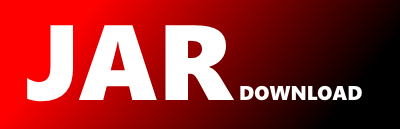
org.apache.tephra.persist.NoOpTransactionStateStorage Maven / Gradle / Ivy
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.tephra.persist;
import com.google.common.util.concurrent.AbstractIdleService;
import com.google.inject.Inject;
import org.apache.tephra.snapshot.SnapshotCodec;
import org.apache.tephra.snapshot.SnapshotCodecProvider;
import java.io.IOException;
import java.io.OutputStream;
import java.util.ArrayList;
import java.util.List;
/**
* Minimal {@link TransactionStateStorage} implementation that does nothing, i.e. does not maintain any actual state.
*/
public class NoOpTransactionStateStorage extends AbstractIdleService implements TransactionStateStorage {
private final SnapshotCodec codec;
@Inject
public NoOpTransactionStateStorage(SnapshotCodecProvider codecProvider) {
codec = codecProvider;
}
@Override
protected void startUp() throws Exception {
}
@Override
protected void shutDown() throws Exception {
}
@Override
public void writeSnapshot(OutputStream out, TransactionSnapshot snapshot) throws IOException {
codec.encode(out, snapshot);
}
@Override
public void writeSnapshot(TransactionSnapshot snapshot) throws IOException {
}
@Override
public TransactionSnapshot getLatestSnapshot() throws IOException {
return null;
}
@Override
public TransactionVisibilityState getLatestTransactionVisibilityState() throws IOException {
return null;
}
@Override
public long deleteOldSnapshots(int numberToKeep) throws IOException {
return 0;
}
@Override
public List listSnapshots() throws IOException {
return new ArrayList<>(0);
}
@Override
public List getLogsSince(long timestamp) throws IOException {
return new ArrayList<>(0);
}
@Override
public TransactionLog createLog(long timestamp) throws IOException {
return new NoOpTransactionLog();
}
@Override
public void deleteLogsOlderThan(long timestamp) throws IOException {
}
@Override
public void setupStorage() throws IOException {
}
@Override
public List listLogs() throws IOException {
return new ArrayList<>(0);
}
@Override
public String getLocation() {
return "no-op";
}
private static class NoOpTransactionLog implements TransactionLog {
private long timestamp = System.currentTimeMillis();
@Override
public String getName() {
return "no-op";
}
@Override
public long getTimestamp() {
return timestamp;
}
@Override
public void append(TransactionEdit edit) throws IOException {
}
@Override
public void append(List edits) throws IOException {
}
@Override
public void close() {
}
@Override
public TransactionLogReader getReader() {
return new TransactionLogReader() {
@Override
public TransactionEdit next() {
return null;
}
@Override
public TransactionEdit next(TransactionEdit reuse) {
return null;
}
@Override
public void close() {
}
};
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy