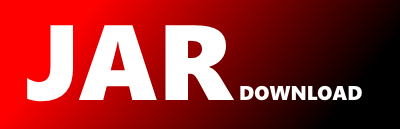
com.google.longrunning.operations.OperationsClient.scala Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pekko-connectors-google-cloud-bigquery-storage_3 Show documentation
Show all versions of pekko-connectors-google-cloud-bigquery-storage_3 Show documentation
Apache Pekko Connectors is a Reactive Enterprise Integration library for Java and Scala, based on Reactive Streams and Pekko.
The newest version!
// Generated by Pekko gRPC. DO NOT EDIT.
package com.google.longrunning.operations
import scala.concurrent.ExecutionContext
import org.apache.pekko
import pekko.actor.ClassicActorSystemProvider
import pekko.grpc.GrpcChannel
import pekko.grpc.GrpcClientCloseException
import pekko.grpc.GrpcClientSettings
import pekko.grpc.scaladsl.PekkoGrpcClient
import pekko.grpc.internal.NettyClientUtils
import pekko.grpc.PekkoGrpcGenerated
import pekko.grpc.scaladsl.SingleResponseRequestBuilder
import pekko.grpc.internal.ScalaUnaryRequestBuilder
// Not sealed so users can extend to write their stubs
@PekkoGrpcGenerated
trait OperationsClient extends Operations with OperationsClientPowerApi with PekkoGrpcClient
@PekkoGrpcGenerated
object OperationsClient {
def apply(settings: GrpcClientSettings)(implicit sys: ClassicActorSystemProvider): OperationsClient =
new DefaultOperationsClient(GrpcChannel(settings), isChannelOwned = true)
def apply(channel: GrpcChannel)(implicit sys: ClassicActorSystemProvider): OperationsClient =
new DefaultOperationsClient(channel, isChannelOwned = false)
private class DefaultOperationsClient(channel: GrpcChannel, isChannelOwned: Boolean)(implicit sys: ClassicActorSystemProvider) extends OperationsClient {
import Operations.MethodDescriptors._
private implicit val ex: ExecutionContext = sys.classicSystem.dispatcher
private val settings = channel.settings
private val options = NettyClientUtils.callOptions(settings)
private def listOperationsRequestBuilder(channel: pekko.grpc.internal.InternalChannel) =
new ScalaUnaryRequestBuilder(listOperationsDescriptor, channel, options, settings)
private def getOperationRequestBuilder(channel: pekko.grpc.internal.InternalChannel) =
new ScalaUnaryRequestBuilder(getOperationDescriptor, channel, options, settings)
private def deleteOperationRequestBuilder(channel: pekko.grpc.internal.InternalChannel) =
new ScalaUnaryRequestBuilder(deleteOperationDescriptor, channel, options, settings)
private def cancelOperationRequestBuilder(channel: pekko.grpc.internal.InternalChannel) =
new ScalaUnaryRequestBuilder(cancelOperationDescriptor, channel, options, settings)
private def waitOperationRequestBuilder(channel: pekko.grpc.internal.InternalChannel) =
new ScalaUnaryRequestBuilder(waitOperationDescriptor, channel, options, settings)
/**
* Lower level "lifted" version of the method, giving access to request metadata etc.
* prefer listOperations(com.google.longrunning.operations.ListOperationsRequest) if possible.
*/
override def listOperations(): SingleResponseRequestBuilder[com.google.longrunning.operations.ListOperationsRequest, com.google.longrunning.operations.ListOperationsResponse] =
listOperationsRequestBuilder(channel.internalChannel)
/**
* For access to method metadata use the parameterless version of listOperations
*/
def listOperations(in: com.google.longrunning.operations.ListOperationsRequest): scala.concurrent.Future[com.google.longrunning.operations.ListOperationsResponse] =
listOperations().invoke(in)
/**
* Lower level "lifted" version of the method, giving access to request metadata etc.
* prefer getOperation(com.google.longrunning.operations.GetOperationRequest) if possible.
*/
override def getOperation(): SingleResponseRequestBuilder[com.google.longrunning.operations.GetOperationRequest, com.google.longrunning.operations.Operation] =
getOperationRequestBuilder(channel.internalChannel)
/**
* For access to method metadata use the parameterless version of getOperation
*/
def getOperation(in: com.google.longrunning.operations.GetOperationRequest): scala.concurrent.Future[com.google.longrunning.operations.Operation] =
getOperation().invoke(in)
/**
* Lower level "lifted" version of the method, giving access to request metadata etc.
* prefer deleteOperation(com.google.longrunning.operations.DeleteOperationRequest) if possible.
*/
override def deleteOperation(): SingleResponseRequestBuilder[com.google.longrunning.operations.DeleteOperationRequest, com.google.protobuf.empty.Empty] =
deleteOperationRequestBuilder(channel.internalChannel)
/**
* For access to method metadata use the parameterless version of deleteOperation
*/
def deleteOperation(in: com.google.longrunning.operations.DeleteOperationRequest): scala.concurrent.Future[com.google.protobuf.empty.Empty] =
deleteOperation().invoke(in)
/**
* Lower level "lifted" version of the method, giving access to request metadata etc.
* prefer cancelOperation(com.google.longrunning.operations.CancelOperationRequest) if possible.
*/
override def cancelOperation(): SingleResponseRequestBuilder[com.google.longrunning.operations.CancelOperationRequest, com.google.protobuf.empty.Empty] =
cancelOperationRequestBuilder(channel.internalChannel)
/**
* For access to method metadata use the parameterless version of cancelOperation
*/
def cancelOperation(in: com.google.longrunning.operations.CancelOperationRequest): scala.concurrent.Future[com.google.protobuf.empty.Empty] =
cancelOperation().invoke(in)
/**
* Lower level "lifted" version of the method, giving access to request metadata etc.
* prefer waitOperation(com.google.longrunning.operations.WaitOperationRequest) if possible.
*/
override def waitOperation(): SingleResponseRequestBuilder[com.google.longrunning.operations.WaitOperationRequest, com.google.longrunning.operations.Operation] =
waitOperationRequestBuilder(channel.internalChannel)
/**
* For access to method metadata use the parameterless version of waitOperation
*/
def waitOperation(in: com.google.longrunning.operations.WaitOperationRequest): scala.concurrent.Future[com.google.longrunning.operations.Operation] =
waitOperation().invoke(in)
override def close(): scala.concurrent.Future[pekko.Done] =
if (isChannelOwned) channel.close()
else throw new GrpcClientCloseException()
override def closed: scala.concurrent.Future[pekko.Done] = channel.closed()
}
}
@PekkoGrpcGenerated
trait OperationsClientPowerApi {
/**
* Lower level "lifted" version of the method, giving access to request metadata etc.
* prefer listOperations(com.google.longrunning.operations.ListOperationsRequest) if possible.
*/
def listOperations(): SingleResponseRequestBuilder[com.google.longrunning.operations.ListOperationsRequest, com.google.longrunning.operations.ListOperationsResponse] = ???
/**
* Lower level "lifted" version of the method, giving access to request metadata etc.
* prefer getOperation(com.google.longrunning.operations.GetOperationRequest) if possible.
*/
def getOperation(): SingleResponseRequestBuilder[com.google.longrunning.operations.GetOperationRequest, com.google.longrunning.operations.Operation] = ???
/**
* Lower level "lifted" version of the method, giving access to request metadata etc.
* prefer deleteOperation(com.google.longrunning.operations.DeleteOperationRequest) if possible.
*/
def deleteOperation(): SingleResponseRequestBuilder[com.google.longrunning.operations.DeleteOperationRequest, com.google.protobuf.empty.Empty] = ???
/**
* Lower level "lifted" version of the method, giving access to request metadata etc.
* prefer cancelOperation(com.google.longrunning.operations.CancelOperationRequest) if possible.
*/
def cancelOperation(): SingleResponseRequestBuilder[com.google.longrunning.operations.CancelOperationRequest, com.google.protobuf.empty.Empty] = ???
/**
* Lower level "lifted" version of the method, giving access to request metadata etc.
* prefer waitOperation(com.google.longrunning.operations.WaitOperationRequest) if possible.
*/
def waitOperation(): SingleResponseRequestBuilder[com.google.longrunning.operations.WaitOperationRequest, com.google.longrunning.operations.Operation] = ???
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy