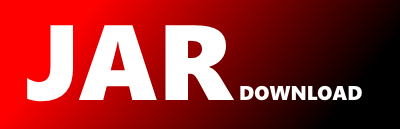
com.google.longrunning.OperationsPowerApiHandlerFactory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pekko-connectors-google-cloud-bigquery-storage_3 Show documentation
Show all versions of pekko-connectors-google-cloud-bigquery-storage_3 Show documentation
Apache Pekko Connectors is a Reactive Enterprise Integration library for Java and Scala, based on Reactive Streams and Pekko.
The newest version!
// Generated by Pekko gRPC. DO NOT EDIT.
package com.google.longrunning;
import java.util.Iterator;
import java.util.concurrent.CompletableFuture;
import java.util.concurrent.CompletionStage;
import org.apache.pekko.japi.function.Function;
import org.apache.pekko.actor.ActorSystem;
import org.apache.pekko.actor.ClassicActorSystemProvider;
import org.apache.pekko.annotation.ApiMayChange;
import org.apache.pekko.stream.Materializer;
import org.apache.pekko.stream.SystemMaterializer;
import org.apache.pekko.grpc.Trailers;
import org.apache.pekko.grpc.javadsl.GrpcMarshalling;
import org.apache.pekko.grpc.javadsl.GrpcExceptionHandler;
import org.apache.pekko.grpc.internal.TelemetryExtension;
import org.apache.pekko.grpc.internal.TelemetrySpi;
import org.apache.pekko.grpc.PekkoGrpcGenerated;
import org.apache.pekko.grpc.javadsl.Metadata;
import org.apache.pekko.grpc.javadsl.MetadataBuilder;
import static com.google.longrunning.Operations.Serializers.*;
/*
* Generated by Pekko gRPC. DO NOT EDIT.
*
* The API of this class may still change in future Apache Pekko gRPC versions, see for instance
* https://github.com/akka/akka-grpc/issues/994
*/
@ApiMayChange
@PekkoGrpcGenerated
public class OperationsPowerApiHandlerFactory {
private static final CompletionStage notFound = CompletableFuture.completedFuture(
org.apache.pekko.http.javadsl.model.HttpResponse.create().withStatus(org.apache.pekko.http.javadsl.model.StatusCodes.NOT_FOUND));
private static final CompletionStage unsupportedMediaType = CompletableFuture.completedFuture(
org.apache.pekko.http.javadsl.model.HttpResponse.create().withStatus(org.apache.pekko.http.javadsl.model.StatusCodes.UNSUPPORTED_MEDIA_TYPE));
/**
* Creates a `HttpRequest` to `HttpResponse` handler that can be used in for example `Http().bindAndHandleAsync`
* for the generated partial function handler and ends with `StatusCodes.NotFound` if the request is not matching.
*
* Use {@link org.apache.pekko.grpc.javadsl.ServiceHandler#concatOrNotFound} with {@link OperationsHandlerFactory#partial} when combining
* several services.
*/
public static Function> create(OperationsPowerApi implementation, ClassicActorSystemProvider system) {
return create(implementation, Operations.name, system);
}
/**
* Creates a `HttpRequest` to `HttpResponse` handler that can be used in for example `Http().bindAndHandleAsync`
* for the generated partial function handler and ends with `StatusCodes.NotFound` if the request is not matching.
*
* Use {@link org.apache.pekko.grpc.javadsl.ServiceHandler#concatOrNotFound} with {@link OperationsHandlerFactory#partial} when combining
* several services.
*/
public static Function> create(OperationsPowerApi implementation, org.apache.pekko.japi.Function> eHandler, ClassicActorSystemProvider system) {
return create(implementation, Operations.name, eHandler, system);
}
/**
* Creates a `HttpRequest` to `HttpResponse` handler that can be used in for example `Http().bindAndHandleAsync`
* for the generated partial function handler and ends with `StatusCodes.NotFound` if the request is not matching.
*
* Use {@link org.apache.pekko.grpc.javadsl.ServiceHandler#concatOrNotFound} with {@link OperationsHandlerFactory#partial} when combining
* several services.
*
* Registering a gRPC service under a custom prefix is not widely supported and strongly discouraged by the specification.
*/
public static Function> create(OperationsPowerApi implementation, String prefix, ClassicActorSystemProvider system) {
return partial(implementation, prefix, SystemMaterializer.get(system).materializer(), GrpcExceptionHandler.defaultMapper(), system);
}
/**
* Creates a `HttpRequest` to `HttpResponse` handler that can be used in for example `Http().bindAndHandleAsync`
* for the generated partial function handler and ends with `StatusCodes.NotFound` if the request is not matching.
*
* Use {@link org.apache.pekko.grpc.javadsl.ServiceHandler#concatOrNotFound} with {@link OperationsHandlerFactory#partial} when combining
* several services.
*
* Registering a gRPC service under a custom prefix is not widely supported and strongly discouraged by the specification.
*/
public static Function> create(OperationsPowerApi implementation, String prefix, org.apache.pekko.japi.Function> eHandler, ClassicActorSystemProvider system) {
return partial(implementation, prefix, SystemMaterializer.get(system).materializer(), eHandler, system);
}
/**
* Creates a `HttpRequest` to `HttpResponse` handler that can be used in for example `Http().bindAndHandleAsync`
* for the generated partial function handler. The generated handler falls back to a reflection handlers for
* `Operations` and ends with `StatusCodes.NotFound` if the request is not matching.
*
* Use {@link org.apache.pekko.grpc.javadsl.ServiceHandler#concatOrNotFound} with {@link OperationsHandlerFactory#partial} when combining
* several services.
*/
@SuppressWarnings("unchecked")
public static Function> createWithServerReflection(OperationsPowerApi implementation, ClassicActorSystemProvider system) {
return org.apache.pekko.grpc.javadsl.ServiceHandler.concatOrNotFound(
OperationsPowerApiHandlerFactory.create(implementation, system),
org.apache.pekko.grpc.javadsl.ServerReflection.create(
java.util.Collections.singletonList(Operations.description),
system));
}
/**
* Creates a `HttpRequest` to `HttpResponse` handler that can be used in for example
* `Http.get(system).bindAndHandleAsync`. It ends with `StatusCodes.NotFound` if the request is not matching.
*
* Use {@link org.apache.pekko.grpc.javadsl.ServiceHandler#concatOrNotFound} when combining several services.
*/
public static Function> partial(OperationsPowerApi implementation, String prefix, ClassicActorSystemProvider system) {
return partial(implementation, prefix, SystemMaterializer.get(system).materializer(), GrpcExceptionHandler.defaultMapper(), system);
}
/**
* Creates a `HttpRequest` to `HttpResponse` handler that can be used in for example
* `Http.get(system).bindAndHandleAsync`. It ends with `StatusCodes.NotFound` if the request is not matching.
*
* Use {@link org.apache.pekko.grpc.javadsl.ServiceHandler#concatOrNotFound} when combining several services.
*/
public static Function> partial(OperationsPowerApi implementation, String prefix, Materializer mat, org.apache.pekko.japi.Function> eHandler, ClassicActorSystemProvider system) {
TelemetrySpi spi = TelemetryExtension.get(system).spi();
return (req -> {
Iterator segments = req.getUri().pathSegments().iterator();
if (segments.hasNext() && segments.next().equals(prefix) && segments.hasNext()) {
String method = segments.next();
if (segments.hasNext()) return notFound; // we don't allow any random `/prefix/Method/anything/here
else {
return handle(spi.onRequest(prefix, method, req), method, implementation, mat, eHandler, system);
}
} else {
return notFound;
}
});
}
public String getServiceName() {
return Operations.name;
}
private static CompletionStage handle(org.apache.pekko.http.javadsl.model.HttpRequest request, String method, OperationsPowerApi implementation, Materializer mat, org.apache.pekko.japi.Function> eHandler, ClassicActorSystemProvider system) {
return GrpcMarshalling.negotiated(request, (reader, writer) -> {
final CompletionStage response;
Metadata metadata = MetadataBuilder.fromHeaders(request.getHeaders());
switch(method) {
case "ListOperations":
response = GrpcMarshalling.unmarshal(request.entity(), ListOperationsRequestSerializer, mat, reader)
.thenCompose(e -> implementation.listOperations(e, metadata))
.thenApply(e -> GrpcMarshalling.marshal(e, ListOperationsResponseSerializer, writer, system, eHandler));
break;
case "GetOperation":
response = GrpcMarshalling.unmarshal(request.entity(), GetOperationRequestSerializer, mat, reader)
.thenCompose(e -> implementation.getOperation(e, metadata))
.thenApply(e -> GrpcMarshalling.marshal(e, OperationSerializer, writer, system, eHandler));
break;
case "DeleteOperation":
response = GrpcMarshalling.unmarshal(request.entity(), DeleteOperationRequestSerializer, mat, reader)
.thenCompose(e -> implementation.deleteOperation(e, metadata))
.thenApply(e -> GrpcMarshalling.marshal(e, google_protobuf_EmptySerializer, writer, system, eHandler));
break;
case "CancelOperation":
response = GrpcMarshalling.unmarshal(request.entity(), CancelOperationRequestSerializer, mat, reader)
.thenCompose(e -> implementation.cancelOperation(e, metadata))
.thenApply(e -> GrpcMarshalling.marshal(e, google_protobuf_EmptySerializer, writer, system, eHandler));
break;
case "WaitOperation":
response = GrpcMarshalling.unmarshal(request.entity(), WaitOperationRequestSerializer, mat, reader)
.thenCompose(e -> implementation.waitOperation(e, metadata))
.thenApply(e -> GrpcMarshalling.marshal(e, OperationSerializer, writer, system, eHandler));
break;
default:
CompletableFuture result = new CompletableFuture<>();
result.completeExceptionally(new UnsupportedOperationException("Not implemented: " + method));
response = result;
}
return response.exceptionally(e -> GrpcExceptionHandler.standard(e, eHandler, writer, system));
})
.orElseGet(() -> unsupportedMediaType);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy