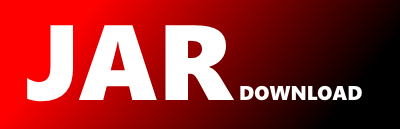
org.apache.phoenix.jdbc.LoggingPhoenixConnection Maven / Gradle / Ivy
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.phoenix.jdbc;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.SQLException;
import java.sql.Statement;
import org.apache.phoenix.util.PhoenixRuntime;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class LoggingPhoenixConnection extends DelegateConnection {
private static final Logger logger = LoggerFactory.getLogger(LoggingPhoenixResultSet.class);
private PhoenixMetricsLog phoenixMetricsLog;
public LoggingPhoenixConnection(Connection conn,
PhoenixMetricsLog phoenixMetricsLog) {
super(conn);
this.phoenixMetricsLog = phoenixMetricsLog;
}
@Override
public Statement createStatement() throws SQLException {
return new LoggingPhoenixStatement(super.createStatement(), phoenixMetricsLog);
}
@Override
public Statement createStatement(int resultSetType, int resultSetConcurrency)
throws SQLException {
return new LoggingPhoenixStatement(
super.createStatement(resultSetType, resultSetConcurrency), phoenixMetricsLog);
}
@Override
public Statement createStatement(int resultSetType, int resultSetConcurrency,
int resultSetHoldability) throws SQLException {
return new LoggingPhoenixStatement(
super.createStatement(resultSetType, resultSetConcurrency, resultSetHoldability),
phoenixMetricsLog);
}
@Override
public PreparedStatement prepareStatement(String sql) throws SQLException {
return new LoggingPhoenixPreparedStatement(super.prepareStatement(sql),
phoenixMetricsLog);
}
@Override
public PreparedStatement prepareStatement(String sql, int resultSetType,
int resultSetConcurrency) throws SQLException {
return new LoggingPhoenixPreparedStatement(
super.prepareStatement(sql, resultSetType, resultSetConcurrency),
phoenixMetricsLog);
}
@Override
public PreparedStatement prepareStatement(String sql, int resultSetType,
int resultSetConcurrency, int resultSetHoldability) throws SQLException {
return new LoggingPhoenixPreparedStatement(super.prepareStatement(sql, resultSetType,
resultSetConcurrency, resultSetHoldability), phoenixMetricsLog);
}
@Override
public PreparedStatement prepareStatement(String sql, int autoGeneratedKeys)
throws SQLException {
return new LoggingPhoenixPreparedStatement(super.prepareStatement(sql, autoGeneratedKeys),
phoenixMetricsLog);
}
@Override
public PreparedStatement prepareStatement(String sql, int[] columnIndexes) throws SQLException {
return new LoggingPhoenixPreparedStatement(super.prepareStatement(sql, columnIndexes),
phoenixMetricsLog);
}
@Override
public PreparedStatement prepareStatement(String sql, String[] columnNames)
throws SQLException {
return new LoggingPhoenixPreparedStatement(super.prepareStatement(sql, columnNames),
phoenixMetricsLog);
}
@Override
public void commit() throws SQLException {
super.commit();
phoenixMetricsLog.logWriteMetricsfoForMutations(logger, PhoenixRuntime.getWriteMetricInfoForMutationsSinceLastReset(conn));
phoenixMetricsLog.logReadMetricInfoForMutationsSinceLastReset(logger, PhoenixRuntime.getReadMetricInfoForMutationsSinceLastReset(conn));
PhoenixRuntime.resetMetrics(conn);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy