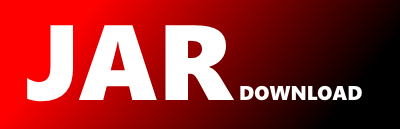
org.apache.polygene.serialization.javaxxml.JavaxXmlSerialization Maven / Gradle / Ivy
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.polygene.serialization.javaxxml;
import org.apache.polygene.api.mixin.Mixins;
import org.apache.polygene.spi.serialization.XmlSerialization;
/**
* javax.xml state serialization.
*
* The XML representations consumed and produced by this service are, by default, verbose, and safe to deserialize.
* This is because the default mapping is purely structural.
* You can customize the XML representations using {@link JavaxXmlSettings} and {@link JavaxXmlAdapters}.
*
* The following describe how state is represented by default.
*
* Because a valid XML document can only contain a single node and it must be an element, all
* {@link org.w3c.dom.Document}s have a root element {@literal <state/>}. This serialization implementation
* also impose that the root element can only contain a single node, of any type.
*
* {@literal null} is represented as {@literal <null/>}.
* Plain values are represented as {@link org.w3c.dom.Text} nodes.
* Iterables and Streams are represented as {@literal <collection/>} {@link org.w3c.dom.Element}s.
* Maps are represented as {@literal <dictionary/>} {@link org.w3c.dom.Element}s.
*
* This is how a {@literal null} plain value is represented: {@literal <state><null/></state>}.
* And a plain {@literal LocalDate}: {@literal <state>2017-01-01</state>}
*
* This is how a fictional value including a collection and a map is represented:
*
* <state>
* <stringProperty>and it's value</stringProperty>
* <bigDecimalProperty>4.22376931348623157E+310</bigDecimalProperty>
* <nullProperty><null/></nullProperty>
* <booleanProperty>false</booleanProperty>
* <stringCollectionProperty>
* <collection>
* item1
* item2 <!-- As multiple text nodes -->
* </collection>
* </stringCollectionProperty>
* <mapProperty>
* <map>
* <foo>bar</foo>
* <bazar>cathedral</bazar>
* </map>
* </mapProperty>
* <complexKeyMapProperty>
* <map>
* <entry>
* <key>
* <foo>bar</foo>
* <bazar>cathedral</bazar>
* </key>
* <value>23</value>
* </entry>
* <entry>
* <key>
* <foo>baz</foo>
* <bazar>bar</bazar>
* </key>
* <value>42</value>
* </entry>
* </map>
* </complexKeyMapProperty>
* </state>
*
*
*/
@Mixins( { JavaxXmlSerializer.class, JavaxXmlDeserializer.class } )
public interface JavaxXmlSerialization extends XmlSerialization
{
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy