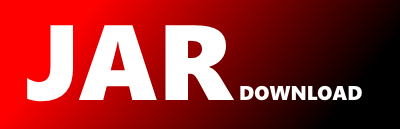
org.apache.phoenix.coprocessor.generated.PTableProtos Maven / Gradle / Ivy
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: PTable.proto
package org.apache.phoenix.coprocessor.generated;
public final class PTableProtos {
private PTableProtos() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
}
/**
* Protobuf enum {@code PTableType}
*/
public enum PTableType
implements com.google.protobuf.ProtocolMessageEnum {
/**
* SYSTEM = 0;
*/
SYSTEM(0, 0),
/**
* USER = 1;
*/
USER(1, 1),
/**
* VIEW = 2;
*/
VIEW(2, 2),
/**
* INDEX = 3;
*/
INDEX(3, 3),
/**
* JOIN = 4;
*/
JOIN(4, 4),
;
/**
* SYSTEM = 0;
*/
public static final int SYSTEM_VALUE = 0;
/**
* USER = 1;
*/
public static final int USER_VALUE = 1;
/**
* VIEW = 2;
*/
public static final int VIEW_VALUE = 2;
/**
* INDEX = 3;
*/
public static final int INDEX_VALUE = 3;
/**
* JOIN = 4;
*/
public static final int JOIN_VALUE = 4;
public final int getNumber() { return value; }
public static PTableType valueOf(int value) {
switch (value) {
case 0: return SYSTEM;
case 1: return USER;
case 2: return VIEW;
case 3: return INDEX;
case 4: return JOIN;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static com.google.protobuf.Internal.EnumLiteMap
internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public PTableType findValueByNumber(int number) {
return PTableType.valueOf(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(index);
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return org.apache.phoenix.coprocessor.generated.PTableProtos.getDescriptor().getEnumTypes().get(0);
}
private static final PTableType[] VALUES = values();
public static PTableType valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int index;
private final int value;
private PTableType(int index, int value) {
this.index = index;
this.value = value;
}
// @@protoc_insertion_point(enum_scope:PTableType)
}
public interface PColumnOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// required bytes columnNameBytes = 1;
/**
* required bytes columnNameBytes = 1;
*/
boolean hasColumnNameBytes();
/**
* required bytes columnNameBytes = 1;
*/
com.google.protobuf.ByteString getColumnNameBytes();
// optional bytes familyNameBytes = 2;
/**
* optional bytes familyNameBytes = 2;
*/
boolean hasFamilyNameBytes();
/**
* optional bytes familyNameBytes = 2;
*/
com.google.protobuf.ByteString getFamilyNameBytes();
// optional string dataType = 3;
/**
* optional string dataType = 3;
*/
boolean hasDataType();
/**
* optional string dataType = 3;
*/
java.lang.String getDataType();
/**
* optional string dataType = 3;
*/
com.google.protobuf.ByteString
getDataTypeBytes();
// optional int32 maxLength = 4;
/**
* optional int32 maxLength = 4;
*/
boolean hasMaxLength();
/**
* optional int32 maxLength = 4;
*/
int getMaxLength();
// optional int32 scale = 5;
/**
* optional int32 scale = 5;
*/
boolean hasScale();
/**
* optional int32 scale = 5;
*/
int getScale();
// required bool nullable = 6;
/**
* required bool nullable = 6;
*/
boolean hasNullable();
/**
* required bool nullable = 6;
*/
boolean getNullable();
// required int32 position = 7;
/**
* required int32 position = 7;
*/
boolean hasPosition();
/**
* required int32 position = 7;
*/
int getPosition();
// optional int32 sortOrder = 8;
/**
* optional int32 sortOrder = 8;
*/
boolean hasSortOrder();
/**
* optional int32 sortOrder = 8;
*/
int getSortOrder();
// optional int32 arraySize = 9;
/**
* optional int32 arraySize = 9;
*/
boolean hasArraySize();
/**
* optional int32 arraySize = 9;
*/
int getArraySize();
// optional bytes viewConstant = 10;
/**
* optional bytes viewConstant = 10;
*/
boolean hasViewConstant();
/**
* optional bytes viewConstant = 10;
*/
com.google.protobuf.ByteString getViewConstant();
// optional bool viewReferenced = 11;
/**
* optional bool viewReferenced = 11;
*/
boolean hasViewReferenced();
/**
* optional bool viewReferenced = 11;
*/
boolean getViewReferenced();
// optional string expression = 12;
/**
* optional string expression = 12;
*/
boolean hasExpression();
/**
* optional string expression = 12;
*/
java.lang.String getExpression();
/**
* optional string expression = 12;
*/
com.google.protobuf.ByteString
getExpressionBytes();
// optional bool isRowTimestamp = 13;
/**
* optional bool isRowTimestamp = 13;
*/
boolean hasIsRowTimestamp();
/**
* optional bool isRowTimestamp = 13;
*/
boolean getIsRowTimestamp();
// optional bool isDynamic = 14;
/**
* optional bool isDynamic = 14;
*/
boolean hasIsDynamic();
/**
* optional bool isDynamic = 14;
*/
boolean getIsDynamic();
// optional bytes columnQualifierBytes = 15;
/**
* optional bytes columnQualifierBytes = 15;
*/
boolean hasColumnQualifierBytes();
/**
* optional bytes columnQualifierBytes = 15;
*/
com.google.protobuf.ByteString getColumnQualifierBytes();
// optional int64 timestamp = 16;
/**
* optional int64 timestamp = 16;
*/
boolean hasTimestamp();
/**
* optional int64 timestamp = 16;
*/
long getTimestamp();
// optional bool derived = 17 [default = false];
/**
* optional bool derived = 17 [default = false];
*/
boolean hasDerived();
/**
* optional bool derived = 17 [default = false];
*/
boolean getDerived();
}
/**
* Protobuf type {@code PColumn}
*/
public static final class PColumn extends
com.google.protobuf.GeneratedMessage
implements PColumnOrBuilder {
// Use PColumn.newBuilder() to construct.
private PColumn(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private PColumn(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final PColumn defaultInstance;
public static PColumn getDefaultInstance() {
return defaultInstance;
}
public PColumn getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private PColumn(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
bitField0_ |= 0x00000001;
columnNameBytes_ = input.readBytes();
break;
}
case 18: {
bitField0_ |= 0x00000002;
familyNameBytes_ = input.readBytes();
break;
}
case 26: {
bitField0_ |= 0x00000004;
dataType_ = input.readBytes();
break;
}
case 32: {
bitField0_ |= 0x00000008;
maxLength_ = input.readInt32();
break;
}
case 40: {
bitField0_ |= 0x00000010;
scale_ = input.readInt32();
break;
}
case 48: {
bitField0_ |= 0x00000020;
nullable_ = input.readBool();
break;
}
case 56: {
bitField0_ |= 0x00000040;
position_ = input.readInt32();
break;
}
case 64: {
bitField0_ |= 0x00000080;
sortOrder_ = input.readInt32();
break;
}
case 72: {
bitField0_ |= 0x00000100;
arraySize_ = input.readInt32();
break;
}
case 82: {
bitField0_ |= 0x00000200;
viewConstant_ = input.readBytes();
break;
}
case 88: {
bitField0_ |= 0x00000400;
viewReferenced_ = input.readBool();
break;
}
case 98: {
bitField0_ |= 0x00000800;
expression_ = input.readBytes();
break;
}
case 104: {
bitField0_ |= 0x00001000;
isRowTimestamp_ = input.readBool();
break;
}
case 112: {
bitField0_ |= 0x00002000;
isDynamic_ = input.readBool();
break;
}
case 122: {
bitField0_ |= 0x00004000;
columnQualifierBytes_ = input.readBytes();
break;
}
case 128: {
bitField0_ |= 0x00008000;
timestamp_ = input.readInt64();
break;
}
case 136: {
bitField0_ |= 0x00010000;
derived_ = input.readBool();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.phoenix.coprocessor.generated.PTableProtos.internal_static_PColumn_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.phoenix.coprocessor.generated.PTableProtos.internal_static_PColumn_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.phoenix.coprocessor.generated.PTableProtos.PColumn.class, org.apache.phoenix.coprocessor.generated.PTableProtos.PColumn.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public PColumn parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new PColumn(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// required bytes columnNameBytes = 1;
public static final int COLUMNNAMEBYTES_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString columnNameBytes_;
/**
* required bytes columnNameBytes = 1;
*/
public boolean hasColumnNameBytes() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required bytes columnNameBytes = 1;
*/
public com.google.protobuf.ByteString getColumnNameBytes() {
return columnNameBytes_;
}
// optional bytes familyNameBytes = 2;
public static final int FAMILYNAMEBYTES_FIELD_NUMBER = 2;
private com.google.protobuf.ByteString familyNameBytes_;
/**
* optional bytes familyNameBytes = 2;
*/
public boolean hasFamilyNameBytes() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional bytes familyNameBytes = 2;
*/
public com.google.protobuf.ByteString getFamilyNameBytes() {
return familyNameBytes_;
}
// optional string dataType = 3;
public static final int DATATYPE_FIELD_NUMBER = 3;
private java.lang.Object dataType_;
/**
* optional string dataType = 3;
*/
public boolean hasDataType() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional string dataType = 3;
*/
public java.lang.String getDataType() {
java.lang.Object ref = dataType_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
dataType_ = s;
}
return s;
}
}
/**
* optional string dataType = 3;
*/
public com.google.protobuf.ByteString
getDataTypeBytes() {
java.lang.Object ref = dataType_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
dataType_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional int32 maxLength = 4;
public static final int MAXLENGTH_FIELD_NUMBER = 4;
private int maxLength_;
/**
* optional int32 maxLength = 4;
*/
public boolean hasMaxLength() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional int32 maxLength = 4;
*/
public int getMaxLength() {
return maxLength_;
}
// optional int32 scale = 5;
public static final int SCALE_FIELD_NUMBER = 5;
private int scale_;
/**
* optional int32 scale = 5;
*/
public boolean hasScale() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional int32 scale = 5;
*/
public int getScale() {
return scale_;
}
// required bool nullable = 6;
public static final int NULLABLE_FIELD_NUMBER = 6;
private boolean nullable_;
/**
* required bool nullable = 6;
*/
public boolean hasNullable() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* required bool nullable = 6;
*/
public boolean getNullable() {
return nullable_;
}
// required int32 position = 7;
public static final int POSITION_FIELD_NUMBER = 7;
private int position_;
/**
* required int32 position = 7;
*/
public boolean hasPosition() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
* required int32 position = 7;
*/
public int getPosition() {
return position_;
}
// optional int32 sortOrder = 8;
public static final int SORTORDER_FIELD_NUMBER = 8;
private int sortOrder_;
/**
* optional int32 sortOrder = 8;
*/
public boolean hasSortOrder() {
return ((bitField0_ & 0x00000080) == 0x00000080);
}
/**
* optional int32 sortOrder = 8;
*/
public int getSortOrder() {
return sortOrder_;
}
// optional int32 arraySize = 9;
public static final int ARRAYSIZE_FIELD_NUMBER = 9;
private int arraySize_;
/**
* optional int32 arraySize = 9;
*/
public boolean hasArraySize() {
return ((bitField0_ & 0x00000100) == 0x00000100);
}
/**
* optional int32 arraySize = 9;
*/
public int getArraySize() {
return arraySize_;
}
// optional bytes viewConstant = 10;
public static final int VIEWCONSTANT_FIELD_NUMBER = 10;
private com.google.protobuf.ByteString viewConstant_;
/**
* optional bytes viewConstant = 10;
*/
public boolean hasViewConstant() {
return ((bitField0_ & 0x00000200) == 0x00000200);
}
/**
* optional bytes viewConstant = 10;
*/
public com.google.protobuf.ByteString getViewConstant() {
return viewConstant_;
}
// optional bool viewReferenced = 11;
public static final int VIEWREFERENCED_FIELD_NUMBER = 11;
private boolean viewReferenced_;
/**
* optional bool viewReferenced = 11;
*/
public boolean hasViewReferenced() {
return ((bitField0_ & 0x00000400) == 0x00000400);
}
/**
* optional bool viewReferenced = 11;
*/
public boolean getViewReferenced() {
return viewReferenced_;
}
// optional string expression = 12;
public static final int EXPRESSION_FIELD_NUMBER = 12;
private java.lang.Object expression_;
/**
* optional string expression = 12;
*/
public boolean hasExpression() {
return ((bitField0_ & 0x00000800) == 0x00000800);
}
/**
* optional string expression = 12;
*/
public java.lang.String getExpression() {
java.lang.Object ref = expression_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
expression_ = s;
}
return s;
}
}
/**
* optional string expression = 12;
*/
public com.google.protobuf.ByteString
getExpressionBytes() {
java.lang.Object ref = expression_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
expression_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional bool isRowTimestamp = 13;
public static final int ISROWTIMESTAMP_FIELD_NUMBER = 13;
private boolean isRowTimestamp_;
/**
* optional bool isRowTimestamp = 13;
*/
public boolean hasIsRowTimestamp() {
return ((bitField0_ & 0x00001000) == 0x00001000);
}
/**
* optional bool isRowTimestamp = 13;
*/
public boolean getIsRowTimestamp() {
return isRowTimestamp_;
}
// optional bool isDynamic = 14;
public static final int ISDYNAMIC_FIELD_NUMBER = 14;
private boolean isDynamic_;
/**
* optional bool isDynamic = 14;
*/
public boolean hasIsDynamic() {
return ((bitField0_ & 0x00002000) == 0x00002000);
}
/**
* optional bool isDynamic = 14;
*/
public boolean getIsDynamic() {
return isDynamic_;
}
// optional bytes columnQualifierBytes = 15;
public static final int COLUMNQUALIFIERBYTES_FIELD_NUMBER = 15;
private com.google.protobuf.ByteString columnQualifierBytes_;
/**
* optional bytes columnQualifierBytes = 15;
*/
public boolean hasColumnQualifierBytes() {
return ((bitField0_ & 0x00004000) == 0x00004000);
}
/**
* optional bytes columnQualifierBytes = 15;
*/
public com.google.protobuf.ByteString getColumnQualifierBytes() {
return columnQualifierBytes_;
}
// optional int64 timestamp = 16;
public static final int TIMESTAMP_FIELD_NUMBER = 16;
private long timestamp_;
/**
* optional int64 timestamp = 16;
*/
public boolean hasTimestamp() {
return ((bitField0_ & 0x00008000) == 0x00008000);
}
/**
* optional int64 timestamp = 16;
*/
public long getTimestamp() {
return timestamp_;
}
// optional bool derived = 17 [default = false];
public static final int DERIVED_FIELD_NUMBER = 17;
private boolean derived_;
/**
* optional bool derived = 17 [default = false];
*/
public boolean hasDerived() {
return ((bitField0_ & 0x00010000) == 0x00010000);
}
/**
* optional bool derived = 17 [default = false];
*/
public boolean getDerived() {
return derived_;
}
private void initFields() {
columnNameBytes_ = com.google.protobuf.ByteString.EMPTY;
familyNameBytes_ = com.google.protobuf.ByteString.EMPTY;
dataType_ = "";
maxLength_ = 0;
scale_ = 0;
nullable_ = false;
position_ = 0;
sortOrder_ = 0;
arraySize_ = 0;
viewConstant_ = com.google.protobuf.ByteString.EMPTY;
viewReferenced_ = false;
expression_ = "";
isRowTimestamp_ = false;
isDynamic_ = false;
columnQualifierBytes_ = com.google.protobuf.ByteString.EMPTY;
timestamp_ = 0L;
derived_ = false;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (!hasColumnNameBytes()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasNullable()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasPosition()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(1, columnNameBytes_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeBytes(2, familyNameBytes_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeBytes(3, getDataTypeBytes());
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeInt32(4, maxLength_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
output.writeInt32(5, scale_);
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
output.writeBool(6, nullable_);
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
output.writeInt32(7, position_);
}
if (((bitField0_ & 0x00000080) == 0x00000080)) {
output.writeInt32(8, sortOrder_);
}
if (((bitField0_ & 0x00000100) == 0x00000100)) {
output.writeInt32(9, arraySize_);
}
if (((bitField0_ & 0x00000200) == 0x00000200)) {
output.writeBytes(10, viewConstant_);
}
if (((bitField0_ & 0x00000400) == 0x00000400)) {
output.writeBool(11, viewReferenced_);
}
if (((bitField0_ & 0x00000800) == 0x00000800)) {
output.writeBytes(12, getExpressionBytes());
}
if (((bitField0_ & 0x00001000) == 0x00001000)) {
output.writeBool(13, isRowTimestamp_);
}
if (((bitField0_ & 0x00002000) == 0x00002000)) {
output.writeBool(14, isDynamic_);
}
if (((bitField0_ & 0x00004000) == 0x00004000)) {
output.writeBytes(15, columnQualifierBytes_);
}
if (((bitField0_ & 0x00008000) == 0x00008000)) {
output.writeInt64(16, timestamp_);
}
if (((bitField0_ & 0x00010000) == 0x00010000)) {
output.writeBool(17, derived_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, columnNameBytes_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, familyNameBytes_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(3, getDataTypeBytes());
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(4, maxLength_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(5, scale_);
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(6, nullable_);
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(7, position_);
}
if (((bitField0_ & 0x00000080) == 0x00000080)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(8, sortOrder_);
}
if (((bitField0_ & 0x00000100) == 0x00000100)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(9, arraySize_);
}
if (((bitField0_ & 0x00000200) == 0x00000200)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(10, viewConstant_);
}
if (((bitField0_ & 0x00000400) == 0x00000400)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(11, viewReferenced_);
}
if (((bitField0_ & 0x00000800) == 0x00000800)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(12, getExpressionBytes());
}
if (((bitField0_ & 0x00001000) == 0x00001000)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(13, isRowTimestamp_);
}
if (((bitField0_ & 0x00002000) == 0x00002000)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(14, isDynamic_);
}
if (((bitField0_ & 0x00004000) == 0x00004000)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(15, columnQualifierBytes_);
}
if (((bitField0_ & 0x00008000) == 0x00008000)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(16, timestamp_);
}
if (((bitField0_ & 0x00010000) == 0x00010000)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(17, derived_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.phoenix.coprocessor.generated.PTableProtos.PColumn)) {
return super.equals(obj);
}
org.apache.phoenix.coprocessor.generated.PTableProtos.PColumn other = (org.apache.phoenix.coprocessor.generated.PTableProtos.PColumn) obj;
boolean result = true;
result = result && (hasColumnNameBytes() == other.hasColumnNameBytes());
if (hasColumnNameBytes()) {
result = result && getColumnNameBytes()
.equals(other.getColumnNameBytes());
}
result = result && (hasFamilyNameBytes() == other.hasFamilyNameBytes());
if (hasFamilyNameBytes()) {
result = result && getFamilyNameBytes()
.equals(other.getFamilyNameBytes());
}
result = result && (hasDataType() == other.hasDataType());
if (hasDataType()) {
result = result && getDataType()
.equals(other.getDataType());
}
result = result && (hasMaxLength() == other.hasMaxLength());
if (hasMaxLength()) {
result = result && (getMaxLength()
== other.getMaxLength());
}
result = result && (hasScale() == other.hasScale());
if (hasScale()) {
result = result && (getScale()
== other.getScale());
}
result = result && (hasNullable() == other.hasNullable());
if (hasNullable()) {
result = result && (getNullable()
== other.getNullable());
}
result = result && (hasPosition() == other.hasPosition());
if (hasPosition()) {
result = result && (getPosition()
== other.getPosition());
}
result = result && (hasSortOrder() == other.hasSortOrder());
if (hasSortOrder()) {
result = result && (getSortOrder()
== other.getSortOrder());
}
result = result && (hasArraySize() == other.hasArraySize());
if (hasArraySize()) {
result = result && (getArraySize()
== other.getArraySize());
}
result = result && (hasViewConstant() == other.hasViewConstant());
if (hasViewConstant()) {
result = result && getViewConstant()
.equals(other.getViewConstant());
}
result = result && (hasViewReferenced() == other.hasViewReferenced());
if (hasViewReferenced()) {
result = result && (getViewReferenced()
== other.getViewReferenced());
}
result = result && (hasExpression() == other.hasExpression());
if (hasExpression()) {
result = result && getExpression()
.equals(other.getExpression());
}
result = result && (hasIsRowTimestamp() == other.hasIsRowTimestamp());
if (hasIsRowTimestamp()) {
result = result && (getIsRowTimestamp()
== other.getIsRowTimestamp());
}
result = result && (hasIsDynamic() == other.hasIsDynamic());
if (hasIsDynamic()) {
result = result && (getIsDynamic()
== other.getIsDynamic());
}
result = result && (hasColumnQualifierBytes() == other.hasColumnQualifierBytes());
if (hasColumnQualifierBytes()) {
result = result && getColumnQualifierBytes()
.equals(other.getColumnQualifierBytes());
}
result = result && (hasTimestamp() == other.hasTimestamp());
if (hasTimestamp()) {
result = result && (getTimestamp()
== other.getTimestamp());
}
result = result && (hasDerived() == other.hasDerived());
if (hasDerived()) {
result = result && (getDerived()
== other.getDerived());
}
result = result &&
getUnknownFields().equals(other.getUnknownFields());
return result;
}
private int memoizedHashCode = 0;
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptorForType().hashCode();
if (hasColumnNameBytes()) {
hash = (37 * hash) + COLUMNNAMEBYTES_FIELD_NUMBER;
hash = (53 * hash) + getColumnNameBytes().hashCode();
}
if (hasFamilyNameBytes()) {
hash = (37 * hash) + FAMILYNAMEBYTES_FIELD_NUMBER;
hash = (53 * hash) + getFamilyNameBytes().hashCode();
}
if (hasDataType()) {
hash = (37 * hash) + DATATYPE_FIELD_NUMBER;
hash = (53 * hash) + getDataType().hashCode();
}
if (hasMaxLength()) {
hash = (37 * hash) + MAXLENGTH_FIELD_NUMBER;
hash = (53 * hash) + getMaxLength();
}
if (hasScale()) {
hash = (37 * hash) + SCALE_FIELD_NUMBER;
hash = (53 * hash) + getScale();
}
if (hasNullable()) {
hash = (37 * hash) + NULLABLE_FIELD_NUMBER;
hash = (53 * hash) + hashBoolean(getNullable());
}
if (hasPosition()) {
hash = (37 * hash) + POSITION_FIELD_NUMBER;
hash = (53 * hash) + getPosition();
}
if (hasSortOrder()) {
hash = (37 * hash) + SORTORDER_FIELD_NUMBER;
hash = (53 * hash) + getSortOrder();
}
if (hasArraySize()) {
hash = (37 * hash) + ARRAYSIZE_FIELD_NUMBER;
hash = (53 * hash) + getArraySize();
}
if (hasViewConstant()) {
hash = (37 * hash) + VIEWCONSTANT_FIELD_NUMBER;
hash = (53 * hash) + getViewConstant().hashCode();
}
if (hasViewReferenced()) {
hash = (37 * hash) + VIEWREFERENCED_FIELD_NUMBER;
hash = (53 * hash) + hashBoolean(getViewReferenced());
}
if (hasExpression()) {
hash = (37 * hash) + EXPRESSION_FIELD_NUMBER;
hash = (53 * hash) + getExpression().hashCode();
}
if (hasIsRowTimestamp()) {
hash = (37 * hash) + ISROWTIMESTAMP_FIELD_NUMBER;
hash = (53 * hash) + hashBoolean(getIsRowTimestamp());
}
if (hasIsDynamic()) {
hash = (37 * hash) + ISDYNAMIC_FIELD_NUMBER;
hash = (53 * hash) + hashBoolean(getIsDynamic());
}
if (hasColumnQualifierBytes()) {
hash = (37 * hash) + COLUMNQUALIFIERBYTES_FIELD_NUMBER;
hash = (53 * hash) + getColumnQualifierBytes().hashCode();
}
if (hasTimestamp()) {
hash = (37 * hash) + TIMESTAMP_FIELD_NUMBER;
hash = (53 * hash) + hashLong(getTimestamp());
}
if (hasDerived()) {
hash = (37 * hash) + DERIVED_FIELD_NUMBER;
hash = (53 * hash) + hashBoolean(getDerived());
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.phoenix.coprocessor.generated.PTableProtos.PColumn parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.phoenix.coprocessor.generated.PTableProtos.PColumn parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.phoenix.coprocessor.generated.PTableProtos.PColumn parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.phoenix.coprocessor.generated.PTableProtos.PColumn parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.phoenix.coprocessor.generated.PTableProtos.PColumn parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.apache.phoenix.coprocessor.generated.PTableProtos.PColumn parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.apache.phoenix.coprocessor.generated.PTableProtos.PColumn parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.apache.phoenix.coprocessor.generated.PTableProtos.PColumn parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.apache.phoenix.coprocessor.generated.PTableProtos.PColumn parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.apache.phoenix.coprocessor.generated.PTableProtos.PColumn parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(org.apache.phoenix.coprocessor.generated.PTableProtos.PColumn prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code PColumn}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements org.apache.phoenix.coprocessor.generated.PTableProtos.PColumnOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.phoenix.coprocessor.generated.PTableProtos.internal_static_PColumn_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.phoenix.coprocessor.generated.PTableProtos.internal_static_PColumn_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.phoenix.coprocessor.generated.PTableProtos.PColumn.class, org.apache.phoenix.coprocessor.generated.PTableProtos.PColumn.Builder.class);
}
// Construct using org.apache.phoenix.coprocessor.generated.PTableProtos.PColumn.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
columnNameBytes_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
familyNameBytes_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000002);
dataType_ = "";
bitField0_ = (bitField0_ & ~0x00000004);
maxLength_ = 0;
bitField0_ = (bitField0_ & ~0x00000008);
scale_ = 0;
bitField0_ = (bitField0_ & ~0x00000010);
nullable_ = false;
bitField0_ = (bitField0_ & ~0x00000020);
position_ = 0;
bitField0_ = (bitField0_ & ~0x00000040);
sortOrder_ = 0;
bitField0_ = (bitField0_ & ~0x00000080);
arraySize_ = 0;
bitField0_ = (bitField0_ & ~0x00000100);
viewConstant_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000200);
viewReferenced_ = false;
bitField0_ = (bitField0_ & ~0x00000400);
expression_ = "";
bitField0_ = (bitField0_ & ~0x00000800);
isRowTimestamp_ = false;
bitField0_ = (bitField0_ & ~0x00001000);
isDynamic_ = false;
bitField0_ = (bitField0_ & ~0x00002000);
columnQualifierBytes_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00004000);
timestamp_ = 0L;
bitField0_ = (bitField0_ & ~0x00008000);
derived_ = false;
bitField0_ = (bitField0_ & ~0x00010000);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.phoenix.coprocessor.generated.PTableProtos.internal_static_PColumn_descriptor;
}
public org.apache.phoenix.coprocessor.generated.PTableProtos.PColumn getDefaultInstanceForType() {
return org.apache.phoenix.coprocessor.generated.PTableProtos.PColumn.getDefaultInstance();
}
public org.apache.phoenix.coprocessor.generated.PTableProtos.PColumn build() {
org.apache.phoenix.coprocessor.generated.PTableProtos.PColumn result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.apache.phoenix.coprocessor.generated.PTableProtos.PColumn buildPartial() {
org.apache.phoenix.coprocessor.generated.PTableProtos.PColumn result = new org.apache.phoenix.coprocessor.generated.PTableProtos.PColumn(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.columnNameBytes_ = columnNameBytes_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.familyNameBytes_ = familyNameBytes_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.dataType_ = dataType_;
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000008;
}
result.maxLength_ = maxLength_;
if (((from_bitField0_ & 0x00000010) == 0x00000010)) {
to_bitField0_ |= 0x00000010;
}
result.scale_ = scale_;
if (((from_bitField0_ & 0x00000020) == 0x00000020)) {
to_bitField0_ |= 0x00000020;
}
result.nullable_ = nullable_;
if (((from_bitField0_ & 0x00000040) == 0x00000040)) {
to_bitField0_ |= 0x00000040;
}
result.position_ = position_;
if (((from_bitField0_ & 0x00000080) == 0x00000080)) {
to_bitField0_ |= 0x00000080;
}
result.sortOrder_ = sortOrder_;
if (((from_bitField0_ & 0x00000100) == 0x00000100)) {
to_bitField0_ |= 0x00000100;
}
result.arraySize_ = arraySize_;
if (((from_bitField0_ & 0x00000200) == 0x00000200)) {
to_bitField0_ |= 0x00000200;
}
result.viewConstant_ = viewConstant_;
if (((from_bitField0_ & 0x00000400) == 0x00000400)) {
to_bitField0_ |= 0x00000400;
}
result.viewReferenced_ = viewReferenced_;
if (((from_bitField0_ & 0x00000800) == 0x00000800)) {
to_bitField0_ |= 0x00000800;
}
result.expression_ = expression_;
if (((from_bitField0_ & 0x00001000) == 0x00001000)) {
to_bitField0_ |= 0x00001000;
}
result.isRowTimestamp_ = isRowTimestamp_;
if (((from_bitField0_ & 0x00002000) == 0x00002000)) {
to_bitField0_ |= 0x00002000;
}
result.isDynamic_ = isDynamic_;
if (((from_bitField0_ & 0x00004000) == 0x00004000)) {
to_bitField0_ |= 0x00004000;
}
result.columnQualifierBytes_ = columnQualifierBytes_;
if (((from_bitField0_ & 0x00008000) == 0x00008000)) {
to_bitField0_ |= 0x00008000;
}
result.timestamp_ = timestamp_;
if (((from_bitField0_ & 0x00010000) == 0x00010000)) {
to_bitField0_ |= 0x00010000;
}
result.derived_ = derived_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.apache.phoenix.coprocessor.generated.PTableProtos.PColumn) {
return mergeFrom((org.apache.phoenix.coprocessor.generated.PTableProtos.PColumn)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.phoenix.coprocessor.generated.PTableProtos.PColumn other) {
if (other == org.apache.phoenix.coprocessor.generated.PTableProtos.PColumn.getDefaultInstance()) return this;
if (other.hasColumnNameBytes()) {
setColumnNameBytes(other.getColumnNameBytes());
}
if (other.hasFamilyNameBytes()) {
setFamilyNameBytes(other.getFamilyNameBytes());
}
if (other.hasDataType()) {
bitField0_ |= 0x00000004;
dataType_ = other.dataType_;
onChanged();
}
if (other.hasMaxLength()) {
setMaxLength(other.getMaxLength());
}
if (other.hasScale()) {
setScale(other.getScale());
}
if (other.hasNullable()) {
setNullable(other.getNullable());
}
if (other.hasPosition()) {
setPosition(other.getPosition());
}
if (other.hasSortOrder()) {
setSortOrder(other.getSortOrder());
}
if (other.hasArraySize()) {
setArraySize(other.getArraySize());
}
if (other.hasViewConstant()) {
setViewConstant(other.getViewConstant());
}
if (other.hasViewReferenced()) {
setViewReferenced(other.getViewReferenced());
}
if (other.hasExpression()) {
bitField0_ |= 0x00000800;
expression_ = other.expression_;
onChanged();
}
if (other.hasIsRowTimestamp()) {
setIsRowTimestamp(other.getIsRowTimestamp());
}
if (other.hasIsDynamic()) {
setIsDynamic(other.getIsDynamic());
}
if (other.hasColumnQualifierBytes()) {
setColumnQualifierBytes(other.getColumnQualifierBytes());
}
if (other.hasTimestamp()) {
setTimestamp(other.getTimestamp());
}
if (other.hasDerived()) {
setDerived(other.getDerived());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasColumnNameBytes()) {
return false;
}
if (!hasNullable()) {
return false;
}
if (!hasPosition()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.phoenix.coprocessor.generated.PTableProtos.PColumn parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.phoenix.coprocessor.generated.PTableProtos.PColumn) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// required bytes columnNameBytes = 1;
private com.google.protobuf.ByteString columnNameBytes_ = com.google.protobuf.ByteString.EMPTY;
/**
* required bytes columnNameBytes = 1;
*/
public boolean hasColumnNameBytes() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required bytes columnNameBytes = 1;
*/
public com.google.protobuf.ByteString getColumnNameBytes() {
return columnNameBytes_;
}
/**
* required bytes columnNameBytes = 1;
*/
public Builder setColumnNameBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
columnNameBytes_ = value;
onChanged();
return this;
}
/**
* required bytes columnNameBytes = 1;
*/
public Builder clearColumnNameBytes() {
bitField0_ = (bitField0_ & ~0x00000001);
columnNameBytes_ = getDefaultInstance().getColumnNameBytes();
onChanged();
return this;
}
// optional bytes familyNameBytes = 2;
private com.google.protobuf.ByteString familyNameBytes_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes familyNameBytes = 2;
*/
public boolean hasFamilyNameBytes() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional bytes familyNameBytes = 2;
*/
public com.google.protobuf.ByteString getFamilyNameBytes() {
return familyNameBytes_;
}
/**
* optional bytes familyNameBytes = 2;
*/
public Builder setFamilyNameBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
familyNameBytes_ = value;
onChanged();
return this;
}
/**
* optional bytes familyNameBytes = 2;
*/
public Builder clearFamilyNameBytes() {
bitField0_ = (bitField0_ & ~0x00000002);
familyNameBytes_ = getDefaultInstance().getFamilyNameBytes();
onChanged();
return this;
}
// optional string dataType = 3;
private java.lang.Object dataType_ = "";
/**
* optional string dataType = 3;
*/
public boolean hasDataType() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional string dataType = 3;
*/
public java.lang.String getDataType() {
java.lang.Object ref = dataType_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
dataType_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string dataType = 3;
*/
public com.google.protobuf.ByteString
getDataTypeBytes() {
java.lang.Object ref = dataType_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
dataType_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string dataType = 3;
*/
public Builder setDataType(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
dataType_ = value;
onChanged();
return this;
}
/**
* optional string dataType = 3;
*/
public Builder clearDataType() {
bitField0_ = (bitField0_ & ~0x00000004);
dataType_ = getDefaultInstance().getDataType();
onChanged();
return this;
}
/**
* optional string dataType = 3;
*/
public Builder setDataTypeBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
dataType_ = value;
onChanged();
return this;
}
// optional int32 maxLength = 4;
private int maxLength_ ;
/**
* optional int32 maxLength = 4;
*/
public boolean hasMaxLength() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional int32 maxLength = 4;
*/
public int getMaxLength() {
return maxLength_;
}
/**
* optional int32 maxLength = 4;
*/
public Builder setMaxLength(int value) {
bitField0_ |= 0x00000008;
maxLength_ = value;
onChanged();
return this;
}
/**
* optional int32 maxLength = 4;
*/
public Builder clearMaxLength() {
bitField0_ = (bitField0_ & ~0x00000008);
maxLength_ = 0;
onChanged();
return this;
}
// optional int32 scale = 5;
private int scale_ ;
/**
* optional int32 scale = 5;
*/
public boolean hasScale() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional int32 scale = 5;
*/
public int getScale() {
return scale_;
}
/**
* optional int32 scale = 5;
*/
public Builder setScale(int value) {
bitField0_ |= 0x00000010;
scale_ = value;
onChanged();
return this;
}
/**
* optional int32 scale = 5;
*/
public Builder clearScale() {
bitField0_ = (bitField0_ & ~0x00000010);
scale_ = 0;
onChanged();
return this;
}
// required bool nullable = 6;
private boolean nullable_ ;
/**
* required bool nullable = 6;
*/
public boolean hasNullable() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* required bool nullable = 6;
*/
public boolean getNullable() {
return nullable_;
}
/**
* required bool nullable = 6;
*/
public Builder setNullable(boolean value) {
bitField0_ |= 0x00000020;
nullable_ = value;
onChanged();
return this;
}
/**
* required bool nullable = 6;
*/
public Builder clearNullable() {
bitField0_ = (bitField0_ & ~0x00000020);
nullable_ = false;
onChanged();
return this;
}
// required int32 position = 7;
private int position_ ;
/**
* required int32 position = 7;
*/
public boolean hasPosition() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
* required int32 position = 7;
*/
public int getPosition() {
return position_;
}
/**
* required int32 position = 7;
*/
public Builder setPosition(int value) {
bitField0_ |= 0x00000040;
position_ = value;
onChanged();
return this;
}
/**
* required int32 position = 7;
*/
public Builder clearPosition() {
bitField0_ = (bitField0_ & ~0x00000040);
position_ = 0;
onChanged();
return this;
}
// optional int32 sortOrder = 8;
private int sortOrder_ ;
/**
* optional int32 sortOrder = 8;
*/
public boolean hasSortOrder() {
return ((bitField0_ & 0x00000080) == 0x00000080);
}
/**
* optional int32 sortOrder = 8;
*/
public int getSortOrder() {
return sortOrder_;
}
/**
* optional int32 sortOrder = 8;
*/
public Builder setSortOrder(int value) {
bitField0_ |= 0x00000080;
sortOrder_ = value;
onChanged();
return this;
}
/**
* optional int32 sortOrder = 8;
*/
public Builder clearSortOrder() {
bitField0_ = (bitField0_ & ~0x00000080);
sortOrder_ = 0;
onChanged();
return this;
}
// optional int32 arraySize = 9;
private int arraySize_ ;
/**
* optional int32 arraySize = 9;
*/
public boolean hasArraySize() {
return ((bitField0_ & 0x00000100) == 0x00000100);
}
/**
* optional int32 arraySize = 9;
*/
public int getArraySize() {
return arraySize_;
}
/**
* optional int32 arraySize = 9;
*/
public Builder setArraySize(int value) {
bitField0_ |= 0x00000100;
arraySize_ = value;
onChanged();
return this;
}
/**
* optional int32 arraySize = 9;
*/
public Builder clearArraySize() {
bitField0_ = (bitField0_ & ~0x00000100);
arraySize_ = 0;
onChanged();
return this;
}
// optional bytes viewConstant = 10;
private com.google.protobuf.ByteString viewConstant_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes viewConstant = 10;
*/
public boolean hasViewConstant() {
return ((bitField0_ & 0x00000200) == 0x00000200);
}
/**
* optional bytes viewConstant = 10;
*/
public com.google.protobuf.ByteString getViewConstant() {
return viewConstant_;
}
/**
* optional bytes viewConstant = 10;
*/
public Builder setViewConstant(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000200;
viewConstant_ = value;
onChanged();
return this;
}
/**
* optional bytes viewConstant = 10;
*/
public Builder clearViewConstant() {
bitField0_ = (bitField0_ & ~0x00000200);
viewConstant_ = getDefaultInstance().getViewConstant();
onChanged();
return this;
}
// optional bool viewReferenced = 11;
private boolean viewReferenced_ ;
/**
* optional bool viewReferenced = 11;
*/
public boolean hasViewReferenced() {
return ((bitField0_ & 0x00000400) == 0x00000400);
}
/**
* optional bool viewReferenced = 11;
*/
public boolean getViewReferenced() {
return viewReferenced_;
}
/**
* optional bool viewReferenced = 11;
*/
public Builder setViewReferenced(boolean value) {
bitField0_ |= 0x00000400;
viewReferenced_ = value;
onChanged();
return this;
}
/**
* optional bool viewReferenced = 11;
*/
public Builder clearViewReferenced() {
bitField0_ = (bitField0_ & ~0x00000400);
viewReferenced_ = false;
onChanged();
return this;
}
// optional string expression = 12;
private java.lang.Object expression_ = "";
/**
* optional string expression = 12;
*/
public boolean hasExpression() {
return ((bitField0_ & 0x00000800) == 0x00000800);
}
/**
* optional string expression = 12;
*/
public java.lang.String getExpression() {
java.lang.Object ref = expression_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
expression_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string expression = 12;
*/
public com.google.protobuf.ByteString
getExpressionBytes() {
java.lang.Object ref = expression_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
expression_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string expression = 12;
*/
public Builder setExpression(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000800;
expression_ = value;
onChanged();
return this;
}
/**
* optional string expression = 12;
*/
public Builder clearExpression() {
bitField0_ = (bitField0_ & ~0x00000800);
expression_ = getDefaultInstance().getExpression();
onChanged();
return this;
}
/**
* optional string expression = 12;
*/
public Builder setExpressionBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000800;
expression_ = value;
onChanged();
return this;
}
// optional bool isRowTimestamp = 13;
private boolean isRowTimestamp_ ;
/**
* optional bool isRowTimestamp = 13;
*/
public boolean hasIsRowTimestamp() {
return ((bitField0_ & 0x00001000) == 0x00001000);
}
/**
* optional bool isRowTimestamp = 13;
*/
public boolean getIsRowTimestamp() {
return isRowTimestamp_;
}
/**
* optional bool isRowTimestamp = 13;
*/
public Builder setIsRowTimestamp(boolean value) {
bitField0_ |= 0x00001000;
isRowTimestamp_ = value;
onChanged();
return this;
}
/**
* optional bool isRowTimestamp = 13;
*/
public Builder clearIsRowTimestamp() {
bitField0_ = (bitField0_ & ~0x00001000);
isRowTimestamp_ = false;
onChanged();
return this;
}
// optional bool isDynamic = 14;
private boolean isDynamic_ ;
/**
* optional bool isDynamic = 14;
*/
public boolean hasIsDynamic() {
return ((bitField0_ & 0x00002000) == 0x00002000);
}
/**
* optional bool isDynamic = 14;
*/
public boolean getIsDynamic() {
return isDynamic_;
}
/**
* optional bool isDynamic = 14;
*/
public Builder setIsDynamic(boolean value) {
bitField0_ |= 0x00002000;
isDynamic_ = value;
onChanged();
return this;
}
/**
* optional bool isDynamic = 14;
*/
public Builder clearIsDynamic() {
bitField0_ = (bitField0_ & ~0x00002000);
isDynamic_ = false;
onChanged();
return this;
}
// optional bytes columnQualifierBytes = 15;
private com.google.protobuf.ByteString columnQualifierBytes_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes columnQualifierBytes = 15;
*/
public boolean hasColumnQualifierBytes() {
return ((bitField0_ & 0x00004000) == 0x00004000);
}
/**
* optional bytes columnQualifierBytes = 15;
*/
public com.google.protobuf.ByteString getColumnQualifierBytes() {
return columnQualifierBytes_;
}
/**
* optional bytes columnQualifierBytes = 15;
*/
public Builder setColumnQualifierBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00004000;
columnQualifierBytes_ = value;
onChanged();
return this;
}
/**
* optional bytes columnQualifierBytes = 15;
*/
public Builder clearColumnQualifierBytes() {
bitField0_ = (bitField0_ & ~0x00004000);
columnQualifierBytes_ = getDefaultInstance().getColumnQualifierBytes();
onChanged();
return this;
}
// optional int64 timestamp = 16;
private long timestamp_ ;
/**
* optional int64 timestamp = 16;
*/
public boolean hasTimestamp() {
return ((bitField0_ & 0x00008000) == 0x00008000);
}
/**
* optional int64 timestamp = 16;
*/
public long getTimestamp() {
return timestamp_;
}
/**
* optional int64 timestamp = 16;
*/
public Builder setTimestamp(long value) {
bitField0_ |= 0x00008000;
timestamp_ = value;
onChanged();
return this;
}
/**
* optional int64 timestamp = 16;
*/
public Builder clearTimestamp() {
bitField0_ = (bitField0_ & ~0x00008000);
timestamp_ = 0L;
onChanged();
return this;
}
// optional bool derived = 17 [default = false];
private boolean derived_ ;
/**
* optional bool derived = 17 [default = false];
*/
public boolean hasDerived() {
return ((bitField0_ & 0x00010000) == 0x00010000);
}
/**
* optional bool derived = 17 [default = false];
*/
public boolean getDerived() {
return derived_;
}
/**
* optional bool derived = 17 [default = false];
*/
public Builder setDerived(boolean value) {
bitField0_ |= 0x00010000;
derived_ = value;
onChanged();
return this;
}
/**
* optional bool derived = 17 [default = false];
*/
public Builder clearDerived() {
bitField0_ = (bitField0_ & ~0x00010000);
derived_ = false;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:PColumn)
}
static {
defaultInstance = new PColumn(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:PColumn)
}
public interface PTableStatsOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// required bytes key = 1;
/**
* required bytes key = 1;
*/
boolean hasKey();
/**
* required bytes key = 1;
*/
com.google.protobuf.ByteString getKey();
// repeated bytes values = 2;
/**
* repeated bytes values = 2;
*/
java.util.List getValuesList();
/**
* repeated bytes values = 2;
*/
int getValuesCount();
/**
* repeated bytes values = 2;
*/
com.google.protobuf.ByteString getValues(int index);
// optional int64 guidePostsByteCount = 3;
/**
* optional int64 guidePostsByteCount = 3;
*/
boolean hasGuidePostsByteCount();
/**
* optional int64 guidePostsByteCount = 3;
*/
long getGuidePostsByteCount();
// optional int64 keyBytesCount = 4;
/**
* optional int64 keyBytesCount = 4;
*/
boolean hasKeyBytesCount();
/**
* optional int64 keyBytesCount = 4;
*/
long getKeyBytesCount();
// optional int32 guidePostsCount = 5;
/**
* optional int32 guidePostsCount = 5;
*/
boolean hasGuidePostsCount();
/**
* optional int32 guidePostsCount = 5;
*/
int getGuidePostsCount();
// optional .PGuidePosts pGuidePosts = 6;
/**
* optional .PGuidePosts pGuidePosts = 6;
*/
boolean hasPGuidePosts();
/**
* optional .PGuidePosts pGuidePosts = 6;
*/
org.apache.phoenix.coprocessor.generated.PGuidePostsProtos.PGuidePosts getPGuidePosts();
/**
* optional .PGuidePosts pGuidePosts = 6;
*/
org.apache.phoenix.coprocessor.generated.PGuidePostsProtos.PGuidePostsOrBuilder getPGuidePostsOrBuilder();
}
/**
* Protobuf type {@code PTableStats}
*/
public static final class PTableStats extends
com.google.protobuf.GeneratedMessage
implements PTableStatsOrBuilder {
// Use PTableStats.newBuilder() to construct.
private PTableStats(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private PTableStats(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final PTableStats defaultInstance;
public static PTableStats getDefaultInstance() {
return defaultInstance;
}
public PTableStats getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private PTableStats(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
bitField0_ |= 0x00000001;
key_ = input.readBytes();
break;
}
case 18: {
if (!((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
values_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000002;
}
values_.add(input.readBytes());
break;
}
case 24: {
bitField0_ |= 0x00000002;
guidePostsByteCount_ = input.readInt64();
break;
}
case 32: {
bitField0_ |= 0x00000004;
keyBytesCount_ = input.readInt64();
break;
}
case 40: {
bitField0_ |= 0x00000008;
guidePostsCount_ = input.readInt32();
break;
}
case 50: {
org.apache.phoenix.coprocessor.generated.PGuidePostsProtos.PGuidePosts.Builder subBuilder = null;
if (((bitField0_ & 0x00000010) == 0x00000010)) {
subBuilder = pGuidePosts_.toBuilder();
}
pGuidePosts_ = input.readMessage(org.apache.phoenix.coprocessor.generated.PGuidePostsProtos.PGuidePosts.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(pGuidePosts_);
pGuidePosts_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000010;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
values_ = java.util.Collections.unmodifiableList(values_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.phoenix.coprocessor.generated.PTableProtos.internal_static_PTableStats_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.phoenix.coprocessor.generated.PTableProtos.internal_static_PTableStats_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.phoenix.coprocessor.generated.PTableProtos.PTableStats.class, org.apache.phoenix.coprocessor.generated.PTableProtos.PTableStats.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public PTableStats parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new PTableStats(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// required bytes key = 1;
public static final int KEY_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString key_;
/**
* required bytes key = 1;
*/
public boolean hasKey() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required bytes key = 1;
*/
public com.google.protobuf.ByteString getKey() {
return key_;
}
// repeated bytes values = 2;
public static final int VALUES_FIELD_NUMBER = 2;
private java.util.List values_;
/**
* repeated bytes values = 2;
*/
public java.util.List
getValuesList() {
return values_;
}
/**
* repeated bytes values = 2;
*/
public int getValuesCount() {
return values_.size();
}
/**
* repeated bytes values = 2;
*/
public com.google.protobuf.ByteString getValues(int index) {
return values_.get(index);
}
// optional int64 guidePostsByteCount = 3;
public static final int GUIDEPOSTSBYTECOUNT_FIELD_NUMBER = 3;
private long guidePostsByteCount_;
/**
* optional int64 guidePostsByteCount = 3;
*/
public boolean hasGuidePostsByteCount() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional int64 guidePostsByteCount = 3;
*/
public long getGuidePostsByteCount() {
return guidePostsByteCount_;
}
// optional int64 keyBytesCount = 4;
public static final int KEYBYTESCOUNT_FIELD_NUMBER = 4;
private long keyBytesCount_;
/**
* optional int64 keyBytesCount = 4;
*/
public boolean hasKeyBytesCount() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional int64 keyBytesCount = 4;
*/
public long getKeyBytesCount() {
return keyBytesCount_;
}
// optional int32 guidePostsCount = 5;
public static final int GUIDEPOSTSCOUNT_FIELD_NUMBER = 5;
private int guidePostsCount_;
/**
* optional int32 guidePostsCount = 5;
*/
public boolean hasGuidePostsCount() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional int32 guidePostsCount = 5;
*/
public int getGuidePostsCount() {
return guidePostsCount_;
}
// optional .PGuidePosts pGuidePosts = 6;
public static final int PGUIDEPOSTS_FIELD_NUMBER = 6;
private org.apache.phoenix.coprocessor.generated.PGuidePostsProtos.PGuidePosts pGuidePosts_;
/**
* optional .PGuidePosts pGuidePosts = 6;
*/
public boolean hasPGuidePosts() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional .PGuidePosts pGuidePosts = 6;
*/
public org.apache.phoenix.coprocessor.generated.PGuidePostsProtos.PGuidePosts getPGuidePosts() {
return pGuidePosts_;
}
/**
* optional .PGuidePosts pGuidePosts = 6;
*/
public org.apache.phoenix.coprocessor.generated.PGuidePostsProtos.PGuidePostsOrBuilder getPGuidePostsOrBuilder() {
return pGuidePosts_;
}
private void initFields() {
key_ = com.google.protobuf.ByteString.EMPTY;
values_ = java.util.Collections.emptyList();
guidePostsByteCount_ = 0L;
keyBytesCount_ = 0L;
guidePostsCount_ = 0;
pGuidePosts_ = org.apache.phoenix.coprocessor.generated.PGuidePostsProtos.PGuidePosts.getDefaultInstance();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (!hasKey()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(1, key_);
}
for (int i = 0; i < values_.size(); i++) {
output.writeBytes(2, values_.get(i));
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeInt64(3, guidePostsByteCount_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeInt64(4, keyBytesCount_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeInt32(5, guidePostsCount_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
output.writeMessage(6, pGuidePosts_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, key_);
}
{
int dataSize = 0;
for (int i = 0; i < values_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeBytesSizeNoTag(values_.get(i));
}
size += dataSize;
size += 1 * getValuesList().size();
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(3, guidePostsByteCount_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(4, keyBytesCount_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(5, guidePostsCount_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(6, pGuidePosts_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.phoenix.coprocessor.generated.PTableProtos.PTableStats)) {
return super.equals(obj);
}
org.apache.phoenix.coprocessor.generated.PTableProtos.PTableStats other = (org.apache.phoenix.coprocessor.generated.PTableProtos.PTableStats) obj;
boolean result = true;
result = result && (hasKey() == other.hasKey());
if (hasKey()) {
result = result && getKey()
.equals(other.getKey());
}
result = result && getValuesList()
.equals(other.getValuesList());
result = result && (hasGuidePostsByteCount() == other.hasGuidePostsByteCount());
if (hasGuidePostsByteCount()) {
result = result && (getGuidePostsByteCount()
== other.getGuidePostsByteCount());
}
result = result && (hasKeyBytesCount() == other.hasKeyBytesCount());
if (hasKeyBytesCount()) {
result = result && (getKeyBytesCount()
== other.getKeyBytesCount());
}
result = result && (hasGuidePostsCount() == other.hasGuidePostsCount());
if (hasGuidePostsCount()) {
result = result && (getGuidePostsCount()
== other.getGuidePostsCount());
}
result = result && (hasPGuidePosts() == other.hasPGuidePosts());
if (hasPGuidePosts()) {
result = result && getPGuidePosts()
.equals(other.getPGuidePosts());
}
result = result &&
getUnknownFields().equals(other.getUnknownFields());
return result;
}
private int memoizedHashCode = 0;
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptorForType().hashCode();
if (hasKey()) {
hash = (37 * hash) + KEY_FIELD_NUMBER;
hash = (53 * hash) + getKey().hashCode();
}
if (getValuesCount() > 0) {
hash = (37 * hash) + VALUES_FIELD_NUMBER;
hash = (53 * hash) + getValuesList().hashCode();
}
if (hasGuidePostsByteCount()) {
hash = (37 * hash) + GUIDEPOSTSBYTECOUNT_FIELD_NUMBER;
hash = (53 * hash) + hashLong(getGuidePostsByteCount());
}
if (hasKeyBytesCount()) {
hash = (37 * hash) + KEYBYTESCOUNT_FIELD_NUMBER;
hash = (53 * hash) + hashLong(getKeyBytesCount());
}
if (hasGuidePostsCount()) {
hash = (37 * hash) + GUIDEPOSTSCOUNT_FIELD_NUMBER;
hash = (53 * hash) + getGuidePostsCount();
}
if (hasPGuidePosts()) {
hash = (37 * hash) + PGUIDEPOSTS_FIELD_NUMBER;
hash = (53 * hash) + getPGuidePosts().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.phoenix.coprocessor.generated.PTableProtos.PTableStats parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.phoenix.coprocessor.generated.PTableProtos.PTableStats parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.phoenix.coprocessor.generated.PTableProtos.PTableStats parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.phoenix.coprocessor.generated.PTableProtos.PTableStats parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.phoenix.coprocessor.generated.PTableProtos.PTableStats parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.apache.phoenix.coprocessor.generated.PTableProtos.PTableStats parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.apache.phoenix.coprocessor.generated.PTableProtos.PTableStats parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.apache.phoenix.coprocessor.generated.PTableProtos.PTableStats parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.apache.phoenix.coprocessor.generated.PTableProtos.PTableStats parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.apache.phoenix.coprocessor.generated.PTableProtos.PTableStats parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(org.apache.phoenix.coprocessor.generated.PTableProtos.PTableStats prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code PTableStats}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements org.apache.phoenix.coprocessor.generated.PTableProtos.PTableStatsOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.phoenix.coprocessor.generated.PTableProtos.internal_static_PTableStats_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.phoenix.coprocessor.generated.PTableProtos.internal_static_PTableStats_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.phoenix.coprocessor.generated.PTableProtos.PTableStats.class, org.apache.phoenix.coprocessor.generated.PTableProtos.PTableStats.Builder.class);
}
// Construct using org.apache.phoenix.coprocessor.generated.PTableProtos.PTableStats.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getPGuidePostsFieldBuilder();
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
key_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
values_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
guidePostsByteCount_ = 0L;
bitField0_ = (bitField0_ & ~0x00000004);
keyBytesCount_ = 0L;
bitField0_ = (bitField0_ & ~0x00000008);
guidePostsCount_ = 0;
bitField0_ = (bitField0_ & ~0x00000010);
if (pGuidePostsBuilder_ == null) {
pGuidePosts_ = org.apache.phoenix.coprocessor.generated.PGuidePostsProtos.PGuidePosts.getDefaultInstance();
} else {
pGuidePostsBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000020);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.phoenix.coprocessor.generated.PTableProtos.internal_static_PTableStats_descriptor;
}
public org.apache.phoenix.coprocessor.generated.PTableProtos.PTableStats getDefaultInstanceForType() {
return org.apache.phoenix.coprocessor.generated.PTableProtos.PTableStats.getDefaultInstance();
}
public org.apache.phoenix.coprocessor.generated.PTableProtos.PTableStats build() {
org.apache.phoenix.coprocessor.generated.PTableProtos.PTableStats result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.apache.phoenix.coprocessor.generated.PTableProtos.PTableStats buildPartial() {
org.apache.phoenix.coprocessor.generated.PTableProtos.PTableStats result = new org.apache.phoenix.coprocessor.generated.PTableProtos.PTableStats(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.key_ = key_;
if (((bitField0_ & 0x00000002) == 0x00000002)) {
values_ = java.util.Collections.unmodifiableList(values_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.values_ = values_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000002;
}
result.guidePostsByteCount_ = guidePostsByteCount_;
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000004;
}
result.keyBytesCount_ = keyBytesCount_;
if (((from_bitField0_ & 0x00000010) == 0x00000010)) {
to_bitField0_ |= 0x00000008;
}
result.guidePostsCount_ = guidePostsCount_;
if (((from_bitField0_ & 0x00000020) == 0x00000020)) {
to_bitField0_ |= 0x00000010;
}
if (pGuidePostsBuilder_ == null) {
result.pGuidePosts_ = pGuidePosts_;
} else {
result.pGuidePosts_ = pGuidePostsBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.apache.phoenix.coprocessor.generated.PTableProtos.PTableStats) {
return mergeFrom((org.apache.phoenix.coprocessor.generated.PTableProtos.PTableStats)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.phoenix.coprocessor.generated.PTableProtos.PTableStats other) {
if (other == org.apache.phoenix.coprocessor.generated.PTableProtos.PTableStats.getDefaultInstance()) return this;
if (other.hasKey()) {
setKey(other.getKey());
}
if (!other.values_.isEmpty()) {
if (values_.isEmpty()) {
values_ = other.values_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureValuesIsMutable();
values_.addAll(other.values_);
}
onChanged();
}
if (other.hasGuidePostsByteCount()) {
setGuidePostsByteCount(other.getGuidePostsByteCount());
}
if (other.hasKeyBytesCount()) {
setKeyBytesCount(other.getKeyBytesCount());
}
if (other.hasGuidePostsCount()) {
setGuidePostsCount(other.getGuidePostsCount());
}
if (other.hasPGuidePosts()) {
mergePGuidePosts(other.getPGuidePosts());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasKey()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.phoenix.coprocessor.generated.PTableProtos.PTableStats parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.phoenix.coprocessor.generated.PTableProtos.PTableStats) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// required bytes key = 1;
private com.google.protobuf.ByteString key_ = com.google.protobuf.ByteString.EMPTY;
/**
* required bytes key = 1;
*/
public boolean hasKey() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required bytes key = 1;
*/
public com.google.protobuf.ByteString getKey() {
return key_;
}
/**
* required bytes key = 1;
*/
public Builder setKey(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
key_ = value;
onChanged();
return this;
}
/**
* required bytes key = 1;
*/
public Builder clearKey() {
bitField0_ = (bitField0_ & ~0x00000001);
key_ = getDefaultInstance().getKey();
onChanged();
return this;
}
// repeated bytes values = 2;
private java.util.List values_ = java.util.Collections.emptyList();
private void ensureValuesIsMutable() {
if (!((bitField0_ & 0x00000002) == 0x00000002)) {
values_ = new java.util.ArrayList(values_);
bitField0_ |= 0x00000002;
}
}
/**
* repeated bytes values = 2;
*/
public java.util.List
getValuesList() {
return java.util.Collections.unmodifiableList(values_);
}
/**
* repeated bytes values = 2;
*/
public int getValuesCount() {
return values_.size();
}
/**
* repeated bytes values = 2;
*/
public com.google.protobuf.ByteString getValues(int index) {
return values_.get(index);
}
/**
* repeated bytes values = 2;
*/
public Builder setValues(
int index, com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureValuesIsMutable();
values_.set(index, value);
onChanged();
return this;
}
/**
* repeated bytes values = 2;
*/
public Builder addValues(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureValuesIsMutable();
values_.add(value);
onChanged();
return this;
}
/**
* repeated bytes values = 2;
*/
public Builder addAllValues(
java.lang.Iterable extends com.google.protobuf.ByteString> values) {
ensureValuesIsMutable();
super.addAll(values, values_);
onChanged();
return this;
}
/**
* repeated bytes values = 2;
*/
public Builder clearValues() {
values_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
// optional int64 guidePostsByteCount = 3;
private long guidePostsByteCount_ ;
/**
* optional int64 guidePostsByteCount = 3;
*/
public boolean hasGuidePostsByteCount() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional int64 guidePostsByteCount = 3;
*/
public long getGuidePostsByteCount() {
return guidePostsByteCount_;
}
/**
* optional int64 guidePostsByteCount = 3;
*/
public Builder setGuidePostsByteCount(long value) {
bitField0_ |= 0x00000004;
guidePostsByteCount_ = value;
onChanged();
return this;
}
/**
* optional int64 guidePostsByteCount = 3;
*/
public Builder clearGuidePostsByteCount() {
bitField0_ = (bitField0_ & ~0x00000004);
guidePostsByteCount_ = 0L;
onChanged();
return this;
}
// optional int64 keyBytesCount = 4;
private long keyBytesCount_ ;
/**
* optional int64 keyBytesCount = 4;
*/
public boolean hasKeyBytesCount() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional int64 keyBytesCount = 4;
*/
public long getKeyBytesCount() {
return keyBytesCount_;
}
/**
* optional int64 keyBytesCount = 4;
*/
public Builder setKeyBytesCount(long value) {
bitField0_ |= 0x00000008;
keyBytesCount_ = value;
onChanged();
return this;
}
/**
* optional int64 keyBytesCount = 4;
*/
public Builder clearKeyBytesCount() {
bitField0_ = (bitField0_ & ~0x00000008);
keyBytesCount_ = 0L;
onChanged();
return this;
}
// optional int32 guidePostsCount = 5;
private int guidePostsCount_ ;
/**
* optional int32 guidePostsCount = 5;
*/
public boolean hasGuidePostsCount() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional int32 guidePostsCount = 5;
*/
public int getGuidePostsCount() {
return guidePostsCount_;
}
/**
* optional int32 guidePostsCount = 5;
*/
public Builder setGuidePostsCount(int value) {
bitField0_ |= 0x00000010;
guidePostsCount_ = value;
onChanged();
return this;
}
/**
* optional int32 guidePostsCount = 5;
*/
public Builder clearGuidePostsCount() {
bitField0_ = (bitField0_ & ~0x00000010);
guidePostsCount_ = 0;
onChanged();
return this;
}
// optional .PGuidePosts pGuidePosts = 6;
private org.apache.phoenix.coprocessor.generated.PGuidePostsProtos.PGuidePosts pGuidePosts_ = org.apache.phoenix.coprocessor.generated.PGuidePostsProtos.PGuidePosts.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
org.apache.phoenix.coprocessor.generated.PGuidePostsProtos.PGuidePosts, org.apache.phoenix.coprocessor.generated.PGuidePostsProtos.PGuidePosts.Builder, org.apache.phoenix.coprocessor.generated.PGuidePostsProtos.PGuidePostsOrBuilder> pGuidePostsBuilder_;
/**
* optional .PGuidePosts pGuidePosts = 6;
*/
public boolean hasPGuidePosts() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* optional .PGuidePosts pGuidePosts = 6;
*/
public org.apache.phoenix.coprocessor.generated.PGuidePostsProtos.PGuidePosts getPGuidePosts() {
if (pGuidePostsBuilder_ == null) {
return pGuidePosts_;
} else {
return pGuidePostsBuilder_.getMessage();
}
}
/**
* optional .PGuidePosts pGuidePosts = 6;
*/
public Builder setPGuidePosts(org.apache.phoenix.coprocessor.generated.PGuidePostsProtos.PGuidePosts value) {
if (pGuidePostsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
pGuidePosts_ = value;
onChanged();
} else {
pGuidePostsBuilder_.setMessage(value);
}
bitField0_ |= 0x00000020;
return this;
}
/**
* optional .PGuidePosts pGuidePosts = 6;
*/
public Builder setPGuidePosts(
org.apache.phoenix.coprocessor.generated.PGuidePostsProtos.PGuidePosts.Builder builderForValue) {
if (pGuidePostsBuilder_ == null) {
pGuidePosts_ = builderForValue.build();
onChanged();
} else {
pGuidePostsBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000020;
return this;
}
/**
* optional .PGuidePosts pGuidePosts = 6;
*/
public Builder mergePGuidePosts(org.apache.phoenix.coprocessor.generated.PGuidePostsProtos.PGuidePosts value) {
if (pGuidePostsBuilder_ == null) {
if (((bitField0_ & 0x00000020) == 0x00000020) &&
pGuidePosts_ != org.apache.phoenix.coprocessor.generated.PGuidePostsProtos.PGuidePosts.getDefaultInstance()) {
pGuidePosts_ =
org.apache.phoenix.coprocessor.generated.PGuidePostsProtos.PGuidePosts.newBuilder(pGuidePosts_).mergeFrom(value).buildPartial();
} else {
pGuidePosts_ = value;
}
onChanged();
} else {
pGuidePostsBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000020;
return this;
}
/**
* optional .PGuidePosts pGuidePosts = 6;
*/
public Builder clearPGuidePosts() {
if (pGuidePostsBuilder_ == null) {
pGuidePosts_ = org.apache.phoenix.coprocessor.generated.PGuidePostsProtos.PGuidePosts.getDefaultInstance();
onChanged();
} else {
pGuidePostsBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000020);
return this;
}
/**
* optional .PGuidePosts pGuidePosts = 6;
*/
public org.apache.phoenix.coprocessor.generated.PGuidePostsProtos.PGuidePosts.Builder getPGuidePostsBuilder() {
bitField0_ |= 0x00000020;
onChanged();
return getPGuidePostsFieldBuilder().getBuilder();
}
/**
* optional .PGuidePosts pGuidePosts = 6;
*/
public org.apache.phoenix.coprocessor.generated.PGuidePostsProtos.PGuidePostsOrBuilder getPGuidePostsOrBuilder() {
if (pGuidePostsBuilder_ != null) {
return pGuidePostsBuilder_.getMessageOrBuilder();
} else {
return pGuidePosts_;
}
}
/**
* optional .PGuidePosts pGuidePosts = 6;
*/
private com.google.protobuf.SingleFieldBuilder<
org.apache.phoenix.coprocessor.generated.PGuidePostsProtos.PGuidePosts, org.apache.phoenix.coprocessor.generated.PGuidePostsProtos.PGuidePosts.Builder, org.apache.phoenix.coprocessor.generated.PGuidePostsProtos.PGuidePostsOrBuilder>
getPGuidePostsFieldBuilder() {
if (pGuidePostsBuilder_ == null) {
pGuidePostsBuilder_ = new com.google.protobuf.SingleFieldBuilder<
org.apache.phoenix.coprocessor.generated.PGuidePostsProtos.PGuidePosts, org.apache.phoenix.coprocessor.generated.PGuidePostsProtos.PGuidePosts.Builder, org.apache.phoenix.coprocessor.generated.PGuidePostsProtos.PGuidePostsOrBuilder>(
pGuidePosts_,
getParentForChildren(),
isClean());
pGuidePosts_ = null;
}
return pGuidePostsBuilder_;
}
// @@protoc_insertion_point(builder_scope:PTableStats)
}
static {
defaultInstance = new PTableStats(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:PTableStats)
}
public interface PTableOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// required bytes schemaNameBytes = 1;
/**
* required bytes schemaNameBytes = 1;
*/
boolean hasSchemaNameBytes();
/**
* required bytes schemaNameBytes = 1;
*/
com.google.protobuf.ByteString getSchemaNameBytes();
// required bytes tableNameBytes = 2;
/**
* required bytes tableNameBytes = 2;
*/
boolean hasTableNameBytes();
/**
* required bytes tableNameBytes = 2;
*/
com.google.protobuf.ByteString getTableNameBytes();
// required .PTableType tableType = 3;
/**
* required .PTableType tableType = 3;
*/
boolean hasTableType();
/**
* required .PTableType tableType = 3;
*/
org.apache.phoenix.coprocessor.generated.PTableProtos.PTableType getTableType();
// optional string indexState = 4;
/**
* optional string indexState = 4;
*/
boolean hasIndexState();
/**
* optional string indexState = 4;
*/
java.lang.String getIndexState();
/**
* optional string indexState = 4;
*/
com.google.protobuf.ByteString
getIndexStateBytes();
// required int64 sequenceNumber = 5;
/**
* required int64 sequenceNumber = 5;
*/
boolean hasSequenceNumber();
/**
* required int64 sequenceNumber = 5;
*/
long getSequenceNumber();
// required int64 timeStamp = 6;
/**
* required int64 timeStamp = 6;
*/
boolean hasTimeStamp();
/**
* required int64 timeStamp = 6;
*/
long getTimeStamp();
// optional bytes pkNameBytes = 7;
/**
* optional bytes pkNameBytes = 7;
*/
boolean hasPkNameBytes();
/**
* optional bytes pkNameBytes = 7;
*/
com.google.protobuf.ByteString getPkNameBytes();
// required int32 bucketNum = 8;
/**
* required int32 bucketNum = 8;
*/
boolean hasBucketNum();
/**
* required int32 bucketNum = 8;
*/
int getBucketNum();
// repeated .PColumn columns = 9;
/**
* repeated .PColumn columns = 9;
*/
java.util.List
getColumnsList();
/**
* repeated .PColumn columns = 9;
*/
org.apache.phoenix.coprocessor.generated.PTableProtos.PColumn getColumns(int index);
/**
* repeated .PColumn columns = 9;
*/
int getColumnsCount();
/**
* repeated .PColumn columns = 9;
*/
java.util.List extends org.apache.phoenix.coprocessor.generated.PTableProtos.PColumnOrBuilder>
getColumnsOrBuilderList();
/**
* repeated .PColumn columns = 9;
*/
org.apache.phoenix.coprocessor.generated.PTableProtos.PColumnOrBuilder getColumnsOrBuilder(
int index);
// repeated .PTable indexes = 10;
/**
* repeated .PTable indexes = 10;
*/
java.util.List
getIndexesList();
/**
* repeated .PTable indexes = 10;
*/
org.apache.phoenix.coprocessor.generated.PTableProtos.PTable getIndexes(int index);
/**
* repeated .PTable indexes = 10;
*/
int getIndexesCount();
/**
* repeated .PTable indexes = 10;
*/
java.util.List extends org.apache.phoenix.coprocessor.generated.PTableProtos.PTableOrBuilder>
getIndexesOrBuilderList();
/**
* repeated .PTable indexes = 10;
*/
org.apache.phoenix.coprocessor.generated.PTableProtos.PTableOrBuilder getIndexesOrBuilder(
int index);
// required bool isImmutableRows = 11;
/**
* required bool isImmutableRows = 11;
*/
boolean hasIsImmutableRows();
/**
* required bool isImmutableRows = 11;
*/
boolean getIsImmutableRows();
// optional bytes dataTableNameBytes = 13;
/**
* optional bytes dataTableNameBytes = 13;
*
*
* Do NOT reuse the tag '12'. Stats are no longer passed
* along with the PTable.
*repeated PTableStats guidePosts = 12;
* TODO remove this field in 5.0 release
*
*/
boolean hasDataTableNameBytes();
/**
* optional bytes dataTableNameBytes = 13;
*
*
* Do NOT reuse the tag '12'. Stats are no longer passed
* along with the PTable.
*repeated PTableStats guidePosts = 12;
* TODO remove this field in 5.0 release
*
*/
com.google.protobuf.ByteString getDataTableNameBytes();
// optional bytes defaultFamilyName = 14;
/**
* optional bytes defaultFamilyName = 14;
*/
boolean hasDefaultFamilyName();
/**
* optional bytes defaultFamilyName = 14;
*/
com.google.protobuf.ByteString getDefaultFamilyName();
// required bool disableWAL = 15;
/**
* required bool disableWAL = 15;
*/
boolean hasDisableWAL();
/**
* required bool disableWAL = 15;
*/
boolean getDisableWAL();
// required bool multiTenant = 16;
/**
* required bool multiTenant = 16;
*/
boolean hasMultiTenant();
/**
* required bool multiTenant = 16;
*/
boolean getMultiTenant();
// optional bytes viewType = 17;
/**
* optional bytes viewType = 17;
*/
boolean hasViewType();
/**
* optional bytes viewType = 17;
*/
com.google.protobuf.ByteString getViewType();
// optional bytes viewStatement = 18;
/**
* optional bytes viewStatement = 18;
*/
boolean hasViewStatement();
/**
* optional bytes viewStatement = 18;
*/
com.google.protobuf.ByteString getViewStatement();
// repeated bytes physicalNames = 19;
/**
* repeated bytes physicalNames = 19;
*/
java.util.List getPhysicalNamesList();
/**
* repeated bytes physicalNames = 19;
*/
int getPhysicalNamesCount();
/**
* repeated bytes physicalNames = 19;
*/
com.google.protobuf.ByteString getPhysicalNames(int index);
// optional bytes tenantId = 20;
/**
* optional bytes tenantId = 20;
*/
boolean hasTenantId();
/**
* optional bytes tenantId = 20;
*/
com.google.protobuf.ByteString getTenantId();
// optional int64 viewIndexId = 21;
/**
* optional int64 viewIndexId = 21;
*/
boolean hasViewIndexId();
/**
* optional int64 viewIndexId = 21;
*/
long getViewIndexId();
// optional bytes indexType = 22;
/**
* optional bytes indexType = 22;
*/
boolean hasIndexType();
/**
* optional bytes indexType = 22;
*/
com.google.protobuf.ByteString getIndexType();
// optional int64 statsTimeStamp = 23;
/**
* optional int64 statsTimeStamp = 23;
*/
boolean hasStatsTimeStamp();
/**
* optional int64 statsTimeStamp = 23;
*/
long getStatsTimeStamp();
// optional bool storeNulls = 24;
/**
* optional bool storeNulls = 24;
*/
boolean hasStoreNulls();
/**
* optional bool storeNulls = 24;
*/
boolean getStoreNulls();
// optional int32 baseColumnCount = 25;
/**
* optional int32 baseColumnCount = 25;
*/
boolean hasBaseColumnCount();
/**
* optional int32 baseColumnCount = 25;
*/
int getBaseColumnCount();
// optional bool rowKeyOrderOptimizable = 26;
/**
* optional bool rowKeyOrderOptimizable = 26;
*/
boolean hasRowKeyOrderOptimizable();
/**
* optional bool rowKeyOrderOptimizable = 26;
*/
boolean getRowKeyOrderOptimizable();
// optional bool transactional = 27;
/**
* optional bool transactional = 27;
*/
boolean hasTransactional();
/**
* optional bool transactional = 27;
*/
boolean getTransactional();
// optional int64 updateCacheFrequency = 28;
/**
* optional int64 updateCacheFrequency = 28;
*/
boolean hasUpdateCacheFrequency();
/**
* optional int64 updateCacheFrequency = 28;
*/
long getUpdateCacheFrequency();
// optional int64 indexDisableTimestamp = 29;
/**
* optional int64 indexDisableTimestamp = 29;
*/
boolean hasIndexDisableTimestamp();
/**
* optional int64 indexDisableTimestamp = 29;
*/
long getIndexDisableTimestamp();
// optional bool isNamespaceMapped = 30;
/**
* optional bool isNamespaceMapped = 30;
*/
boolean hasIsNamespaceMapped();
/**
* optional bool isNamespaceMapped = 30;
*/
boolean getIsNamespaceMapped();
// optional string autoParititonSeqName = 31;
/**
* optional string autoParititonSeqName = 31;
*/
boolean hasAutoParititonSeqName();
/**
* optional string autoParititonSeqName = 31;
*/
java.lang.String getAutoParititonSeqName();
/**
* optional string autoParititonSeqName = 31;
*/
com.google.protobuf.ByteString
getAutoParititonSeqNameBytes();
// optional bool isAppendOnlySchema = 32;
/**
* optional bool isAppendOnlySchema = 32;
*/
boolean hasIsAppendOnlySchema();
/**
* optional bool isAppendOnlySchema = 32;
*/
boolean getIsAppendOnlySchema();
// optional bytes parentNameBytes = 33;
/**
* optional bytes parentNameBytes = 33;
*/
boolean hasParentNameBytes();
/**
* optional bytes parentNameBytes = 33;
*/
com.google.protobuf.ByteString getParentNameBytes();
// optional bytes storageScheme = 34;
/**
* optional bytes storageScheme = 34;
*/
boolean hasStorageScheme();
/**
* optional bytes storageScheme = 34;
*/
com.google.protobuf.ByteString getStorageScheme();
// optional bytes encodingScheme = 35;
/**
* optional bytes encodingScheme = 35;
*/
boolean hasEncodingScheme();
/**
* optional bytes encodingScheme = 35;
*/
com.google.protobuf.ByteString getEncodingScheme();
// repeated .EncodedCQCounter encodedCQCounters = 36;
/**
* repeated .EncodedCQCounter encodedCQCounters = 36;
*/
java.util.List
getEncodedCQCountersList();
/**
* repeated .EncodedCQCounter encodedCQCounters = 36;
*/
org.apache.phoenix.coprocessor.generated.PTableProtos.EncodedCQCounter getEncodedCQCounters(int index);
/**
* repeated .EncodedCQCounter encodedCQCounters = 36;
*/
int getEncodedCQCountersCount();
/**
* repeated .EncodedCQCounter encodedCQCounters = 36;
*/
java.util.List extends org.apache.phoenix.coprocessor.generated.PTableProtos.EncodedCQCounterOrBuilder>
getEncodedCQCountersOrBuilderList();
/**
* repeated .EncodedCQCounter encodedCQCounters = 36;
*/
org.apache.phoenix.coprocessor.generated.PTableProtos.EncodedCQCounterOrBuilder getEncodedCQCountersOrBuilder(
int index);
// optional bool useStatsForParallelization = 37;
/**
* optional bool useStatsForParallelization = 37;
*/
boolean hasUseStatsForParallelization();
/**
* optional bool useStatsForParallelization = 37;
*/
boolean getUseStatsForParallelization();
// optional int32 transactionProvider = 38;
/**
* optional int32 transactionProvider = 38;
*/
boolean hasTransactionProvider();
/**
* optional int32 transactionProvider = 38;
*/
int getTransactionProvider();
// optional int32 viewIndexIdType = 39 [default = 5];
/**
* optional int32 viewIndexIdType = 39 [default = 5];
*/
boolean hasViewIndexIdType();
/**
* optional int32 viewIndexIdType = 39 [default = 5];
*/
int getViewIndexIdType();
// optional bool viewModifiedUpdateCacheFrequency = 40;
/**
* optional bool viewModifiedUpdateCacheFrequency = 40;
*/
boolean hasViewModifiedUpdateCacheFrequency();
/**
* optional bool viewModifiedUpdateCacheFrequency = 40;
*/
boolean getViewModifiedUpdateCacheFrequency();
// optional bool viewModifiedUseStatsForParallelization = 41;
/**
* optional bool viewModifiedUseStatsForParallelization = 41;
*/
boolean hasViewModifiedUseStatsForParallelization();
/**
* optional bool viewModifiedUseStatsForParallelization = 41;
*/
boolean getViewModifiedUseStatsForParallelization();
// optional int64 phoenixTTL = 42;
/**
* optional int64 phoenixTTL = 42;
*/
boolean hasPhoenixTTL();
/**
* optional int64 phoenixTTL = 42;
*/
long getPhoenixTTL();
// optional int64 phoenixTTLHighWaterMark = 43;
/**
* optional int64 phoenixTTLHighWaterMark = 43;
*/
boolean hasPhoenixTTLHighWaterMark();
/**
* optional int64 phoenixTTLHighWaterMark = 43;
*/
long getPhoenixTTLHighWaterMark();
// optional bool viewModifiedPhoenixTTL = 44;
/**
* optional bool viewModifiedPhoenixTTL = 44;
*/
boolean hasViewModifiedPhoenixTTL();
/**
* optional bool viewModifiedPhoenixTTL = 44;
*/
boolean getViewModifiedPhoenixTTL();
// optional int64 lastDDLTimestamp = 45;
/**
* optional int64 lastDDLTimestamp = 45;
*/
boolean hasLastDDLTimestamp();
/**
* optional int64 lastDDLTimestamp = 45;
*/
long getLastDDLTimestamp();
// optional bool changeDetectionEnabled = 46;
/**
* optional bool changeDetectionEnabled = 46;
*/
boolean hasChangeDetectionEnabled();
/**
* optional bool changeDetectionEnabled = 46;
*/
boolean getChangeDetectionEnabled();
}
/**
* Protobuf type {@code PTable}
*/
public static final class PTable extends
com.google.protobuf.GeneratedMessage
implements PTableOrBuilder {
// Use PTable.newBuilder() to construct.
private PTable(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private PTable(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final PTable defaultInstance;
public static PTable getDefaultInstance() {
return defaultInstance;
}
public PTable getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private PTable(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
int mutable_bitField1_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
bitField0_ |= 0x00000001;
schemaNameBytes_ = input.readBytes();
break;
}
case 18: {
bitField0_ |= 0x00000002;
tableNameBytes_ = input.readBytes();
break;
}
case 24: {
int rawValue = input.readEnum();
org.apache.phoenix.coprocessor.generated.PTableProtos.PTableType value = org.apache.phoenix.coprocessor.generated.PTableProtos.PTableType.valueOf(rawValue);
if (value == null) {
unknownFields.mergeVarintField(3, rawValue);
} else {
bitField0_ |= 0x00000004;
tableType_ = value;
}
break;
}
case 34: {
bitField0_ |= 0x00000008;
indexState_ = input.readBytes();
break;
}
case 40: {
bitField0_ |= 0x00000010;
sequenceNumber_ = input.readInt64();
break;
}
case 48: {
bitField0_ |= 0x00000020;
timeStamp_ = input.readInt64();
break;
}
case 58: {
bitField0_ |= 0x00000040;
pkNameBytes_ = input.readBytes();
break;
}
case 64: {
bitField0_ |= 0x00000080;
bucketNum_ = input.readInt32();
break;
}
case 74: {
if (!((mutable_bitField0_ & 0x00000100) == 0x00000100)) {
columns_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000100;
}
columns_.add(input.readMessage(org.apache.phoenix.coprocessor.generated.PTableProtos.PColumn.PARSER, extensionRegistry));
break;
}
case 82: {
if (!((mutable_bitField0_ & 0x00000200) == 0x00000200)) {
indexes_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000200;
}
indexes_.add(input.readMessage(org.apache.phoenix.coprocessor.generated.PTableProtos.PTable.PARSER, extensionRegistry));
break;
}
case 88: {
bitField0_ |= 0x00000100;
isImmutableRows_ = input.readBool();
break;
}
case 106: {
bitField0_ |= 0x00000200;
dataTableNameBytes_ = input.readBytes();
break;
}
case 114: {
bitField0_ |= 0x00000400;
defaultFamilyName_ = input.readBytes();
break;
}
case 120: {
bitField0_ |= 0x00000800;
disableWAL_ = input.readBool();
break;
}
case 128: {
bitField0_ |= 0x00001000;
multiTenant_ = input.readBool();
break;
}
case 138: {
bitField0_ |= 0x00002000;
viewType_ = input.readBytes();
break;
}
case 146: {
bitField0_ |= 0x00004000;
viewStatement_ = input.readBytes();
break;
}
case 154: {
if (!((mutable_bitField0_ & 0x00020000) == 0x00020000)) {
physicalNames_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00020000;
}
physicalNames_.add(input.readBytes());
break;
}
case 162: {
bitField0_ |= 0x00008000;
tenantId_ = input.readBytes();
break;
}
case 168: {
bitField0_ |= 0x00010000;
viewIndexId_ = input.readInt64();
break;
}
case 178: {
bitField0_ |= 0x00020000;
indexType_ = input.readBytes();
break;
}
case 184: {
bitField0_ |= 0x00040000;
statsTimeStamp_ = input.readInt64();
break;
}
case 192: {
bitField0_ |= 0x00080000;
storeNulls_ = input.readBool();
break;
}
case 200: {
bitField0_ |= 0x00100000;
baseColumnCount_ = input.readInt32();
break;
}
case 208: {
bitField0_ |= 0x00200000;
rowKeyOrderOptimizable_ = input.readBool();
break;
}
case 216: {
bitField0_ |= 0x00400000;
transactional_ = input.readBool();
break;
}
case 224: {
bitField0_ |= 0x00800000;
updateCacheFrequency_ = input.readInt64();
break;
}
case 232: {
bitField0_ |= 0x01000000;
indexDisableTimestamp_ = input.readInt64();
break;
}
case 240: {
bitField0_ |= 0x02000000;
isNamespaceMapped_ = input.readBool();
break;
}
case 250: {
bitField0_ |= 0x04000000;
autoParititonSeqName_ = input.readBytes();
break;
}
case 256: {
bitField0_ |= 0x08000000;
isAppendOnlySchema_ = input.readBool();
break;
}
case 266: {
bitField0_ |= 0x10000000;
parentNameBytes_ = input.readBytes();
break;
}
case 274: {
bitField0_ |= 0x20000000;
storageScheme_ = input.readBytes();
break;
}
case 282: {
bitField0_ |= 0x40000000;
encodingScheme_ = input.readBytes();
break;
}
case 290: {
if (!((mutable_bitField1_ & 0x00000004) == 0x00000004)) {
encodedCQCounters_ = new java.util.ArrayList();
mutable_bitField1_ |= 0x00000004;
}
encodedCQCounters_.add(input.readMessage(org.apache.phoenix.coprocessor.generated.PTableProtos.EncodedCQCounter.PARSER, extensionRegistry));
break;
}
case 296: {
bitField0_ |= 0x80000000;
useStatsForParallelization_ = input.readBool();
break;
}
case 304: {
bitField1_ |= 0x00000001;
transactionProvider_ = input.readInt32();
break;
}
case 312: {
bitField1_ |= 0x00000002;
viewIndexIdType_ = input.readInt32();
break;
}
case 320: {
bitField1_ |= 0x00000004;
viewModifiedUpdateCacheFrequency_ = input.readBool();
break;
}
case 328: {
bitField1_ |= 0x00000008;
viewModifiedUseStatsForParallelization_ = input.readBool();
break;
}
case 336: {
bitField1_ |= 0x00000010;
phoenixTTL_ = input.readInt64();
break;
}
case 344: {
bitField1_ |= 0x00000020;
phoenixTTLHighWaterMark_ = input.readInt64();
break;
}
case 352: {
bitField1_ |= 0x00000040;
viewModifiedPhoenixTTL_ = input.readBool();
break;
}
case 360: {
bitField1_ |= 0x00000080;
lastDDLTimestamp_ = input.readInt64();
break;
}
case 368: {
bitField1_ |= 0x00000100;
changeDetectionEnabled_ = input.readBool();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000100) == 0x00000100)) {
columns_ = java.util.Collections.unmodifiableList(columns_);
}
if (((mutable_bitField0_ & 0x00000200) == 0x00000200)) {
indexes_ = java.util.Collections.unmodifiableList(indexes_);
}
if (((mutable_bitField0_ & 0x00020000) == 0x00020000)) {
physicalNames_ = java.util.Collections.unmodifiableList(physicalNames_);
}
if (((mutable_bitField1_ & 0x00000004) == 0x00000004)) {
encodedCQCounters_ = java.util.Collections.unmodifiableList(encodedCQCounters_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.phoenix.coprocessor.generated.PTableProtos.internal_static_PTable_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.phoenix.coprocessor.generated.PTableProtos.internal_static_PTable_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.phoenix.coprocessor.generated.PTableProtos.PTable.class, org.apache.phoenix.coprocessor.generated.PTableProtos.PTable.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public PTable parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new PTable(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
private int bitField1_;
// required bytes schemaNameBytes = 1;
public static final int SCHEMANAMEBYTES_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString schemaNameBytes_;
/**
* required bytes schemaNameBytes = 1;
*/
public boolean hasSchemaNameBytes() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required bytes schemaNameBytes = 1;
*/
public com.google.protobuf.ByteString getSchemaNameBytes() {
return schemaNameBytes_;
}
// required bytes tableNameBytes = 2;
public static final int TABLENAMEBYTES_FIELD_NUMBER = 2;
private com.google.protobuf.ByteString tableNameBytes_;
/**
* required bytes tableNameBytes = 2;
*/
public boolean hasTableNameBytes() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required bytes tableNameBytes = 2;
*/
public com.google.protobuf.ByteString getTableNameBytes() {
return tableNameBytes_;
}
// required .PTableType tableType = 3;
public static final int TABLETYPE_FIELD_NUMBER = 3;
private org.apache.phoenix.coprocessor.generated.PTableProtos.PTableType tableType_;
/**
* required .PTableType tableType = 3;
*/
public boolean hasTableType() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* required .PTableType tableType = 3;
*/
public org.apache.phoenix.coprocessor.generated.PTableProtos.PTableType getTableType() {
return tableType_;
}
// optional string indexState = 4;
public static final int INDEXSTATE_FIELD_NUMBER = 4;
private java.lang.Object indexState_;
/**
* optional string indexState = 4;
*/
public boolean hasIndexState() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional string indexState = 4;
*/
public java.lang.String getIndexState() {
java.lang.Object ref = indexState_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
indexState_ = s;
}
return s;
}
}
/**
* optional string indexState = 4;
*/
public com.google.protobuf.ByteString
getIndexStateBytes() {
java.lang.Object ref = indexState_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
indexState_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// required int64 sequenceNumber = 5;
public static final int SEQUENCENUMBER_FIELD_NUMBER = 5;
private long sequenceNumber_;
/**
* required int64 sequenceNumber = 5;
*/
public boolean hasSequenceNumber() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* required int64 sequenceNumber = 5;
*/
public long getSequenceNumber() {
return sequenceNumber_;
}
// required int64 timeStamp = 6;
public static final int TIMESTAMP_FIELD_NUMBER = 6;
private long timeStamp_;
/**
* required int64 timeStamp = 6;
*/
public boolean hasTimeStamp() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* required int64 timeStamp = 6;
*/
public long getTimeStamp() {
return timeStamp_;
}
// optional bytes pkNameBytes = 7;
public static final int PKNAMEBYTES_FIELD_NUMBER = 7;
private com.google.protobuf.ByteString pkNameBytes_;
/**
* optional bytes pkNameBytes = 7;
*/
public boolean hasPkNameBytes() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
* optional bytes pkNameBytes = 7;
*/
public com.google.protobuf.ByteString getPkNameBytes() {
return pkNameBytes_;
}
// required int32 bucketNum = 8;
public static final int BUCKETNUM_FIELD_NUMBER = 8;
private int bucketNum_;
/**
* required int32 bucketNum = 8;
*/
public boolean hasBucketNum() {
return ((bitField0_ & 0x00000080) == 0x00000080);
}
/**
* required int32 bucketNum = 8;
*/
public int getBucketNum() {
return bucketNum_;
}
// repeated .PColumn columns = 9;
public static final int COLUMNS_FIELD_NUMBER = 9;
private java.util.List columns_;
/**
* repeated .PColumn columns = 9;
*/
public java.util.List getColumnsList() {
return columns_;
}
/**
* repeated .PColumn columns = 9;
*/
public java.util.List extends org.apache.phoenix.coprocessor.generated.PTableProtos.PColumnOrBuilder>
getColumnsOrBuilderList() {
return columns_;
}
/**
* repeated .PColumn columns = 9;
*/
public int getColumnsCount() {
return columns_.size();
}
/**
* repeated .PColumn columns = 9;
*/
public org.apache.phoenix.coprocessor.generated.PTableProtos.PColumn getColumns(int index) {
return columns_.get(index);
}
/**
* repeated .PColumn columns = 9;
*/
public org.apache.phoenix.coprocessor.generated.PTableProtos.PColumnOrBuilder getColumnsOrBuilder(
int index) {
return columns_.get(index);
}
// repeated .PTable indexes = 10;
public static final int INDEXES_FIELD_NUMBER = 10;
private java.util.List indexes_;
/**
* repeated .PTable indexes = 10;
*/
public java.util.List getIndexesList() {
return indexes_;
}
/**
* repeated .PTable indexes = 10;
*/
public java.util.List extends org.apache.phoenix.coprocessor.generated.PTableProtos.PTableOrBuilder>
getIndexesOrBuilderList() {
return indexes_;
}
/**
* repeated .PTable indexes = 10;
*/
public int getIndexesCount() {
return indexes_.size();
}
/**
* repeated .PTable indexes = 10;
*/
public org.apache.phoenix.coprocessor.generated.PTableProtos.PTable getIndexes(int index) {
return indexes_.get(index);
}
/**
* repeated .PTable indexes = 10;
*/
public org.apache.phoenix.coprocessor.generated.PTableProtos.PTableOrBuilder getIndexesOrBuilder(
int index) {
return indexes_.get(index);
}
// required bool isImmutableRows = 11;
public static final int ISIMMUTABLEROWS_FIELD_NUMBER = 11;
private boolean isImmutableRows_;
/**
* required bool isImmutableRows = 11;
*/
public boolean hasIsImmutableRows() {
return ((bitField0_ & 0x00000100) == 0x00000100);
}
/**
* required bool isImmutableRows = 11;
*/
public boolean getIsImmutableRows() {
return isImmutableRows_;
}
// optional bytes dataTableNameBytes = 13;
public static final int DATATABLENAMEBYTES_FIELD_NUMBER = 13;
private com.google.protobuf.ByteString dataTableNameBytes_;
/**
* optional bytes dataTableNameBytes = 13;
*
*
* Do NOT reuse the tag '12'. Stats are no longer passed
* along with the PTable.
*repeated PTableStats guidePosts = 12;
* TODO remove this field in 5.0 release
*
*/
public boolean hasDataTableNameBytes() {
return ((bitField0_ & 0x00000200) == 0x00000200);
}
/**
* optional bytes dataTableNameBytes = 13;
*
*
* Do NOT reuse the tag '12'. Stats are no longer passed
* along with the PTable.
*repeated PTableStats guidePosts = 12;
* TODO remove this field in 5.0 release
*
*/
public com.google.protobuf.ByteString getDataTableNameBytes() {
return dataTableNameBytes_;
}
// optional bytes defaultFamilyName = 14;
public static final int DEFAULTFAMILYNAME_FIELD_NUMBER = 14;
private com.google.protobuf.ByteString defaultFamilyName_;
/**
* optional bytes defaultFamilyName = 14;
*/
public boolean hasDefaultFamilyName() {
return ((bitField0_ & 0x00000400) == 0x00000400);
}
/**
* optional bytes defaultFamilyName = 14;
*/
public com.google.protobuf.ByteString getDefaultFamilyName() {
return defaultFamilyName_;
}
// required bool disableWAL = 15;
public static final int DISABLEWAL_FIELD_NUMBER = 15;
private boolean disableWAL_;
/**
* required bool disableWAL = 15;
*/
public boolean hasDisableWAL() {
return ((bitField0_ & 0x00000800) == 0x00000800);
}
/**
* required bool disableWAL = 15;
*/
public boolean getDisableWAL() {
return disableWAL_;
}
// required bool multiTenant = 16;
public static final int MULTITENANT_FIELD_NUMBER = 16;
private boolean multiTenant_;
/**
* required bool multiTenant = 16;
*/
public boolean hasMultiTenant() {
return ((bitField0_ & 0x00001000) == 0x00001000);
}
/**
* required bool multiTenant = 16;
*/
public boolean getMultiTenant() {
return multiTenant_;
}
// optional bytes viewType = 17;
public static final int VIEWTYPE_FIELD_NUMBER = 17;
private com.google.protobuf.ByteString viewType_;
/**
* optional bytes viewType = 17;
*/
public boolean hasViewType() {
return ((bitField0_ & 0x00002000) == 0x00002000);
}
/**
* optional bytes viewType = 17;
*/
public com.google.protobuf.ByteString getViewType() {
return viewType_;
}
// optional bytes viewStatement = 18;
public static final int VIEWSTATEMENT_FIELD_NUMBER = 18;
private com.google.protobuf.ByteString viewStatement_;
/**
* optional bytes viewStatement = 18;
*/
public boolean hasViewStatement() {
return ((bitField0_ & 0x00004000) == 0x00004000);
}
/**
* optional bytes viewStatement = 18;
*/
public com.google.protobuf.ByteString getViewStatement() {
return viewStatement_;
}
// repeated bytes physicalNames = 19;
public static final int PHYSICALNAMES_FIELD_NUMBER = 19;
private java.util.List physicalNames_;
/**
* repeated bytes physicalNames = 19;
*/
public java.util.List
getPhysicalNamesList() {
return physicalNames_;
}
/**
* repeated bytes physicalNames = 19;
*/
public int getPhysicalNamesCount() {
return physicalNames_.size();
}
/**
* repeated bytes physicalNames = 19;
*/
public com.google.protobuf.ByteString getPhysicalNames(int index) {
return physicalNames_.get(index);
}
// optional bytes tenantId = 20;
public static final int TENANTID_FIELD_NUMBER = 20;
private com.google.protobuf.ByteString tenantId_;
/**
* optional bytes tenantId = 20;
*/
public boolean hasTenantId() {
return ((bitField0_ & 0x00008000) == 0x00008000);
}
/**
* optional bytes tenantId = 20;
*/
public com.google.protobuf.ByteString getTenantId() {
return tenantId_;
}
// optional int64 viewIndexId = 21;
public static final int VIEWINDEXID_FIELD_NUMBER = 21;
private long viewIndexId_;
/**
* optional int64 viewIndexId = 21;
*/
public boolean hasViewIndexId() {
return ((bitField0_ & 0x00010000) == 0x00010000);
}
/**
* optional int64 viewIndexId = 21;
*/
public long getViewIndexId() {
return viewIndexId_;
}
// optional bytes indexType = 22;
public static final int INDEXTYPE_FIELD_NUMBER = 22;
private com.google.protobuf.ByteString indexType_;
/**
* optional bytes indexType = 22;
*/
public boolean hasIndexType() {
return ((bitField0_ & 0x00020000) == 0x00020000);
}
/**
* optional bytes indexType = 22;
*/
public com.google.protobuf.ByteString getIndexType() {
return indexType_;
}
// optional int64 statsTimeStamp = 23;
public static final int STATSTIMESTAMP_FIELD_NUMBER = 23;
private long statsTimeStamp_;
/**
* optional int64 statsTimeStamp = 23;
*/
public boolean hasStatsTimeStamp() {
return ((bitField0_ & 0x00040000) == 0x00040000);
}
/**
* optional int64 statsTimeStamp = 23;
*/
public long getStatsTimeStamp() {
return statsTimeStamp_;
}
// optional bool storeNulls = 24;
public static final int STORENULLS_FIELD_NUMBER = 24;
private boolean storeNulls_;
/**
* optional bool storeNulls = 24;
*/
public boolean hasStoreNulls() {
return ((bitField0_ & 0x00080000) == 0x00080000);
}
/**
* optional bool storeNulls = 24;
*/
public boolean getStoreNulls() {
return storeNulls_;
}
// optional int32 baseColumnCount = 25;
public static final int BASECOLUMNCOUNT_FIELD_NUMBER = 25;
private int baseColumnCount_;
/**
* optional int32 baseColumnCount = 25;
*/
public boolean hasBaseColumnCount() {
return ((bitField0_ & 0x00100000) == 0x00100000);
}
/**
* optional int32 baseColumnCount = 25;
*/
public int getBaseColumnCount() {
return baseColumnCount_;
}
// optional bool rowKeyOrderOptimizable = 26;
public static final int ROWKEYORDEROPTIMIZABLE_FIELD_NUMBER = 26;
private boolean rowKeyOrderOptimizable_;
/**
* optional bool rowKeyOrderOptimizable = 26;
*/
public boolean hasRowKeyOrderOptimizable() {
return ((bitField0_ & 0x00200000) == 0x00200000);
}
/**
* optional bool rowKeyOrderOptimizable = 26;
*/
public boolean getRowKeyOrderOptimizable() {
return rowKeyOrderOptimizable_;
}
// optional bool transactional = 27;
public static final int TRANSACTIONAL_FIELD_NUMBER = 27;
private boolean transactional_;
/**
* optional bool transactional = 27;
*/
public boolean hasTransactional() {
return ((bitField0_ & 0x00400000) == 0x00400000);
}
/**
* optional bool transactional = 27;
*/
public boolean getTransactional() {
return transactional_;
}
// optional int64 updateCacheFrequency = 28;
public static final int UPDATECACHEFREQUENCY_FIELD_NUMBER = 28;
private long updateCacheFrequency_;
/**
* optional int64 updateCacheFrequency = 28;
*/
public boolean hasUpdateCacheFrequency() {
return ((bitField0_ & 0x00800000) == 0x00800000);
}
/**
* optional int64 updateCacheFrequency = 28;
*/
public long getUpdateCacheFrequency() {
return updateCacheFrequency_;
}
// optional int64 indexDisableTimestamp = 29;
public static final int INDEXDISABLETIMESTAMP_FIELD_NUMBER = 29;
private long indexDisableTimestamp_;
/**
* optional int64 indexDisableTimestamp = 29;
*/
public boolean hasIndexDisableTimestamp() {
return ((bitField0_ & 0x01000000) == 0x01000000);
}
/**
* optional int64 indexDisableTimestamp = 29;
*/
public long getIndexDisableTimestamp() {
return indexDisableTimestamp_;
}
// optional bool isNamespaceMapped = 30;
public static final int ISNAMESPACEMAPPED_FIELD_NUMBER = 30;
private boolean isNamespaceMapped_;
/**
* optional bool isNamespaceMapped = 30;
*/
public boolean hasIsNamespaceMapped() {
return ((bitField0_ & 0x02000000) == 0x02000000);
}
/**
* optional bool isNamespaceMapped = 30;
*/
public boolean getIsNamespaceMapped() {
return isNamespaceMapped_;
}
// optional string autoParititonSeqName = 31;
public static final int AUTOPARITITONSEQNAME_FIELD_NUMBER = 31;
private java.lang.Object autoParititonSeqName_;
/**
* optional string autoParititonSeqName = 31;
*/
public boolean hasAutoParititonSeqName() {
return ((bitField0_ & 0x04000000) == 0x04000000);
}
/**
* optional string autoParititonSeqName = 31;
*/
public java.lang.String getAutoParititonSeqName() {
java.lang.Object ref = autoParititonSeqName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
autoParititonSeqName_ = s;
}
return s;
}
}
/**
* optional string autoParititonSeqName = 31;
*/
public com.google.protobuf.ByteString
getAutoParititonSeqNameBytes() {
java.lang.Object ref = autoParititonSeqName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
autoParititonSeqName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional bool isAppendOnlySchema = 32;
public static final int ISAPPENDONLYSCHEMA_FIELD_NUMBER = 32;
private boolean isAppendOnlySchema_;
/**
* optional bool isAppendOnlySchema = 32;
*/
public boolean hasIsAppendOnlySchema() {
return ((bitField0_ & 0x08000000) == 0x08000000);
}
/**
* optional bool isAppendOnlySchema = 32;
*/
public boolean getIsAppendOnlySchema() {
return isAppendOnlySchema_;
}
// optional bytes parentNameBytes = 33;
public static final int PARENTNAMEBYTES_FIELD_NUMBER = 33;
private com.google.protobuf.ByteString parentNameBytes_;
/**
* optional bytes parentNameBytes = 33;
*/
public boolean hasParentNameBytes() {
return ((bitField0_ & 0x10000000) == 0x10000000);
}
/**
* optional bytes parentNameBytes = 33;
*/
public com.google.protobuf.ByteString getParentNameBytes() {
return parentNameBytes_;
}
// optional bytes storageScheme = 34;
public static final int STORAGESCHEME_FIELD_NUMBER = 34;
private com.google.protobuf.ByteString storageScheme_;
/**
* optional bytes storageScheme = 34;
*/
public boolean hasStorageScheme() {
return ((bitField0_ & 0x20000000) == 0x20000000);
}
/**
* optional bytes storageScheme = 34;
*/
public com.google.protobuf.ByteString getStorageScheme() {
return storageScheme_;
}
// optional bytes encodingScheme = 35;
public static final int ENCODINGSCHEME_FIELD_NUMBER = 35;
private com.google.protobuf.ByteString encodingScheme_;
/**
* optional bytes encodingScheme = 35;
*/
public boolean hasEncodingScheme() {
return ((bitField0_ & 0x40000000) == 0x40000000);
}
/**
* optional bytes encodingScheme = 35;
*/
public com.google.protobuf.ByteString getEncodingScheme() {
return encodingScheme_;
}
// repeated .EncodedCQCounter encodedCQCounters = 36;
public static final int ENCODEDCQCOUNTERS_FIELD_NUMBER = 36;
private java.util.List encodedCQCounters_;
/**
* repeated .EncodedCQCounter encodedCQCounters = 36;
*/
public java.util.List getEncodedCQCountersList() {
return encodedCQCounters_;
}
/**
* repeated .EncodedCQCounter encodedCQCounters = 36;
*/
public java.util.List extends org.apache.phoenix.coprocessor.generated.PTableProtos.EncodedCQCounterOrBuilder>
getEncodedCQCountersOrBuilderList() {
return encodedCQCounters_;
}
/**
* repeated .EncodedCQCounter encodedCQCounters = 36;
*/
public int getEncodedCQCountersCount() {
return encodedCQCounters_.size();
}
/**
* repeated .EncodedCQCounter encodedCQCounters = 36;
*/
public org.apache.phoenix.coprocessor.generated.PTableProtos.EncodedCQCounter getEncodedCQCounters(int index) {
return encodedCQCounters_.get(index);
}
/**
* repeated .EncodedCQCounter encodedCQCounters = 36;
*/
public org.apache.phoenix.coprocessor.generated.PTableProtos.EncodedCQCounterOrBuilder getEncodedCQCountersOrBuilder(
int index) {
return encodedCQCounters_.get(index);
}
// optional bool useStatsForParallelization = 37;
public static final int USESTATSFORPARALLELIZATION_FIELD_NUMBER = 37;
private boolean useStatsForParallelization_;
/**
* optional bool useStatsForParallelization = 37;
*/
public boolean hasUseStatsForParallelization() {
return ((bitField0_ & 0x80000000) == 0x80000000);
}
/**
* optional bool useStatsForParallelization = 37;
*/
public boolean getUseStatsForParallelization() {
return useStatsForParallelization_;
}
// optional int32 transactionProvider = 38;
public static final int TRANSACTIONPROVIDER_FIELD_NUMBER = 38;
private int transactionProvider_;
/**
* optional int32 transactionProvider = 38;
*/
public boolean hasTransactionProvider() {
return ((bitField1_ & 0x00000001) == 0x00000001);
}
/**
* optional int32 transactionProvider = 38;
*/
public int getTransactionProvider() {
return transactionProvider_;
}
// optional int32 viewIndexIdType = 39 [default = 5];
public static final int VIEWINDEXIDTYPE_FIELD_NUMBER = 39;
private int viewIndexIdType_;
/**
* optional int32 viewIndexIdType = 39 [default = 5];
*/
public boolean hasViewIndexIdType() {
return ((bitField1_ & 0x00000002) == 0x00000002);
}
/**
* optional int32 viewIndexIdType = 39 [default = 5];
*/
public int getViewIndexIdType() {
return viewIndexIdType_;
}
// optional bool viewModifiedUpdateCacheFrequency = 40;
public static final int VIEWMODIFIEDUPDATECACHEFREQUENCY_FIELD_NUMBER = 40;
private boolean viewModifiedUpdateCacheFrequency_;
/**
* optional bool viewModifiedUpdateCacheFrequency = 40;
*/
public boolean hasViewModifiedUpdateCacheFrequency() {
return ((bitField1_ & 0x00000004) == 0x00000004);
}
/**
* optional bool viewModifiedUpdateCacheFrequency = 40;
*/
public boolean getViewModifiedUpdateCacheFrequency() {
return viewModifiedUpdateCacheFrequency_;
}
// optional bool viewModifiedUseStatsForParallelization = 41;
public static final int VIEWMODIFIEDUSESTATSFORPARALLELIZATION_FIELD_NUMBER = 41;
private boolean viewModifiedUseStatsForParallelization_;
/**
* optional bool viewModifiedUseStatsForParallelization = 41;
*/
public boolean hasViewModifiedUseStatsForParallelization() {
return ((bitField1_ & 0x00000008) == 0x00000008);
}
/**
* optional bool viewModifiedUseStatsForParallelization = 41;
*/
public boolean getViewModifiedUseStatsForParallelization() {
return viewModifiedUseStatsForParallelization_;
}
// optional int64 phoenixTTL = 42;
public static final int PHOENIXTTL_FIELD_NUMBER = 42;
private long phoenixTTL_;
/**
* optional int64 phoenixTTL = 42;
*/
public boolean hasPhoenixTTL() {
return ((bitField1_ & 0x00000010) == 0x00000010);
}
/**
* optional int64 phoenixTTL = 42;
*/
public long getPhoenixTTL() {
return phoenixTTL_;
}
// optional int64 phoenixTTLHighWaterMark = 43;
public static final int PHOENIXTTLHIGHWATERMARK_FIELD_NUMBER = 43;
private long phoenixTTLHighWaterMark_;
/**
* optional int64 phoenixTTLHighWaterMark = 43;
*/
public boolean hasPhoenixTTLHighWaterMark() {
return ((bitField1_ & 0x00000020) == 0x00000020);
}
/**
* optional int64 phoenixTTLHighWaterMark = 43;
*/
public long getPhoenixTTLHighWaterMark() {
return phoenixTTLHighWaterMark_;
}
// optional bool viewModifiedPhoenixTTL = 44;
public static final int VIEWMODIFIEDPHOENIXTTL_FIELD_NUMBER = 44;
private boolean viewModifiedPhoenixTTL_;
/**
* optional bool viewModifiedPhoenixTTL = 44;
*/
public boolean hasViewModifiedPhoenixTTL() {
return ((bitField1_ & 0x00000040) == 0x00000040);
}
/**
* optional bool viewModifiedPhoenixTTL = 44;
*/
public boolean getViewModifiedPhoenixTTL() {
return viewModifiedPhoenixTTL_;
}
// optional int64 lastDDLTimestamp = 45;
public static final int LASTDDLTIMESTAMP_FIELD_NUMBER = 45;
private long lastDDLTimestamp_;
/**
* optional int64 lastDDLTimestamp = 45;
*/
public boolean hasLastDDLTimestamp() {
return ((bitField1_ & 0x00000080) == 0x00000080);
}
/**
* optional int64 lastDDLTimestamp = 45;
*/
public long getLastDDLTimestamp() {
return lastDDLTimestamp_;
}
// optional bool changeDetectionEnabled = 46;
public static final int CHANGEDETECTIONENABLED_FIELD_NUMBER = 46;
private boolean changeDetectionEnabled_;
/**
* optional bool changeDetectionEnabled = 46;
*/
public boolean hasChangeDetectionEnabled() {
return ((bitField1_ & 0x00000100) == 0x00000100);
}
/**
* optional bool changeDetectionEnabled = 46;
*/
public boolean getChangeDetectionEnabled() {
return changeDetectionEnabled_;
}
private void initFields() {
schemaNameBytes_ = com.google.protobuf.ByteString.EMPTY;
tableNameBytes_ = com.google.protobuf.ByteString.EMPTY;
tableType_ = org.apache.phoenix.coprocessor.generated.PTableProtos.PTableType.SYSTEM;
indexState_ = "";
sequenceNumber_ = 0L;
timeStamp_ = 0L;
pkNameBytes_ = com.google.protobuf.ByteString.EMPTY;
bucketNum_ = 0;
columns_ = java.util.Collections.emptyList();
indexes_ = java.util.Collections.emptyList();
isImmutableRows_ = false;
dataTableNameBytes_ = com.google.protobuf.ByteString.EMPTY;
defaultFamilyName_ = com.google.protobuf.ByteString.EMPTY;
disableWAL_ = false;
multiTenant_ = false;
viewType_ = com.google.protobuf.ByteString.EMPTY;
viewStatement_ = com.google.protobuf.ByteString.EMPTY;
physicalNames_ = java.util.Collections.emptyList();
tenantId_ = com.google.protobuf.ByteString.EMPTY;
viewIndexId_ = 0L;
indexType_ = com.google.protobuf.ByteString.EMPTY;
statsTimeStamp_ = 0L;
storeNulls_ = false;
baseColumnCount_ = 0;
rowKeyOrderOptimizable_ = false;
transactional_ = false;
updateCacheFrequency_ = 0L;
indexDisableTimestamp_ = 0L;
isNamespaceMapped_ = false;
autoParititonSeqName_ = "";
isAppendOnlySchema_ = false;
parentNameBytes_ = com.google.protobuf.ByteString.EMPTY;
storageScheme_ = com.google.protobuf.ByteString.EMPTY;
encodingScheme_ = com.google.protobuf.ByteString.EMPTY;
encodedCQCounters_ = java.util.Collections.emptyList();
useStatsForParallelization_ = false;
transactionProvider_ = 0;
viewIndexIdType_ = 5;
viewModifiedUpdateCacheFrequency_ = false;
viewModifiedUseStatsForParallelization_ = false;
phoenixTTL_ = 0L;
phoenixTTLHighWaterMark_ = 0L;
viewModifiedPhoenixTTL_ = false;
lastDDLTimestamp_ = 0L;
changeDetectionEnabled_ = false;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (!hasSchemaNameBytes()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasTableNameBytes()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasTableType()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasSequenceNumber()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasTimeStamp()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasBucketNum()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasIsImmutableRows()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasDisableWAL()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasMultiTenant()) {
memoizedIsInitialized = 0;
return false;
}
for (int i = 0; i < getColumnsCount(); i++) {
if (!getColumns(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
for (int i = 0; i < getIndexesCount(); i++) {
if (!getIndexes(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
for (int i = 0; i < getEncodedCQCountersCount(); i++) {
if (!getEncodedCQCounters(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(1, schemaNameBytes_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeBytes(2, tableNameBytes_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeEnum(3, tableType_.getNumber());
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeBytes(4, getIndexStateBytes());
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
output.writeInt64(5, sequenceNumber_);
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
output.writeInt64(6, timeStamp_);
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
output.writeBytes(7, pkNameBytes_);
}
if (((bitField0_ & 0x00000080) == 0x00000080)) {
output.writeInt32(8, bucketNum_);
}
for (int i = 0; i < columns_.size(); i++) {
output.writeMessage(9, columns_.get(i));
}
for (int i = 0; i < indexes_.size(); i++) {
output.writeMessage(10, indexes_.get(i));
}
if (((bitField0_ & 0x00000100) == 0x00000100)) {
output.writeBool(11, isImmutableRows_);
}
if (((bitField0_ & 0x00000200) == 0x00000200)) {
output.writeBytes(13, dataTableNameBytes_);
}
if (((bitField0_ & 0x00000400) == 0x00000400)) {
output.writeBytes(14, defaultFamilyName_);
}
if (((bitField0_ & 0x00000800) == 0x00000800)) {
output.writeBool(15, disableWAL_);
}
if (((bitField0_ & 0x00001000) == 0x00001000)) {
output.writeBool(16, multiTenant_);
}
if (((bitField0_ & 0x00002000) == 0x00002000)) {
output.writeBytes(17, viewType_);
}
if (((bitField0_ & 0x00004000) == 0x00004000)) {
output.writeBytes(18, viewStatement_);
}
for (int i = 0; i < physicalNames_.size(); i++) {
output.writeBytes(19, physicalNames_.get(i));
}
if (((bitField0_ & 0x00008000) == 0x00008000)) {
output.writeBytes(20, tenantId_);
}
if (((bitField0_ & 0x00010000) == 0x00010000)) {
output.writeInt64(21, viewIndexId_);
}
if (((bitField0_ & 0x00020000) == 0x00020000)) {
output.writeBytes(22, indexType_);
}
if (((bitField0_ & 0x00040000) == 0x00040000)) {
output.writeInt64(23, statsTimeStamp_);
}
if (((bitField0_ & 0x00080000) == 0x00080000)) {
output.writeBool(24, storeNulls_);
}
if (((bitField0_ & 0x00100000) == 0x00100000)) {
output.writeInt32(25, baseColumnCount_);
}
if (((bitField0_ & 0x00200000) == 0x00200000)) {
output.writeBool(26, rowKeyOrderOptimizable_);
}
if (((bitField0_ & 0x00400000) == 0x00400000)) {
output.writeBool(27, transactional_);
}
if (((bitField0_ & 0x00800000) == 0x00800000)) {
output.writeInt64(28, updateCacheFrequency_);
}
if (((bitField0_ & 0x01000000) == 0x01000000)) {
output.writeInt64(29, indexDisableTimestamp_);
}
if (((bitField0_ & 0x02000000) == 0x02000000)) {
output.writeBool(30, isNamespaceMapped_);
}
if (((bitField0_ & 0x04000000) == 0x04000000)) {
output.writeBytes(31, getAutoParititonSeqNameBytes());
}
if (((bitField0_ & 0x08000000) == 0x08000000)) {
output.writeBool(32, isAppendOnlySchema_);
}
if (((bitField0_ & 0x10000000) == 0x10000000)) {
output.writeBytes(33, parentNameBytes_);
}
if (((bitField0_ & 0x20000000) == 0x20000000)) {
output.writeBytes(34, storageScheme_);
}
if (((bitField0_ & 0x40000000) == 0x40000000)) {
output.writeBytes(35, encodingScheme_);
}
for (int i = 0; i < encodedCQCounters_.size(); i++) {
output.writeMessage(36, encodedCQCounters_.get(i));
}
if (((bitField0_ & 0x80000000) == 0x80000000)) {
output.writeBool(37, useStatsForParallelization_);
}
if (((bitField1_ & 0x00000001) == 0x00000001)) {
output.writeInt32(38, transactionProvider_);
}
if (((bitField1_ & 0x00000002) == 0x00000002)) {
output.writeInt32(39, viewIndexIdType_);
}
if (((bitField1_ & 0x00000004) == 0x00000004)) {
output.writeBool(40, viewModifiedUpdateCacheFrequency_);
}
if (((bitField1_ & 0x00000008) == 0x00000008)) {
output.writeBool(41, viewModifiedUseStatsForParallelization_);
}
if (((bitField1_ & 0x00000010) == 0x00000010)) {
output.writeInt64(42, phoenixTTL_);
}
if (((bitField1_ & 0x00000020) == 0x00000020)) {
output.writeInt64(43, phoenixTTLHighWaterMark_);
}
if (((bitField1_ & 0x00000040) == 0x00000040)) {
output.writeBool(44, viewModifiedPhoenixTTL_);
}
if (((bitField1_ & 0x00000080) == 0x00000080)) {
output.writeInt64(45, lastDDLTimestamp_);
}
if (((bitField1_ & 0x00000100) == 0x00000100)) {
output.writeBool(46, changeDetectionEnabled_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, schemaNameBytes_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, tableNameBytes_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(3, tableType_.getNumber());
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(4, getIndexStateBytes());
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(5, sequenceNumber_);
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(6, timeStamp_);
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(7, pkNameBytes_);
}
if (((bitField0_ & 0x00000080) == 0x00000080)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(8, bucketNum_);
}
for (int i = 0; i < columns_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(9, columns_.get(i));
}
for (int i = 0; i < indexes_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(10, indexes_.get(i));
}
if (((bitField0_ & 0x00000100) == 0x00000100)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(11, isImmutableRows_);
}
if (((bitField0_ & 0x00000200) == 0x00000200)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(13, dataTableNameBytes_);
}
if (((bitField0_ & 0x00000400) == 0x00000400)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(14, defaultFamilyName_);
}
if (((bitField0_ & 0x00000800) == 0x00000800)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(15, disableWAL_);
}
if (((bitField0_ & 0x00001000) == 0x00001000)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(16, multiTenant_);
}
if (((bitField0_ & 0x00002000) == 0x00002000)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(17, viewType_);
}
if (((bitField0_ & 0x00004000) == 0x00004000)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(18, viewStatement_);
}
{
int dataSize = 0;
for (int i = 0; i < physicalNames_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeBytesSizeNoTag(physicalNames_.get(i));
}
size += dataSize;
size += 2 * getPhysicalNamesList().size();
}
if (((bitField0_ & 0x00008000) == 0x00008000)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(20, tenantId_);
}
if (((bitField0_ & 0x00010000) == 0x00010000)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(21, viewIndexId_);
}
if (((bitField0_ & 0x00020000) == 0x00020000)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(22, indexType_);
}
if (((bitField0_ & 0x00040000) == 0x00040000)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(23, statsTimeStamp_);
}
if (((bitField0_ & 0x00080000) == 0x00080000)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(24, storeNulls_);
}
if (((bitField0_ & 0x00100000) == 0x00100000)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(25, baseColumnCount_);
}
if (((bitField0_ & 0x00200000) == 0x00200000)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(26, rowKeyOrderOptimizable_);
}
if (((bitField0_ & 0x00400000) == 0x00400000)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(27, transactional_);
}
if (((bitField0_ & 0x00800000) == 0x00800000)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(28, updateCacheFrequency_);
}
if (((bitField0_ & 0x01000000) == 0x01000000)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(29, indexDisableTimestamp_);
}
if (((bitField0_ & 0x02000000) == 0x02000000)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(30, isNamespaceMapped_);
}
if (((bitField0_ & 0x04000000) == 0x04000000)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(31, getAutoParititonSeqNameBytes());
}
if (((bitField0_ & 0x08000000) == 0x08000000)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(32, isAppendOnlySchema_);
}
if (((bitField0_ & 0x10000000) == 0x10000000)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(33, parentNameBytes_);
}
if (((bitField0_ & 0x20000000) == 0x20000000)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(34, storageScheme_);
}
if (((bitField0_ & 0x40000000) == 0x40000000)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(35, encodingScheme_);
}
for (int i = 0; i < encodedCQCounters_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(36, encodedCQCounters_.get(i));
}
if (((bitField0_ & 0x80000000) == 0x80000000)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(37, useStatsForParallelization_);
}
if (((bitField1_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(38, transactionProvider_);
}
if (((bitField1_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(39, viewIndexIdType_);
}
if (((bitField1_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(40, viewModifiedUpdateCacheFrequency_);
}
if (((bitField1_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(41, viewModifiedUseStatsForParallelization_);
}
if (((bitField1_ & 0x00000010) == 0x00000010)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(42, phoenixTTL_);
}
if (((bitField1_ & 0x00000020) == 0x00000020)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(43, phoenixTTLHighWaterMark_);
}
if (((bitField1_ & 0x00000040) == 0x00000040)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(44, viewModifiedPhoenixTTL_);
}
if (((bitField1_ & 0x00000080) == 0x00000080)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(45, lastDDLTimestamp_);
}
if (((bitField1_ & 0x00000100) == 0x00000100)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(46, changeDetectionEnabled_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.phoenix.coprocessor.generated.PTableProtos.PTable)) {
return super.equals(obj);
}
org.apache.phoenix.coprocessor.generated.PTableProtos.PTable other = (org.apache.phoenix.coprocessor.generated.PTableProtos.PTable) obj;
boolean result = true;
result = result && (hasSchemaNameBytes() == other.hasSchemaNameBytes());
if (hasSchemaNameBytes()) {
result = result && getSchemaNameBytes()
.equals(other.getSchemaNameBytes());
}
result = result && (hasTableNameBytes() == other.hasTableNameBytes());
if (hasTableNameBytes()) {
result = result && getTableNameBytes()
.equals(other.getTableNameBytes());
}
result = result && (hasTableType() == other.hasTableType());
if (hasTableType()) {
result = result &&
(getTableType() == other.getTableType());
}
result = result && (hasIndexState() == other.hasIndexState());
if (hasIndexState()) {
result = result && getIndexState()
.equals(other.getIndexState());
}
result = result && (hasSequenceNumber() == other.hasSequenceNumber());
if (hasSequenceNumber()) {
result = result && (getSequenceNumber()
== other.getSequenceNumber());
}
result = result && (hasTimeStamp() == other.hasTimeStamp());
if (hasTimeStamp()) {
result = result && (getTimeStamp()
== other.getTimeStamp());
}
result = result && (hasPkNameBytes() == other.hasPkNameBytes());
if (hasPkNameBytes()) {
result = result && getPkNameBytes()
.equals(other.getPkNameBytes());
}
result = result && (hasBucketNum() == other.hasBucketNum());
if (hasBucketNum()) {
result = result && (getBucketNum()
== other.getBucketNum());
}
result = result && getColumnsList()
.equals(other.getColumnsList());
result = result && getIndexesList()
.equals(other.getIndexesList());
result = result && (hasIsImmutableRows() == other.hasIsImmutableRows());
if (hasIsImmutableRows()) {
result = result && (getIsImmutableRows()
== other.getIsImmutableRows());
}
result = result && (hasDataTableNameBytes() == other.hasDataTableNameBytes());
if (hasDataTableNameBytes()) {
result = result && getDataTableNameBytes()
.equals(other.getDataTableNameBytes());
}
result = result && (hasDefaultFamilyName() == other.hasDefaultFamilyName());
if (hasDefaultFamilyName()) {
result = result && getDefaultFamilyName()
.equals(other.getDefaultFamilyName());
}
result = result && (hasDisableWAL() == other.hasDisableWAL());
if (hasDisableWAL()) {
result = result && (getDisableWAL()
== other.getDisableWAL());
}
result = result && (hasMultiTenant() == other.hasMultiTenant());
if (hasMultiTenant()) {
result = result && (getMultiTenant()
== other.getMultiTenant());
}
result = result && (hasViewType() == other.hasViewType());
if (hasViewType()) {
result = result && getViewType()
.equals(other.getViewType());
}
result = result && (hasViewStatement() == other.hasViewStatement());
if (hasViewStatement()) {
result = result && getViewStatement()
.equals(other.getViewStatement());
}
result = result && getPhysicalNamesList()
.equals(other.getPhysicalNamesList());
result = result && (hasTenantId() == other.hasTenantId());
if (hasTenantId()) {
result = result && getTenantId()
.equals(other.getTenantId());
}
result = result && (hasViewIndexId() == other.hasViewIndexId());
if (hasViewIndexId()) {
result = result && (getViewIndexId()
== other.getViewIndexId());
}
result = result && (hasIndexType() == other.hasIndexType());
if (hasIndexType()) {
result = result && getIndexType()
.equals(other.getIndexType());
}
result = result && (hasStatsTimeStamp() == other.hasStatsTimeStamp());
if (hasStatsTimeStamp()) {
result = result && (getStatsTimeStamp()
== other.getStatsTimeStamp());
}
result = result && (hasStoreNulls() == other.hasStoreNulls());
if (hasStoreNulls()) {
result = result && (getStoreNulls()
== other.getStoreNulls());
}
result = result && (hasBaseColumnCount() == other.hasBaseColumnCount());
if (hasBaseColumnCount()) {
result = result && (getBaseColumnCount()
== other.getBaseColumnCount());
}
result = result && (hasRowKeyOrderOptimizable() == other.hasRowKeyOrderOptimizable());
if (hasRowKeyOrderOptimizable()) {
result = result && (getRowKeyOrderOptimizable()
== other.getRowKeyOrderOptimizable());
}
result = result && (hasTransactional() == other.hasTransactional());
if (hasTransactional()) {
result = result && (getTransactional()
== other.getTransactional());
}
result = result && (hasUpdateCacheFrequency() == other.hasUpdateCacheFrequency());
if (hasUpdateCacheFrequency()) {
result = result && (getUpdateCacheFrequency()
== other.getUpdateCacheFrequency());
}
result = result && (hasIndexDisableTimestamp() == other.hasIndexDisableTimestamp());
if (hasIndexDisableTimestamp()) {
result = result && (getIndexDisableTimestamp()
== other.getIndexDisableTimestamp());
}
result = result && (hasIsNamespaceMapped() == other.hasIsNamespaceMapped());
if (hasIsNamespaceMapped()) {
result = result && (getIsNamespaceMapped()
== other.getIsNamespaceMapped());
}
result = result && (hasAutoParititonSeqName() == other.hasAutoParititonSeqName());
if (hasAutoParititonSeqName()) {
result = result && getAutoParititonSeqName()
.equals(other.getAutoParititonSeqName());
}
result = result && (hasIsAppendOnlySchema() == other.hasIsAppendOnlySchema());
if (hasIsAppendOnlySchema()) {
result = result && (getIsAppendOnlySchema()
== other.getIsAppendOnlySchema());
}
result = result && (hasParentNameBytes() == other.hasParentNameBytes());
if (hasParentNameBytes()) {
result = result && getParentNameBytes()
.equals(other.getParentNameBytes());
}
result = result && (hasStorageScheme() == other.hasStorageScheme());
if (hasStorageScheme()) {
result = result && getStorageScheme()
.equals(other.getStorageScheme());
}
result = result && (hasEncodingScheme() == other.hasEncodingScheme());
if (hasEncodingScheme()) {
result = result && getEncodingScheme()
.equals(other.getEncodingScheme());
}
result = result && getEncodedCQCountersList()
.equals(other.getEncodedCQCountersList());
result = result && (hasUseStatsForParallelization() == other.hasUseStatsForParallelization());
if (hasUseStatsForParallelization()) {
result = result && (getUseStatsForParallelization()
== other.getUseStatsForParallelization());
}
result = result && (hasTransactionProvider() == other.hasTransactionProvider());
if (hasTransactionProvider()) {
result = result && (getTransactionProvider()
== other.getTransactionProvider());
}
result = result && (hasViewIndexIdType() == other.hasViewIndexIdType());
if (hasViewIndexIdType()) {
result = result && (getViewIndexIdType()
== other.getViewIndexIdType());
}
result = result && (hasViewModifiedUpdateCacheFrequency() == other.hasViewModifiedUpdateCacheFrequency());
if (hasViewModifiedUpdateCacheFrequency()) {
result = result && (getViewModifiedUpdateCacheFrequency()
== other.getViewModifiedUpdateCacheFrequency());
}
result = result && (hasViewModifiedUseStatsForParallelization() == other.hasViewModifiedUseStatsForParallelization());
if (hasViewModifiedUseStatsForParallelization()) {
result = result && (getViewModifiedUseStatsForParallelization()
== other.getViewModifiedUseStatsForParallelization());
}
result = result && (hasPhoenixTTL() == other.hasPhoenixTTL());
if (hasPhoenixTTL()) {
result = result && (getPhoenixTTL()
== other.getPhoenixTTL());
}
result = result && (hasPhoenixTTLHighWaterMark() == other.hasPhoenixTTLHighWaterMark());
if (hasPhoenixTTLHighWaterMark()) {
result = result && (getPhoenixTTLHighWaterMark()
== other.getPhoenixTTLHighWaterMark());
}
result = result && (hasViewModifiedPhoenixTTL() == other.hasViewModifiedPhoenixTTL());
if (hasViewModifiedPhoenixTTL()) {
result = result && (getViewModifiedPhoenixTTL()
== other.getViewModifiedPhoenixTTL());
}
result = result && (hasLastDDLTimestamp() == other.hasLastDDLTimestamp());
if (hasLastDDLTimestamp()) {
result = result && (getLastDDLTimestamp()
== other.getLastDDLTimestamp());
}
result = result && (hasChangeDetectionEnabled() == other.hasChangeDetectionEnabled());
if (hasChangeDetectionEnabled()) {
result = result && (getChangeDetectionEnabled()
== other.getChangeDetectionEnabled());
}
result = result &&
getUnknownFields().equals(other.getUnknownFields());
return result;
}
private int memoizedHashCode = 0;
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptorForType().hashCode();
if (hasSchemaNameBytes()) {
hash = (37 * hash) + SCHEMANAMEBYTES_FIELD_NUMBER;
hash = (53 * hash) + getSchemaNameBytes().hashCode();
}
if (hasTableNameBytes()) {
hash = (37 * hash) + TABLENAMEBYTES_FIELD_NUMBER;
hash = (53 * hash) + getTableNameBytes().hashCode();
}
if (hasTableType()) {
hash = (37 * hash) + TABLETYPE_FIELD_NUMBER;
hash = (53 * hash) + hashEnum(getTableType());
}
if (hasIndexState()) {
hash = (37 * hash) + INDEXSTATE_FIELD_NUMBER;
hash = (53 * hash) + getIndexState().hashCode();
}
if (hasSequenceNumber()) {
hash = (37 * hash) + SEQUENCENUMBER_FIELD_NUMBER;
hash = (53 * hash) + hashLong(getSequenceNumber());
}
if (hasTimeStamp()) {
hash = (37 * hash) + TIMESTAMP_FIELD_NUMBER;
hash = (53 * hash) + hashLong(getTimeStamp());
}
if (hasPkNameBytes()) {
hash = (37 * hash) + PKNAMEBYTES_FIELD_NUMBER;
hash = (53 * hash) + getPkNameBytes().hashCode();
}
if (hasBucketNum()) {
hash = (37 * hash) + BUCKETNUM_FIELD_NUMBER;
hash = (53 * hash) + getBucketNum();
}
if (getColumnsCount() > 0) {
hash = (37 * hash) + COLUMNS_FIELD_NUMBER;
hash = (53 * hash) + getColumnsList().hashCode();
}
if (getIndexesCount() > 0) {
hash = (37 * hash) + INDEXES_FIELD_NUMBER;
hash = (53 * hash) + getIndexesList().hashCode();
}
if (hasIsImmutableRows()) {
hash = (37 * hash) + ISIMMUTABLEROWS_FIELD_NUMBER;
hash = (53 * hash) + hashBoolean(getIsImmutableRows());
}
if (hasDataTableNameBytes()) {
hash = (37 * hash) + DATATABLENAMEBYTES_FIELD_NUMBER;
hash = (53 * hash) + getDataTableNameBytes().hashCode();
}
if (hasDefaultFamilyName()) {
hash = (37 * hash) + DEFAULTFAMILYNAME_FIELD_NUMBER;
hash = (53 * hash) + getDefaultFamilyName().hashCode();
}
if (hasDisableWAL()) {
hash = (37 * hash) + DISABLEWAL_FIELD_NUMBER;
hash = (53 * hash) + hashBoolean(getDisableWAL());
}
if (hasMultiTenant()) {
hash = (37 * hash) + MULTITENANT_FIELD_NUMBER;
hash = (53 * hash) + hashBoolean(getMultiTenant());
}
if (hasViewType()) {
hash = (37 * hash) + VIEWTYPE_FIELD_NUMBER;
hash = (53 * hash) + getViewType().hashCode();
}
if (hasViewStatement()) {
hash = (37 * hash) + VIEWSTATEMENT_FIELD_NUMBER;
hash = (53 * hash) + getViewStatement().hashCode();
}
if (getPhysicalNamesCount() > 0) {
hash = (37 * hash) + PHYSICALNAMES_FIELD_NUMBER;
hash = (53 * hash) + getPhysicalNamesList().hashCode();
}
if (hasTenantId()) {
hash = (37 * hash) + TENANTID_FIELD_NUMBER;
hash = (53 * hash) + getTenantId().hashCode();
}
if (hasViewIndexId()) {
hash = (37 * hash) + VIEWINDEXID_FIELD_NUMBER;
hash = (53 * hash) + hashLong(getViewIndexId());
}
if (hasIndexType()) {
hash = (37 * hash) + INDEXTYPE_FIELD_NUMBER;
hash = (53 * hash) + getIndexType().hashCode();
}
if (hasStatsTimeStamp()) {
hash = (37 * hash) + STATSTIMESTAMP_FIELD_NUMBER;
hash = (53 * hash) + hashLong(getStatsTimeStamp());
}
if (hasStoreNulls()) {
hash = (37 * hash) + STORENULLS_FIELD_NUMBER;
hash = (53 * hash) + hashBoolean(getStoreNulls());
}
if (hasBaseColumnCount()) {
hash = (37 * hash) + BASECOLUMNCOUNT_FIELD_NUMBER;
hash = (53 * hash) + getBaseColumnCount();
}
if (hasRowKeyOrderOptimizable()) {
hash = (37 * hash) + ROWKEYORDEROPTIMIZABLE_FIELD_NUMBER;
hash = (53 * hash) + hashBoolean(getRowKeyOrderOptimizable());
}
if (hasTransactional()) {
hash = (37 * hash) + TRANSACTIONAL_FIELD_NUMBER;
hash = (53 * hash) + hashBoolean(getTransactional());
}
if (hasUpdateCacheFrequency()) {
hash = (37 * hash) + UPDATECACHEFREQUENCY_FIELD_NUMBER;
hash = (53 * hash) + hashLong(getUpdateCacheFrequency());
}
if (hasIndexDisableTimestamp()) {
hash = (37 * hash) + INDEXDISABLETIMESTAMP_FIELD_NUMBER;
hash = (53 * hash) + hashLong(getIndexDisableTimestamp());
}
if (hasIsNamespaceMapped()) {
hash = (37 * hash) + ISNAMESPACEMAPPED_FIELD_NUMBER;
hash = (53 * hash) + hashBoolean(getIsNamespaceMapped());
}
if (hasAutoParititonSeqName()) {
hash = (37 * hash) + AUTOPARITITONSEQNAME_FIELD_NUMBER;
hash = (53 * hash) + getAutoParititonSeqName().hashCode();
}
if (hasIsAppendOnlySchema()) {
hash = (37 * hash) + ISAPPENDONLYSCHEMA_FIELD_NUMBER;
hash = (53 * hash) + hashBoolean(getIsAppendOnlySchema());
}
if (hasParentNameBytes()) {
hash = (37 * hash) + PARENTNAMEBYTES_FIELD_NUMBER;
hash = (53 * hash) + getParentNameBytes().hashCode();
}
if (hasStorageScheme()) {
hash = (37 * hash) + STORAGESCHEME_FIELD_NUMBER;
hash = (53 * hash) + getStorageScheme().hashCode();
}
if (hasEncodingScheme()) {
hash = (37 * hash) + ENCODINGSCHEME_FIELD_NUMBER;
hash = (53 * hash) + getEncodingScheme().hashCode();
}
if (getEncodedCQCountersCount() > 0) {
hash = (37 * hash) + ENCODEDCQCOUNTERS_FIELD_NUMBER;
hash = (53 * hash) + getEncodedCQCountersList().hashCode();
}
if (hasUseStatsForParallelization()) {
hash = (37 * hash) + USESTATSFORPARALLELIZATION_FIELD_NUMBER;
hash = (53 * hash) + hashBoolean(getUseStatsForParallelization());
}
if (hasTransactionProvider()) {
hash = (37 * hash) + TRANSACTIONPROVIDER_FIELD_NUMBER;
hash = (53 * hash) + getTransactionProvider();
}
if (hasViewIndexIdType()) {
hash = (37 * hash) + VIEWINDEXIDTYPE_FIELD_NUMBER;
hash = (53 * hash) + getViewIndexIdType();
}
if (hasViewModifiedUpdateCacheFrequency()) {
hash = (37 * hash) + VIEWMODIFIEDUPDATECACHEFREQUENCY_FIELD_NUMBER;
hash = (53 * hash) + hashBoolean(getViewModifiedUpdateCacheFrequency());
}
if (hasViewModifiedUseStatsForParallelization()) {
hash = (37 * hash) + VIEWMODIFIEDUSESTATSFORPARALLELIZATION_FIELD_NUMBER;
hash = (53 * hash) + hashBoolean(getViewModifiedUseStatsForParallelization());
}
if (hasPhoenixTTL()) {
hash = (37 * hash) + PHOENIXTTL_FIELD_NUMBER;
hash = (53 * hash) + hashLong(getPhoenixTTL());
}
if (hasPhoenixTTLHighWaterMark()) {
hash = (37 * hash) + PHOENIXTTLHIGHWATERMARK_FIELD_NUMBER;
hash = (53 * hash) + hashLong(getPhoenixTTLHighWaterMark());
}
if (hasViewModifiedPhoenixTTL()) {
hash = (37 * hash) + VIEWMODIFIEDPHOENIXTTL_FIELD_NUMBER;
hash = (53 * hash) + hashBoolean(getViewModifiedPhoenixTTL());
}
if (hasLastDDLTimestamp()) {
hash = (37 * hash) + LASTDDLTIMESTAMP_FIELD_NUMBER;
hash = (53 * hash) + hashLong(getLastDDLTimestamp());
}
if (hasChangeDetectionEnabled()) {
hash = (37 * hash) + CHANGEDETECTIONENABLED_FIELD_NUMBER;
hash = (53 * hash) + hashBoolean(getChangeDetectionEnabled());
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.phoenix.coprocessor.generated.PTableProtos.PTable parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.phoenix.coprocessor.generated.PTableProtos.PTable parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.phoenix.coprocessor.generated.PTableProtos.PTable parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.phoenix.coprocessor.generated.PTableProtos.PTable parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.phoenix.coprocessor.generated.PTableProtos.PTable parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.apache.phoenix.coprocessor.generated.PTableProtos.PTable parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.apache.phoenix.coprocessor.generated.PTableProtos.PTable parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.apache.phoenix.coprocessor.generated.PTableProtos.PTable parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.apache.phoenix.coprocessor.generated.PTableProtos.PTable parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.apache.phoenix.coprocessor.generated.PTableProtos.PTable parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(org.apache.phoenix.coprocessor.generated.PTableProtos.PTable prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code PTable}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements org.apache.phoenix.coprocessor.generated.PTableProtos.PTableOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.phoenix.coprocessor.generated.PTableProtos.internal_static_PTable_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.phoenix.coprocessor.generated.PTableProtos.internal_static_PTable_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.phoenix.coprocessor.generated.PTableProtos.PTable.class, org.apache.phoenix.coprocessor.generated.PTableProtos.PTable.Builder.class);
}
// Construct using org.apache.phoenix.coprocessor.generated.PTableProtos.PTable.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getColumnsFieldBuilder();
getIndexesFieldBuilder();
getEncodedCQCountersFieldBuilder();
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
schemaNameBytes_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
tableNameBytes_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000002);
tableType_ = org.apache.phoenix.coprocessor.generated.PTableProtos.PTableType.SYSTEM;
bitField0_ = (bitField0_ & ~0x00000004);
indexState_ = "";
bitField0_ = (bitField0_ & ~0x00000008);
sequenceNumber_ = 0L;
bitField0_ = (bitField0_ & ~0x00000010);
timeStamp_ = 0L;
bitField0_ = (bitField0_ & ~0x00000020);
pkNameBytes_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000040);
bucketNum_ = 0;
bitField0_ = (bitField0_ & ~0x00000080);
if (columnsBuilder_ == null) {
columns_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000100);
} else {
columnsBuilder_.clear();
}
if (indexesBuilder_ == null) {
indexes_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000200);
} else {
indexesBuilder_.clear();
}
isImmutableRows_ = false;
bitField0_ = (bitField0_ & ~0x00000400);
dataTableNameBytes_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000800);
defaultFamilyName_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00001000);
disableWAL_ = false;
bitField0_ = (bitField0_ & ~0x00002000);
multiTenant_ = false;
bitField0_ = (bitField0_ & ~0x00004000);
viewType_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00008000);
viewStatement_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00010000);
physicalNames_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00020000);
tenantId_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00040000);
viewIndexId_ = 0L;
bitField0_ = (bitField0_ & ~0x00080000);
indexType_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00100000);
statsTimeStamp_ = 0L;
bitField0_ = (bitField0_ & ~0x00200000);
storeNulls_ = false;
bitField0_ = (bitField0_ & ~0x00400000);
baseColumnCount_ = 0;
bitField0_ = (bitField0_ & ~0x00800000);
rowKeyOrderOptimizable_ = false;
bitField0_ = (bitField0_ & ~0x01000000);
transactional_ = false;
bitField0_ = (bitField0_ & ~0x02000000);
updateCacheFrequency_ = 0L;
bitField0_ = (bitField0_ & ~0x04000000);
indexDisableTimestamp_ = 0L;
bitField0_ = (bitField0_ & ~0x08000000);
isNamespaceMapped_ = false;
bitField0_ = (bitField0_ & ~0x10000000);
autoParititonSeqName_ = "";
bitField0_ = (bitField0_ & ~0x20000000);
isAppendOnlySchema_ = false;
bitField0_ = (bitField0_ & ~0x40000000);
parentNameBytes_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x80000000);
storageScheme_ = com.google.protobuf.ByteString.EMPTY;
bitField1_ = (bitField1_ & ~0x00000001);
encodingScheme_ = com.google.protobuf.ByteString.EMPTY;
bitField1_ = (bitField1_ & ~0x00000002);
if (encodedCQCountersBuilder_ == null) {
encodedCQCounters_ = java.util.Collections.emptyList();
bitField1_ = (bitField1_ & ~0x00000004);
} else {
encodedCQCountersBuilder_.clear();
}
useStatsForParallelization_ = false;
bitField1_ = (bitField1_ & ~0x00000008);
transactionProvider_ = 0;
bitField1_ = (bitField1_ & ~0x00000010);
viewIndexIdType_ = 5;
bitField1_ = (bitField1_ & ~0x00000020);
viewModifiedUpdateCacheFrequency_ = false;
bitField1_ = (bitField1_ & ~0x00000040);
viewModifiedUseStatsForParallelization_ = false;
bitField1_ = (bitField1_ & ~0x00000080);
phoenixTTL_ = 0L;
bitField1_ = (bitField1_ & ~0x00000100);
phoenixTTLHighWaterMark_ = 0L;
bitField1_ = (bitField1_ & ~0x00000200);
viewModifiedPhoenixTTL_ = false;
bitField1_ = (bitField1_ & ~0x00000400);
lastDDLTimestamp_ = 0L;
bitField1_ = (bitField1_ & ~0x00000800);
changeDetectionEnabled_ = false;
bitField1_ = (bitField1_ & ~0x00001000);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.phoenix.coprocessor.generated.PTableProtos.internal_static_PTable_descriptor;
}
public org.apache.phoenix.coprocessor.generated.PTableProtos.PTable getDefaultInstanceForType() {
return org.apache.phoenix.coprocessor.generated.PTableProtos.PTable.getDefaultInstance();
}
public org.apache.phoenix.coprocessor.generated.PTableProtos.PTable build() {
org.apache.phoenix.coprocessor.generated.PTableProtos.PTable result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.apache.phoenix.coprocessor.generated.PTableProtos.PTable buildPartial() {
org.apache.phoenix.coprocessor.generated.PTableProtos.PTable result = new org.apache.phoenix.coprocessor.generated.PTableProtos.PTable(this);
int from_bitField0_ = bitField0_;
int from_bitField1_ = bitField1_;
int to_bitField0_ = 0;
int to_bitField1_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.schemaNameBytes_ = schemaNameBytes_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.tableNameBytes_ = tableNameBytes_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.tableType_ = tableType_;
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000008;
}
result.indexState_ = indexState_;
if (((from_bitField0_ & 0x00000010) == 0x00000010)) {
to_bitField0_ |= 0x00000010;
}
result.sequenceNumber_ = sequenceNumber_;
if (((from_bitField0_ & 0x00000020) == 0x00000020)) {
to_bitField0_ |= 0x00000020;
}
result.timeStamp_ = timeStamp_;
if (((from_bitField0_ & 0x00000040) == 0x00000040)) {
to_bitField0_ |= 0x00000040;
}
result.pkNameBytes_ = pkNameBytes_;
if (((from_bitField0_ & 0x00000080) == 0x00000080)) {
to_bitField0_ |= 0x00000080;
}
result.bucketNum_ = bucketNum_;
if (columnsBuilder_ == null) {
if (((bitField0_ & 0x00000100) == 0x00000100)) {
columns_ = java.util.Collections.unmodifiableList(columns_);
bitField0_ = (bitField0_ & ~0x00000100);
}
result.columns_ = columns_;
} else {
result.columns_ = columnsBuilder_.build();
}
if (indexesBuilder_ == null) {
if (((bitField0_ & 0x00000200) == 0x00000200)) {
indexes_ = java.util.Collections.unmodifiableList(indexes_);
bitField0_ = (bitField0_ & ~0x00000200);
}
result.indexes_ = indexes_;
} else {
result.indexes_ = indexesBuilder_.build();
}
if (((from_bitField0_ & 0x00000400) == 0x00000400)) {
to_bitField0_ |= 0x00000100;
}
result.isImmutableRows_ = isImmutableRows_;
if (((from_bitField0_ & 0x00000800) == 0x00000800)) {
to_bitField0_ |= 0x00000200;
}
result.dataTableNameBytes_ = dataTableNameBytes_;
if (((from_bitField0_ & 0x00001000) == 0x00001000)) {
to_bitField0_ |= 0x00000400;
}
result.defaultFamilyName_ = defaultFamilyName_;
if (((from_bitField0_ & 0x00002000) == 0x00002000)) {
to_bitField0_ |= 0x00000800;
}
result.disableWAL_ = disableWAL_;
if (((from_bitField0_ & 0x00004000) == 0x00004000)) {
to_bitField0_ |= 0x00001000;
}
result.multiTenant_ = multiTenant_;
if (((from_bitField0_ & 0x00008000) == 0x00008000)) {
to_bitField0_ |= 0x00002000;
}
result.viewType_ = viewType_;
if (((from_bitField0_ & 0x00010000) == 0x00010000)) {
to_bitField0_ |= 0x00004000;
}
result.viewStatement_ = viewStatement_;
if (((bitField0_ & 0x00020000) == 0x00020000)) {
physicalNames_ = java.util.Collections.unmodifiableList(physicalNames_);
bitField0_ = (bitField0_ & ~0x00020000);
}
result.physicalNames_ = physicalNames_;
if (((from_bitField0_ & 0x00040000) == 0x00040000)) {
to_bitField0_ |= 0x00008000;
}
result.tenantId_ = tenantId_;
if (((from_bitField0_ & 0x00080000) == 0x00080000)) {
to_bitField0_ |= 0x00010000;
}
result.viewIndexId_ = viewIndexId_;
if (((from_bitField0_ & 0x00100000) == 0x00100000)) {
to_bitField0_ |= 0x00020000;
}
result.indexType_ = indexType_;
if (((from_bitField0_ & 0x00200000) == 0x00200000)) {
to_bitField0_ |= 0x00040000;
}
result.statsTimeStamp_ = statsTimeStamp_;
if (((from_bitField0_ & 0x00400000) == 0x00400000)) {
to_bitField0_ |= 0x00080000;
}
result.storeNulls_ = storeNulls_;
if (((from_bitField0_ & 0x00800000) == 0x00800000)) {
to_bitField0_ |= 0x00100000;
}
result.baseColumnCount_ = baseColumnCount_;
if (((from_bitField0_ & 0x01000000) == 0x01000000)) {
to_bitField0_ |= 0x00200000;
}
result.rowKeyOrderOptimizable_ = rowKeyOrderOptimizable_;
if (((from_bitField0_ & 0x02000000) == 0x02000000)) {
to_bitField0_ |= 0x00400000;
}
result.transactional_ = transactional_;
if (((from_bitField0_ & 0x04000000) == 0x04000000)) {
to_bitField0_ |= 0x00800000;
}
result.updateCacheFrequency_ = updateCacheFrequency_;
if (((from_bitField0_ & 0x08000000) == 0x08000000)) {
to_bitField0_ |= 0x01000000;
}
result.indexDisableTimestamp_ = indexDisableTimestamp_;
if (((from_bitField0_ & 0x10000000) == 0x10000000)) {
to_bitField0_ |= 0x02000000;
}
result.isNamespaceMapped_ = isNamespaceMapped_;
if (((from_bitField0_ & 0x20000000) == 0x20000000)) {
to_bitField0_ |= 0x04000000;
}
result.autoParititonSeqName_ = autoParititonSeqName_;
if (((from_bitField0_ & 0x40000000) == 0x40000000)) {
to_bitField0_ |= 0x08000000;
}
result.isAppendOnlySchema_ = isAppendOnlySchema_;
if (((from_bitField0_ & 0x80000000) == 0x80000000)) {
to_bitField0_ |= 0x10000000;
}
result.parentNameBytes_ = parentNameBytes_;
if (((from_bitField1_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x20000000;
}
result.storageScheme_ = storageScheme_;
if (((from_bitField1_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x40000000;
}
result.encodingScheme_ = encodingScheme_;
if (encodedCQCountersBuilder_ == null) {
if (((bitField1_ & 0x00000004) == 0x00000004)) {
encodedCQCounters_ = java.util.Collections.unmodifiableList(encodedCQCounters_);
bitField1_ = (bitField1_ & ~0x00000004);
}
result.encodedCQCounters_ = encodedCQCounters_;
} else {
result.encodedCQCounters_ = encodedCQCountersBuilder_.build();
}
if (((from_bitField1_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x80000000;
}
result.useStatsForParallelization_ = useStatsForParallelization_;
if (((from_bitField1_ & 0x00000010) == 0x00000010)) {
to_bitField1_ |= 0x00000001;
}
result.transactionProvider_ = transactionProvider_;
if (((from_bitField1_ & 0x00000020) == 0x00000020)) {
to_bitField1_ |= 0x00000002;
}
result.viewIndexIdType_ = viewIndexIdType_;
if (((from_bitField1_ & 0x00000040) == 0x00000040)) {
to_bitField1_ |= 0x00000004;
}
result.viewModifiedUpdateCacheFrequency_ = viewModifiedUpdateCacheFrequency_;
if (((from_bitField1_ & 0x00000080) == 0x00000080)) {
to_bitField1_ |= 0x00000008;
}
result.viewModifiedUseStatsForParallelization_ = viewModifiedUseStatsForParallelization_;
if (((from_bitField1_ & 0x00000100) == 0x00000100)) {
to_bitField1_ |= 0x00000010;
}
result.phoenixTTL_ = phoenixTTL_;
if (((from_bitField1_ & 0x00000200) == 0x00000200)) {
to_bitField1_ |= 0x00000020;
}
result.phoenixTTLHighWaterMark_ = phoenixTTLHighWaterMark_;
if (((from_bitField1_ & 0x00000400) == 0x00000400)) {
to_bitField1_ |= 0x00000040;
}
result.viewModifiedPhoenixTTL_ = viewModifiedPhoenixTTL_;
if (((from_bitField1_ & 0x00000800) == 0x00000800)) {
to_bitField1_ |= 0x00000080;
}
result.lastDDLTimestamp_ = lastDDLTimestamp_;
if (((from_bitField1_ & 0x00001000) == 0x00001000)) {
to_bitField1_ |= 0x00000100;
}
result.changeDetectionEnabled_ = changeDetectionEnabled_;
result.bitField0_ = to_bitField0_;
result.bitField1_ = to_bitField1_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.apache.phoenix.coprocessor.generated.PTableProtos.PTable) {
return mergeFrom((org.apache.phoenix.coprocessor.generated.PTableProtos.PTable)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.phoenix.coprocessor.generated.PTableProtos.PTable other) {
if (other == org.apache.phoenix.coprocessor.generated.PTableProtos.PTable.getDefaultInstance()) return this;
if (other.hasSchemaNameBytes()) {
setSchemaNameBytes(other.getSchemaNameBytes());
}
if (other.hasTableNameBytes()) {
setTableNameBytes(other.getTableNameBytes());
}
if (other.hasTableType()) {
setTableType(other.getTableType());
}
if (other.hasIndexState()) {
bitField0_ |= 0x00000008;
indexState_ = other.indexState_;
onChanged();
}
if (other.hasSequenceNumber()) {
setSequenceNumber(other.getSequenceNumber());
}
if (other.hasTimeStamp()) {
setTimeStamp(other.getTimeStamp());
}
if (other.hasPkNameBytes()) {
setPkNameBytes(other.getPkNameBytes());
}
if (other.hasBucketNum()) {
setBucketNum(other.getBucketNum());
}
if (columnsBuilder_ == null) {
if (!other.columns_.isEmpty()) {
if (columns_.isEmpty()) {
columns_ = other.columns_;
bitField0_ = (bitField0_ & ~0x00000100);
} else {
ensureColumnsIsMutable();
columns_.addAll(other.columns_);
}
onChanged();
}
} else {
if (!other.columns_.isEmpty()) {
if (columnsBuilder_.isEmpty()) {
columnsBuilder_.dispose();
columnsBuilder_ = null;
columns_ = other.columns_;
bitField0_ = (bitField0_ & ~0x00000100);
columnsBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getColumnsFieldBuilder() : null;
} else {
columnsBuilder_.addAllMessages(other.columns_);
}
}
}
if (indexesBuilder_ == null) {
if (!other.indexes_.isEmpty()) {
if (indexes_.isEmpty()) {
indexes_ = other.indexes_;
bitField0_ = (bitField0_ & ~0x00000200);
} else {
ensureIndexesIsMutable();
indexes_.addAll(other.indexes_);
}
onChanged();
}
} else {
if (!other.indexes_.isEmpty()) {
if (indexesBuilder_.isEmpty()) {
indexesBuilder_.dispose();
indexesBuilder_ = null;
indexes_ = other.indexes_;
bitField0_ = (bitField0_ & ~0x00000200);
indexesBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getIndexesFieldBuilder() : null;
} else {
indexesBuilder_.addAllMessages(other.indexes_);
}
}
}
if (other.hasIsImmutableRows()) {
setIsImmutableRows(other.getIsImmutableRows());
}
if (other.hasDataTableNameBytes()) {
setDataTableNameBytes(other.getDataTableNameBytes());
}
if (other.hasDefaultFamilyName()) {
setDefaultFamilyName(other.getDefaultFamilyName());
}
if (other.hasDisableWAL()) {
setDisableWAL(other.getDisableWAL());
}
if (other.hasMultiTenant()) {
setMultiTenant(other.getMultiTenant());
}
if (other.hasViewType()) {
setViewType(other.getViewType());
}
if (other.hasViewStatement()) {
setViewStatement(other.getViewStatement());
}
if (!other.physicalNames_.isEmpty()) {
if (physicalNames_.isEmpty()) {
physicalNames_ = other.physicalNames_;
bitField0_ = (bitField0_ & ~0x00020000);
} else {
ensurePhysicalNamesIsMutable();
physicalNames_.addAll(other.physicalNames_);
}
onChanged();
}
if (other.hasTenantId()) {
setTenantId(other.getTenantId());
}
if (other.hasViewIndexId()) {
setViewIndexId(other.getViewIndexId());
}
if (other.hasIndexType()) {
setIndexType(other.getIndexType());
}
if (other.hasStatsTimeStamp()) {
setStatsTimeStamp(other.getStatsTimeStamp());
}
if (other.hasStoreNulls()) {
setStoreNulls(other.getStoreNulls());
}
if (other.hasBaseColumnCount()) {
setBaseColumnCount(other.getBaseColumnCount());
}
if (other.hasRowKeyOrderOptimizable()) {
setRowKeyOrderOptimizable(other.getRowKeyOrderOptimizable());
}
if (other.hasTransactional()) {
setTransactional(other.getTransactional());
}
if (other.hasUpdateCacheFrequency()) {
setUpdateCacheFrequency(other.getUpdateCacheFrequency());
}
if (other.hasIndexDisableTimestamp()) {
setIndexDisableTimestamp(other.getIndexDisableTimestamp());
}
if (other.hasIsNamespaceMapped()) {
setIsNamespaceMapped(other.getIsNamespaceMapped());
}
if (other.hasAutoParititonSeqName()) {
bitField0_ |= 0x20000000;
autoParititonSeqName_ = other.autoParititonSeqName_;
onChanged();
}
if (other.hasIsAppendOnlySchema()) {
setIsAppendOnlySchema(other.getIsAppendOnlySchema());
}
if (other.hasParentNameBytes()) {
setParentNameBytes(other.getParentNameBytes());
}
if (other.hasStorageScheme()) {
setStorageScheme(other.getStorageScheme());
}
if (other.hasEncodingScheme()) {
setEncodingScheme(other.getEncodingScheme());
}
if (encodedCQCountersBuilder_ == null) {
if (!other.encodedCQCounters_.isEmpty()) {
if (encodedCQCounters_.isEmpty()) {
encodedCQCounters_ = other.encodedCQCounters_;
bitField1_ = (bitField1_ & ~0x00000004);
} else {
ensureEncodedCQCountersIsMutable();
encodedCQCounters_.addAll(other.encodedCQCounters_);
}
onChanged();
}
} else {
if (!other.encodedCQCounters_.isEmpty()) {
if (encodedCQCountersBuilder_.isEmpty()) {
encodedCQCountersBuilder_.dispose();
encodedCQCountersBuilder_ = null;
encodedCQCounters_ = other.encodedCQCounters_;
bitField1_ = (bitField1_ & ~0x00000004);
encodedCQCountersBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getEncodedCQCountersFieldBuilder() : null;
} else {
encodedCQCountersBuilder_.addAllMessages(other.encodedCQCounters_);
}
}
}
if (other.hasUseStatsForParallelization()) {
setUseStatsForParallelization(other.getUseStatsForParallelization());
}
if (other.hasTransactionProvider()) {
setTransactionProvider(other.getTransactionProvider());
}
if (other.hasViewIndexIdType()) {
setViewIndexIdType(other.getViewIndexIdType());
}
if (other.hasViewModifiedUpdateCacheFrequency()) {
setViewModifiedUpdateCacheFrequency(other.getViewModifiedUpdateCacheFrequency());
}
if (other.hasViewModifiedUseStatsForParallelization()) {
setViewModifiedUseStatsForParallelization(other.getViewModifiedUseStatsForParallelization());
}
if (other.hasPhoenixTTL()) {
setPhoenixTTL(other.getPhoenixTTL());
}
if (other.hasPhoenixTTLHighWaterMark()) {
setPhoenixTTLHighWaterMark(other.getPhoenixTTLHighWaterMark());
}
if (other.hasViewModifiedPhoenixTTL()) {
setViewModifiedPhoenixTTL(other.getViewModifiedPhoenixTTL());
}
if (other.hasLastDDLTimestamp()) {
setLastDDLTimestamp(other.getLastDDLTimestamp());
}
if (other.hasChangeDetectionEnabled()) {
setChangeDetectionEnabled(other.getChangeDetectionEnabled());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasSchemaNameBytes()) {
return false;
}
if (!hasTableNameBytes()) {
return false;
}
if (!hasTableType()) {
return false;
}
if (!hasSequenceNumber()) {
return false;
}
if (!hasTimeStamp()) {
return false;
}
if (!hasBucketNum()) {
return false;
}
if (!hasIsImmutableRows()) {
return false;
}
if (!hasDisableWAL()) {
return false;
}
if (!hasMultiTenant()) {
return false;
}
for (int i = 0; i < getColumnsCount(); i++) {
if (!getColumns(i).isInitialized()) {
return false;
}
}
for (int i = 0; i < getIndexesCount(); i++) {
if (!getIndexes(i).isInitialized()) {
return false;
}
}
for (int i = 0; i < getEncodedCQCountersCount(); i++) {
if (!getEncodedCQCounters(i).isInitialized()) {
return false;
}
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.phoenix.coprocessor.generated.PTableProtos.PTable parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.phoenix.coprocessor.generated.PTableProtos.PTable) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private int bitField1_;
// required bytes schemaNameBytes = 1;
private com.google.protobuf.ByteString schemaNameBytes_ = com.google.protobuf.ByteString.EMPTY;
/**
* required bytes schemaNameBytes = 1;
*/
public boolean hasSchemaNameBytes() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required bytes schemaNameBytes = 1;
*/
public com.google.protobuf.ByteString getSchemaNameBytes() {
return schemaNameBytes_;
}
/**
* required bytes schemaNameBytes = 1;
*/
public Builder setSchemaNameBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
schemaNameBytes_ = value;
onChanged();
return this;
}
/**
* required bytes schemaNameBytes = 1;
*/
public Builder clearSchemaNameBytes() {
bitField0_ = (bitField0_ & ~0x00000001);
schemaNameBytes_ = getDefaultInstance().getSchemaNameBytes();
onChanged();
return this;
}
// required bytes tableNameBytes = 2;
private com.google.protobuf.ByteString tableNameBytes_ = com.google.protobuf.ByteString.EMPTY;
/**
* required bytes tableNameBytes = 2;
*/
public boolean hasTableNameBytes() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required bytes tableNameBytes = 2;
*/
public com.google.protobuf.ByteString getTableNameBytes() {
return tableNameBytes_;
}
/**
* required bytes tableNameBytes = 2;
*/
public Builder setTableNameBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
tableNameBytes_ = value;
onChanged();
return this;
}
/**
* required bytes tableNameBytes = 2;
*/
public Builder clearTableNameBytes() {
bitField0_ = (bitField0_ & ~0x00000002);
tableNameBytes_ = getDefaultInstance().getTableNameBytes();
onChanged();
return this;
}
// required .PTableType tableType = 3;
private org.apache.phoenix.coprocessor.generated.PTableProtos.PTableType tableType_ = org.apache.phoenix.coprocessor.generated.PTableProtos.PTableType.SYSTEM;
/**
* required .PTableType tableType = 3;
*/
public boolean hasTableType() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* required .PTableType tableType = 3;
*/
public org.apache.phoenix.coprocessor.generated.PTableProtos.PTableType getTableType() {
return tableType_;
}
/**
* required .PTableType tableType = 3;
*/
public Builder setTableType(org.apache.phoenix.coprocessor.generated.PTableProtos.PTableType value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
tableType_ = value;
onChanged();
return this;
}
/**
* required .PTableType tableType = 3;
*/
public Builder clearTableType() {
bitField0_ = (bitField0_ & ~0x00000004);
tableType_ = org.apache.phoenix.coprocessor.generated.PTableProtos.PTableType.SYSTEM;
onChanged();
return this;
}
// optional string indexState = 4;
private java.lang.Object indexState_ = "";
/**
* optional string indexState = 4;
*/
public boolean hasIndexState() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional string indexState = 4;
*/
public java.lang.String getIndexState() {
java.lang.Object ref = indexState_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
indexState_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string indexState = 4;
*/
public com.google.protobuf.ByteString
getIndexStateBytes() {
java.lang.Object ref = indexState_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
indexState_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string indexState = 4;
*/
public Builder setIndexState(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
indexState_ = value;
onChanged();
return this;
}
/**
* optional string indexState = 4;
*/
public Builder clearIndexState() {
bitField0_ = (bitField0_ & ~0x00000008);
indexState_ = getDefaultInstance().getIndexState();
onChanged();
return this;
}
/**
* optional string indexState = 4;
*/
public Builder setIndexStateBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
indexState_ = value;
onChanged();
return this;
}
// required int64 sequenceNumber = 5;
private long sequenceNumber_ ;
/**
* required int64 sequenceNumber = 5;
*/
public boolean hasSequenceNumber() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* required int64 sequenceNumber = 5;
*/
public long getSequenceNumber() {
return sequenceNumber_;
}
/**
* required int64 sequenceNumber = 5;
*/
public Builder setSequenceNumber(long value) {
bitField0_ |= 0x00000010;
sequenceNumber_ = value;
onChanged();
return this;
}
/**
* required int64 sequenceNumber = 5;
*/
public Builder clearSequenceNumber() {
bitField0_ = (bitField0_ & ~0x00000010);
sequenceNumber_ = 0L;
onChanged();
return this;
}
// required int64 timeStamp = 6;
private long timeStamp_ ;
/**
* required int64 timeStamp = 6;
*/
public boolean hasTimeStamp() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* required int64 timeStamp = 6;
*/
public long getTimeStamp() {
return timeStamp_;
}
/**
* required int64 timeStamp = 6;
*/
public Builder setTimeStamp(long value) {
bitField0_ |= 0x00000020;
timeStamp_ = value;
onChanged();
return this;
}
/**
* required int64 timeStamp = 6;
*/
public Builder clearTimeStamp() {
bitField0_ = (bitField0_ & ~0x00000020);
timeStamp_ = 0L;
onChanged();
return this;
}
// optional bytes pkNameBytes = 7;
private com.google.protobuf.ByteString pkNameBytes_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes pkNameBytes = 7;
*/
public boolean hasPkNameBytes() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
* optional bytes pkNameBytes = 7;
*/
public com.google.protobuf.ByteString getPkNameBytes() {
return pkNameBytes_;
}
/**
* optional bytes pkNameBytes = 7;
*/
public Builder setPkNameBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000040;
pkNameBytes_ = value;
onChanged();
return this;
}
/**
* optional bytes pkNameBytes = 7;
*/
public Builder clearPkNameBytes() {
bitField0_ = (bitField0_ & ~0x00000040);
pkNameBytes_ = getDefaultInstance().getPkNameBytes();
onChanged();
return this;
}
// required int32 bucketNum = 8;
private int bucketNum_ ;
/**
* required int32 bucketNum = 8;
*/
public boolean hasBucketNum() {
return ((bitField0_ & 0x00000080) == 0x00000080);
}
/**
* required int32 bucketNum = 8;
*/
public int getBucketNum() {
return bucketNum_;
}
/**
* required int32 bucketNum = 8;
*/
public Builder setBucketNum(int value) {
bitField0_ |= 0x00000080;
bucketNum_ = value;
onChanged();
return this;
}
/**
* required int32 bucketNum = 8;
*/
public Builder clearBucketNum() {
bitField0_ = (bitField0_ & ~0x00000080);
bucketNum_ = 0;
onChanged();
return this;
}
// repeated .PColumn columns = 9;
private java.util.List columns_ =
java.util.Collections.emptyList();
private void ensureColumnsIsMutable() {
if (!((bitField0_ & 0x00000100) == 0x00000100)) {
columns_ = new java.util.ArrayList(columns_);
bitField0_ |= 0x00000100;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
org.apache.phoenix.coprocessor.generated.PTableProtos.PColumn, org.apache.phoenix.coprocessor.generated.PTableProtos.PColumn.Builder, org.apache.phoenix.coprocessor.generated.PTableProtos.PColumnOrBuilder> columnsBuilder_;
/**
* repeated .PColumn columns = 9;
*/
public java.util.List getColumnsList() {
if (columnsBuilder_ == null) {
return java.util.Collections.unmodifiableList(columns_);
} else {
return columnsBuilder_.getMessageList();
}
}
/**
* repeated .PColumn columns = 9;
*/
public int getColumnsCount() {
if (columnsBuilder_ == null) {
return columns_.size();
} else {
return columnsBuilder_.getCount();
}
}
/**
* repeated .PColumn columns = 9;
*/
public org.apache.phoenix.coprocessor.generated.PTableProtos.PColumn getColumns(int index) {
if (columnsBuilder_ == null) {
return columns_.get(index);
} else {
return columnsBuilder_.getMessage(index);
}
}
/**
* repeated .PColumn columns = 9;
*/
public Builder setColumns(
int index, org.apache.phoenix.coprocessor.generated.PTableProtos.PColumn value) {
if (columnsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureColumnsIsMutable();
columns_.set(index, value);
onChanged();
} else {
columnsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .PColumn columns = 9;
*/
public Builder setColumns(
int index, org.apache.phoenix.coprocessor.generated.PTableProtos.PColumn.Builder builderForValue) {
if (columnsBuilder_ == null) {
ensureColumnsIsMutable();
columns_.set(index, builderForValue.build());
onChanged();
} else {
columnsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .PColumn columns = 9;
*/
public Builder addColumns(org.apache.phoenix.coprocessor.generated.PTableProtos.PColumn value) {
if (columnsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureColumnsIsMutable();
columns_.add(value);
onChanged();
} else {
columnsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .PColumn columns = 9;
*/
public Builder addColumns(
int index, org.apache.phoenix.coprocessor.generated.PTableProtos.PColumn value) {
if (columnsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureColumnsIsMutable();
columns_.add(index, value);
onChanged();
} else {
columnsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .PColumn columns = 9;
*/
public Builder addColumns(
org.apache.phoenix.coprocessor.generated.PTableProtos.PColumn.Builder builderForValue) {
if (columnsBuilder_ == null) {
ensureColumnsIsMutable();
columns_.add(builderForValue.build());
onChanged();
} else {
columnsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .PColumn columns = 9;
*/
public Builder addColumns(
int index, org.apache.phoenix.coprocessor.generated.PTableProtos.PColumn.Builder builderForValue) {
if (columnsBuilder_ == null) {
ensureColumnsIsMutable();
columns_.add(index, builderForValue.build());
onChanged();
} else {
columnsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .PColumn columns = 9;
*/
public Builder addAllColumns(
java.lang.Iterable extends org.apache.phoenix.coprocessor.generated.PTableProtos.PColumn> values) {
if (columnsBuilder_ == null) {
ensureColumnsIsMutable();
super.addAll(values, columns_);
onChanged();
} else {
columnsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .PColumn columns = 9;
*/
public Builder clearColumns() {
if (columnsBuilder_ == null) {
columns_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000100);
onChanged();
} else {
columnsBuilder_.clear();
}
return this;
}
/**
* repeated .PColumn columns = 9;
*/
public Builder removeColumns(int index) {
if (columnsBuilder_ == null) {
ensureColumnsIsMutable();
columns_.remove(index);
onChanged();
} else {
columnsBuilder_.remove(index);
}
return this;
}
/**
* repeated .PColumn columns = 9;
*/
public org.apache.phoenix.coprocessor.generated.PTableProtos.PColumn.Builder getColumnsBuilder(
int index) {
return getColumnsFieldBuilder().getBuilder(index);
}
/**
* repeated .PColumn columns = 9;
*/
public org.apache.phoenix.coprocessor.generated.PTableProtos.PColumnOrBuilder getColumnsOrBuilder(
int index) {
if (columnsBuilder_ == null) {
return columns_.get(index); } else {
return columnsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .PColumn columns = 9;
*/
public java.util.List extends org.apache.phoenix.coprocessor.generated.PTableProtos.PColumnOrBuilder>
getColumnsOrBuilderList() {
if (columnsBuilder_ != null) {
return columnsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(columns_);
}
}
/**
* repeated .PColumn columns = 9;
*/
public org.apache.phoenix.coprocessor.generated.PTableProtos.PColumn.Builder addColumnsBuilder() {
return getColumnsFieldBuilder().addBuilder(
org.apache.phoenix.coprocessor.generated.PTableProtos.PColumn.getDefaultInstance());
}
/**
* repeated .PColumn columns = 9;
*/
public org.apache.phoenix.coprocessor.generated.PTableProtos.PColumn.Builder addColumnsBuilder(
int index) {
return getColumnsFieldBuilder().addBuilder(
index, org.apache.phoenix.coprocessor.generated.PTableProtos.PColumn.getDefaultInstance());
}
/**
* repeated .PColumn columns = 9;
*/
public java.util.List
getColumnsBuilderList() {
return getColumnsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
org.apache.phoenix.coprocessor.generated.PTableProtos.PColumn, org.apache.phoenix.coprocessor.generated.PTableProtos.PColumn.Builder, org.apache.phoenix.coprocessor.generated.PTableProtos.PColumnOrBuilder>
getColumnsFieldBuilder() {
if (columnsBuilder_ == null) {
columnsBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
org.apache.phoenix.coprocessor.generated.PTableProtos.PColumn, org.apache.phoenix.coprocessor.generated.PTableProtos.PColumn.Builder, org.apache.phoenix.coprocessor.generated.PTableProtos.PColumnOrBuilder>(
columns_,
((bitField0_ & 0x00000100) == 0x00000100),
getParentForChildren(),
isClean());
columns_ = null;
}
return columnsBuilder_;
}
// repeated .PTable indexes = 10;
private java.util.List indexes_ =
java.util.Collections.emptyList();
private void ensureIndexesIsMutable() {
if (!((bitField0_ & 0x00000200) == 0x00000200)) {
indexes_ = new java.util.ArrayList(indexes_);
bitField0_ |= 0x00000200;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
org.apache.phoenix.coprocessor.generated.PTableProtos.PTable, org.apache.phoenix.coprocessor.generated.PTableProtos.PTable.Builder, org.apache.phoenix.coprocessor.generated.PTableProtos.PTableOrBuilder> indexesBuilder_;
/**
* repeated .PTable indexes = 10;
*/
public java.util.List getIndexesList() {
if (indexesBuilder_ == null) {
return java.util.Collections.unmodifiableList(indexes_);
} else {
return indexesBuilder_.getMessageList();
}
}
/**
* repeated .PTable indexes = 10;
*/
public int getIndexesCount() {
if (indexesBuilder_ == null) {
return indexes_.size();
} else {
return indexesBuilder_.getCount();
}
}
/**
* repeated .PTable indexes = 10;
*/
public org.apache.phoenix.coprocessor.generated.PTableProtos.PTable getIndexes(int index) {
if (indexesBuilder_ == null) {
return indexes_.get(index);
} else {
return indexesBuilder_.getMessage(index);
}
}
/**
* repeated .PTable indexes = 10;
*/
public Builder setIndexes(
int index, org.apache.phoenix.coprocessor.generated.PTableProtos.PTable value) {
if (indexesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureIndexesIsMutable();
indexes_.set(index, value);
onChanged();
} else {
indexesBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .PTable indexes = 10;
*/
public Builder setIndexes(
int index, org.apache.phoenix.coprocessor.generated.PTableProtos.PTable.Builder builderForValue) {
if (indexesBuilder_ == null) {
ensureIndexesIsMutable();
indexes_.set(index, builderForValue.build());
onChanged();
} else {
indexesBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .PTable indexes = 10;
*/
public Builder addIndexes(org.apache.phoenix.coprocessor.generated.PTableProtos.PTable value) {
if (indexesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureIndexesIsMutable();
indexes_.add(value);
onChanged();
} else {
indexesBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .PTable indexes = 10;
*/
public Builder addIndexes(
int index, org.apache.phoenix.coprocessor.generated.PTableProtos.PTable value) {
if (indexesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureIndexesIsMutable();
indexes_.add(index, value);
onChanged();
} else {
indexesBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .PTable indexes = 10;
*/
public Builder addIndexes(
org.apache.phoenix.coprocessor.generated.PTableProtos.PTable.Builder builderForValue) {
if (indexesBuilder_ == null) {
ensureIndexesIsMutable();
indexes_.add(builderForValue.build());
onChanged();
} else {
indexesBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .PTable indexes = 10;
*/
public Builder addIndexes(
int index, org.apache.phoenix.coprocessor.generated.PTableProtos.PTable.Builder builderForValue) {
if (indexesBuilder_ == null) {
ensureIndexesIsMutable();
indexes_.add(index, builderForValue.build());
onChanged();
} else {
indexesBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .PTable indexes = 10;
*/
public Builder addAllIndexes(
java.lang.Iterable extends org.apache.phoenix.coprocessor.generated.PTableProtos.PTable> values) {
if (indexesBuilder_ == null) {
ensureIndexesIsMutable();
super.addAll(values, indexes_);
onChanged();
} else {
indexesBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .PTable indexes = 10;
*/
public Builder clearIndexes() {
if (indexesBuilder_ == null) {
indexes_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000200);
onChanged();
} else {
indexesBuilder_.clear();
}
return this;
}
/**
* repeated .PTable indexes = 10;
*/
public Builder removeIndexes(int index) {
if (indexesBuilder_ == null) {
ensureIndexesIsMutable();
indexes_.remove(index);
onChanged();
} else {
indexesBuilder_.remove(index);
}
return this;
}
/**
* repeated .PTable indexes = 10;
*/
public org.apache.phoenix.coprocessor.generated.PTableProtos.PTable.Builder getIndexesBuilder(
int index) {
return getIndexesFieldBuilder().getBuilder(index);
}
/**
* repeated .PTable indexes = 10;
*/
public org.apache.phoenix.coprocessor.generated.PTableProtos.PTableOrBuilder getIndexesOrBuilder(
int index) {
if (indexesBuilder_ == null) {
return indexes_.get(index); } else {
return indexesBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .PTable indexes = 10;
*/
public java.util.List extends org.apache.phoenix.coprocessor.generated.PTableProtos.PTableOrBuilder>
getIndexesOrBuilderList() {
if (indexesBuilder_ != null) {
return indexesBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(indexes_);
}
}
/**
* repeated .PTable indexes = 10;
*/
public org.apache.phoenix.coprocessor.generated.PTableProtos.PTable.Builder addIndexesBuilder() {
return getIndexesFieldBuilder().addBuilder(
org.apache.phoenix.coprocessor.generated.PTableProtos.PTable.getDefaultInstance());
}
/**
* repeated .PTable indexes = 10;
*/
public org.apache.phoenix.coprocessor.generated.PTableProtos.PTable.Builder addIndexesBuilder(
int index) {
return getIndexesFieldBuilder().addBuilder(
index, org.apache.phoenix.coprocessor.generated.PTableProtos.PTable.getDefaultInstance());
}
/**
* repeated .PTable indexes = 10;
*/
public java.util.List
getIndexesBuilderList() {
return getIndexesFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
org.apache.phoenix.coprocessor.generated.PTableProtos.PTable, org.apache.phoenix.coprocessor.generated.PTableProtos.PTable.Builder, org.apache.phoenix.coprocessor.generated.PTableProtos.PTableOrBuilder>
getIndexesFieldBuilder() {
if (indexesBuilder_ == null) {
indexesBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
org.apache.phoenix.coprocessor.generated.PTableProtos.PTable, org.apache.phoenix.coprocessor.generated.PTableProtos.PTable.Builder, org.apache.phoenix.coprocessor.generated.PTableProtos.PTableOrBuilder>(
indexes_,
((bitField0_ & 0x00000200) == 0x00000200),
getParentForChildren(),
isClean());
indexes_ = null;
}
return indexesBuilder_;
}
// required bool isImmutableRows = 11;
private boolean isImmutableRows_ ;
/**
* required bool isImmutableRows = 11;
*/
public boolean hasIsImmutableRows() {
return ((bitField0_ & 0x00000400) == 0x00000400);
}
/**
* required bool isImmutableRows = 11;
*/
public boolean getIsImmutableRows() {
return isImmutableRows_;
}
/**
* required bool isImmutableRows = 11;
*/
public Builder setIsImmutableRows(boolean value) {
bitField0_ |= 0x00000400;
isImmutableRows_ = value;
onChanged();
return this;
}
/**
* required bool isImmutableRows = 11;
*/
public Builder clearIsImmutableRows() {
bitField0_ = (bitField0_ & ~0x00000400);
isImmutableRows_ = false;
onChanged();
return this;
}
// optional bytes dataTableNameBytes = 13;
private com.google.protobuf.ByteString dataTableNameBytes_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes dataTableNameBytes = 13;
*
*
* Do NOT reuse the tag '12'. Stats are no longer passed
* along with the PTable.
*repeated PTableStats guidePosts = 12;
* TODO remove this field in 5.0 release
*
*/
public boolean hasDataTableNameBytes() {
return ((bitField0_ & 0x00000800) == 0x00000800);
}
/**
* optional bytes dataTableNameBytes = 13;
*
*
* Do NOT reuse the tag '12'. Stats are no longer passed
* along with the PTable.
*repeated PTableStats guidePosts = 12;
* TODO remove this field in 5.0 release
*
*/
public com.google.protobuf.ByteString getDataTableNameBytes() {
return dataTableNameBytes_;
}
/**
* optional bytes dataTableNameBytes = 13;
*
*
* Do NOT reuse the tag '12'. Stats are no longer passed
* along with the PTable.
*repeated PTableStats guidePosts = 12;
* TODO remove this field in 5.0 release
*
*/
public Builder setDataTableNameBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000800;
dataTableNameBytes_ = value;
onChanged();
return this;
}
/**
* optional bytes dataTableNameBytes = 13;
*
*
* Do NOT reuse the tag '12'. Stats are no longer passed
* along with the PTable.
*repeated PTableStats guidePosts = 12;
* TODO remove this field in 5.0 release
*
*/
public Builder clearDataTableNameBytes() {
bitField0_ = (bitField0_ & ~0x00000800);
dataTableNameBytes_ = getDefaultInstance().getDataTableNameBytes();
onChanged();
return this;
}
// optional bytes defaultFamilyName = 14;
private com.google.protobuf.ByteString defaultFamilyName_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes defaultFamilyName = 14;
*/
public boolean hasDefaultFamilyName() {
return ((bitField0_ & 0x00001000) == 0x00001000);
}
/**
* optional bytes defaultFamilyName = 14;
*/
public com.google.protobuf.ByteString getDefaultFamilyName() {
return defaultFamilyName_;
}
/**
* optional bytes defaultFamilyName = 14;
*/
public Builder setDefaultFamilyName(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00001000;
defaultFamilyName_ = value;
onChanged();
return this;
}
/**
* optional bytes defaultFamilyName = 14;
*/
public Builder clearDefaultFamilyName() {
bitField0_ = (bitField0_ & ~0x00001000);
defaultFamilyName_ = getDefaultInstance().getDefaultFamilyName();
onChanged();
return this;
}
// required bool disableWAL = 15;
private boolean disableWAL_ ;
/**
* required bool disableWAL = 15;
*/
public boolean hasDisableWAL() {
return ((bitField0_ & 0x00002000) == 0x00002000);
}
/**
* required bool disableWAL = 15;
*/
public boolean getDisableWAL() {
return disableWAL_;
}
/**
* required bool disableWAL = 15;
*/
public Builder setDisableWAL(boolean value) {
bitField0_ |= 0x00002000;
disableWAL_ = value;
onChanged();
return this;
}
/**
* required bool disableWAL = 15;
*/
public Builder clearDisableWAL() {
bitField0_ = (bitField0_ & ~0x00002000);
disableWAL_ = false;
onChanged();
return this;
}
// required bool multiTenant = 16;
private boolean multiTenant_ ;
/**
* required bool multiTenant = 16;
*/
public boolean hasMultiTenant() {
return ((bitField0_ & 0x00004000) == 0x00004000);
}
/**
* required bool multiTenant = 16;
*/
public boolean getMultiTenant() {
return multiTenant_;
}
/**
* required bool multiTenant = 16;
*/
public Builder setMultiTenant(boolean value) {
bitField0_ |= 0x00004000;
multiTenant_ = value;
onChanged();
return this;
}
/**
* required bool multiTenant = 16;
*/
public Builder clearMultiTenant() {
bitField0_ = (bitField0_ & ~0x00004000);
multiTenant_ = false;
onChanged();
return this;
}
// optional bytes viewType = 17;
private com.google.protobuf.ByteString viewType_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes viewType = 17;
*/
public boolean hasViewType() {
return ((bitField0_ & 0x00008000) == 0x00008000);
}
/**
* optional bytes viewType = 17;
*/
public com.google.protobuf.ByteString getViewType() {
return viewType_;
}
/**
* optional bytes viewType = 17;
*/
public Builder setViewType(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00008000;
viewType_ = value;
onChanged();
return this;
}
/**
* optional bytes viewType = 17;
*/
public Builder clearViewType() {
bitField0_ = (bitField0_ & ~0x00008000);
viewType_ = getDefaultInstance().getViewType();
onChanged();
return this;
}
// optional bytes viewStatement = 18;
private com.google.protobuf.ByteString viewStatement_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes viewStatement = 18;
*/
public boolean hasViewStatement() {
return ((bitField0_ & 0x00010000) == 0x00010000);
}
/**
* optional bytes viewStatement = 18;
*/
public com.google.protobuf.ByteString getViewStatement() {
return viewStatement_;
}
/**
* optional bytes viewStatement = 18;
*/
public Builder setViewStatement(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00010000;
viewStatement_ = value;
onChanged();
return this;
}
/**
* optional bytes viewStatement = 18;
*/
public Builder clearViewStatement() {
bitField0_ = (bitField0_ & ~0x00010000);
viewStatement_ = getDefaultInstance().getViewStatement();
onChanged();
return this;
}
// repeated bytes physicalNames = 19;
private java.util.List physicalNames_ = java.util.Collections.emptyList();
private void ensurePhysicalNamesIsMutable() {
if (!((bitField0_ & 0x00020000) == 0x00020000)) {
physicalNames_ = new java.util.ArrayList(physicalNames_);
bitField0_ |= 0x00020000;
}
}
/**
* repeated bytes physicalNames = 19;
*/
public java.util.List
getPhysicalNamesList() {
return java.util.Collections.unmodifiableList(physicalNames_);
}
/**
* repeated bytes physicalNames = 19;
*/
public int getPhysicalNamesCount() {
return physicalNames_.size();
}
/**
* repeated bytes physicalNames = 19;
*/
public com.google.protobuf.ByteString getPhysicalNames(int index) {
return physicalNames_.get(index);
}
/**
* repeated bytes physicalNames = 19;
*/
public Builder setPhysicalNames(
int index, com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensurePhysicalNamesIsMutable();
physicalNames_.set(index, value);
onChanged();
return this;
}
/**
* repeated bytes physicalNames = 19;
*/
public Builder addPhysicalNames(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensurePhysicalNamesIsMutable();
physicalNames_.add(value);
onChanged();
return this;
}
/**
* repeated bytes physicalNames = 19;
*/
public Builder addAllPhysicalNames(
java.lang.Iterable extends com.google.protobuf.ByteString> values) {
ensurePhysicalNamesIsMutable();
super.addAll(values, physicalNames_);
onChanged();
return this;
}
/**
* repeated bytes physicalNames = 19;
*/
public Builder clearPhysicalNames() {
physicalNames_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00020000);
onChanged();
return this;
}
// optional bytes tenantId = 20;
private com.google.protobuf.ByteString tenantId_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes tenantId = 20;
*/
public boolean hasTenantId() {
return ((bitField0_ & 0x00040000) == 0x00040000);
}
/**
* optional bytes tenantId = 20;
*/
public com.google.protobuf.ByteString getTenantId() {
return tenantId_;
}
/**
* optional bytes tenantId = 20;
*/
public Builder setTenantId(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00040000;
tenantId_ = value;
onChanged();
return this;
}
/**
* optional bytes tenantId = 20;
*/
public Builder clearTenantId() {
bitField0_ = (bitField0_ & ~0x00040000);
tenantId_ = getDefaultInstance().getTenantId();
onChanged();
return this;
}
// optional int64 viewIndexId = 21;
private long viewIndexId_ ;
/**
* optional int64 viewIndexId = 21;
*/
public boolean hasViewIndexId() {
return ((bitField0_ & 0x00080000) == 0x00080000);
}
/**
* optional int64 viewIndexId = 21;
*/
public long getViewIndexId() {
return viewIndexId_;
}
/**
* optional int64 viewIndexId = 21;
*/
public Builder setViewIndexId(long value) {
bitField0_ |= 0x00080000;
viewIndexId_ = value;
onChanged();
return this;
}
/**
* optional int64 viewIndexId = 21;
*/
public Builder clearViewIndexId() {
bitField0_ = (bitField0_ & ~0x00080000);
viewIndexId_ = 0L;
onChanged();
return this;
}
// optional bytes indexType = 22;
private com.google.protobuf.ByteString indexType_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes indexType = 22;
*/
public boolean hasIndexType() {
return ((bitField0_ & 0x00100000) == 0x00100000);
}
/**
* optional bytes indexType = 22;
*/
public com.google.protobuf.ByteString getIndexType() {
return indexType_;
}
/**
* optional bytes indexType = 22;
*/
public Builder setIndexType(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00100000;
indexType_ = value;
onChanged();
return this;
}
/**
* optional bytes indexType = 22;
*/
public Builder clearIndexType() {
bitField0_ = (bitField0_ & ~0x00100000);
indexType_ = getDefaultInstance().getIndexType();
onChanged();
return this;
}
// optional int64 statsTimeStamp = 23;
private long statsTimeStamp_ ;
/**
* optional int64 statsTimeStamp = 23;
*/
public boolean hasStatsTimeStamp() {
return ((bitField0_ & 0x00200000) == 0x00200000);
}
/**
* optional int64 statsTimeStamp = 23;
*/
public long getStatsTimeStamp() {
return statsTimeStamp_;
}
/**
* optional int64 statsTimeStamp = 23;
*/
public Builder setStatsTimeStamp(long value) {
bitField0_ |= 0x00200000;
statsTimeStamp_ = value;
onChanged();
return this;
}
/**
* optional int64 statsTimeStamp = 23;
*/
public Builder clearStatsTimeStamp() {
bitField0_ = (bitField0_ & ~0x00200000);
statsTimeStamp_ = 0L;
onChanged();
return this;
}
// optional bool storeNulls = 24;
private boolean storeNulls_ ;
/**
* optional bool storeNulls = 24;
*/
public boolean hasStoreNulls() {
return ((bitField0_ & 0x00400000) == 0x00400000);
}
/**
* optional bool storeNulls = 24;
*/
public boolean getStoreNulls() {
return storeNulls_;
}
/**
* optional bool storeNulls = 24;
*/
public Builder setStoreNulls(boolean value) {
bitField0_ |= 0x00400000;
storeNulls_ = value;
onChanged();
return this;
}
/**
* optional bool storeNulls = 24;
*/
public Builder clearStoreNulls() {
bitField0_ = (bitField0_ & ~0x00400000);
storeNulls_ = false;
onChanged();
return this;
}
// optional int32 baseColumnCount = 25;
private int baseColumnCount_ ;
/**
* optional int32 baseColumnCount = 25;
*/
public boolean hasBaseColumnCount() {
return ((bitField0_ & 0x00800000) == 0x00800000);
}
/**
* optional int32 baseColumnCount = 25;
*/
public int getBaseColumnCount() {
return baseColumnCount_;
}
/**
* optional int32 baseColumnCount = 25;
*/
public Builder setBaseColumnCount(int value) {
bitField0_ |= 0x00800000;
baseColumnCount_ = value;
onChanged();
return this;
}
/**
* optional int32 baseColumnCount = 25;
*/
public Builder clearBaseColumnCount() {
bitField0_ = (bitField0_ & ~0x00800000);
baseColumnCount_ = 0;
onChanged();
return this;
}
// optional bool rowKeyOrderOptimizable = 26;
private boolean rowKeyOrderOptimizable_ ;
/**
* optional bool rowKeyOrderOptimizable = 26;
*/
public boolean hasRowKeyOrderOptimizable() {
return ((bitField0_ & 0x01000000) == 0x01000000);
}
/**
* optional bool rowKeyOrderOptimizable = 26;
*/
public boolean getRowKeyOrderOptimizable() {
return rowKeyOrderOptimizable_;
}
/**
* optional bool rowKeyOrderOptimizable = 26;
*/
public Builder setRowKeyOrderOptimizable(boolean value) {
bitField0_ |= 0x01000000;
rowKeyOrderOptimizable_ = value;
onChanged();
return this;
}
/**
* optional bool rowKeyOrderOptimizable = 26;
*/
public Builder clearRowKeyOrderOptimizable() {
bitField0_ = (bitField0_ & ~0x01000000);
rowKeyOrderOptimizable_ = false;
onChanged();
return this;
}
// optional bool transactional = 27;
private boolean transactional_ ;
/**
* optional bool transactional = 27;
*/
public boolean hasTransactional() {
return ((bitField0_ & 0x02000000) == 0x02000000);
}
/**
* optional bool transactional = 27;
*/
public boolean getTransactional() {
return transactional_;
}
/**
* optional bool transactional = 27;
*/
public Builder setTransactional(boolean value) {
bitField0_ |= 0x02000000;
transactional_ = value;
onChanged();
return this;
}
/**
* optional bool transactional = 27;
*/
public Builder clearTransactional() {
bitField0_ = (bitField0_ & ~0x02000000);
transactional_ = false;
onChanged();
return this;
}
// optional int64 updateCacheFrequency = 28;
private long updateCacheFrequency_ ;
/**
* optional int64 updateCacheFrequency = 28;
*/
public boolean hasUpdateCacheFrequency() {
return ((bitField0_ & 0x04000000) == 0x04000000);
}
/**
* optional int64 updateCacheFrequency = 28;
*/
public long getUpdateCacheFrequency() {
return updateCacheFrequency_;
}
/**
* optional int64 updateCacheFrequency = 28;
*/
public Builder setUpdateCacheFrequency(long value) {
bitField0_ |= 0x04000000;
updateCacheFrequency_ = value;
onChanged();
return this;
}
/**
* optional int64 updateCacheFrequency = 28;
*/
public Builder clearUpdateCacheFrequency() {
bitField0_ = (bitField0_ & ~0x04000000);
updateCacheFrequency_ = 0L;
onChanged();
return this;
}
// optional int64 indexDisableTimestamp = 29;
private long indexDisableTimestamp_ ;
/**
* optional int64 indexDisableTimestamp = 29;
*/
public boolean hasIndexDisableTimestamp() {
return ((bitField0_ & 0x08000000) == 0x08000000);
}
/**
* optional int64 indexDisableTimestamp = 29;
*/
public long getIndexDisableTimestamp() {
return indexDisableTimestamp_;
}
/**
* optional int64 indexDisableTimestamp = 29;
*/
public Builder setIndexDisableTimestamp(long value) {
bitField0_ |= 0x08000000;
indexDisableTimestamp_ = value;
onChanged();
return this;
}
/**
* optional int64 indexDisableTimestamp = 29;
*/
public Builder clearIndexDisableTimestamp() {
bitField0_ = (bitField0_ & ~0x08000000);
indexDisableTimestamp_ = 0L;
onChanged();
return this;
}
// optional bool isNamespaceMapped = 30;
private boolean isNamespaceMapped_ ;
/**
* optional bool isNamespaceMapped = 30;
*/
public boolean hasIsNamespaceMapped() {
return ((bitField0_ & 0x10000000) == 0x10000000);
}
/**
* optional bool isNamespaceMapped = 30;
*/
public boolean getIsNamespaceMapped() {
return isNamespaceMapped_;
}
/**
* optional bool isNamespaceMapped = 30;
*/
public Builder setIsNamespaceMapped(boolean value) {
bitField0_ |= 0x10000000;
isNamespaceMapped_ = value;
onChanged();
return this;
}
/**
* optional bool isNamespaceMapped = 30;
*/
public Builder clearIsNamespaceMapped() {
bitField0_ = (bitField0_ & ~0x10000000);
isNamespaceMapped_ = false;
onChanged();
return this;
}
// optional string autoParititonSeqName = 31;
private java.lang.Object autoParititonSeqName_ = "";
/**
* optional string autoParititonSeqName = 31;
*/
public boolean hasAutoParititonSeqName() {
return ((bitField0_ & 0x20000000) == 0x20000000);
}
/**
* optional string autoParititonSeqName = 31;
*/
public java.lang.String getAutoParititonSeqName() {
java.lang.Object ref = autoParititonSeqName_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
autoParititonSeqName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string autoParititonSeqName = 31;
*/
public com.google.protobuf.ByteString
getAutoParititonSeqNameBytes() {
java.lang.Object ref = autoParititonSeqName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
autoParititonSeqName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string autoParititonSeqName = 31;
*/
public Builder setAutoParititonSeqName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x20000000;
autoParititonSeqName_ = value;
onChanged();
return this;
}
/**
* optional string autoParititonSeqName = 31;
*/
public Builder clearAutoParititonSeqName() {
bitField0_ = (bitField0_ & ~0x20000000);
autoParititonSeqName_ = getDefaultInstance().getAutoParititonSeqName();
onChanged();
return this;
}
/**
* optional string autoParititonSeqName = 31;
*/
public Builder setAutoParititonSeqNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x20000000;
autoParititonSeqName_ = value;
onChanged();
return this;
}
// optional bool isAppendOnlySchema = 32;
private boolean isAppendOnlySchema_ ;
/**
* optional bool isAppendOnlySchema = 32;
*/
public boolean hasIsAppendOnlySchema() {
return ((bitField0_ & 0x40000000) == 0x40000000);
}
/**
* optional bool isAppendOnlySchema = 32;
*/
public boolean getIsAppendOnlySchema() {
return isAppendOnlySchema_;
}
/**
* optional bool isAppendOnlySchema = 32;
*/
public Builder setIsAppendOnlySchema(boolean value) {
bitField0_ |= 0x40000000;
isAppendOnlySchema_ = value;
onChanged();
return this;
}
/**
* optional bool isAppendOnlySchema = 32;
*/
public Builder clearIsAppendOnlySchema() {
bitField0_ = (bitField0_ & ~0x40000000);
isAppendOnlySchema_ = false;
onChanged();
return this;
}
// optional bytes parentNameBytes = 33;
private com.google.protobuf.ByteString parentNameBytes_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes parentNameBytes = 33;
*/
public boolean hasParentNameBytes() {
return ((bitField0_ & 0x80000000) == 0x80000000);
}
/**
* optional bytes parentNameBytes = 33;
*/
public com.google.protobuf.ByteString getParentNameBytes() {
return parentNameBytes_;
}
/**
* optional bytes parentNameBytes = 33;
*/
public Builder setParentNameBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x80000000;
parentNameBytes_ = value;
onChanged();
return this;
}
/**
* optional bytes parentNameBytes = 33;
*/
public Builder clearParentNameBytes() {
bitField0_ = (bitField0_ & ~0x80000000);
parentNameBytes_ = getDefaultInstance().getParentNameBytes();
onChanged();
return this;
}
// optional bytes storageScheme = 34;
private com.google.protobuf.ByteString storageScheme_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes storageScheme = 34;
*/
public boolean hasStorageScheme() {
return ((bitField1_ & 0x00000001) == 0x00000001);
}
/**
* optional bytes storageScheme = 34;
*/
public com.google.protobuf.ByteString getStorageScheme() {
return storageScheme_;
}
/**
* optional bytes storageScheme = 34;
*/
public Builder setStorageScheme(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField1_ |= 0x00000001;
storageScheme_ = value;
onChanged();
return this;
}
/**
* optional bytes storageScheme = 34;
*/
public Builder clearStorageScheme() {
bitField1_ = (bitField1_ & ~0x00000001);
storageScheme_ = getDefaultInstance().getStorageScheme();
onChanged();
return this;
}
// optional bytes encodingScheme = 35;
private com.google.protobuf.ByteString encodingScheme_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes encodingScheme = 35;
*/
public boolean hasEncodingScheme() {
return ((bitField1_ & 0x00000002) == 0x00000002);
}
/**
* optional bytes encodingScheme = 35;
*/
public com.google.protobuf.ByteString getEncodingScheme() {
return encodingScheme_;
}
/**
* optional bytes encodingScheme = 35;
*/
public Builder setEncodingScheme(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField1_ |= 0x00000002;
encodingScheme_ = value;
onChanged();
return this;
}
/**
* optional bytes encodingScheme = 35;
*/
public Builder clearEncodingScheme() {
bitField1_ = (bitField1_ & ~0x00000002);
encodingScheme_ = getDefaultInstance().getEncodingScheme();
onChanged();
return this;
}
// repeated .EncodedCQCounter encodedCQCounters = 36;
private java.util.List encodedCQCounters_ =
java.util.Collections.emptyList();
private void ensureEncodedCQCountersIsMutable() {
if (!((bitField1_ & 0x00000004) == 0x00000004)) {
encodedCQCounters_ = new java.util.ArrayList(encodedCQCounters_);
bitField1_ |= 0x00000004;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
org.apache.phoenix.coprocessor.generated.PTableProtos.EncodedCQCounter, org.apache.phoenix.coprocessor.generated.PTableProtos.EncodedCQCounter.Builder, org.apache.phoenix.coprocessor.generated.PTableProtos.EncodedCQCounterOrBuilder> encodedCQCountersBuilder_;
/**
* repeated .EncodedCQCounter encodedCQCounters = 36;
*/
public java.util.List getEncodedCQCountersList() {
if (encodedCQCountersBuilder_ == null) {
return java.util.Collections.unmodifiableList(encodedCQCounters_);
} else {
return encodedCQCountersBuilder_.getMessageList();
}
}
/**
* repeated .EncodedCQCounter encodedCQCounters = 36;
*/
public int getEncodedCQCountersCount() {
if (encodedCQCountersBuilder_ == null) {
return encodedCQCounters_.size();
} else {
return encodedCQCountersBuilder_.getCount();
}
}
/**
* repeated .EncodedCQCounter encodedCQCounters = 36;
*/
public org.apache.phoenix.coprocessor.generated.PTableProtos.EncodedCQCounter getEncodedCQCounters(int index) {
if (encodedCQCountersBuilder_ == null) {
return encodedCQCounters_.get(index);
} else {
return encodedCQCountersBuilder_.getMessage(index);
}
}
/**
* repeated .EncodedCQCounter encodedCQCounters = 36;
*/
public Builder setEncodedCQCounters(
int index, org.apache.phoenix.coprocessor.generated.PTableProtos.EncodedCQCounter value) {
if (encodedCQCountersBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureEncodedCQCountersIsMutable();
encodedCQCounters_.set(index, value);
onChanged();
} else {
encodedCQCountersBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .EncodedCQCounter encodedCQCounters = 36;
*/
public Builder setEncodedCQCounters(
int index, org.apache.phoenix.coprocessor.generated.PTableProtos.EncodedCQCounter.Builder builderForValue) {
if (encodedCQCountersBuilder_ == null) {
ensureEncodedCQCountersIsMutable();
encodedCQCounters_.set(index, builderForValue.build());
onChanged();
} else {
encodedCQCountersBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .EncodedCQCounter encodedCQCounters = 36;
*/
public Builder addEncodedCQCounters(org.apache.phoenix.coprocessor.generated.PTableProtos.EncodedCQCounter value) {
if (encodedCQCountersBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureEncodedCQCountersIsMutable();
encodedCQCounters_.add(value);
onChanged();
} else {
encodedCQCountersBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .EncodedCQCounter encodedCQCounters = 36;
*/
public Builder addEncodedCQCounters(
int index, org.apache.phoenix.coprocessor.generated.PTableProtos.EncodedCQCounter value) {
if (encodedCQCountersBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureEncodedCQCountersIsMutable();
encodedCQCounters_.add(index, value);
onChanged();
} else {
encodedCQCountersBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .EncodedCQCounter encodedCQCounters = 36;
*/
public Builder addEncodedCQCounters(
org.apache.phoenix.coprocessor.generated.PTableProtos.EncodedCQCounter.Builder builderForValue) {
if (encodedCQCountersBuilder_ == null) {
ensureEncodedCQCountersIsMutable();
encodedCQCounters_.add(builderForValue.build());
onChanged();
} else {
encodedCQCountersBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .EncodedCQCounter encodedCQCounters = 36;
*/
public Builder addEncodedCQCounters(
int index, org.apache.phoenix.coprocessor.generated.PTableProtos.EncodedCQCounter.Builder builderForValue) {
if (encodedCQCountersBuilder_ == null) {
ensureEncodedCQCountersIsMutable();
encodedCQCounters_.add(index, builderForValue.build());
onChanged();
} else {
encodedCQCountersBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .EncodedCQCounter encodedCQCounters = 36;
*/
public Builder addAllEncodedCQCounters(
java.lang.Iterable extends org.apache.phoenix.coprocessor.generated.PTableProtos.EncodedCQCounter> values) {
if (encodedCQCountersBuilder_ == null) {
ensureEncodedCQCountersIsMutable();
super.addAll(values, encodedCQCounters_);
onChanged();
} else {
encodedCQCountersBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .EncodedCQCounter encodedCQCounters = 36;
*/
public Builder clearEncodedCQCounters() {
if (encodedCQCountersBuilder_ == null) {
encodedCQCounters_ = java.util.Collections.emptyList();
bitField1_ = (bitField1_ & ~0x00000004);
onChanged();
} else {
encodedCQCountersBuilder_.clear();
}
return this;
}
/**
* repeated .EncodedCQCounter encodedCQCounters = 36;
*/
public Builder removeEncodedCQCounters(int index) {
if (encodedCQCountersBuilder_ == null) {
ensureEncodedCQCountersIsMutable();
encodedCQCounters_.remove(index);
onChanged();
} else {
encodedCQCountersBuilder_.remove(index);
}
return this;
}
/**
* repeated .EncodedCQCounter encodedCQCounters = 36;
*/
public org.apache.phoenix.coprocessor.generated.PTableProtos.EncodedCQCounter.Builder getEncodedCQCountersBuilder(
int index) {
return getEncodedCQCountersFieldBuilder().getBuilder(index);
}
/**
* repeated .EncodedCQCounter encodedCQCounters = 36;
*/
public org.apache.phoenix.coprocessor.generated.PTableProtos.EncodedCQCounterOrBuilder getEncodedCQCountersOrBuilder(
int index) {
if (encodedCQCountersBuilder_ == null) {
return encodedCQCounters_.get(index); } else {
return encodedCQCountersBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .EncodedCQCounter encodedCQCounters = 36;
*/
public java.util.List extends org.apache.phoenix.coprocessor.generated.PTableProtos.EncodedCQCounterOrBuilder>
getEncodedCQCountersOrBuilderList() {
if (encodedCQCountersBuilder_ != null) {
return encodedCQCountersBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(encodedCQCounters_);
}
}
/**
* repeated .EncodedCQCounter encodedCQCounters = 36;
*/
public org.apache.phoenix.coprocessor.generated.PTableProtos.EncodedCQCounter.Builder addEncodedCQCountersBuilder() {
return getEncodedCQCountersFieldBuilder().addBuilder(
org.apache.phoenix.coprocessor.generated.PTableProtos.EncodedCQCounter.getDefaultInstance());
}
/**
* repeated .EncodedCQCounter encodedCQCounters = 36;
*/
public org.apache.phoenix.coprocessor.generated.PTableProtos.EncodedCQCounter.Builder addEncodedCQCountersBuilder(
int index) {
return getEncodedCQCountersFieldBuilder().addBuilder(
index, org.apache.phoenix.coprocessor.generated.PTableProtos.EncodedCQCounter.getDefaultInstance());
}
/**
* repeated .EncodedCQCounter encodedCQCounters = 36;
*/
public java.util.List
getEncodedCQCountersBuilderList() {
return getEncodedCQCountersFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
org.apache.phoenix.coprocessor.generated.PTableProtos.EncodedCQCounter, org.apache.phoenix.coprocessor.generated.PTableProtos.EncodedCQCounter.Builder, org.apache.phoenix.coprocessor.generated.PTableProtos.EncodedCQCounterOrBuilder>
getEncodedCQCountersFieldBuilder() {
if (encodedCQCountersBuilder_ == null) {
encodedCQCountersBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
org.apache.phoenix.coprocessor.generated.PTableProtos.EncodedCQCounter, org.apache.phoenix.coprocessor.generated.PTableProtos.EncodedCQCounter.Builder, org.apache.phoenix.coprocessor.generated.PTableProtos.EncodedCQCounterOrBuilder>(
encodedCQCounters_,
((bitField1_ & 0x00000004) == 0x00000004),
getParentForChildren(),
isClean());
encodedCQCounters_ = null;
}
return encodedCQCountersBuilder_;
}
// optional bool useStatsForParallelization = 37;
private boolean useStatsForParallelization_ ;
/**
* optional bool useStatsForParallelization = 37;
*/
public boolean hasUseStatsForParallelization() {
return ((bitField1_ & 0x00000008) == 0x00000008);
}
/**
* optional bool useStatsForParallelization = 37;
*/
public boolean getUseStatsForParallelization() {
return useStatsForParallelization_;
}
/**
* optional bool useStatsForParallelization = 37;
*/
public Builder setUseStatsForParallelization(boolean value) {
bitField1_ |= 0x00000008;
useStatsForParallelization_ = value;
onChanged();
return this;
}
/**
* optional bool useStatsForParallelization = 37;
*/
public Builder clearUseStatsForParallelization() {
bitField1_ = (bitField1_ & ~0x00000008);
useStatsForParallelization_ = false;
onChanged();
return this;
}
// optional int32 transactionProvider = 38;
private int transactionProvider_ ;
/**
* optional int32 transactionProvider = 38;
*/
public boolean hasTransactionProvider() {
return ((bitField1_ & 0x00000010) == 0x00000010);
}
/**
* optional int32 transactionProvider = 38;
*/
public int getTransactionProvider() {
return transactionProvider_;
}
/**
* optional int32 transactionProvider = 38;
*/
public Builder setTransactionProvider(int value) {
bitField1_ |= 0x00000010;
transactionProvider_ = value;
onChanged();
return this;
}
/**
* optional int32 transactionProvider = 38;
*/
public Builder clearTransactionProvider() {
bitField1_ = (bitField1_ & ~0x00000010);
transactionProvider_ = 0;
onChanged();
return this;
}
// optional int32 viewIndexIdType = 39 [default = 5];
private int viewIndexIdType_ = 5;
/**
* optional int32 viewIndexIdType = 39 [default = 5];
*/
public boolean hasViewIndexIdType() {
return ((bitField1_ & 0x00000020) == 0x00000020);
}
/**
* optional int32 viewIndexIdType = 39 [default = 5];
*/
public int getViewIndexIdType() {
return viewIndexIdType_;
}
/**
* optional int32 viewIndexIdType = 39 [default = 5];
*/
public Builder setViewIndexIdType(int value) {
bitField1_ |= 0x00000020;
viewIndexIdType_ = value;
onChanged();
return this;
}
/**
* optional int32 viewIndexIdType = 39 [default = 5];
*/
public Builder clearViewIndexIdType() {
bitField1_ = (bitField1_ & ~0x00000020);
viewIndexIdType_ = 5;
onChanged();
return this;
}
// optional bool viewModifiedUpdateCacheFrequency = 40;
private boolean viewModifiedUpdateCacheFrequency_ ;
/**
* optional bool viewModifiedUpdateCacheFrequency = 40;
*/
public boolean hasViewModifiedUpdateCacheFrequency() {
return ((bitField1_ & 0x00000040) == 0x00000040);
}
/**
* optional bool viewModifiedUpdateCacheFrequency = 40;
*/
public boolean getViewModifiedUpdateCacheFrequency() {
return viewModifiedUpdateCacheFrequency_;
}
/**
* optional bool viewModifiedUpdateCacheFrequency = 40;
*/
public Builder setViewModifiedUpdateCacheFrequency(boolean value) {
bitField1_ |= 0x00000040;
viewModifiedUpdateCacheFrequency_ = value;
onChanged();
return this;
}
/**
* optional bool viewModifiedUpdateCacheFrequency = 40;
*/
public Builder clearViewModifiedUpdateCacheFrequency() {
bitField1_ = (bitField1_ & ~0x00000040);
viewModifiedUpdateCacheFrequency_ = false;
onChanged();
return this;
}
// optional bool viewModifiedUseStatsForParallelization = 41;
private boolean viewModifiedUseStatsForParallelization_ ;
/**
* optional bool viewModifiedUseStatsForParallelization = 41;
*/
public boolean hasViewModifiedUseStatsForParallelization() {
return ((bitField1_ & 0x00000080) == 0x00000080);
}
/**
* optional bool viewModifiedUseStatsForParallelization = 41;
*/
public boolean getViewModifiedUseStatsForParallelization() {
return viewModifiedUseStatsForParallelization_;
}
/**
* optional bool viewModifiedUseStatsForParallelization = 41;
*/
public Builder setViewModifiedUseStatsForParallelization(boolean value) {
bitField1_ |= 0x00000080;
viewModifiedUseStatsForParallelization_ = value;
onChanged();
return this;
}
/**
* optional bool viewModifiedUseStatsForParallelization = 41;
*/
public Builder clearViewModifiedUseStatsForParallelization() {
bitField1_ = (bitField1_ & ~0x00000080);
viewModifiedUseStatsForParallelization_ = false;
onChanged();
return this;
}
// optional int64 phoenixTTL = 42;
private long phoenixTTL_ ;
/**
* optional int64 phoenixTTL = 42;
*/
public boolean hasPhoenixTTL() {
return ((bitField1_ & 0x00000100) == 0x00000100);
}
/**
* optional int64 phoenixTTL = 42;
*/
public long getPhoenixTTL() {
return phoenixTTL_;
}
/**
* optional int64 phoenixTTL = 42;
*/
public Builder setPhoenixTTL(long value) {
bitField1_ |= 0x00000100;
phoenixTTL_ = value;
onChanged();
return this;
}
/**
* optional int64 phoenixTTL = 42;
*/
public Builder clearPhoenixTTL() {
bitField1_ = (bitField1_ & ~0x00000100);
phoenixTTL_ = 0L;
onChanged();
return this;
}
// optional int64 phoenixTTLHighWaterMark = 43;
private long phoenixTTLHighWaterMark_ ;
/**
* optional int64 phoenixTTLHighWaterMark = 43;
*/
public boolean hasPhoenixTTLHighWaterMark() {
return ((bitField1_ & 0x00000200) == 0x00000200);
}
/**
* optional int64 phoenixTTLHighWaterMark = 43;
*/
public long getPhoenixTTLHighWaterMark() {
return phoenixTTLHighWaterMark_;
}
/**
* optional int64 phoenixTTLHighWaterMark = 43;
*/
public Builder setPhoenixTTLHighWaterMark(long value) {
bitField1_ |= 0x00000200;
phoenixTTLHighWaterMark_ = value;
onChanged();
return this;
}
/**
* optional int64 phoenixTTLHighWaterMark = 43;
*/
public Builder clearPhoenixTTLHighWaterMark() {
bitField1_ = (bitField1_ & ~0x00000200);
phoenixTTLHighWaterMark_ = 0L;
onChanged();
return this;
}
// optional bool viewModifiedPhoenixTTL = 44;
private boolean viewModifiedPhoenixTTL_ ;
/**
* optional bool viewModifiedPhoenixTTL = 44;
*/
public boolean hasViewModifiedPhoenixTTL() {
return ((bitField1_ & 0x00000400) == 0x00000400);
}
/**
* optional bool viewModifiedPhoenixTTL = 44;
*/
public boolean getViewModifiedPhoenixTTL() {
return viewModifiedPhoenixTTL_;
}
/**
* optional bool viewModifiedPhoenixTTL = 44;
*/
public Builder setViewModifiedPhoenixTTL(boolean value) {
bitField1_ |= 0x00000400;
viewModifiedPhoenixTTL_ = value;
onChanged();
return this;
}
/**
* optional bool viewModifiedPhoenixTTL = 44;
*/
public Builder clearViewModifiedPhoenixTTL() {
bitField1_ = (bitField1_ & ~0x00000400);
viewModifiedPhoenixTTL_ = false;
onChanged();
return this;
}
// optional int64 lastDDLTimestamp = 45;
private long lastDDLTimestamp_ ;
/**
* optional int64 lastDDLTimestamp = 45;
*/
public boolean hasLastDDLTimestamp() {
return ((bitField1_ & 0x00000800) == 0x00000800);
}
/**
* optional int64 lastDDLTimestamp = 45;
*/
public long getLastDDLTimestamp() {
return lastDDLTimestamp_;
}
/**
* optional int64 lastDDLTimestamp = 45;
*/
public Builder setLastDDLTimestamp(long value) {
bitField1_ |= 0x00000800;
lastDDLTimestamp_ = value;
onChanged();
return this;
}
/**
* optional int64 lastDDLTimestamp = 45;
*/
public Builder clearLastDDLTimestamp() {
bitField1_ = (bitField1_ & ~0x00000800);
lastDDLTimestamp_ = 0L;
onChanged();
return this;
}
// optional bool changeDetectionEnabled = 46;
private boolean changeDetectionEnabled_ ;
/**
* optional bool changeDetectionEnabled = 46;
*/
public boolean hasChangeDetectionEnabled() {
return ((bitField1_ & 0x00001000) == 0x00001000);
}
/**
* optional bool changeDetectionEnabled = 46;
*/
public boolean getChangeDetectionEnabled() {
return changeDetectionEnabled_;
}
/**
* optional bool changeDetectionEnabled = 46;
*/
public Builder setChangeDetectionEnabled(boolean value) {
bitField1_ |= 0x00001000;
changeDetectionEnabled_ = value;
onChanged();
return this;
}
/**
* optional bool changeDetectionEnabled = 46;
*/
public Builder clearChangeDetectionEnabled() {
bitField1_ = (bitField1_ & ~0x00001000);
changeDetectionEnabled_ = false;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:PTable)
}
static {
defaultInstance = new PTable(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:PTable)
}
public interface EncodedCQCounterOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// required string colFamily = 1;
/**
* required string colFamily = 1;
*/
boolean hasColFamily();
/**
* required string colFamily = 1;
*/
java.lang.String getColFamily();
/**
* required string colFamily = 1;
*/
com.google.protobuf.ByteString
getColFamilyBytes();
// required int32 counter = 2;
/**
* required int32 counter = 2;
*/
boolean hasCounter();
/**
* required int32 counter = 2;
*/
int getCounter();
}
/**
* Protobuf type {@code EncodedCQCounter}
*/
public static final class EncodedCQCounter extends
com.google.protobuf.GeneratedMessage
implements EncodedCQCounterOrBuilder {
// Use EncodedCQCounter.newBuilder() to construct.
private EncodedCQCounter(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private EncodedCQCounter(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final EncodedCQCounter defaultInstance;
public static EncodedCQCounter getDefaultInstance() {
return defaultInstance;
}
public EncodedCQCounter getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private EncodedCQCounter(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
bitField0_ |= 0x00000001;
colFamily_ = input.readBytes();
break;
}
case 16: {
bitField0_ |= 0x00000002;
counter_ = input.readInt32();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.phoenix.coprocessor.generated.PTableProtos.internal_static_EncodedCQCounter_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.phoenix.coprocessor.generated.PTableProtos.internal_static_EncodedCQCounter_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.phoenix.coprocessor.generated.PTableProtos.EncodedCQCounter.class, org.apache.phoenix.coprocessor.generated.PTableProtos.EncodedCQCounter.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public EncodedCQCounter parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new EncodedCQCounter(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// required string colFamily = 1;
public static final int COLFAMILY_FIELD_NUMBER = 1;
private java.lang.Object colFamily_;
/**
* required string colFamily = 1;
*/
public boolean hasColFamily() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required string colFamily = 1;
*/
public java.lang.String getColFamily() {
java.lang.Object ref = colFamily_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
colFamily_ = s;
}
return s;
}
}
/**
* required string colFamily = 1;
*/
public com.google.protobuf.ByteString
getColFamilyBytes() {
java.lang.Object ref = colFamily_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
colFamily_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// required int32 counter = 2;
public static final int COUNTER_FIELD_NUMBER = 2;
private int counter_;
/**
* required int32 counter = 2;
*/
public boolean hasCounter() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required int32 counter = 2;
*/
public int getCounter() {
return counter_;
}
private void initFields() {
colFamily_ = "";
counter_ = 0;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (!hasColFamily()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasCounter()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(1, getColFamilyBytes());
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeInt32(2, counter_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, getColFamilyBytes());
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(2, counter_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.phoenix.coprocessor.generated.PTableProtos.EncodedCQCounter)) {
return super.equals(obj);
}
org.apache.phoenix.coprocessor.generated.PTableProtos.EncodedCQCounter other = (org.apache.phoenix.coprocessor.generated.PTableProtos.EncodedCQCounter) obj;
boolean result = true;
result = result && (hasColFamily() == other.hasColFamily());
if (hasColFamily()) {
result = result && getColFamily()
.equals(other.getColFamily());
}
result = result && (hasCounter() == other.hasCounter());
if (hasCounter()) {
result = result && (getCounter()
== other.getCounter());
}
result = result &&
getUnknownFields().equals(other.getUnknownFields());
return result;
}
private int memoizedHashCode = 0;
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptorForType().hashCode();
if (hasColFamily()) {
hash = (37 * hash) + COLFAMILY_FIELD_NUMBER;
hash = (53 * hash) + getColFamily().hashCode();
}
if (hasCounter()) {
hash = (37 * hash) + COUNTER_FIELD_NUMBER;
hash = (53 * hash) + getCounter();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.phoenix.coprocessor.generated.PTableProtos.EncodedCQCounter parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.phoenix.coprocessor.generated.PTableProtos.EncodedCQCounter parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.phoenix.coprocessor.generated.PTableProtos.EncodedCQCounter parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.phoenix.coprocessor.generated.PTableProtos.EncodedCQCounter parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.phoenix.coprocessor.generated.PTableProtos.EncodedCQCounter parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.apache.phoenix.coprocessor.generated.PTableProtos.EncodedCQCounter parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.apache.phoenix.coprocessor.generated.PTableProtos.EncodedCQCounter parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.apache.phoenix.coprocessor.generated.PTableProtos.EncodedCQCounter parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.apache.phoenix.coprocessor.generated.PTableProtos.EncodedCQCounter parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.apache.phoenix.coprocessor.generated.PTableProtos.EncodedCQCounter parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(org.apache.phoenix.coprocessor.generated.PTableProtos.EncodedCQCounter prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code EncodedCQCounter}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements org.apache.phoenix.coprocessor.generated.PTableProtos.EncodedCQCounterOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.phoenix.coprocessor.generated.PTableProtos.internal_static_EncodedCQCounter_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.phoenix.coprocessor.generated.PTableProtos.internal_static_EncodedCQCounter_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.phoenix.coprocessor.generated.PTableProtos.EncodedCQCounter.class, org.apache.phoenix.coprocessor.generated.PTableProtos.EncodedCQCounter.Builder.class);
}
// Construct using org.apache.phoenix.coprocessor.generated.PTableProtos.EncodedCQCounter.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
colFamily_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
counter_ = 0;
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.phoenix.coprocessor.generated.PTableProtos.internal_static_EncodedCQCounter_descriptor;
}
public org.apache.phoenix.coprocessor.generated.PTableProtos.EncodedCQCounter getDefaultInstanceForType() {
return org.apache.phoenix.coprocessor.generated.PTableProtos.EncodedCQCounter.getDefaultInstance();
}
public org.apache.phoenix.coprocessor.generated.PTableProtos.EncodedCQCounter build() {
org.apache.phoenix.coprocessor.generated.PTableProtos.EncodedCQCounter result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.apache.phoenix.coprocessor.generated.PTableProtos.EncodedCQCounter buildPartial() {
org.apache.phoenix.coprocessor.generated.PTableProtos.EncodedCQCounter result = new org.apache.phoenix.coprocessor.generated.PTableProtos.EncodedCQCounter(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.colFamily_ = colFamily_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.counter_ = counter_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.apache.phoenix.coprocessor.generated.PTableProtos.EncodedCQCounter) {
return mergeFrom((org.apache.phoenix.coprocessor.generated.PTableProtos.EncodedCQCounter)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.phoenix.coprocessor.generated.PTableProtos.EncodedCQCounter other) {
if (other == org.apache.phoenix.coprocessor.generated.PTableProtos.EncodedCQCounter.getDefaultInstance()) return this;
if (other.hasColFamily()) {
bitField0_ |= 0x00000001;
colFamily_ = other.colFamily_;
onChanged();
}
if (other.hasCounter()) {
setCounter(other.getCounter());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasColFamily()) {
return false;
}
if (!hasCounter()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.phoenix.coprocessor.generated.PTableProtos.EncodedCQCounter parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.phoenix.coprocessor.generated.PTableProtos.EncodedCQCounter) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// required string colFamily = 1;
private java.lang.Object colFamily_ = "";
/**
* required string colFamily = 1;
*/
public boolean hasColFamily() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required string colFamily = 1;
*/
public java.lang.String getColFamily() {
java.lang.Object ref = colFamily_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
colFamily_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* required string colFamily = 1;
*/
public com.google.protobuf.ByteString
getColFamilyBytes() {
java.lang.Object ref = colFamily_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
colFamily_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* required string colFamily = 1;
*/
public Builder setColFamily(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
colFamily_ = value;
onChanged();
return this;
}
/**
* required string colFamily = 1;
*/
public Builder clearColFamily() {
bitField0_ = (bitField0_ & ~0x00000001);
colFamily_ = getDefaultInstance().getColFamily();
onChanged();
return this;
}
/**
* required string colFamily = 1;
*/
public Builder setColFamilyBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
colFamily_ = value;
onChanged();
return this;
}
// required int32 counter = 2;
private int counter_ ;
/**
* required int32 counter = 2;
*/
public boolean hasCounter() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required int32 counter = 2;
*/
public int getCounter() {
return counter_;
}
/**
* required int32 counter = 2;
*/
public Builder setCounter(int value) {
bitField0_ |= 0x00000002;
counter_ = value;
onChanged();
return this;
}
/**
* required int32 counter = 2;
*/
public Builder clearCounter() {
bitField0_ = (bitField0_ & ~0x00000002);
counter_ = 0;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:EncodedCQCounter)
}
static {
defaultInstance = new EncodedCQCounter(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:EncodedCQCounter)
}
private static com.google.protobuf.Descriptors.Descriptor
internal_static_PColumn_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_PColumn_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_PTableStats_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_PTableStats_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_PTable_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_PTable_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_EncodedCQCounter_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_EncodedCQCounter_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n\014PTable.proto\032\021PGuidePosts.proto\"\357\002\n\007PC" +
"olumn\022\027\n\017columnNameBytes\030\001 \002(\014\022\027\n\017family" +
"NameBytes\030\002 \001(\014\022\020\n\010dataType\030\003 \001(\t\022\021\n\tmax" +
"Length\030\004 \001(\005\022\r\n\005scale\030\005 \001(\005\022\020\n\010nullable\030" +
"\006 \002(\010\022\020\n\010position\030\007 \002(\005\022\021\n\tsortOrder\030\010 \001" +
"(\005\022\021\n\tarraySize\030\t \001(\005\022\024\n\014viewConstant\030\n " +
"\001(\014\022\026\n\016viewReferenced\030\013 \001(\010\022\022\n\nexpressio" +
"n\030\014 \001(\t\022\026\n\016isRowTimestamp\030\r \001(\010\022\021\n\tisDyn" +
"amic\030\016 \001(\010\022\034\n\024columnQualifierBytes\030\017 \001(\014" +
"\022\021\n\ttimestamp\030\020 \001(\003\022\026\n\007derived\030\021 \001(\010:\005fa",
"lse\"\232\001\n\013PTableStats\022\013\n\003key\030\001 \002(\014\022\016\n\006valu" +
"es\030\002 \003(\014\022\033\n\023guidePostsByteCount\030\003 \001(\003\022\025\n" +
"\rkeyBytesCount\030\004 \001(\003\022\027\n\017guidePostsCount\030" +
"\005 \001(\005\022!\n\013pGuidePosts\030\006 \001(\0132\014.PGuidePosts" +
"\"\262\t\n\006PTable\022\027\n\017schemaNameBytes\030\001 \002(\014\022\026\n\016" +
"tableNameBytes\030\002 \002(\014\022\036\n\ttableType\030\003 \002(\0162" +
"\013.PTableType\022\022\n\nindexState\030\004 \001(\t\022\026\n\016sequ" +
"enceNumber\030\005 \002(\003\022\021\n\ttimeStamp\030\006 \002(\003\022\023\n\013p" +
"kNameBytes\030\007 \001(\014\022\021\n\tbucketNum\030\010 \002(\005\022\031\n\007c" +
"olumns\030\t \003(\0132\010.PColumn\022\030\n\007indexes\030\n \003(\0132",
"\007.PTable\022\027\n\017isImmutableRows\030\013 \002(\010\022\032\n\022dat" +
"aTableNameBytes\030\r \001(\014\022\031\n\021defaultFamilyNa" +
"me\030\016 \001(\014\022\022\n\ndisableWAL\030\017 \002(\010\022\023\n\013multiTen" +
"ant\030\020 \002(\010\022\020\n\010viewType\030\021 \001(\014\022\025\n\rviewState" +
"ment\030\022 \001(\014\022\025\n\rphysicalNames\030\023 \003(\014\022\020\n\010ten" +
"antId\030\024 \001(\014\022\023\n\013viewIndexId\030\025 \001(\003\022\021\n\tinde" +
"xType\030\026 \001(\014\022\026\n\016statsTimeStamp\030\027 \001(\003\022\022\n\ns" +
"toreNulls\030\030 \001(\010\022\027\n\017baseColumnCount\030\031 \001(\005" +
"\022\036\n\026rowKeyOrderOptimizable\030\032 \001(\010\022\025\n\rtran" +
"sactional\030\033 \001(\010\022\034\n\024updateCacheFrequency\030",
"\034 \001(\003\022\035\n\025indexDisableTimestamp\030\035 \001(\003\022\031\n\021" +
"isNamespaceMapped\030\036 \001(\010\022\034\n\024autoParititon" +
"SeqName\030\037 \001(\t\022\032\n\022isAppendOnlySchema\030 \001(" +
"\010\022\027\n\017parentNameBytes\030! \001(\014\022\025\n\rstorageSch" +
"eme\030\" \001(\014\022\026\n\016encodingScheme\030# \001(\014\022,\n\021enc" +
"odedCQCounters\030$ \003(\0132\021.EncodedCQCounter\022" +
"\"\n\032useStatsForParallelization\030% \001(\010\022\033\n\023t" +
"ransactionProvider\030& \001(\005\022\032\n\017viewIndexIdT" +
"ype\030\' \001(\005:\0015\022(\n viewModifiedUpdateCacheF" +
"requency\030( \001(\010\022.\n&viewModifiedUseStatsFo",
"rParallelization\030) \001(\010\022\022\n\nphoenixTTL\030* \001" +
"(\003\022\037\n\027phoenixTTLHighWaterMark\030+ \001(\003\022\036\n\026v" +
"iewModifiedPhoenixTTL\030, \001(\010\022\030\n\020lastDDLTi" +
"mestamp\030- \001(\003\022\036\n\026changeDetectionEnabled\030" +
". \001(\010\"6\n\020EncodedCQCounter\022\021\n\tcolFamily\030\001" +
" \002(\t\022\017\n\007counter\030\002 \002(\005*A\n\nPTableType\022\n\n\006S" +
"YSTEM\020\000\022\010\n\004USER\020\001\022\010\n\004VIEW\020\002\022\t\n\005INDEX\020\003\022\010" +
"\n\004JOIN\020\004B@\n(org.apache.phoenix.coprocess" +
"or.generatedB\014PTableProtosH\001\210\001\001\240\001\001"
};
com.google.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner assigner =
new com.google.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner() {
public com.google.protobuf.ExtensionRegistry assignDescriptors(
com.google.protobuf.Descriptors.FileDescriptor root) {
descriptor = root;
internal_static_PColumn_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_PColumn_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_PColumn_descriptor,
new java.lang.String[] { "ColumnNameBytes", "FamilyNameBytes", "DataType", "MaxLength", "Scale", "Nullable", "Position", "SortOrder", "ArraySize", "ViewConstant", "ViewReferenced", "Expression", "IsRowTimestamp", "IsDynamic", "ColumnQualifierBytes", "Timestamp", "Derived", });
internal_static_PTableStats_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_PTableStats_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_PTableStats_descriptor,
new java.lang.String[] { "Key", "Values", "GuidePostsByteCount", "KeyBytesCount", "GuidePostsCount", "PGuidePosts", });
internal_static_PTable_descriptor =
getDescriptor().getMessageTypes().get(2);
internal_static_PTable_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_PTable_descriptor,
new java.lang.String[] { "SchemaNameBytes", "TableNameBytes", "TableType", "IndexState", "SequenceNumber", "TimeStamp", "PkNameBytes", "BucketNum", "Columns", "Indexes", "IsImmutableRows", "DataTableNameBytes", "DefaultFamilyName", "DisableWAL", "MultiTenant", "ViewType", "ViewStatement", "PhysicalNames", "TenantId", "ViewIndexId", "IndexType", "StatsTimeStamp", "StoreNulls", "BaseColumnCount", "RowKeyOrderOptimizable", "Transactional", "UpdateCacheFrequency", "IndexDisableTimestamp", "IsNamespaceMapped", "AutoParititonSeqName", "IsAppendOnlySchema", "ParentNameBytes", "StorageScheme", "EncodingScheme", "EncodedCQCounters", "UseStatsForParallelization", "TransactionProvider", "ViewIndexIdType", "ViewModifiedUpdateCacheFrequency", "ViewModifiedUseStatsForParallelization", "PhoenixTTL", "PhoenixTTLHighWaterMark", "ViewModifiedPhoenixTTL", "LastDDLTimestamp", "ChangeDetectionEnabled", });
internal_static_EncodedCQCounter_descriptor =
getDescriptor().getMessageTypes().get(3);
internal_static_EncodedCQCounter_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_EncodedCQCounter_descriptor,
new java.lang.String[] { "ColFamily", "Counter", });
return null;
}
};
com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
org.apache.phoenix.coprocessor.generated.PGuidePostsProtos.getDescriptor(),
}, assigner);
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy