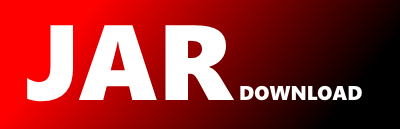
com.google.longrunning.Operations Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pekko-connectors-google-cloud-bigquery-storage_3 Show documentation
Show all versions of pekko-connectors-google-cloud-bigquery-storage_3 Show documentation
Apache Pekko Connectors is a Reactive Enterprise Integration library for Java and Scala, based on Reactive Streams and Pekko.
The newest version!
// Generated by Pekko gRPC. DO NOT EDIT.
package com.google.longrunning;
import org.apache.pekko.grpc.ProtobufSerializer;
import org.apache.pekko.grpc.javadsl.GoogleProtobufSerializer;
import org.apache.pekko.grpc.PekkoGrpcGenerated;
/**
* Manages long-running operations with an API service.
* When an API method normally takes long time to complete, it can be designed
* to return [Operation][google.longrunning.Operation] to the client, and the client can use this
* interface to receive the real response asynchronously by polling the
* operation resource, or pass the operation resource to another API (such as
* Google Cloud Pub/Sub API) to receive the response. Any API service that
* returns long-running operations should implement the `Operations` interface
* so developers can have a consistent client experience.
*/
public interface Operations {
/**
* Lists operations that match the specified filter in the request. If the
* server doesn't support this method, it returns `UNIMPLEMENTED`.
* NOTE: the `name` binding allows API services to override the binding
* to use different resource name schemes, such as `users/*/operations`. To
* override the binding, API services can add a binding such as
* `"/v1/{name=users/*}/operations"` to their service configuration.
* For backwards compatibility, the default name includes the operations
* collection id, however overriding users must ensure the name binding
* is the parent resource, without the operations collection id.
*/
java.util.concurrent.CompletionStage listOperations(com.google.longrunning.ListOperationsRequest in);
/**
* Gets the latest state of a long-running operation. Clients can use this
* method to poll the operation result at intervals as recommended by the API
* service.
*/
java.util.concurrent.CompletionStage getOperation(com.google.longrunning.GetOperationRequest in);
/**
* Deletes a long-running operation. This method indicates that the client is
* no longer interested in the operation result. It does not cancel the
* operation. If the server doesn't support this method, it returns
* `google.rpc.Code.UNIMPLEMENTED`.
*/
java.util.concurrent.CompletionStage deleteOperation(com.google.longrunning.DeleteOperationRequest in);
/**
* Starts asynchronous cancellation on a long-running operation. The server
* makes a best effort to cancel the operation, but success is not
* guaranteed. If the server doesn't support this method, it returns
* `google.rpc.Code.UNIMPLEMENTED`. Clients can use
* [Operations.GetOperation][google.longrunning.Operations.GetOperation] or
* other methods to check whether the cancellation succeeded or whether the
* operation completed despite cancellation. On successful cancellation,
* the operation is not deleted; instead, it becomes an operation with
* an [Operation.error][google.longrunning.Operation.error] value with a [google.rpc.Status.code][google.rpc.Status.code] of 1,
* corresponding to `Code.CANCELLED`.
*/
java.util.concurrent.CompletionStage cancelOperation(com.google.longrunning.CancelOperationRequest in);
/**
* Waits until the specified long-running operation is done or reaches at most
* a specified timeout, returning the latest state. If the operation is
* already done, the latest state is immediately returned. If the timeout
* specified is greater than the default HTTP/RPC timeout, the HTTP/RPC
* timeout is used. If the server does not support this method, it returns
* `google.rpc.Code.UNIMPLEMENTED`.
* Note that this method is on a best-effort basis. It may return the latest
* state before the specified timeout (including immediately), meaning even an
* immediate response is no guarantee that the operation is done.
*/
java.util.concurrent.CompletionStage waitOperation(com.google.longrunning.WaitOperationRequest in);
static String name = "google.longrunning.Operations";
static org.apache.pekko.grpc.ServiceDescription description = new org.apache.pekko.grpc.internal.ServiceDescriptionImpl(name, OperationsProto.getDescriptor());
@PekkoGrpcGenerated
public static class Serializers {
public static ProtobufSerializer ListOperationsRequestSerializer = new GoogleProtobufSerializer<>(com.google.longrunning.ListOperationsRequest.parser());
public static ProtobufSerializer GetOperationRequestSerializer = new GoogleProtobufSerializer<>(com.google.longrunning.GetOperationRequest.parser());
public static ProtobufSerializer DeleteOperationRequestSerializer = new GoogleProtobufSerializer<>(com.google.longrunning.DeleteOperationRequest.parser());
public static ProtobufSerializer CancelOperationRequestSerializer = new GoogleProtobufSerializer<>(com.google.longrunning.CancelOperationRequest.parser());
public static ProtobufSerializer WaitOperationRequestSerializer = new GoogleProtobufSerializer<>(com.google.longrunning.WaitOperationRequest.parser());
public static ProtobufSerializer ListOperationsResponseSerializer = new GoogleProtobufSerializer<>(com.google.longrunning.ListOperationsResponse.parser());
public static ProtobufSerializer OperationSerializer = new GoogleProtobufSerializer<>(com.google.longrunning.Operation.parser());
public static ProtobufSerializer google_protobuf_EmptySerializer = new GoogleProtobufSerializer<>(com.google.protobuf.Empty.parser());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy