Option(__v))
def getPublishing: com.google.api.client.Publishing = publishing.getOrElse(com.google.api.client.Publishing.defaultInstance)
def clearPublishing: Service = copy(publishing = _root_.scala.None)
def withPublishing(__v: com.google.api.client.Publishing): Service = copy(publishing = Option(__v))
def getConfigVersion: _root_.scala.Int = configVersion.getOrElse(com.google.api.service.Service._typemapper_configVersion.toCustom(com.google.protobuf.wrappers.UInt32Value.defaultInstance))
def clearConfigVersion: Service = copy(configVersion = _root_.scala.None)
def withConfigVersion(__v: _root_.scala.Int): Service = copy(configVersion = Option(__v))
def withUnknownFields(__v: _root_.scalapb.UnknownFieldSet) = copy(unknownFields = __v)
def discardUnknownFields = copy(unknownFields = _root_.scalapb.UnknownFieldSet.empty)
def getFieldByNumber(__fieldNumber: _root_.scala.Int): _root_.scala.Any = {
(__fieldNumber: @_root_.scala.unchecked) match {
case 1 => {
val __t = name
if (__t != "") __t else null
}
case 2 => {
val __t = title
if (__t != "") __t else null
}
case 22 => {
val __t = producerProjectId
if (__t != "") __t else null
}
case 33 => {
val __t = id
if (__t != "") __t else null
}
case 3 => apis
case 4 => types
case 5 => enums
case 6 => documentation.orNull
case 8 => backend.orNull
case 9 => http.orNull
case 10 => quota.orNull
case 11 => authentication.orNull
case 12 => context.orNull
case 15 => usage.orNull
case 18 => endpoints
case 21 => control.orNull
case 23 => logs
case 24 => metrics
case 25 => monitoredResources
case 26 => billing.orNull
case 27 => logging.orNull
case 28 => monitoring.orNull
case 29 => systemParameters.orNull
case 37 => sourceInfo.orNull
case 45 => publishing.orNull
case 20 => configVersion.map(com.google.api.service.Service._typemapper_configVersion.toBase(_)).orNull
}
}
def getField(__field: _root_.scalapb.descriptors.FieldDescriptor): _root_.scalapb.descriptors.PValue = {
_root_.scala.Predef.require(__field.containingMessage eq companion.scalaDescriptor)
(__field.number: @_root_.scala.unchecked) match {
case 1 => _root_.scalapb.descriptors.PString(name)
case 2 => _root_.scalapb.descriptors.PString(title)
case 22 => _root_.scalapb.descriptors.PString(producerProjectId)
case 33 => _root_.scalapb.descriptors.PString(id)
case 3 => _root_.scalapb.descriptors.PRepeated(apis.iterator.map(_.toPMessage).toVector)
case 4 => _root_.scalapb.descriptors.PRepeated(types.iterator.map(_.toPMessage).toVector)
case 5 => _root_.scalapb.descriptors.PRepeated(enums.iterator.map(_.toPMessage).toVector)
case 6 => documentation.map(_.toPMessage).getOrElse(_root_.scalapb.descriptors.PEmpty)
case 8 => backend.map(_.toPMessage).getOrElse(_root_.scalapb.descriptors.PEmpty)
case 9 => http.map(_.toPMessage).getOrElse(_root_.scalapb.descriptors.PEmpty)
case 10 => quota.map(_.toPMessage).getOrElse(_root_.scalapb.descriptors.PEmpty)
case 11 => authentication.map(_.toPMessage).getOrElse(_root_.scalapb.descriptors.PEmpty)
case 12 => context.map(_.toPMessage).getOrElse(_root_.scalapb.descriptors.PEmpty)
case 15 => usage.map(_.toPMessage).getOrElse(_root_.scalapb.descriptors.PEmpty)
case 18 => _root_.scalapb.descriptors.PRepeated(endpoints.iterator.map(_.toPMessage).toVector)
case 21 => control.map(_.toPMessage).getOrElse(_root_.scalapb.descriptors.PEmpty)
case 23 => _root_.scalapb.descriptors.PRepeated(logs.iterator.map(_.toPMessage).toVector)
case 24 => _root_.scalapb.descriptors.PRepeated(metrics.iterator.map(_.toPMessage).toVector)
case 25 => _root_.scalapb.descriptors.PRepeated(monitoredResources.iterator.map(_.toPMessage).toVector)
case 26 => billing.map(_.toPMessage).getOrElse(_root_.scalapb.descriptors.PEmpty)
case 27 => logging.map(_.toPMessage).getOrElse(_root_.scalapb.descriptors.PEmpty)
case 28 => monitoring.map(_.toPMessage).getOrElse(_root_.scalapb.descriptors.PEmpty)
case 29 => systemParameters.map(_.toPMessage).getOrElse(_root_.scalapb.descriptors.PEmpty)
case 37 => sourceInfo.map(_.toPMessage).getOrElse(_root_.scalapb.descriptors.PEmpty)
case 45 => publishing.map(_.toPMessage).getOrElse(_root_.scalapb.descriptors.PEmpty)
case 20 => configVersion.map(com.google.api.service.Service._typemapper_configVersion.toBase(_).toPMessage).getOrElse(_root_.scalapb.descriptors.PEmpty)
}
}
def toProtoString: _root_.scala.Predef.String = _root_.scalapb.TextFormat.printToUnicodeString(this)
def companion: com.google.api.service.Service.type = com.google.api.service.Service
// @@protoc_insertion_point(GeneratedMessage[google.api.Service])
}
object Service extends scalapb.GeneratedMessageCompanion[com.google.api.service.Service] {
implicit def messageCompanion: scalapb.GeneratedMessageCompanion[com.google.api.service.Service] = this
def parseFrom(`_input__`: _root_.com.google.protobuf.CodedInputStream): com.google.api.service.Service = {
var __name: _root_.scala.Predef.String = ""
var __title: _root_.scala.Predef.String = ""
var __producerProjectId: _root_.scala.Predef.String = ""
var __id: _root_.scala.Predef.String = ""
val __apis: _root_.scala.collection.immutable.VectorBuilder[com.google.protobuf.api.Api] = new _root_.scala.collection.immutable.VectorBuilder[com.google.protobuf.api.Api]
val __types: _root_.scala.collection.immutable.VectorBuilder[com.google.protobuf.`type`.Type] = new _root_.scala.collection.immutable.VectorBuilder[com.google.protobuf.`type`.Type]
val __enums: _root_.scala.collection.immutable.VectorBuilder[com.google.protobuf.`type`.Enum] = new _root_.scala.collection.immutable.VectorBuilder[com.google.protobuf.`type`.Enum]
var __documentation: _root_.scala.Option[com.google.api.documentation.Documentation] = _root_.scala.None
var __backend: _root_.scala.Option[com.google.api.backend.Backend] = _root_.scala.None
var __http: _root_.scala.Option[com.google.api.http.Http] = _root_.scala.None
var __quota: _root_.scala.Option[com.google.api.quota.Quota] = _root_.scala.None
var __authentication: _root_.scala.Option[com.google.api.auth.Authentication] = _root_.scala.None
var __context: _root_.scala.Option[com.google.api.context.Context] = _root_.scala.None
var __usage: _root_.scala.Option[com.google.api.usage.Usage] = _root_.scala.None
val __endpoints: _root_.scala.collection.immutable.VectorBuilder[com.google.api.endpoint.Endpoint] = new _root_.scala.collection.immutable.VectorBuilder[com.google.api.endpoint.Endpoint]
var __control: _root_.scala.Option[com.google.api.control.Control] = _root_.scala.None
val __logs: _root_.scala.collection.immutable.VectorBuilder[com.google.api.log.LogDescriptor] = new _root_.scala.collection.immutable.VectorBuilder[com.google.api.log.LogDescriptor]
val __metrics: _root_.scala.collection.immutable.VectorBuilder[com.google.api.metric.MetricDescriptor] = new _root_.scala.collection.immutable.VectorBuilder[com.google.api.metric.MetricDescriptor]
val __monitoredResources: _root_.scala.collection.immutable.VectorBuilder[com.google.api.monitored_resource.MonitoredResourceDescriptor] = new _root_.scala.collection.immutable.VectorBuilder[com.google.api.monitored_resource.MonitoredResourceDescriptor]
var __billing: _root_.scala.Option[com.google.api.billing.Billing] = _root_.scala.None
var __logging: _root_.scala.Option[com.google.api.logging.Logging] = _root_.scala.None
var __monitoring: _root_.scala.Option[com.google.api.monitoring.Monitoring] = _root_.scala.None
var __systemParameters: _root_.scala.Option[com.google.api.system_parameter.SystemParameters] = _root_.scala.None
var __sourceInfo: _root_.scala.Option[com.google.api.source_info.SourceInfo] = _root_.scala.None
var __publishing: _root_.scala.Option[com.google.api.client.Publishing] = _root_.scala.None
var __configVersion: _root_.scala.Option[_root_.scala.Int] = _root_.scala.None
var `_unknownFields__`: _root_.scalapb.UnknownFieldSet.Builder = null
var _done__ = false
while (!_done__) {
val _tag__ = _input__.readTag()
_tag__ match {
case 0 => _done__ = true
case 10 =>
__name = _input__.readStringRequireUtf8()
case 18 =>
__title = _input__.readStringRequireUtf8()
case 178 =>
__producerProjectId = _input__.readStringRequireUtf8()
case 266 =>
__id = _input__.readStringRequireUtf8()
case 26 =>
__apis += _root_.scalapb.LiteParser.readMessage[com.google.protobuf.api.Api](_input__)
case 34 =>
__types += _root_.scalapb.LiteParser.readMessage[com.google.protobuf.`type`.Type](_input__)
case 42 =>
__enums += _root_.scalapb.LiteParser.readMessage[com.google.protobuf.`type`.Enum](_input__)
case 50 =>
__documentation = _root_.scala.Option(__documentation.fold(_root_.scalapb.LiteParser.readMessage[com.google.api.documentation.Documentation](_input__))(_root_.scalapb.LiteParser.readMessage(_input__, _)))
case 66 =>
__backend = _root_.scala.Option(__backend.fold(_root_.scalapb.LiteParser.readMessage[com.google.api.backend.Backend](_input__))(_root_.scalapb.LiteParser.readMessage(_input__, _)))
case 74 =>
__http = _root_.scala.Option(__http.fold(_root_.scalapb.LiteParser.readMessage[com.google.api.http.Http](_input__))(_root_.scalapb.LiteParser.readMessage(_input__, _)))
case 82 =>
__quota = _root_.scala.Option(__quota.fold(_root_.scalapb.LiteParser.readMessage[com.google.api.quota.Quota](_input__))(_root_.scalapb.LiteParser.readMessage(_input__, _)))
case 90 =>
__authentication = _root_.scala.Option(__authentication.fold(_root_.scalapb.LiteParser.readMessage[com.google.api.auth.Authentication](_input__))(_root_.scalapb.LiteParser.readMessage(_input__, _)))
case 98 =>
__context = _root_.scala.Option(__context.fold(_root_.scalapb.LiteParser.readMessage[com.google.api.context.Context](_input__))(_root_.scalapb.LiteParser.readMessage(_input__, _)))
case 122 =>
__usage = _root_.scala.Option(__usage.fold(_root_.scalapb.LiteParser.readMessage[com.google.api.usage.Usage](_input__))(_root_.scalapb.LiteParser.readMessage(_input__, _)))
case 146 =>
__endpoints += _root_.scalapb.LiteParser.readMessage[com.google.api.endpoint.Endpoint](_input__)
case 170 =>
__control = _root_.scala.Option(__control.fold(_root_.scalapb.LiteParser.readMessage[com.google.api.control.Control](_input__))(_root_.scalapb.LiteParser.readMessage(_input__, _)))
case 186 =>
__logs += _root_.scalapb.LiteParser.readMessage[com.google.api.log.LogDescriptor](_input__)
case 194 =>
__metrics += _root_.scalapb.LiteParser.readMessage[com.google.api.metric.MetricDescriptor](_input__)
case 202 =>
__monitoredResources += _root_.scalapb.LiteParser.readMessage[com.google.api.monitored_resource.MonitoredResourceDescriptor](_input__)
case 210 =>
__billing = _root_.scala.Option(__billing.fold(_root_.scalapb.LiteParser.readMessage[com.google.api.billing.Billing](_input__))(_root_.scalapb.LiteParser.readMessage(_input__, _)))
case 218 =>
__logging = _root_.scala.Option(__logging.fold(_root_.scalapb.LiteParser.readMessage[com.google.api.logging.Logging](_input__))(_root_.scalapb.LiteParser.readMessage(_input__, _)))
case 226 =>
__monitoring = _root_.scala.Option(__monitoring.fold(_root_.scalapb.LiteParser.readMessage[com.google.api.monitoring.Monitoring](_input__))(_root_.scalapb.LiteParser.readMessage(_input__, _)))
case 234 =>
__systemParameters = _root_.scala.Option(__systemParameters.fold(_root_.scalapb.LiteParser.readMessage[com.google.api.system_parameter.SystemParameters](_input__))(_root_.scalapb.LiteParser.readMessage(_input__, _)))
case 298 =>
__sourceInfo = _root_.scala.Option(__sourceInfo.fold(_root_.scalapb.LiteParser.readMessage[com.google.api.source_info.SourceInfo](_input__))(_root_.scalapb.LiteParser.readMessage(_input__, _)))
case 362 =>
__publishing = _root_.scala.Option(__publishing.fold(_root_.scalapb.LiteParser.readMessage[com.google.api.client.Publishing](_input__))(_root_.scalapb.LiteParser.readMessage(_input__, _)))
case 162 =>
__configVersion = _root_.scala.Option(com.google.api.service.Service._typemapper_configVersion.toCustom(__configVersion.map(com.google.api.service.Service._typemapper_configVersion.toBase(_)).fold(_root_.scalapb.LiteParser.readMessage[com.google.protobuf.wrappers.UInt32Value](_input__))(_root_.scalapb.LiteParser.readMessage(_input__, _))))
case tag =>
if (_unknownFields__ == null) {
_unknownFields__ = new _root_.scalapb.UnknownFieldSet.Builder()
}
_unknownFields__.parseField(tag, _input__)
}
}
com.google.api.service.Service(
name = __name,
title = __title,
producerProjectId = __producerProjectId,
id = __id,
apis = __apis.result(),
types = __types.result(),
enums = __enums.result(),
documentation = __documentation,
backend = __backend,
http = __http,
quota = __quota,
authentication = __authentication,
context = __context,
usage = __usage,
endpoints = __endpoints.result(),
control = __control,
logs = __logs.result(),
metrics = __metrics.result(),
monitoredResources = __monitoredResources.result(),
billing = __billing,
logging = __logging,
monitoring = __monitoring,
systemParameters = __systemParameters,
sourceInfo = __sourceInfo,
publishing = __publishing,
configVersion = __configVersion,
unknownFields = if (_unknownFields__ == null) _root_.scalapb.UnknownFieldSet.empty else _unknownFields__.result()
)
}
implicit def messageReads: _root_.scalapb.descriptors.Reads[com.google.api.service.Service] = _root_.scalapb.descriptors.Reads{
case _root_.scalapb.descriptors.PMessage(__fieldsMap) =>
_root_.scala.Predef.require(__fieldsMap.keys.forall(_.containingMessage eq scalaDescriptor), "FieldDescriptor does not match message type.")
com.google.api.service.Service(
name = __fieldsMap.get(scalaDescriptor.findFieldByNumber(1).get).map(_.as[_root_.scala.Predef.String]).getOrElse(""),
title = __fieldsMap.get(scalaDescriptor.findFieldByNumber(2).get).map(_.as[_root_.scala.Predef.String]).getOrElse(""),
producerProjectId = __fieldsMap.get(scalaDescriptor.findFieldByNumber(22).get).map(_.as[_root_.scala.Predef.String]).getOrElse(""),
id = __fieldsMap.get(scalaDescriptor.findFieldByNumber(33).get).map(_.as[_root_.scala.Predef.String]).getOrElse(""),
apis = __fieldsMap.get(scalaDescriptor.findFieldByNumber(3).get).map(_.as[_root_.scala.Seq[com.google.protobuf.api.Api]]).getOrElse(_root_.scala.Seq.empty),
types = __fieldsMap.get(scalaDescriptor.findFieldByNumber(4).get).map(_.as[_root_.scala.Seq[com.google.protobuf.`type`.Type]]).getOrElse(_root_.scala.Seq.empty),
enums = __fieldsMap.get(scalaDescriptor.findFieldByNumber(5).get).map(_.as[_root_.scala.Seq[com.google.protobuf.`type`.Enum]]).getOrElse(_root_.scala.Seq.empty),
documentation = __fieldsMap.get(scalaDescriptor.findFieldByNumber(6).get).flatMap(_.as[_root_.scala.Option[com.google.api.documentation.Documentation]]),
backend = __fieldsMap.get(scalaDescriptor.findFieldByNumber(8).get).flatMap(_.as[_root_.scala.Option[com.google.api.backend.Backend]]),
http = __fieldsMap.get(scalaDescriptor.findFieldByNumber(9).get).flatMap(_.as[_root_.scala.Option[com.google.api.http.Http]]),
quota = __fieldsMap.get(scalaDescriptor.findFieldByNumber(10).get).flatMap(_.as[_root_.scala.Option[com.google.api.quota.Quota]]),
authentication = __fieldsMap.get(scalaDescriptor.findFieldByNumber(11).get).flatMap(_.as[_root_.scala.Option[com.google.api.auth.Authentication]]),
context = __fieldsMap.get(scalaDescriptor.findFieldByNumber(12).get).flatMap(_.as[_root_.scala.Option[com.google.api.context.Context]]),
usage = __fieldsMap.get(scalaDescriptor.findFieldByNumber(15).get).flatMap(_.as[_root_.scala.Option[com.google.api.usage.Usage]]),
endpoints = __fieldsMap.get(scalaDescriptor.findFieldByNumber(18).get).map(_.as[_root_.scala.Seq[com.google.api.endpoint.Endpoint]]).getOrElse(_root_.scala.Seq.empty),
control = __fieldsMap.get(scalaDescriptor.findFieldByNumber(21).get).flatMap(_.as[_root_.scala.Option[com.google.api.control.Control]]),
logs = __fieldsMap.get(scalaDescriptor.findFieldByNumber(23).get).map(_.as[_root_.scala.Seq[com.google.api.log.LogDescriptor]]).getOrElse(_root_.scala.Seq.empty),
metrics = __fieldsMap.get(scalaDescriptor.findFieldByNumber(24).get).map(_.as[_root_.scala.Seq[com.google.api.metric.MetricDescriptor]]).getOrElse(_root_.scala.Seq.empty),
monitoredResources = __fieldsMap.get(scalaDescriptor.findFieldByNumber(25).get).map(_.as[_root_.scala.Seq[com.google.api.monitored_resource.MonitoredResourceDescriptor]]).getOrElse(_root_.scala.Seq.empty),
billing = __fieldsMap.get(scalaDescriptor.findFieldByNumber(26).get).flatMap(_.as[_root_.scala.Option[com.google.api.billing.Billing]]),
logging = __fieldsMap.get(scalaDescriptor.findFieldByNumber(27).get).flatMap(_.as[_root_.scala.Option[com.google.api.logging.Logging]]),
monitoring = __fieldsMap.get(scalaDescriptor.findFieldByNumber(28).get).flatMap(_.as[_root_.scala.Option[com.google.api.monitoring.Monitoring]]),
systemParameters = __fieldsMap.get(scalaDescriptor.findFieldByNumber(29).get).flatMap(_.as[_root_.scala.Option[com.google.api.system_parameter.SystemParameters]]),
sourceInfo = __fieldsMap.get(scalaDescriptor.findFieldByNumber(37).get).flatMap(_.as[_root_.scala.Option[com.google.api.source_info.SourceInfo]]),
publishing = __fieldsMap.get(scalaDescriptor.findFieldByNumber(45).get).flatMap(_.as[_root_.scala.Option[com.google.api.client.Publishing]]),
configVersion = __fieldsMap.get(scalaDescriptor.findFieldByNumber(20).get).flatMap(_.as[_root_.scala.Option[com.google.protobuf.wrappers.UInt32Value]]).map(com.google.api.service.Service._typemapper_configVersion.toCustom(_))
)
case _ => throw new RuntimeException("Expected PMessage")
}
def javaDescriptor: _root_.com.google.protobuf.Descriptors.Descriptor = ServiceProto.javaDescriptor.getMessageTypes().get(0)
def scalaDescriptor: _root_.scalapb.descriptors.Descriptor = ServiceProto.scalaDescriptor.messages(0)
def messageCompanionForFieldNumber(__number: _root_.scala.Int): _root_.scalapb.GeneratedMessageCompanion[_] = {
var __out: _root_.scalapb.GeneratedMessageCompanion[_] = null
(__number: @_root_.scala.unchecked) match {
case 3 => __out = com.google.protobuf.api.Api
case 4 => __out = com.google.protobuf.`type`.Type
case 5 => __out = com.google.protobuf.`type`.Enum
case 6 => __out = com.google.api.documentation.Documentation
case 8 => __out = com.google.api.backend.Backend
case 9 => __out = com.google.api.http.Http
case 10 => __out = com.google.api.quota.Quota
case 11 => __out = com.google.api.auth.Authentication
case 12 => __out = com.google.api.context.Context
case 15 => __out = com.google.api.usage.Usage
case 18 => __out = com.google.api.endpoint.Endpoint
case 21 => __out = com.google.api.control.Control
case 23 => __out = com.google.api.log.LogDescriptor
case 24 => __out = com.google.api.metric.MetricDescriptor
case 25 => __out = com.google.api.monitored_resource.MonitoredResourceDescriptor
case 26 => __out = com.google.api.billing.Billing
case 27 => __out = com.google.api.logging.Logging
case 28 => __out = com.google.api.monitoring.Monitoring
case 29 => __out = com.google.api.system_parameter.SystemParameters
case 37 => __out = com.google.api.source_info.SourceInfo
case 45 => __out = com.google.api.client.Publishing
case 20 => __out = com.google.protobuf.wrappers.UInt32Value
}
__out
}
lazy val nestedMessagesCompanions: Seq[_root_.scalapb.GeneratedMessageCompanion[_ <: _root_.scalapb.GeneratedMessage]] = Seq.empty
def enumCompanionForFieldNumber(__fieldNumber: _root_.scala.Int): _root_.scalapb.GeneratedEnumCompanion[_] = throw new MatchError(__fieldNumber)
lazy val defaultInstance = com.google.api.service.Service(
name = "",
title = "",
producerProjectId = "",
id = "",
apis = _root_.scala.Seq.empty,
types = _root_.scala.Seq.empty,
enums = _root_.scala.Seq.empty,
documentation = _root_.scala.None,
backend = _root_.scala.None,
http = _root_.scala.None,
quota = _root_.scala.None,
authentication = _root_.scala.None,
context = _root_.scala.None,
usage = _root_.scala.None,
endpoints = _root_.scala.Seq.empty,
control = _root_.scala.None,
logs = _root_.scala.Seq.empty,
metrics = _root_.scala.Seq.empty,
monitoredResources = _root_.scala.Seq.empty,
billing = _root_.scala.None,
logging = _root_.scala.None,
monitoring = _root_.scala.None,
systemParameters = _root_.scala.None,
sourceInfo = _root_.scala.None,
publishing = _root_.scala.None,
configVersion = _root_.scala.None
)
implicit class ServiceLens[UpperPB](_l: _root_.scalapb.lenses.Lens[UpperPB, com.google.api.service.Service]) extends _root_.scalapb.lenses.ObjectLens[UpperPB, com.google.api.service.Service](_l) {
def name: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Predef.String] = field(_.name)((c_, f_) => c_.copy(name = f_))
def title: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Predef.String] = field(_.title)((c_, f_) => c_.copy(title = f_))
def producerProjectId: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Predef.String] = field(_.producerProjectId)((c_, f_) => c_.copy(producerProjectId = f_))
def id: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Predef.String] = field(_.id)((c_, f_) => c_.copy(id = f_))
def apis: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Seq[com.google.protobuf.api.Api]] = field(_.apis)((c_, f_) => c_.copy(apis = f_))
def types: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Seq[com.google.protobuf.`type`.Type]] = field(_.types)((c_, f_) => c_.copy(types = f_))
def enums: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Seq[com.google.protobuf.`type`.Enum]] = field(_.enums)((c_, f_) => c_.copy(enums = f_))
def documentation: _root_.scalapb.lenses.Lens[UpperPB, com.google.api.documentation.Documentation] = field(_.getDocumentation)((c_, f_) => c_.copy(documentation = _root_.scala.Option(f_)))
def optionalDocumentation: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Option[com.google.api.documentation.Documentation]] = field(_.documentation)((c_, f_) => c_.copy(documentation = f_))
def backend: _root_.scalapb.lenses.Lens[UpperPB, com.google.api.backend.Backend] = field(_.getBackend)((c_, f_) => c_.copy(backend = _root_.scala.Option(f_)))
def optionalBackend: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Option[com.google.api.backend.Backend]] = field(_.backend)((c_, f_) => c_.copy(backend = f_))
def http: _root_.scalapb.lenses.Lens[UpperPB, com.google.api.http.Http] = field(_.getHttp)((c_, f_) => c_.copy(http = _root_.scala.Option(f_)))
def optionalHttp: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Option[com.google.api.http.Http]] = field(_.http)((c_, f_) => c_.copy(http = f_))
def quota: _root_.scalapb.lenses.Lens[UpperPB, com.google.api.quota.Quota] = field(_.getQuota)((c_, f_) => c_.copy(quota = _root_.scala.Option(f_)))
def optionalQuota: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Option[com.google.api.quota.Quota]] = field(_.quota)((c_, f_) => c_.copy(quota = f_))
def authentication: _root_.scalapb.lenses.Lens[UpperPB, com.google.api.auth.Authentication] = field(_.getAuthentication)((c_, f_) => c_.copy(authentication = _root_.scala.Option(f_)))
def optionalAuthentication: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Option[com.google.api.auth.Authentication]] = field(_.authentication)((c_, f_) => c_.copy(authentication = f_))
def context: _root_.scalapb.lenses.Lens[UpperPB, com.google.api.context.Context] = field(_.getContext)((c_, f_) => c_.copy(context = _root_.scala.Option(f_)))
def optionalContext: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Option[com.google.api.context.Context]] = field(_.context)((c_, f_) => c_.copy(context = f_))
def usage: _root_.scalapb.lenses.Lens[UpperPB, com.google.api.usage.Usage] = field(_.getUsage)((c_, f_) => c_.copy(usage = _root_.scala.Option(f_)))
def optionalUsage: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Option[com.google.api.usage.Usage]] = field(_.usage)((c_, f_) => c_.copy(usage = f_))
def endpoints: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Seq[com.google.api.endpoint.Endpoint]] = field(_.endpoints)((c_, f_) => c_.copy(endpoints = f_))
def control: _root_.scalapb.lenses.Lens[UpperPB, com.google.api.control.Control] = field(_.getControl)((c_, f_) => c_.copy(control = _root_.scala.Option(f_)))
def optionalControl: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Option[com.google.api.control.Control]] = field(_.control)((c_, f_) => c_.copy(control = f_))
def logs: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Seq[com.google.api.log.LogDescriptor]] = field(_.logs)((c_, f_) => c_.copy(logs = f_))
def metrics: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Seq[com.google.api.metric.MetricDescriptor]] = field(_.metrics)((c_, f_) => c_.copy(metrics = f_))
def monitoredResources: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Seq[com.google.api.monitored_resource.MonitoredResourceDescriptor]] = field(_.monitoredResources)((c_, f_) => c_.copy(monitoredResources = f_))
def billing: _root_.scalapb.lenses.Lens[UpperPB, com.google.api.billing.Billing] = field(_.getBilling)((c_, f_) => c_.copy(billing = _root_.scala.Option(f_)))
def optionalBilling: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Option[com.google.api.billing.Billing]] = field(_.billing)((c_, f_) => c_.copy(billing = f_))
def logging: _root_.scalapb.lenses.Lens[UpperPB, com.google.api.logging.Logging] = field(_.getLogging)((c_, f_) => c_.copy(logging = _root_.scala.Option(f_)))
def optionalLogging: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Option[com.google.api.logging.Logging]] = field(_.logging)((c_, f_) => c_.copy(logging = f_))
def monitoring: _root_.scalapb.lenses.Lens[UpperPB, com.google.api.monitoring.Monitoring] = field(_.getMonitoring)((c_, f_) => c_.copy(monitoring = _root_.scala.Option(f_)))
def optionalMonitoring: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Option[com.google.api.monitoring.Monitoring]] = field(_.monitoring)((c_, f_) => c_.copy(monitoring = f_))
def systemParameters: _root_.scalapb.lenses.Lens[UpperPB, com.google.api.system_parameter.SystemParameters] = field(_.getSystemParameters)((c_, f_) => c_.copy(systemParameters = _root_.scala.Option(f_)))
def optionalSystemParameters: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Option[com.google.api.system_parameter.SystemParameters]] = field(_.systemParameters)((c_, f_) => c_.copy(systemParameters = f_))
def sourceInfo: _root_.scalapb.lenses.Lens[UpperPB, com.google.api.source_info.SourceInfo] = field(_.getSourceInfo)((c_, f_) => c_.copy(sourceInfo = _root_.scala.Option(f_)))
def optionalSourceInfo: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Option[com.google.api.source_info.SourceInfo]] = field(_.sourceInfo)((c_, f_) => c_.copy(sourceInfo = f_))
def publishing: _root_.scalapb.lenses.Lens[UpperPB, com.google.api.client.Publishing] = field(_.getPublishing)((c_, f_) => c_.copy(publishing = _root_.scala.Option(f_)))
def optionalPublishing: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Option[com.google.api.client.Publishing]] = field(_.publishing)((c_, f_) => c_.copy(publishing = f_))
def configVersion: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Int] = field(_.getConfigVersion)((c_, f_) => c_.copy(configVersion = _root_.scala.Option(f_)))
def optionalConfigVersion: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Option[_root_.scala.Int]] = field(_.configVersion)((c_, f_) => c_.copy(configVersion = f_))
}
final val NAME_FIELD_NUMBER = 1
final val TITLE_FIELD_NUMBER = 2
final val PRODUCER_PROJECT_ID_FIELD_NUMBER = 22
final val ID_FIELD_NUMBER = 33
final val APIS_FIELD_NUMBER = 3
final val TYPES_FIELD_NUMBER = 4
final val ENUMS_FIELD_NUMBER = 5
final val DOCUMENTATION_FIELD_NUMBER = 6
final val BACKEND_FIELD_NUMBER = 8
final val HTTP_FIELD_NUMBER = 9
final val QUOTA_FIELD_NUMBER = 10
final val AUTHENTICATION_FIELD_NUMBER = 11
final val CONTEXT_FIELD_NUMBER = 12
final val USAGE_FIELD_NUMBER = 15
final val ENDPOINTS_FIELD_NUMBER = 18
final val CONTROL_FIELD_NUMBER = 21
final val LOGS_FIELD_NUMBER = 23
final val METRICS_FIELD_NUMBER = 24
final val MONITORED_RESOURCES_FIELD_NUMBER = 25
final val BILLING_FIELD_NUMBER = 26
final val LOGGING_FIELD_NUMBER = 27
final val MONITORING_FIELD_NUMBER = 28
final val SYSTEM_PARAMETERS_FIELD_NUMBER = 29
final val SOURCE_INFO_FIELD_NUMBER = 37
final val PUBLISHING_FIELD_NUMBER = 45
final val CONFIG_VERSION_FIELD_NUMBER = 20
@transient
private[service] val _typemapper_configVersion: _root_.scalapb.TypeMapper[com.google.protobuf.wrappers.UInt32Value, _root_.scala.Int] = implicitly[_root_.scalapb.TypeMapper[com.google.protobuf.wrappers.UInt32Value, _root_.scala.Int]]
def of(
name: _root_.scala.Predef.String,
title: _root_.scala.Predef.String,
producerProjectId: _root_.scala.Predef.String,
id: _root_.scala.Predef.String,
apis: _root_.scala.Seq[com.google.protobuf.api.Api],
types: _root_.scala.Seq[com.google.protobuf.`type`.Type],
enums: _root_.scala.Seq[com.google.protobuf.`type`.Enum],
documentation: _root_.scala.Option[com.google.api.documentation.Documentation],
backend: _root_.scala.Option[com.google.api.backend.Backend],
http: _root_.scala.Option[com.google.api.http.Http],
quota: _root_.scala.Option[com.google.api.quota.Quota],
authentication: _root_.scala.Option[com.google.api.auth.Authentication],
context: _root_.scala.Option[com.google.api.context.Context],
usage: _root_.scala.Option[com.google.api.usage.Usage],
endpoints: _root_.scala.Seq[com.google.api.endpoint.Endpoint],
control: _root_.scala.Option[com.google.api.control.Control],
logs: _root_.scala.Seq[com.google.api.log.LogDescriptor],
metrics: _root_.scala.Seq[com.google.api.metric.MetricDescriptor],
monitoredResources: _root_.scala.Seq[com.google.api.monitored_resource.MonitoredResourceDescriptor],
billing: _root_.scala.Option[com.google.api.billing.Billing],
logging: _root_.scala.Option[com.google.api.logging.Logging],
monitoring: _root_.scala.Option[com.google.api.monitoring.Monitoring],
systemParameters: _root_.scala.Option[com.google.api.system_parameter.SystemParameters],
sourceInfo: _root_.scala.Option[com.google.api.source_info.SourceInfo],
publishing: _root_.scala.Option[com.google.api.client.Publishing],
configVersion: _root_.scala.Option[_root_.scala.Int]
): _root_.com.google.api.service.Service = _root_.com.google.api.service.Service(
name,
title,
producerProjectId,
id,
apis,
types,
enums,
documentation,
backend,
http,
quota,
authentication,
context,
usage,
endpoints,
control,
logs,
metrics,
monitoredResources,
billing,
logging,
monitoring,
systemParameters,
sourceInfo,
publishing,
configVersion
)
// @@protoc_insertion_point(GeneratedMessageCompanion[google.api.Service])
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy